Nelson 1.11.0.0
Nelson is a powerful, open-source numerical computational language, developed to provide a comprehensive and intuitive environment for engineers, scientists, and students. With over 1,200 built-in functions, Nelson supports a wide range of tasks, from basic algebra to advanced numerical simulations.
Originally inspired by languages like MATLAB© and GNU Octave, Nelson offers users a lightweight yet feature-rich experience. It is designed to be easy to learn and use, with an emphasis on performance and flexibility.
Try it now!
Features
Data Types Managed by Nelson
- Double and Complex Double: Supports scalars, vectors, 2D matrices, N-dimensional arrays, and sparse matrices.
- Single and Complex Single: Includes scalars, vectors, 2D matrices, N-dimensional arrays, and sparse matrices.
- Logical: Handles scalars, vectors, 2D matrices, N-dimensional arrays, and sparse matrices.
- Character Arrays: Supports UNICODE characters.
- String Arrays: Fully supports UNICODE.
- Integers: 8, 16, 32, and 64-bit signed and unsigned scalars, vectors, 2D matrices, and N-dimensional arrays.
- Handle Objects: For object-oriented functionality.
- Anonymous Functions: Allows creation and manipulation of functions without names.
- Data Structures: Supports dictionaries and tables.
- Overloading: All types can be overloaded for custom behavior.
Performance Enhancements
- OpenMP and SIMD: Utilizes parallel processing and vectorization for faster computations.
Visualization & Interface
- 2D and 3D Plotting: High-level commands for visualizations.
- User Interface Controls: Built-in controls for creating custom interfaces.
- Desktop Environment: Comes with history tracking, a file explorer, and workspace browser.
Advanced Modules
- Parallel Computing: Enables efficient use of multi-core processors.
- Fast Fourier Transform (FFT): High-performance FFT functions based on FFTW and MKL.
- SLICOT Interface: Optional support for the Systems and Control Theory subroutine library.
- Control System Module: Tools for control theory and system design.
- MPI (Message Passing Interface): Functions for distributed parallel computing.
Data Formats & Interfacing
- JSON Support: Read and write JSON files.
- HDF5 Functions: High-level I/O functions, with HDF5 as the default file format for
.nh5
workspaces. - MAT-File Compatibility: Load and save workspaces in MAT-file format.
- Foreign Function Interface (FFI): Build and load C/Fortran code on the fly.
- MEX C API Compatibility: Interfacing with MEX-compatible C APIs.
- Nelson Engine API: Use Nelson as a backend engine within C code, compatible with the MEX Engine API.
- Python Interfacing: Call Python scripts and functions from Nelson.
- RESTful API: Enables Nelson to interact with web services.
Additional Capabilities
- Inter-Process Communication: Communicate between Nelson processes.
- QML Engine: Use Qt’s QML framework to display and manipulate graphical content.
- Component Object Model (COM): Interface with COM components, especially on Windows.
- Excel File Support: Write and read
.xlsx
files using COM on Windows. - Embedded Code Editor: Integrated editor for Nelson scripts.
Help & Testing Tools
- Help Engine: Generate and view help files in various formats like HTML, Markdown, PDF, or GitBook.
- Test Engine: Validate algorithms using built-in functions, with support for xUnit report export.
Profiling & Code Coverage
- Profiler: Built-in profiler to analyze and optimize code performance.
- Code Coverage: Measure the coverage of your tests to ensure thorough validation.
Cloud & Extensibility
- Nelson Cloud: Instant access to Nelson from any web browser via Nelson Cloud.
- Module Skeleton: Templates for extending Nelson:
- Nelson Modules Manager (nmm): A package manager to install and manage extensions for Nelson.
Changelogs
- Changelog v1.x.x
- Changelog v0.7.x
- Changelog v0.6.x
- Changelog v0.5.x
- Changelog v0.4.x
- Changelog v0.3.x
- Changelog v0.2.x
- Changelog v0.1.x
License
Functions manager
Functions manager
Description
functions manager
- addpath - Add directories to functions search path.
- builtin - Executes built-in function.
- clearfun - Clear an built-in function.
- feval - Evaluates function.
- inmem - Names of functions, MEX-files.
- isbuiltin - Check for the existence of a builtin.
- ismacro - Check for the existence of a macro (function).
- ismex - Check for the existence of a mex function.
- macroargs - Returns variables names of a function.
- path - Modify or display Nelson’s load path.
- private functions - Private functions.
- rehash - Reinitialize Nelson’s search path directory cache.
- restoredefaultpath - Restore Nelson’s path to its initial state at startup.
- rmpath - Remove directory from search path.
- userpath - Displays or modify default user functions directory.
- what - Get Nelson builtin and macro list.
- which - Locates functions and built-in.
addpath
Add directories to functions search path.
Syntax
- addpath(dirname)
- addpath(dirname, ..., dirname)
- addpath(dirname, ..., dirname, '-begin')
- addpath(dirname, ..., dirname, '-end')
- addpath(dirname, ..., dirname, '-frozen')
- previous = addpath(dirname)
- previous = addpath(dirname, ..., dirname)
- previous = addpath(dirname, ..., dirname, '-begin')
- previous = addpath(dirname, ..., dirname, '-end')
Input argument
- dirname - a string: a directory
- '-end' or '-begin' - append dirname at the end or begin of the list.
- '-frozen' - disables folder change detection for the folders being added or modified.
Output argument
- previous - returns previous path before adding
Description
addpath add directories to search path.
It is also possible to add lists of directory names separated by pathsep.
Non-existent path will not be added and a warning will be issued.
files watchers is disabled for internal modules.
Example
path()
addpath(tempdir())
path
rmpath(tempdir())
path
See also
path, rmpath, restoredefaultpath.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
builtin
Executes built-in function.
Syntax
- builtin(function_name; x1, ..., xn)
- builtin(function_handle; x1, ..., xn)
- [r1, ..., rn] = builtin(function_name, x1, ..., xn)
- [r1, ..., rn] = builtin(function_handle, x1, ..., xn)
Input argument
- function_name - a string: function name.
- function_handle - a function handle.
- x1, ..., xn - input arguments of the builtin.
Output argument
- r1, ..., rn - output arguments returned by the builtin
Description
builtin calls the base built-in described by its name or function handle and input arguments.
Example
a = builtin('cos', 0)
b = builtin(str2func('cos'), 0)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
clearfun
Clear an built-in function.
Syntax
- l = clearfun(function_name)
- l = clearfun(function_handle)
Input argument
- function_name - a string: function name.
- function_handle - a function handle.
Output argument
- l - a logical
Description
clearfun clears built-in.
Example
cos(3)
a = clearfun('cos')
cos(3)
sin(3)
b = clearfun(str2func('sin'))
sin(3)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
feval
Evaluates function.
Syntax
- feval(function_name; x1, ..., xn)
- feval(function_handle; x1, ..., xn)
- [r1, ..., rn] = feval(function_name, x1, ..., xn)
- [r1, ..., rn] = feval(function_handle, x1, ..., xn)
Input argument
- function_name - a string: function name.
- function_handle - a function handle.
- x1, ..., xn - input arguments of the function.
Output argument
- r1, ..., rn - output arguments returned by the function
Description
function calls the base function or built-in described by its name or function handle and input arguments.
Example
a = feval('cos', 0)
b = feval(str2func('cos'), 0)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
inmem
Names of functions, MEX-files.
Syntax
- F = inmem()
- [F, M] = inmem()
- F = inmem('-completenames')
- [F, M] = inmem('-completenames')
Input argument
- '-completenames' - a string: mex function name.
Output argument
- F - cell array of character vectors containing the names of the macros that are loaded.
- M - cell array of character vectors containing the names of the mex that are loaded.
Description
inmem returns cells array of names of functions and mex currently loaded.
Example
clear all
tand(3)
inmem()
inmem('-completenames')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
isbuiltin
Check for the existence of a builtin.
Syntax
- tf = isbuiltin(name)
Input argument
- name - a string: builtin name.
Output argument
- tf - a logical: true if builtin exists.
Description
isbuiltin checks for the existence of a builtin.
Example
isbuiltin('isbuiltin')
isbuiltin('exist')
ismacro('exist')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
ismacro
Check for the existence of a macro (function).
Syntax
- tf = ismacro(name)
Input argument
- name - a string: macro name.
Output argument
- tf - a logical: true if macro exists.
Description
isbuiltin checks for the existence of a macro.
Example
ismacro('isbuiltin')
ismacro('exist')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
ismex
Check for the existence of a mex function.
Syntax
- tf = ismex(name)
Input argument
- name - a string: mex function name.
Output argument
- tf - a logical: true if mex exists.
Description
ismex checks for the existence of a mex function.
Example
ismex('isbuiltin')
ismex('exist')
ismex('exist')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
macroargs
Returns variables names of a function.
Syntax
- [argOut, argIn] = macroarg(function_name)
Input argument
- function_name - a string: function name.
Output argument
- argOut - a cell with output arguments.
- argIn - a cell with input arguments.
Description
macroargs returns input and output variables used by the function.
Example
[out_args, in_args] = macroarg('getfield')
[out_args, in_args] = macroarg('deal')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
path
Modify or display Nelson’s load path.
Syntax
- path()
- p = path()
- path(dirname)
- path(path(), dirname)
- path(dirname, path())
Input argument
- dirname - a directory name or an suite of directory names using pathsep()
Output argument
- p - string: the specified paths
Description
path modifies or displays Nelson’s load path.
Example
path
p = path()
path(p, tempdir())
path
path(p)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
private functions
Private functions.
Description
Private functions serve a valuable purpose when you wish to restrict the accessibility of a function.
In numerous instances, a single function may require access to one or more auxiliary functions.
when a solitary auxiliary function is utilized by multiple functions, it becomes necessary to relocate these auxiliary functions to a dedicated subdirectory named "private", positioned within the directory where the functions that require access to these auxiliary functions are located.
To illustrate this concept, consider a function, let's call it function1, that relies on a helper function, function2, to perform a substantial portion of its tasks, as shown in below example.
In this scenario, if the path to func1 is directory/function1.m and function2 is found in the directory directory/private/function2.m, then function2 is only accessible to functions within directory, such as function1.
Examples
directory/function1.m
function y = function1(x)
y = function2(x) + 1;
end
directory/private/function2.m
function y = function2(x)
y = 41;
end
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
rehash
Reinitialize Nelson’s search path directory cache.
Syntax
- rehash
Description
rehash() reinitializes Nelson’s search path directory cache.
This happens each time Nelson displays the prompt.
You should use rehash() only when you run a .m file that updates another .m file
Example
rehash()
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
restoredefaultpath
Restore Nelson’s path to its initial state at startup.
Syntax
- restoredefaultpath
Description
restoredefaultpath restores Nelson's search path to its startup state.
Example
path
path('')
path
restoredefaultpath
path
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
rmpath
Remove directory from search path.
Syntax
- rmpath(dirname)
- previouspaths = rmpath(dirname)
Input argument
- dirname - name of directory to remove
Output argument
- previouspaths - a string: path prior to removing the specified paths
Description
rmpath removes directory from search path.
Example
path
addpath(tempdir())
path
rmpath(tempdir())
path
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
userpath
Displays or modify default user functions directory.
Syntax
- p = userpath()
- userpath(dirname)
- userpath('reset')
- userpath('clear')
Input argument
- dirname - an existing directory name
- 'clear' - removes the first directory for current and next sessions of Nelson.
- 'reset' - resets the first directory to the default for your platform.
Output argument
- p - string: the specified user path
Description
userpath modifies or displays user’s load path.
By default, userpath directory is platform-dependant:
Windows platforms: %USERPROFILE%/Documents/Nelson
Others platforms: $home/Documents/Nelson
It is possible to force userpath by define an environment variable: NELSON_USERPATH with an existing path.
Example
path
userpath
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
what
Get Nelson builtin and macro list.
Syntax
- list_builtin = what()
- [list_builtin, list_macro] = what()
Output argument
- list_builtin - a cell of strings
- list_macro - a cell of strings
Description
what returns the list of all builtin and macro available in current Nelson's session.
Example
l = what()
[l, m] = what()
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
which
Locates functions and built-in.
Syntax
- which(function_name)
- p = which(function_name)
- c = which(function_name, '-all')
- m = which(function_name, '-module')
Input argument
- function_name - a string: function name.
Output argument
- p - a string: path of the function or built-in
- c - a cell of strings: paths of the function or built-in.
- m - a cell of strings: name of the modules where function or built-in is available.
Description
which returns the path of a function or a built-in.
Example
which('cos')
p = which('cos')
c = which('cos', '-all')
m = which('cos', '-module')
See also
what.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
Modules manager
Modules manager
Description
modules manager functions
- addgateway - Adds dynamically builtin at runtime.
- addmodule - Add module to Nelson.
- gatewayinfo - Returns information about an gateway.
- getmodules - Returns list of modules loaded in Nelson.
- ismodule - Checks if a module is loaded.
- module.json - module.json description
- modulepath - Returns path of a module.
- nmm - Nelson Modules Manager.
- nmm_build_help - helper's function to build help of an external module
- nmm_build_loader - helper's function to build main loader.m of an external module
- removegateway - Removes dynamically builtin at runtime.
- removemodule - remove a module from Nelson.
- requiremodule - Returns an error if module is not loaded in Nelson.
- semver - semantic versioner.
- toolboxdir - Returns path of a module.
- usermodulesdir - Returns path where external modules are saved.
addgateway
Adds dynamically builtin at runtime.
Syntax
- addgateway(dyn_lib_path)
Input argument
- dyn_lib_path - a string: path of a dynamic library prepared for Nelson.
Description
addgateway(dyn_lib_path) adds dynamically builtin at runtime.
The dynamic library loaded must have at least an C entry point AddGateway.
If gateway was already loaded, no error or warning will be raised.
Example
Add gateway for string module:
addgateway(modulepath('time', 'builtin'))
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
addmodule
Add module to Nelson.
Syntax
- addmodule(module_path, module_short_name)
Input argument
- module_path - a string: root path of a module. path must exist.
- module_short_name - a string: short module's name. This name must not be already used.
Description
addmodule registers a new module designed by his path and short name.
Example
See module skeleton for example
ismodule('module_skeleton')
addmodule([nelsonroot(), '/module_skeleton'], 'module_skeleton')
ismodule('module_skeleton')
removemodule('module_skeleton')
See also
ismodule, removemodule, getmodules.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
gatewayinfo
Returns information about an gateway.
Syntax
- [gateway_name, builtin_list] = gatewayinfo(dyn_lib_path)
Input argument
- dyn_lib_path - a string: path of a dynamic library prepared for Nelson.
Output argument
- gateway_name - a string: gateway name
- builtin_list - a cell of strings: list of builtin in this gateway
Description
[gateway_name, builtin_list] = gatewayinfo(dyn_lib_path) get information about an gateway.
The dynamic library must have at least an C entry point GetGatewayInfo.
If file does not exist an error is raised.
Example
[gateway_name, builtin_list] = gatewayinfo(modulepath('time', 'builtin'))
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
getmodules
Returns list of modules loaded in Nelson.
Syntax
- modules_name = getmodules()
- [modules_name, modules_root_path, modules_version, modules_protected] = getmodules()
Output argument
- modules_name - a cell of strings: modules names.
- modules_root_path - a cell of strings: path of modules.
- modules_version - a cell of vector: [major, minor, patch].
- modules_protected - a vector of logical: true if module can be removed or not.
Description
getmodules returns list of modules loaded in Nelson.
all core's modules are protected and cannot removed during an nelson's session.
Example
[modules_name, modules_root_path, modules_version, modules_protected] = getmodules()
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
ismodule
Checks if a module is loaded.
Syntax
- state = ismodule(module_short_name)
- state = ismodule(module_short_name, 'isprotected')
Input argument
- module_short_name - a string: short module's name to test.
- 'isprotected' - check module isprotected (ie. internal module).
Output argument
- state - a logical.
Description
ismodule returns true if module is loaded otherwise false.
Example
ismodule('core')
ismodule('mymodule')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
1.11.0 | 'isprotected' second argument. |
Author
Allan CORNET
module.json
module.json description
Description
A module.json file is required for each nelson's external module, it allows to manager easily with nmm function.
module: unique identifier module short name (alphanumeric characters), example: "module_skeleton_basic"
title: complete module name (human friendly name), example: "Module skeleton basic"
summary: one line description, example: "Skeleton of a basic nelson package"
version: version number using semantic versioning, example: "1.0.0"
platforms: platforms supported.
"all" for all platforms
others platforms:
"win32": windows 32 bits
"win64": windows 64 bits
"maci64": macos 64 bits build
"maci32": macos 32 bits build
"glnxa64": linux 64 bits build
"glnxa32": linux 64 bits build
example: ["win64", "glnxa64"], module will be available only on windows and linux 64 bits platforms.
nelson: nelson's supported versions, example: "<2.0.0" (default)
builtin: true if module requires C/C++ compiler, false if module have only macros.
author: Author information: name, email and website
Example:
{
"name": "Allan CORNET",
"email": "nelson.numerical.computation@gmail.com",
"url": "https://nelson-lang.github.io/nelson-website/"
}
homepage: homepage of the module, example "https://github.com/nelson-lang/module_skeleton_basic"
description: full description of the module, markdown format supported, example: "nelson's module skeleton (macros only)"
copyrightcopyright description, example: "Copyright © 2019-present Allan CORNET"
license: License under which the toolbox will be published, example: "BSD" or "LGPLv2", ...
keywords: keywords describing your module.
Example:
["interpreter", "scientific-computing", "programming-language", "matrix-functions", "skeleton"]
dependencies: list of modules dependencies {} (default) or name : url values
{
"module_a": "https://module_a.git#v1.0.0",
"module_b": "https://module_b.git#v1.0.0"
}
Example
Deploy module_skeleton and module_skeleton_basic template
if ~ismodule('module_skeleton_basic')
nmm('install', 'https://github.com/nelson-lang/module_skeleton_basic.git#v1.0.0');
end
if ~ismodule('module_skeleton')
nmm('install', 'https://github.com/nelson-lang/module_skeleton.git#v1.0.0');
end
modules_installed = nmm('list');
edit([modules_installed.module_skeleton.path, 'module.json']);
edit([modules_installed.module_skeleton_basic.path, 'module.json']);
See also
nmm.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
modulepath
Returns path of a module.
Syntax
- p = modulepath(module_short_name)
- p = modulepath(module_short_name, option)
Input argument
- module_short_name or 'nelson' - a string: short module's name. module must exist in nelson session.
- option - a string: 'etc', 'bin', 'root', 'builtin', 'tests'.
Output argument
- p - a string: path or subpath of the module.
Description
modulepath is an helper's function to return module root path or a subdirectory.
modulepath('nelson') is equivalent to modulepath('nelson', 'root')
modulepath('nelson', 'bin') return path of nelson's executables.
modulepath('nelson', 'builtin') returns path of nelson's dynamic libraries.
Example
modulepath('core')
modulepath('core', 'root')
modulepath('core', 'etc')
modulepath('core', 'bin')
modulepath('core', 'builtin')
modulepath('core', 'tests')
modulepath('nelson', 'root')
modulepath('nelson', 'bin')
modulepath('nelson', 'builtin')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
nmm
Nelson Modules Manager.
Syntax
- st = nmm('list')
- nmm('load', module_name)
- l = nmm('autoload', module_name)
- nmm('autoload', module_name, state)
- nmm('install', git_url)
- nmm('uninstall', module_name)
- package_filename = nmm('package', module_name, destination_dir)
Input argument
- module_name - a string: short module's name.
- state - a logical: true will autoload module at startup, false disable autoload for this module.
- git_url - a string: a git url (http/https protocol).
- destination_dir - a string: an existing destination directory where archive will be created.
Output argument
- st - a struct: list of installed modules.
- l - a logical: current state of autoload.
- package_filename - a string: filename.
Description
nmm is the Nelson Modules Manager.
Source-based distribution packages allows to have optimized packages for your computer and allows to have distributed repositories.
Installed modules are locally built and can require an C/C++.
st = nmm('list') get list of installed modules.
nmm('install', git_url) install a distant module.
About git_url, in this example 'https://github.com/nelson-lang/module_skeleton_basic.git#v1.0.0'
'#v1.0.0' is defined as #<commit-ish>, it allows to clone exactly an commit.
The commit-ish can be a tag (exact version), and an sha1 (exac commit) or an branch name.
Without commit-ish, master branch will be used.
nmm('install', filename_nmz) install an prebuilt external module.
nmm('load', module_name) load an installed module for current session.
l = nmm('autoload', module_name returns current state autoload for module_name.
nmm('autoload', module_name, state) marks an installed modules "marked" as autoload at startup.
By default modules are marked as autoload.
nmm('uninstall', module_name) uninstall an installed module.
nmm('package', module_name, destination_dir) packages an module as a zip file.
Examples
Deploy module_skeleton_basic template
if ~ismodule('module_skeleton_basic')
nmm('install', 'https://github.com/nelson-lang/module_skeleton_basic.git#v1.0.0');
macro_sum(3, 4)
nmm('uninstall', 'module_skeleton_basic')
end
Package easily a module
if ~ismodule('module_skeleton_basic')
nmm('install', 'https://github.com/nelson-lang/module_skeleton_basic.git#v1.0.0');
end
package_filename = nmm('package', 'module_skeleton_basic', tempdir())
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
nmm_build_help
helper's function to build help of an external module
Syntax
- nmm_build_help(module_short_name, module_root_path)
Input argument
- module_short_name - a string: short module's name.
- module_root_path - a string: path of the module named 'module_short_name'.
Description
nmm_build_help generates help of an external module.
Example
See module skeleton for example
% see builder.m
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
nmm_build_loader
helper's function to build main loader.m of an external module
Syntax
- nmm_build_loader(module_short_name, module_root_path)
Input argument
- module_short_name - a string: short module's name.
- module_root_path - a string: path of the module named 'module_short_name'.
Description
nmm_build_loader generates main loader.m of an external module.
Example
See module skeleton for example
% see builder.m
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
removegateway
Removes dynamically builtin at runtime.
Syntax
- removegateway(dyn_lib_path)
Input argument
- dyn_lib_path - a string: path of a dynamic library prepared for Nelson.
Description
removegateway(dyn_lib_path) removes dynamically builtin at runtime.
The dynamic library loaded must have at least an C entry point RemoveGateway.
If gateway was not loaded, no error or warning will be raised. If file does not exist an error is raised.
Example
removes time builtin
calendar
removegateway(modulepath('time', 'builtin'))
calendar
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
removemodule
remove a module from Nelson.
Syntax
- removemodule(module_short_name)
Input argument
- module_short_name - a string: short module's name.
Description
removemodule remove a module designed by his short name.
all core's modules are protected and cannot removed during an nelson's session.
Example
See module skeleton for example
ismodule('module_skeleton')
addmodule([nelsonroot(), '/module_skeleton'], 'module_skeleton')
ismodule('module_skeleton')
removemodule('module_skeleton')
ismodule('module_skeleton')
See also
ismodule, addmodule, getmodules.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
requiremodule
Returns an error if module is not loaded in Nelson.
Syntax
- requiremodule(module_short_name)
Input argument
- module_short_name - a string: short module's name.
Description
requiremodule returns an error if desired module is not loaded.
This function is usefull to verify a dependency on another module.
Example
See module skeleton for example
ismodule('module_skeleton')
requiremodule('module_skeleton')
addmodule([nelsonroot(), '/module_skeleton'], 'module_skeleton')
ismodule('module_skeleton')
requiremodule('module_skeleton')
See also
ismodule, addmodule, getmodules.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
semver
semantic versioner.
Syntax
- r = semver(version_str, version_range)
Input argument
- version_str - a string: current version.
- version_range - a string: version to compare or range.
Output argument
- r - a double: -1, 0 or 1.
Description
semver compares a version string to an version or an range version.
if an range version is used, r return 0 (not satisfied) or 1 (satisfied).
if an simple version is used, an comparaison value r is returned -1 (inferior), 0 (equal) or 1 (superior).
supported range operators:
= - Equality
>= - Higher or equal to
<= - Lower or equal to
< - Lower than
> - Higher than
^ - Caret operator comparison
~ - Tilde operator comparison
Used function(s)
semver.c
Bibliography
https://semver.org/
Example
semver('1.5.10', '2.3.0')
semver('2.3.0', '1.5.10');
semver('1.5.10', '1.5.10')
semver('1.2.3', '~1.2.3')
semver('1.5.3', '~1.2.3')
semver('1.0.3', '~1')
semver('2.0.3', '~1')
semver('1.2.3-alpha', '>1.2.3-beta')
semver('1.2.3-alpha', '<1.2.3-beta')
semver('1.2.3', '^1.2.3')
semver('1.2.2', '^1.2.3')
semver('1.9.9', '^1.2.3')
semver('2.0.1', '^1.2.3')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
toolboxdir
Returns path of a module.
Syntax
- p = toolboxdir(module_short_name)
Input argument
- module_short_name - a string: short module's name.
Output argument
- p - a string: path of the module.
Description
toolboxdir is an helper's function to return module root path.
Example
toolboxdir('core')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
usermodulesdir
Returns path where external modules are saved.
Syntax
- p = usermodulesdir()
Output argument
- p - a string: path where are external modules.
Description
usermodulesdir is an helper's function to return path where users modules are saved.
This path can be overloaded by defining NELSON_EXTERNAL_MODULES_PATH environment variable on your system.
Example
usermodulesdir()
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
Core
Core
Description
core functions
- banner - Shows Nelson banner.
- eval - Evaluate Nelson code in string.
- evalc - Evaluate Nelson code with console capture.
- evalin - Evaluate Nelson code in string in an specified scope.
- execstr - Execute Nelson code in strings.
- exist - Check for the existence.
- exit - Terminate Nelson program (same as quit)
- feature - undocumented features.
- inputname - Get variable name of function input.
- isunicodesupported - Detect whether the current terminal supports Unicode.
- license - Get license information for Nelson.
- maxNumCompThreads - Set/Get maximum number of computional threads.
- namelengthmax - Return the maximum variable name length.
- nargin - Returns the number of input arguments.
- narginchk - Checks the number of input arguments.
- nargout - Returns the number of output arguments.
- nargoutchk - Checks the number of output arguments.
- nelsonroot - Returns Nelson's root folder.
- nfilename - Returns the name of the currently executing file.
- pause - Pauses script execution.
- prefdir - Return the preferences directory used by Nelson.
- quit - Terminate Nelson application
- run - Executes a script file (.m).
- sha256 - Get sha256 checksum.
- version - Return the version of Nelson.
banner
Shows Nelson banner.
Syntax
- banner
Description
banner shows Nelson banner.
Example
clc();banner
See also
clc.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
eval
Evaluate Nelson code in string.
Syntax
- eval(str)
- eval(str, catch_str)
- [r1, ... rn] = eval(str)
- [r1, ... rn] = eval(str, catch_str)
Input argument
- str - a string: Nelson instruction to execute
- catch_str - a string: Nelson instruction to execute if an error is detected.
Output argument
- [r1, ... rn] - results: output variables
Description
eval executes Nelson instructions given in a string.
Please use try catch end block instead than eval, if you need to capture an error message for higher performance.
Examples
eval('B=4')
This example will fail and returns an error message.
C = eval('B=4')
D = eval(4)
This example will not fail and return false.
eval('error(''blabla'')', 'l = lasterror(); disp([''lasterror message: '', l.message])')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
evalc
Evaluate Nelson code with console capture.
Syntax
- t = evalc(str)
- t = evalc(str)
- [t, r1, ... rn] = evalc(str)
Input argument
- str - a string: Nelson instruction to execute
Output argument
- T - output text captured in t variable
- [r1, ... rn] - results: output variables
Description
evalc executes Nelson instructions given in a string.
console display is redirected into a variable.
diary, more, and input are disabled when evalc is used.
Examples
evalc('B=4')
t = evalc('dir')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
evalin
Evaluate Nelson code in string in an specified scope.
Syntax
- evalin(scope, str)
- [r1, ... rn] = evalin(scope, str)
Input argument
- scope - a string: 'base' or 'caller'.
- str - a string: Nelson instruction to execute
Output argument
- [r1, ... rn] - results: output variables
Description
eval executes Nelson instructions given in a string in 'base' or 'caller' scope.
Example
R = evalin('base', 'evalin(''caller'',''pi'')')
See also
eval, acquirevar, execstr, evalc.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
execstr
Execute Nelson code in strings.
Syntax
- execstr(str)
- execstr(str, 'nocatch')
- bSuccess = execstr(str, 'errcatch')
Input argument
- str - a string: Nelson instruction to execute
Output argument
- bSuccess - a logical: true or false if command fails
Description
execstr executes Nelson instructions given in a string.
execstr(str, 'nocatch') is equivalent to execstr(str)
execstr can be used as alternative to try ... catch ... end block.
Examples
execstr('b = ''hello''; disp(b);')
This example will fail and returns an error message.
execstr('b = yyyy')
This example will fail and returns an error message.
execstr('b = yyyy', 'nocatch')
This example will not fail and return false.
r = execstr('b = yyyy', 'errcatch')
See also
run.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
exist
Check for the existence.
Syntax
- res = exist(name)
- res = exist(name, category)
Input argument
- name - a string: name of variable, function, file or directory.
- category - a string: 'var', 'builtin', 'file', or 'dir'.
Output argument
- res - a integer value.
Description
exists checks for the existence of variable, builtin, file or directory.
exists returns:
0 does not exist
1 is an variable
2 is a file
3 is a mex function
5 is a builtin or function
7 is a directory
Example
exist('fileread')
fileread = 3;
exist('fileread')
clear fileread
exist('fileread')
See also
isbuiltin, ismacro, isfile, isdir, isvar.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
exit
Terminate Nelson program (same as quit)
Syntax
- exit
- exit(status)
- exit('force')
- exit('cancel')
- exit(status, 'force')
Description
This function is equivalent to the quit function.
See also
quit.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
feature
undocumented features.
Syntax
- ret = feature(name)
- ret = feature(name, newValue)
Input argument
- name - a string: name of the feature.
- newValue - a variable
Output argument
- ret - result: result returned
Description
feature is an entirely undocumented and unsupported Nelson function.
It is a helper function for debugging Nelson.
featurecan change without prior notice between Nelson releases, so be very careful when using this function in your code.
History
Version | Description |
---|---|
1.2.0 | initial version |
Author
Allan CORNET
inputname
Get variable name of function input.
Syntax
- s = inputname(argNumber)
Input argument
- argNumber - a scalar, real, positive integer value: Number of function input argument
Output argument
- s - character vector: variable name
Description
inputname get variable name of function input.
inputname is only useable within a function
Example
function R = getinputname(varargin)
R = string([]);
for i = 1:nargin
R = [R, string(inputname(i))];
end
end
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
isunicodesupported
Detect whether the current terminal supports Unicode.
Syntax
- tf = isunicodesupported()
Output argument
- tf - a logical: true or false.
Description
isunicodesupported: returns if current terminal supports Unicode.
value returned can be overloaded if environment variable 'NELSON_TERM_IS_UNICODE_SUPPORTED' is 'TRUE'
Example
isunicodesupported()
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
license
Get license information for Nelson.
Syntax
- license
- r = license
- [r, txt] = license
Output argument
- r - a string: minimal string description about license
- txt - a string: complete license text.
Description
license get license information for Nelson.
Example
license()
r = license()
[r,txt] = license()
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
maxNumCompThreads
Set/Get maximum number of computional threads.
Syntax
- T = maxNumCompThreads()
- PREVIOUS_T = maxNumCompThreads(T)
- PREVIOUS_T = maxNumCompThreads('automatic')
Input argument
- T - an integer value: number of threads used by Nelson for computations.
Output argument
- T - an integer value: number of threads used by Nelson for computations.
- PREVIOUS_T - an integer value: previous number of threads used by Nelson for computations.
Description
maxNumCompThreads returns the number of threads used by Nelson for computations.
maxNumCompThreads(T) sets the maximum number of computational threads. This modification is only available for current session.
By default, maxNumCompThreads uses OMP_NUM_THREADS environment variable or numbers of detected physical cores on Windows and logical cores on others platforms.
Limitation: On Windows 32 bits, due to MKL and OpenMP, maxNumCompThreads returns 4 max even if there is more core.
Example
maxNumCompThreads
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
namelengthmax
Return the maximum variable name length.
Syntax
- R = namelengthmax
Output argument
- R - a double: the maximum variable name length
Description
namelengthmax: Nelson allows 4096 as maximum length for variables and structures field names.
Examples
Working: identifier length 4096 characters
ID = ['A', char(double('0') * ones(1, namelengthmax -1 ))];
length(ID)
STR = [ID, ' = 3'];
execstr(STR)
Not Working: identifier length 4097 characters
ID = ['A', char(double('0') * ones(1, namelengthmax))];
length(ID)
STR = [ID, ' = 3'];
execstr(STR)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
nargin
Returns the number of input arguments.
Syntax
- R = nargin()
- R = nargin(function_name)
- R = nargin(function_handle)
Input argument
- function_name - a string: function name
- function_handle - a function handle
Output argument
- R - an integer value: number of input argument
Description
nargin returns the number of input arguments of an function.
If the last input argument of the function is varargin the returned value is negative.
Examples
With an macro function:
nargin('getfield')
With an builtin function:
nargin('cos')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
narginchk
Checks the number of input arguments.
Syntax
- narginchk(minArgs, maxArgs)
Input argument
- minArgs - minimum number of accepted inputs (scalar integer value).
- maxArgs - maximum number of accepted inputs (scalar integer value).
Description
narginchk checks the number of input arguments of an function.
To ensure that a minimum number of arguments is provided, while allowing an unlimited maximum number by setting maxArgs to inf. For instance, use narginchk(2, inf) to throw an error if fewer than two inputs are supplied.
Example
With an macro function:
narginchk(1, 2)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
1.10.0 | narginchk(3, Inf) managed |
Author
Allan CORNET
nargout
Returns the number of output arguments.
Syntax
- R = nargout()
- R = nargout(function_name)
- R = nargout(function_handle)
Input argument
- function_name - a string: function name
- function_handle - a function handle
Output argument
- R - an integer value: number of output argument
Description
nargout returns the number of output arguments of an function.
If the last output argument of the function is varargout the returned value is negative.
Examples
With an macro function:
nargout('cellstr')
With an builtin function:
nargout('cos')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
nargoutchk
Checks the number of output arguments.
Syntax
- nargoutchk(minArgs, maxArgs)
- msg = nargoutchk(minArgs, maxArgs, numArgs)
- st = nargoutchk(minArgs, maxArgs, numArgs, 'struct')
Input argument
- minArgs - minimum number of accepted outputs (scalar integer value).
- maxArgs - maximum number of accepted outputs (scalar integer value).
- numArgs - number of function outputs (scalar integer value).
Output argument
- msg - a string: error message.
- st - a struct with error message and identifier.
Description
nargoutchk checks the number of output arguments of an function.
To ensure a minimum number of outputs while imposing no maximum limit, set maxArgs to inf. For example, nargoutchk(2, inf) generates an error if fewer than two outputs are specified.
Example
With an macro function:
nargoutchk(1, 2, 3)
nargoutchk(1, 2, 3, 'struct')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
1.10.0 | nargoutchk(3, Inf) managed |
Author
Allan CORNET
nelsonroot
Returns Nelson's root folder.
Syntax
- nelson_path = nelsonroot
Output argument
- nelson_path - a string: the root folder of Nelson.
Description
nelsonroot returns the root folder of Nelson.
Example
pwd
cd(nelsonroot)
pwd
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
nfilename
mfilename
Returns the name of the currently executing file.
Syntax
- R = nfilename()
- R = nfilename('fullpath')
- R = nfilename('fullpathext')
Output argument
- R - a string: the path of current function
Description
R = nfilename() returns the name of the currently executing file.
nfilename() called from outside an nlf file returns an empty string.
With the input argument 'fullpathext', the string includes the directory part of the macro filename, and the filename extension.
With the input argument 'fullpath', the string includes the directory part of the macro filename, but not the extension.
mfilename is an alias on nfilename added for basic script compatibility.
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
pause
Pauses script execution.
Syntax
- state = pause()
- pause(t)
- pause(newState)
- previousState = pause(newState)
- currentState = pause('query')
Input argument
- t - t: double value. time (seconds) before to continue.
- newState - a string: 'on' (enable pause) or 'off' (disable pause setting)
Output argument
- previousState, currentState - a string: 'on' or 'off'
Description
pause(t) suspends execution for t seconds.
pause without input argument wait until return key is pressed.
Example
an example
state = pause
echo('press return to continue.')
pause
pause('off')
pause
pause('on')
pause(5)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
prefdir
Return the preferences directory used by Nelson.
Syntax
- pref_path = prefdir
Output argument
- pref_path - a string: the preferences directory
Description
pref_path = prefdir() returns the preferences directory used by Nelson.
Example
an example
cd(prefdir)
See also
cd.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
quit
Terminate Nelson application
Syntax
- quit
- quit(status)
- quit('force')
- quit('cancel')
- quit(status, 'force')
Description
quit terminates current Nelson application.
quit('cancel') command is designed specifically for utilization within a finish.m script, preventing the termination process.
Its functionality is restricted to this context.
On the other hand, quit('force') disregards the finish.m script and immediately concludes Nelson.
Employ this syntax when you need to override the finish script, ensuring a smooth exit in case the script poses obstacles to quitting.
When you utilize quit(code), Nelson exits with the specified value as the exit code.
If you append "force" to this command quit(code, 'force') it enforces an immediate termination, bypassing finish.m and incorporating the provided exit code.
The exit code, denoted by "code" and specified as a signed integer, determines the status of Nelson termination.
On Windows® platforms, Nelson furnishes exit codes within the range of INT_MIN to INT_MAX (-2147483647 to 2147483647).
On Linux® and macOS platforms, Nelson confines exit codes to the range of 0 to 255.
This distinction should be considered when interpreting or handling exit codes in Nelson scripts or processes.
Example
Beware this example will close Nelson
quit
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
run
Executes a script file (.m).
Syntax
- run(script_file)
- run(script_file, 'nocatch')
- bsuccess = run(script_file, 'errcatch')
Input argument
- script_file - a string: path of a script
- 'nocatch' - a string: default option (no error catch)
- 'errcatch' - a string: error catched
Output argument
- bsuccess - a logical: true if no error detected during script execution
Description
run(script_file) executes a Nelson's script file (.m file extension).
Examples
Creates two .m in temp directory to use as example:
fd = fopen([tempdir(), 'example_run_ok.m'], 'wt');
fprintf(fd, ['A = 1;', char(10)]);
fprintf(fd, ['B = 2;', char(10)]);
fprintf(fd, ['C = A + B', char(10)]);
fclose(fd);
fd = fopen([tempdir(), 'example_run_not_ok.m'], 'wt');
fprintf(fd, ['AA = 1;', char(10)]);
fprintf(fd, ['CC = AA + BB', char(10)]);
fclose(fd);
run a script without error.
run([tempdir(), 'example_run_ok.m']);
run a script and catch error (no error).
bsuccess = run([tempdir(), 'example_run_ok.m'], 'errcatch')
run a script and catch error (with error).
bsuccess = run([tempdir(), 'example_run_not_ok.m'], 'errcatch')
run a script and no catch error.
run([tempdir(), 'example_run_not_ok.m'], 'nocatch');
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
sha256
Get sha256 checksum.
Syntax
- hexa_hash = sha256(str)
- hexa_hash = sha256(filename)
- hexa_hash = sha256(str, '-file')
- hexa_hash = sha256(str, '-string')
Input argument
- str - a character vector, cell of string or array of strings: content of string will be hashed.
- filename - a string: existing filename: content of the file will be hashed.
- '-file' or '-string' - force to hash as file or string content.
Output argument
- hexa_hash - a character vector, cell of string or array of strings: hashed result (checksum).
Description
sha256 get sha256 checksum.
Examples
R = sha256('Nelson')
R = sha256({'Hello', 'World'})
R = sha256(["Hello"; "World"])
R = sha256([modulepath('matio', 'tests'), '/mat/test_char_array_unicode_7.4_GLNX86.mat'])
R = sha256([modulepath('matio', 'tests'), '/mat/test_char_array_unicode_7.4_GLNX86.mat'], '-file')
R = sha256([modulepath('matio', 'tests'), '/mat/test_char_array_unicode_7.4_GLNX86.mat'], '-string')
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
version
Return the version of Nelson.
Syntax
- ver_str = version
- ver_date = version('-date')
- ver_desc = version('-description')
- ver_comp = version('-compiler')
- ver_hash = version('-commit_hash')
- ver_number = version('-number')
- ver_release = version('-release')
- [ver_str, ver_release] = version()
Input argument
- '-date' - a string to get release date
- '-description' - a string to get release description
- '-semantic' - a string to get semantic version
- '-release' - a string to get release number
- '-compiler' - a string to get compiler used to build Nelson
- '-number' - a string to get semantic version
- '-commit_hash' - a string to get commit hash
Output argument
- ver_str - a string : version
- ver_date - a string: version date
- ver_desc - a string: version description
- ver_release - a string: release info
- ver_commit - a string: commit hash
- ver_compiler - a cell of string: {compiler used, arch}
- ver_number - a matrix of integer values: [MAJOR, MINOR, MAINTENANCE, BUILD]
Description
version the version of Nelson.
Examples
ver = version
ver_date = version('-date')
ver_date = version('-description')
ver_date = version('-release')
ver_version_vector] = version('-semantic')
ver_version_vector = version('-number')
compiler_info = version('-compiler')
[ver, release] = version()
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
1.2.0 | -semantic option added. |
Author
Allan CORNET
Engine
Engine
Description
nelson engine functions
- argv - Nelson command line arguments.
- executable - Executables to start Nelson software.
- finish - User-defined termination script for Nelson.
- getnelsonmode - Returns current Nelson mode.
- isquietmode - Return true if Nelson started with --quiet option.
- #! shebang - On Unix, Linux operating systems, Parses the rest of the script's initial line as an interpreter directive.
- startup - User-defined startup script for Nelson.
argv
Nelson command line arguments.
Syntax
- args = argv()
Output argument
- args - a cell array of strings.
Description
argv() returns a cell array of strings containing the arguments of the Nelson command line.
The first element of the cell returned contains the path of the launched executable.
Example
argv()
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
executable
Executables to start Nelson software.
Syntax
- nelson arg1 ... argn
- nelson-cli arg1 ... argn
- nelson-adv-cli arg1 ... argn
- nelson-gui arg1 ... argn
Input argument
- -cli - equivalent to call 'nelson-cli'.
- -adv-cli - equivalent to call 'nelson-adv-cli'.
- -gui - equivalent to call 'nelson-gui'.
- -e "nelson instructions" - If this option is present then Nelson instruction is executed just after startup file execution into Nelson. -e and -f options are mutually exclusive.
- -f filename - Nelson script file is executed just after startup file execution) into Nelson. -e and -f options are mutually exclusive.
- -F filename - If this option is present then Nelson script file is executed just after startup file execution) into an existing Nelson's process or creates it.
- --help - help about program options.
- --version - Return Nelson version.
- --vscode - enable Visual Studio Code mode.
- --open - opens files arg2 ... argN must be valid/existing filenames.
- --mat - load files arg2 ... argN must be valid/existing .nh5 or .mat filenames.
- --nostartup - disable the main Nelson script file executed at startup.
- --nousermodules - disable the load of user's modules. loaded before user's script.
- --nouserstartup - disable the user script file executed at startup after the main startup file.
- --minimize - minimize main GUI Windows (GUI mode only).
- --noipc - disable interprocess features (files association, ipc builtin).
- --withoutfilewatcher - disable file watcher feature for this session.
- --noaudio - disable audio module.
- --without_python - disable python_engine module.
- --language lang - If this option is present it fixes the user language. Currently, lang can be: fr_FR en_US.
- --quiet - If this option is present no banner and version displayed.
Description
nelson-cli: basic terminal, no gui (no dependency to gui framework), no history, no completion (iso latin encoding)
nelson-adv-cli: advanced terminal, no graphical console, history, completion available (UTF-16 support)
nelson-gui: graphical console, history, completion available (UTF-16 support)
If you have installed Nelson on Windows, the NELSON_RUNTIME_PATH environment variable will be defined.
It allows to call easily Nelson "%NELSON_RUNTIME_PATH%\nelson.bat".
Examples
nelson-adv-cli -q -e "a = 1 + 2"
nelson-gui -v
nelson-gui --help
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
1.4.0 | --without_python added |
1.11.0 | About NELSON_RUNTIME_PATH environment variable added |
1.11.0 | --vsocde argument |
Author
Allan CORNET
finish
User-defined termination script for Nelson.
Description
startup.m in Nelson initiates user-specified commands upon Nelson startup.
It executes any file named startup.m that is located on the search path.
To leverage this feature, create a file named startup.m in the userpath folder, which is included in the Nelson search path.
Embed commands within this file that you wish to be executed during Nelson startup.
This could involve setting physical constants, defining defaults for graphics properties, incorporating engineering conversion factors, or predefining any other elements desired in your workspace.
Customizing the startup.m file allows you to establish a tailored environment every time Nelson is launched.
See also
exit, quit, startup, userpath.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
getnelsonmode
Returns current Nelson mode.
Syntax
- m = getnelsonmode()
Output argument
- m - a string.
Description
getnelsonmode() returns current Nelson mode used.
There are 5 modes:
BASIC_ENGINE: Nelson used as engine without any graphics.
ADVANCED_ENGINE: Nelson used as engine with graphics/gui.
BASIC_TERMINAL: Nelson launched as terminal without graphics.
ADVANCED_TERMINAL: Nelson launched as terminal with graphics/gui.
BASIC_SIO_CLIENT: Nelson launched as socket IO client.
ADVANCED_SIO_CLIENT: Nelson launched as socket IO client with graphics/gui.
GUI: Nelson launched as a graphical application (default).
Example
getnelsonmode()
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
isquietmode
Return true if Nelson started with --quiet option.
Syntax
- res = isquietmode()
Output argument
- res - a logical true or false
Description
isquietmode returns a logical 1 if Nelson started with --quiet option and a logical 0 otherwise.
Example
disp(isquietmode());
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
#! shebang
On Unix, Linux operating systems, Parses the rest of the script's initial line as an interpreter directive.
Description
On Unix, Linux and MacOs X, shebang allows to execute directly a NelSon script.
Example
#!nelson-adv-cli -q -f
argv()
disp('shebang example line 1')
disp('shebang example line 2')
exit()
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
startup
User-defined startup script for Nelson.
Description
startup.m in Nelson initiates user-specified commands upon Nelson startup.
It executes any file named startup.m that is located on the search path.
To leverage this feature, create a file named startup.m in the userpath folder, which is included in the Nelson search path.
Embed commands within this file that you wish to be executed during Nelson startup.
This could involve setting physical constants, defining defaults for graphics properties, incorporating engineering conversion factors, or predefining any other elements desired in your workspace.
Customizing the startup.m file allows you to establish a tailored environment every time Nelson is launched.
See also
finish.m, exit, quit, path, userpath.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
Interpreter functions
Interpreter functions
Description
interpreter functions
- abort - stop evaluation.
- break - exit evaluation loop.
- continue - continue evaluation in loop.
- for - for loop.
- function - function declaration.
- if - conditional statement.
- iskeyword - Returns all Nelson keywords.
- keyboard - Stops script execution and enter in debug mode.
- max_recursion_depth - Internal limit on the number of times a function may be called recursively.
- numeric types - About integer and floating-point data.
- parsefile - Parse a Nelson file.
- parsestring - Parse a string.
- switch - switch statement.
- try - try/catch statement.
- while - while loop.
abort
return
stop evaluation.
Syntax
- abort
- return
Description
return or abort stops current evaluation.
Example
for i=1:10,a = i,abort,end
a
See also
for.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
break
exit evaluation loop.
Syntax
- break
Description
break statement is used to exit a loop prematurely.
break statement can be used inside a for or a while loop.
Example
for i = 1:10
if i == 5
disp('i == 5');
break;
end
disp(i)
end
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
continue
continue evaluation in loop.
Syntax
- continue
Description
continue statement can be used inside a for or a while loop.
continue statement is used to pass control to the next iteration of a loop.
Example
for i=1:10
if (i == 5)
continue;
disp('never here')
disp(i)
else
disp(i)
end
end
See also
for.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
for
parfor
for loop.
Syntax
- for variable = expression, statements, end
- for variable, statements, end
Description
for loop executes a set of statements with an index variable looping through each element in a vector.
parfor is currently an alias on for keyword.
Examples
for i = 1:10, disp(i), end
for i = [1, 2; 3 4], disp(i), disp('next'), end
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
function
endfunction
function declaration.
Syntax
- function [out_1,...,out_M,varargout] = fname(in_1, ... , in_N, varargin)
- function fname(in_1, ... , in_N, varargin)
- function [out_1,...,out_M,varargout] = fname()
- function fname()
Description
function opens a function definition.
endfunction closes a function definition (optional, but strongly recommended).
Example
in a file: demo_function.m
function r = demo_function(a, b)
r = a + b;
endfunction
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
if
conditional statement.
Syntax
- if conditional_expression_1, statements_1, elseif conditional_expression_2, statements_2, else statements_N end
Description
if and else statements form a control structure for conditional execution.
Example
i = 0;
if i == 0
disp('ok')
elseif i == 1
disp('not ok 1')
else
disp('not ok 2')
end
See also
for.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
iskeyword
Returns all Nelson keywords.
Syntax
- state = iskeyword(name)
- ce = iskeyword()
Input argument
- name - a string.
Output argument
- state - a logical: true if is an Nelson keyword.
- ce - a cell of strings: list of Nelson's keywords.
Description
iskeyword returns a list of all Nelson keywords.
Example
iskeyword('for')
ce = iskeyword()
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
keyboard
Stops script execution and enter in debug mode.
Syntax
- keyboard()
Description
keyboard stops script execution and enter in debug mode. prompt is modified and displays debug level.
Example
keyboard()
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
max_recursion_depth
Internal limit on the number of times a function may be called recursively.
Syntax
- current_val = max_recursion_depth()
- previous_val = max_recursion_depth(new_val)
Input argument
- new_val - a integer value: new value
Output argument
- current_val - a integer value.
- previous_val - a integer value.
Description
max_recursion_depth specifies the recursion depth max to prevent Nelson from recursing infinitely.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
numeric types
About integer and floating-point data.
Description
In Nelson you can specify the data type of a numeric literal by using a suffix or a type specifier.
Here are some common suffixes for specifying the data type of numeric literals:
literal number suffix | Nelson type |
---|---|
f32 | single (float single precision) |
f64 | double (float double precision) |
i8 | int8 (8-bit signed integer) |
i16 | int16 (16-bit signed integer) |
i32 | int32 (32-bit signed integer) |
i64 | int64 (64-bit signed integer) |
u8 | uint8 (8-bit unsigned integer) |
u16 | uint16 (16-bit unsigned integer) |
u32 | uint32 (32-bit unsigned integer) |
u64 | uint64 (64-bit unsigned integer) |
i64: To specify a 64-bit signed integer, you can use the i64 suffix. example: A = 42i64
f32: To specify a 32-bit floating-point number (single precision), you can use the f64 suffix. example: 3.14f32
These suffixes help the Nelson infer the correct data type for the literal.
Nelson automatically infer data type by default as double and you don't need to specify this suffixe explicitly. example: A = 3.14
Unless you have specific requirements or need to disambiguate between data types, you often don't need to explicitly specify the type of numeric literals.
But when you create a numeric array of large integers in Nelson, especially when they exceed the maximum precision representable by double (larger than flintmax), Nelson initially stores these values as double-precision floating-point numbers by default.
Examples
explicit single number
single(3.1415)
3.1415f32
implicit-explicit double number
3.1415
3.1415f64
values exceed maximum precision representable by double
R1 = uint64([72057594035891654 81997179153022975])
R2 = [72057594035891654u64 81997179153022975u64]
See also
double, single, int8, int16, int32, int64, uint8, uint16, uint32, uint64.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
parsefile
Parse a Nelson file.
Syntax
- status = parsefile(filename)
Input argument
- filename - a string: a filename to parse.
Output argument
- status - a string: 'script', 'function', 'error'.
Description
parsefile parse a file and returns if it is a valid script, a valid function or an error.
Example
parsefile([nelsonroot(), '/etc/startup.m'])
parsefile([nelsonroot(), '/modules/data_structures/functions/cellstr.m'])
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
parsestring
Parse a string.
Syntax
- status = parsestring(str)
Input argument
- str - a string: a string to parse.
Output argument
- status - a string: 'script', 'function', 'error'.
Description
parsestring parse a string and returns if it is a valid script, a valid function or an error.
Example
parsestring('1 + 1')
parsestring('1 +++ 1')
parsestring('1 +*+ 1')
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
switch
switch statement.
Syntax
- switch(expression), case test_expression_1, statements, case test_expression_2, statements, otherwise statements, end
Description
switch statement is used to selective execute code based on the value of either scalar value or a string.
otherwise clause is optional.
Examples
demo_switch.m
function c = demo_switch(a)
switch(a)
case {'hello', 'world'}
c = 'message';
case {'red', 'green', 'blue'}
c = 'color';
otherwise
c = 'not sure';
end
end
demo_switch('hello')
demo_switch('red')
demo_switch('?')
See also
for.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
try
catch
try/catch statement.
Syntax
- try, statements_1, catch, statements_2, end
- try, statements_1, catch exception, statements_2, end
Description
try and catch statements are used for error handling and control in files.
exception is an MException object that allows you to identify the error.
The catch block assigns the current exception object to the variable in exception.
Examples
try/catch in a script file
try
error('an error')
catch
disp('error catched')
end
try/catch in a script file
try
error('an error')
catch ME
ME
end
See also
run, execstr, MException.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
while
while loop.
Syntax
- while test_expression, statements, end
Description
while loop executes a set of statements as long as a the test condition remains true.
Example
i = 0;
while lt(i, 10)
disp(i)
i = i + 1;
end
See also
for.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
Display format
Display format
Description
output display format functions
- disp - Display a variable.
- display - Show information about variable or result of expression.
- echo - Controls the echoing during their execution.
- format - Display format and number printing.
- formattedDisplayText - Capture display output as string.
disp
Display a variable.
Syntax
- disp(V)
Input argument
- V - a variable
Description
disp(V) displays the value of the variable V.
disp uses current format setting to display numeric values.
Examples
disp('Hello Nelson')
disp(pi)
disp(eye(3, 3))
disp always ends with a newline.
disp('')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
display
Show information about variable or result of expression.
Syntax
- display(V)
- display(V, name)
Input argument
- V - Result of executing a statement or expression
- name - a character vector: variable name displayed.
Description
display(V) displays information about the variable V.
Nelson calls display function whenever an object is referred to in a statement that is not terminated by a semicolon.
Examples
display(33, 'Hello')
display('Hello Nelson')
display(pi)
A = eye(3, 3); disp(A)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
echo
Controls the echoing during their execution.
Syntax
- state = echo()
- echo()
- echo('on')
- echo('off')
Input argument
- 'on' - enable echo mode (default)
- 'off' - disable echo mode
Output argument
- state - a string: 'on' or 'off'
Description
echo('off') disable echo mode.
Without input and output arguments, echo toggles the current echo state.
Example
an example
R = echo
echo('on')
A = 1+1
echo('off')
A = A+1
echo(R)
A
See also
disp.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
format
Display format and number printing.
Syntax
- fmt = format()
- format()
- format('default')
- format(new_style)
Input argument
- new_style - a string
Output argument
- fmt - DisplayFormatOptions object: format used
Description
format(new_style) changes the display format and number printing of the current session.
format('default') will reset to default format (short, loose).
Styles supported:
short
long
shortE
longE
shortEng
longEng
plus
rational
hex
Line Spacing Format supported:
loose
compact
Example
an example
current_style = format()
pi
format('short')
pi
format('long')
pi
format('shortE')
pi
format('longE')
pi
format('hex')
pi
format('+')
pi
format('rational')
pi
format('compact')
pi
format(current_style)
pi
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
formattedDisplayText
Capture display output as string.
Syntax
- str = formattedDisplayText(V)
- str = formattedDisplayText(V, Name, Value)
Input argument
- V - Variable to return as string
- Name, Value - Name-Value Pair Arguments, Name: 'NumericFormat' or 'LineSpacing'.
Output argument
- str - a string
Description
str = formattedDisplayText(V) returns the display output of V as a string.
The string contains equivalent to disp(V).
Example
R = eye(3, 3)
str = formattedDisplayText(R)
R = rand(3, 3);
disp(R)
str = formattedDisplayText(R)
str = formattedDisplayText(R, 'NumericFormat', 'bank', 'LineSpacing', 'compact')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
Localization functions
Localization functions
Description
localization functions
- getavailablelanguages - Returns available languages in Nelson.
- getdefaultlanguage - Returns the default language used in Nelson.
- getlanguage - Returns the current language in Nelson.
- setlanguage - Changes the language used in Nelson.
getavailablelanguages
Returns available languages in Nelson.
Syntax
- ce = getavailablelanguages()
Output argument
- ce - a cell of strings: supported languages.
Description
getavailablelanguages returns the list of currently supported languages in Nelson.
Example
getavailablelanguages()
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
getdefaultlanguage
Returns the default language used in Nelson.
Syntax
- lang = getdefaultlanguage()
Output argument
- lang - a string: 'en_US' by default.
Description
getdefaultlanguage returns the default language used by Nelson.
Example
getdefaultlanguage()
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
getlanguage
Returns the current language in Nelson.
Syntax
- lang = getlanguage()
Output argument
- lang - a string: current language used in Nelson.
Description
getlanguage returns the current language used in Nelson.
Example
l = getlanguage()
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
setlanguage
Changes the language used in Nelson.
Syntax
- setlanguage(language)
Input argument
- language - a string: 'en_US', 'fr_FR' or others by default.
Description
setlanguage changes the language used by Nelson and saves this changes for subsequent runs of Nelson.
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
I18n functions
I18n functions
Description
internalization functions
- gettext - Get text translated into the current locale.
- i18nHelpers - Internationalization (i18n) utility functions
- poheader - Generates po file header.
gettext
_
Get text translated into the current locale.
Syntax
- translated_string = gettext(your_string)
- translated*string = *(your_string))
Input argument
- your_string - a string: message to be translated.
Output argument
- translated_string - a string: message translated.
Description
translated_string = gettext(your_string) gets the translation of a string your_string to the current locale in the Nelson domain.
_(your_string) is an alias of gettext(your_string).
Example
disp(_('function not found.'))
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
i18nHelpers
Internationalization (i18n) utility functions
Syntax
- i18nHelpers('convert', potFile, jsonFile)
- i18nHelpers('merge', jsonFile1, jsonFile2)
- i18nHelpers('sort', jsonFileA, jsonFileB)
Input argument
- potFile - String: Path to the source .po/.pot translation template file
- jsonFile - String: Path to JSON translation file destination
- jsonFile1 - String: Path to the source JSON translation file
- jsonFile2 - String: Path to the destination JSON translation file
- jsonFileA - String: Path to the source JSON file to sort
- jsonFileB - String: Path to the sorted JSON file
Description
i18nHelpers provides essential utility functions for managing internationalization files. The main functions include:
- 'convert': Converts a .po/.pot translation template file into JSON format for easier manipulation.
- 'merge': Merges two JSON translation files. The entries from jsonFile1
are added to jsonFile2
, and entries exclusive to jsonFile2
are removed.
- 'sort': Sorts and organizes entries in a JSON translation file. jsonFileA
and jsonFileB
may refer to the same file if in-place sorting is desired.
This utility is intended for internal use and may be updated over time.
See also
History
Version | Description |
---|---|
1.10.0 | Initial version |
Author
Allan CORNET
poheader
Generates po file header.
Syntax
- ce = poheader(domain, language)
Input argument
- domain - a string: domain message.
- language - a string: language, examples 'en_US' or 'fr_FR'.
Output argument
- ce - a cell of string: po file header.
Description
ce = poheader(domain, language) generates po file header.
Example
poheader('nelson', 'en_US')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
Characters encoding
Characters encoding
Description
characters encoding functions
- native2unicode - Converts bytes representation to unicode characters
- nativecharset - Find all charset matches that appear to be consistent with the input
- unicode2native - Converts unicode characters representation to bytes
native2unicode
Converts bytes representation to unicode characters
Syntax
- str = native2unicode(bytes, charset)
Input argument
- bytes - a uint8 vector
- charset - an scalar string or vector characters array.
Output argument
- str - an vector characters array.
Description
native2unicode converts an uint8 vector to unicode characters.
str = native2unicode(bytes) converts an uint8 vector to unicode characters (using the native character set of the machine).
str = native2unicode(bytes, charset) converts an uint8 vector to unicode characters (character set charset instead of the native character set).
List of characters set: http://www.iana.org/assignments/character-sets/character-sets.xhtml
Bibliography
ICU library
Example
native2unicode(uint8([149 208 137 188 150 188]), 'SHIFT_JIS')
See also
unicode2native, native2unicode, char.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
nativecharset
Find all charset matches that appear to be consistent with the input
Syntax
- ce = nativecharset(bytes)
Input argument
- bytes - a uint8 vector, or string or row characters array
Output argument
- ce - a cell of strings.
Description
nativecharset find all charset matches that appear to be consistent with the input, returning a cell of string with results.
The results are ordered with the best quality match first.
List of characters set: http://www.iana.org/assignments/character-sets/character-sets.xhtml
Bibliography
ICU library
Example
C = uint8([194 232 240 242 243 224 235 252 237 224 255]);
nativecharset(R)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
unicode2native
Converts unicode characters representation to bytes
Syntax
- bytes = unicode2native(str, charset)
Input argument
- str - an scalar string or vector characters array.
- charset - an scalar string or vector characters array.
Output argument
- bytes - a uint8 vector
Description
unicode2native converts unicode characters to an numeric array.
bytes = unicode2native(str) converts unicode characters to an numeric array (the native character set of the machine).
bytes = unicode2native(str, charset) converts unicode characters to an numeric array (character set charset instead of the native character set).
List of characters set: http://www.iana.org/assignments/character-sets/character-sets.xhtml
Bibliography
ICU library
Example
R = unicode2native('片仮名', 'SHIFT_JIS')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
Types module
Types module
Description
module about types management.
- class - Return classname of object or creates a named object.
- isa - Return true if var is an object from the class str.
- iscell - Return true if variable var is a cell array.
- ischar - Return true if variable var is a char array.
- isclass - Return true if variable var is a class object.
- isdouble - Return true if variable var is a double matrix.
- isempty - Return true if variable var is an empty matrix.
- isfloat - Return true if variable var is a single or double matrix.
- ishandle - Return true if variable var is a handle object.
- isint16 - Return true if variable var is a signed 16-bit integer type array.
- isint32 - Return true if variable var is a signed 32-bit integer type array.
- isint64 - Return true if variable var is a signed 64-bit integer type array.
- isint8 - Return true if variable var is a signed 8-bit integer type array.
- isinteger - Return true if variable var is a integer type array.
- islogical - Return true if variable var is a logical.
- isnumeric - Return true if variable var is a numeric array.
- isobject - Return true if variable var is an object.
- isreal - Return true if all imaginary part is a zero array.
- issingle - Return true if variable var is a single matrix.
- issparse - Return true if variable var is a sparse array.
- isstring - Return true if variable var is a string array.
- isstruct - Return true if variable var is a structure.
- isuint16 - Return true if variable var is an unsigned 16-bit integer type array.
- isuint32 - Return true if variable var is an unsigned 32-bit integer type array.
- isuint64 - Return true if variable var is an unsigned 64-bit integer type array.
- isuint8 - Return true if variable var is an unsigned 8-bit integer type array.
- isvarname - Return true if input is valid variable name.
class
Return classname of object or creates a named object.
Syntax
- name = class(var)
- obj = class(st, strname)
Input argument
- var - a variable
- st - a struct
- strname - a string: classname desired
Output argument
- name - a string
- obj - an object of type 'strname' based on struct 'st'
Description
name = class(var) returns the class of var variable.
Standard classes are:
'cell'
'struct'
'single'
'double'
'logical'
'char'
'int8'
'int16'
'int32'
'int64'
'uint8'
'uint16'
'uint32'
'uint64'
'function_handle'
Examples
A = 3;
res = class(A)
C = [1 ; 3];
res = class(C)
addpath([nelsonroot(), '/modules/overload/examples/complex']);
c = complexObj(3,4);
class(c)
See also
isa, isdouble, isfloat, ischar, isstruct, iscell.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
isa
Return true if var is an object from the class str.
Syntax
- res = isa(var, str)
Input argument
- var - a variable
- str - a string: classname expected
Output argument
- res - a logical: true or false
Description
isa returns a logical 1 if the argument is a cell array and a logical 0 otherwise.
str can also be 'numeric', 'float', or 'integer':
numeric: floating point or integer array: double, single, int8, uint8, int16, uint16, int32, uint32, int64, uint64
float: single or double precision floating-point array: double, single
integer: unsigned or signed integer array: int8, uint8, int16, uint16, int32, uint32, int64, uint64
If var is a handle object, str can be 'handle' or type name of the handle.
Examples
A = 3;
res = isa(A, 'double')
B = {'NelSon', 3, true};
res = isa(B, 'cell')
B = {'NelSon', 3, true};
res = isa(B, 'cell')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
iscell
Return true if variable var is a cell array.
Syntax
- res = iscell(var)
Input argument
- var - a variable
Output argument
- res - a logical: true or false
Description
iscell returns a logical 1 if the argument is a cell array and a logical 0 otherwise.
Examples
A = 3;
res = iscell(A)
B = {'NelSon', 3, true};
res = iscell(B)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
ischar
Return true if variable var is a char array.
Syntax
- res = ischar(var)
Input argument
- var - a variable
Output argument
- res - a logical: true or false
Description
ischar returns a logical 1 if the argument is a char array and a logical 0 otherwise.
Examples
A = 3;
res = ischar(A)
B = 'NelSon';
res = ischar(B)
C = [1 ; 3];
res = ischar(C)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
isclass
Return true if variable var is a class object.
Syntax
- res = isclass(var)
Input argument
- var - a variable
Output argument
- res - a logical: true or false
Description
isclass returns a logical 1 if the argument is a class object and a logical 0 otherwise.
Example
A = 3;
res = isclass(A)
addpath([nelsonroot(), '/modules/overload/examples/complex']);
c = complexObj(3,4);
res = isclass(c)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
isdouble
Return true if variable var is a double matrix.
Syntax
- res = isdouble(var)
Input argument
- var - a variable
Output argument
- res - a logical: true or false
Description
isdouble returns a logical 1 if the argument is a double matrix and a logical 0 otherwise.
Examples
A = 3;
res = isdouble(A)
A = single(3);
res = isdouble(A)
A = single([3, i]);
res = isdouble(A)
A = [3, i];
res = isdouble(A)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
isempty
Return true if variable var is an empty matrix.
Syntax
- res = isempty(var)
Input argument
- var - a variable
Output argument
- res - a logical: true or false
Description
isempty returns a logical true if the argument is an empty matrix.
Any one of its dimensions is zero.
Examples
A = rand(3, 3, 3);
res = isempty(A)
A(:, :, :) = [];
res = isempty(A)
B = {};
res = isempty(B)
C = struct()
res = isempty(C)
C = struct([])
res = isempty(C)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
isfloat
Return true if variable var is a single or double matrix.
Syntax
- res = isfloat(var)
Input argument
- var - a variable
Output argument
- res - a logical: true or false
Description
isfloat returns a logical 1 if the argument is a single or double matrix and a logical 0 otherwise.
Examples
A = 3;
res = isfloat(A)
A = single(3);
res = isfloat(A)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
ishandle
Return true if variable var is a handle object.
Syntax
- res = ishandle(var)
Input argument
- var - a variable
Output argument
- res - a logical: true or false
Description
ishandle returns a logical 1 if the argument is a handle object and a logical 0 otherwise.
Example
A = 3;
res = ishandle(A)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
isint16
Return true if variable var is a signed 16-bit integer type array.
Syntax
- res = isint16(var)
Input argument
- var - a variable
Output argument
- res - a logical: true or false
Description
isint16 returns a logical 1if the argument is a signed 16-bit integer array and a logical 0 otherwise.
Examples
A = 3;
res = isint16(A)
B = int16(3);
res = isint16(B)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
isint32
Return true if variable var is a signed 32-bit integer type array.
Syntax
- res = isint32(var)
Input argument
- var - a variable
Output argument
- res - a logical: true or false
Description
isint32 returns a logical 1if the argument is a signed 32-bit integer array and a logical 0 otherwise.
Examples
A = 3;
res = isint32(A)
B = int32(3);
res = isint32(B)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
isint64
Return true if variable var is a signed 64-bit integer type array.
Syntax
- res = isint64(var)
Input argument
- var - a variable
Output argument
- res - a logical: true or false
Description
isint64 returns a logical 1if the argument is a signed 64-bit integer array and a logical 0 otherwise.
Examples
A = 3;
res = isint64(A)
B = int64(3);
res = isint64(B)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
isint8
Return true if variable var is a signed 8-bit integer type array.
Syntax
- res = isint8(var)
Input argument
- var - a variable
Output argument
- res - a logical: true or false
Description
isint8 returns a logical 1if the argument is a signed 8-bit integer array and a logical 0 otherwise.
Examples
A = 3;
res = isint8(A)
B = int8(3);
res = isint8(B)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
isinteger
Return true if variable var is a integer type array.
Syntax
- res = isinteger(var)
Input argument
- var - a variable
Output argument
- res - a logical: true or false
Description
isinteger returns a logical 1 if the argument is a integer type (int8, int16 ...) array and a logical 0 otherwise.
Examples
A = 3;
res = isinteger(A)
B = uint8(3);
res = isinteger(B)
A = single([3, i]);
res = isinteger(A)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
islogical
Return true if variable var is a logical.
Syntax
- res = islogical(var)
Input argument
- var - a variable
Output argument
- res - a logical: true or false
Description
islogical returns a logical 1 if the argument is a logical array and a logical 0 otherwise.
Examples
A = 1;
res = islogical(A)
B = logical(1);
res = islogical(B)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
isnumeric
Return true if variable var is a numeric array.
Syntax
- res = isnumeric(var)
Input argument
- var - a variable
Output argument
- res - a logical: true or false
Description
isnumeric returns a logical 1 if the argument is a numeric array and a logical 0 otherwise.
List of numeric types:
single : single precision
double : double precision
int8 : 8 bit signed integer
int16 : 16 bit signed integer
int32 : 32 bit signed integer
int64 : 64 bit signed integer
uint8 : 8 bit unsigned integer
uint16 : 16 bit unsigned integer
uint32 : 32 bit unsigned integer
uint64 : 64 bit unsigned integer
Examples
A = 1;
res = isnumeric(A)
B = single(1+i);
res = isnumeric(B)
C = logical(1);
res = isnumeric(C)
See also
islogical, isinteger, isdouble, issingle.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
isobject
Return true if variable var is an object.
Syntax
- res = isobject(var)
Input argument
- var - a variable
Output argument
- res - a logical: true or false
Description
ishandle returns a logical 1 if the argument is an object and a logical 0 otherwise.
Example
A = 3;
res = isobject(A)
addpath([modulepath('overload', 'root'), '/examples/complex']);
A = complexObj(1, 2);
res = isobject(A)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
isreal
Return true if all imaginary part is a zero array.
Syntax
- res = isreal(var)
Input argument
- var - a variable
Output argument
- res - a logical: true or false
Description
isreal returns a logical true if var is a non-complex matrix or scalar and a logical false otherwise.
Examples
A = 1 + 0i;
res = isreal(A)
B = uint8(3);
res = isreal(B)
A = single([3, i]);
res = isreal(A)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
issingle
Return true if variable var is a single matrix.
Syntax
- res = issingle(var)
Input argument
- var - a variable
Output argument
- res - a logical: true or false
Description
issingle returns a logical 1 if the argument is a single matrix and a logical 0 otherwise.
Examples
A = 3.6;
res = issingle(A)
B = single([1 ; 3]);
res = issingle(B)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
issparse
Return true if variable var is a sparse array.
Syntax
- res = issparse(var)
Input argument
- var - a variable
Output argument
- res - a logical: true or false
Description
issparse returns a logical 1 if the argument is a sparse array and a logical 0 otherwise.
Examples
A = 1;
res = issparse(A)
B = sparse(1);
res = issparse(B)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
isstring
Return true if variable var is a string array.
Syntax
- res = isstring(var)
Input argument
- var - a variable
Output argument
- res - a logical: true or false
Description
isstring returns a logical 1 if the argument is a string array and a logical 0 otherwise.
Examples
A = 3;
res = isstring(A)
B = "NelSon";
res = isstring(B)
C = [1 ; 3];
res = isstring(C)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
isstruct
Return true if variable var is a structure.
Syntax
- res = isstruct(var)
Input argument
- var - a variable
Output argument
- res - a logical: true or false
Description
isstruct returns a logical 1 if the argument is a struct (structure) and a logical 0 otherwise.
Examples
A = 1;
res = isstruct(A)
B = struct();
res = isstruct(B)
C.a = 1;
C.B = 'hello';
res = isstruct(C)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
isuint16
Return true if variable var is an unsigned 16-bit integer type array.
Syntax
- res = isuint16(var)
Input argument
- var - a variable
Output argument
- res - a logical: true or false
Description
isuint16 returns a logical 1if the argument is an unsigned 16-bit integer array and a logical 0 otherwise.
Examples
A = 3;
res = isuint16(A)
B = uint16(3);
res = isuint16(B)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
isuint32
Return true if variable var is an unsigned 32-bit integer type array.
Syntax
- res = isuint32(var)
Input argument
- var - a variable
Output argument
- res - a logical: true or false
Description
isuint32 returns a logical 1if the argument is an unsigned 32-bit integer array and a logical 0 otherwise.
Examples
A = 3;
res = isuint32(A)
B = uint32(3);
res = isuint32(B)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
isuint64
Return true if variable var is an unsigned 64-bit integer type array.
Syntax
- res = isuint64(var)
Input argument
- var - a variable
Output argument
- res - a logical: true or false
Description
isuint64 returns a logical 1if the argument is an unsigned 64-bit integer array and a logical 0 otherwise.
Examples
A = 3;
res = isuint64(A)
B = uint64(3);
res = isuint64(B)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
isuint8
Return true if variable var is an unsigned 8-bit integer type array.
Syntax
- res = isuint8(var)
Input argument
- var - a variable
Output argument
- res - a logical: true or false
Description
isuint8 returns a logical 1if the argument is an unsigned 8-bit integer array and a logical 0 otherwise.
Examples
A = 3;
res = isuint8(A)
B = uint8(3);
res = isuint8(B)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
isvarname
Return true if input is valid variable name.
Syntax
- res = isvarname(var)
Input argument
- var - a variable
Output argument
- res - a logical: true or false
Description
isvarname returns a logical 1 if the argument is a valid variable name and a logical 0 otherwise.
Example
isvarname(4)
isvarname('t')
isvarname('8t')
isvarname('t8t')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
Overloading
Overloading
Description
Overloading capabilities on functions and operators.
- overloading - Customizing Operators and Functions
overloading
Customizing Operators and Functions
Description
In various scenarios, you may find it necessary to modify the behavior of Nelson's operators and functions when they operate on objects or basic types.
This customization can be achieved by overloading the relevant functions, allowing them to handle diverse types and quantities of input arguments and execute the appropriate operation for the highest-priority object.
Overloading Operators:
Each built-in operator corresponds to a specific function name (e.g., the - operator is associated with the minus.m function).
You can overload any operator by creating an M-file with the appropriate name within the class directory.
For instance, if either A or B is an object of type classname, the expression A - B triggers a call to a function @classname/minus.m, provided it exists.
When A and B belong to different classes, Nelson employs precedence rules to determine which method to apply.
The table below provides a list of function names associated with most of the Nelson operators:
Description | Operator | Function |
---|---|---|
Binary addition | a + b | plus(a, b) |
Binary subtraction | a - b | minus(a, b) |
Unary minus | -a | uminus(a) |
Unary plus | +a | uplus(a) |
Element-wise multiplication | a .* b | times(a, b) |
Matrix multiplication | a * b | mtimes(a, b) |
Right element-wise division | a ./ b | rdivide(a, b) |
Left element-wise division | a .\ b | ldivide(a, b) |
Matrix right division | a / b | mrdivide(a, b) |
Matrix left division | a \ b | mldivide(a, b) |
Element-wise power | a .^ b | power(a, b) |
Matrix power | a ^ b | mpower(a, b) |
Less than | a < b | lt(a, b) |
Greater than | a > b | gt(a, b) |
Less than or equal to | a <= b | le(a, b) |
Greater than or equal to | a >= b | ge(a, b) |
Not equal to | a ~= b | ne(a, b) |
Equality | a == b | eq(a, b) |
Logical AND | a & b | and(a, b) |
Logical OR | a | b | or(a, b) |
Logical NOT | ~a | not(a) |
Colon operator | a:d:b | colon(a, d, b) |
Complex conjugate transpose | a' | ctranspose(a) |
Matrix transpose | a.' | transpose(a) |
Display method | command window output | display(a) |
Horizontal concatenation | [a, b] | horzcat(a, b, ...) |
Vertical concatenation | [a; b] | vertcat(a, b, ...) |
Subscripted reference | a(s1, s2, ... , sn) | subsref(a, s) |
Subscripted assignment | a(s1, ... , sn) = b | subsasgn(a, s, b) |
Subscript index | b(a) | subsindex(a) |
Example
Overload minus operator with double
% save in @double directory, as minus.m
function r = minus(A, B)
disp('minus was called')
% to call minus builtin
r = builtin('minus', A, B)
end
See also
plus, minus, uminus, uplus, times, mtimes, rdivide, ldivide, mrdivide, mldivide, power, mpower, lt, gt, le, ge, ne, eq, and, or, not, colon, ctranspose, transpose, display, horzcat, vertcat, subsref, subsasgn, subsindex.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
Logical type functions
Logical type functions
Description
logical type functions
- false - Logical false.
- logical - Converts a numeric value to logical type.
- true - Logical true.
- xor - Exclusive or.
false
Logical false.
Syntax
- false
- l = false(n)
- l = false(sz)
- l = false(n, m, ..., k)
- l = false(n, m, 'like', sp)
Input argument
- n - a integer value.
- sz - a size vector.
- n, m, ..., k - a n -by- m - ... -by- k array to indicate size.
- sp - a sparse or array.
Output argument
- l - a logical value: false.
Description
false build a matrix of false.
Example
false
false(4)
false(4, 1, 4)
L = logical(sparse(1, 2))
L2 = false(3,'like', L);
See also
true.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
logical
Converts a numeric value to logical type.
Syntax
- Y = logical(X)
Input argument
- X - a numeric value.
Output argument
- Y - a logical value.
Description
logical converts a numeric value to logical type.
Nonzero value converted to true and zeros values converted to false.
Complex numbers returns an error.
Example
A = eye(2, 2)
B = logical(A)
islogical(B)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
true
Logical true.
Syntax
- true
- l = true(n)
- l = true(sz)
- l = true(n, m, ..., k)
- l = true(n, m, 'like', sp)
Input argument
- n - a integer value.
- sz - a size vector.
- n, m, ..., k - a n -by- m - ... -by- k array to indicate size.
- sp - a sparse or array.
Output argument
- l - a logical value: true.
Description
true build a matrix of true.
Example
true
true(4)
true(4, 1, 4)
L = logical(sparse(1, 2))
L2 = true(3,'like', L);
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
xor
Exclusive or.
Syntax
- R = xor(V1, V2)
- R = xor(V1, V2, ... , VN)
Input argument
- V1 - a matrix.
- V2 - a matrix, same dimensions than V1.
- VN - a matrix, same dimensions than V1.
Output argument
- R - a logical matrix.
Description
xor performs a logical exclusive-OR.
Example
x = [0 1 0 1];
y = [0 0 1 1];
R = xor(x, y)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
Single type
Single type
Description
single type functions
- single - Converts a variable to single precision type.
single
Converts a variable to single precision type.
Syntax
- S = single(V)
Input argument
- V - a variable.
Output argument
- S - a single.
Description
single(V) converts to the single-precision type.
Examples
single('Nelson')
A = single(pi)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
Double
Double
Description
double type functions
- double - Converts a variable to double precision type.
- flintmax - Largest consecutive integer in floating-point format.
- realmax - Largest positive floating-point number.
- realmin - Smallest positive floating-point number.
double
Converts a variable to double precision type.
Syntax
- D = double(V)
Input argument
- V - a variable.
Output argument
- D - a double.
Description
double(V) converts to the double-precision type.
Examples
double('Nelson')
A = single(pi)
B = double(A)
B - A
A = ["3.134", "NaN"; "Inf", "-5"];
B = double(A)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
flintmax
Largest consecutive integer in floating-point format.
Syntax
- R = flintmax()
- R = flintmax('double')
- R = flintmax('single')
- R = flintmax('like', V)
Input argument
- V - a double or single variable.
Output argument
- R - a double or single.
Description
flintmax returns largest consecutive integer in floating-point format.
Example
flintmax
flintmax('double')
flintmax('like', pi)
flintmax('single')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
realmax
Largest positive floating-point number.
Syntax
- R = realmax()
- R = realtmax('double')
- R = realmax('single')
Output argument
- R - a double or single.
Description
realmax returns largest positive floating-point number.
Example
realmax
realmax('double')
realmax('single')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
realmin
Smallest positive floating-point number.
Syntax
- R = realmin()
- R = realmin('double')
- R = realmin('single')
Output argument
- R - a double or single.
Description
realmin returns smallest positive floating-point number.
Example
realmin
realmin('double')
realmin('single')
See also
History
Version | Description |
---|---|
1.10.0 | initial version |
Author
Allan CORNET
Data structures
Data structures
Description
data structures functions
- cell - Create cell array of empty matrices.
- cell2mat - Transform a cell array containing matrices into a single, concatenated matrix.
- cell2struct - Creates a struct from a cell.
- celldisp - Display cell array contents.
- cellfun - Evaluates an function on a cell.
- cellstr - Converts to cell of character array.
- fieldnames - Returns field names of a structure or an handle.
- getfield - Returns value of a field in a struct.
- iscellstr - Returns if a variable is a cell of strings.
- isfield - Checks if a fieldname exists in a struct.
- namedargs2cell - Converts a struct containing name-value pairs to a cell.
- num2cell - Convert array to cell array with consistently sized cells.
- orderfields - Reorganize the fields of a structured array.
- rmfield - Remove fields from structure.
- setfield - Set structure field contents.
- struct - Creates a struct.
- struct2cell - Creates a cell from a structure.
cell
Create cell array of empty matrices.
Syntax
- C = cell()
- C = cell(m)
- C = cell(m, n)
- C = cell(m, n, ... , p)
- C = cell(sz)
- C = cell(A)
Input argument
- m, n, ... , p - dimensions of the cell to create.
- sz - a vector of integer values (dimensions of the cell to create).
- A - a string array.
Output argument
- C - a cell
Description
cell returns a cell array of empty matrices.
cell() is equivalent to cell(0)
cell(A) with A a string array converts to cell.
Examples
A = eye(2, 4);
sz = size(A)
C = cell(sz)
A = ["Nel", "son"; "open", "source"];
C = cell(A)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
cell2mat
Transform a cell array containing matrices into a single, concatenated matrix.
Syntax
- M = cell2smat(ce)
Input argument
- ce - a cell.
Output argument
- M - array.
Description
M = cell2smat(ce) creates a single matrix by merging all elements within the cell array ce into a multi-dimensional array. The elements in c can consist of numeric, logical, or character matrices, cell arrays, or structs, and they must be compatible for concatenation using cat function.
Example
C = {[10], [20 30 40]; [90; 50], [60 76 88; 110 111 112]};
M = cell2mat(C)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
cell2struct
Creates a struct from a cell.
Syntax
- st = cell2struct(ce, fields)
- st = cell2struct(ce, fields, dim)
Input argument
- ce - a cell.
- fields - a cell of strings.
- dim - dimension along cell is converted.
Output argument
- st - a struct array.
Description
st = cell2struct(ce, fields) creates a struct from a cell.
Example
ce = {85, 50, 68; 'Pierre', 'Anna', 'Roberto'}
fields = {'Height','Name'}
A = cell2struct (ce, fields, 1)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
celldisp
Display cell array contents.
Syntax
- celldisp(C)
- celldisp(C, name)
Input argument
- C - cell array.
- name - displayed name of cell array.
Description
celldisp recursively display the contents of a cell array.
Example
C = {2, 22, 'ff', {331, 332}};
celldisp(C)
celldisp(C, 'var_name')
See also
disp.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
cellfun
Evaluates an function on a cell.
Syntax
- R = cellfun(function_name, ce)
- R = cellfun(function_handle, ce)
- [R1, ... , Rp] = cellfun(function_handle, ce1, ..., cep)
- [R1, ... , Rp] = cellfun(function_handle, ce1, ..., cep, name, value)
Input argument
- function_handle - a function handle.
- ce1, ... , cep - cells with p inputs required for function_handle.
- name, value pair - 'UniformOutput': true or false, 'ErrorHandler': a error function.
Output argument
- R1, ... , Rp - Outputs from function
Description
cellfun applies function to each cell elements.
Examples
greetings = {'Hello', 'Guten Tag', 'Sawadee', 'Bonjour', 'Namaste', ''};
R = cellfun('size', greetings, 1)
R1 = cellfun('size', greetings, 2)
C = {1:10, eye(3,4), eye(5,6)};
f = str2func('size');
[nrows_1, ncols_1] = cellfun(f, C,'UniformOutput', false)
[nrows_2, ncols_2] = cellfun(f, C,'UniformOutput', true)
functions to define for next example:
function r = fun1(x, y)
r = x > y;
end
function result = errorfun(S, varargin)
disp(nargin())
disp(S)
disp(class(varargin))
disp(size(varargin))
disp(varargin{1})
disp(varargin{2})
result = false;
end
R = str2func('fun1');
H = str2func('errorfun');
A = {rand(3)};
B = {rand(5)};
AgtA = cellfun(R, A, B, 'ErrorHandler', H, 'UniformOutput', true)
AgtB = cellfun(R, A, B, 'ErrorHandler', H, 'UniformOutput', false)
See also
cell.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
cellstr
Converts to cell of character array.
Syntax
- ce = cellstr(A)
Input argument
- A - a string, a string array, cell of character array.
Output argument
- ce - a cell of character array
Description
cellstr(A) converts to cell of character array.
Examples
cellstr('Nelson')
cellstr({'Nelson'})
cellstr({})
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
fieldnames
Returns field names of a structure or an handle.
Syntax
- names = fieldnames(st)
- names = fieldnames(h)
- names = fieldnames(h, '-full')
Input argument
- st - a structure
- h - a handle object
Output argument
- names - a cell of strings
Description
names = fieldnames(st) returns a cell of strings with the names of the fields in the input structure.
names = fieldnames(h) returns a cell of strings with the names of the properties in the handle (without hidden).
names = fieldnames(h, '-full') returns a cell of strings with the names of the all properties in the handle.
Example
fieldnames(dir())
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
getfield
Returns value of a field in a struct.
Syntax
- value = getfield(st, field)
Input argument
- st - a structure.
- field - a string.
Output argument
- value - the value of a field from a structure.
Description
value = getfield(st, field) returns the value of the field named field from a structure.
Example
example.a = 1
example.b = 'nelson'
example.c = []
getfield(example, 'b')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
iscellstr
Returns if a variable is a cell of strings.
Syntax
- true_or_false = iscellstr(A)
Input argument
- A - a variable
Output argument
- true_or_false - a logical
Description
iscellstr(A) returns true if A is a cell of strings or an empty cell).
Examples
iscellstr('Nelson')
iscellstr({'Nelson'})
iscellstr({})
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
isfield
Checks if a fieldname exists in a struct.
Syntax
- res = isfield(S, name)
- res = isfield(S, C)
Input argument
- S - a struct
- name - a string
- C - a cell
Output argument
- res - a logical
Description
isfield(A) returns true if name is a fieldname of S.
Examples
S.Nelson = 1;
isfield(S, 'Nel')
isfield(S, 'Nelson')
S.nel = 1;
S.son = 2;
isfield(S,{ 1, 'nel'; 2, 'son'})
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
namedargs2cell
Converts a struct containing name-value pairs to a cell.
Syntax
- ce = namedargs2cell(st)
Input argument
- st - a scalar structure.
Output argument
- ce - a cell.
Description
ce = namedargs2cell(st) returns an cell containing name-value pairs.
Example
S = struct();
S.CharacterEncoding = 'auto';
S.Timeout = 5;
S.Username = "";
S.logical = false;
R = namedargs2cell(S)
See also
struct2cell, struct, fieldnames.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
num2cell
Convert array to cell array with consistently sized cells.
Syntax
- C = num2cell(A)
- C = num2cell(A, dim)
Input argument
- A - any type of multidimensional array.
- dim - positive integer value or positive vector of integers.
Output argument
- C - a cell array.
Description
num2cell function converts a numeric array into a cell array, where each element of the numeric array is placed in its own cell in the resulting cell array.
If A is a character array, num2cell will convert each row of the array into a separate cell in the resulting cell array.
Example
A = [1 2; 3 4; 5 6];
C = num2cell(A)
C = num2cell(A, 1)
C = num2cell(A, 2)
See also
cell.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
orderfields
Reorganize the fields of a structured array.
Syntax
- S = orderfields(S1)
- S = orderfields(S1, S2)
- S = orderfields(S1, C)
- S = orderfields(S1, P)
- [S, Pout] = orderfields(...)
Input argument
- S1 - structure array: Input structure.
- S2 - structure array: Field order by structure.
- C - cell array of character vectors or string array: Field order by name
- P - numeric vector: Field order by number.
Output argument
- S - structure array: Reordered structure.
- Pout - numeric vector: Output field order.
Description
S = orderfields(S1) sorts the fields in S1 alphabetically by their names, considering uppercase letters before lowercase ones, and digits and underscores are also accounted for.
S = orderfields(S1,S2) returns a copy of S1 with its fields rearranged to match the order of fields in S2.Both S1 and S2 must share the same field names.
S = orderfields(S1, C) matches the order specified in the input array C. Each field name from S1 must appear once in C.
S = orderfields(S1, P) reorders fields based on the permutation vector P. P contains integers from 1 to n, where n is the number of fields in S1. This syntax is useful for maintaining consistent ordering across multiple structure arrays.
[S, Pout] = orderfields(...) also returns a permutation vector Pout, indicating the changes in field order. Pout consists of integers from 1 to n, reflecting the rearranged field positions. This syntax is compatible with any of the previously mentioned arguments.
orderfields function exclusively arranges the top-level fields and doesn't operate recursively.
Example
s = struct ("d", 4, "b", 2, "a", 1, "c", 3);
tA = orderfields (s)
t = struct ("d", {}, "c", {}, "b", {}, "a", {});
tB = orderfields (s, tA)
See also
History
Version | Description |
---|---|
1.5.0 | initial version |
Author
Allan CORNET
rmfield
Remove fields from structure.
Syntax
- s = rmfield(st, field)
Input argument
- st - a structure.
- field - a string, cell of strings, or char.
Output argument
- s - a structure without field.
Description
s = rmfield(st, field) removes the specified field from structure array.
Example
example.a = 1
example.b = 'nelson'
example.c = []
rmfield(example, 'b')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
setfield
Set structure field contents.
Syntax
- stOut = setfield(stIn, fieldname, fieldvalue)
- stOut = setfield(stIn, fieldname1, fieldvalue1, ..., fieldnameN, fieldvalueN)
Input argument
- stIn - a structure.
- fieldname - a string or characters vector.
- fieldvalue - a variable value.
Output argument
- stOut - a structure: result.
Description
Set the contents of the specified field to the value.
Alternative syntax: S.(fieldname) = fieldvalue
Alternative syntax: S(idx1, idx2).(fieldname) = fieldvalue
Example
A = {};
setfield(A, 'vv', 3)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
struct
Creates a struct.
Syntax
- st = struct()
- st = struct([])
- st = struct(object)
- st = struct(field, value)
- st = struct(field, value, field2, value2, ..., fieldn, valuen)
Input argument
- field, field2, ... , fieldn - strings : field names, valid names are same than variable identifiers.
- value, value2, ..., valuen - all data types supported by Nelson.
- object - an object created with 'class' builtin.
Output argument
- st - a struct
Description
struct returns a structure.
Examples
struct()
struct([])
date_st = struct('day', 15, 'month' ,'August','year', 1974)
Other way to create a struct:
date_st.day = 15
date_st.month = 'August'
date_st.year = 1974)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
1.3.0 | Scalar String allowed as field name. |
Author
Allan CORNET
struct2cell
Creates a cell from a structure.
Syntax
- ce = struct2cell(st)
Input argument
- st - a structure.
Output argument
- ce - a cell.
Description
ce = struct2cell(st) returns a new cell from the structure.
Example
names = {'Pierre', 'Anna', 'Roberto'}
values = {45, 42, 13}
st = struct ('name', names, 'age', values);
ce = struct2cell(st)
See also
cell, struct, fieldnames.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
Dictionaries
Dictionaries
Description
Map data with keys that index values.
- configureDictionary - Generate a dictionary with defined key and value types.
- dictionary - Object that maps unique keys to values.
- entries - Key-value pairs of dictionary.
- insert - Add entries to a dictionary.
- isConfigured - Check if dictionary has types assigned to keys and values.
- isKey - Check if dictionary contains key
- keyHash - Create a hash code for a dictionary key.
- keyMatch - Check whether two dictionary keys are same.
- keys - Keys of dictionary.
- lookup - Find value in dictionary by key.
- numEntries - Number of key-value pairs in dictionary.
- remove - Remove dictionary entries.
- types - Types of dictionary keys and values.
- values - Values of dictionary.
configureDictionary
Generate a dictionary with defined key and value types.
Syntax
- d = configureDictionary(keyType, valueType)
Input argument
- keyType - Key data type: string scalar or character vector.
- valueType - Value data type: string scalar or character vector.
Output argument
- d - scalar: a dictionary object.
Description
d = configureDictionary(keyType, valueType) initializes an empty dictionary that enforces keys of type keyType and values of type valueType.
Example
d1 = configureDictionary("string", "single")
d2 = configureDictionary("cell", "struct")
See also
History
Version | Description |
---|---|
1.5.0 | initial version |
Author
Allan CORNET
dictionary
Object that maps unique keys to values.
Syntax
- d = dictionary()
- d = dictionary(d1)
- d = dictionary(keys, values)
- d = dictionary(key1, value1, ... , keyN, valueN)
Input argument
- d1 - a dictionary or py.dict object.
- keys - scalar or array
- values - scalar, array or cell array
- key1, value1, ... , keyN, valueN - Key-value pairs
Output argument
- d - scalar: a dictionary object.
Description
d = dictionary(): This command initializes an empty dictionary with no keys or values.
Initially, the dictionary has no specific data types assigned to its keys or values. Once entries are added, the data types for keys and values are determined based on these entries.
d = dictionary(keys, values): This creates a dictionary using the provided keys and values.
The resulting dictionary is a 1-by-1 scalar object. If a key appears multiple times, only the last corresponding value is kept. If the values parameter is a scalar, each key is assigned this value. When keys and values are arrays, they must have matching sizes, resulting in key-value pairs accordingly.
Dictionaries are typed according to their entries. All keys must share the same data type, and all values must share a different, consistent data type. If a new entry has parts that don't match the existing data types, Nelson will attempt to convert them. Keys and values can have different data types, and character row vectors are converted to string scalars.
d = dictionary(key1, value1, ... , keyN, valueN): This syntax creates a dictionary with the specified key-value pairs.
If a key is repeated, only the last key-value pair for that key is kept.
Removing an Entry from a Dictionary:
d(keys) = []: This command removes the entry associated with the specified key from the dictionary.
Assigning Values to Entries:
d(keys) = newValues: This command assigns the elements of newValues to the entries specified by the corresponding keys.
If a specified key does not exist in the dictionary, a new entry is created. If a key appears multiple times, only the last assigned value is kept. Assigning a new value to an existing key overwrites its previous value.
Looking Up a Value:
bvalue = d(keys): This command retrieves the value corresponding to the specified keys from the dictionary.
Storing Multiple Data Types in a Dictionary:
value = d{keys} retrieves the value associated with keys and returns the contents of the cell. If keys is an array, a comma-separated list of the corresponding values is returned. An error is thrown if the dictionary's values are configured to a datatype other than cell.
d{keys} = values assigns cells containing the elements of values to the entries specified by the corresponding keys. An error is thrown if the dictionary's values are configured to a datatype other than cell.
Examples
d = dictionary()
d('apple') = 1
d('banana') = 2
d('kiwi') = 3
d('banana') = []
Values = {{'a','b'},["ff", "cc"],struct,[1 2 3 4]}
Keys = ["letters" "words" "a structure" "numeric array"]
d = dictionary(Keys, Values)
d{"numeric array"}
d{"a new entry"} = 'table'
dictionary conversion nelson -- python
wheels = [1 2 3];
names = ["Monocycle" "Bicycle" "Tricycle"];
d = dictionary(wheels, names)
R = pyrun("A = d", "A", 'd', d)
dictionary(R)
See also
lookup, remove, insert, keyMatch.
History
Version | Description |
---|---|
1.5.0 | initial version |
Author
Allan CORNET
entries
Key-value pairs of dictionary.
Syntax
- E = entries(d)
- E = entries(d, format)
Input argument
- d - scalar: dictionary object.
- format - format: string scalar or character vector: 'cell', 'struct', 'table' (not yet implemented) .
Output argument
- E - table, struct or cell.
Description
E = entries(d) retrieves a table containing the key-value pairs from the given dictionary, d.
E = entries(d) currently not implemented.
E = entries(d, format) specifies the output format as either a table or a structure. For instance, entries(d, "struct") returns a structure containing the key-value pairs of d. This option is useful for data types that are not compatible with tables.
Example
names = ["Biil" "John" "Yann"];
wheels = [1 2 3];
d = dictionary(wheels, names)
E = entries(d, 'struct')
E = entries(d, 'cell')
See also
History
Version | Description |
---|---|
1.5.0 | initial version |
Author
Allan CORNET
insert
Add entries to a dictionary.
Syntax
- db = insert(da, key, value)
- db = insert(da, key, value, 'Overwrite', tf)
Input argument
- da - scalar: a dictionary object.
- key - scalar or array: key
- value - scalar or array: value. size of key must be compatible with the size of value.
- tf - true force to Overwrite, false do not overwrite and ignore change
Output argument
- db - scalar: a dictionary object.
Description
db = insert(da, key, value) adds the key-value pair to the dictionary da.
If the key already exists, its value is updated.
d = insert(d, key, value) is equivalent to d[key] = value.
db = insert(da, key, value, 'overwrite', tf) specifies whether to overwrite an existing value for the key based on the boolean parameter Overwrite.
Example
names = ["Apple" "Banana" "Kiwi"];
wheels = [1 2 3];
d = dictionary(wheels, names)
d = insert(d, [2 4] ,["Orange" "Citra"], 'Overwrite', false)
See also
History
Version | Description |
---|---|
1.5.0 | initial version |
Author
Allan CORNET
isConfigured
Check if dictionary has types assigned to keys and values.
Syntax
- tf = isConfigured(d)
Input argument
- d - scalar: dictionary object.
Output argument
- tf - scalar logical: true if configured, false if not.
Description
tf = isConfigured(d) returns a logical true if the specified dictionary is configured, and a logical false if it is not.
A dictionary is considered configured when it has assigned types for its keys and values. Adding entries to an unconfigured dictionary will configure it.
Example
names = ["Biil" "John" "Yann"];
wheels = [1 2 3];
d = dictionary(wheels, names)
tf = isConfigured(d)
d2 = dictionary()
tf = isConfigured(d2)
See also
dictionary, configureDictionary, insert, values.
History
Version | Description |
---|---|
1.5.0 | initial version |
Author
Allan CORNET
isKey
Check if dictionary contains key
Syntax
- tf = isKey(d)
Input argument
- d - scalar: dictionary object.
Output argument
- tf - scalar logical: true if key, false if not.
Description
tf = isKey(d, key) returns a logical true if the specified key exists in the configured dictionary, and a logical false if it does not.
If d is an unconfigured dictionary, isKey throws an error.
If key is an array of multiple keys, then tf is a logical array of the same size.
Example
names = ["Biil" "John" "Yann"];
wheels = [1 2 3];
d = dictionary(wheels, names)
tf = isKey(d, "John")
tf = isKey(d, ["biil" , "Yannis")
See also
dictionary, configureDictionary, keys.
History
Version | Description |
---|---|
1.5.0 | initial version |
Author
Allan CORNET
keyHash
Create a hash code for a dictionary key.
Syntax
- H = keyHash(A)
Input argument
- A - array
Output argument
- H - scalar: uint64, Hash code.
Description
H = keyHash(A) returns a uint64 scalar representing the input array, A.
The keyHash function computes a hash code derived from the characteristics of the input.
For custom classes, keyHash might require overloading to guarantee proper equivalence.
Example
keyHash({'a', 'b', 1})
keyHash({1, 'a', 'b'})
See also
History
Version | Description |
---|---|
1.5.0 | initial version |
Author
Allan CORNET
keyMatch
Check whether two dictionary keys are same.
Syntax
- tf = keyMatch(A, B)
Input argument
- A - array
- B - array
Output argument
- tf - logical: true or false.
Description
tf = keyMatch(A, B) returns true if arrays A and B have identical classes, properties, dimensions, and values, and returns false otherwise.
For custom classes, overloading keyMatch may be necessary to ensure accurate equivalence.
Example
A = {'a', 'b', 1};
B = {1, 'a', 'b'};
C = A;
D = B;
keyMatch(A, B)
keyMatch(A, C)
keyMatch(B, D)
See also
History
Version | Description |
---|---|
1.5.0 | initial version |
Author
Allan CORNET
keys
Keys of dictionary.
Syntax
- k = keys(d)
- k = keys(d, 'cell')
Input argument
- d - scalar: dictionary object.
Output argument
- k - keys.
Description
k = keys(d) retrieves an array containing the keys of the specified dictionary, d.
k = keys(d, 'cell') optionally returns the keys as a cell array.
Example
names = ["Biil" "John" "Yann"];
wheels = [1 2 3];
d = dictionary(wheels, names)
k = keys(d)
k = keys(d, 'cell')
See also
History
Version | Description |
---|---|
1.5.0 | initial version |
Author
Allan CORNET
lookup
Find value in dictionary by key.
Syntax
- value = lookup(d, key)
- value = lookup(d, key, 'FallbackValue', fallback)
Input argument
- d - scalar: dictionary object.
- key - key type must match or be convertible to the data type of keys in d.
- fallback - scalar: Fallback value
Output argument
- value - value.
Description
value = lookup(d, key) retrieves the value associated with the given key in dictionary d.
If the key does not exist, an error is raised.
value = lookup(d, key) is equivalent to value = d[key].
value = lookup(d, key, 'FallbackValue', fallback) specifies a fallback value to return if the key is not found in d.
lookup function only validates the fallback if it is needed. An error is raised only if the key is not found and no valid fallback is provided.
Example
names = ["Apple" "Banana" "Kiwi"];
wheels = [1 2 3];
d = dictionary(wheels, names)
v = lookup(d,[3,5], 'FallbackValue', "Orange")
See also
History
Version | Description |
---|---|
1.5.0 | initial version |
Author
Allan CORNET
numEntries
Number of key-value pairs in dictionary.
Syntax
- n = numEntries(d)
Input argument
- d - scalar: dictionary object.
Output argument
- n - scalar: number of entries.
Description
n = numEntries(d) retrieves the number of key-value pairs stored in the dictionary.
If d is an unconfigured dictionary, then numEntries returns 0.
Example
names = ["Biil" "John" "Yann"];
wheels = [1 2 3];
d = dictionary(wheels, names)
n = numEntries(d)
See also
dictionary, entries, keys, values.
History
Version | Description |
---|---|
1.5.0 | initial version |
Author
Allan CORNET
remove
Remove dictionary entries.
Syntax
- db = remove(da, key)
Input argument
- da - scalar: a dictionary object.
- key - scalar or array: key
Output argument
- db - scalar: a dictionary object.
Description
db = remove(da, key) deletes the entry associated with the key from dictionary da.
d = remove(d, key) is equivalent to d[key] = [].
Example
names = ["Apple" "Banana" "Kiwi"];
wheels = [1 2 3];
d = dictionary(wheels, names)
d = remove(d, 2)
See also
History
Version | Description |
---|---|
1.5.0 | initial version |
Author
Allan CORNET
types
Types of dictionary keys and values.
Syntax
- [keyType, valueType] = types(d)
- keyType = types(d)
Input argument
- d - scalar: dictionary object.
Output argument
- keyType - string scalar: Data type of dictionary keys.
- valueType - string scalar: Data type of dictionary values.
Description
keyType = types(d) returns the data type of the keys in the dictionary.
[keyType, valueType] = types(d) returns the data types of the keys and values in the specified dictionary. If the dictionary d is not configured, types returns a string scalar indicating missing.
Example
names = ["Biil" "John" "Yann"];
wheels = [1 2 3];
d = dictionary(wheels, names)
[keyType, valueType] = types(d)
See also
dictionary, keys, values.
History
Version | Description |
---|---|
1.5.0 | initial version |
Author
Allan CORNET
values
Values of dictionary.
Syntax
- v = values(d)
- v = values(d, 'cell')
Input argument
- d - scalar: dictionary object.
Output argument
- v - values.
Description
v = values(d) retrieves an array containing the values of the specified dictionary, d.
v = values(d, 'cell') optionally returns the values as a cell array.
Example
names = ["Biil" "John" "Yann"];
wheels = [1 2 3];
d = dictionary(wheels, names)
v = values(d)
v = values(d, 'cell')
See also
History
Version | Description |
---|---|
1.5.0 | initial version |
Author
Allan CORNET
Tables
Tables
Description
Tables of arrays with named columns, each potentially containing different data types.
- Accessing and Manipulating Tables in Nelson
- Direct computation with Table
- Read/Write table to files
- array2table - Convert homogeneous array to table.
- cell2table - Convert cell array to table.
- head - Get top rows of table or array.
- height - Number of table rows
- istable - Determine if input is table.
- removevars - Delete variables from table.
- renamevars - Rename variables in table.
- struct2table - Convert a structure array into a tabular format.
- table - A table-like array with named variables, capable of holding different data types
- table2array - Convert table to homogeneous array.
- table2cell - Convert table to cell array
- table2struct - Convert table to structure array
- tail - Get bottom rows of table or array.
- width - Number of table variables
Accessing and Manipulating Tables in Nelson
Description
Insertion into a Table
To insert new data into a table, use dot notation or curly braces {} for specific element-wise insertion. You can add new rows, columns, or update existing data.
see examples: Adding a New Column and Updating an Existing Element
Extraction from a Table
You can extract specific rows, columns, or individual elements using indexing or by referencing variable names.
see examples: Extracting Specific Columns and Extracting Specific Rows
Removing Data from a Table
In Nelson, you can remove rows, columns, or specific elements from a table by using indexing or the removevars function. Rows or columns can be removed by setting the indices to empty brackets [].
see examples: Removing Rows and Removing Columns
Horizontal Concatenation (horzcat)
You can concatenate tables horizontally (side by side) using the horzcat function. This function combines tables by appending the columns of one table to the columns of another table.
see examples: Horizontal Concatenation
Vertical Concatenation (vertcat)
You can concatenate tables vertically (one below the other) using the vertcat function. This function combines tables by appending the rows of one table to the rows of another table.
see examples: Vertical Concatenation
Convert variable types
You can convert table variables by using the VariableTypes property.
see examples: VariableTypes example
Summary
In Nelson, tables provide a flexible way to store and manipulate heterogeneous data. You can easily insert data, extract parts of the table, and concatenate tables both horizontally and vertically using built-in functionality like dot notation and concatenation functions (horzcat, vertcat), making table manipulation intuitive and powerful for data analysis.
Examples
Adding a New Column
T = table([1; 2], {'A'; 'B'}, 'VariableNames', {'ID', 'Label'})
% Insert a new column 'Score'
T.Score = [10; 20]
Updating an Existing Element
T = table([1; 2], {'A'; 'B'}, 'VariableNames', {'ID', 'Label'})
% Insert a new column 'Score'
T.Score = [10; 20]
% Update the value in row 1, column 'Score'
T{1, 'Score'} = 15
Extracting Specific Columns
T = table([1; 2], {'A'; 'B'}, 'VariableNames', {'ID', 'Label'})
% Insert a new column 'Score'
T.Score = [10; 20]
% Update the value in row 1, column 'Score'
T{1, 'Score'} = 15
% Extract the 'ID' column from the table
ID_column = T.ID
Extracting Specific Rows
T = table([1; 2], {'A'; 'B'}, 'VariableNames', {'ID', 'Label'})
% Insert a new column 'Score'
T.Score = [10; 20]
% Update the value in row 1, column 'Score'
T{1, 'Score'} = 15
% Extract the first two rows of the table
rows_1_2 = T(1:2, :)
Removing a Column
T = table([1; 2], {'A'; 'B'}, 'VariableNames', {'ID', 'Label'})
% Insert a new column 'Score'
T.Score = [10; 20]
% Remove the 'Score' column from the table
T(:, 'Score') = [];
Removing a Row
T = table([1; 2], {'A'; 'B'}, 'VariableNames', {'ID', 'Label'})
% Insert a new column 'Score'
T.Score = [10; 20]
% Remove the second row from the table
T(2, :) = [];
Horizontal Concatenation
% Create two tables with the same number of rows
T1 = table([1; 2], {'A'; 'B'}, 'VariableNames', {'ID', 'Label'});
T2 = table([10; 20], {'X'; 'Y'}, 'VariableNames', {'Score', 'Grade'});
% Concatenate horizontally
T_horz = [T1, T2] % or T_horz = horzcat(T1, T2);
Vertical Concatenation
T1 = table([1; 2], {'A'; 'B'}, 'VariableNames', {'ID', 'Label'});
% Create two tables with the same column names
T3 = table([3; 4], {'C'; 'D'}, 'VariableNames', {'ID', 'Label'});
% Concatenate vertically
T_vert = [T1; T3] % or T_vert = vertcat(T1, T3)
Convert variable types
Names = {'John'; 'Alice'; 'Bob'; 'Diana'};
Age = [28; 34; 22; 30];
Height = [175; 160; 180; 165];
Weight = [70; 55; 80; 60];
T = table(Names, Age, Height, Weight);
T.Properties.VariableTypes
T{:,1}
T{:,2}
T.Properties.VariableTypes = ["string" "int8" "double" "double"];
T{:,1}
T{:,2}
T.Properties.VariableTypes
See also
table, Direct computation with Table.
History
Version | Description |
---|---|
1.8.0 | initial version |
1.10.0 | VariableTypes property |
Author
Allan CORNET
Direct computation with Table
Description
You can perform calculations directly on tableswithout needing to index into them.
To perform such operations using the same syntax as you would for arrays, your tables must meet several criteria:
All variables within the table must have data types that support the intended calculations (e.g., numeric or logical types).
When performing an operation where only one operand is a table, the other operand must be either a numeric or logical array.
For operations involving two tables, they must have compatible sizes (i.e., the same number of rows and columns or the operation must make sense for the structures involved).
Below is an example that demonstrates how to perform calculations without explicitly indexing into the table.
Example
Direct computation on Tables
% Create a sample table with sensor data
T = table([1.5; -2.3; 4.7], [0.5; 1.1; -0.7], [-1; 2; 3], ...
'VariableNames', {'Voltage', 'Current', 'Resistance'});
% Apply functions directly to the table columns
abs(T)
acos(T)
acosh(T)
T > 1
T + 2
T .* T
abs(sin(T)) + 1
See also
abs, acos, acosh, acot, acotd, acoth, acsc, acscd, acsch, asec, asecd, asech, asin, asind, asinh, atan, atand, atanh, ceil, cosd, cosh, cospi, cot, cotd, coth, csc, cscd, csch, exp, fix, floor, log, log10, log1p, log2, nextpow2, round, sec, secd, sech, sin, sind, sinh, sinpi, sqrt, tan, tand, tanh, var, acosd, not, plus, minus, times, eq, ge, gt, le, ne, lt, mrdivide, rem, power, pow2, or, mod, ldivide.
History
Version | Description |
---|---|
1.9.0 | initial version |
Author
Allan CORNET
Read/Write table to files
Description
Nelson provides extensive capabilities for reading and writing tables to files, supporting both text-based and binary storage formats to meet different data management needs.
Text files (.csv, .txt, etc.):
- writetable() exports tables to delimited text files with customizable separators
- readtable() imports tables from delimited text files with automatic format detection
- Text files preserve variable names and data in human-readable format
Binary file:
- Nelson HDF5 format (.nh5):
- Efficient binary storage using HDF5 format
- Preserves all table metadata and data types
- Use save -nh5 and load commands
Binary format is recommended for preserving exact numeric precision and working with large datasets.
Examples
Read/Write table to .nh5 file
% Create a sample table with sensor data
T = table([1.5; -2.3; 4.7], [0.5; 1.1; -0.7], [-1; 2; 3], 'VariableNames', {'Voltage', 'Current', 'Resistance'});
R = T;
filename = [tempdir(), 'table_example.nh5'];
save(filename, '-nh5', 'T');
clear T
load(filename, 'T');
assert(isequal(T, R));
T
Read/Write table to text file
% Create a sample table with sensor data
T = table([1.5; -2.3; 4.7], [0.5; 1.1; -0.7], [-1; 2; 3], 'VariableNames', {'Voltage', 'Current', 'Resistance'});
filename = [tempdir(), 'table_example.csv'];
writetable(T, filename);
T2 = readtable(filename);
See also
writetable, readtable, load, save.
History
Version | Description |
---|---|
1.10.0 | initial version |
Author
Allan CORNET
array2table
Convert homogeneous array to table.
Syntax
- T = array2table(A)
Input argument
- A - matrix: single, double, integer types, logical, char, string, struct, cell.
Output argument
- T - Table object.
Description
T = array2table(A) converts an m-by-n array A into an m-by-n table, where each column of A becomes a variable in the resulting table T.
By default, array2table uses the name of the input array, combined with the column number, to create variable names in the table. If these names are not valid identifiers, it assigns default names of the form 'Var1', 'Var2', ... , 'VarN', where N is the number of columns in A.
Example
A = magic(6);
T = array2table(A)
T = array2table(magic(6))
See also
History
Version | Description |
---|---|
1.8.0 | initial version |
Author
Allan CORNET
cell2table
Convert cell array to table.
Syntax
- T = cell2table(C)
Input argument
- C - 2-D cell array.
Output argument
- T - Table object.
Description
T = cell2table(C) converts the contents of an m-by-n cell array C into an m-by-n table.
Each column of the input cell array becomes the data for a corresponding variable in the output table.
To generate variable names in the output table, cell2table appends the column numbers to the name of the input array.
If the input array does not have a name, cell2table assigns default variable names in the format "Var1", "Var2", ... , "VarN", where N is the number of columns in the cell array.
Example
C = {'John', 28, true; 'Alice', 35, false; 'Bob', 42, true};
% Convert the cell array to a table
T = cell2table(C)
See also
History
Version | Description |
---|---|
1.8.0 | initial version |
Author
Allan CORNET
head
Get top rows of table or array.
Syntax
- head(A)
- head(A, k)
- B = head(...)
Input argument
- A - Input array (table or other).
Output argument
- k - a integer value: Number of rows to extract (k = 8 by default).
Description
head(A) displays the first eight rows of an array, or table A in the Command Window without assigning it to a variable.
head(A, k) displays the first k rows of A.
B = head(...) returns the specified rows of A for any of the previous syntaxes, with B having the same data type as A.
Examples
LastName = {'Sanchez';'Johnson';'Li';'Diaz';'Brown'};
Age = [38;43;38;40;49];
Smoker = logical([1;0;1;0;1]);
Height = [71;69;64;67;64];
Weight = [176;163;131;133;119];
BloodPressure = [124 93; 109 77; 125 83; 117 75; 122 80];
T = table(LastName, Age, Smoker, Height, Weight, BloodPressure)
head(T, 2)
A = repmat((1:50)',1, 3);
head(A)
See also
History
Version | Description |
---|---|
1.9.0 | initial version |
Author
Allan CORNET
height
Number of table rows
Syntax
- H = height(T)
Input argument
- T - Input array (table or other).
Output argument
- H - a integer value: Number of table rows in Table or size(T, 1).
Description
H = height(T) returns the number of rows in the table T.
The function height(T) is equivalent to size(T, 1), which also provides the number of rows in the table.
Example
T = table();
height(T)
C = {'John', 28, true; 'Alice', 35, false; 'Bob', 42, true};
T = cell2table(C);
height(T)
See also
History
Version | Description |
---|---|
1.8.0 | initial version |
Author
Allan CORNET
istable
Determine if input is table.
Syntax
- tf = istable(A)
Input argument
- A - Input array.
Output argument
- tf - a logical: true if it is a table.
Description
tf = istable(A) returns true if A is a table, and false if it is not.
Example
T = table();
istable(T)
M = magic(6);
istable(M)
See also
History
Version | Description |
---|---|
1.8.0 | initial version |
Author
Allan CORNET
removevars
Delete variables from table.
Syntax
- TB = removevars(TA, varsNames)
Input argument
- TA - Input table.
- varsNames - Variable names in input table to remove: character vector, string array or cell array of character vectors.
Output argument
- TB - Table object modified.
Description
TB = removevars(TA, varsNames) removes the variables specified by varsNames from the table TA and stores the remaining variables in T2.
You can specify the variables by name, position, or using logical indices.
You can also remove variables from a table using T(:, varsNames) = [].
Example
C = {'John', 28, true; 'Alice', 35, false; 'Bob', 42, true};
% Convert the cell array to a table
T1 = cell2table(C)
T2 = removevars(T1, 'C2')
See also
History
Version | Description |
---|---|
1.9.0 | initial version |
Author
Allan CORNET
renamevars
Rename variables in table.
Syntax
- TB = renamevars(TA, varsNames, newNames)
Input argument
- TA - Input table.
- varsNames - Variable names in input table: character vector, string array or cell array of character vectors.
- newNames - New names for variables: character vector, string array or cell array of character vectors.
Output argument
- TB - Table object with variable names modified.
Description
TB = renamevars(TA, varsNames, newNames) renames the variables in the table TA as specified by varsNames and assigns them the new names provided in newNames.
You can also rename all the variables in a table by assigning new names to its VariableNames property using T.Properties.VariableNames = newNames.
In this case, newNames must be a string array or a cell array of character vectors.
Example
C = {'John', 28, true; 'Alice', 35, false; 'Bob', 42, true};
% Convert the cell array to a table
T1 = cell2table(C);
T2 = renamevars(T1, {'C1', 'C2'}, {'Name', 'Age'})
T3 = cell2table(C);
T3.Properties.VariableNames = {'Name', 'Age', 'Married'};
T3
See also
History
Version | Description |
---|---|
1.9.0 | initial version |
Author
Allan CORNET
struct2table
Convert a structure array into a tabular format.
Syntax
- T = struct2table(S)
Input argument
- S - structure: Array provided as a structure.
Output argument
- T - A table object.
Description
T = struct2table(S) transforms a structure array into a table, where each field of the input structure is represented as a variable in the resulting table.
If the input is a scalar structure containing 𝑛 fields, each with 𝑚 rows, the output will be an 𝑚×𝑛 table.
If the input is either an 𝑚×1 or a 1×𝑚 structure array with 𝑛 fields, the output will also be an 𝑚×𝑛 table.
Examples
% Define a structure array
S(1).Name = 'Alice';
S(1).Age = 30;
S(1).Height = 5.5;
S(2).Name = 'Bob';
S(2).Age = 25;
S(2).Height = 6.0;
% Convert the structure array to a table
T = struct2table(S)
S = struct();
S(1).a = [10 20];
S(2).a = [30 40];
S(1).b = 50;
S(2).b = 60;
T = struct2table(S)
S = struct();
S.a = [1;2;3]
S.b = [4 5;6 7;8 9]
T = struct2table(S)
S = struct();
S(1).a = [10 20];
S(2).a = [30 40 50];
S(1).b = 70;
S(2).b = 80;
T = struct2table(S)
See also
History
Version | Description |
---|---|
1.8.0 | initial version |
Author
Allan CORNET
table
A table-like array with named variables, capable of holding different data types
Syntax
- T = table()
- T = table(var1, ... , varN)
- T = table(... , Name, Value)
Input argument
- var1, ... , varN - Input variables: Input variables are specified as arrays that all have the same number of rows. These variables can differ in size and data type.
- Name, Value - Optional arguments are specified as pairs in the format Name1, Value1, ... , NameN, ValueN, where Name represents the argument name and Value is its corresponding value. These name-value pairs must come after any other arguments, but the order of the pairs themselves is flexible
Output argument
- T - A table object.
Description
Table arrays are designed to store column-oriented, such as columns from text files or spreadsheets.
Each column of data is stored in a variable within the table, and these variables can have different data types and sizes, provided they all share the same number of rows.
Table variables have names, similar to structure fields.
To access data in a table, use the following methods:
- Dot notation (T.varname) to extract a single variable.
- Curly braces (T{rows, vars}) to extract an array from specific rows and variables.
- Parentheses (T(rows, vars)) to return a subset of the table.
T = table(var1, ..., varN) creates a table from the specified input variables var1,...,varN.
The variables can vary in size and data type, but they must all have the same number of rows.
If the inputs are workspace variables, their names are used as the variable names in the resulting table.
Otherwise, the table assigns default names in the format 'Var1', 'Var2', and so on, where N is the total number of variables.
T = table(..., Name, Value) allows you to specify additional options using one or more name-value pair arguments.
For instance, you can set custom variable names by using the 'VariableNames' name-value pair.
This syntax can be used in combination with any of the input arguments from the previous forms.
T = table() creates an empty table with 0 rows and 0 columns.
Examples
Names = {'John'; 'Alice'; 'Bob'; 'Diana'};
Age = [28; 34; 22; 30];
Height = [175; 160; 180; 165];
Weight = [70; 55; 80; 60];
T = table(Names, Age, Height, Weight)
T.Names
T{2, 2}
T{'Alice', 'Age'}
T{2, 'Age'}
T(:, 'Age')
T(2:3,1:3)
N = {'John'; 'Alice'; 'Bob'; 'Diana'};
A = [28; 34; 22; 30];
H = [175; 160; 180; 165];
W = [70; 55; 80; 60];
T = table(N, A, H, W, 'VariableNames', {'Name', 'Age', 'Height', 'Weight'})
N = {'John'; 'Alice'; 'Bob'; 'Diana'};
A = [28; 34; 22; 30];
H = [175; 160; 180; 165];
W = [70; 55; 80; 60];
% Define the row names
RowNames = {'Person1', 'Person2', 'Person3', 'Person4'};
% Create the table with row names
T = table(A, H, W, 'RowNames', RowNames, 'VariableNames', {'Age', 'Height_cm', 'Weight_kg'})
T('Person2', 1:2)
See also
Accessing and Manipulating Tables in Nelson, Direct computation with Table, cell2table, array2table, struct2table.
History
Version | Description |
---|---|
1.8.0 | initial version |
Author
Allan CORNET
table2array
Convert table to homogeneous array.
Syntax
- A = table2array(T)
Input argument
- T - table object.
Output argument
- A - matrix: single, double, integer types, logical, char, string, struct, cell.
Description
A = table2array(T) converts the input table T into a homogeneous array A, where the variables in T become the columns of A.
The output A does not retain the table properties from T.Properties.
If T is a table with row names, these row names will not be included in A.
Example
A = magic(6);
T = array2table(A);
A = table2array(T)
See also
History
Version | Description |
---|---|
1.8.0 | initial version |
Author
Allan CORNET
table2cell
Convert table to cell array
Syntax
- S = table2cell(T)
- S = table2cell(T, "ToScalar", true)
Input argument
- T - a table object
Output argument
- C - Cell array.
Description
C = table2cell(T) converts the table T into a cell array C, where each variable in T is transformed into a column of cells in C.
The output C does not include any properties from T.Properties.
If T contains row names, these will not be included in C.
Example
S = ["Y";"Y";"N";"N";"N"];
A = [38;43;38;40;49];
B = [124 93;109 77; 125 83; 117 75; 122 80];
T = table(S, A, B, 'VariableNames',["Smoker" "Age" "BloodPressure"], 'RowNames',["Chang" "Brown" "Ruiz" "Lee" "Garcia"])
C = table2cell(T)
See also
History
Version | Description |
---|---|
1.8.0 | initial version |
Author
Allan CORNET
table2struct
Convert table to structure array
Syntax
- S = table2struct(T)
- S = table2struct(T, "ToScalar", true)
Input argument
- T - a table object
Output argument
- S - Structure.
Description
S = table2struct(T) converts the table T into a structure array S, where each variable in T is represented as a field in S.
If T is an m-by-n table, S will be an m-by-1 structure array with n fields.
the output S will not contain any table properties from T.Properties.
S = table2struct(T, "ToScalar", true) converts the table T into a scalar structure S, where each variable in T becomes a field in S.
If T is an m-by-n table, S will contain n fields, and each field will have m rows.
Example
Names = {'John'; 'Alice'; 'Bob'; 'Diana'};
Age = [28; 34; 22; 30];
Height = [175; 160; 180; 165];
Weight = [70; 55; 80; 60];
T = table(Names, Age, Height, Weight)
S1 = table2struct(T)
S1 = table2struct(T, "ToScalar", true)
See also
History
Version | Description |
---|---|
1.8.0 | initial version |
Author
Allan CORNET
tail
Get bottom rows of table or array.
Syntax
- tail(A)
- tail(A, k)
- B = tail(...)
Input argument
- A - Input array (table or other).
Output argument
- k - a integer value: Number of rows to extract (k = 8 by default).
Description
tail(A) displays the last eight rows of an array, or table A in the Command Window without assigning it to a variable.
tail(A, k) displays the last k rows of A.
B = tail(...) returns the specified rows of A for any of the previous syntaxes, with B having the same data type as A.
Examples
LastName = {'Sanchez';'Johnson';'Li';'Diaz';'Brown'};
Age = [38;43;38;40;49];
Smoker = logical([1;0;1;0;1]);
Height = [71;69;64;67;64];
Weight = [176;163;131;133;119];
BloodPressure = [124 93; 109 77; 125 83; 117 75; 122 80];
T = table(LastName, Age, Smoker, Height, Weight, BloodPressure)
tail(T, 2)
A = repmat((1:50)',1, 3);
tail(A)
See also
History
Version | Description |
---|---|
1.9.0 | initial version |
Author
Allan CORNET
width
Number of table variables
Syntax
- W = width(T)
Input argument
- T - Input array (table or other).
Output argument
- W - a integer value: Number of Variables in Table or size(T, 2).
Description
W = width(T) returns the number of variables in the table T.
The function width(T) is equivalent to size(T, 2), which also provides the number of columns in the table.
Example
T = table();
width(T)
C = {'John', 28, true; 'Alice', 35, false; 'Bob', 42, true};
T = cell2table(C);
width(T)
See also
History
Version | Description |
---|---|
1.8.0 | initial version |
Author
Allan CORNET
Integers type
Integers type
Description
These functions convert and store data to 1, 2 or 4 bytes integers. These data types are especially useful to store big objects such as images.
- int16 - Converts to 16-bit signed integer.
- int32 - Converts to 32-bit signed integer.
- int64 - Converts to 64-bit signed integer.
- int8 - Converts to 8-bit signed integer.
- intmax - Return the largest integer that can be represented in an integer type.
- intmin - Return the smallest integer that can be represented in an integer type.
- uint16 - Converts to 16-bit unsigned integer.
- uint32 - Converts to 32-bit unsigned integer.
- uint64 - Converts to 64-bit unsigned integer.
- uint8 - Converts to 8-bit unsigned integer.
int16
Converts to 16-bit signed integer.
Syntax
- Y = int16(X)
Input argument
- X - a matrix of double, single or integers.
Output argument
- Y - a matrix of 16-bit integer.
Description
int16 converts value to 16-bit integer type.
The value is rounded to the nearest int16 value on conversion. A value that is above or below the range for an int16 class is mapped to one of the endpoints of the range [-32768, 32767].
Example
A = [1 -32769 -120 127 32767 32768]
B = int16(A)
See also
intmax, intmin, numeric types.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
int32
Converts to 32-bit signed integer.
Syntax
- Y = int32(X)
Input argument
- X - a matrix of double, single or integers.
Output argument
- Y - a matrix of 32-bit integer.
Description
int32 converts value to 32-bit integer type.
The value is rounded to the nearest int32 value on conversion. A value that is above or below the range for an int32 class is mapped to one of the endpoints of the range [-2147483648, 2147483647].
Example
A = [1 -2147483649 -120 127 2147483647 2147483648]
B = int32(A)
See also
intmax, intmin, numeric types.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
int64
Converts to 64-bit signed integer.
Syntax
- Y = int64(X)
Input argument
- X - a matrix of double, single or integers.
Output argument
- Y - a matrix of 64-bit integer.
Description
int64 converts value to 64-bit integer type.
The value is rounded to the nearest int64 value on conversion. A value that is above or below the range for an int64 class is mapped to one of the endpoints of the range [-9223372036854775808,9223372036854775807].
When you create a numeric array of large integers in Nelson, especially when they exceed the maximum precision representable by double (larger than flintmax), Nelson initially stores these values as double-precision floating-point numbers by default.
This default representation can lead to a loss of precision, as floating-point numbers have limited precision.
To maintain the full precision of these large integer values, it's advisable to explicitly convert each scalar element of the array to the int64 or uint64 data type using the i64 or u64 notation (see example).
This way, you ensure that the values are stored with their full precision as 64-bit signed or unsigned integers, respectively, rather than as double-precision floating-point numbers.
Example
A = [1 12 -120 127 -9e24 9e23]
B = int64(A)
R1 = int64([72057594035891654 81997179153022975])
R2 = [72057594035891654i64 81997179153022975i64]
See also
intmax, intmin, numeric types.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
int8
Converts to 8-bit signed integer.
Syntax
- Y = int8(X)
Input argument
- X - a matrix of double, single or integers.
Output argument
- Y - a matrix of 8-bit integer.
Description
int8 converts value to 8-bit integer type.
The value is rounded to the nearest int8 value on conversion. A value that is above or below the range for an int8 class is mapped to one of the endpoints of the range [-128, 127].
Example
A = [1 -255 -120 127 128 215]
B = int8(A)
See also
intmax, intmin, numeric types.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
intmax
Return the largest integer that can be represented in an integer type.
Syntax
- imax = intmax()
- imax = intmax(classname)
Input argument
- classname - a string: by default: int32
Output argument
- imax - largest integer
Description
imax = intmax(classname)the largest integer that can be represented in an integer type.
Supported values for the string classname are:
'int8'
'uint8'
'int16'
'uint16'
'int32'
'uint32'
'int64'
'uint64'
Examples
A = intmax('int64')
res = class(A)
A = intmax('uint32')
res = class(C)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
intmin
Return the smallest integer that can be represented in an integer type.
Syntax
- imin = intmin()
- imin = intmin(classname)
Input argument
- classname - a string: by default: int32
Output argument
- imin - smallest integer
Description
imin = intmin(classname)the smallest integer that can be represented in an integer type.
Supported values for the string classname are:
'int8'
'uint8'
'int16'
'uint16'
'int32'
'uint32'
'int64'
'uint64'
Examples
A = intmin('int64')
res = class(A)
A = intmin('uint32')
res = class(C)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
uint16
Converts to 16-bit unsigned integer.
Syntax
- Y = uint16(X)
Input argument
- X - a matrix of double, single or integers.
Output argument
- Y - a matrix of 16-bit unsigned integer.
Description
uint16 converts value to 16-bit unsigned integer type.
The value is rounded to the nearest uint16 value on conversion. A value that is above or below the range for an uint16 class is mapped to one of the endpoints of the range [0, 65535].
Example
A = [1 -32769 -120 127 32767 32768]
B = uint16(A)
See also
intmax, intmin, numeric types.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
uint32
Converts to 32-bit unsigned integer.
Syntax
- Y = uint32(X)
Input argument
- X - a matrix of double, single or integers.
Output argument
- Y - a matrix of 32-bit unsigned integer.
Description
uint32 converts value to 32-bit unsigned integer type.
The value is rounded to the nearest uint32 value on conversion. A value that is above or below the range for an uint32 class is mapped to one of the endpoints of the range [0, 4294967295].
Example
A = [1 -2147483649 -120 127 2147483647 2147483648]
B = uint32(A)
See also
intmax, intmin, numeric types.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
uint64
Converts to 64-bit unsigned integer.
Syntax
- Y = uint64(X)
Input argument
- X - a matrix of double, single or integers.
Output argument
- Y - a matrix of 64-bit unsigned integer.
Description
uint64 converts value to 64-bit unsigned integer type.
The value is rounded to the nearest uint64 value on conversion. A value that is above or below the range for an uint64 class is mapped to one of the endpoints of the range [0, 18446744073709551616].
When you create a numeric array of large integers in Nelson, especially when they exceed the maximum precision representable by double (larger than flintmax), Nelson initially stores these values as double-precision floating-point numbers by default.
This default representation can lead to a loss of precision, as floating-point numbers have limited precision.
To maintain the full precision of these large integer values, it's advisable to explicitly convert each scalar element of the array to the int64 or uint64 data type using the i64 or u64 notation (see example).
This way, you ensure that the values are stored with their full precision as 64-bit signed or unsigned integers, respectively, rather than as double-precision floating-point numbers.
Example
A = [1 12 -120 127 -9e24 9e23]
B = uint64(A)
R1 = uint64([72057594035891654 81997179153022975])
R2 = [72057594035891654u64 81997179153022975u64]
See also
intmax, intmin, numeric types.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
uint8
Converts to 8-bit unsigned integer.
Syntax
- Y = uint8(X)
Input argument
- X - a matrix of double, single or integers.
Output argument
- Y - a matrix of 8-bit unsigned integer.
Description
uint8 converts value to 8-bit unsigned integer type.
The value is rounded to the nearest uint8 value on conversion. A value that is above or below the range for an uint8 class is mapped to one of the endpoints of the range [0, 255].
Example
A = [1 256 -120 127 -1 215]
B = uint8(A)
See also
intmax, intmin, numeric types.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
Sparse type
Sparse type
Description
sparse type functions
- IJV - Returns I,J,V triplets from a sparse matrix.
- full - Sparse to full matrix conversion.
- nnz - Return the number of nonzero elements.
- nzmax - Reserved size for nonzero elements.
- sparse - Sparse matrix definition.
- speye - Sparse identity matrix.
- spones - Replaces non zero sparse matrix elements with ones.
IJV
Returns I,J,V triplets from a sparse matrix.
Syntax
- [iv, jv, vv, m, n, nzmax] = IJV(sp)
Input argument
- sp - a sparse matrix.
Output argument
- iv - a vector: row indices of the nonzero elements.
- jv - a vector: column indices of the nonzero elements.
- vv - a vector: values of the nonzero elements.
- m - an integer value: number of rows in the matrix.
- n - an integer value: number of columns in the matrix.
- nzmax - an integer value: reserved size for nonzero elements..
Description
IJV converts a sparse matrix into its COO format.
Example
sp = sparse(eye(3,3))
[IV, JV, VV, m, n, nzmax] = IJV(sp)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
full
Sparse to full matrix conversion.
Syntax
- M = full(sp)
Input argument
- sp - a matrix: double or logical, sparse.
Output argument
- M - a matrix.
Description
full converts a sparse matrix into its full representation.
If input argument is already full then output argument will be equal to input argument.
Example
sp = sparse(eye(3,3))
F = full(sp)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
nnz
Return the number of nonzero elements.
Syntax
- v = nnz(M)
Input argument
- M - a matrix: double or logical, sparse or not.
Output argument
- v - a integer value.
Description
nnz returns the number of non zero elements in an matrix.
Example
I = [1 2 3];
J = [3 1 2];
V = [32 42 53];
sp = sparse(I, J, V, 5, 4, 10)
size(sp)
nnz(sp)
nzmax(sp)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
nzmax
Reserved size for nonzero elements.
Syntax
- v = nzmax(M)
Input argument
- M - a matrix: double or logical, sparse or not.
Output argument
- v - a integer value.
Description
nzmax returns the amount of storage allocated for nonzero elements.
Example
I = [1 2 3];
J = [3 1 2];
V = [32 42 53];
sp = sparse(I, J, V, 5, 4, 10)
size(sp)
nnz(sp)
nzmax(sp)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
sparse
Sparse matrix definition.
Syntax
- sp = sparse(M)
- sp = sparse(m, n)
- sp = sparse(I, J, V)
- sp = sparse(I, J, V, m, n)
- sp = sparse(I, J, V, m, n, nz)
Input argument
- M - a matrix: double or logical.
- m - an integer value: rows dimension.
- n - an integer value: columns dimension
- I - a vector.
- J - a vector.
- V - a vector.
- nz - an integer value: storage allocation for nonzero elements.
Output argument
- S - a single.
Description
sparse is used to build a sparse matrix. Only non-zero entries are stored.
If Mis a full matrix, sparse converts it to a sparse matrix representation, removing all zero values.
If nz is not specified, sparse uses as default value: nz = max([numel(i), numel(j), numel(v)])
If multiple values are specified with the same i, j indices, the associated value will be the sum of the values at the repeated index.
Examples
sp = sparse(eye(3,3))
sp = sparse(3, 3)
I = [1 2 3];
J = [3 1 2];
V = [32 42 53];
sp = sparse(I, J, V)
size(sp)
I = [1 2 3];
J = [3 1 2];
V = [32 42 53];
sp = sparse(I, J, V, 5, 4)
size(sp)
nnz(sp)
nzmax(sp)
I = [1 2 3];
J = [3 1 2];
V = [32 42 53];
sp = sparse(I, J, V, 5, 4, 10)
size(sp)
nnz(sp)
nzmax(sp)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
speye
Sparse identity matrix.
Syntax
- S = speye()
- S = speye(n)
- S = speye(n, m)
- S = speye(sz)
Input argument
- n, m - dimension sizes: nonnegative integer scalar.
- sz - dimension sizes: two-element row vector.
Output argument
- S - a sparse matrix.
Description
S = speye() returns a sparse scalar 1.
S = speye(n) returns a sparse n-by-n identity matrix, with ones on the main diagonal.
S = speye(n, m) returns a sparse n-by-m matrix, with ones on the main diagonal.
S = speye(sz) returns a matrix with ones on the main diagonal.
Example
tic();S = speye(5000, 5000);toc()
tic();S = sparse(eye(5000, 5000));toc()
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
spones
Replaces non zero sparse matrix elements with ones.
Syntax
- s = spones(S)
Input argument
- S - Sparse or 2D matrix.
Output argument
- S - a sparse matrix.
Description
s = spones(S) returns a matrix s with the same sparsity structure as S, but with one's in the nonzero positions.
Example
S = sparse([1,0;3,4]);
R = spones(S)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
String type
String type
Description
string type functions
- append - combines strings horizontally.
- blanks - creates an string of blank characters.
- char - Converts to a character array.
- contains - checks if string contains with pattern.
- convertCharsToStrings - Convert chars arrays to string arrays.
- convertStringsToChars - Convert string arrays to character arrays.
- count - Computes the number of occurrences of an pattern.
- deblank - Remove trailing whitespace.
- endsWith - checks if string ends with pattern.
- int2str - Convert an integer array to a string
- isStringScalar - checks if input is string array with one element.
- isletter - Determine which characters are letters.
- isspace - Determine which characters are space.
- join - Combine strings.
- mat2str - Matrix to String.
- matches - Determine if pattern matches with strings.
- newline - Returns a newline character.
- num2str - Converts numbers to character array.
- replace - Replaces strings in another.
- sprintf - Writes data to a string.
- startsWith - checks if string starts with pattern.
- str2double - Converts a string to double.
- strcat - concatenate strings horizontally.
- strcmp - Strings comparaison.
- strcmpi - Strings comparaison (case insensitive).
- strfind - Find a string in another.
- string - string array constructor.
- strings - Create string array without characters.
- strjust - Justify strings
- strlength - Length of strings in cell of strings or string array.
- strncmp - Compares first n characters of strings.
- strncmpi - Compares first n characters of strings (case sensitive).
- strrep - Replaces strings in another.
- strtrim - Remove leading and trailing whitespace.
- tolower - Lower case conversion.
- toupper - Upper case conversion.
append
combines strings horizontally.
Syntax
- res = append(s1, s2, ..., sN)
Input argument
- s1, s2, ..., sN - a string, string array or cell of strings.
Output argument
- res - a string, string array or cell of strings.
Description
strcat combines strings horizontally.
If all inputs are character arrays, then res is a character array.
If any input is a string array, then the res is a string array.
If any input is a cell array, and none are string arrays, then res is a cell array of character vectors.
append does not remove trailing white space.
Example
append("Nelson", 'nelSon')
A = {'abcde','fghi'};
B = {'jkl','mn'};
C = append(A, B)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
blanks
creates an string of blank characters.
Syntax
- r = blanks(n)
Input argument
- n - an value integer, number of blanks.
Output argument
- r - a character array with n blanks
Description
blanks creates an string of blank characters.
Example
blanks(4)
See also
char.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
char
Converts to a character array.
Syntax
- res = char(var)
- res = char(var1, var2)
- res = char(var1, var2, ..., varN)
Input argument
- var - a cell of strings, string array or an numeric array.
- var1, var2, ..., varN - strings or an numeric arrays.
Output argument
- res - a string
Description
char converts numerical input into character data by taking the corresponding unicode character for each element.
Examples
M = [ 104 101 108 108 111;
20320 22909 32 32 32];
char(M)
R = char('these', 'are', 'test', 'strings')
R = char(["these"; "are"; "test"; "strings"])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
contains
checks if string contains with pattern.
Syntax
- tf = contains(str, pattern)
- tf = contains(str, pattern,'IgnoreCase', true)
- tf = contains(str, pattern,'IgnoreCase', false)
Input argument
- str - a string, string array or cell of strings.
- pattern - a string to find.
Output argument
- tf - a matrix of logical.
Description
contains returns true if str contains pattern.
Example
str = 'To make a mountain out of a molehill';
k = contains (str, 'hill')
k = contains (str, 'molehill')
k = contains (str, 'Hill', 'IgnoreCase', true)
A = {'Nel', 'son'; 'Nelson', 'Modules'}
k = contains(A, 'son')
A = ["Nel", "son"; "Nelson", "Modules"]
k = contains(A, 'son')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
convertCharsToStrings
Convert chars arrays to string arrays.
Syntax
- S = convertCharsToStrings(C)
- [B1, B2, ..., BN] = convertCharsToStrings(A1, A2, ..., AN)
Input argument
- C - if C is a char array, output S will be converted to an string array.
- A1, A2, ..., AN - variables to convert to string array if it is a char array.
Output argument
- S - a string array or unaltered variable
- B1, B2, ..., BN - variables converted to string array if it is a char array or cell of char array.
Description
convertCharsToStrings converts chars arrays to string arrays.
Example
[A, B, C, D] = convertCharsToStrings("one", 2, 'three', {'four' ; 'NaN' ;'five'})
R = convertCharsToStrings(['Nelson' ; ' is '; ' good'])
See also
convertStringsToChars, cellstr, string, char.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
convertStringsToChars
Convert string arrays to character arrays.
Syntax
- C = convertStringsToChars(S)
- [B1, B2, ..., BN] = convertStringsToChars(A1, A2, ..., AN)
Input argument
- S - if S is a string array, output C will be converted to an cell of strings or an character vector (if S is scalar).
- A1, A2, ..., AN - variables to convert to char array if it is a string array.
Output argument
- C - a char array or unaltered variable
- B1, B2, ..., BN - variables converted to char array if it is a string array.
Description
convertStringsToChars converts string arrays to character arrays.
Example
A = convertStringsToChars("Nelson")
A = convertStringsToChars(["Nelson", string(NaN)])
See also
convertCharsToStringss, cellstr, string, char.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
count
Computes the number of occurrences of an pattern.
Syntax
- nbocc = count(str, pattern)
- nbocc = count(str, pattern,'IgnoreCase', true)
- nbocc = count(str, pattern,'IgnoreCase', false)
Input argument
- str - a string, string array or cell of strings.
- pattern - a string or string array or cell of strings to find.
Output argument
- nbocc - a matrix of integer values.
Description
count computes the number of occurrences of an pattern.
Example
str = 'To make a mountain out of a molehill';
k = count(str, 'hill')
k = count(str, 'molehill')
k = count(str, 'Hill', 'IgnoreCase', true)
A = {'Nel', 'son'; 'Nelson', 'Modules'}
k = count(A, 'son')
A = ["Nel", "son"; "Nelson", "Modules"]
k = count(A, 'son')
See also
startsWith, endsWith, contains.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
deblank
Remove trailing whitespace.
Syntax
- res = deblank(str)
Input argument
- str - a string, a cell of strings or a string array.
Output argument
- res - a string without trailing whitespace.
Description
deblank removes trailing whitespace.
deblank does not remove all significant whitespace (only characters ' \t\n\r\f\v' removed).
Examples
deblank(' Nel Son ')
deblank(" Nel Son ")
deblank([' Nel Son ', char(160)])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
endsWith
checks if string ends with pattern.
Syntax
- tf = endsWith(str, pattern)
- tf = endsWith(str, pattern,'IgnoreCase', true)
- tf = endsWith(str, pattern,'IgnoreCase', false)
Input argument
- str - a string, string array or cell of strings.
- pattern - a string to find.
Output argument
- tf - a matrix of logical.
Description
endsWith returns true if str ends with pattern.
Example
str = 'To make a mountain out of a molehill';
k = endsWith (str, 'hill')
k = endsWith (str, 'molehill')
k = endsWith (str, 'Hill', 'IgnoreCase', true)
A = {'Nel', 'son'; 'Nelson', 'Modules'}
k = endsWith(A, 'son')
A = ["Nel", "son"; "Nelson", "Modules"]
k = endsWith(A, "son")
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
int2str
Convert an integer array to a string
Syntax
- res = int2str(var)
Input argument
- var - an numeric array.
Output argument
- res - a string
Description
int2str converts an numeric array to a string with integer format. Inputs are rounded before conversion.
Examples
R = int2str ([-Inf, 2, NaN; 4, Inf, 6])
R = int2str(uint64(intmax('uint64')))
See also
char.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
isStringScalar
checks if input is string array with one element.
Syntax
- r = isStringScalar(str)
Input argument
- str - a string, string array or cell of strings.
Output argument
- r - a logical, true if res is string type and scalar.
Description
isStringScalar checks if input is string array with one element.
Example
r = isStringScalar('hello')
r = isStringScalar("hello")
r = isStringScalar(["hello", "world"])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
isletter
Determine which characters are letters.
Syntax
- res = isletter(str)
Input argument
- str - scalar, vector, matrix or multidimensional array.
Output argument
- res - logical array
Description
isletter determines which characters are letters.
Examples
isletter('Nel Son')
isletter("六書 six writings")
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
isspace
Determine which characters are space.
Syntax
- res = isspace(str)
Input argument
- str - scalar, vector, matrix or multidimensional array.
Output argument
- res - logical array
Description
isletter determines which characters are space characters.
Examples
isspace('Nel Son')
isspace("六書 six writings")
See also
History
Version | Description |
---|---|
1.3.0 | initial version |
Author
Allan CORNET
join
Combine strings.
Syntax
- res = join(str)
- res = join(str, delimiter)
- res = join(str, dim)
- res = join(str, delimiter, dim)
Input argument
- str - a string, string array or cell of strings.
- delimiter - a string, string array or cell of strings:Characters used to separate and join strings.
- dim - positive integer: Dimension along which to join strings.
Output argument
- res - a string, string array or cell of strings.
Description
res = join(str) combines the elements of str into a single text by joining them with a space character as the default delimiter.
The input, str, can be either a string array or a cell array of character vectors. The output, res, has the same data type as str.
If str is a 1-by-N or N-by-1 string array or cell array, res will be a string scalar or a cell array containing a single character vector.
If str is an M-by-N string array or cell array, res will be an M-by-1 string array or cell array.
For arrays of any size, join concatenates elements along the last dimension with a size greater than 1.
res = join(str, delimiter) joins the elements of str using the specified delimiter instead of the default space character.
If delimiter is an array of multiple delimiters, and str has N elements along the joining dimension, delimiter must have N–1 elements along the same dimension. All other dimensions of delimiter must either have size 1 or match the size of the corresponding dimensions of str.
res = join(str, dim) combines the elements of str along the specified dimension dim.
res = join(str, delimiter, dim) joins the elements of str along the specified dimension dim, using delimiter to separate them.
Example
str = ["x","y","z"; "a","b","c"];
delimiters = [" + "," = "; " - "," = "];
R = join(str, delimiters)
See also
History
Version | Description |
---|---|
1.10.0 | initial version |
Author
Allan CORNET
mat2str
Matrix to String.
Syntax
- res = mat2str(M)
- res = mat2str(M, 'class')
- res = mat2str(M, P, 'class')
Input argument
- M - a numerical 2D matrix or logical.
- P - an integer value: precision, 15 by default.
Output argument
- res - a string
Description
mat2str converts a matrix to a string.
This string may be used to reconstruct the original matrix with execstr function.
Example
R = mat2str(pi)
R = mat2str(pi, 'class')
R = mat2str(pi, 4)
R = mat2str(pi + i, 'class')
execstr(['RB = ', R])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
matches
Determine if pattern matches with strings.
Syntax
- res = matches(str, pattern)
- res = matches(str, pattern, 'IgnoreCase', true)
Input argument
- str - a string, string array or cell of strings.
- pattern - a string, string array or cell of strings.
Output argument
- res - a logical: true if the two matches and false otherwise.
Description
matches determines if pattern matches with strings.
Example
matches("Nelson", 'nelSon')
matches("Nelson", 'Nelson')
str = ["yellow", "green", "blue", "brown"];
R = matches(str, ["yellow", "Brown"], 'IgnoreCase', true);
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
newline
Returns a newline character.
Syntax
- ch = newline()
Output argument
- ch - a char: equivalent to char(10)
Description
newline returns a newline character.
Example
double(newline)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
num2str
Converts numbers to character array.
Syntax
- S = num2str(A)
- S = num2str(A, precision)
- S = num2str(A, formatSpec)
Input argument
- A - a numerical matrix or logical.
- precision - an positive integer value: Maximum number of significant digits.
- formatSpec - a character array: Format of output fields.
Output argument
- S - a character array: text representation of input array.
Description
num2str converts numbers to character array.
num2str trims any leading spaces from a character array. For better control over the results, use sprintf.
Example
R = num2str(pi, 4)
R = num2str(magic(3))
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
replace
Replaces strings in another.
Syntax
- res = replace(str, old, new)
Input argument
- str - a string, string array or cell of strings.
- old - a string, string array or cell of strings to find.
- new - a string, string array or cell of strings.
Output argument
- res - a string, string array or cell of strings.
Description
replace replaces strings in another.
replace and strrep replace strings but replace is recommended.
Example
r = replace('This is a string.', 'is', 'is not')
r = replace({'cccc','ccbbcca'},{'cc','bb'},{'cc'})
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
sprintf
Writes data to a string.
Syntax
- sprintf(format, v1, ... , vn)
Input argument
- format - a string describing the format to used_function.
- v1, ... , vn - data to convert and print according to the previous format parameter.
Description
Write data in text form to a string.
The format follows C fprintf syntax.
Value type | format | comment |
---|---|---|
Integer | %i | base 10 |
Integer signed | %d | base 10 |
Integer unsigned | %u | base 10 |
Integer | %o | Octal (base 8) |
Integer | %x | Hexadecimal (lowercase) |
Integer | %X | Hexadecimal (uppercase) |
Floating-point number | %f | Fixed-point notation |
Floating-point number | %e | Exponential notation (lowercase) |
Floating-point number | %E | Exponential notation (uppercase) |
Floating-point number | %g | Exponential notation (compact format, lowercase) |
Floating-point number | %G | Exponential notation (compact format, uppercase) |
Character | %c | Single character |
String | %s | Character vector. |
To display a percent sign, you need to use a double percent sign (%%) in the format string.
Examples
sprintf('an example of %s.', 'text')
sprintf("an example of %s.", "text")
sprintf('an value %g.', pi)
Display a percent sign
sprintf('%d%%.', 95)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
startsWith
checks if string starts with pattern.
Syntax
- tf = startsWith(str, pattern)
- tf = startsWith(str, pattern,'IgnoreCase', true)
- tf = startsWith(str, pattern,'IgnoreCase', false)
Input argument
- str - a string, string array or cell of strings.
- pattern - a string to find.
Output argument
- tf - a matrix of logical.
Description
startsWith returns true if str starts with pattern.
Example
str = 'To make a mountain out of a molehill';
k = startsWith (str, 'in')
k = startsWith (str, 'to')
k = startsWith (str, 'to', 'IgnoreCase', true)
A = {'Nel', 'son'; 'Nelson', 'Modules'}
k = startsWith(A, 'Nel')
A = ["Nel", "son"; "Nelson", "Modules"];
k = startsWith(A, "Nel")
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
str2double
Converts a string to double.
Syntax
- res = str2double(str)
Input argument
- str - a cell of strings, string array or a string.
Output argument
- res - a double
Description
str2double converts any complex number as a whole into a complex numeric field, converting the real and imaginary parts to the specified numeric type.
If str2double cannot convert string to a number, then it returns a Not An Number value.
Example
R = str2double('2.6 + 3j')
R = str2double('+NaNi')
R = str2double({'2.71' '3.1415'})
R = str2double(["2.71" "3.1415"])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
strcat
concatenate strings horizontally.
Syntax
- res = strcat(s1, s2, ..., sN)
Input argument
- s1, s2, ..., sN - a string, string array or cell of strings.
Output argument
- res - a string, string array or cell of strings.
Description
strcat concatenate strings horizontally.
If all inputs are character arrays, then res is a character array.
If any input is a string array, then the res is a string array.
If any input is a cell array, and none are string arrays, then res is a cell array of character vectors.
For cell and string array inputs, strcat does not remove trailing white space.
For character array inputs, strcat removes trailing ASCII white-space characters.
Example
strcat("Nelson", 'nelSon')
A = {'abcde','fghi'};
B = {'jkl','mn'};
C = strcat(A, B)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
strcmp
Strings comparaison.
Syntax
- res = strcmp(s1, s2)
Input argument
- s1 - a string, string array or cell of strings.
- s2 - a string, string array or cell of strings.
Output argument
- res - a logical: true if the two are identical and false otherwise.
Description
strcmp compares two strings.
Example
strcmp('Nelson', 'nelSon')
strcmp('Nelson', 'Nelson')
A = {'Nel', 'son'; 'Toolboxes', 'Modules'}
B = {'Handle', 'Struct'; 'Toolboxes', 'Modules'}
C = {'C', 'Contents'; 'Nel', 'son'}
strcmp(A, B)
strcmp(A, C)
strcmp(C, 'C')
See also
char.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
strcmpi
Strings comparaison (case insensitive).
Syntax
- res = strcmpi(s1, s2)
Input argument
- s1 - a string, string array or cell of strings.
- s2 - a string, string array or cell of strings.
Output argument
- res - a logical: true if the two are identical (case insensitive) and false otherwise.
Description
strcmpi compares two strings (case insensitive).
Example
strcmpi('Nelson', 'nelSon')
strcmpi('Nelson', 'Nelson')
A = {'Nel', 'son'; 'Toolboxes', 'Modules'}
B = {'Handle', 'Struct'; 'Toolboxes', 'Modules'}
C = {'C', 'Contents'; 'Nel', 'son'}
strcmpi(A, B)
strcmpi(A, C)
strcmpi(C, 'C')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
strfind
Find a string in another.
Syntax
- occ = strfind(str, pattern)
- occ = strfind(str, pattern,'ForceCellOutput', ouput)
Input argument
- str - a string or cell of strings.
- pattern - a string to find.
- output - a logical.
Output argument
- occ - a cell or matrix of integer values: occurences position.
Description
strfind find a string in another.
Example
str = 'To make a mountain out of a molehill';
k = strfind (str, 'in')
k= strfind(str, ' ')
k = strfind ({'abababada', 'beabebe', 'ab'}, 'aba')
A = {'Nel', 'son'; 'Toolboxes', 'Modules'}
k = strfind(A, 'o')
str = 'No pain no gain.';
k = strfind(str,'in','ForceCellOutput',true)
k = strfind(str,'in','ForceCellOutput',false)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
string
string array constructor.
Syntax
- res = string(var)
Input argument
- var - characters, a cell of characters, or an logical or numeric array.
Output argument
- res - a string array
Description
string converts input into string array.
Examples
R = string({'these', 'are'; 'test', 'strings'})
R2 = ["these", "are"; "test", "strings"];
M = [ 104 101 108 108 111;
20320 22909 32 32 32];
R = string(M)
D = double(R)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
strings
Create string array without characters.
Syntax
- C = strings()
- C = strings(m)
- C = strings(m, n)
- C = strings(m, n, ... , p)
- C = strings(sz)
Input argument
- m, n, ... , p - dimensions of the string array to create.
- sz - a vector of integer values (dimensions of the cell to create).
Output argument
- C - a string array
Description
strings returns a cell array of empty matrices.
Example
A = eye(2, 4);
sz = size(A)
C = strings(sz)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
strjust
Justify strings
Syntax
- J = strjust(str)
- J = strjust(str, side)
Input argument
- str - characters vector, cell of characters or string array.
- side - 'left', 'center', 'right' (default).
Output argument
- J - justified text
Description
J = strjust(str, side) returns the text that is justified on the side specified by side.
Examples
S = ["a"; "ab"; "abc"; "abcd"];
J = strjust (S)
J = strjust (S, 'left')
J = strjust (S, 'center')
J = strjust (S, 'right')
J = strjust(' text', 'center')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
strlength
Length of strings in cell of strings or string array.
Syntax
- len = strlength(ce)
Input argument
- ce - a string, string array or cell of strings.
Output argument
- len - a matrix of integer values: length of strings.
Description
strlength returns length of strings.
Example
str = 'To make a mountain out of a molehill';
k = strlength(str)
A = {'Nel', 'son'; 'Toolboxes', 'Modules'}
k = strlength(A)
B = ["Nel", NaN, "son"; "is", "open", "source"];
k = strlength(B)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
strncmp
Compares first n characters of strings.
Syntax
- res = strncmp(s1, s2, n)
Input argument
- s1 - a string, string array or cell of strings.
- s2 - a string, string array or cell of strings.
- n - an integer value: numbers of characters to compare.
Output argument
- res - a logical: true if the two are identical and false otherwise.
Description
strncmp compares the first n characters of two strings (case sensitive).
Example
strncmp('Nelson', 'nelSon', 3)
strncmp('Nelson', 'Nelson', 3)
A = {'Nel', 'son'; 'Toolboxes', 'Modules'}
B = {'Handle', 'Struct'; 'Toolboxes', 'Modules'}
C = {'C', 'Contents'; 'Nel', 'son'}
strncmp(A, B, 2)
strncmp(A, C, 2)
strncmp(C, 'C', 4)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
strncmpi
Compares first n characters of strings (case sensitive).
Syntax
- res = strncmpi(s1, s2, n)
Input argument
- s1 - a string, string array or cell of strings.
- s2 - a string, string array or cell of strings.
- n - an integer value: numbers of characters to compare.
Output argument
- res - a logical: true if the two are identical and false otherwise.
Description
strncmpi compares the first n characters of two strings (case insensitive).
Example
strncmpi('Nelson', 'nelSon', 3)
strncmpi('Nelson', 'Nelson', 3)
A = {'Nel', 'son'; 'Toolboxes', 'Modules'}
B = {'Handle', 'Struct'; 'Toolboxes', 'Modules'}
C = {'C', 'Contents'; 'Nel', 'son'}
strncmpi(A, B, 2)
strncmpi(A, C, 2)
strncmpi(C, 'C', 4)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
strrep
Replaces strings in another.
Syntax
- res = strrep(str, old, new)
Input argument
- str - a string, string array or cell of strings.
- old - a string, string array or cell of strings to find.
- new - a string, string array or cell of strings.
Output argument
- res - a string, string array or cell of strings.
Description
replace replaces strings in another.
replace and strrep replace strings but replace is recommended.
Example
r = strrep('This is a string.', 'is', 'is not')
r = strrep({'cccc','ccbbcca'},{'cc','bb'},{'cc'})
r = strrep("This is a string.", "is", 'is not')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
strtrim
Remove leading and trailing whitespace.
Syntax
- res = strtrim(str)
Input argument
- str - a string, a cell of strings or a string array.
Output argument
- res - a string without leading or trailing whitespace.
Description
strtrim removes leading and trailing whitespace.
strtrim does not remove all significant whitespace (only characters ' \t\n\r\f\v' removed).
Examples
strtrim(' Nel Son')
strtrim(" Nel Son")
strtrim([' Nel Son', char(160)])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
tolower
Lower case conversion.
Syntax
- res = tolower(str)
Input argument
- str - a row character array, a cell of strings or an string array.
Output argument
- res - lower case equivalent
Description
tolower converts a string to lower case.
Examples
tolower('NelSon')
tolower(["NelSon", "is", "open"])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
toupper
Upper case conversion.
Syntax
- res = toupper(str)
Input argument
- str - a row character array, a cell of strings or an string array.
Output argument
- res - a string upper case
Description
toupper converts a string to upper case.
Examples
toupper('NelSon')
upper(["NelSon", "is", "open"])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
Function_handle functions
Function_handle functions
Description
function_handle functions
- Anonymous Functions - Anonymous Functions.
- func2str - Return a function handle constructed from a string.
- isfunction_handle - Checks if value is a function handle.
- str2func - Returns a function handle from a string.
Anonymous Functions
Anonymous Functions.
Description
Anonymous functions provide a convenient way to swiftly create straightforward functions without the need to generate separate M-files on every occasion.
These anonymous functions can be built either directly at the command line or within any M-file function or script.
To create an anonymous function from an expression, use the following syntax:
function_handle = @(argument_list) expression
Breaking down this syntax, expression represents the body of the function, which contains the code that performs the primary task of your function.
This part consists of a valid expression. Next, there's argument_list, which is a comma-separated list of input arguments to be passed to the function.
These components are similar to the body and argument list of any regular function.
At the beginning of this syntax statement, you'll notice the @ sign.
This @ sign is the operator that constructs a function handle.
Creating a function handle for an anonymous function allows you to invoke the function and is useful when passing your anonymous function as an argument to another function.
The @ sign is a necessary part of the anonymous function definition.
It's worth noting that function handles not only apply to anonymous functions but also to any function.
The syntax for creating a function handle to a regular function is different and looks like this:
function_handle = @function_name
For example: f = @cos
You have the option to store function handles along with their associated values in a MAT-file.
Later, in a different session, you can retrieve and utilize them using the save and load functions.
for example a = 1;b = 2; f = @(x) a + b + x; save('test.nH5', f);
Only .nh5 files allows to save and load function_handle type as expected.
You can create an anonymous function that takes multiple input arguments, x and y.
Assuming that variables A and B are already defined, you can define the function as follows:
A = 10; B = 100; r = @(x, y) (A*y + B*x);
Examples
A = 10;
f1 = @() sqr(A);
clear A
f1
f1()
f2 = @cos;
f2
f2(0.6)
f3 = @(x)cos(x) + 1;
f2
f3(0.6)
Multiple input arguments
A = 10;
B = 100;
f4 = @(x, y) (A*y + B*x);
f4
f4(0.6, 0.2)
Save/Load function handle
a = 1;
b = 2;
f5 = @(x) a + b + x;
save([tempdir(), 'test.nh5'], 'f5');
clear all
load([tempdir(), 'test.nh5'])
f5
f5(10)
Multiple output arguments
P = pi * 3;
mymeshgrid = @(X, Y) meshgrid((-X:X/P:X),(-Y:Y/P:Y));
[x, y] = mymeshgrid(pi, 2 * pi);
z = cos(x) + sin(y);
mesh(x, y, z)
See also
func2str, str2func, isfunction_handle.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
func2str
Return a function handle constructed from a string.
Syntax
- func_handle = str2func(str)
Input argument
- str - a string.
Output argument
- func_handle - a function handle
Description
func_handle = str2func(str) returns a function handle constructed from the string str.
Example
fh = str2func('cos')
class(fh)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
isfunction_handle
Checks if value is a function handle.
Syntax
- l = isfunction_handle(func_handle)
Input argument
- func_handle - a function handle or other variable type.
Output argument
- l - a logical
Description
l = isfunction_handle(func_handle) checks if func_handle is a function handle. Returning true if it is.
Example
fh = str2func('cos')
isfunction_handle(fh)
fh = 3
isfunction_handle(fh)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
str2func
Returns a function handle from a string.
Syntax
- func_handle = str2func(str)
Input argument
- str - a string
Output argument
- func_handle - a function handle.
Description
function_handle = str2func(str) returns a function handle function_handle for the function named in the string str
str function name or representation of anonymous function.
Examples
fh = str2func('cos')
str = func2str(fh)
myFind = str2func('@(x, y) find(x > y)')
M = rand(4, 3, 5);
[R, C] = myFind(M, 0.9)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
Handle
Handle
Description
handle object functions
- delete - delete handle objects.
- get - Retrieve a property value from an handle object.
- invoke - Invoke method on an handle object.
- ismethod - Return true if method of handle object.
- isprop - Return true if property of handle object.
- isvalid - Return true if variable h is a valid handle.
- methods - Returns the methods name of an object handle.
- properties - Returns the properties name of an object handle.
- set - Set a property value of an handle object.
delete
delete handle objects.
Syntax
- delete(h)
Input argument
- h - a handle object: scalar or matrix.
Description
delete(h) removes from memory the handle objects referenced by h.
When deleted, any references to the objects in h become invalid.
To remove the handle variables, use the clear function.
See clear function about how to force delete with clear function.
Example
f = figure();
ax = gca();
img = image();
hold on
P = plot(magic(5));
children1 = ax.Children;
delete(img);
size(children1)
children2 = ax.Children;
size(children2)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
get
Retrieve a property value from an handle object.
Syntax
- R = get(h, property_name)
Input argument
- h - an handle object.
- property_name - a string: property name.
Output argument
- R - The data type of the return value depends on the invoked method.
Description
R = get(h, property_name) returns the value of property asked.
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
invoke
Invoke method on an handle object.
Syntax
- R = invoke(h)
- R = invoke(h, 'methodname')
- R = invoke(h, 'methodname', arg1, arg2, ... , argN)
Input argument
- h - an handle object.
Output argument
- R - The data type of the return value depends on the invoked method.
Description
invoke(h) returns a struct with a list of all callable methods.
R = invoke(h, 'methodname') calls the method specified by methodname, and returns an output value.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
ismethod
Return true if method of handle object.
Syntax
- res = ismethod(h, methodname)
Input argument
- h - a handle object
- methodname - a string
Output argument
- res - a logical: true or false
Description
ismethod returns a logical 1 if the argument is a valid handle with method name and a logical 0 otherwise.
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
isprop
Return true if property of handle object.
Syntax
- res = isprop(h, propertyname)
Input argument
- h - a handle object
- propertyname - a string
Output argument
- res - a logical: true or false
Description
isprop returns a logical 1 if the argument is a valid handle with property name and a logical 0 otherwise.
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
isvalid
Return true if variable h is a valid handle.
Syntax
- res = isvalid(h)
Input argument
- h - an handle object
Output argument
- res - a logical: true or false
Description
isvalid returns a logical 1 if the argument is a valid handle and a logical 0 otherwise.
See also
isa.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
methods
Returns the methods name of an object handle.
Syntax
- c = methods(h)
Input argument
- h - a handle object
Output argument
- c - a cell of strings
Description
methods returns a cell of strings with methods name.
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
properties
Returns the properties name of an object handle.
Syntax
- properties(h)
- c = properties(h)
Input argument
- h - a handle object
Output argument
- c - a cell of strings
Description
properties returns a cell of strings with properties name.
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
set
Set a property value of an handle object.
Syntax
- R = set(h, property_name, value)
Input argument
- h - an handle object.
- property_name - a string: property name.
- value - a variable.
Output argument
- R - user-settable properties and possible values for the object identified by h.
Description
This routine can be used to modify the value of a specified property from an handle object.
See also
QObject_set (set), get, invoke.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
Error manager
Error manager
Description
error handling functions
- MException - Matrix Exception information.
- error - Raise an error message.
- getLastReport - Returns last recorded formatted error message.
- lasterror - Returns last recorded error message.
- lastwarn - Returns last recorded warning message.
- rethrow - rethrow error.
- throw - throw error.
- throwAsCaller - Throw exception as if occurs within calling function.
- warning - Display a warning message.
MException
Matrix Exception information.
Syntax
- ME = MException(identifier, message)
- ME = MException('last')
- MException('reset')
Input argument
- identifier - a string: error identifier.
- message - a string.
Output argument
- ME - a MException object.
Description
All Nelson code that detects an error and throws an exception constructs an MException object.
identifier includes one or more component fields and a mnemonic field (example: 'nelson:matrix:empty')
ME = MException('last') return last exception.
MException('reset') clears last exception.
Example
ME = MException('nelson:identifier', 'your error message.')
throw(ME)
See also
error, try, throw, rethrow, throwAsCaller.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
error
Raise an error message.
Syntax
- error(id, msg)
- error(msg)
- error(error_structure)
Input argument
- id - a string: error identifier.
- msg - a string.
- error_structure - error message structure.
Description
error stops the current script execution.
error('') will be ignored and the script will continue to run.
identifier includes one or more component fields and a mnemonic field (example: 'nelson:matrix:empty')
Examples
error('your error message.')
error('nelson:identifier', 'your error message.')
error('')
1 / [1 2 3]
a = lasterror()
lasterror('reset')
b = lasterror()
error(a)
c = lasterror()
See also
MException, lasterror, warning.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
getLastReport
Returns last recorded formatted error message.
Syntax
- messageText = getLastReport()
Output argument
- messageText - a character vector: formatted error message.
Description
getLastReport returns last formatted error message.
Examples
lasterror('reset')
getLastReport()
state = execstr('xxxxxx', 'errcatch')
l = lasterror()
getLastReport
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
lasterror
Returns last recorded error message.
Syntax
- last_err = lasterror()
- lasterror('reset')
- lasterror(error_struct)
Output argument
- last_err - error message structure.
Description
l = lasterror() returns a structure containing the last error message and information as an struct.
lasterror('reset') clears last error.
lasterror(error_struct) set last error.
Examples
state = execstr('xxxxxx', 'errcatch')
if ~state
l = lasterror()
end
state = execstr('xxxxxx', 'errcatch')
l = lasterror();
lasterror('reset');
lasterror()
lasterror(l);
lasterror()
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
lastwarn
Returns last recorded warning message.
Syntax
- last_message = lastwarn()
- [last_message, last_identifier] = lastwarn()
- lastwarn('')
- lastwarn(new_message)
- lastwarn(new_message, new_identifier)
- [last_message, last_identifier] = lastwarn('')
- [last_message, last_identifier] = lastwarn(new_message)
- [last_message, last_identifier] = lastwarn(new_message, new_identifier)
Output argument
- last_message - string: last warning message.
- last_identifier - string: identifier.
Description
last_message = lastwarn() returns a string containing the last warning message.
lastwarn('') clears last warning.
Example
[1:3]:3
lastwarn
[msg, id] = lastwarn()
lastwarn('')
[msg, id] = lastwarn()
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
rethrow
rethrow error.
Syntax
- rethrow(MException)
Input argument
- MException - MException object
Description
rethrow(MException) reissues the error specified by MException.
Example
try
a
catch ME
disp(ME)
rethrow(ME)
end
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
throw
throw error.
Syntax
- throw(MException)
Input argument
- MException - MException object
Description
throw(MException) throws an exception based on the information contained in the MException object, exception.
Example
ME = MException('nelson:errorId', 'my error')
throw(ME)
See also
MException, rethrow, throwAsCaller.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
throwAsCaller
Throw exception as if occurs within calling function.
Syntax
- throwAsCaller(MException)
Input argument
- MException - MException object
Description
It throws an exception as if it occurs within the calling function.
Example
function test_throwAsCaller()
ME = MException('n:m', 'your error')
throwAsCaller(ME)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
warning
Display a warning message.
Syntax
- warning()
- warning(msg)
- warning(id, msg)
- warning(state)
- warning(state, id)
- st = warning()
- warning(st)
Input argument
- id - a string: identifier for the warning.
- msg - a string: message to warn.
- state - a string: 'on', 'off', 'aserror', 'all' or 'query'.
- st - a struct: set warning settings.
Output argument
- st - a struct, warning settings.
Description
warning displays a warning message.
warning('') resets lastwarn state.
Examples
warning('your warning message.')
warning('on', 'myModule:identifier');
warning('myModule:identifier', 'my message 1 on');
warning('off', 'myModule:identifier');
warning('myModule:identifier', 'my message 2 off');
warning('aserror', 'myModule:identifier');
warning('myModule:identifier', 'my message 3 as error');
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
Debugger functions
Debugger functions
Description
debugger functions
- dbstack - call stack.
dbstack
call stack.
Syntax
- dbstack
- st = dbstack()
- dbstack('-completenames')
- st = dbstack('-completenames')
- dbstack('-completenames', omit)
- st = dbstack('-completenames', omit)
Input argument
- omit - an integer value: Number of frames to omit (must be positive).
Output argument
- st - a struct
Description
dbstack displays the file names and line numbers of the function calls.
dbstack('-completenames') displays the full file names.
Example
Creates a myfun.m and calls it.
function myfun(x)
dbstack();
end
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
Profiling tools
Profiling tools
Description
Profile to Improve Performance
- profile - Profile execution time for Macro functions.
- profsave - Save profile result to HTML format.
profile
Profile execution time for Macro functions.
Syntax
- profile on
- profile off
- profile resume
- profile clear
- status = profile('status')
- p = profile('info')
- profile('show', sortOption)
- profile('show', sortOption, nbLines)
Input argument
- sortOption - a string: 'nfl' by name file line, 'line' by line, 'percalls', 'totaltime', 'filename', 'function' or 'nbcalls'.
- nbLines - a integer value: number of lines to display.
Description
Profiling is a way to measure where Macro function spend times.
s = profile('status') returns a structure with the current status of the profiler.
p = profile('info') returns a structure with collected profiling data.
profile('on') starts profiler.
profile('off') stops profiler. Collected profiling data will be retrieved later with p = profile ('info').
profile('clear') clears collected profiling data.
profile('resume') restarts and continue and extends collected profiling data.
Examples
profile on
sind(5)
profile off
profile('show')
profile('show', 'totaltime')
profile('show', 'totaltime', 4)
profile on
sind(5)
profile off
profsave(profile('info'), [tempdir(), 'profile_results'])
unix([tempdir(), 'profile_results/index.html'])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
profsave
Save profile result to HTML format.
Syntax
- profsave
- profsave(profile_info)
- profsave(profile_info, dirname)
Input argument
- profile_info - a struct: result of profile('info')
- dirname - a string: output directory destination.
Description
profsave exports the profiling data into a series of HTML files.
The input profile_info is the structure returned by profile('info').
If unspecified, profsave will use the current profile.
Example
profile on
sind(5)
profile off
profsave(profile('info'), [tempdir(), 'profile_results'])
unix([tempdir(), 'profile_results/index.html'])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
Constructors functions
Constructors functions
Description
Create scalars, vectors, or matrices
- Inf - Infinity
- NaN - Creates an Not-a-Number
- diag - Get diagonal elements of matrix or create diagonal matrix.
- eps - Creates an epsilon (machine precision)
- eye - Creates an identity matrix.
- i - Pure Imaginary number.
- ones - Creates an matrix made of ones.
- pi - Ratio of circle's circumference to its diameter.
- zeros - Creates an matrix made of zeros.
Inf
Infinity
Syntax
- Inf
- inf
- Inf(n)
- Inf(n, m)
Input argument
- n - a variable: n-by-n matrix
- m - a variable: n-by-m matrix
Description
NaN returns the IEEE symbol Inf (Infinity).
Examples
Inf
-Inf + Inf
1.e1000
See also
nan.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
NaN
Creates an Not-a-Number
Syntax
- NaN
- nan
- NaN(n)
- NaN(n, m)
Input argument
- n - a variable: n-by-n matrix
- m - a variable: n-by-m matrix
Description
NaN returns the IEEE symbol NaN (Not a Number).
NaN is the result of operations which do not produce a well defined numerical result.
Beware, you must never compare NaN with NaN, in this case, please use isnan.
Examples
NaN
3 + NaN
NaN != NaN
isnan(NaN)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
diag
Get diagonal elements of matrix or create diagonal matrix.
Syntax
- D = diag(V)
- X = diag(A)
- D = diag(V, k)
- X = diag(A, k)
Input argument
- V - Diagonal elements
- A - Input matrix
Output argument
- D - vector
- X - matrix
Description
diag returns diagonal elements of matrix or create diagonal matrix.
Example
diag(eye(3))
diag(diag(eye(3)))
See also
ones.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
eps
Creates an epsilon (machine precision)
Syntax
- eps
- eps
- eps(n)
- eps(n, m)
- eps('double')
- eps('single')
Input argument
- n - a variable: n-by-n matrix
- m - a variable: n-by-m matrix
Description
eps returns the machine precision 2^(-52) for double and 2^(-23) for single.
eps(Inf), eps(-Inf) and eps(NaN) return NaN.
Examples
eps
eps('double')
eps('single')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
eye
Creates an identity matrix.
Syntax
- R = eye
- R = eye(n)
- R = eye(n, m)
- R = eye(n, m, ..., z)
- R = eye(n, m, ..., z, 'like', V)
- R = eye(n, m, ..., z, classname)
Input argument
- n - a variable: n-by-n matrix
- m - a variable: n-by-m matrix
Description
eye returns an identity matrix.
Examples
eye(3)
eye(3,1,3,'single')
A = single([3 3])
B = eye(2,4,'like', A)
A = eye(0, 4)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
i
Pure Imaginary number.
Syntax
- i
- 0i
- 3*i
Description
i, or j returns a pure imaginary number equivalent to sqrt(-1).
Beware, i and j can be redefined and used as ordinary variables, in this case, you must use clear to restore default behavior.
Examples
A = 3i
A = single(3i)
i = 33;
disp(i);
clear('i');
disp(i);
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
ones
Creates an matrix made of ones.
Syntax
- R = ones
- R = ones(n)
- R = ones(n, m)
- R = ones(n, m, ..., z)
- R = ones(n, m, ..., z, 'like', V)
- R = ones(n, m, ..., z, classname)
Input argument
- n - a variable: n-by-n matrix
- m - a variable: n-by-m matrix
Description
ones returns a matrix made of ones.
Examples
ones(3,2)
ones(3,1,3,'single')
A = single([3 3])
B = ones(2,4,'like', A)
tic(); single(1) * ones(1000); toc()
tic();ones(1000,'single'); toc()
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
pi
Ratio of circle's circumference to its diameter.
Syntax
- pi
Description
pi eturns the floating-point number nearest the value of π .
Examples
cos(pi)
sin(pi)
4*atan(1) == pi
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
zeros
Creates an matrix made of zeros.
Syntax
- R = zeros
- R = zeros(n)
- R = zeros(n, m)
- R = zeros(n, m, ..., z)
- R = zeros(n, m, ..., z, 'like', V)
- R = zeros(n, m, ..., z, classname)
Input argument
- n - a variable
- m - a variable
Description
zeros returns a matrix made of zeros.
Examples
zeros(3, 2)
zeros(3, 1, 3, 'single')
A = single([3 3])
B = zeros(2, 4, 'like', A)
tic(); single(1) * zeros(1000); toc()
tic();zeros(1000, 'single'); toc()
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
Operators
Operators
Description
Operators
- all - all of the elements of a matrix satisfy some condition.
- and - logical 'AND' operator, &
- any - any of the elements of a matrix satisfy some condition.
- bitand - Bit-wise AND
- bitor - Bit-wise OR
- bitxor - Bit-wise XOR
- cat - Concatenate arrays.
- colon - colon operator ':'.
- ctranspose - Returns complex conjugate transpose: ' operator.
- eq - equality, == operator.
- ge - greater than or equal, >= operator.
- gt - greater than, > operator.
- horzcat - Horizontal concatenation.
- ismember - Array elements that are members of another array.
- ldivide - Left division, .\ operator.
- le - less than or equal, ≤ operator.
- lt - less than, < operator.
- minus - Subtraction, - operator
- mldivide - Matrix left division, \ operator.
- mpower - Matrix power, ^ operator
- mrdivide - Matrix right division, / operator.
- mtimes - Matrix multiplication, * operator
- ne - Inequality, ~= operator
- not - not logical, ~ operator
- or - logical 'OR' operator, |
- plus - Addition, + operator
- power - Element wise power, .^ operator
- rdivide - Right division, ./ operator
- shortcutand - Short circuit 'AND' operator, &&
- shortcutor - Short circuit 'OR' operator, ||
- subsasgn - Redefine subscripted assignment.
- subsindex - Convert an object to an index vector.
- subsref - Subscripted reference.
- mtimes - Element wise multiplication, .* operator
- transpose - Returns vector or matrix transpose: .' operator.
- uminus - Unary minus, - operator
- uplus - Unary plus, + operator
- vertcat - Vertical concatenation.
all
all of the elements of a matrix satisfy some condition.
Syntax
- R = all(M)
- R = all(M, dim)
- R = all(M, 'all')
Input argument
- M - a matrix.
- dim - a integer value: dimension along it works.
- 'all' - tests over all elements of M.
Output argument
- R - a logical matrix.
Description
all returns true if all of the elements of a matrix satisfy some condition.
Example
all([33, 22; 11, 0])
all([33, 22; 11, 0], 2)
See also
any.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
and
logical 'AND' operator, &
Syntax
- C = and(A, B)
- C = A & B
Input argument
- A - a variable
- B - a variable
Output argument
- C - result of A & B
Description
C = and(A, B) performs a logical AND operation.
Example
A = [6 8 0; 0 3 89; 15 0 0]
B = [66 56 0; 11 33 55; -11 0 0]
C = A & B
D = and(B, A)
C == D
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
any
any of the elements of a matrix satisfy some condition.
Syntax
- R = any(M)
- R = any(M, dim)
- R = any(M, 'all')
Input argument
- M - a matrix.
- dim - a integer value: dimension along it works.
- 'all' - tests over all elements of M.
Output argument
- R - a logical matrix.
Description
any returns true if any of the elements of a matrix satisfy some condition.
Example
any([33, 22; 11, 0])
any([33, 22; 11, 0], 2)
See also
all.
History
Version | Description |
---|---|
1.0.0 | initial version |
1.6.0 | manages input argument 'all' |
Author
anyan CORNET
bitand
Bit-wise AND
Syntax
- C = bitand(A, B)
- C = bitand(A, B, assumedtype)
Input argument
- A - a variable: double, logical, integer
- B - a variable: double, logical, integer
- assumedtype - 'int64', 'int32', 'int16', 'int8', 'uint64', 'uint32', 'uint16' or 'uint8'.
Output argument
- C - Bit-wise AND result
Description
C = bitand(A, B) returns the bit-wise AND of A and B.
Example
A = uint16([0 1; 0 1]);
B = uint16([0 0; 1 1]);
R = bitand(A, B)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
bitor
Bit-wise OR
Syntax
- C = bitor(A, B)
- C = bitor(A, B, assumedtype)
Input argument
- A - a variable: double, logical, integer
- B - a variable: double, logical, integer
- assumedtype - 'int64', 'int32', 'int16', 'int8', 'uint64', 'uint32', 'uint16' or 'uint8'.
Output argument
- C - Bit-wise OR result
Description
C = bitor(A, B) returns the bit-wise OR of A and B.
Example
A = uint16([0 1; 0 1]);
B = uint16([0 0; 1 1]);
R = bitor(A, B)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
bitxor
Bit-wise XOR
Syntax
- C = bitxor(A, B)
- C = bitxor(A, B, assumedtype)
Input argument
- A - a variable: double, logical, integer
- B - a variable: double, logical, integer
- assumedtype - 'int64', 'int32', 'int16', 'int8', 'uint64', 'uint32', 'uint16' or 'uint8'.
Output argument
- C - Bit-wise XOR result
Description
C = bitxor(A, B) returns the bit-wise XOR of A and B.
Example
A = uint16([0 1; 0 1]);
B = uint16([0 0; 1 1]);
R = bitxor(A, B)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
cat
Concatenate arrays.
Syntax
- R = cat(dim, A, B)
- R = cat(dim, A1, A2, ..., An)
Input argument
- dim - Dimension to operate along: positive integer scalar.
- A - a variable: first input.
- B - a variable: second input.
- A1, A2, ..., An - List of inputs to concatenate
Output argument
- R - concatenated array
Description
R = cat(dim, M1, M2, ... , MN) returns the concatenation of M1, M2, ... , MN along the dimension dim.
Example
A = eye(2, 2);
B = ones(2, 2);
C = cat(2, A, B)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
colon
colon operator ':'.
Syntax
- R = colon(base, limit)
- R = colon(base, increment, limit
Input argument
- base - a variable
- limit - a variable
- increment - a variable (optional)
Output argument
- C - result
Description
colon creates vectors. It is an usefull function for loop, extraction and insertion.
colon(base, limit) is equivalent to base:limit
colon(base, increment, limit) is equivalent to base:increment:limit
Examples
1:0.5:4
A = 1:6
B = 1:4:12
C = rand(3, 4)
C(:)
C(:, 3)
C(2, :)
C(:, 1, 1)
C(:) = rand(3, 4)
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
ctranspose
Returns complex conjugate transpose: ' operator.
Syntax
- C= ctranspose(A)
- C = A'
Input argument
- A - a variable
Output argument
- C - result: complex conjugate transpose of A.
Description
C = ctranspose(A) returns the complex conjugate transpose of A.
Examples
A = 3
B = A'
A = -i
B = A'
A = sparse(eye(3, 4) * i)
B = A'
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
eq
equality, == operator.
Syntax
- C = eq(A, B)
- C = (A == B)
Input argument
- A - a variable
- B - a variable
Output argument
- C - result of A == B
Description
C = eq(A, B) returns a logical array with elements set to logical true where arrays A and B are equals.
eq compares both real and imaginary parts of numeric arrays.
Examples
eye(2,2) == ones(2, 2)
0 == i
'Nelson' == 'Noslen'
"Nelson" == "Noslen"
'Nelson' == 'l'
eq(0.8-0.6-0.2, 0)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
ge
greater than or equal, >= operator.
Syntax
- C = ge(A, B)
- C = (A >= B)
Input argument
- A - a variable
- B - a variable
Output argument
- C - result of A >= B
Description
C = ge(A, B) returns a logical array with elements set to logical true A is greater than or equal to B.
ge compares only the real part of numeric arrays.
Examples
eye(2,2) >= ones(2, 2)
0 >= i
'Nelson' >= 'Noslen'
'Nelson' >= 'l'
ge(0.8-0.6-0.2, 0)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
gt
greater than, > operator.
Syntax
- C = gt(A, B)
- C = (A > B)
Input argument
- A - a variable
- B - a variable
Output argument
- C - result of A > B
Description
C = gt(A, B) returns a logical array with elements set to logical true A is greater than B.
gt compares only the real part of numeric arrays.
Examples
eye(2,2) > ones(2, 2)
0 > i
'Nelson' > 'Noslen'
'Nelson' > 'l'
gt(0.8 - 0.6 - 0.2, 0)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
horzcat
Horizontal concatenation.
Syntax
- R = horzcat(M1, M2, ... , MN)
- R = [M1, M2, ... , MN]
Input argument
- M1 - a variable
- M2 - a variable
- MN - a variable
Output argument
- R - result of [M1, M2, ... , MN]
Description
R = horzcat(M1, M2, ... , MN) returns the horizontal concatenation of M1, M2, ... , MN along the dimension 2.
Examples
A = eye(2, 2);
B = ones(2, 2);
C = horzcat(A, B)
D = [A, B]
A = 'nel';
B = 'son';
C = horzcat(A, B)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
ismember
Array elements that are members of another array.
Syntax
- T = ismember(A, B)
Input argument
- A - a variable
- B - a variable
Output argument
- T - result of ismember.
Description
T = ismember(A, B) returns an array of logical where the data in A is found in B.
Example
A = [50 30 40 20];
B = [20 40 40 40 60 80];
T = ismember(A, B)
T = ismember(["a","b","f"], ["b", "f", "c"])
See also
sort.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
ldivide
Left division, .\ operator.
Syntax
- C = ldivide(A, B)
- C = A .\ B
Input argument
- A - a variable
- B - a variable
Output argument
- C - result of A .\ B
Description
C = ldivide(A, B) returns the element-by-element left division of A and B.
Examples
B = ones(3, 4)
A = B *2
A .\ B
B = 2
A = B *2
A .\ B
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
le
less than or equal, ≤ operator.
Syntax
- C = le(A, B)
Input argument
- A - a variable
- B - a variable
Output argument
- C - result of le(A, B)
Description
C = le(A, B) returns a logical array with elements set to logical true A is less than or equal to B.
le compares only the real part of numeric arrays.
Examples
eye(2,2) <= ones(2, 2)
0 <= i
'Nelson' <= 'Noslen'
'Nelson' <= 'l'
le(0.8 - 0.6 - 0.2, 0)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
lt
less than, < operator.
Syntax
- C = lt(A, B)
Input argument
- A - a variable
- B - a variable
Output argument
- C - result of lt(A, B)
Description
C = lt(A, B) returns a logical array with elements set to logical true A is less than B.
lt compares only the real part of numeric arrays.
Examples
eye(2,2) < ones(2, 2)
0 < i
'Nelson' < 'Noslen'
'Nelson' < 'l'
lt(0.8 - 0.6 - 0.2, 0)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
minus
Subtraction, - operator
Syntax
- C = minus(A, B)
- C = A - B
Input argument
- A - a variable
- B - a variable
Output argument
- C - result of A - B
Description
C = minus(A, B) performs subtraction A - B variables.
Examples
minus(3, 4)
3 - 4
[1, 2] - 1
minus([1, 2], 1)
ones(0, 0) - 1
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
mldivide
Matrix left division, \ operator.
Syntax
- C = mldivide(A, B)
- C = A \ B
Input argument
- A - a variable
- B - a variable
Output argument
- C - result of A \ B
Description
C = mldivide(A, B) returns the matrix left division of A and B.
Example
B = ones(3, 4)
A = B *2
A \ B
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
mpower
Matrix power, ^ operator
Syntax
- C = mpower(A, B)
- C = A ^ B
Input argument
- A - a variable
- B - a variable
Output argument
- C - result of A^B
Description
C = mpower(A, B) performs matrix power operation: A^B
Example
mpower(3, 4)
3^4
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
mrdivide
Matrix right division, / operator.
Syntax
- C = mrdivide(A, B)
- C = A / B
Input argument
- A - a variable
- B - a variable
Output argument
- C - result of A / B
Description
C = mrdivide(A, B) returns the matrix right division of A and B.
Example
B = ones(3, 4)
A = B *2
A / B
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
mtimes
Matrix multiplication, * operator
Syntax
- C = mtimes(A, B)
- C = A * B
Input argument
- A - a variable
- B - a variable
Output argument
- C - result of A * B
Description
C = mtimes(A, B) performs matrix multiplication operation: A * B.
Examples
mtimes(3, 4)
3 * 4
M1 = [2 6 10; 4 8 70];
M2 = [-25 88 1; 23 29 41; 24 40 0];
M1 * M2
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
ne
Inequality, ~= operator
Syntax
- C = ne(A, B)
- C = A ~= B
Input argument
- A - a variable
- B - a variable
Output argument
- C - result of A ~= B
Description
C = ne(A, B) performs inequality operation: A ~= B variables.
ne compares both real and imaginary parts of numeric arrays.
Example
ne(3, 4)
3 ~= 4
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
not
not logical, ~ operator
Syntax
- C = not(A)
- C = ~A
Input argument
- A - a variable
Output argument
- C - result of ~A
Description
C = not(A) performs not logical ~A.
Example
M = false(3, 3);
~M
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
or
logical 'OR' operator, |
Syntax
- C = or(A, B)
- C = A | B
Input argument
- A - a variable
- B - a variable
Output argument
- C - result of A | B
Description
C = or(A, B) performs a logical OR operation.
Example
A = [6 8 0; 0 3 89; 15 0 0]
B = [66 56 0; 11 33 55; -11 0 0]
C = A | B
D = or(B, A)
C == D
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
plus
Addition, + operator
Syntax
- C = plus(A, B)
- C = A + B
Input argument
- A - a variable
- B - a variable
Output argument
- C - result of A + B
Description
C = plus(A, B) performs addition A + B variables.
Examples
plus(3, 4)
3 + 4
[1, 2] + 1
plus([1, 2], 1)
ones(0, 0) + 1
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
power
Element wise power, .^ operator
Syntax
- C = power(A, B)
- C = A .^ B
Input argument
- A - a variable
- B - a variable
Output argument
- C - result of A.^B
Description
C = power(A, B) performs an element wise power operation: A .^ B .
Example
power(3, 4)
3.^4
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
rdivide
Right division, ./ operator
Syntax
- C = rdivide(A, B)
- C = A ./ B
Input argument
- A - a variable
- B - a variable
Output argument
- C - result of A ./ B
Description
C = rdivide(A, B) performs right division operation: A ./* B.
Examples
rdivide(3, 4)
3 ./ 4
M1 = [2];
M2 = [-25 88 1];
M1 ./ M2
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
shortcutand
Short circuit 'AND' operator, &&
Syntax
- C = A && B
Input argument
- A - a variable
- B - a variable
Output argument
- C - result of A && B
Description
C = A && B performs a logical AND operation, the second operand is evaluated only when the result is not fully determined by the first operand.
Example
A = [6 8 0; 0 3 89; 15 0 0]
B = [66 56 0; 11 33 55; -11 0 0]
C = A && B
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
shortcutor
Short circuit 'OR' operator, ||
Syntax
- C = A || B
Input argument
- A - a variable
- B - a variable
Output argument
- C - result of A || B
Description
C = A || B performs a logical OR operation, the second operand is evaluated only when the result is not fully determined by the first operand.
Example
A = [6 8 0; 0 3 89; 15 0 0]
B = [66 56 0; 11 33 55; -11 0 0]
C = A || B
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
subsasgn
Redefine subscripted assignment.
Syntax
- B = subsasgn(A, S, B)
Input argument
- A - Object used in indexing operation
- S - Structure with two fields: 'type' and 'subs'.
- B - The assigned value, located on the right side of the assignment statement.
Output argument
- R - The outcome of the assignment statement is the object that has been modified, and this modified object is provided as the first argument.
Description
B = subsasgn(A, S, B) assigns a value to an element of a cell or matrix.
Example
Parentheses Indexing
R1 = {1, 'GoodBye', [1, 2;3, 4]};
S = substruct('{}', {1, 3});
R2 = subsasgn(R1, S, 'Hello')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
subsindex
Convert an object to an index vector.
Syntax
- r = subsindex(O)
Input argument
- O - a variable
Description
If O is an object then subsindex is the overloading method that allows to convert this object to a valid indexing vector.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
subsref
Subscripted reference.
Syntax
- B = subsref(A, S)
Input argument
- A - Indexed object array
- B - Indexing structure
Output argument
- B - Result of indexing expression
Description
B = subsref(A, S) is invoked when using the syntax A(i), A{i}, or A.i with an object A.
Examples
Parentheses Indexing
A = magic(5);
S.type='()';
S.subs={1:2,':'};
R = subsref(A, S)
Brace Indexing
C = {"one", 2, 'three'};
S = [];
S.type = '{}';
S.subs = {[1 2]};
[R1, R2] = subsref(C, S);
Dot Indexing
A = struct('number', 10);
S = [];
S.type = '.';
S.subs = 'number';
R = subsref(A, S)
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
mtimes
Element wise multiplication, .* operator
Syntax
- C = times(A, B)
- C = A .* B
Input argument
- A - a variable
- B - a variable
Output argument
- C - result of A .* B
Description
C = times(A, B) performs element wise multiplication operation: A .* B.
Examples
times(3, 4)
3 .* 4
M1 = [2 6 10; 4 8 70];
M2 = [-25 88 1; 23 29 41];
M1 .* M2
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
transpose
Returns vector or matrix transpose: .' operator.
Syntax
- C= transpose(A)
- C = A .'
Input argument
- A - a variable
Output argument
- C - result: transpose of A.
Description
C = transpose(A) returns the transpose of A.
Examples
A = 3
B = A.'
A = -i
B = A.'
A = sparse(eye(3, 4) * i)
B = A.'
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
uminus
Unary minus, - operator
Syntax
- C = uminus(A)
- C = -A
Input argument
- A - a variable
Output argument
- C - result of -A
Description
C = uminus(A) performs unary minus ie -A.
Example
M = 3;
-M
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
uplus
Unary plus, + operator
Syntax
- C = uplus(A)
- C = +A
Input argument
- A - a variable
Output argument
- C - result of +A
Description
C = uplus(A) performs unary plus ie +A.
Example
M =-3;
+M
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
vertcat
Vertical concatenation.
Syntax
- R = vertcat(M1, M2, ... , MN)
- R = [M1; M2; ... ; MN]
Input argument
- M1 - a variable
- M2 - a variable
- MN - a variable
Output argument
- R - result of [M1; M2; ... ; MN]
Description
R = vertcat(M1, M2, ... , MN) returns the vertical concatenation of M1, M2, ... , MN along the dimension 1.
Examples
A = eye(2, 2);
B = ones(2, 2);
C = vertcat(A, B)
D = [A; B]
A = 'nel';
B = 'son';
C = vertcat(A, B)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
Elementary functions
Elementary functions
Description
elementary functions
- abs - Absolute value
- allfinite - Check if all array elements are finite.
- angle - Phase angle
- base2dec - Convert number in a base to decimal.
- bernsteinMatrix - Bernstein matrix
- bin2dec - Convert number in base 2 to decimal.
- bin2num - Convert two's complement binary string to number.
- blkdiag - Block diagonal matrix
- cast - Converts variable to a different data type
- ceil - Round up
- circshift - Circular shift
- complex - Creates an complex number.
- conj - Complex conjugate
- deal - Distribute inputs to outputs.
- dec2base - Convert decimal number to another base.
- dec2bin - Convert decimal number to base 2.
- dec2hex - Convert decimal number to base 16.
- exp - Exponential
- factorial - Factorial function
- filter - 1-D digital filter
- find - Find Non-zero Elements
- fix - Round towards zero
- flip - Flip order of elements
- flipdim - Flip array along specified dimension
- fliplr - Flip order of elements left to right
- flipud - Flip order of elements up to dow
- floor - Round down
- hadamard - Hadamard matrix
- hankel - Hankel matrix
- hex2dec - Convert number in base 16 to decimal.
- hilb - Hilbert matrix
- hypot - Square root of sum of squares
- imag - Imaginary part of an complex number.
- ind2sub - Linear index to matrix subscript values
- invhilb - Inverse of Hilbert matrix
- ipermute - Inverse permute array dimensions.
- isapprox - Return true if arguments are approximately equal, within the precision.
- iscolumn - Determine whether input is column vector.
- istriu - Checks if matrix is diagonal.
- isequal - Return true if all arguments x1, x2, ... , xn are equal (same dimensions, same values).
- isequaln - Return true if all arguments x1, x2, ... , xn are equal (same dimensions, same values or NaNs).
- isequalto - Return true if all arguments x1, x2, ... , xn are equal (same type, same dimensions, same values or NaNs).
- isfinite - Check for finite entries.
- isinf - Check for Infinity entries.
- ismatrix - determines whether input is matrix or not
- isnan - Check for Not a Number entries.
- isrow - Determine whether input is row vector.
- isscalar - Check if the input is a scalar
- istril - Checks if matrix is lower triangular.
- istriu - Checks if matrix is upper triangular.
- isvector - Checks input is vector.
- length - Length of an object.
- linspace - linearly spaced vector constructor.
- log - Natural logarithm.
- log10 - Common logarithm (base 10).
- log1p - log(1 + x) accurately for small values of x.
- log2 - dissect floating-point numbers into base 2 exponent and mantissa.
- logspace - logarithmically spaced vector constructor.
- magic - Magic square
- meshgrid - Cartesian rectangular grid in 2-D or 3-D.
- minus - Subtraction, - operator
- mod - Modulus after division.
- ndgrid - Rectangular grid in N-D space
- ndims - Number of dimensions of an array.
- nextpow2 - Exponent of next higher power of 2
- norm - Matrix and vector norms
- normest - 2-norm estimate
- nthroot - The real 𝑛th root of real number.
- num2bin - Convert number to binary representation.
- numel - Number of elements.
- permute - Permute array dimensions.
- pinv - Moore-Penrose pseudoinverse
- pow2 - Base 2 exponentiation and scaling of floating-point numbers.
- real - Real part of an complex number.
- rem - Remainder after division.
- repmat - Replicate and tile an array.
- reshape - Reshapes a vector or a matrix to a different size matrix.
- rosser - Classic symmetric eigenvalue test problem.
- rot90 - Rotate array 90 degrees.
- round - Round to nearest integer
- shiftdim - Shift array dimensions
- sign - Find the sign function of a number.
- size - Size of an object.
- sqrt - Square root.
- squeeze - Remove dimensions of length 1.
- sub2ind - Matrix subscript values to linear index
- substruct - Create structure argument for subsasgn or subsref
- swapbytes - Swap byte ordering.
- toeplitz - Toeplitz matrix
- tril - Lower triangular part of matrix
- triu - Upper triangular part of matrix
- vander - Vandermonde matrix
- wilkinson - Wilkinson's eigenvalue test matrix
abs
Absolute value
Syntax
- R = abs(M)
Input argument
- M - a variable
Output argument
- R - result of abs: absolute value.
Description
abs computes the absolute value.
If input argument is a complex number, abs computes the complex magnitude.
Example
x = [1+i,-i;i,2i];
r = abs(x)
See also
conj.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
allfinite
Check if all array elements are finite.
Syntax
- tf = allfinite(M)
Input argument
- M - a variable
Output argument
- tf - logical: result of 'allfinite'.
Description
allfinite returns a logical scalar which is true where elements of M are all finite values.
Example
X = sparse([1 2 NaN 3 0 Inf 0 4]);
R = allfinite(X)
R2 = isfinite(X)
See also
History
Version | Description |
---|---|
1.6.0 | initial version |
Author
Allan CORNET
angle
Phase angle
Syntax
- R = angle(Z)
Input argument
- Z - a variable (double, single, complex)
Output argument
- R - result of angle function.
Description
angle computes the phase angle, equivalent to atan2(imag(Z), real(Z)).
Example
x = [1+i,-i;i,2i];
r = angle(x)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
base2dec
Convert number in a base to decimal.
Syntax
- D = base2dec(TXT, B)
Input argument
- TXT - a char array.
- B - an integer value: [2, 36].
Output argument
- D - result of base2dec: an integer value.
Description
base2dec converts number in a base to decimal.
Note:
- dec2base and base2dec are inverses of one another.
- values are cached to speed up next computation base2dec('', 2) to clear cache.
Example
base2dec('313', 3)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
bernsteinMatrix
Bernstein matrix
Syntax
- B = bernsteinMatrix(n, t)
Input argument
- n - nonnegative integer: Approximation order.
- t - number or vector: Evaluation point.
Output argument
- B - Bernstein Matrix: length(t) - by - n+1 matrix.
Description
B = bernsteinMatrix(n, t) constructs a Bernstein matrix B with dimensions length(t) - by - (n+1), where t is a vector.
The Bernstein matrix is also referred to as the Bezier matrix.
This function can be utilized to calculate the points of a Bezier curve.
Example
t = 0:1/100:1;
B = bernsteinMatrix(3, t);
P = [0 0 0; 1 2 1; 1 -2 3; 5 2 4];
bezierCurve = B * P;
plot3(bezierCurve(:,1), bezierCurve(:,2), bezierCurve(:,3))
History
Version | Description |
---|---|
1.5.0 | initial version |
Author
Allan CORNET
bin2dec
Convert number in base 2 to decimal.
Syntax
- D = bin2dec(TXT)
Input argument
- TXT - a char array.
Output argument
- D - result of bin2dec: an integer value.
Description
bin2dec converts number in base 2 to decimal.
Note:
- bin2dec and dec2bin are inverses of one another.
Example
bin2dec('11')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
bin2num
Convert two's complement binary string to number.
Syntax
- R = bin2num(M)
Input argument
- M - a char array with.
Output argument
- R - result of num2bin: logical, single or double.
Description
bin2num converts binary character arry to a numeric array.
Note:
- num2bin always returns the binary representations in a column
- bin2num and num2bin are inverses of one another.
Used function(s)
C++ std::bitset
Bibliography
http://www.oxfordmathcenter.com/drupal7/node/43
Example
X = [65535 128; 1 0]
Y = num2bin(X)
bin2num(Y)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
blkdiag
Block diagonal matrix
Syntax
- R = blkdiag(M1, ... , MN)
Input argument
- M1, ..., MN - a numeric 2D matrix
Output argument
- R - a matrix.
Description
R = blkdiag(M1, ... , MN) build the block diagonal matrix created by aligning the input matrices M1, ... , MN along the diagonal of R.
Example
blkdiag(magic(2), magic(3), magic(4))
See also
diag.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
cast
Converts variable to a different data type
Syntax
- R = cast(V, type_destination)
- R = cast(V, 'like', W)
Input argument
- V - a variable
- type_destination - a string: name of destination data type.
- W - a variable
Output argument
- R - a variable with new data type.
Description
cast converts variable to a different data type.
R = cast(V, 'like', W) converts varible V to sparsity and same data type than W.
Example
r = cast([3.6 1.2 -2.4], 'like', int64(3))
r = cast([3.6 1.2 -2.4], 'int64')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
ceil
Round up
Syntax
- C = ceil(A)
Input argument
- A - a variable
Output argument
- C - result of ceil.
Description
ceil returns an integer or complex matrix made of rounded up elements.
Example
ceil(pi)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
circshift
Circular shift
Syntax
- R = circshift(M, N)
- R = circshift(M, N, DIM)
Input argument
- M - a variable
- N - shift
- DIM - dimension to operate
Output argument
- R - result of 'circshift'.
Description
circshift computes circular shift.
Example
x = [10, 20, 30; 40, 50, 60; 70, 80, 90];
circshift (x, 1
circshift (x, -2))
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
complex
Creates an complex number.
Syntax
- cpx = complex(a)
- cpx = complex(a, b)
Input argument
- a - a variable: real part
- b - a variable: imaginary part
Output argument
- cplx - result of a + b*i
Description
complex returns a complex value from real arguments.
With only one input argument, complex returns a complex value a + 0*i.
Example
z = complex(3, 2)
z2 = complex(Inf, Inf)
z3 = Inf + Inf * i
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
conj
Complex conjugate
Syntax
- CZ = conj(M)
Input argument
- M - a variable
Output argument
- CZ - result of conj: complex conjugate.
Description
conj returns the complex conjugate.
Example
x = [1+i,-i;i,2i];
r = conj(x)
See also
real.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
deal
Distribute inputs to outputs.
Syntax
- [R1, ... , Rn] = deal(A1, ... , An)
- [R1, ... , Rn] = deal(A)
Input argument
- A1, ... , An - variables
Output argument
- R1, ... , Rn - variables
Description
deal replicates the input parameters to the corresponding output parameters.
If a singular input parameter is provided, its value will be duplicated across all outputs.
Examples
[A1, A2, A3] = deal(pi)
S = [];
S.A = [];
S(2).A = [];
S(3).A = [];
A1 = 200;
A2 = 'fifo';
A3 = 1:11;
[S.A] = deal(A1, A2, A3)
C = cell(1,3)
A1 = 200;
A2 = 'fifo';
A3 = 1:11;
[C{:}] = deal(A1, A2, A3)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
dec2base
Convert decimal number to another base.
Syntax
- R = dec2base(D, B)
- R = dec2base(D, B, N)
Input argument
- D - a nonnegative integer smaller than the value returned by flintmax.
- B - an integer value [2, 36].
- N - an integer value. number of digits.
Output argument
- R - result of dec2base: char array.
Description
dec2base converts decimal number to another base.
values are cached to speed up next computation dec2base([], 2) to clear cache.
Example
X = [65535 128; 1 0]
Y = dec2base(X, 2)
Y = dec2base(X, 2, 26)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
dec2bin
Convert decimal number to base 2.
Syntax
- R = dec2bin(D)
- R = dec2bin(D, N)
Input argument
- D - a non negative integer smaller than the value returned by flintmax.
- N - an integer value. number of digits.
Output argument
- R - result of dec2bin: char array.
Description
dec2bin converts decimal number to base 2.
Example
Y = dec2bin(2)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
dec2hex
Convert decimal number to base 16.
Syntax
- R = dec2hex(D)
- R = dec2hex(D, N)
Input argument
- D - a non negative integer smaller than the value returned by flintmax.
- N - an integer value. number of digits.
Output argument
- R - result of dec2hex: char array.
Description
dec2hex converts decimal number to base 16.
Example
Y = dec2hex(12)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
exp
Exponential
Syntax
- R = exp(M)
Input argument
- M - a variable
Output argument
- R - result of exp: exponential.
Description
exp computes the exponential value.
If input argument is a complex number, exp computes exp(x) * (cos(y) + i * sin(y)) with z = x + i * y.
Example
x = [1+i,-i;i,2i];
r = exp(x)
See also
conj.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
factorial
Factorial function
Syntax
- R = factorial(M)
Input argument
- M - a integer, real single or real double matrix.
Output argument
- R - result of factorial function.
Description
factorial computes the factorial function: the product of all integers values: 1 * 2 * ... * M
Example
R = factorial([1:10])
R = factorial(int8(4))
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
filter
1-D digital filter
Syntax
- y = filter(b, a, x)
Input argument
- b - Numerator coefficients of rational transfer function: vector.
- a - Denominator coefficients of rational transfer function: vector.
- x - Input data: matrix.
Output argument
- y - Filtered data: matrix.
Description
The function filter(b, a, x) applies a rational transfer function to filter the input data array x.
This transfer function is defined by the coefficients of the numerator (b) and denominator (a).
If the first coefficient of a (a(1)) is not equal to 1, the filter normalizes the coefficients by a(1). It is crucial for a(1) to be nonzero.
When x is a vector, the function returns a vector of the same size as x containing the filtered data.
Example
f = figure();
rng default
t = linspace(-pi,pi,100);
X = sin(t) + (0.33 * rand(size(t)));
windowSize = 7;
b = (1/windowSize)*ones(1,windowSize);
a = 1;
y = filter(b, a, X);
plot(t, X)
hold on
plot(t, y)
legend(_('Input Data'), _('Filtered Data'));
See also
conv.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
find
Find Non-zero Elements
Syntax
- K = find(M)
- [R, C] = find(M)
- [R, C, V] = find(M)
- K = find(M, N)
- [R, C] = find(M, N)
- [R, C, V] = find(M, N)
- K = find(M, N, D)
- [R, C] = find(M, N, D)
- [R, C, V] = find(M, N, D)
Input argument
- M - a scalar, vector, matrix, or multidimensional array.
- N - positive integer scalar value: number of nonzeros to find.
- D - direction: 'first' (default) or 'last'.
Output argument
- K - indices to nonzero elements (vector).
- R - row subscripts (vector).
- C - column subscripts (vector).
- V - nonzero elements of M (vector).
Description
K = find(M) returns a vector with the linear indices of each nonzero element of M.
Example
M = rand(4, 3, 5);
[R, C, V] = find(M > 0.9)
M(R(1),C(1),V(1))
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
fix
Round towards zero
Syntax
- C = fix(A)
Input argument
- A - a variable
Output argument
- C - result of fix.
Description
fix returns an integer matrix made of nearest rounded integers toward zeros.
Example
fix(pi)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
flip
Flip order of elements
Syntax
- B = flip(A, dim)
Input argument
- A - an array
- dim - an positive integer value
Output argument
- B - flipped array.
Description
flip return an new array of A flipped about the dimension dim.
Example
x = eye(3, 2);
y = flip(x, 1)
y = flip(x, 2)
y = flip(x, 3)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
flipdim
Flip array along specified dimension
Syntax
- B = flipdim(A, dim)
Input argument
- A - an array
- dim - an positive integer value
Output argument
- B - flipped array.
Description
flipdim return an new array of A flipped about the dimension dim.
flipdim is similar to flip and available for compatibility with old existing scripts.
Example
x = eye(3, 2);
y = flipdim(x, 1)
y = flipdim(x, 2)
y = flipdim(x, 3)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
fliplr
Flip order of elements left to right
Syntax
- B = fliplr(A)
Input argument
- A - an array
Output argument
- B - flipped array.
Description
fliplr return an new array of A flipped left to right.
Example
x = eye(3, 2);
y = fliplr(x)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
flipud
Flip order of elements up to dow
Syntax
- B = flipud(A)
Input argument
- A - an array
Output argument
- B - flipped array.
Description
fliplr return an new array of A flipped up to down.
Example
x = eye(3, 2);
y = flipud(x)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
floor
Round down
Syntax
- C = floor(A)
Input argument
- A - a variable
Output argument
- C - result of floor.
Description
floor returns an integer matrix made of nearest rounded down integers.
Example
floor(pi)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
hadamard
Hadamard matrix
Syntax
- H = hadamard(n)
- H = hadamard(n, classname)
Input argument
- n - scalar integer value: order.
- classname - row character vector or scalar string: class name desired ('double' by default).
Output argument
- H - Hadamard Matrix.
Description
H = hadamard(n) returns the Hadamard Matrix of order n.
Bibliography
https://en.wikipedia.org/wiki/Hadamard_matrix , https://mathworld.wolfram.com/HadamardMatrix.html
Example
H = hadamard(4)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
hankel
Hankel matrix
Syntax
- H = hankel(c)
- H = hankel(c, r)
Input argument
- c - First column of Hankel matrix: vector or scalar.
- r - Last row of Hankel matrix: vector or scalar.
Output argument
- H - Hankel Matrix.
Description
H = hankel(c) returns a square Hankel Matrix with c the first column of the matrix and the elements are zero below the main anti-diagonal.
H = hankel(c, r) returns a Hankel matrix with c as its first column and r as its last row.
If last element of c differs from the first element of r, then Hankel issues a warning and uses the last element of c for the anti-diagonal.
Example
c = [1 2 3 4 5];
hankel(c)
See also
hilb.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
hex2dec
Convert number in base 16 to decimal.
Syntax
- D = hex2dec(TXT)
Input argument
- TXT - a char array.
Output argument
- D - result of hex2dec: an integer value.
Description
hex2dec converts number in base 16 to decimal.
Note:
- hex2dec and dec2hex are inverses of one another.
Example
bin2dec('11')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
hilb
Hilbert matrix
Syntax
- h = invhilb(n)
- h = invhilb(n, className)
Input argument
- n - a scalar, nonnegative integer.
- className - 'single' or 'double' (default).
Output argument
- h - Hilbert matrix.
Description
hilb computes the exact inverse of the exact Hilbert matrix.
Bibliography
https://en.wikipedia.org/wiki/David_Hilbert, and Thanks to https://nhigham.com/2020/06/30/what-is-the-hilbert-matrix/
Example
h = invhilb(5)
See also
hilb.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
hypot
Square root of sum of squares
Syntax
- C = hypot(A, B)
Input argument
- A - a variable: scalars, vectors, matrices, multidimensional arrays single or double
- B - a variable: scalars, vectors, matrices, multidimensional arrays single or double
Output argument
- R - result of hypot: hypothenuse.
Description
hypot computes the hypothenuse.
If one or both inputs is NaN, then hypot returns NaN.
Example
R = hypot(1e308, 1e308)
R = hypot(1e309, 1e309)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
imag
Imaginary part of an complex number.
Syntax
- im = imag(M)
Input argument
- M - a variable
Output argument
- R - imaginary part of the elements of the complex array M.
Description
R = imag(M) Return the imaginary part of M.
Example
cplx = 22+34*i;
r = imag(cplx)
See also
real.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
ind2sub
Linear index to matrix subscript values
Syntax
- [row, col] = ind2sub(sz, ind)
- [I1, I2, ..., In] = ind2sub(sz, ind)
Input argument
- sz - size of array: vector of positive integers.
- ind - linear indices.
Output argument
- row - row subscripts.
- col - column subscripts.
- I1, I2, ..., In - multidimensional subscripts.
Description
ind2sub converts linear indices to subscript.
Example
ind = [4 5 6 7];
sz = [4 4];
[row,col] = ind2sub(sz,ind)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
invhilb
Inverse of Hilbert matrix
Syntax
- h = hilb(n)
- h = hilb(n, className)
Input argument
- n - a scalar, nonnegative integer.
- className - 'single' or 'double' (default).
Output argument
- h - Hilbert matrix.
Description
hilb computes the Hilbert matrix.
Bibliography
https://en.wikipedia.org/wiki/David_Hilbert, and Thanks to https://nhigham.com/2020/06/30/what-is-the-hilbert-matrix/
Example
h = hilb(5)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
ipermute
Inverse permute array dimensions.
Syntax
- R = ipermute(A, order)
Input argument
- A - an array.
- order - Dimension order: row vector
Output argument
- R - result array rearranged with new dimension order.
Description
ipermute permutes the dimensions of an array (in inverse order of permute).
Example
x = [1 2 3; 4 5 6]
y = permute(x,[3 1 2])
x2 = ipermute(y,[3 1 2])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
isapprox
Return true if arguments are approximately equal, within the precision.
Syntax
- res = isapprox(x1, x2)
- res = isapprox(x1, x2, precision)
Input argument
- x1 - a matrix, a sparse matrix of doubles, or a multidimensional matrix.
- x2 - a matrix, a sparse matrix of doubles, or a multidimensional matrix.
- precision - a double value: 0. by default
Output argument
- res - a logical value
Description
For matrices, the comparison is done using the Hilbert-Schmidt norm (aka Frobenius norm L2 norm).
isapprox manages complex numbers. In this case, the real parts of the input arguments are compared. If this fails, it returns false. If this succeeds, the imaginary parts are compared.
To compare values, NaN, Inf, -Inf and remaining values, are separated. As it is impossible to compare NaN values between them, we compare the indices where NaN value occurs. For infinity values, we also compare the indices where Inf values occurs.
Examples
A = pi
B = single(pi)
res = isapprox(A, B)
A = pi
B = single(pi)
res = isapprox(A, B, 1e-4)
A = [pi NaN]
res = isapprox(A, A)
A = [pi NaN]
B = [pi + 2*eps, NaN]
res = isapprox(A, B)
res = isapprox(A, B, eps)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
iscolumn
Determine whether input is column vector.
Syntax
- tf = iscolumn(V)
Input argument
- V - a variable
Output argument
- tf - logical: result of 'iscolumn'.
Description
iscolumn(V) returns logical true if size(V) returns [n, 1] with a nonnegative integer value n, and logical false otherwise.
Example
iscolumn([1:4])
iscolumn([1:4]')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
istriu
Checks if matrix is diagonal.
Syntax
- tf = isdiag(M)
Input argument
- M - a numeric array
Output argument
- tf - logical: result of 'isdiag'.
Description
isdiag returns an scalar logical if entry is diag.
Example
A = eye(3, 3);
R = isdiag(A)
R = isdiag(A(:,1))
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
isequal
Return true if all arguments x1, x2, ... , xn are equal (same dimensions, same values).
Syntax
- res = isequal(x1, x2)
- res = isequal(x1, x2, xn)
Input argument
- x1 - a value
- x2 - a value
- xn - a value
Output argument
- res - a logical value
Description
isequal returns true if x1 and x2 are the same size and their contents are of equal value; otherwise, it returns false. isequal compares real and imaginary parts of numeric arrays. NaN (Not a Number) values are considered to be unequal to other elements.
Examples
A = eye(3, 3);
res = isequal(A, A)
A = eye(3, 3);
B = single(A)
res = isequal(A, B)
res = isequalto(A, B)
res = isequal('nel', 'son')
res = isequalnNaN, NaN)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
isequaln
Return true if all arguments x1, x2, ... , xn are equal (same dimensions, same values or NaNs).
Syntax
- res = isequaln(x1, x2)
- res = isequaln(x1, x2, xn)
Input argument
- x1 - a value
- x2 - a value
- xn - a value
Output argument
- res - a logical value
Description
isequaln returns true if x1 and x2 are the same size and same values; otherwise, it returns false. isequaln compares real and imaginary parts of numeric arrays. NaN (Not a Number) values are considered to be equal to other elements.
Examples
A = eye(3, 3);
res = isequaln(A, A)
A = eye(3, 3);
B = single(A)
res = isequaln(A, B)
res = isequalto(A, B)
res = isequaln('nel', 'son')
res = isequaln(NaN, NaN)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
isequalto
Return true if all arguments x1, x2, ... , xn are equal (same type, same dimensions, same values or NaNs).
Syntax
- res = isequalto(x1, x2)
- res = isequalto(x1, x2, xn)
Input argument
- x1 - a value
- x2 - a value
- xn - a value
Output argument
- res - a logical value
Description
isequalto returns true if x1 and x2 are the same type, same size and same values; otherwise, it returns false.
Example
A = eye(3, 3);
res = isequal(A, single(A))
res = isequalto(A, single(A))
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
isfinite
Check for finite entries.
Syntax
- tf = isfinite(M)
Input argument
- M - a variable
Output argument
- tf - logical: result of 'isfinite'.
Description
isfinite returns a logical array which is true where elements of M are finite values.
Example
isfinite(pi)
isfinite(Inf)
isfinite(-Inf)
isfinite(int32(3))
X = sparse([1 2 NaN 3 0 Inf 0 4]);
R = isfinite(X)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
isinf
Check for Infinity entries.
Syntax
- tf = isinf(M)
Input argument
- M - a variable
Output argument
- tf - logical: result of 'isinf'.
Description
isnan returns a logical array which is true where elements of M are Infinity values.
Example
isnan(pi)
isinf(Inf)
isinf(-Inf)
isinf(int32(3))
X = sparse([1 2 NaN 3 0 Inf 0 4]);
R = isinf(X)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
ismatrix
determines whether input is matrix or not
Syntax
- TF = ismatrix(A)
Input argument
- A - input array as a scalar, vector, matrix, or multidimensional array.
Output argument
- TF - a logical: true if it is a matrix.
Description
TF = ismatrix(A) returns true if A is a matrix.
A matrix is a two-dimensional array that has a size of m-by-n, where m and n are nonnegative integers.
Example
x = [1+i,-i;i,2i];
ismatrix(x)
ismatrix(ones(3,1,2))
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
isnan
Check for Not a Number entries.
Syntax
- tf = isnan(M)
Input argument
- M - a variable
Output argument
- tf - logical: result of 'isnan'.
Description
isnan returns a logical array which is true where elements of M are "Not a Number" values.
Example
isnan(pi)
isnan(NaN)
isnan(int32(3))
X = sparse([1 2 NaN 3 0 NaN 0 4]);
R = isnan(X)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
isrow
Determine whether input is row vector.
Syntax
- tf = isrow(V)
Input argument
- V - a variable
Output argument
- tf - logical: result of 'isrow'.
Description
isrow(V) returns logical true if size(V) returns [1, n] with a nonnegative integer value n, and logical false otherwise.
Example
isrow([1:4])
isrow([1:4]')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
isscalar
Check if the input is a scalar
Syntax
- TF = iscalar(A)
Input argument
- A - input array as a scalar, vector, matrix, or multidimensional array.
Output argument
- TF - a logical: true if it is a scalar.
Description
TF = isscalar(A) returns logical true if A is a scalar, meaning it is a 1-by-1 two-dimensional array.
Otherwise, it returns logical false.
Example
x = [1+i, -i ; i, 2i];
isscalar(x)
isscalar(1)
See also
History
Version | Description |
---|---|
1.10.0 | initial version |
Author
Allan CORNET
istril
Checks if matrix is lower triangular.
Syntax
- tf = istril(M)
Input argument
- M - a numeric array
Output argument
- tf - logical: result of 'istril'.
Description
istril returns an scalar logical if entry is lower triangular.
Example
A = eye(3, 3);
R = istriu(A)
R = istriu(A(:,1))
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
istriu
Checks if matrix is upper triangular.
Syntax
- tf = istriu(M)
Input argument
- M - a numeric array
Output argument
- tf - logical: result of 'istriu'.
Description
istriu returns an scalar logical if entry is upper triangular.
Example
A = eye(3, 3);
R = istriu(A)
R = istriu(A(:,1))
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
isvector
Checks input is vector.
Syntax
- tf = isvector(M)
Input argument
- M - a variable
Output argument
- tf - logical: result of 'isvector'.
Description
isvector returns an scalar logical if entry is an vector.
Example
A = eye(3, 3);
R = isvector(A)
R = isvector(A(:,1))
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
length
Length of an object.
Syntax
- l = length(M)
Input argument
- M - a variable
Output argument
- l - the length of the largest array dimension in M.
Description
For matrix or N-dimensional array, length returns the number of elements along the largest dimension. For empty object, length returns 0. For scalar, length returns 1. For a vector, length returns the number of elements.
Example
length(ones(3, 0))
length(3)
length([1 2 3 4 5])
length(ones(3, 4, 5))
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
linspace
linearly spaced vector constructor.
Syntax
- V = linspace(s, e)
- V = linspace(s, e, n)
Input argument
- s - first value: a scalar, single or double.
- e - last value: a scalar, single or double.
- n - Number of points: a scalar, single or double (by default 100).
Output argument
- V - result of linspace: an linearly spaced vector.
Description
linspace generates an linearly spaced vector.
Example
V = linspace(1+2i, 10+10i, 4)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
log
Natural logarithm.
Syntax
- R = log(M)
Input argument
- M - a variable
Output argument
- R - result of log: Natural logarithm.
Description
log computes the natural logarithm.
If input argument is a complex number or negative, log(z) computes log(abs(z)) + angle(z) * i.
Example
x = [1+i,-i;i,2i];
r = log(x)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
log10
Common logarithm (base 10).
Syntax
- R = log10(M)
Input argument
- M - a variable
Output argument
- R - result of log: base 10.
Description
log10 computes common logarithm (base 10).
For negative real and complex values of M, log10 function returns complex values.
Example
x = [1+i,-i;i,2i];
r = log10(x)
See also
log.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
log1p
log(1 + x) accurately for small values of x.
Syntax
- R = log1p(M)
Input argument
- M - a variable
Output argument
- R - result of log(1 + x) accurately for small values of x.
Description
log1p computes log(1 + x) accurately for small values of x.
Example
x = [1+i,-i;i,2i];
r = log1p(x)
See also
log.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
log2
dissect floating-point numbers into base 2 exponent and mantissa.
Syntax
- R = log2(M)
- [F, E] = log2(M)
Input argument
- M - a variable: a matrix
Output argument
- R - result of log2: computes the base 2 logarithm of the elements of X.
- F - Mantissa values that satisfy this equation: M= F.*2.^E
- E - Exponent values that satisfy this equation: M= F.*2.^E
Description
log2 dissects several numbers into the exponent and mantissa.
[F, E] = log2(M), any zeros in M produce F = 0 and E = 0.
Input values of Inf, -Inf, or NaN are returned unchanged in F with a corresponding exponent of E = 0.
Used function(s)
std::frexp and std::logb C++ functions
Example
x = [1+i,-i;i,2i];
R = log2(x)
[F, E] = log2(x)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
logspace
logarithmically spaced vector constructor.
Syntax
- V = logspace(s, e)
- V = logspace(s, e, n)
Input argument
- s - first value: a scalar, single or double.
- e - last value: a scalar, single or double.
- n - Number of points: a scalar, single or double (by default 100).
Output argument
- V - result of logspace: an logarithmically spaced vector.
Description
logspace generates an logarithmically spaced vector.
Example
V = logspace(1+2i, 10+10i, 4)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
magic
Magic square
Syntax
- M = magic(N)
Input argument
- N - Matrix order, specified as a scalar integer.
Output argument
- M - result of magic function.
Description
M = magic(N) computes an square matrix constructed as an arrangement of the 1:n^2 such that the row sums, column sums, and diagonal sums are all equal to the same value.
Example
M = magic(3)
See also
ones.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
meshgrid
Cartesian rectangular grid in 2-D or 3-D.
Syntax
- [X, Y] = meshgrid(x, y)
- [X, Y] = meshgrid(x)
- [X, Y, Z] = meshgrid(x, y, z)
- [X, Y, Z] = meshgrid(x)
Input argument
- x - x-coordinates of points: vector
- y - y-coordinates of points: vector
- z - z-coordinates of points: vector
Output argument
- X - x-coordinates over grid: 2-D or 3-D array.
- Y - y-coordinates over grid: 2-D or 3-D array.
- Z - z-coordinates over grid: 3-D array.
Description
meshgrid creates Cartesian rectangular grid in 2-D or 3-D.
Example
x = -1:0.4:1;
y = -1:0.4:1;
[X, Y] = meshgrid(x, y)
x = 0:2:6;
y = 0:1:6;
z = 0:3:6;
[X,Y,Z] = meshgrid(x, y, z)
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
minus
Subtraction, - operator
Syntax
- C = minus(A, B)
- C = A - B
Input argument
- A - a variable
- B - a variable
Output argument
- C - result of A - B
Description
C = minus(A, B) performs subtraction A - B variables.
Examples
minus(3, 4)
3 - 4
[1, 2] - 1
minus([1, 2], 1)
ones(0, 0) - 1
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
mod
Modulus after division.
Syntax
- C = mod(A, B)
Input argument
- A - a variable: dividend
- B - a variable: divisor
Output argument
- C - result of mod(A, B)
Description
C = mod(A, B) computes the modulo of A and B, i.e : A - B .* floor (A ./ B).
This function manages also negative values.
Example
mod (-1, 3)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
ndgrid
Rectangular grid in N-D space
Syntax
- [X1, X2, ..., Xn] = ndgrid(x1, x2, ... , xn)
- [X1, X2, ..., Xn] = ndgrid(xg)
Input argument
- x1, x2, … , xn - vector: grid vectors as separate arguments.
- xg - vector: grid vector for all dimensions.
Output argument
- X1, X2, … , Xn - array: full grid representation.
Description
[X1, X2, … , Xn] = ndgrid(x1, x2, … , xn) generates an n-dimensional full grid by replicating each grid vector.
[X1, X2, … , Xn] = ndgrid(xg) In this scenario, the single grid vector xg is used for all dimensions. The number of output arguments determines the dimensionality n of the resulting grid.
Examples
M = {'apple', 'banana', 'cherry'};
N = {'blue', 'green', 'red'};
ndgrid(M , N)
[X, Y] = ndgrid(1:2:19, 2:2:12)
See also
History
Version | Description |
---|---|
1.6.0 | initial version |
Author
Allan CORNET
ndims
Number of dimensions of an array.
Syntax
- n = ndims(M)
Input argument
- M - a variable
Output argument
- n - a integer value: Number of dimensions of M.
Description
n = ndims(M) return the number of dimension of the array M.
M is greater than or equal to 2.
Example
ndims(ones(3, 0))
ndims(3)
ndims([1 2 3 4 5])
ndims(ones(3, 4, 5))
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
nextpow2
Exponent of next higher power of 2
Syntax
- R = nextpow2(M)
Input argument
- M - a variable
Output argument
- R - result of nextpow2: next higher power of 2.
Description
if M is a vector or a matrix nextpow2(M) applies element-wise.
If M is a scalar, nextpow2(M) returns the first p such that 2^p >= abs(M).
Example
R = nextpow2([10, Inf, 30, -Inf, 90, NaN])
M = uint32([1020 4000 32700]);
R = nextpow2(M)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
norm
Matrix and vector norms
Syntax
- R = norm(V)
- R = norm(V, p)
- R = norm(V, 'fro')
- R = norm(M)
- R = norm(M, 1)
- R = norm(M, 2)
- R = norm(M, Inf)
- R = norm(M, 'fro')
Input argument
- M - a 2D matrix single or double
- V - a vector single or double
- p - a scalar (p-norm)
Output argument
- R - result of norm: scalar.
Description
norm computes the norm of a vector or a matrix.
Frobenius norm of M is equal to sqrt (sum (diag (M' * M))) .
Examples
M = [1 2; 3 4];
norm(M)
norm(M, 1)
norm(M, 2)
norm(M, Inf)
norm(M, 'fro')
V = [1 2 3 4];
norm(V)
norm(V, 1)
norm(V, 2)
norm(V, Inf)
norm(V, 'fro')
x = ones(3000, 3000);
tic();R = norm(x);toc
See also
svd.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
normest
2-norm estimate
Syntax
- nrm = normest(A)
- [nrm, count] = normest(A)
- nrm = normest(A, tolerance)
- [nrm, count] = normest(A, tolerance)
Input argument
- A - Input matrix
- tolerance - Relative error tolerance
Output argument
- nrm - Matrix norm: scalar.
- count - Number of power iterations: scalar.
Description
nrm = normest(A) returns an estimate of the 2-norm of the matrix A.
Example
M = [ 0 2.4495 0 0 0 0 0
2.4495 0 3.1623 0 0 0 0
0 3.1623 0 3.4641 0 0 0
0 0 3.4641 0 3.4641 0 0
0 0 0 3.4641 0 3.1623 0
0 0 0 0 3.1623 0 2.4495
0 0 0 0 0 2.4495 0];
[nrm, count] = normest(M)
norm(M)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
nthroot
The real 𝑛th root of real number.
Syntax
- Y = nthroot(X, N)
Input argument
- X - Input array: scalar, vector, matrix or multidimensional array.
- N - Roots to calculate: scalar or array of same size as X.
Output argument
- Y - result of 'nthroot'.
Description
𝑌 = nthroot(𝑋, 𝑁) returns the real 𝑛th root of the elements of 𝑋.
Both 𝑋 and 𝑁 must be real scalars or arrays of the same size. If an element in 𝑋 is negative, the corresponding element in 𝑁 must be an odd integer.
When computing roots where both real and complex roots exist, the power function efficiently computes only the complex roots.
To obtain the real root in such cases, use the nthroot function instead.
Example
X = [-2 -3 -2; 4 -2 -5]
N = [1 -1 3; 1/2 5 3]
Y = nthroot(X, N)
See also
History
Version | Description |
---|---|
1.6.0 | initial version |
Author
Allan CORNET
num2bin
Convert number to binary representation.
Syntax
- R = num2bin(M)
Input argument
- M - a variable: logical, integer, single or double real full matrix.
Output argument
- R - result of num2bin: char array.
Description
num2bin returns a char array giving the literal bit representation of a number.
Used function(s)
C++ std::bitset
Bibliography
http://www.oxfordmathcenter.com/drupal7/node/43
Example
X = [65535 128; 1 0]
Y = num2bin(X)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
numel
Number of elements.
Syntax
- nbel = numel(M)
Input argument
- M - a variable
Output argument
- nbel - the number of elements.
Description
Return the number of elements in the object M.
Example
numel(ones(3, 0))
numel(ones(3,4))
numel(ones(3,4,5))
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
permute
Permute array dimensions.
Syntax
- R = permute(A, order)
Input argument
- A - an array.
- order - Dimension order: row vector
Output argument
- R - result array rearranged with new dimension order.
Description
permute permutes the dimensions of an array.
Example
x = [1 2 3; 4 5 6]
y = permute(x,[3 1 2])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
pinv
Moore-Penrose pseudoinverse
Syntax
- y = pinv(A)
- y = pinv(A, tol)
Input argument
- A - matrix: input matrix
- tol - scalar: singular value tolerance
Output argument
- y - Moore-Penrose Pseudoinverse of matrix A.
Description
pinv returns Moore-Penrose Pseudoinverse of matrix A.
Example
A = [1, 2, 3; 4, 5, 6];
R = pinv(A)
R = pinv(A, 2)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
pow2
Base 2 exponentiation and scaling of floating-point numbers.
Syntax
- Y = pow2(E)
- Y = pow2(X, E)
Input argument
- E - Exponent values
- X - Significand values
Output argument
- Y - result of pow2.
Description
Y = pow2(E) computes 2 to the power of E.
Y = pow2(X, E) computes X times 2 to the power of E.
Example
R = pow2([1, 2, 3; 4, 5, 6], [6, 5, 4; 3, 2, 1])
See also
log2.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
real
Real part of an complex number.
Syntax
- R = real(M)
Input argument
- M - a variable
Output argument
- R - real part of the elements of the complex array M.
Description
R = real(M) Return the real part of M.
Example
cplx = 22+34*i;
r = real(cplx)
See also
imag.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
rem
Remainder after division.
Syntax
- C = rem(A, B)
Input argument
- A - a variable: dividend
- B - a variable: divisor
Output argument
- C - result of rem(A, B)
Description
C = rem(A, B) computes the remainder of A and B, i.e : A - fix(A ./ B) .* B.
This function manages also negative values.
mod(A, 0) = A , whereas rem(A, 0) = NaN.
mod(A, B) has the sign of B, while rem(A, B) has the sign of A.
mod and rem are equals if A and B have the same sign.
Example
rem (-1, 3)
mod(-1, 3)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
repmat
Replicate and tile an array.
Syntax
- R = repmat(A, m)
- R = repmat(A, m, n)
- R = repmat(A, m, n, p …)
- R = repmat(A, [m n])
- R = repmat(A, [m n p …])
Input argument
- A - an array.
- m, n, p … - a value: integer
Output argument
- R - result array form by tiling.
Description
repmat repeats matrix or N-D array.
Examples
repmat(1:5, 2)
repmat(1:5, [2 3])
repmat(1:5, [2 3 4])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
reshape
Reshapes a vector or a matrix to a different size matrix.
Syntax
- M2 = reshape(M1, s1, ... ,sN)
- M2 = reshape(M1, ..., [], ...)
- M2 = reshape(M1, size)
Input argument
- M1 - a vector or an matrix
- size - a size vector
- s1, ... ,sN - a s1 - by - ... - by - sN array where s1, ..., sN indicates the size of each dimension.
Output argument
- M2 - Matrix reshaped
Description
reshape performs a reshape to a different size matrix. If only one dimension is specified, reshape will determine complementary size automatically. [ ] is used to unspecify the dimension.
Example
M1 = ones(3, 4, 5);
M2 = reshape(M1, [5, 3, 4])
M2 = reshape(M1, 5, [], 4)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
rosser
Classic symmetric eigenvalue test problem.
Syntax
- R = rosser()
- R = rosser(classname)
Input argument
- classname - row character vector or scalar string: class name desired ('double' by default).
Output argument
- R - Rosser Matrix.
Description
R = rosser() returns the Rosser Matrix.
Bibliography
https://archive.org/details/jresv47n4p291
Example
R = rosser()
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
rot90
Rotate array 90 degrees.
Syntax
- B = rot90(A)
- B = rot90(A, k)
Input argument
- A - an array
- k - an positive integer value: Rotation constant.
Output argument
- B - rotated array.
Description
B = rot90(A, k) rotates array A counter clockwise by k * 90 degrees, with k is an integer value.
Consider flip function to flip arrays in any dimension.
Example
x = eye(3, 2);
y = rot90(x, 0)
y = rot90(x, 1)
y = rot90(x, 2)
y = rot90(x, 3)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
round
Round to nearest integer
Syntax
- C = round(A)
Input argument
- A - a variable
Output argument
- C - result of round.
Description
round rounds the elements to the nearest integers.
Example
round(pi)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
shiftdim
Shift array dimensions
Syntax
- B = shiftdim(A, n)
- B = shiftdim(A)
- [B, m] = shiftdim(A)
Input argument
- A - Input array: vector, matrix or multidimensional array.
- n - Number of positions: integer value.
Output argument
- B - vector, matrix, or multidimensional array.
- m - Number of dimensions removed: non-negative integer.
Description
shiftdim(A, n) reorganizes the dimensions of an array A by n positions.
Specifically, when n is a positive integer, it shifts the dimensions to the left, and when n is a negative integer, it shifts the dimensions to the right.
Example
A = rand(2, 3, 4);
size(A)
% Shift the dimensions of array A by 2 positions to the left
B = shiftdim(A, 2)
See also
History
Version | Description |
---|---|
1.3.0 | initial version |
Author
Allan CORNET
sign
Find the sign function of a number.
Syntax
- R = sign(M)
Input argument
- M - a variable
Output argument
- R - result of sign.
Description
sign find the sign function of a number.
-1 if the corresponding element of M is less than 0.
0 if the corresponding element of M equals 0.
1 if the corresponding element of M is greater than 0.
If input argument is a complex number, sign computes M ./ abs(M).
Example
V = [-1 0 15 NaN Inf];
sign(V)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
size
Size of an object.
Syntax
- s = size(X)
- sdim = size(X, dim)
- vec = size(X, dims)
- [r, c] = size(X)
- [s1, ... , sn] = size(X)
Input argument
- X - a variable
- dim - a variable: a positive integer to get the dimth dimension.
- dims - a variable: a vector of positive integer to get the dimth dimensions.
Output argument
- s - a row vector whose elements contain the length of the corresponding dimension of X.
- sdim - the length of dimension dim.
- vec - length of dimensions dims.
- [r, c] - number of rows and columns.
- [s1, ... , sn] - numbers with integer values.
Description
Examples
X = rand(3, 4, 5, 6);
size(X)
size(X, 3)
size(X, [2 4])
[r, c] =size(X)
[s1, s2, s3, s4] = size(X)
size(cell(4,3))
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
sqrt
Square root.
Syntax
- R = sqrt(M)
Input argument
- M - a variable
Output argument
- R - result of sqrt: square root.
Description
sqrt computes the square root.
If input argument is a complex number or negative, sqrt(z) computes: sqrt(r) * (cos(phi/2) + sin(phi/2) * i) with
r = sqrt((real(z) * real(z)) + (imag(z) * imag(z))) and phi = atan2(imag(z), real(z))
Example
x = -3:3;
r = sqrt(x)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
squeeze
Remove dimensions of length 1.
Syntax
- B = squeeze(A)
Input argument
- A - input array: multidimensional array
Output argument
- B - output array.
Description
B = squeeze(A) returns an array with the same elements as the input array A, but with dimensions of length 1 removed.
Example
A = zeros(1, 1, 3);
A(:, :, 1:3) = [1 20 3];
R = squeeze(A)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
sub2ind
Matrix subscript values to linear index
Syntax
- ind = sub2ind(sz, row, col)
- ind = sub2ind(sz, I1, I2, ..., In)
Input argument
- sz - size of array: vector of positive integers.
- row - row subscripts.
- col - column subscripts.
- I1, I2, ..., In - multidimensional subscripts.
Output argument
- ind - linear indices.
Description
ind2sub converts subscripts to linear indices. .
Example
row = [2 3 4 2];
col = [2 2 2 3];
sz = [3 3];
ind = sub2ind(sz, row, col)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
substruct
Create structure argument for subsasgn or subsref
Syntax
- S = substruct(type1, subs1, type2, subs2, ...)
Description
S = substruct(type1, subs1, type2, subs2, ...) generates a structure containing fields necessary for an overloaded subsref or subsasgn method.
Each type char vector is limited to '.', '()', or '{}'.
The associated subs argument should be a field name (for the '.' type) or a cell array containing index vectors (for the '()' or '{}' types).
Example
S = struct('field1', 10, 'field2', 'Hello', 'field3', [1, 2, 3]);
% Create a substruct for accessing the 'field2'
s = substruct('.', 'field2');
% Use subsref to get the value of 'field2'
value = subsref(S, s);
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
swapbytes
Swap byte ordering.
Syntax
- R = swapbytes(M)
Input argument
- M - a variable: integer, single or double real full matrix.
Output argument
- R - result of swapbytes: reversed byte order of M.
Description
swapbytes Swap byte ordering.
endian (little - big) converter
Example
X = uint16([65535 128; 1 0])
Y = swapbytes(X)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
toeplitz
Toeplitz matrix
Syntax
- T = toeplitz(c, r)
- T = toeplitz(r)
Input argument
- c - a scalar or vector: column of Toeplitz matrix.
- r - a scalar or vector: row of Toeplitz matrix.
Output argument
- T - Toeplitz matrix.
Description
T = toeplitz(c, r) returns the Toeplitz matrix whose first row is r and first column is c.
T = toeplitz(c) returns the symmetric Toeplitz matrix.
Bibliography
https://en.wikipedia.org/wiki/Toeplitz_matrix
Example
T = toeplitz(1:5, 1:2:7)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
tril
Lower triangular part of matrix
Syntax
- T = tril(M)
- T = tril(M, k)
Input argument
- M - 2D input matrix
- k - Diagonals to include: integer real value
Output argument
- R - Lower Triangular Portions of Matrix
Description
tril computes Lower Triangular Portions of Matrix.
R = tril(M, k) returns the elements on and above the kth diagonal of M.
Example
x = [1+i,-i;i,2i];
r = tril(x)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
triu
Upper triangular part of matrix
Syntax
- T = triu(M)
- T = triu(M, k)
Input argument
- M - 2D input matrix
- k - Diagonals to include: integer real value
Output argument
- R - Upper Triangular Portions of Matrix
Description
triu computes Upper Triangular Portions of Matrix.
R = triu(M, k) returns the elements on and above the kth diagonal of M.
Example
x = [1+i,-i;i,2i];
r = triu(x)
See also
diag.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
vander
Vandermonde matrix
Syntax
- A = vander(v)
Input argument
- v - a numeric vector.
Output argument
- A - Vandermonde Matrix.
Description
A = vander(v) returns the Vandermonde Matrix.
Bibliography
https://en.wikipedia.org/wiki/Vandermonde_matrix
Example
A = vander(1:.5:3)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
wilkinson
Wilkinson's eigenvalue test matrix
Syntax
- W = wilkinson(n)
- W = wilkinson(n, classname)
Input argument
- n - scalar integer value: order.
- classname - row character vector or scalar string: class name desired ('double' by default).
Output argument
- W - Wilkinson's eigenvalue test matrix.
Description
W = wilkinson(n) returns the wilkinson Matrix of order n.
Bibliography
https://en.wikipedia.org/wiki/Wilkinson_matrix
Example
W = wilkinson(4)
See also
diag.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
Data analysis
Data analysis
Description
data analysis
- conv - Convolution and polynomial multiplication.
- conv2 - 2-D convolution.
- cumprod - Cumulative product of array elements.
- cumsum - Cumulative sum of array elements.
- ismissing - Check for missing values.
- issorted - Determine if array is sorted.
- max - Maximum elements of an array.
- min - Minimum elements of an array.
- prod - Product of array elements.
- sort - Sort array elements by quick sort algorithm.
- sum - Sum of array elements.
- unique - Unique values.
conv
Convolution and polynomial multiplication.
Syntax
- C = conv(u, v)
- C = conv(u, v, shape)
Input argument
- u - input vectors, specified as either row or column vectors.
- v - input vectors, specified as either row or column vectors.
- shape - subsection of convolution: 'full' (default: full 2-D convolution), 'same' (central part of the convolution) or 'valid' (parts of the convolution that are computed without zero-padded edges).
Output argument
- C - convolution, returned as a vector or matrix.
Description
conv returns the convolution of vectors u and v.
Example
U = [-1 2 3 -2 0 1 2];
V = [2 4 -1 1];
R = conv(U, V, 'same')
See also
conv.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
conv2
2-D convolution.
Syntax
- C = conv2(A, B)
- C = conv2(u, v, A)
- C = conv2(A, B, shape)
- C = conv2(u, v, A, shape)
Input argument
- A - vector or matrix.
- B - vector or matrix.
- u - row or column vector.
- v - row or column vector.
- shape - subsection of convolution: 'full' (default: full 2-D convolution), 'same' (central part of the convolution) or 'valid' (parts of the convolution that are computed without zero-padded edges).
Output argument
- C - 2-D convolution, returned as a vector or matrix.
Description
conv2 returns the two-dimensional convolution.
Example
A = magic(3);
B = magic(4);
R = conv2(A, B, 'same')
See also
conv.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
cumprod
Cumulative product of array elements.
Syntax
- R = cumprod(M)
- R = cumprod(M, d)
- R = cumprod(M, d, direction)
- R = cumprod(M, d, direction, nanflag)
Input argument
- M - an array of double, single, integers, ...
- d - dimension to operate along: positive integer scalar.
- direction - a string: 'reverse', 'forward' (default).
- nanflag - a string: 'includenan' (default) or 'omitnan'.
Output argument
- R - Cumulative Product of array elements.
Description
R = cumprod(M) returns the cumulative product of the array elements of M.
Example
M = uint8([10:30:70;20:30:80;30:30:90]);
R = cumprid(M)
R = cumprod(M, 'reverse')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
cumsum
Cumulative sum of array elements.
Syntax
- R = cumsum(M)
- R = cumsum(M, d)
- R = cumsum(M, d, direction)
- R = cumsum(M, d, direction, nanflag)
Input argument
- M - an array of double, single, integers, ...
- d - dimension to operate along: positive integer scalar.
- direction - a string: 'reverse', 'forward' (default).
- nanflag - a string: 'includenan' (default) or 'omitnan'.
Output argument
- R - Cumulative Sum of array elements.
Description
R = cumsum(M) returns the cumulative sum of the array elements of M.
Example
M = uint8([10:30:70;20:30:80;30:30:90]);
R = cumsum(M)
R = cumsum(M, 'reverse')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
ismissing
Check for missing values.
Syntax
- tf = ismissing(M)
Input argument
- M - a variable
Output argument
- tf - logical: result of 'ismissing'.
Description
ismissing returns a logical array which is true where elements of M are missing values.
missing data are defined as:
NaN for double or single
missing for string array
' ' for character array
'' for cell of character array
Example
A = ["Nel", NaN, "son"];
ismissing(A)
B = [1 2 NaN Inf];
ismissing(B)
C = 'Nel son'
ismissing(C)
D = {'Nel' '' 'son'}
ismissing(D)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
issorted
Determine if array is sorted.
Syntax
- tf = issorted(A)
- tf = issorted(A, dim)
- tf = issorted(..., direction)
- tf = issorted(A, 'rows')
Input argument
- A - an nelson's variable (double, single, int8, int16, int32, int64, uint8, uint16, uint32, uint64, logical, char, string, cell).
- dim - Dimension to operate along: positive integer scalar.
- direction - Sorting direction: 'ascend' (default) or 'descend'.
- 'rows' - returns true when the elements of the first column of a matrix are sorted.
Output argument
- tf - a logical: true if array is sorted.
Description
tf = issorted(A) returns true if the elements of A are sorted in ascending order, and false otherwise.
Example
x = [1 2 3 4];
issorted(x) % returns true
x = [1 3 2 4];
issorted(x) % returns false
% Check if a matrix is sorted by rows
A = [1 2 3; 4 5 6; 7 8 9];
issorted(A, 'rows') % returns true
A = [1 2 3; 7 8 9; 4 5 6];
issorted(A, 'rows') % returns false
See also
sort.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
max
Maximum elements of an array.
Syntax
- M = max(A)
- [M, I] = max(A)
- M = max(A, [], dim)
- [M, I] = max(A, [], dim)
- M = max(A, [], dim, 'omitnan')
- [M, I] = max(A, [], dim, 'includenan')
- [M, I] = max(A, [], 'all')
- [M, I] = max(A, [], 'all', 'omitnan')
- [M, I] = max(A, [], 'all', 'includenan')
- C = max(A, B)
- C = max(A, B, 'omitnan')
- C = max(A, B, 'includenan')
Input argument
- A - a variable
- dim - a positive integer scalar (Dimension to operate along)
- 'omitnan' - ignore all NaN values. default behaviour. max will return the first element, if all elements are NaN.
- 'includenan' - include the NaN values.
- 'all' - it finds the maximum over all elements.
Output argument
- M - Maximum values of A.
- I - Index to maximum values of A.
- C - Maximum elements from A or B.
Description
max find maximum values in an array.
If A is a matrix then M = max(A) is a row vector containing the maximum value of each column.
If A is a vector then M = max(A) will return the maximum of A.
If A If A is complex number then M = max(A) will return founded complex number with the largest magnitude.
Example
A = [1 2 3; 4 5 6];
M = max(A)
M = max(A, [], 'all')
See also
min.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
min
Minimum elements of an array.
Syntax
- M = min(A)
- [M, I] = min(A)
- M = min(A, [], dim)
- [M, I] = min(A, [], dim)
- M = min(A, [], dim, 'omitnan')
- [M, I] = min(A, [], dim, 'includenan')
- [M, I] = min(A, [], 'all')
- [M, I] = min(A, [], 'all', 'omitnan')
- [M, I] = min(A, [], 'all', 'includenan')
- C = min(A, B)
- C = min(A, B, 'omitnan')
- C = min(A, B, 'includenan')
Input argument
- A - a variable
- dim - a positive integer scalar (Dimension to operate along)
- 'omitnan' - ignore all NaN values. default behaviour. min will return the first element, if all elements are NaN.
- 'includenan' - include the NaN values.
- 'all' - it finds the minimum over all elements.
Output argument
- M - minimum values of A.
- I - Index to minimum values of A.
- C - minimum elements from A or B.
Description
min find minimum values in an array.
If A is a matrix then M = min(A) is a row vector containing the minimum value of each column.
If A is a vector then M = min(A) will return the minimum of A.
If A If A is complex number then M = min(A) will return founded complex number with the largest magnitude.
Example
A = [1 2 3; 4 5 6];
M = min(A)
M = min(A, [], 'all')
See also
max.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
prod
Product of array elements.
Syntax
- R = prod(M)
- R = prod(M, d)
- R = prod(M, d)
- R = prod(M, d, t)
- R = prod(M, d, t, f)
Input argument
- M - an array of double, single, integers, ...
- d - dimension to operate along: positive integer scalar.
- t - a string: 'default', 'double' or 'native'.
- f - a string: 'includenan' or 'omitnan'.
Output argument
- R - Product of array elements.
Description
R = prod(M) returns the product of the array elements of M.
Example
M = uint8([10:30:70;20:30:80;30:30:90]);
R = prod(M, 'native')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
sort
Sort array elements by quick sort algorithm.
Syntax
- B = sort(A)
- B = sort(A, dim)
- B = sort(..., direction)
- B = sort(..., name, value)
- [B, I] = sort(...)
Input argument
- A - an nelson's variable (double, single, int8, int16, int32, int64, uint8, uint16, uint32, uint64, logical, char, string, cell).
- dim - Dimension to operate along: positive integer scalar.
- direction - Sorting direction: 'ascend' (default) or 'descend'.
- name, value - name-value pair arguments.
Output argument
- B - sorted array.
- I - sort index.
Description
sort implements quick sort algorithm.
name-value pair arguments:
'MissingPlacement' - Placement of missing values: 'auto' (default), 'first', 'last'.
'ComparisonMethod' - Element comparison method: 'auto' (default), 'real', 'abs'.
Used function(s)
qsort (stl)
Bibliography
Quick sort algorithm from Bentley and McIlroy's "Engineering a Sort Function". Software - Practice and Experience
Examples
ComparisonMethod
A = [10+20i 30+i 10i 0 -10i];
B = sort(A,'ComparisonMethod', 'auto')
B = sort(A, 'ComparisonMethod', 'real')
B = sort(A, 'ComparisonMethod', 'abs')
MissingPlacement
A = [NaN 3 6 0 NaN];
[B, I] = sort(A, 'MissingPlacement', 'auto')
[B, I] = sort(A, 'MissingPlacement', 'first')
[B, I] = sort(A, 'MissingPlacement', 'last')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
sum
Sum of array elements.
Syntax
- R = sum(M)
- R = sum(M, d)
- R = sum(M, d)
- R = sum(M, d, t)
- R = sum(M, d, t, f)
Input argument
- M - an array of double, single, integers, ...
- d - dimension to operate along: positive integer scalar.
- t - a string: 'default', 'double' or 'native'.
- f - a string: 'includenan' or 'omitnan'.
Output argument
- R - Sum of array elements.
Description
R = sum(M) returns the sum of the array elements of M.
Example
M = uint8([10:30:70;20:30:80;30:30:90]);
R = sum(M, 'native')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
unique
Unique values.
Syntax
- C = unique(A)
- C = unique(A, 'rows')
- [C, ia, ic] = unique(...)
Input argument
- A - an nelson's variable (double, single, int8, int16, int32, int64, uint8, uint16, uint32, uint64, logical, char, string, cell).
Output argument
- C - Unique data of A.
- ia - Index to A: column vector.
- ic - Index to C: column vector.
Description
C = unique(A) returns the unique elements of array A in sorted order.
C = unique(A, 'rows') considers each row of A as a unique entity and returns the unique rows in sorted order.
Note that the 'rows' option does not support cell arrays.
[C, ia, ic] = unique(...) extends any of the previous syntaxes to also return index vectors ia and ic.
For a vector A, the relationships are C = A(ia) and A = C(ic).
For a matrix or array A, the relationships are C = A(ia) and A(:) = C(ic).
If the 'rows' option is used, the relationships are C = A(ia, :) and A = C(ic, :).
Used function(s)
std::sort, std::unique (stl)
Examples
A = [10+20i 30+i 10i 0 -10i];
[C, ia, ic] = unique(A)
A = {'hi', 'good'; 'good', 'tell'; 'hi', 'bye'}
[C, ia, ic] = unique(A)
See also
sort.
History
Version | Description |
---|---|
1.6.0 | initial version |
Author
Allan CORNET
Trigonometric functions
Trigonometric functions
Description
module about sine, cosine, and related functions.
- acos - Computes the inverse cosine in radians for each element of x.
- acosd - Inverse cosine in degrees.
- acosh - Inverse hyperbolic cosine.
- acot - Inverse cotangent of angle in radians
- acotd - Inverse cotangent of angle in degrees
- acoth - Inverse hyperbolic cotangent.
- acsc - Inverse cosecant in radians.
- acscd - Inverse cosecant in degrees.
- acsch - Inverse hyperbolic cosecant.
- asec - Inverse secant of angle in radians.
- asecd - Inverse secant of argument in degrees.
- asech - Inverse hyperbolic secant of angle in radians.
- asin - Computes the inverse sine in radians for each element of x.
- asind - Inverse sine in degrees.
- asinh - Inverse hyperbolic sine function
- atan - Computes the inverse tangent in radians for each element of x.
- atan2 - Computes the four-quadrant inverse tangent.
- atan2d - Four-quadrant inverse tangent in degrees.
- atand - Inverse tangent in degrees.
- atanh - Computes the inverse hyperbolic tangent.
- cart2pol - Transforms Cartesian coordinates to polar or cylindrical.
- cart2sph - Transforms Cartesian to spherical coordinates.
- cos - Computes the cosine in radians for each element of x.
- cosd - Computes the cosine in degree for each element of x.
- cosh - Computes the hyperbolic cosine in radians for each element of x.
- cosm - Computes the matrix cosine of a square matrix.
- cospi - Computes cos(X * pi) accurately.
- cot - Cotangent of angle in radians
- cotd - Cotangent of argument in degrees
- coth - Hyperbolic cotangent.
- csc - Cosecant of input angle in radians.
- cscd - Cosecant of argument in degrees.
- csch - Hyperbolic cosecant.
- deg2rad - Convert angle from degrees to radians.
- pol2cart - Transforms polar or cylindrical coordinates to Cartesian.
- rad2deg - Convert angle from radians to degrees.
- sec - Secant of angle in radians.
- secd - Secant of argument in degrees.
- sech - Hyperbolic secant.
- sin - Computes the sine in radians for each element of x.
- sind - Computes the sine in degree for each element of x.
- sinh - Computes the hyperbolic sine in radians for each element of x.
- sinm - Computes the matrix sinus of a square matrix.
- sinpi - Computes sin(X * pi) accurately.
- sph2cart - Transform spherical coordinates to Cartesian.
- tan - Computes the tangent in radians for each element of x.
- tand - Computes the tangent in degree for each element of x.
- tanh - Computes the hyperbolic tangent in radians for each element of x.
- tanm - Computes the matrix tangent of a square matrix.
acos
Computes the inverse cosine in radians for each element of x.
Syntax
- res = acos(x)
Input argument
- x - a numeric value
Output argument
- res - a numeric value
Description
acos computes the inverse cosine in radians for each element of x.
Example
A = eye(3, 3);
res = acos(A)
See also
cos.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
acosd
Inverse cosine in degrees.
Syntax
- res = acosd(x)
Input argument
- x - a numeric value
Output argument
- res - a numeric value
Description
atand computes the inverse cosine in degrees for each element of x.
Example
x = [1 -20 0 2 5];
y = acosd(x)
See also
cosd.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
acosh
Inverse hyperbolic cosine.
Syntax
- res = acosh(x)
Input argument
- x - a numeric value
Output argument
- res - a numeric value
Description
acos computes the inverse hyperbolic cosine.
Example
A = [1+2i, 2, -3];
res = acosh(A)
See also
cosh.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
acot
Inverse cotangent of angle in radians
Syntax
- res = acot(x)
Input argument
- x - a numeric value
Output argument
- res - a numeric value
Description
acot computes the inverse cotangent of angle for each element of x.
Example
R = acot([-i pi+i*pi/2 -1+i*4])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
acotd
Inverse cotangent of angle in degrees
Syntax
- res = acotd(x)
Input argument
- x - a numeric value
Output argument
- res - a numeric value
Description
acotd computes the inverse cotangent of angle for each element of x in degrees.
Example
R = acotd([-i pi+i*pi/2 -1+i*4])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
acoth
Inverse hyperbolic cotangent.
Syntax
- res = acoth(x)
Input argument
- x - a numeric value
Output argument
- res - a numeric value
Description
acoth computes the inverse hyperbolic cotangent.
Example
A = [1+2i, 2, -3];
res = acoth(A)
See also
coth.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
acsc
Inverse cosecant in radians.
Syntax
- res = acsc(x)
Input argument
- x - a numeric value
Output argument
- res - a numeric value
Description
acsc computes the inverse cosecant of argument in radians for each element of x.
Example
R = acsc(3)
R = acsc(0.5)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
acscd
Inverse cosecant in degrees.
Syntax
- res = acsc(x)
Input argument
- x - a numeric value
Output argument
- res - a numeric value
Description
acscd computes the inverse cosecant of argument in degrees for each element of x.
Example
x = [0 1 20 10 Inf];
y = acscd(x)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
acsch
Inverse hyperbolic cosecant.
Syntax
- res = acsch(x)
Input argument
- x - a numeric value
Output argument
- res - a numeric value
Description
acsch computes the inverse hyperbolic cosecant for each element of x.
Example
X = [3*pi, 2*pi, pi, 0];
R = acsch(X)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
asec
Inverse secant of angle in radians.
Syntax
- res = asec(x)
Input argument
- x - a numeric value
Output argument
- res - a numeric value
Description
asec computes the inverse secant of argument in radians for each element of x.
Example
x = -pi:0.75:pi;
R = asec(x)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
asecd
Inverse secant of argument in degrees.
Syntax
- res = asecd(x)
Input argument
- x - a numeric value
Output argument
- res - a numeric value
Description
asecd computes the inverse secant of argument in degrees for each element of x.
Example
R = asecd([1, 10+3i, 15+2i, 35+i])
See also
asec.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
asech
Inverse hyperbolic secant of angle in radians.
Syntax
- res = asech(x)
Input argument
- x - a numeric value
Output argument
- res - a numeric value
Description
asech computes the inverse hyperbolic secant of argument in radians for each element of x.
Example
x = -pi:0.75:pi;
R = asech(x)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
asin
Computes the inverse sine in radians for each element of x.
Syntax
- res = asin(x)
Input argument
- x - a numeric value
Output argument
- res - a numeric value
Description
asin computes the inverse sine in radians for each element of x.
Example
A = eye(3, 3);
res = asin(A)
See also
sin.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
asind
Inverse sine in degrees.
Syntax
- res = asind(x)
Input argument
- x - a numeric value
Output argument
- res - a numeric value
Description
asind computes the inverse sine in degrees for each element of x.
Example
x = [-50 -20 0 20 50];
y = asind(x)
See also
sind.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
asinh
Inverse hyperbolic sine function
Syntax
- res = asinh(x)
Input argument
- x - a numeric value
Output argument
- res - a numeric value
Description
cosh computes the inverse hyperbolic sine in radians for each element of x.
Example
A = eye(3, 3);
res = asinh(A)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
atan
Computes the inverse tangent in radians for each element of x.
Syntax
- res = atan(x)
Input argument
- x - a numeric value
Output argument
- res - a numeric value
Description
atan computes the inverse tangent in radians for each element of x.
Example
A = eye(3, 3);
res = atan(A)
See also
tan.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
atan2
Computes the four-quadrant inverse tangent.
Syntax
- res = atan2(y, x)
Input argument
- y - a numeric value (double or single real)
- x - a numeric value (double or single real)
Output argument
- res - a numeric value
Description
atan2 computes the four-quadrant inverse tangent.
Example
atan2(1, 0)
See also
atan.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
atan2d
Four-quadrant inverse tangent in degrees.
Syntax
- d = atan2d(y, x)
Input argument
- y - a numeric value
- x - a numeric value
Output argument
- d - a numeric value
Description
d = atan2d(y, x) returns the four-quadrant inverse tangent (tan-1) of y and x, which must be real.
Example
x = [1 0 -1 0];
y = [0 1 0 -1];
d = atan2d(y, x)
See also
tand.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
atand
Inverse tangent in degrees.
Syntax
- res = atand(x)
Input argument
- x - a numeric value
Output argument
- res - a numeric value
Description
atand computes the inverse tangent in degrees for each element of x.
Example
x = [-50 -20 0 20 50];
y = atand(x)
See also
tand.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
atanh
Computes the inverse hyperbolic tangent.
Syntax
- res = atanh(x)
Input argument
- x - a numeric value
Output argument
- res - a numeric value
Description
acos computes the inverse hyperbolic tangent.
Example
A = [1+2i, 2, -3];
res = atanh(A)
See also
tanh.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
cart2pol
Transforms Cartesian coordinates to polar or cylindrical.
Syntax
- [theta, rho] = cart2pol(x, y)
- [theta, rho, z] = cart2pol(x, y, z)
Input argument
- x - a numeric value (double or single real): Cartesian coordinates
- y - a numeric value (double or single real): Cartesian coordinates
- z - a numeric value (double or single real): Cartesian coordinates
Output argument
- theta - a numeric value: Angular coordinate.
- rho - a numeric value: Radial coordinate.
- z - a numeric value: Elevation coordinate.
Description
cart2pol transforms Cartesian coordinates to polar or cylindrical.
Examples
x = [5 3.5355 0 -10];
y = [0 3.5355 10 0];
[theta, rho] = cart2pol(x, y)
x = [1 2.1213 0 -5];
y = [0 2.1213 4 0];
z = [7 8 9 10];
[theta, rho, el] = cart2pol(x, y, z)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
cart2sph
Transforms Cartesian to spherical coordinates.
Syntax
- [azimuth, elevation, r] = cart2sph(x, y, z)
Input argument
- x - a numeric value (double or single real): Cartesian coordinates
- y - a numeric value (double or single real): Cartesian coordinates
- z - a numeric value (double or single real): Cartesian coordinates
Output argument
- azimuth - a numeric value: Azimuth angle.
- elevation - a numeric value: Elevation angle.
- r - a numeric value: Radius.
Description
cart2sph transforms Cartesian to spherical coordinates.
Example
x = [1 1 1 1; -1 -1 -1 -1];
y = [1 1 -1 -1; 1 1 -1 -1];
z = [1 -1 1 -1; 1 -1 1 -1];
[az, el, r] = cart2sph(x, y, z)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
cos
Computes the cosine in radians for each element of x.
Syntax
- res = cos(x)
Input argument
- x - a numeric value
Output argument
- res - a numeric value
Description
cos computes the cosine in radians for each element of x.
Example
A = eye(3, 3);
res = cos(A)
See also
acos.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
cosd
Computes the cosine in degree for each element of x.
Syntax
- res = cosd(x)
Input argument
- x - a numeric value
Output argument
- res - a numeric value
Description
cosd computes the cosine in degree for each element of x.
Example
A = [0 30 45 60 90 360];;
res = cosd(A)
See also
cos.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
cosh
Computes the hyperbolic cosine in radians for each element of x.
Syntax
- res = cosh(x)
Input argument
- x - a numeric value
Output argument
- res - a numeric value
Description
cosh computes the hyperbolic cosine in radians for each element of x.
Example
A = eye(3, 3);
res = cosh(A)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
cosm
Computes the matrix cosine of a square matrix.
Syntax
- res = cosm(x)
Input argument
- x - a numeric value: scalar or square matrix
Output argument
- res - a numeric value: a square matrix
Description
cosm(x) computes the matrix cosine of x.
Example
A = eye(3, 3);
res = cosm(A)
A = [1, 2; 3, 4];
res = cosm(A)
See also
cos.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
cospi
Computes cos(X * pi) accurately.
Syntax
- res = cospi(x)
Input argument
- x - a numeric value
Output argument
- res - a numeric value
Description
res = cospi(x) computes cos(x * pi) accurately.
For integers, cospi(x) is +1 or -1.
For odd integers, cospi(x / 2) is exactly zero.
Example
x = [0, 1/2, 1, 3/2, 2];
r = cos(x * pi)
res = cospi(x)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
cot
Cotangent of angle in radians
Syntax
- res = cot(x)
Input argument
- x - a numeric value
Output argument
- res - a numeric value
Description
acot computes the cotangent of angle for each element of x.
Example
R = cot([-i pi+i*pi/2 -1+i*4])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
cotd
Cotangent of argument in degrees
Syntax
- res = cotd(x)
Input argument
- x - a numeric value
Output argument
- res - a numeric value
Description
cotd computes the cotangent of argument in degrees for each element of x.
Example
R = cotd(35 + 5i)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
coth
Hyperbolic cotangent.
Syntax
- res = coth(x)
Input argument
- x - a numeric value
Output argument
- res - a numeric value
Description
csch computes the hyperbolic cotangent for each element of x.
Example
X = [3*pi, 2*pi, pi, 0];
R = coth(X)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
csc
Cosecant of input angle in radians.
Syntax
- res = csc(x)
Input argument
- x - a numeric value
Output argument
- res - a numeric value
Description
csc computes the cosecant of argument in radians for each element of x.
Example
R = csc(-pi+0.01:0.01:-0.01)
See also
cscd.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
cscd
Cosecant of argument in degrees.
Syntax
- res = cscd(x)
Input argument
- x - a numeric value
Output argument
- res - a numeric value
Description
cscd computes the cosecant of argument in degrees for each element of x.
Example
R = cscd([35+i 15+2i 10+3i])
See also
csc.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
csch
Hyperbolic cosecant.
Syntax
- res = csch(x)
Input argument
- x - a numeric value
Output argument
- res - a numeric value
Description
csch computes the hyperbolic cosecant for each element of x.
Example
X = [3*pi, 2*pi, pi, 0];
R = csch(X)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
deg2rad
Convert angle from degrees to radians.
Syntax
- r = deg2rad(d)
Input argument
- d - a numeric value (double or single)
Output argument
- r - a numeric value
Description
d = deg2rad(r) converts angle units from degrees to radians for each element of r.
Example
D = 64.7;
R = deg2rad(D);
radEarth = 6371;
dist = radEarth * R
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
pol2cart
Transforms polar or cylindrical coordinates to Cartesian.
Syntax
- [x, y] = pol2cart(theta, rho)
- [x, y, z] = pol2cart(theta, rho, z)
Input argument
- theta - a numeric value: Angular coordinate.
- rho - a numeric value: Radial coordinate.
- z - a numeric value: Elevation coordinate.
Output argument
- x - a numeric value (double or single real): Cartesian coordinates
- y - a numeric value (double or single real): Cartesian coordinates
- z - a numeric value (double or single real): Cartesian coordinates
Description
pol2cart transforms polar or cylindrical coordinates to Cartesian.
Example
theta = [0 pi/4 pi/2 pi];
rho = [5 5 10 10];
[x, y] = pol2cart(theta, rho)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
rad2deg
Convert angle from radians to degrees.
Syntax
- d = rad2deg(r)
Input argument
- r - a numeric value (double or single)
Output argument
- d - a numeric value
Description
d = rad2deg(r) converts angle units from radians to degrees for each element of r.
Example
dist = 7194;
radEarth = 6371;
D = rad2deg(dist / radEarth)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
sec
Secant of angle in radians.
Syntax
- res = sec(x)
Input argument
- x - a numeric value
Output argument
- res - a numeric value
Description
sec computes the secant of argument in radians for each element of x.
Example
x = -pi:0.75:pi;
R = sec(x)
See also
secd.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
secd
Secant of argument in degrees.
Syntax
- res = secd(x)
Input argument
- x - a numeric value
Output argument
- res - a numeric value
Description
secd computes the secant of argument in degrees for each element of x.
Example
R = secd([1, 10+3i, 15+2i, 35+i])
See also
sec.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
sech
Hyperbolic secant.
Syntax
- res = sech(x)
Input argument
- x - a numeric value
Output argument
- res - a numeric value
Description
sech computes the Hyperbolic secant for each element of x.
Example
X = [3*pi, 2*pi, pi, 0];
R = sech(X)
See also
cosh.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
sin
Computes the sine in radians for each element of x.
Syntax
- res = sin(x)
Input argument
- x - a numeric value
Output argument
- res - a numeric value
Description
sin computes the sine in radians for each element of x.
Example
A = eye(3, 3);
res = sin(A)
See also
asin.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
sind
Computes the sine in degree for each element of x.
Syntax
- res = sind(x)
Input argument
- x - a numeric value
Output argument
- res - a numeric value
Description
sind computes the sine in degree for each element of x.
Example
A = [0 30 45 60 90 360];
sind(A)
See also
sin.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
sinh
Computes the hyperbolic sine in radians for each element of x.
Syntax
- res = sinh(x)
Input argument
- x - a numeric value
Output argument
- res - a numeric value
Description
sinh computes the hyperbolic sine in radians for each element of x.
Example
A = eye(3, 3);
res = sinh(A)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
sinm
Computes the matrix sinus of a square matrix.
Syntax
- res = sinm(x)
Input argument
- x - a numeric value: scalar or square matrix
Output argument
- res - a numeric value: a square matrix
Description
sinm(x) computes the matrix sinus of x.
Example
A = eye(3, 3);
res = sinm(A)
A = [1, 2; 3, 4];
res = sinm(A)
See also
sin.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
sinpi
Computes sin(X * pi) accurately.
Syntax
- res = sinpi(x)
Input argument
- x - a numeric value
Output argument
- res - a numeric value
Description
res = sinpi(x) computes sin(x * pi) accurately.
For odd integers, sinpi(x / 2) is +1 or -1.
For integers, sinpi(x) is exactly zero.
Example
x = [0, 1/2, 1, 3/2, 2];
r = sin(x * pi)
res = sinpi(x)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
sph2cart
Transform spherical coordinates to Cartesian.
Syntax
- [x, y, z] = sph2cart(azimuth, elevation, r)
Input argument
- azimuth - a numeric value: Azimuth angle.
- elevation - a numeric value: Elevation angle.
- r - a numeric value: Radius.
Output argument
- x - a numeric value (double or single real): Cartesian coordinates
- y - a numeric value (double or single real): Cartesian coordinates
- z - a numeric value (double or single real): Cartesian coordinates
Description
sph2cart transforms Cartesian to spherical coordinates.
Example
azimut = [0.7854, 0.7854, -0.7854, -0.7854; 2.3562, 2.3562, -2.3562, -2.3562];
elevation = [0.6155, -0.6155, 0.6155, -0.6155; 0.6155, -0.6155, 0.6155, -0.6155];
radius = 1.7321 * ones(2, 4);
[x, y, z] = sph2cart(azimut, elevation, radius)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
tan
Computes the tangent in radians for each element of x.
Syntax
- res = tan(x)
Input argument
- x - a numeric value
Output argument
- res - a numeric value
Description
tan computes the tangent in radians for each element of x.
Example
A = eye(3, 3);
res = tan(A)
See also
atan.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
tand
Computes the tangent in degree for each element of x.
Syntax
- res = tand(x)
Input argument
- x - a numeric value
Output argument
- res - a numeric value
Description
tand computes the tangent in degree for each element of x.
Example
A = [0 30 45 60 90 360];
res = tand(A)
See also
tan.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
tanh
Computes the hyperbolic tangent in radians for each element of x.
Syntax
- res = tanh(x)
Input argument
- x - a numeric value
Output argument
- res - a numeric value
Description
tanhcomputes the hyperbolic tangent in radians for each element of x.
Example
A = eye(3, 3);
res = tanh(A)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
tanm
Computes the matrix tangent of a square matrix.
Syntax
- res = tanm(x)
Input argument
- x - a numeric value: scalar or square matrix
Output argument
- res - a numeric value: a square matrix
Description
tanm(x) computes the matrix tangent of x.
Example
A = eye(3, 3);
res = tanm(A)
A = [1, 2; 3, 4];
res = tanm(A)
See also
tan.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
Linear algebra
Linear algebra
Description
linear algebra functions
- balance - Diagonal scaling to improve eigenvalue accuracy.
- bandwidth - Lower and upper matrix bandwidth.
- chol - Cholesky factorization.
- cond - Condition number for inversion.
- condeig - Condition number with respect to eigenvalues.
- det - Matrix determinant.
- diff - Differences and approximate derivatives.
- eig - Eigenvalues and eigenvectors.
- expm - Computes the matrix exponential of a square matrix.
- gradient - Numerical gradient.
- inv - Matrix inverse.
- isbanded - Determine if matrix is within specific bandwidth.
- ishermitian - Computes if matrix is hermitian or skew-hermitian.
- issymmetric - Computes if matrix is symmetric.
- kron - Kronecker tensor product.
- logm - Computes the matrix logarithm of a square matrix.
- lu - LU matrix factorization.
- orth - Range space of a matrix.
- planerot - Givens plane rotation.
- rank - Rank of matrix.
- rcond - Inverse condition number.
- rref - Gauss-Jordan elimination.
- rsf2csf - Convert real Schur form to complex Schur form.
- schur - Schur decomposition.
- sqrtm - Computes the matrix square root of a square matrix.
- subspace - Angle between two subspaces.
- svd - Singular Value Decomposition.
- trace - Matrix trace.
- vecnorm - Vector-wise norm.
balance
Diagonal scaling to improve eigenvalue accuracy.
Syntax
- B = balance(A)
- B = balance(A,'noperm')
- [T, B] = balance(A)
- [S, P, B] = balance(A)
Input argument
- A - a matrix: square, finite single or double.
Output argument
- B - balanced matrix.
- T - similarity transformation: Rearrange the elements of a diagonal matrix containing integer powers of two in order to minimize the impact of roundoff errors.
- S - scaling vector
- P - permutation vector
Description
B = balance(A) returns the balanced matrix B.
B = balance(A, 'noperm') scales A without permuting its rows and columns.
Used function(s)
LAPACKE_dgebal, LAPACKE_sgebal, LAPACKE_zgebal, LAPACKE_cgebal
Example
A = [10 1000 100000; .1 10 1000; .001 .1 10]
F = balance(A)
See also
eig.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
bandwidth
Lower and upper matrix bandwidth.
Syntax
- [lower, upper] = bandwidth(A)
- R = bandwidth(A, type)
Input argument
- A - Input matrix
- type - 'upper' or 'lower'
Output argument
- lower, upper - lower bandwidth,
- R - lower or upper bandwidth.
Description
[lower, upper] = bandwidth(A) returns lower and upper bandwidths of matrix A.
Example
M = [10 -20 40; -50 20 0; 10 0 30]
[lower, upper] = bandwidth(M)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
chol
Cholesky factorization.
Syntax
- F = chol(A)
Input argument
- A - a matrix: square and symmetric positive definite.
Output argument
- F - Cholesky factor.
Description
F = chol(A) factorizes symmetric positive definite matrix A into an upper triangular F that satisfies A = F' * F.
Example
A = [10 0 10; 0 20 0; 10 0 30];
F = chol(A)
See also
det.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
cond
Condition number for inversion.
Syntax
- c = rcond(A, p)
Input argument
- A - a numeric value: square or rectangular (double or single)
- p - norm type: Inf, 'fro', 1, 2 (default)
Output argument
- c - a numeric value: a scalar.
Description
c = cond(A) returns the 2-norm condition number for inversion.
c = cond(A, p) returns the p-norm condition number, where p can be 1, 2, Inf, or 'fro'.
Example
X = rand(10, 10);
r = cond(X)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
condeig
Condition number with respect to eigenvalues.
Syntax
- C = condeig(A)
- [V, D, S] = condeig(A)
Input argument
- A - Input matrix
Output argument
- C - a vector of condition numbers for the eigenvalues of A.
Description
C = condeig(A) returns a vector of condition numbers for the eigenvalues of A.
Example
A = [10, 20; 30, 40];
S = condeig(A)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
det
Matrix determinant.
Syntax
- res = det(x)
Input argument
- x - a numeric value: scalar or square matrix (double or single)
Output argument
- res - real or complex number (double or single), the determinant base 10.
Description
res = det(x) returns the determinant of square matrix x.
Example
A = [10 -20 40; -50 20 0; 10 0 30]
D = det(A)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
diff
Differences and approximate derivatives.
Syntax
- Y = diff(X)
- Y = diff(X, n)
- Y = diff(X, n, dim)
Input argument
- X - vector or matrix (real or single)
- n - difference order: positive integer scalar or []
- dim - dimension: positive integer scalar
Output argument
- Y - difference array: vector or matrix.
Description
If X is a vector of length n, result of diff(X) is a vector of first differences X(2) - X(1), ..., X(n) - X(n-1).
If X is a matrix, result of diff(X) is a matrix of column differences along the first non-singleton dimension.
Example
h = .01; x = 0:h:pi;
X = sin(x.^2);
R = diff(X)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
eig
Eigenvalues and eigenvectors.
Syntax
- e = eig(A)
- [V, D] = eig(A)
- e = eig(A, balanceOption)
- [V, D] = eig(A, balanceOption)
- e = eig(A, B)
- [V, D] = eig(A, B)
- e = eig(A, B, balanceOption)
- [V, D] = eig(A, B, balanceOption)
Input argument
- A - a numeric value: scalar or square matrix (double or single, complex or real)
- B - a numeric value: scalar or square matrix (double or single, complex or real)
- balanceOption - a string: 'nobalance' (disable preliminary balancing) or 'balance' (default).
Output argument
- e - real or complex number (double or single), Eigenvalues (returned as column vector).
- V - real or complex number (double or single), square right eigenvectors.
- D - real or complex number (double or single), Eigenvalues (returned as diagonal matrix).
Description
eig(A) returns the Eigenvalues and eigenvectors.
eig(A, B) returns the generalized Eigenvalues and eigenvectors.
Bibliography
[1] Anderson, E., Z. Bai, C. Bischof, S. Blackford, J. Demmel, J. Dongarra, J. Du Croz, A. Greenbaum, S. Hammarling, A. McKenney, and D. Sorensen, LAPACK User's Guide (http://www.netlib.org/lapack/lug/ lapack_lug.html), Third Edition, SIAM, Philadelphia, 1999.
Examples
A = [10 -20 40; -50 20 0; 10 0 30]
e = eig(A)
[V, D] = eig(A)
A = [1/sqrt(2) 0; 0 1];
B = [0 1; -1/sqrt(2) 0];
[V, D] = eig(A, B)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
expm
Computes the matrix exponential of a square matrix.
Syntax
- res = expm(x)
Input argument
- x - a numeric value: scalar or square matrix (double or single)
Output argument
- res - a numeric value: a square matrix
Description
expm(x) computes the matrix exponential of x.
The computation is performed by first block-diagonalizing x and then applying a Pade approximation on each block.
Example
A = eye(3, 3);
res = expm(A)
res = expm(A+i)
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
gradient
Numerical gradient.
Syntax
- FX = gradient(F)
- [FX, FY] = gradient(F)
- [FX, FY, FZ, ..., FN] = gradient(F)
- [...] = gradient(F, h)
- [...] = gradient(F, hx, hy, ... , hN)
Input argument
- F - Input array: vector, matrix or multidimensional array.
- h - Uniform spacing between points: scalar or 1 (default).
- hx, hy, ..., hN - Spacing between points: vector, scalar or 1 (default).
Output argument
- FX, FY, FZ, ..., FN - Numerical gradients: array.
Description
gradient(F) calculates the one-dimensional numerical gradient of the vector or matrix F.
The output FX represents the differences in the x (horizontal) direction, corresponding to ∂F/∂x.
It assumes that the spacing between points is 1.
gradient(F, h) allows for specifying a uniform spacing h between points in each direction.
This uniform spacing can also be individually specified for each dimension of F using gradient(F, hx, hy, ..., hN).
Example
[X, Y] = meshgrid(-2:0.2:2);
Z = X .* exp(-X.^2 - Y.^2);
[U, V] = gradient(Z, 0.2, 0.2);
See also
diff.
History
Version | Description |
---|---|
1.3.0 | initial version |
Author
Allan CORNET
inv
Matrix inverse.
Syntax
- res = inv(x)
Input argument
- x - a numeric value: scalar or square matrix (double or single)
Output argument
- res - a numeric value: a square matrix
Description
inv(x) computes the matrix inverse of x.
Example
X = rand(10, 10);
Y = inv(X);
Y * X
See also
expm.
History
Version | Description |
---|---|
1.0.0 | initial version |
1.4.0 | warning about 'Matrix is singular to working precision' |
Author
Allan CORNET
isbanded
Determine if matrix is within specific bandwidth.
Syntax
- tf = isbanded(A, lower, upper)
Input argument
- A - Input matrix
- lower, upper - lower bandwidth,
Output argument
- tf - logical
Description
tf = isbanded(A, lower, upper) returns true if matrix A is within the specified lower bandwidth, lower, and upper bandwidth, upper.
Example
M = [1 0 0 0 0; 2 1 0 0 0; 3 2 1 0 0]
TF = isbanded(M, 2, 0)
TF = isbanded(M, 2, 1)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
ishermitian
Computes if matrix is hermitian or skew-hermitian.
Syntax
- res = ishermitian(x)
- res = ishermitian(x, 'skew')
- res = ishermitian(x, 'nonskew')
Input argument
- x - a numeric value: scalar or matrix (double or single, integers, logical).
Output argument
- res - a logical.
Description
ishermitian(x) computes if matrix is hermitian or skew-hermitian.
A matrix is skew-hermitian if the complex conjugate transpose of the matrix is equal to the negative of the original matrix.
Example
ishermitian([1 0 1i; 0 1 0; -1i 0 1])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
issymmetric
Computes if matrix is symmetric.
Syntax
- res = issymmetric(x)
- res = issymmetric(x, 'skew')
- res = issymmetric(x, 'nonskew')
- res = issymmetric(x, tol)
Input argument
- x - a numeric value: scalar or matrix (double or single, integers, logical).
- tol - a numeric value: finite and >= 0.
Output argument
- res - a logical.
Description
issymmetric(x) computes if matrix is symmetric.
With 'nonskew' argument, x square matrix, x is symmetric if it is equal to its nonconjugate transpose, x = x.'
With 'skew' argument, x square matrix, x is symmetric if it is equal to its nonconjugate transpose, x = -x.'
Example
issymmetric([1, 2; 2, 1])
issymmetric([1, 2.1; 2, 1.1], 0.2)
A = [0 1 -2 5; -1 0 3 -4; 2 -3 0 6; -5 4 -6 0];
issymmetric(A, 'skew')
issymmetric(A, 'nonskew')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
kron
Kronecker tensor product.
Syntax
- K = kron(A, B)
Input argument
- A - a matrix: scalars, vectors or matrices.
- B - a matrix: scalars, vectors or matrices.
Output argument
- K - result: Kronecker Tensor Product.
Description
K = kron(A, B) computes the Kronecker tensor product of matrices A and B.
Bibliography
https://en.wikipedia.org/wiki/Kronecker_product
Example
A = [1, 2; 3, 4];
B = [0, 5; 6, 7];
K = kron(A, B)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
logm
Computes the matrix logarithm of a square matrix.
Syntax
- res = logm(x)
Input argument
- x - a numeric value: scalar or square matrix (double or single)
Output argument
- res - a numeric value: a square matrix
Description
expm(x) computes the matrix logarithm of x.
The computation is performed by first block-diagonalizing x and then applying a Pade approximation on each block.
Example
A = eye(3, 3);
res = logm(A)
res = logm(A+i)
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
lu
LU matrix factorization.
Syntax
- [L, U] = lu(A)
- [L, U, P] = lu(A)
Input argument
- A - a matrix: square, finite single or double.
Output argument
- L - Lower triangular factor: matrix (same type A)
- U - Upper triangular factor: matrix (same type A).
- P - Row permutation: matrix (same type A).
Description
[L, U] = lu(A) function decomposes a full matrix A into two matrices: an upper triangular matrix U and a permuted lower triangular matrix L.
This factorization satisfies the equation A = L * U.
[L, U, P] = lu(A) function, when used with three output arguments, provides a permutation matrix P in addition to the unit lower triangular matrix L and the upper triangular matrix U.
This factorization is expressed as A = P'LU, where L is unit lower triangular, and U is upper triangular.
Used function(s)
LAPACKE_dgetrf, LAPACKE_sgetrf, LAPACKE_zgetrf, LAPACKE_cgetrf
Examples
A = magic(5)
[L, U] = lu(A)
L * U
A = magic(5)
[L, U, P] = lu(A);
subplot(1, 2, 1)
spy(L)
title(_('L factor'))
subplot(1, 2, 2)
spy(U)
title(_('U factor'))
See also
cond.
History
Version | Description |
---|---|
1.1.0 | initial version |
Author
Allan CORNET
orth
Range space of a matrix.
Syntax
- O = orth(A)
- O = orth(A, tol)
Input argument
- A - Input matrix
- tol - a numeric value: scalar, singular value tolerance
Output argument
- O - real or complex number (double or single).
Description
O = orth(A) returns an orthonormal basis for the range of A.
Example
M = [10 -20 40; -50 20 0; 10 0 30]
O = orth(M)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
planerot
Givens plane rotation.
Syntax
- [G, Y] = planerot(X)
Input argument
- X - two-element column vector.
Output argument
- G - 2 by 2 orthogonal matrix.
- Y - Y = G * X with Y(2) = 0.
Description
[G, Y] = planerot(X) computes the Givens rotation matrix for the two-element column vector X.
Example
X = [4; 5];
[G, X] = planerot(X)
See also
norm.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
rank
Rank of matrix.
Syntax
- r = rank(A)
- r = rank(A, tol)
Input argument
- A - matrix: double or single
- tol - tolerance
Output argument
- r - a numeric value: a scalar.
Description
rank(A) returns the number of linearly independent columns in a matrix (rank of the matrix).
Example
X = rand(10, 10);
r = rank(X)
See also
svd.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
rcond
Inverse condition number.
Syntax
- res = rcond(x)
Input argument
- x - a numeric value: scalar or square matrix (double or single)
Output argument
- res - a numeric value: a scalar.
Description
rcond(x) computes the reciprocal of the condition of x in the 1-norm.
Example
X = rand(10, 10);
r = rcond(X);
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
rref
Gauss-Jordan elimination.
Syntax
- R = rref(A)
- R = rref(A, tol)
- [R, p] = rref(A)
- [R, p] = rref(A, tol)
Input argument
- A - input matrix (double or single)
- tol - tolerance: scalar or max(rows, cols) _ eps(class(A)) _ norm(A, inf) (default)
Output argument
- R - a matrix: reduced row echelon form of A.
- p - a vector: nonzero pivot columns.
Description
R = rref(A) returns the reduced row echelon form of A.
[R, p] = rref(A) returns also the nonzero pivots p.
Bibliography
https://en.wikipedia.org/wiki/Gaussian_elimination
Example
A = [magic(4), eye(4)]
[R, p] = rref(A)
See also
rank.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
rsf2csf
Convert real Schur form to complex Schur form.
Syntax
- [Uc, Tc] = rsf2csf(U, T)
Input argument
- U - unitary matrix (double or single, real or complex)
- T - schur form (double or single, real or complex)
Output argument
- Uc - transformed unitary matrix
- Tc - transformed schur form
Description
[Uc, Tc] = rsf2csf(U, T) transforms the outputs of [U, T] = schur(X) for real matrices X from real Schur form to complex Schur form.
Example
X = [1, 1, 1, 3;
1, 2, 1, 1;
1, 1, 3, 1;
-2, 1, 1, 4];
[U, T] = schur(X)
[Uc, Tc] = rsf2csf(U, T)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
schur
Schur decomposition.
Syntax
- T = schur(M)
- T = schur(M, 'real')
- T = schur(M, 'complex')
- [U, T] = schur(M)
- [U, T] = schur(M, 'complex')
- [U, T] = schur(M, 'real')
Input argument
- M - a numeric value: scalar or square matrix (double or single)
Output argument
- U - unitary matrix
- T - upper triangular matrix
Description
schur(M) computes the schur decomposition.
With the flag 'complex', the complex schur form is upper triangular with the eigenvalues of M on the diagonal.
If A is real, the real schur form is returned.
With the flag 'real', the real schur form has the real eigenvalues on the diagonal and the complex eigenvalues in 2-by-2 blocks on the diagonal.
Example
X = [1 2; 3 4];
[U, T] = schur(X)
[U, T] = schur(X * i, 'complex')
[U, T] = schur(X * i, 'real')
See also
eig.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
sqrtm
Computes the matrix square root of a square matrix.
Syntax
- res = sqrtm(x)
Input argument
- x - a numeric value: scalar or square matrix (double or single)
Output argument
- res - a numeric value: a square matrix
Description
expm(x) computes the matrix square root of x.
Example
A = eye(3, 3);
res = sqrtm(A)
res = sqrtm(A+i)
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
subspace
Angle between two subspaces.
Syntax
- T = subspace(A, B)
Input argument
- A - vector or matrix (real or single)
- B - vector or matrix (real or single)
Output argument
- T - scalar: angle.
Description
T = subspace(A, B) finds the angle between two subspaces specified by the columns of A and B.
Example
M = [1 1 1 1 1 1 1 1;
1 -1 1 -1 1 -1 1 -1;
1 1 -1 -1 1 1 -1 -1;
1 -1 -1 1 1 -1 -1 1;
1 1 1 1 -1 -1 -1 -1;
1 -1 1 -1 -1 1 -1 1;
1 1 -1 -1 -1 -1 1 1;
1 -1 -1 1 -1 1 1 -1];
A = M(:, 2:4);
B = M(:, 5:8);
R = subspace(A, B)
See also
orth.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
svd
Singular Value Decomposition.
Syntax
- s = svd(M)
- [U, S, V] = svd(M)
- [U, S, V] = svd(M, 0)
- [U, S, V] = svd(M, 'econ')
Input argument
- M - a numeric value: matrix (double or single)
Output argument
- s - real vector (singular values) by descending order.
- U - left singular values.
- S - real diagonal matrix (singular values)
- V - right singular values.
Description
[U, S, V] = svd(M) produces a diagonal matrix S of the same dimension as M and with nonnegative diagonal elements in decreasing order, and unitary matrices U and V so that X = U*S*V'.
[U, S, V] = svd(M, 0) produces the 'economy size' decomposition. If M is m-by-n with m > n then only the first n columns of U are computed and S is n-by-n.
[U, S, V] = svd(M,0) produces a different economy-size decomposition of m-by-n matrix M. If m > n then svd(M, 0) is equivalent to svd(M,'econ'). If m <= n then svd(M, 0) is equivalent to svd(M).
Example
X = eye(3, 3);
s = svd(X)
[U, S, V] = svd(X)
See also
eig.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
trace
Matrix trace.
Syntax
- res = trace(x)
Input argument
- x - a numeric value: scalar or matrix (double or single)
Output argument
- res - a numeric value: a scalar
Description
trace(x) computes the trace of x, the sum of the elements along the main diagonal.
Example
X = [1 0; 0 3];
Y = trace(X)
See also
eig.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
vecnorm
Vector-wise norm.
Syntax
- N = vecnorm(A)
- N = vecnorm(A, p)
- N = vecnorm(A, p, dim)
Input argument
- A - vector, matrix or multidimensional array
- p - Norm type: 2 (default), a positive scalar, or Inf.
- dim - positive integer scalar
Output argument
- n - norm: scalar or vector
Description
vecnorm computes the 2-norm or Euclidean norm of the input array A
If A is a vector, vecnorm returns the norm of the vector.
If A is a matrix, vecnorm returns the norm of each column.
For multidimensional arrays, vecnorm returns the norm along the first array dimension whose size does not equal 1.
To compute the generalized vector p-norm, you can use the syntax N = vecnorm(A, p).
To operate along a specific dimension dim, the function can be called as N = vecnorm(A, p, dim).
In this case, the size of the specified dimension reduces to 1, while the sizes of all other dimensions remain unchanged.
Example
A = [1, 2, 3; 4, 5, 6; 7, 8, 9];
n = vecnorm(A)
n = vecnorm(A, 2, 2)
n = vecnorm(A, 1)
See also
norm.
History
Version | Description |
---|---|
1.3.0 | initial version |
Author
Allan CORNET
Statistics
Statistics
Description
statistics functions
- corrcoef - Correlation coefficients
- cov - Covariance
- mean - Mean of array elements.
- normpdf - Normal probability density function
- var - Variance
corrcoef
Correlation coefficients
Syntax
- R = corrcoef(M)
Input argument
- M - a vector or matrix
Output argument
- R - Correlation coefficients of M.
Description
R = corrcoef(M) returns the matrix of correlation coefficients for M, where the columns of M represent random variables and the rows represent observations.
Example
M = [4 -7 3; 1 4 -2; 10 7 9];
R = corrcoef(M)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
cov
Covariance
Syntax
- C = cov(M)
Input argument
- M - a vector or matrix
Output argument
- V - Covariance of M.
Description
C = cov(M) returns the covariance.
Example
M = [4 -7 3; 1 4 -2; 10 7 9];
C = cov(M)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
mean
Mean of array elements.
Syntax
- R = mean(M)
- R = mean(M, d)
- R = mean(M, 'all')
- R = mean(M, d, t)
- R = mean(M, 'all', t)
- R = mean(M, d, t, f)
- R = mean(M, 'all', t, f)
Input argument
- M - an array of double, single, integers, ...
- d - dimension to operate along: positive integer scalar.
- t - a string: 'default', 'double' or 'native'.
- f - a string: 'includenan' or 'omitnan'.
Output argument
- R - Mean of array elements.
Description
R = mean(M) returns the mean of the array elements of M.
Example
M = uint8([10:30:70;20:30:80;30:30:90]);
R = mean(M, 'native')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
normpdf
Normal probability density function
Syntax
- y = normpdf(x)
- y = normpdf(x, mu)
- y = normpdf(x, mu, sigma)
Input argument
- x - scalar value or array: Values at which to evaluate pdf.
- mu - scalar value, 0 (default) or array: Mean.
- sigma - positive scalar value, 1 (default) or array of positive values: Standard deviation.
Output argument
- y - scalar value or array: pdf values.
Description
y = normpdf(x) calculates the probability density function (pdf) of the standard normal distribution at the given values in x.
y = normpdf(x, mu) computes the pdf of the normal distribution with a mean of mu and a standard deviation of 1, evaluated at the provided values in x.
y = normpdf(x, mu, sigma) determines the pdf of the normal distribution with a mean of mu and a standard deviation of sigma, evaluated at the specified values in x.
Bibliography
Evans, M., N. Hastings, and B. Peacock. Statistical Distributions. 2nd ed. Hoboken, NJ: John Wiley and Sons, Inc., 1993.
Example
x = [-0.2, -0.1, 0, 0.1, 0.2];
R = normpdf(x);
x = [-0.2, -0.1, 0, 0.1, 0.2];
R = normpdf(x, 2, 1);
R = normpdf(0, [-0.2, -0.1, 0, 0.1, 0.2], 1);
See also
mean.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
var
Variance
Syntax
- V = var(M)
Input argument
- M - a vector or matrix
Output argument
- V - Variance of M.
Description
V = var(M) returns the variance of the elements of M along the first array dimension whose size does not equal 1.
Example
M = [4 -7 3; 1 4 -2; 10 7 9];
V = var(M)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
Polynomials
Polynomials
Description
Polynomial functions
- deconv - Deconvolution and polynomial division.
- poly - Polynomial with specified roots or characteristic polynomial.
- polyder - Polynomial differentiation.
- polyfit - Polynomial curve fitting.
- polyint - Polynomial integration.
- polyval - Polynomial evaluation.
- polyvalm - Matrix polynomial evaluation.
- roots - Find polynomial roots.
deconv
Deconvolution and polynomial division.
Syntax
- [q, r] = deconv(b, a)
Input argument
- a - row or column vectors
- b - row or column vectors
Output argument
- q - quotient: row or column vector
- r - remainder: row or column vector
Description
[q, r] = deconv(b, a) performs deconvolution on vector b by vector a using long division.
It returns the quotient q and remainder r such that b = conv(a, q) + r.
In the context of polynomial coefficients, deconvolving vectors b and a is akin to dividing the polynomial represented by b by the polynomial represented by a.
Example
b = [1; 2; -1]; % Dividend (x^2 + 2x - 1)
a = [1; 1]; % Divisor (x + 1)
[q, r] = deconv(b, a)
See also
History
Version | Description |
---|---|
1.3.0 | initial version |
Author
Allan CORNET
poly
Polynomial with specified roots or characteristic polynomial.
Syntax
- p = poly(r)
- p = poly(A)
Input argument
- r - vector: polynomial roots
- A - matrix: input matrix
Output argument
- p - row vector: polynomial coefficients
Description
If A is a square matrix, p = poly(A) computes an n+1 element row vector. This result is composed the coefficients of the characteristic polynomial.
If r is a vector, p = poly(r) computes a row vector. This result is composed the coefficients of the polynomial roots of which are the elements of r.
Example
A = [1 2 3;
4 5 6;
7 8 1];
p = poly(A)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
polyder
Polynomial differentiation.
Syntax
- k = polyder(p)
- k = polyder(a, b)
- [q, d] = polyder(a, b)
Input argument
- p - vector: polynomial coefficients
- a - row vector: polynomial coefficients
- b - row vector: polynomial coefficients
Output argument
- k - row vector: differentiated polynomial coefficients
- q - row vector: numerator polynomial
- d - row vector: denominator polynomial
Description
k = polyder(p) return the coefficients of the derivative of the polynomial whose coefficients are given by the vector p.
k = polyder(a, b) returns the derivative of the product of the polynomials a and b.
[q, d] = polyder(a, b) returns the derivative of the quotient of the polynomials a and b.
Example
p = [30 0 -20 0 10 50];
q = polyder(p)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
polyfit
Polynomial curve fitting.
Syntax
- p = polyfit(x, y, n)
Input argument
- x - vector: query points
- y - vector: fitted values at query points
- n - positive scalar: degree of polynomial fit
Output argument
- p - vector: Least-squares fit polynomial coefficients
Description
p = polyfit(x, y, n) returns the coefficients for a polynomial p(x) of degree n that is a best fit for the data in y.
Example
x = linspace(0, 8 * pi, 15);
y = sin(x);
p = polyfit(x, y, 7)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
polyint
Polynomial integration.
Syntax
- q = polyint(p, k)
- q = polyint(p)
Input argument
- p - vector: polynomial coefficients
- k - numeric scalr: constant of integration
Output argument
- q - row vector: integrated polynomial coefficients
Description
polyint returns the integral of the polynomial represented by the coefficients in p using a constant of integration k (0 by default).
Example
p = [10, 0, -10, 0, 0, 10];
v = [10, 0, 10];
k = 3;
q = polyint(conv(p,v),k)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
polyval
Polynomial evaluation.
Syntax
- y = polyval(p, x)
Input argument
- p - vector: polynomial coefficients
- x - query points
Output argument
- y - vector: Function values
Description
polyval evaluates polynomial at several points.
Example
p = [3 2 1];
x = [5 7 9];
R = polyval(p, x)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
polyvalm
Matrix polynomial evaluation.
Syntax
- Y = polyvalm(p, X)
Input argument
- p - vector: polynomial coefficients
- X - square matrix: input matrix
Output argument
- Y - row vector: Output polynomial coefficients
Description
polyvalm evaluates matrix polynomial.
Example
R = polyvalm ([1, 2, 3, 4], [3, -4, 1; -2, 0, 2; -1, 4, -3])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
roots
Find polynomial roots.
Syntax
- r = roots(p)
Input argument
- p - vector: polynomial coefficients
Output argument
- r - roots
Description
r = roots(c) finds the roots of the polynomial c. r is a column vector.
This function uses the companion matrix of the polynomial to find the roots.
Example
p = [1 0 0 0 -1];
r = roots(p)
See also
poly.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
Signal Processing
Signal Processing
Description
Functions to analyze sampled signals.
- bartlett - Bartlett window.
- blackman - Blackman window.
- db2mag - Convert a gain in decibels (dB) to a magnitude.
- db2pow - Convert a gain in decibels (dB) to power.
- filter2 - 2-D digital filter.
- hamming - Hamming window.
- hann - Hann window.
- mag2db - Convert a magnitude to decibels (dB).
- pow2db - Convert power to decibel.
- sinc - Sinc function.
- xcorr2 - 2-D cross-correlation.
- zp2tf - Zero-pole to transfer function conversion.
bartlett
Bartlett window.
Syntax
- c = bartlett(m)
Input argument
- m - positive integer: window length
Output argument
- c - column vector
Description
c = bartlett(m) an L-point symmetric Bartlett window.
Example
c = bartlett(8)
Bibliography
Oppenheim, Alan V., Ronald W. Schafer, and John R. Buck. Discrete-Time Signal Processing. Upper Saddle River, NJ: Prentice Hall, 1999, pp. 468–471.
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
blackman
Blackman window.
Syntax
- c = blackman(m)
- c = blackman(m, opt)
Input argument
- m - positive integer: window length
- opt - string: 'symetric' (default) or 'periodic'
Output argument
- c - column vector
Description
c = blackman(m) computes coefficients of a Blackman window of length m.
Example
c = blackman(8)
c = blackman(8, 'periodic')
Bibliography
Oppenheim, Alan V., Ronald W. Schafer, and John R. Buck. Discrete-Time Signal Processing. Upper Saddle River, NJ: Prentice Hall, 1999, pp. 468–471.
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
db2mag
Convert a gain in decibels (dB) to a magnitude.
Syntax
- mag = db2mag(db)
Input argument
- db - input array: scalar, vector or matrix.
Output argument
- mag - corresponding magnitude
Description
mag = db2mag(db) returns corresponding magnitude.
Example
mag = db2mag([0, -20])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
db2pow
Convert a gain in decibels (dB) to power.
Syntax
- pow = db2pow(db)
Input argument
- db - input array: scalar, vector or matrix.
Output argument
- pow - corresponding power
Description
pow = db2pow(db) returns corresponding power.
Example
pow = db2pow([0, -20])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
filter2
2-D digital filter.
Syntax
- Y = filter2(H, X)
- Y = filter2(H, X, shape)
Input argument
- H - coefficients of rational transfer function.
- X - input data.
- shape - 'same' (default), 'valid' or 'full'.
Output argument
- Y - result: 2-D digital filter.
Description
Y = filter2(H, X) applies a finite impulse response filter to a matrix of data X according to coefficients in a matrix H.
Example
A = zeros(10);
A(3:7, 3:7) = ones(5);
H = [1 2 1; 0 0 0; -1 -2 -1];
R = filter2(H, A, 'valid')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
hamming
Hamming window.
Syntax
- c = hamming(m)
- c = hamming(m, opt)
Input argument
- m - positive integer: window length
- opt - string: 'symetric' (default) or 'periodic'
Output argument
- c - column vector
Description
c = hamming(m) computes coefficients of a Hamming window of length m.
Example
c = hamming(8)
c = hamming(8, 'periodic')
Bibliography
Oppenheim, Alan V., Ronald W. Schafer, and John R. Buck. Discrete-Time Signal Processing. Upper Saddle River, NJ: Prentice Hall, 1999.
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
hann
Hann window.
Syntax
- c = hann(m)
- c = hann(m, opt)
Input argument
- m - positive integer: window length
- opt - string: 'symetric' (default) or 'periodic'
Output argument
- c - column vector
Description
c = hann(m) computes coefficients of a Hanning window of length m.
Example
c = hann(8)
c = hann(8, 'periodic')
Bibliography
Oppenheim, Alan V., Ronald W. Schafer, and John R. Buck. Discrete-Time Signal Processing. Upper Saddle River, NJ: Prentice Hall, 1999.
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
mag2db
Convert a magnitude to decibels (dB).
Syntax
- db = mag2db(mag)
Input argument
- mag - input array: scalar, vector or matrix.
Output argument
- db - corresponding values in decibels
Description
db = mag2db(mag) returns corresponding values in decibels.
Example
DB = mag2db([1, 0.01])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
pow2db
Convert power to decibel.
Syntax
- db = pow2db(pow)
Input argument
- pow - input array: scalar, vector or matrix.
Output argument
- db - corresponding values in decibels
Description
db = pow2db(pow) returns corresponding values in decibels.
Example
DB = pow2db([1, 0.01])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
sinc
Sinc function.
Syntax
- c = sinc(m)
Input argument
- m - input array: scalar, vector or matrix.
Output argument
- c - sinc of input
Description
c = sinc(m) returns an array c whose elements are the sinc of the elements of the input: m.
Example
c = sinc(pi)
See also
sin.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
xcorr2
2-D cross-correlation.
Syntax
- C = xcorr2(A)
- C = xcorr2(A, B)
Input argument
- A - matrices
- B - matrices
Output argument
- C - 2-D cross-correlation or autocorrelation matrix
Description
xcorr2(A, B) calculates the cross-correlation between two matrices, A and B, in two dimensions, without any scaling applied.
Example
X = ones(2, 3);
H = [1 2; 3 4; 5 6];
C = xcorr2(H, X)
See also
History
Version | Description |
---|---|
1.3.0 | initial version |
Author
Allan CORNET
zp2tf
Zero-pole to transfer function conversion.
Syntax
- [NUM, DEN] = zp2tf(Z, P, K)
Input argument
- Z - Locations of zeros, organized in columns for each system output.
- P - Locations of poles, recorded as a column vector.
- K - Gains.
Output argument
- NUM - Coefficients in the numerator, organized by rows corresponding to each system output.
- DEN - Coefficients in the denominator, arranged as a row vector.
Description
[NUM, DEN] = zp2tf(Z, P, K) returns polynomial transfer function representation from zeros and poles.
Example
p = [0.5;0.45+0.5i;0.45-0.5i];
z = [-1;i;-i];
k = 1;
[n, d] = zp2tf(z, p, k)
Bibliography
zpk2tf scipy implementation (MIT)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
Subroutine Library In COntrol Theory
Subroutine Library In COntrol Theory
Description
SLICOT provides numerical algorithms for computations in systems and control theory.
- SLICOT License - About SLICOT license.
- slicot_ab01od - Staircase form for multi-input systems using orthogonal state and input transformations.
- slicot_ab04md - Discrete-time / continuous-time systems conversion by a bilinear transformation.
- slicot_ab07nd - Inverse of a given linear system.
- slicot_ab08nd - Construction of a regular pencil for a given system such that its generalized eigenvalues are invariant zeros of the system.
- slicot_ag08bd - Zeros and Kronecker structure of a descriptor system pencil.
- slicot_mb02md - Solution of Total Least-Squares problem using a SVD approach.
- slicot_mb03od - Matrix rank determination by incremental condition estimation.
- slicot_mb03pd - Matrix rank determination by incremental condition estimation (row pivoting).
- slicot_mb03rd - Reduction of a real Schur form matrix to a block-diagonal form.
- slicot_mb04gd - RQ factorization with row pivoting of a matrix.
- slicot_mb04md - Balancing a general real matrix.
- slicot_mb05od - Matrix exponential for a real matrix, with accuracy estimate.
- slicot_mc01td - Checking stability of a given real polynomial.
- slicot_sb01bd - Pole assignment for a given matrix pair (A,B).
- slicot_sb02od - Solution of continuous- or discrete-time algebraic Riccati equations (generalized Schur vectors method).
- slicot_sb03md - Solution of continuous- or discrete-time Lyapunov equations and separation estimation.
- slicot_sb03od - Solution of stable continuous- or discrete-time Lyapunov equations (Cholesky factor).
- slicot_sb04md - Solution of continuous-time Sylvester equations (Hessenberg-Schur method).
- slicot_sb04qd - Solution of discrete-time Sylvester equations (Hessenberg-Schur method).
- slicot_sb10jd - Converting a descriptor state-space system into regular state-space form.
- slicot_sg02ad - Solution of continuous- or discrete-time algebraic Riccati equations for descriptor systems.
- slicot_tb01id - Balancing a system matrix corresponding to a triplet (A, B, C).
- slicot_tg01ad - Balancing the matrices of the system pencil corresponding to a descriptor triple (A-lambda E, B, C).
SLICOT License
About SLICOT license.
Description
Note that SLICOT is licensed under GPLv2 or higher, but the bindings to the library in this nelson's module, is licensed under LGPL v3.0.
This means that code using the SLICOT library via SLICOT bindings is subject to SLICOT's licensing terms.
If you distribute a derived or combined work, i.e. a program that links to and is distributed with the SLICOT library, then that distribution falls under the terms of the GPL.
if SLICOT library is available on platform, and user chooses to use it, distribution falls under the terms of the GPL.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
slicot_ab01od
Staircase form for multi-input systems using orthogonal state and input transformations.
Syntax
- [A_OUT, B_OUT, U_OUT, V, NCONT_OUT, INDCON_OUT, KSTAIR_OUT, INFO] = slicot_ab01od(STAGES, JOBU, JOBV, A_IN, B_IN, U_IN, NCONT_IN, INDCON_IN, KSTAIR_IN, TOL)
Input argument
- STAGES - Specifies the reduction stage: 'F': Perform the forward stage only; 'B': Perform the backward stage only; 'A': Perform both (all) stages.
- JOBU - Indicates whether the user wishes to accumulate in a matrix U the state-space transformations: 'N': Do not form U; 'I': U is internally initialized to the unit matrix
- JOBV - Indicates whether the user wishes to accumulate in a matrix V the input-space transformations: 'N': Do not form V; I': V is initialized to the unit matrix and the orthogonal transformation matrix V is returned.
- A_IN - the leading N-by-N part of this array must contain the state transition matrix A to be transformed
- B_IN - the leading N-by-M part of this array must contain the input matrix B to be transformed.
- U_IN - If STAGES ~= 'B' or JOBU = 'N', then U need not be set on entry. If STAGES = 'B' and JOBU = 'I', then, on entry, the leading N-by-N part of this array must contain the transformation matrix U that reduced the pair to the orthogonal canonical form.
- NCONT_IN - The order of the controllable state-space representation. NCONT_IN is input only if STAGES = 'B'.
- INDCON_IN - The number of stairs in the staircase form (also, the controllability index of the controllable part of the system representation).
- TOL - The tolerance to be used in rank determination when transforming (A, B).
Output argument
- A_OUT - On exit, the leading N-by-N part of this array contains the transformed state transition matrix U' _ A _ U. The leading NCONT-by-NCONT part contains the upper block Hessenberg state matrix Acont in Ac, given by U' _ A _ U, of a controllable realization for the original system. The elements below the first block-subdiagonal are set to zero. If STAGES ~='F', the subdiagonal blocks of A are triangularized by RQ factorization, and the annihilated elements are explicitly zeroed.
- B_OUT - On exit with STAGES = 'F', the leading N-by-M part of this array contains the transformed input matrix U' _ B, with all elements but the first block set to zero. On exit with STAGES ~= 'F', the leading N-by-M part of this array contains the transformed input matrix U' _ B * V, with all elements but the first block set to zero and the first block in upper triangular form.
- U_OUT - if JOBU = 'I', the leading N-by-N part of this array contains the transformation matrix U that performed the specified reduction. If JOBU = 'N', the array U is not referenced and can be supplied as a dummy array.
- V - If JOBV = 'I', then the leading M-by-M part of this array contains the transformation matrix V.
- NCONT_OUT - NCONT_OUT is input only if STAGES = 'B'.
- INDCON_OUT - INDCON is input only if STAGES = 'B'.
- KSTAIR_OUT - KSTAIR is input if STAGES = 'B', and output otherwise.
- INFO - 0: successful exit; if INFO = -i, the i-th argument had an illegal value.
Description
To reduce the matrices A and B using (and optionally accumulating) state-space and input-space transformations U and V respectively, such that the pair of matrices
Ac = U' * A * U, Bc = U' * B * V
Used function(s)
AB01OD
Bibliography
http://slicot.org/objects/software/shared/doc/AB01OD.html
Example
N = 5;
M = 2;
TOL = 0.
STAGES = 'F';
JOBU = 'N';
JOBV = 'N';
A_IN = [17.0 24.0 1.0 8.0 15.0;
23.0 5.0 7.0 14.0 16.0;
4.0 6.0 13.0 20.0 22.0;
10.0 12.0 19.0 21.0 3.0;
11.0 18.0 25.0 2.0 9.0];
% SLICOT 5.0 have an error in the example.
A_IN = A_IN.';
B_IN = [ -1.0 -4.0;
4.0 9.0;
-9.0 -16.0;
16.0 25.0;
-25.0 -36.0];
U_IN = zeros(N, N);
INDCON_IN = N;
NCONT_IN = 1;
KSTAIR_IN = zeros(1,N);
[A_OUT, B_OUT, U_OUT, V, NCONT_OUT, INDCON_OUT, KSTAIR_OUT, INFO] = slicot_ab01od(STAGES, JOBU, JOBV, A_IN, B_IN, U_IN, NCONT_IN, INDCON_IN, KSTAIR_IN, TOL)
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
SLICOT Documentation
slicot_ab04md
Discrete-time / continuous-time systems conversion by a bilinear transformation.
Syntax
- [A_OUT, B_OUT, C_OUT, D_OUT, INFO] = slicot_ab04md(TYPE, ALPHA, BETA, A_IN, B_IN, C_IN, D_IN)
Input argument
- TYPE - a character: 'D' discrete or 'C' continuous time
- ALPHA, BETA - Parameters specifying the bilinear transformation. Recommended values for stable systems: ALPHA = 1, BETA = 1. ALPHA ~= 0, BETA ~= 0
- A_IN - N-by-N part of this array must contain the state matrix A of the original system.
- B_IN - N-by-M part of this array must contain the input matrix B of the original system.
- C_IN - P-by-N part of this array must contain the output matrix C of the original system.
- D_IN - P-by-M part of this array must contain the input/output matrix D for the original system.
Output argument
- A_OUT - N-by-N part of this array contains the state matrix _A of the transformed system.
- B_OUT - N-by-M part of this array contains the input matrix _B of the transformed system.
- C_OUT - the leading P-by-N part of this array contains the output matrix _C of the transformed system.
- D_OUT - P-by-M part of this array contains the input/output matrix _D of the transformed system.
- INFO - Error Indicator: = 0: successful exit; less than 0: if INFO = -i, the i-th argument had an illegal value; = 1: if the matrix (ALPHAI + A) is exactly singular; = 2: if the matrix (BETAI - A) is exactly singular.
Description
To perform a transformation on the parameters (A, B, C, D) of a system, which is equivalent to a bilinear transformation of the corresponding transfer function matrix.
Used function(s)
AB04MD
Bibliography
http://slicot.org/objects/software/shared/doc/AB04MD.html
Example
N = 2;
M = 2;
P = 2;
TYPE= 'C';
ALPHA = 1;
BETA = 1;
A = [ 1.0 0.5; 0.5 1.0];
B = [ 0.0 -1.0; 1.0 0.0];
C = [ -1.0 0.0; 0.0 1.0];
D = [ 1.0 0.0; 0.0 -1.0];
[A_OUT, B_OUT, C_OUT, D_OUT, INFO] = slicot_ab04md(TYPE, ALPHA, BETA, A, B, C, D)
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
SLICOT Documentation
slicot_ab07nd
Inverse of a given linear system.
Syntax
- [A_OUT, B_OUT, C_OUT, D_OUT, RCOND, INFO] = slicot_ab07nd(A_IN, B_IN, C_IN, D_IN)
Input argument
- A_IN - The leading N-by-N part of this array must contain the state matrix A of the original system.
- B_IN - The leading N-by-M part of this array must contain the input matrix B of the original system.
- C_IN - The leading M-by-N part of this array must contain the output matrix C of the original system.
- D_IN - The leading M-by-M part of this array must contain the feedthrough matrix D of the original system.
Output argument
- A_OUT - The leading N-by-N part of this array contains the state matrix Ai of the inverse system.
- B_OUT - The leading N-by-M part of this array contains the input matrix Bi of the inverse system.
- C_OUT - The leading M-by-N part of this array contains the output matrix Ci of the inverse system.
- D_OUT - The leading M-by-M part of this array contains the feedthrough matrix Di of the inverse system.
- RCOND - The estimated reciprocal condition number of the feedthrough matrix D of the original system.
- INFO - = 0: successful exit;
Description
To compute the inverse (Ai, Bi, Ci, Di) of a given system (A, B, C, D).
Used function(s)
AB07ND
Bibliography
http://slicot.org/objects/software/shared/doc/AB07ND.html
Example
A_IN = [1.0 2.0 0.0;
4.0 -1.0 0.0;
0.0 0.0 1.0];
B_IN = [1.0 0.0;
0.0 1.0;
1.0 0.0];
C_IN = [0.0 1.0 -1.0;
0.0 0.0 1.0];
D_IN = [4.0 0.0;
0.0 1.0];
[A_OUT, B_OUT, C_OUT, D_OUT, RCOND, INFO] = slicot_ab07nd(A_IN, B_IN, C_IN, D_IN)
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
SLICOT Documentation
slicot_ab08nd
Construction of a regular pencil for a given system such that its generalized eigenvalues are invariant zeros of the system.
Syntax
- [NU, RANK, DINFZ, NKROR, NKROL, INFZ, KRONR, KRONL, AF, BF, INFO] = slicot_ab08nd(EQUIL, N, M, P, A, B, C, D, TOL)
Input argument
- EQUIL - 'S': Perform balancing (scaling) or 'N': Do not perform balancing.
- N - The number of state variables, i.e., the order of the matrix A.
- M - The number of system inputs.
- P - The number of system outputs.
- A - The leading N-by-N part of this array must contain the state dynamics matrix A of the system.
- B - The leading N-by-M part of this array must contain the input/state matrix B of the system.
- C - The leading P-by-N part of this array must contain the state/output matrix C of the system.
- D - The leading P-by-M part of this array must contain the direct transmission matrix D of the system.
- TOL - A tolerance used in rank decisions.
Output argument
- NU - The number of (finite) invariant zeros.
- RANK - The normal rank of the transfer function matrix.
- DINFZ - The maximum degree of infinite elementary divisors.
- NKROR - The number of right Kronecker indices.
- NKROL - The number of left Kronecker indices.
- INFZ - The leading DINFZ elements of INFZ contain information on the infinite elementary divisors as follows: the system has INFZ(i) infinite elementary divisors of degree i, where i = 1,2,...,DINFZ.
- KRONR - The leading NKROR elements of this array contain the right Kronecker (column) indices.
- KRONL - The leading NKROL elements of this array contain the left Kronecker (row) indices.
- AF - The leading NU-by-NU part of this array contains the coefficient matrix A of the reduced pencil.
- BF - The leading NU-by-NU part of this array contains the coefficient matrix B of the reduced pencil.
- INFO - 0: successful exit; if INFO = -i, the i-th argument had an illegal value.
Description
To construct for a linear multivariable system described by a state-space model (A,B,C,D) a regular pencil (A - lambda*B ) which has the invariant zeros of the system as generalized eigenvalues.
The routine also computes the orders of the infinite zeros and the right and left Kronecker indices of the system (A,B,C,D).
Used function(s)
AB08ND
Bibliography
http://slicot.org/objects/software/shared/doc/AB08ND.html
Example
N = 6;
M = 2;
P = 3;
TOL = 0.0;
EQUIL = 'N';
%=============================================================================
A = [1.0 0.0 0.0 0.0 0.0 0.0;
0.0 1.0 0.0 0.0 0.0 0.0;
0.0 0.0 3.0 0.0 0.0 0.0;
0.0 0.0 0.0 -4.0 0.0 0.0;
0.0 0.0 0.0 0.0 -1.0 0.0;
0.0 0.0 0.0 0.0 0.0 3.0];
%=============================================================================
B = [0.0 -1.0;
-1.0 0.0;
1.0 -1.0;
0.0 0.0;
0.0 1.0;
-1.0 -1.0];
%=============================================================================
C = [1.0 0.0 0.0 1.0 0.0 0.0;
0.0 1.0 0.0 1.0 0.0 1.0;
0.0 0.0 1.0 0.0 0.0 1.0];
D = [0.0 0.0;
0.0 0.0;
0.0 0.0];
%=============================================================================
% Check the observability and compute the ordered set of the observability indices (call the routine with M = 0).
[NU, RANK, DINFZ, NKROR, NKROL, INFZ, KRONR, KRONL, AF, BF, INFO] = slicot_ab08nd(EQUIL, N, 0, P, A, B, C, D, TOL)
% Check the controllability and compute the ordered set of the controllability indices (call the routine with P = 0)
[NU, RANK, DINFZ, NKROR, NKROL, INFZ, KRONR, KRONL, AF, BF, INFO] = slicot_ab08nd(EQUIL, N, M, 0, A, B, C, D, TOL)
% Compute the structural invariants of the given system.
[NU, RANK, DINFZ, NKROR, NKROL, INFZ, KRONR, KRONL, AF, BF, INFO] = slicot_ab08nd(EQUIL, N, M, P, A, B, C, D, TOL)
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
SLICOT Documentation
slicot_ag08bd
Zeros and Kronecker structure of a descriptor system pencil.
Syntax
- [A_OUT, E_OUT, NFZ, NRANK, NIZ, DINFZ, NKROR, NINFE, NKROL, INFZ, KRONR, INFE, KRONL, INFO] = slicot_ag08bd(EQUIL, M, P, A_IN, E_IN, B, C, D, TOL)
Input argument
- EQUIL - = 'S': Perform balancing (scaling); = 'N': Do not perform balancing.
- M - The number of columns of matrix B.
- P - The number of rows of matrix C.
- A_IN - The leading L-by-N part of this array must contain the state dynamics matrix A of the system.
- E_IN - The leading L-by-N part of this array must contain the descriptor matrix E of the system.
- B - The leading L-by-M part of this array must contain the input/state matrix B of the system.
- C - The leading P-by-N part of this array must contain the state/output matrix C of the system.
- D - The leading P-by-M part of this array must contain the direct transmission matrix D of the system.
- TOL - A tolerance used in rank decisions to determine the effective rank, which is defined as the order of the largest leading (or trailing) triangular submatrix in the QR (or RQ) factorization with column (or row) pivoting whose estimated condition number is less than 1/TOL.
Output argument
- A_OUT - The leading NFZ-by-NFZ part of this array contains the matrix Af of the reduced pencil.
- E_OUT - The leading NFZ-by-NFZ part of this array contains the matrix Ef of the reduced pencil.
- NFZ - The number of finite zeros.
- NRANK - The normal rank of the system pencil.
- NIZ - The number of infinite zeros.
- DINFZ - The maximal multiplicity of infinite Smith zeros.
- NKROR - The number of right Kronecker indices.
- NINFE - The number of elementary infinite blocks.
- NKROL - The number of left Kronecker indices.
- INFZ - The leading DINFZ elements of INFZ contain information on the infinite elementary divisors
- KRONR - The leading NKROR elements of this array contain the right Kronecker (column) indices.
- KRONL - The leading NKROL elements of this array contain the left Kronecker (row) indices.
- INFO - = 0: successful exit;
Description
To extract from the system pencil a regular pencil Af-lambda*Ef which has the finite Smith zeros of S(lambda) as generalized eigenvalues. The routine also computes the orders of the infinite Smith zeros and determines the singular and infinite Kronecker structure of system pencil, i.e., the right and left Kronecker indices, and the multiplicities of infinite eigenvalues.
Used function(s)
AG08BD
Bibliography
http://slicot.org/objects/software/shared/doc/AG08BD.html
Example
L = 9;
N = 9;
M = 3;
P = 3;
TOL= 1.e-7;
EQUIL='N';
A_IN = [1 0 0 0 0 0 0 0 0;
0 1 0 0 0 0 0 0 0;
0 0 1 0 0 0 0 0 0;
0 0 0 1 0 0 0 0 0;
0 0 0 0 1 0 0 0 0;
0 0 0 0 0 1 0 0 0;
0 0 0 0 0 0 1 0 0;
0 0 0 0 0 0 0 1 0;
0 0 0 0 0 0 0 0 1];
E_IN = [0 0 0 0 0 0 0 0 0;
1 0 0 0 0 0 0 0 0;
0 1 0 0 0 0 0 0 0;
0 0 0 0 0 0 0 0 0;
0 0 0 1 0 0 0 0 0;
0 0 0 0 1 0 0 0 0;
0 0 0 0 0 0 0 0 0;
0 0 0 0 0 0 1 0 0;
0 0 0 0 0 0 0 1 0];
B =[-1 0 0;
0 0 0;
0 0 0;
0 -1 0;
0 0 0;
0 0 0;
0 0 -1;
0 0 0;
0 0 0];
C = [ 0 1 1 0 3 4 0 0 2;
0 1 0 0 4 0 0 2 0;
0 0 1 0 -1 4 0 -2 2];
D = [ 1 2 -2;
0 -1 -2;
0 0 0];
%=============================================================================
% default call for the fortran routine
M = 3; P = 3;
[A_OUT, E_OUT, NFZ, NRANK, NIZ, DINFZ, NKROR, NINFE, NKROL, INFZ, KRONR, INFE, KRONL, INFO] = slicot_ag08bd(EQUIL, M, P, A_IN, E_IN, B, C, D, TOL)
%=============================================================================
% Compute poles (we need tp call fortran routine with M = 0, P = 0)
M = 0; P = 0;
[A_OUT, E_OUT, NFZ, NRANK, NIZ, DINFZ, NKROR, NINFE, NKROL, INFZ, KRONR, INFE, KRONL, INFO] = slicot_ag08bd(EQUIL, M, P, A_IN, E_IN, B, C, D, TOL)
%=============================================================================
% Check the observability and compute the ordered set of the observability indices (call the routine with M = 0).
M = 0; P = 3;
[A_OUT, E_OUT, NFZ, NRANK, NIZ, DINFZ, NKROR, NINFE, NKROL, INFZ, KRONR, INFE, KRONL, INFO] = slicot_ag08bd(EQUIL, M, P, A_IN, E_IN, B, C, D, TOL)
%=============================================================================
% Check the controllability and compute the ordered set of the controllability indices (call the routine with P = 0)
M = 3; P = 0;
[A_OUT, E_OUT, NFZ, NRANK, NIZ, DINFZ, NKROR, NINFE, NKROL, INFZ, KRONR, INFE, KRONL, INFO] = slicot_ag08bd(EQUIL, M, P, A_IN, E_IN, B, C, D, TOL)
%=============================================================================
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
SLICOT Documentation
slicot_mb02md
Solution of Total Least-Squares problem using a SVD approach.
Syntax
- [RANK_OUT, C_OUT, S, X, IWARN, INFO] = slicot_mb02md(JOB, M, N, L, RANK_IN, C_IN, TOL)
Input argument
- JOB - Determines whether the values of the parameters RANK and TOL are to be specified by the user or computed by the routine as follows: = 'R': Compute RANK only; = 'T': Compute TOL only; = 'B': Compute both RANK and TOL; = 'N': Compute neither RANK nor TOL.
- M - The number of rows in the data matrix A and the observation matrix B.
- N - The number of columns in the data matrix A.
- L - The number of columns in the observation matrix B.
- RANK_IN - if JOB = 'T' or JOB = 'N', then RANK must specify r, the rank of the TLS approximation [A + DA | B + DB].
- C_IN - the leading M-by-(N+L) part of this array must contain the matrices A and B.
- TOL - A tolerance used to determine the rank of the TLS approximation [A+DA|B+DB] and to check the multiplicity of the singular values of matrix C.
Output argument
- RANK_OUT - if JOB = 'R' or JOB = 'B', and INFO = 0, then RANK contains the computed (effective) rank of the TLS approximation [A + DA | B + DB].
- C_OUT - the leading (N+L)-by-(N+L) part of this array contains the (transformed) right singular vectors, including null space vectors, if any, of C = [A | B].
- S - If INFO = 0, the singular values of matrix C
- X - If INFO = 0, the leading N-by-L part of this array contains the solution X to the TLS problem specified by A and B.
- IWARN - = 0: no warnings; = 1: if the rank of matrix C has been lowered because a singular value of multiplicity greater than 1 was found; = 2: if the rank of matrix C has been lowered because the upper triangular matrix F is (numerically) singular.
- INFO - = 0: successful exit;
Description
To solve the Total Least Squares (TLS) problem using a Singular Value Decomposition (SVD) approach. The TLS problem assumes an overdetermined set of linear equations AX = B, where both the data matrix A as well as the observation matrix B are inaccurate. The routine also solves determined and underdetermined sets of equations by computing the minimum norm solution. It is assumed that all preprocessing measures (scaling, coordinate transformations, whitening, ... ) of the data have been performed in advance.
Used function(s)
MB02MD
Bibliography
http://slicot.org/objects/software/shared/doc/MB02MD.html
Example
M = 6;
N = 3;
L = 1;
JOB = 'B';
TOL = 0.0;
RANK_IN = 1;
C_IN = [0.80010 0.39985 0.60005 0.89999;
0.29996 0.69990 0.39997 0.82997;
0.49994 0.60003 0.20012 0.79011;
0.90013 0.20016 0.79995 0.85002;
0.39998 0.80006 0.49985 0.99016;
0.20002 0.90007 0.70009 1.02994];
[RANK_OUT, C_OUT, S, X, IWARN, INFO] = slicot_mb02md(JOB, M, N, L, RANK_IN, C_IN, TOL)
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
SLICOT Documentation
slicot_mb03od
Matrix rank determination by incremental condition estimation.
Syntax
- [A_OUT, JPVT_OUT, TAU, RANK, SVAL, INFO] = slicot_mb03od(JOBQR, A_IN, JPVT_IN, RCOND, SVLMAX)
Input argument
- JOBQR - = 'Q': Perform a QR factorization with column pivoting; = 'N': Do not perform the QR factorization (but assume that it has been done outside).
- A_IN - with JOBQR = 'Q', the leading M by N part of this array must contain the given matrix A.
- JPVT_IN - with JOBQR = 'Q', if JPVT(i) != 0, the i-th column of A is an initial column, otherwise it is a free column. Before the QR factorization of A, all initial columns are permuted to the leading positions; only the remaining free columns are moved as a result of column pivoting during the factorization. For rank determination it is preferable that all columns be free.
- RCOND - RCOND is used to determine the effective rank of A, which is defined as the order of the largest leading triangular submatrix R11 in the QR factorization with pivoting of A, whose estimated condition number is less than 1/RCOND.
- SVLMAX - If A is a submatrix of another matrix B, and the rank decision should be related to that matrix, then SVLMAX should be an estimate of the largest singular value of B
Output argument
- A_OUT - with JOBQR = 'Q', the leading min(M,N) by N upper triangular part of A contains the triangular factor R, and the elements below the diagonal, with the array TAU, represent the orthogonal matrix Q as a product of min(M,N) elementary reflectors.
- JPVT_OUT - with JOBQR = 'Q', if JPVT(i) = k, then the i-th column of A*P was the k-th column of A.
- TAU - with JOBQR = 'Q', the leading min(M,N) elements of TAU contain the scalar factors of the elementary reflectors.
- RANK - The effective (estimated) rank of A, i.e. the order of the submatrix R11.
- SVAL - The estimates of some of the singular values of the triangular factor R
- INFO - = 0: successful exit
Description
To compute (optionally) a rank-revealing QR factorization of a real general M-by-N matrix A, which may be rank-deficient, and estimate its effective rank using incremental condition estimation.
Used function(s)
MB03OD
Bibliography
http://slicot.org/objects/software/shared/doc/MB03OD.html
Example
M = 6;
N = 5;
JOBQR = 'Q';
RCOND = 5.D-16;
SVLMAX = 0.0;
JPVT_IN = zeros(1, N);
A_IN = [1. 2. 6. 3. 5.;
-2. -1. -1. 0. -2.;
5. 5. 1. 5. 1.;
-2. -1. -1. 0. -2.;
4. 8. 4. 20. 4.;
-2. -1. -1. 0. -2.];
[A_OUT, JPVT_OUT, TAU, RANK, SVAL, INFO] = slicot_mb03od(JOBQR, A_IN, JPVT_IN, RCOND, SVLMAX)
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
SLICOT Documentation
slicot_mb03pd
Matrix rank determination by incremental condition estimation (row pivoting).
Syntax
- [A_OUT, JPVT_OUT, TAU, RANK, SVAL, INFO] = slicot_mb03pd(JOBRQ, A_IN, JPVT_IN, RCOND, SVLMAX)
Input argument
- JOBRQ - = 'R': Perform an RQ factorization with row pivoting; = 'N': Do not perform the RQ factorization (but assume that it has been done outside).
- A_IN - with JOBRQ = 'R', the leading M-by-N part of this array must contain the given matrix A.
- JPVT_IN - with JOBRQ = 'R', if JPVT(i) != 0, the i-th row of A is a final row, otherwise it is a free row. Before the RQ factorization of A, all final rows are permuted to the trailing positions; only the remaining free rows are moved as a result of row pivoting during the factorization. For rank determination it is preferable that all rows be free.
- RCOND - RCOND is used to determine the effective rank of A, which is defined as the order of the largest trailing triangular submatrix R22 in the RQ factorization with pivoting of A, whose estimated condition number is less than 1/RCOND.
- SVLMAX - If A is a submatrix of another matrix B, and the rank decision should be related to that matrix, then SVLMAX should be an estimate of the largest singular value of B (for instance, the Frobenius norm of B). If this is not the case, the input value SVLMAX = 0 should work.
Output argument
- A_OUT - with JOBRQ = 'R', if M less or equal than N, the upper triangle of the subarray A(1:M,N-M+1:N) contains the M-by-M upper triangular matrix R;
- JPVT_OUT - with JOBRQ = 'R', if JPVT(i) = k, then the i-th row of P*A was the k-th row of A.
- TAU - with JOBRQ = 'R', the leading min(M,N) elements of TAU contain the scalar factors of the elementary reflectors.
- RANK - The effective (estimated) rank of A, i.e. the order of the submatrix R22.
- SVAL - The estimates of some of the singular values of the triangular factor R.
- INFO - = 0: successful exit
Description
To compute (optionally) a rank-revealing RQ factorization of a real general M-by-N matrix A, which may be rank-deficient, and estimate its effective rank using incremental condition estimation.
Used function(s)
MB03PD
Bibliography
http://slicot.org/objects/software/shared/doc/MB03PD.html
Example
M = 6;
N = 5;
JOBRQ = 'R';
RCOND = 5.D-16;
SVLMAX = 0.0;
JPVT_IN = zeros(1, M);
A_IN = [ 1. 2. 6. 3. 5.;
-2. -1. -1. 0. -2.;
5. 5. 1. 5. 1.;
-2. -1. -1. 0. -2.;
4. 8. 4. 20. 4.;
-2. -1. -1. 0. -2.];
[A_OUT, JPVT_OUT, TAU, RANK, SVAL, INFO] = slicot_mb03pd(JOBRQ, A_IN, JPVT_IN, RCOND, SVLMAX)
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
SLICOT Documentation
slicot_mb03rd
Reduction of a real Schur form matrix to a block-diagonal form.
Syntax
- [A_OUT, X_OUT, NBLCKS, BLSIZE, WR, WI, INFO] = slicot_mb03rd(JOBX, SORT, PMAX, A_IN, X_IN, TOL)
Input argument
- JOBX - Specifies whether or not the transformations are accumulated, as follows: = 'N': The transformations are not accumulated; = 'U': The transformations are accumulated in X (the given matrix X is updated)
- SORT - Specifies whether or not the diagonal blocks of the real Schur form are reordered, as follows: = 'N': The diagonal blocks are not reordered; = 'S': The diagonal blocks are reordered before each step of reduction, so that clustered eigenvalues appear in the same block; = 'C': The diagonal blocks are not reordered, but the "closest-neighbour" strategy is used instead of the standard "closest to the mean" strategy. = 'B': The diagonal blocks are reordered before each step of reduction, and the "closest-neighbour" strategy is used.
- PMAX - An upper bound for the infinity norm of elementary submatrices of the individual transformations used for reduction
- A_IN - the leading N-by-N part of this array must contain the matrix A to be block-diagonalized, in real Schur form.
- X_IN - if JOBX = 'U', the leading N-by-N part of this array must contain a given matrix X.
- TOL - The tolerance to be used in the ordering of the diagonal blocks of the real Schur form matrix.
Output argument
- A_OUT - the leading N-by-N part of this array contains the computed block-diagonal matrix, in real Schur canonical form. The non-diagonal blocks are set to zero.
- X_OUT - if JOBX = 'U', the leading N-by-N part of this array contains the product of the given matrix X and the transformation matrix that reduced A to block-diagonal form. The transformation matrix is itself a product of non-orthogonal similarity transformations having elements with magnitude less than or equal to PMAX. If JOBX = 'N', this array is not referenced
- NBLCKS - The number of diagonal blocks of the matrix A.
- BLSIZE - The first NBLCKS elements of this array contain the orders of the resulting diagonal blocks of the matrix A.
- WR - real parts of the eigenvalues of the matrix A.
- WI - imaginary parts of the eigenvalues of the matrix A.
- INFO - = 0: successful exit;
Description
To reduce a matrix A in real Schur form to a block-diagonal form using well-conditioned non-orthogonal similarity transformations. The condition numbers of the transformations used for reduction are roughly bounded by PMAX*PMAX, where PMAX is a given value. The transformations are optionally postmultiplied in a given matrix X. The real Schur form is optionally ordered, so that clustered eigenvalues are grouped in the same block.
Used function(s)
MB03RD
Bibliography
http://slicot.org/objects/software/shared/doc/MB03RD.html
Example
N = 8;
PMAX = 1.D03;
TOL = 1.D-2;
JOBX = 'U';
SORT = 'S';
A_IN = [1. -1. 1. 2. 3. 1. 2. 3.;
1. 1. 3. 4. 2. 3. 4. 2.;
0. 0. 1. -1. 1. 5. 4. 1.;
0. 0. 0. 1. -1. 3. 1. 2.;
0. 0. 0. 1. 1. 2. 3. -1.;
0. 0. 0. 0. 0. 1. 5. 1.;
0. 0. 0. 0. 0. 0. 0.99999999 -0.99999999;
0. 0. 0. 0. 0. 0. 0.99999999 0.99999999];
X_IN = zeros(N, N);
[A_OUT, X_OUT, NBLCKS, BLSIZE, WR, WI, INFO] = slicot_mb03rd(JOBX, SORT, PMAX, A_IN, X_IN, TOL)
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
SLICOT Documentation
slicot_mb04gd
RQ factorization with row pivoting of a matrix.
Syntax
- [A_OUT, JPVT_OUT, TAU, INFO] = slicot_mb04gd(A_IN, JPVT_IN)
Input argument
- A_IN - The m-by-n matrix A.
- JPVT_IN - if JPVT(i) .ne. 0, the i-th row of A is permuted to the bottom of P*A (a trailing row); if JPVT(i) = 0, the i-th row of A is a free row.
Output argument
- A_OUT - if m less or equal than n, the upper triangle of the subarray A(1:m,n-m+1:n) contains the m-by-m upper triangular matrix R; if m greater or equal than n, the elements on and above the (m-n)-th subdiagonal contain the m-by-n upper trapezoidal matrix R; the remaining elements, with the array TAU, represent the orthogonal matrix Q as a product of min(m,n) elementary reflectors
- JPVT_OUT - if JPVT(i) = k, then the i-th row of P*A was the k-th row of A.
- TAU - The scalar factors of the elementary reflectors.
- INFO - = 0: successful exit.
Description
To compute an RQ factorization with row pivoting of a real m-by-n matrix A: P * A = R * Q.
Used function(s)
MB04GD
Bibliography
http://slicot.org/objects/software/shared/doc/MB04GD.html
Example
M = 6;
N = 5;
A_IN = [1. 2. 6. 3. 5.;
-2. -1. -1. 0. -2.;
5. 5. 1. 5. 1.;
-2. -1. -1. 0. -2.;
4. 8. 4. 20. 4.;
-2. -1. -1. 0. -2.];
JPVT_IN = zeros(1, M);
[A_OUT, JPVT_OUT, TAU, INFO] = slicot_mb04gd(A_IN, JPVT_IN)
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
SLICOT Documentation
slicot_mb04md
Balancing a general real matrix.
Syntax
- [MAXRED_OUT, A_OUT, SCALE, INFO] = slicot_mb04md(MAXRED_IN, A_IN)
Input argument
- MAXRED_IN - The maximum allowed reduction in the 1-norm of A (in an iteration) if zero rows or columns are encountered. If MAXRED greater than 0.0, MAXRED must be larger than one (to enable the norm reduction). If MAXRED less or equal than 0.0, then the value 10.0 for MAXRED is used.
- A_IN - The leading N-by-N part of this array must contain the input matrix A.
Output argument
- MAXRED_OUT - If the 1-norm of the given matrix A is non-zero, the ratio between the 1-norm of the given matrix and the 1-norm of the balanced matrix. Usually, this ratio will be larger than one, but it can sometimes be one, or even less than one (for instance, for some companion matrices).
- A_OUT - The leading N-by-N part of this array contains the balanced matrix.
- SCALE - The scaling factors applied to A. If D(j) is the scaling factor applied to row and column j, then SCALE(j) = D(j), for j = 1,...,N.
- INFO - = 0: successful exit.
Description
To reduce the 1-norm of a general real matrix A by balancing. This involves diagonal similarity transformations applied iteratively to A to make the rows and columns as close in norm as possible.
Used function(s)
MB04MD
Bibliography
http://slicot.org/objects/software/shared/doc/MB04MD.html
Example
MAXRED_IN = 0.0;
A_IN = [1.0 0.0 0.0 0.0;
300.0 400.0 500.0 600.0;
1.0 2.0 0.0 0.0;
1.0 1.0 1.0 1.0];
[MAXRED_OUT, A_OUT, SCALE, INFO] = slicot_mb04md(MAXRED_IN, A_IN)
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
SLICOT Documentation
slicot_mb05od
Matrix exponential for a real matrix, with accuracy estimate.
Syntax
- [A_OUT, MDIG, IDIG, IWARN, INFO] = slicot_mb05od(BALANC, NDIAG, DELTA, A_IN)
Input argument
- BALANC - Specifies whether or not a balancing transformation (done by SLICOT Library routine MB04MD) is required, as follows: = 'N', do not use balancing; = 'S', use balancing (scaling).
- NDIAG - The specified order of the diagonal Pade approximant. In the absence of further information NDIAG should be set to 9. NDIAG should not exceed 15. NDIAG greater or equal than 1.
- DELTA - The scalar value delta of the problem.
- A_IN - The leading N-by-N part of this array must contain the matrix A of the problem.
Output argument
- A_OUT - if INFO = 0, the leading N-by-N part of this array contains the solution matrix exp(A * delta).
- MDIG - The minimal number of accurate digits in the 1-norm of exp(A*delta).
- IDIG - The number of accurate digits in the 1-norm of exp(A*delta) at 95% confidence level.
- IWARN - = 0: no warning; = 1: if MDIG = 0 and IDIG greater than 0, warning for possible inaccuracy (the exponential has been computed); = 2: if MDIG = 0 and IDIG = 0, warning for severe inaccuracy (the exponential has been computed); = 3: if balancing has been requested, but it failed to reduce the matrix norm and was not actually used.
- INFO - = 0: successful exit; = 1: if the norm of matrix A*delta (after a possible balancing) is too large to obtain an accurate result; = 2: if the coefficient matrix (the denominator of the Pade approximant) is exactly singular; try a different value of NDIAG; = 3: if the solution exponential would overflow, possibly due to a too large value DELTA; the calculations stopped prematurely. This error is not likely to appear.
Description
To compute exp(A*delta) where A is a real N-by-N matrix and delta is a scalar value. The routine also returns the minimal number of accurate digits in the 1-norm of exp(A*delta) and the number of accurate digits in the 1-norm of exp(A*delta) at 95% confidence level.
Used function(s)
MB05OD
Bibliography
http://slicot.org/objects/software/shared/doc/MB05OD.html
Example
N = 3;
NDIAG = 9;
DELTA = 1.0;
BALANC = 'S';
A_IN = [2.0 1.0 1.0;
0.0 3.0 2.0;
1.0 0.0 4.0];
[A_OUT, MDIG, IDIG, IWARN, INFO] = slicot_mb05od(BALANC, NDIAG, DELTA, A_IN)
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
SLICOT Documentation
slicot_mc01td
Checking stability of a given real polynomial.
Syntax
- [DP_OUT, STABLE, NZ, IWARN, INFO] = slicot_mc01td(DICO, DP_IN, P)
Input argument
- DICO - Indicates whether the stability test to be applied to P(x) is in the continuous-time or discrete-time case as follows: = 'C': Continuous-time case; = 'D': Discrete-time case.
- DP_IN - The degree of the polynomial P(x).
- P - This array must contain the coefficients of P(x) in increasing powers of x.
Output argument
- DP_OUT - if P(DP+1) = 0.0 on entry, then DP contains the index of the highest power of x for which P(DP+1) != 0.0.
- STABLE - Contains the value int32(1) if P(x) is stable and the value int32(0) otherwise.
- NZ - If INFO = 0, contains the number of unstable zeros - that is, the number of zeros of P(x) in the right half-plane if DICO = 'C' or the number of zeros of P(x) outside the unit circle if DICO = 'D'.
- IWARN - = 0: no warning;
- INFO - = 0: successful exit; = 1: if on entry, P(x) is the zero polynomial;= 2: if the polynomial P(x) is most probably unstable.
Description
To determine whether or not a given polynomial P(x) with real coefficients is stable, either in the continuous-time or discrete-time case.
A polynomial is said to be stable in the continuous-time case if all its zeros lie in the left half-plane, and stable in the discrete-time case if all its zeros lie inside the unit circle.
Used function(s)
MC01TD
Bibliography
http://slicot.org/objects/software/shared/doc/MC01TD.html
Example
DICO = 'C';
DP_IN = 4;
P = [2.0 0.0 1.0 -1.0 1.0];
[DP, STABLE, NZ, IWARN, INFO] = slicot_mc01td(DICO, DP_IN, P)
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
SLICOT Documentation
slicot_sb01bd
Pole assignment for a given matrix pair (A,B).
Syntax
- [A_OUT, WR_OUT, WI_OUT, NFP, NAP, NUP, F, Z, IWARN, INFO] = slicot_sb01bd(DICO, ALPHA, A_IN, B_IN, WR_IN, WI_IN, TOL)
Input argument
- DICO - Specifies the type of the original system.'C': continuous-time system;'D': discrete-time system.
- ALPHA - Specifies the maximum admissible value.
- A_IN - the leading N-by-N part of this array must contain the state dynamics matrix A.
- B_IN - The leading N-by-M part of this array must contain the input/state matrix.
- WR_IN - contains the real parts of the desired eigenvalues of the closed-loop system state-matrix A+B*F.
- WI_IN - contains the imaginary parts of the desired eigenvalues of the closed-loop system state-matrix A+B*F.
- TOL - The absolute tolerance level below which the elements of A or B are considered zero (used for controllability tests).
Output argument
- A_OUT - the leading N-by-N part of this array contains the matrix Z'(A+BF)*Z in a real Schur form.
- WR_OUT - if INFO = 0, the leading NAP elements of these arrays contain the real parts of the assigned eigenvalues. The trailing NP-NAP elements contain the unassigned eigenvalues.
- WI_OUT - if INFO = 0, the leading NAP elements of these arrays contain the imaginary parts of the assigned eigenvalues. The trailing NP-NAP elements contain the unassigned eigenvalues.
- NFP - The number of eigenvalues of A having real parts less than ALPHA, if DICO = 'C', or moduli less than ALPHA, if DICO = 'D'. These eigenvalues are not modified by the eigenvalue assignment algorithm.
- NAP - The number of assigned eigenvalues. If INFO = 0 on exit, then NAP = N-NFP-NUP.
- NUP - The number of uncontrollable eigenvalues detected by the eigenvalue assignment algorithm.
- F - The leading M-by-N part of this array contains the state feedback F, which assigns NAP closed-loop eigenvalues and keeps unaltered N-NAP open-loop eigenvalues.
- Z - The leading N-by-N part of this array contains the orthogonal matrix Z which reduces the closed-loop system state matrix A + B*F to upper real Schur form.
- IWARN - = 0: no warning; = K: K violations of the numerical stability condition.
- INFO - = 0: successful exit;
Description
To determine the state feedback matrix F for a given system (A,B) such that the closed-loop state matrix A+B*F has specified eigenvalues.
Used function(s)
SB01BD
Bibliography
http://slicot.org/objects/software/shared/doc/SB01BD.html
Example
N = 4;
M = 2;
NP = 2;
ALPHA = -.4;
TOL = 1.E-8;
DICO = 'C';
A_IN = [ -6.8000 0.0000 -207.0000 0.0000;
1.0000 0.0000 0.0000 0.0000;
43.2000 0.0000 0.0000 -4.2000;
0.0000 0.0000 1.0000 0.0000];
B_IN = [ 5.6400 0.0000;
0.0000 0.0000;
0.0000 1.1800;
0.0000 0.0000];
WR_IN = [-0.5000; -0.5000];
WI_IN = [ 0.1500; -0.1500];
[A_OUT, WR_OUT, WI_OUT, NFP, NAP, NUP, F, Z, IWARN, INFO] = slicot_sb01bd(DICO, ALPHA, A_IN, B_IN, WR_IN, WI_IN, TOL)
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
SLICOT Documentation
slicot_sb02od
Solution of continuous- or discrete-time algebraic Riccati equations (generalized Schur vectors method).
Syntax
- [RCOND, X, ALFAR, ALFAI, BETA, S, T, U, INFO] = slicot_sb02od(DICO, JOBB, FACT, UPLO, JOBL, SORT, P, A, B, Q, R, L, TOL)
Input argument
- DICO - Specifies the type of Riccati equation to be solved as follows: = 'C': continuous-time case; 'D': discrete-time case.
- JOBB - Specifies whether or not the matrix G is given, instead of the matrices B and R, as follows: = 'B': B and R are given; = 'G': G is given.
- FACT - Specifies whether or not the matrices Q and/or R (if JOBB = 'B') are factored, as follows: = 'N': Not factored, Q and R are given; = 'C': C is given, and Q = C'C; = 'D': D is given, and R = D'D; = 'B': Both factors C and D are given, Q = C'C, R = D'D.
- UPLO - If JOBB = 'G', or FACT = 'N', specifies which triangle of the matrices G and Q (if FACT = 'N'), or Q and R (if JOBB = 'B'), is stored, as follows: = 'U': Upper triangle is stored; = 'L': Lower triangle is stored.
- JOBL - Specifies whether or not the matrix L is zero, as follows: = 'Z': L is zero; = 'N': L is nonzero. JOBL is not used if JOBB = 'G' and JOBL = 'Z' is assumed. SLICOT Library routine SB02MT should be called just before SB02OD, for obtaining the results when JOBB = 'G' and JOBL = 'N'.
- SORT - Specifies which eigenvalues should be obtained in the top of the generalized Schur form, as follows: = 'S': Stable eigenvalues come first;= 'U': Unstable eigenvalues come first.
- P - The number of system outputs. If FACT = 'C' or 'D' or 'B', P is the number of rows of the matrices C and/or D. P >= 0. Otherwise, P is not used.
- A - The leading N-by-N part of this array must contain the state matrix A of the system.
- B - If JOBB = 'B', the leading N-by-M part of this array must contain the input matrix B of the system.
- Q - If FACT = 'N' or 'D', the leading N-by-N upper triangular part (if UPLO = 'U') or lower triangular part (if UPLO = 'L') of this array must contain the upper triangular part or lower triangular part, respectively, of the symmetric state weighting matrix Q. The stricly lower triangular part (if UPLO = 'U') or stricly upper triangular part (if UPLO = 'L') is not referenced.
- R - If FACT = 'N' or 'C', the leading M-by-M upper triangular part (if UPLO = 'U') or lower triangular part (if UPLO = 'L') of this array must contain the upper triangular part or lower triangular part, respectively, of the symmetric input weighting matrix R. The stricly lower triangular part (if UPLO = 'U') or stricly upper triangular part (if UPLO = 'L') is not referenced.
- L - If JOBL = 'N' (and JOBB = 'B'), the leading N-by-M part of this array must contain the cross weighting matrix L. This part is modified internally, but is restored on exit. If JOBL = 'Z' or JOBB = 'G', this array is not referenced.
- TOL - The tolerance to be used to test for near singularity of the original matrix pencil, specifically of the triangular factor obtained during the reduction process.
Output argument
- RCOND - An estimate of the reciprocal of the condition number (in the 1-norm) of the N-th order system of algebraic equations from which the solution matrix X is obtained.
- X - The leading N-by-N part of this array contains the solution matrix X of the problem.
- ALFAR, ALFAI, BETA - The generalized eigenvalues of the 2N-by-2N matrix pair, ordered as specified by SORT (if INFO = 0).
- S - The leading 2N-by-2N part of this array contains the ordered real Schur form S of the first matrix in the reduced matrix pencil associated to the optimal problem, or of the corresponding Hamiltonian matrix, if DICO = 'C' and JOBB = 'G'.
- T - If DICO = 'D' or JOBB = 'B', the leading 2N-by-2N part of this array contains the ordered upper triangular form T of the second matrix in the reduced matrix pencil associated to the optimal problem.
- U - The leading 2N-by-2N part of this array contains the right transformation matrix U which reduces the 2N-by-2N matrix pencil to the ordered generalized real Schur form (S,T), or the Hamiltonian matrix to the ordered real Schur form S, if DICO = 'C' and JOBB = 'G'.
- INFO - = 0: successful exit;
Description
Solution of continuous- or discrete-time algebraic Riccati equations (generalized Schur vectors method)
The routine uses the method of deflating subspaces, based on reordering the eigenvalues in a generalized Schur matrix pair.
A standard eigenproblem is solved in the continuous-time case if G is given.
Used function(s)
SB02OD
Bibliography
http://slicot.org/objects/software/shared/doc/SB02OD.html
Example
N = 2;
M = 1;
P = 3;
TOL = 0.0;
DICO = 'C';
JOBB = 'B';
FACT = 'B';
UPLO = 'U';
JOBL = 'Z';
SORT = 'S';
A = [0.0 1.0;
0.0 0.0];
B = [0.0; 1.0];
Q = [1.0 0.0;
0.0 1.0;
0.0 0.0];
R = [0.0;
0.0;
1.0];
L = zeros(N, M);
[RCOND, X, ALFAR, ALFAI, BETA, S, T, U, INFO] = slicot_sb02od(DICO, JOBB, FACT, UPLO, JOBL, SORT, P, A, B, Q, R, L, TOL)
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
SLICOT Documentation
slicot_sb03md
Solution of continuous- or discrete-time Lyapunov equations and separation estimation.
Syntax
- [U_OUT, C_OUT, SCALE, SEP, FERR, WR, WI, INFO] = slicot_sb03md(DICO, JOB, FACT, TRANA, A, U_IN, C_IN)
Input argument
- DICO - Specifies the type of Lyapunov equation to be solved as follows: = 'C': continuous-time case; 'D': discrete-time case.
- JOB - Specifies the computation to be performed: 'X': Compute the solution only;= 'S': Compute the separation only; = 'B': Compute both the solution and the separation.
- FACT - Specifies whether or not the real Schur factorization of the matrix A is supplied on entry. = 'F': On entry, A and Q contain the factors from the real Schur factorization of the matrix A; = 'N': The Schur factorization of A will be computed and the factors will be stored in A and Q.
- TRANA - Specifies the form of op(A) to be used: = 'N': op(A) = A (No transpose); = 'T': op(A) = AT (Transpose); = 'C': op(A) = AT (Conjugate transpose = Transpose).
- A - the leading N-by-N part of this array must contain the matrix A. If FACT = 'F', then A contains an upper quasi-triangular matrix in Schur canonical form; the elements below the upper Hessenberg part of the array A are not referenced.
- U_IN - If FACT = 'N', zeros(N, N); If FACT = 'F', then U is an input argument and on entry the leading N-by-N part of this array must contain the orthogonal matrix U of the real Schur factorization of A.
- C_IN - With JOB = 'X' or 'B', the leading N-by-N part of this array must contain the symmetric matrix C.
Output argument
- U_OUT - if INFO = 0 or INFO = N+1, it contains the orthogonal N-by-N matrix from the real Schur factorization of A.
- C_OUT - With JOB = 'X' or 'B', if INFO = 0 or INFO = N+1, the leading N-by-N part of C has been overwritten by the symmetric solution matrix X.
- SCALE - The scale factor, scale, set less than or equal to 1 to prevent the solution overflowing.
- SEP - If JOB = 'S' or JOB = 'B', and INFO = 0 or INFO = N+1, SEP contains the estimated separation of the matrices op(A) and -op(A)', if DICO = 'C' or of op(A) and op(A)', if DICO = 'D'.
- FERR - If JOB = 'B', and INFO = 0 or INFO = N+1, FERR contains an estimated forward error bound for the solution X.
- WR - If FACT = 'N', and INFO = 0 or INFO = N+1, WR contains the real parts of the eigenvalues of A.
- WI - If FACT = 'N', and INFO = 0 or INFO = N+1, WI contains the imaginary parts of the eigenvalues of A.
- INFO - = 0: successful exit;
Description
To solve for X either the real continuous-time Lyapunov equation
op(A)'*X + X*op(A) = scale*C
or the real discrete-time Lyapunov equation
op(A)'*X*op(A) - X = scale*C
and/or estimate an associated condition number, called separation, where op(A) = A or A' (A**T) and C is symmetric (C = C').
Used function(s)
SB03MD
Bibliography
http://slicot.org/objects/software/shared/doc/SB03MD.html
Example
N = 3;
DICO = 'D';
FACT = 'N';
JOB = 'X';
TRANA = 'N';
A = [3.0 1.0 1.0;
1.0 3.0 0.0;
0.0 0.0 3.0];
U_IN = zeros(N, N);
C_IN = [25.0 24.0 15.0;
24.0 32.0 8.0;
15.0 8.0 40.0];
[U_OUT, C_OUT, SCALE, SEP, FERR, WR, WI, INFO] = slicot_sb03md(DICO, JOB, FACT, TRANA, A, U_IN, C_IN)
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
SLICOT Documentation
slicot_sb03od
Solution of stable continuous- or discrete-time Lyapunov equations (Cholesky factor).
Syntax
- [Q_OUT, B_OUT, SCALE, WR, WI, INFO] = slicot_sb03od(DICO, FACT, TRANS, A, Q_IN, B_IN)
Input argument
- DICO - Specifies the type of Lyapunov equation to be solved as follows: = 'C': continuous-time case; 'D': discrete-time case.
- FACT - Specifies whether or not the real Schur factorization of the matrix A is supplied on entry. = 'F': On entry, A and Q contain the factors from the real Schur factorization of the matrix A; = 'N': The Schur factorization of A will be computed and the factors will be stored in A and Q.
- TRANS - Specifies the form of op(K) to be used. = 'N': op(K) = K (No transpose); = 'T': op(K) = K**T (Transpose).
- A - the leading N-by-N part of this array must contain the matrix A. If FACT = 'F', then A contains an upper quasi-triangular matrix S in Schur canonical form; the elements below the upper Hessenberg part of the array A are not referenced.
- Q_IN - if FACT = 'F', then the leading N-by-N part of this array must contain the orthogonal matrix Q of the Schur factorization of A. Otherwise, Q need not be set on entry.
- B_IN - if TRANS = 'N', and dimension (LDB,max(M,N)), if TRANS = 'T'. On entry, if TRANS = 'N', the leading M-by-N part of this array must contain the coefficient matrix B of the equation. On entry, if TRANS = 'T', the leading N-by-M part of this array must contain the coefficient matrix B of the equation.
Output argument
- Q_OUT - the leading N-by-N part of this array contains the orthogonal matrix Q of the Schur factorization of A. The contents of array Q is not modified if FACT = 'F'.
- B_OUT - the leading N-by-N part of this array contains the upper triangular Cholesky factor U of the solution matrix X of the problem, X = op(U)'*op(U). If M = 0 and N > 0, then U is set to zero.
- SCALE - The scale factor, scale, set less than or equal to 1 to prevent the solution overflowing.
- WR - If FACT = 'N', and INFO >= 0 and INFO less than 2, WR contains the real parts of the eigenvalues of A.
- WI - If FACT = 'N', and INFO >= 0 and INFO less than 2, WI contains the imaginary parts of the eigenvalues of A.
- INFO - = 0: successful exit.
Description
To solve for X = op(U)'*op(U) either the stable non-negative definite continuous-time Lyapunov equation or the convergent non-negative definite discrete-time Lyapunov equation.
Used function(s)
SB03OD
Bibliography
http://slicot.org/objects/software/shared/doc/SB03OD.html
Example
A = [-0.5000 0.5000 0;
0 0 0;
-0.5000 0 0.5000];
B_IN = [0.5000 1.5000 1.0000;
1.0000 1.0000 1.0000;
0.5000 1.0000 1.5000];
DICO = 'D';
FACT = 'N';
Q_IN = zeros(3, 3);
[Q_OUT, B_OUT, SCALE, WR, WI, INFO] = slicot_sb03od(DICO, FACT, TRANS, A, Q_IN, B_IN)
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
SLICOT Documentation
slicot_sb04md
Solution of continuous-time Sylvester equations (Hessenberg-Schur method).
Syntax
- [A_OUT, B_OUT, C_OUT, Z, INFO] = slicot_sb04md(A_IN, B_IN, C_IN)
Input argument
- A_IN - The leading N-by-N part of this array must contain the coefficient matrix A of the equation.
- B_IN - The leading M-by-M part of this array must contain the coefficient matrix B of the equation.
- C_IN - The leading N-by-M part of this array must contain the coefficient matrix C of the equation.
Output argument
- A_OUT - The leading N-by-N upper Hessenberg part of this array contains the matrix H, and the remainder of the leading N-by-N part, together with the elements 2,3,...,N of array DWORK, contain the orthogonal transformation matrix U (stored in factored form).
- B_OUT - The leading M-by-M part of this array contains the quasi-triangular Schur factor S of the matrix B'.
- C_OUT - The leading N-by-M part of this array contains the solution matrix X of the problem.
- Z - The leading M-by-M part of this array contains the orthogonal matrix Z used to transform B' to real upper Schur form.
- INFO - = 0: successful exit;
Description
To solve for X the continuous-time Sylvester equation AX + XB = C where A, B, C and X are general N-by-N, M-by-M, N-by-M and N-by-M matrices respectively.
Used function(s)
SB04MD
Bibliography
http://slicot.org/objects/software/shared/doc/SB04MD.html
Example
N = 3;
M = 2;
A_IN = [2.0 1.0 3.0;
0.0 2.0 1.0;
6.0 1.0 2.0];
B_IN = [2.0 1.0;
1.0 6.0];
C_IN = [2.0 1.0;
1.0 4.0;
0.0 5.0];
[A_OUT, B_OUT, C_OUT, Z, INFO] = slicot_sb04md(A_IN, B_IN, C_IN)
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
SLICOT Documentation
slicot_sb04qd
Solution of discrete-time Sylvester equations (Hessenberg-Schur method).
Syntax
- [A_OUT, B_OUT, C_OUT, Z, INFO] = slicot_sb04qd(A_IN, B_IN, C_IN)
Input argument
- A_IN - The leading N-by-N part of this array must contain the coefficient matrix A of the equation.
- B_IN - The leading M-by-M part of this array must contain the coefficient matrix B of the equation.
- C_IN - The leading N-by-M part of this array must contain the coefficient matrix C of the equation.
Output argument
- A_OUT - The leading N-by-N upper Hessenberg part of this array contains the matrix H, and the remainder of the leading N-by-N part, together with the elements 2,3,...,N of array DWORK, contain the orthogonal transformation matrix U (stored in factored form).
- B_OUT - The leading M-by-M part of this array contains the quasi-triangular Schur factor S of the matrix B'.
- C_OUT - The leading N-by-M part of this array contains the solution matrix X of the problem.
- Z - The leading M-by-M part of this array contains the orthogonal matrix Z used to transform B' to real upper Schur form.
- INFO - = 0: successful exit;
Description
To solve for X the discrete-time Sylvester equation X + AXB = C, where A, B, C and X are general N-by-N, M-by-M, N-by-M and N-by-M matrices respectively. A Hessenberg-Schur method, which reduces A to upper Hessenberg form, H = U'AU, and B' to real Schur form, S = Z'B'Z (with U, Z orthogonal matrices), is used.
Used function(s)
SB04QD
Bibliography
http://slicot.org/objects/software/shared/doc/SB04QD.html
Example
N = 3;
M = 3;
A_IN = [1.0 2.0 3.0;
6.0 7.0 8.0;
9.0 2.0 3.0];
B_IN = [7.0 2.0 3.0;
2.0 1.0 2.0;
3.0 4.0 1.0];
C_IN = [271.0 135.0 147.0;
923.0 494.0 482.0;
578.0 383.0 287.0];
[A_OUT, B_OUT, C_OUT, Z, INFO] = slicot_sb04qd(A_IN, B_IN, C_IN)
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
SLICOT Documentation
slicot_sb10jd
Converting a descriptor state-space system into regular state-space form.
Syntax
- [A_OUT, B_OUT, C_OUT, D_OUT, E_OUT, NSYS, INFO] = slicot_sb10jd(A_IN, B_IN, C_IN, D_IN, E_IN)
Input argument
- A_IN - the leading N-by-N part of this array must contain the state matrix A of the descriptor system.
- B_IN - the leading N-by-M part of this array must contain the input matrix B of the descriptor system.
- C_IN - the leading NP-by-N part of this array must contain the output matrix C of the descriptor system.
- D_IN - the leading NP-by-M part of this array must contain the matrix D of the descriptor system.
- E_IN - the leading N-by-N part of this array must contain the matrix E of the descriptor system.
Output argument
- A_OUT - the leading NSYS-by-NSYS part of this array contains the state matrix Ad of the converted system.
- B_OUT - the leading NSYS-by-M part of this array contains the input matrix Bd of the converted system.
- C_OUT - the leading NP-by-NSYS part of this array contains the output matrix Cd of the converted system.
- D_OUT - the leading NP-by-M part of this array contains the matrix Dd of the converted system.
- E_OUT - this array contains no useful information.
- NSYS - The order of the converted state-space system.
- INFO - = 0: successful exit; = 1: the iteration for computing singular value decomposition did not converge.
Description
To convert the descriptor state-space system into regular state-space form.
Used function(s)
SB10JD
Bibliography
http://slicot.org/objects/software/shared/doc/SB10JD.html
Example
A_IN = [2 -4; 4 2];
B_IN = [0 -1; 0 0.5];
C_IN = [0 -0.5; 0 -2];
D_IN = [0 0; 0 -1];
E_IN = [1 0; -3 0.5];
[A_OUT, B_OUT, C_OUT, D_OUT, E_OUT, NSYS, INFO] = slicot_sb10jd(A_IN, B_IN, C_IN, D_IN, E_IN)
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
SLICOT Documentation
slicot_sg02ad
Solution of continuous- or discrete-time algebraic Riccati equations for descriptor systems.
Syntax
- [RCONDU, X, ALFAR, ALFAI, BETA, S, T, U, IWARN, INFO] = slicot_sg02ad(DICO, JOBB, FACT, UPLO, JOBL, SCAL, SORT, ACC, P, A, E, B, Q, R, L, TOL)
Input argument
- DICO - Specifies the type of Riccati equation to be solved as follows: = 'C': Equation (1), continuous-time case; = 'D': Equation (2), discrete-time case.
- JOBB - Specifies whether or not the matrix G is given, instead of the matrices B and R, as follows: = 'B': B and R are given; = 'G': G is given.
- FACT - Specifies whether or not the matrices Q and/or R (if JOBB = 'B') are factored, as follows: = 'N': Not factored, Q and R are given; = 'C': C is given, and Q = C'C; = 'D': D is given, and R = D'D; = 'B': Both factors C and D are given, Q = C'C, R = D'D.
- UPLO - If JOBB = 'G', or FACT = 'N', specifies which triangle of the matrices G, or Q and R, is stored, as follows: = 'U': Upper triangle is stored; = 'L': Lower triangle is stored.
- JOBL - Specifies whether or not the matrix L is zero, as follows: = 'Z': L is zero; = 'N': L is nonzero. JOBL is not used if JOBB = 'G' and JOBL = 'Z' is assumed. SLICOT Library routine SB02MT should be called just before SG02AD, for obtaining the results when JOBB = 'G' and JOBL = 'N'.
- SCAL - If JOBB = 'B', specifies whether or not a scaling strategy should be used to scale Q, R, and L, as follows: = 'G': General scaling should be used; = 'N': No scaling should be used. SCAL is not used if JOBB = 'G'.
- SORT - Specifies which eigenvalues should be obtained in the top of the generalized Schur form, as follows: = 'S': Stable eigenvalues come first; = 'U': Unstable eigenvalues come first.
- ACC - Specifies whether or not iterative refinement should be used to solve the system of algebraic equations giving the solution matrix X, as follows: = 'R': Use iterative refinement; = 'N': Do not use iterative refinement.
- P - The number of system outputs. If FACT = 'C' or 'D' or 'B', P is the number of rows of the matrices C and/or D.
- A - The leading N-by-N part of this array must contain the state matrix A of the descriptor system.
- E - The leading N-by-N part of this array must contain the matrix E of the descriptor system.
- B - If JOBB = 'B', the leading N-by-M part of this array must contain the input matrix B of the system.
- Q - If FACT = 'N' or 'D', the leading N-by-N upper triangular part (if UPLO = 'U') or lower triangular part (if UPLO = 'L') of this array must contain the upper triangular part or lower triangular part, respectively, of the symmetric state weighting matrix Q. The stricly lower triangular part (if UPLO = 'U') or stricly upper triangular part (if UPLO = 'L') is not referenced. If FACT = 'C' or 'B', the leading P-by-N part of this array must contain the output matrix C of the system. If JOBB = 'B' and SCAL = 'G', then Q is modified internally, but is restored on exit.
- R - If FACT = 'N' or 'C', the leading M-by-M upper triangular part (if UPLO = 'U') or lower triangular part (if UPLO = 'L') of this array must contain the upper triangular part or lower triangular part, respectively, of the symmetric input weighting matrix R. The stricly lower triangular part (if UPLO = 'U') or stricly upper triangular part (if UPLO = 'L') is not referenced. If FACT = 'D' or 'B', the leading P-by-M part of this array must contain the direct transmission matrix D of the system. If JOBB = 'B' and SCAL = 'G', then R is modified internally, but is restored on exit.
- L - If JOBL = 'N' and JOBB = 'B', the leading N-by-M part of this array must contain the cross weighting matrix L. If JOBB = 'B' and SCAL = 'G', then L is modified internally, but is restored on exit.
- TOL - The tolerance to be used to test for near singularity of the original matrix pencil, specifically of the triangular M-by-M factor obtained during the reduction process.
Output argument
- RCONDU - If N > 0 and INFO = 0 or INFO = 7, an estimate of the reciprocal of the condition number (in the 1-norm) of the N-th order system of algebraic equations from which the solution matrix X is obtained.
- X - If INFO = 0, the leading N-by-N part of this array contains the solution matrix X of the problem.
- ALFAR - The generalized eigenvalues of the 2N-by-2N matrix pair, ordered as specified by SORT (if INFO = 0, or INFO >= 5).
- ALFAI - The generalized eigenvalues of the 2N-by-2N matrix pair, ordered as specified by SORT (if INFO = 0, or INFO >= 5).
- BETA - The generalized eigenvalues of the 2N-by-2N matrix pair, ordered as specified by SORT (if INFO = 0, or INFO >= 5).
- S - The leading 2N-by-2N part of this array contains the ordered real Schur form S of the first matrix in the reduced matrix pencil associated to the optimal problem, corresponding to the scaled Q, R, and L, if JOBB = 'B' and SCAL = 'G'.
- T - The leading 2N-by-2N part of this array contains the ordered upper triangular form T of the second matrix in the reduced matrix pencil associated to the optimal problem, corresponding to the scaled Q, R, and L, if JOBB = 'B' and SCAL = 'G'.
- U - The leading 2N-by-2N part of this array contains the right transformation matrix U which reduces the 2N-by-2N matrix pencil to the ordered generalized real Schur form (S,T).
- IWARN - = 0: no warning; = 1: the computed solution may be inaccurate due to poor scaling or eigenvalues too close to the boundary of the stability domain (the imaginary axis, if DICO = 'C', or the unit circle, if DICO = 'D').
- INFO - = 0: successful exit; = 1: if the computed extended matrix pencil is singular, possibly due to rounding errors; = 2: if the QZ algorithm failed; = 3: if reordering of the generalized eigenvalues failed; = 4: if after reordering, roundoff changed values of some complex eigenvalues so that leading eigenvalues in the generalized Schur form no longer satisfy the stability condition; this could also be caused due to scaling; = 5: if the computed dimension of the solution does not equal N; = 6: if the spectrum is too close to the boundary of the stability domain; = 7: if a singular matrix was encountered during the computation of the solution matrix X.
Description
To solve for X either the continuous-time algebraic Riccatiequation or the discrete-time algebraic Riccati equation
Used function(s)
SG02AD
Bibliography
http://slicot.org/objects/software/shared/doc/SG02AD.html
Example
N = 2;
M = 1;
P = 3;
TOL = 0.0;
DICO = 'C';
JOBB = 'B';
FACT = 'B';
UPLO = 'U';
JOBL = 'Z';
SCAL = 'N';
SORT = 'S';
ACC = 'N';
A = [0.0 1.0;
0.0 0.0];
E = [1.0 0.0;
0.0 1.0];
B = [0.0;
1.0];
Q = [1.0 0.0;
0.0 1.0;
0.0 0.0];
R = [0.0;
0.0;
1.0];
L = zeros(N, N);
[RCONDU, X, ALFAR, ALFAI, BETA, S, T, U, IWARN, INFO] = slicot_sg02ad(DICO, JOBB, FACT, UPLO, JOBL, SCAL, SORT, ACC, P, A, E, B, Q, R, L, TOL)
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
SLICOT Documentation
slicot_tb01id
Balancing a system matrix corresponding to a triplet (A, B, C).
Syntax
- [MAXRED_OUT, A_OUT, B_OUT, C_OUT, SCALE, INFO] = slicot_tb01id(JOB, MAXRED_IN, A_IN, B_IN, C_IN)
Input argument
- JOB - = 'A': All matrices are involved in balancing; = 'B': B and A matrices are involved in balancing; = 'C': C and A matrices are involved in balancing; = 'N': B and C matrices are not involved in balancing.
- MAXRED_IN - the maximum allowed reduction in the 1-norm of S (in an iteration) if zero rows or columns are encountered.
- A_IN - The leading N-by-N part of this array must contain the system state matrix A.
- B_IN - The leading N-by-M part of this array must contain the system input matrix B.
- C_IN - The leading P-by-N part of this array must contain the system output matrix C.
Output argument
- MAXRED_OUT - if the 1-norm of the given matrix S is non-zero, the ratio between the 1-norm of the given matrix and the 1-norm of the balanced matrix.
- A_OUT - The leading N-by-N part of this array contains the balanced matrix inv(D)AD.
- B_OUT - The leading N-by-M part of this array contains the balanced matrix inv(D)*B.
- C_OUT - The leading P-by-N part of this array contains the balanced matrix C*D.
- SCALE - The scaling factors applied to S.
- INFO - = 0: successful exit.
Description
To reduce the 1-norm of a system matrix corresponding to the triple (A,B,C), by balancing.
Used function(s)
TB01ID
Bibliography
http://slicot.org/objects/software/shared/doc/TB01ID.html
Example
N = 5;
M = 2;
P = 5;
JOB = 'A';
MAXRED_IN = 0.0;
A_IN = [0.0 1.0000e+000 0.0 0.0 0.0;
-1.5800e+006 -1.2570e+003 0.0 0.0 0.0;
3.5410e+014 0.0 -1.4340e+003 0.0 -5.3300e+011;
0.0 0.0 0.0 0.0 1.0000e+000;
0.0 0.0 0.0 -1.8630e+004 -1.4820e+000];
B_IN = [0.0 0.0;
1.1030e+002 0.0;
0.0 0.0;
0.0 0.0;
0.0 8.3330e-003];
C_IN = [1.0000e+000 0.0 0.0 0.0 0.0;
0.0 0.0 1.0000e+000 0.0 0.0;
0.0 0.0 0.0 1.0000e+000 0.0;
6.6640e-001 0.0 -6.2000e-013 0.0 0.0;
0.0 0.0 -1.0000e-003 1.8960e+006 1.5080e+002];
[MAXRED_OUT, A_OUT, B_OUT, C_OUT, SCALE, INFO] = slicot_tb01id(JOB, MAXRED_IN, A_IN, B_IN, C_IN)
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
SLICOT Documentation
slicot_tg01ad
Balancing the matrices of the system pencil corresponding to a descriptor triple (A-lambda E, B, C).
Syntax
- [A_OUT, E_OUT, B_OUT, C_OUT, LSCALE, RSCALE, INFO] = slicot_tg01ad(JOB, THRESH, A_IN, E_IN, B_IN, C_IN)
Input argument
- JOB - = 'A': All matrices are involved in balancing; = 'B': B, A and E matrices are involved in balancing; = 'C': C, A and E matrices are involved in balancing; = 'N': B and C matrices are not involved in balancing.
- THRESH - Threshold value for magnitude of elements: elements with magnitude less than or equal to THRESH are ignored for balancing.
- A_IN - The leading L-by-N part of this array must contain the state dynamics matrix A.
- E_IN - The leading L-by-N part of this array must contain the descriptor matrix E.
- B_IN - The leading L-by-M part of this array must contain the input/state matrix B.
- C_IN - The leading P-by-N part of this array must contain the state/output matrix C.
Output argument
- A_OUT - The leading L-by-N part of this array contains the balanced matrix DlADr.
- E_OUT - The leading L-by-N part of this array contains the balanced matrix DlEDr.
- B_OUT - The leading L-by-M part of this array contains the balanced matrix Dl*B.
- C_OUT - The leading P-by-N part of this array contains the balanced matrix C*Dr.
- LSCALE - The scaling factors applied to S from left.
- RSCALE - The scaling factors applied to S from right.
- INFO - = 0: successful exit.
Description
To balance the matrices of the system pencil corresponding to the descriptor triple (A-lambda E,B,C), by balancing.
Used function(s)
TG01AD
Bibliography
http://slicot.org/objects/software/shared/doc/TG01AD.html
Example
L = 4;
N = 4;
M = 2;
P = 2;
JOB = 'A';
THRESH = 0;
A_IN = [ -1 0 0 0.003;
0 0 0.1000 0.02;
100 10 0 0.4;
0 0 0 0.0];
E_IN = [1 0.2 0 0.0;
0 1 0 0.01;
300 90 6 0.3;
0 0 20 0.0];
B_IN = [10 0;
0 0;
0 1000;
10000 10000];
C_IN = [-0.1 0.0 0.001 0.0;
0.0 0.01 -0.001 0.0001];
[A_OUT, E_OUT, B_OUT, C_OUT, LSCALE, RSCALE, INFO] = slicot_tg01ad(JOB, THRESH, A_IN, E_IN, B_IN, C_IN)
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
SLICOT Documentation
Special functions
Special functions
Description
special functions
- betainc - Incomplete beta function
- cross - Cross product.
- dot - Dot product.
- factor - Prime factors
- gamma - Gamma special function
- gammaln - Logarithm of gamma function
- gcd - Greatest common divisor
- interp1 - Linear 1-D data interpolation
- peaks - Peaks function
- primes - Prime numbers less than or equal to input value
betainc
Incomplete beta function
Syntax
- R = betainc(X, Z, W)
- R = betainc(X, Z, W, tail)
Input argument
- X - a real single or real double matrix. It must be in the closed interval [0, 1].
- Z - a real single or real double matrix. It must be nonnegative.
- W - a real single or real double matrix. It must be nonnegative.
- tail - a string 'upper' or 'lower' (default).
Output argument
- R - result of betainc function.
Description
betainc computes the Incomplete beta function.
All arrays must be the same size or any of them can be scalar.
Example
R = betainc(0.5, 1:10, 3)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
cross
Cross product.
Syntax
- R = cross(A, B)
- R = cross(A, B, dim)
Input argument
- A, B - numeric arrays.
- dim - positive integer scalar: Dimension to operate along.
Output argument
- R - Vector cross Product.
Description
R = cross(A, B) returns the cross product of A and B.
Bibliography
https://en.wikipedia.org/wiki/Cross_product
Example
A = [1 2 3;4 5 6;7 8 9];
B = [9 8 7;6 5 4;3 2 1];
R = cross(A, B)
R = cross(A, B, 2)
See also
dot.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
dot
Dot product.
Syntax
- R = dot(A, B)
- R = dot(A, B, dim)
Input argument
- A, B - numeric arrays.
- dim - positive integer scalar: Dimension to operate along.
Output argument
- R - Scalar Dot Product.
Description
R = dot(A, B) returns the scalar dot product of A and B.
Bibliography
https://en.wikipedia.org/wiki/Dot_product
Example
A = [1 2 3;4 5 6;7 8 9];
B = [9 8 7;6 5 4;3 2 1];
R = dot(A, B)
R = dot(A, B, 2)
See also
conj.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
factor
Prime factors
Syntax
- f = factor(n)
Input argument
- n - real, nonnegative integer scalar
Output argument
- p - vector with Prime factors.
Description
f = factor(n) returns a row vector with the prime factors of n.
Vector f is of the same data type as n.
Example
f = factor(204)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
gamma
Gamma special function
Syntax
- R = gamma(M)
Input argument
- M - a real single or real double matrix.
Output argument
- R - result of gamma function.
Description
gamma computes the gamma function.
gamma is an extension of the factorial function.
Example
R = gamma([-pi:0.1:pi])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
gammaln
Logarithm of gamma function
Syntax
- R = gammaln(M)
Input argument
- M - a real single or real double matrix.
Output argument
- R - result of gammaln function.
Description
The function gammaln(A) computes the natural logarithm of the gamma function for a given input A, expressed as gammaln(A) = log(gamma(A)).
It's important to note that A must be a nonnegative real number.
Using gammaln helps prevent potential underflow and overflow issues that might arise if directly computing log(gamma(A)).
Example
R = gammaln([0:0.1:pi])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
gcd
Greatest common divisor
Syntax
- G = gcd(A, B)
Input argument
- A - a scalar, vector, or matrix of real integer values.
- B - a scalar, vector, or matrix of real integer values.
Output argument
- G - result of gcd function (Greatest common divisor).
Description
G = gcd(A, B) computes the greatest common divisor using the Euclidian algorithm.
Bibliography
Knuth, D. “Algorithms A and X.” The Art of Computer Programming, Vol. 2, Section 4.5.2. Reading, MA: Addison-Wesley, 1973.
Example
A = [-5 7; 10 0];
B = [-15 3; 50 0];
G = gcd(A, B)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
interp1
Linear 1-D data interpolation
Syntax
- vq = interp1(x, v, xq)
- vq = interp1(x, v, xq, 'linear')
- vq = interp1(v, xq)
- vq = interp1(v, xq, 'linear')
Input argument
- x - Sample points: vector.
- v - Sample values: vector, matrix.
- xq - Query points: scalar, vector, matrix.
Output argument
- vq - Interpolated values: scalar, vector, matrix.
Description
vq = interp1(x, v, xq) returns interpolated values of a 1-D function at specific query points using linear interpolation.
Bibliography
de Boor, C., A Practical Guide to Splines, Springer-Verlag, 1978.
Example
f = figure();
v = [0 1.41 2 1.41 0 -1.41 -2 -1.41 0];
xq = 1.5:8.5;
vq = interp1(v,xq);
plot(1:9, v, 'o', xq, vq, '*');
legend('v','vq');
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
peaks
Peaks function
Syntax
- Z = peaks()
- Z = peaks(n)
- Z = peaks(Xi, Yi)
- [X, Y, Z] = peaks()
- [X, Y, Z] = peaks(n)
- [X, Y, Z] = peaks(Xi, Yi)
Input argument
- n - Value representing 2-D grid: scalar or vector.
- Xi - x-coordinates of points.
- Yi - y-coordinates of points.
Output argument
- X - x-coordinates of points.
- Y - y-coordinates of points.
- Z - z-coordinates of points.
Description
peaks function has the form:
f(x, y) = 3*(1-x)^2*exp(-x^2 - (y+1)^2) - 10*(x/5 - x^3 - y^5)*exp(-x^2-y^2) - 1/3*exp(-(x+1)^2 - y^2)
Example
x = -2:0.5:2;
y = 1:0.2:2;
[X, Y] = meshgrid(x, y);
Z = peaks(X, Y)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
primes
Prime numbers less than or equal to input value
Syntax
- p = primes(n)
Input argument
- n - scalar, real integer value
Output argument
- p - vector with prime numbers.
Description
p = primes(n) returns a row vector containing all the prime numbers less than or equal to n.
The data type of p is the same as that of n.
Example
p = primes(15)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
FFTW
FFTW
Description
Fastest Fourier Transform in the West
- FFTW License - About FFTW license.
- FFTWwrapper - load/free FFTW library dynamically.
- fft - Fast Fourier transform.
- fft2 - 2-D fast Fourier transform.
- fftn - N-Dimensions fast Fourier transform.
- fftshift - Shift the zero-frequency component to the center of the spectrum.
- fftw - function for determining FFT algorithm.
- ifft - Inverse Fast Fourier transform.
- ifftn - Inverse multidimensional fast Fourier transform.
- ifftshift - inverse of fftshift
FFTW License
About FFTW license.
Description
The FFTW library is no longer distributed as part of Nelson.
Note that FFTW is licensed under GPLv2 or higher (see http://www.fftw.org/doc/License-and-Copyright.html), but the bindings to the library in this nelson's module, is licensed under LGPL v3.0.
This means that code using the FFTW library via FFTW bindings is subject to FFTW's licensing terms.
Code using alternative implementations of the FFTW API, such as MKL's FFTW3 interface (https://software.intel.com/en-us/mkl-developer-reference-c-fftw3-interface-to-intel-math-kernel-library) are instead subject to the alternative's license.
If you distribute a derived or combined work, i.e. a program that links to and is distributed with the FFTW library, then that distribution falls under the terms of the GPL.
On Windows platforms, MKL FFTW implementation is used and distributed with Nelson.
On others plaforms, if FFTW library is available, and user chooses to use it, distribution falls under the terms of the GPL.
See also
fft.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
FFTWwrapper
load/free FFTW library dynamically.
Syntax
- r = FFTWwrapper('load')
- r = FFTWwrapper('load', fftwlibraryname, fftwflibraryname)
- r = FFTWwrapper('free')
Input argument
- 'load' - load FFTW library.
- 'free' - free FFTW library.
- fftwlibraryname - a string: fftw library name.
- fftwflibraryname - a string: fftw float library name.
Output argument
- r - a logical.
Description
FFTWwrapper is an internal builtin used to load FFTW library dynamically.
See also
fft.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
fft
Fast Fourier transform.
Syntax
- Y = fft(X)
- Y = fft(X, n)
- Y = fft(X, n, dim)
Input argument
- X - a vector, matrix or N-D array (double, single, integer, logical).
- n - transform length: a non negative integer scalar or [] (default).
- dim - dimension: a positive integer scalar.
Output argument
- Y - a vector, matrix, N-D array: frequency domain representation.
Description
fft(X) computes the discrete Fourier transform of X using a Fast Fourier Transform (FFT) algorithm based on FFTW library.
Example
% Sampling frequency
Fs = 150;
% Time vector of 1 second
t = 0:1*inv(Fs):1;
% Creates a sine wave of f Hz.
f = 5;
x = sin(2 * pi * t * f);
% Length of FFT
nfft = 1024;
% Take fft, padding with zeros so that length(X) is equal to nfft
X = fft(x, nfft)
% FFT is symmetrix
X = X(1:nfft*inv(2))
% Frequency vector
f = (0:nfft *inv(2) -1)*Fs * inv(nfft);
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
fft2
2-D fast Fourier transform.
Syntax
- Y = fft2(X)
- Y = fft2(X, m, n)
Input argument
- X - Input array.
- m - Number of transform rows.
- n - Number of transform columns.
Output argument
- Y - a vector, matrix, N-D array: frequency domain representation.
Description
Y = fft2(X) returns the two-dimensional Fourier transform of X using a Fast Fourier Transform (FFT) algorithm.
Optional arguments m and n may be used specify the number of rows and columns of X to use.
If either of these is larger than the size of X, X is resized and padded with zeros.
If X is a multi-dimensional matrix, each two-dimensional sub-matrix of X is treated separately.
Example
R = fft2(eye(5, 5), 2, 3)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
fftn
N-Dimensions fast Fourier transform.
Syntax
- Y = fftn(X)
- Y = fftn(X, sz)
Input argument
- X - a vector, matrix or N-D array (double, single, integer, logical).
- sz - a multidimensional array
Output argument
- Y - a vector, matrix, N-D array: frequency domain representation.
Description
Y = fftn(X, sz) pads X with zeros, or truncates X, to create a multidimensional array of size sz before performing the transform.
The size of the result Y is sz.
Y = fftn(X) performs the N-dimensional fast Fourier transform.
The result Y is the same size as X.
Example
f = zeros(5, 5);
f(1:5,4:5) = 1;
Y = ifftn(fftn(f));
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
fftshift
Shift the zero-frequency component to the center of the spectrum.
Syntax
- Y = fftshift(X)
- Y = fftshift(X, DIM)
Input argument
- X - a vector, matrix or N-D array (double, single, integer).
- DIM - axes over which to shift.
Output argument
- Y - shifted array.
Description
fftshift(X) shift the zero-frequency component to the center of the spectrum.
Example
M = [ 0., 10., 20.; 30., 40., -40.; -30., -20., -10.]
fftshift(M)
fftshift(M, 1)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
fftw
function for determining FFT algorithm.
Syntax
- m = fftw('planner')
- fftw('planner', m)
- w = fftw('dwisdom')
- fftw('dwisdom', w)
- w = fftw('swisdom')
- fftw('swisdom', w)
Input argument
- m - method for setting transform parameters: 'estimate', 'measure', 'patient', 'exhaustive', or 'hybrid'.
- w - a string: wisdom data.
Output argument
- m - method: 'estimate', 'measure', 'patient', 'exhaustive', or 'hybrid'.
- w - a string: wisdom data.
Description
The default method is 'estimate'.
Example
w = fftw('dwisdom')
M = rand(1000);
tic; fft(M); toc
fftw('dwisdom', w)
tic; fft(M); toc
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
ifft
Inverse Fast Fourier transform.
Syntax
- Y = ifft(X)
- Y = ifft(X, n)
- Y = ifft(X, n, dim)
Input argument
- X - a vector, matrix or N-D array (double, single, integer, logical).
- n - transform length: a non negative integer scalar or [] (default).
- dim - dimension: a positive integer scalar.
Output argument
- Y - a vector, matrix, N-D array: frequency domain representation.
Description
ifft(X) computes the inverse discrete Fourier transform of X using a Fast Fourier Transform (FFT) algorithm based on FFTW library.
Example
A = [1:10]
Y = fft(A)
R = ifft(Y)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
ifftn
Inverse multidimensional fast Fourier transform.
Syntax
- Y = ifftn(X)
- Y = ifftn(X, sz)
Input argument
- X - a vector, matrix or N-D array (double, single, integer, logical).
- sz - a multidimensional array.
Output argument
- Y - a vector, matrix, N-D array: frequency domain representation.
Description
Y = ifftn(X, sz) pads X with zeros, or truncates X, to create a multidimensional array of size sz before performing the transform.
The size of the result Y is sz.
Y = ifftn(X) performs the N-dimensional inverse fast Fourier transform.
The result Y is the same size as X.
Example
f = zeros(5, 5);
f(1:5,4:5) = 1;
Y = ifftn(fftn(f));
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
ifftshift
inverse of fftshift
Syntax
- Y = ifftshift(X)
- Y = ifftshift(X, DIM)
Input argument
- X - a vector, matrix or N-D array (double, single, integer).
- DIM - axes over which to shift.
Output argument
- Y - shifted array.
Description
fftshift(X) computes the inverse fftshift.
Example
M = [ 0., 10., 20.; 30., 40., -40.; -30., -20., -10.]
ifftshift(M)
ifftshift(M, 1)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
OS functions
OS functions
Description
operating system functions
- cmdsep - Command separator for current operating system.
- computer - System information.
- createGUID - Creates a GUID.
- getenv - Get the value of an environment variable.
- hostname - get host name of this computer.
- ismac - Checks if version is for MacOS platform.
- ispc - Checks if version is for Windows platform.
- isunix - Checks if version is for GNU Linux or Unix platform.
- searchenv - Searches for a file using environment paths.
- setenv - Set the value of an environment variable.
- system - Shell command execution.
- username - get user name currently used.
- winopen - Open file in appropriate application (Windows only).
- winqueryreg - Read the Windows registry (Windows only).
cmdsep
Command separator for current operating system.
Syntax
- sep = cmdsep()
Output argument
- sep - a string: on windows "&&", on linux ";"
Description
cmdsep returns the command separator for current operating system.
This function is used by Nelson to build command lines for unix and dos operating systems.
Example
unix("cd c:/ " + cmdsep() + " nelson")
See also
unix.
History
Version | Description |
---|---|
1.11.0 | initial version |
Author
Allan CORNET
computer
System information.
Syntax
- c = computer()
- [c, maxsize] = computer()
- [c, maxsize, endian] = computer()
- arch = computer('arch')
Input argument
- 'arch' - a string: returns the architecture of the computer.
Output argument
- c - a string: computer type: 'PCWIN', 'PCWIN64', 'GLNXA64', 'GLNXA32', 'MACI32', 'MACI64'
- maxsize - a integer value: maximum number of elements allowed in an array.
- endian - a string: 'L' for little-endian, 'B' for big-endian.
- arch - a string: architecture type: 'win64', 'win32', 'glnxa64', 'glnxa32', 'maci64', 'maci32'.
Description
computers identifies the type of computer that Nelson is running on.
Example
c = computer()
[c, maxsize] = computer()
[c, maxsize, endian] = computer()
arch = computer('arch')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
createGUID
Creates a GUID.
Syntax
- s = createGUID()
- c = createGUID(numbers_of_GUID)
Input argument
- numbers_of_GUID - an integer value: numbers of GUID to create.
Output argument
- s - a string
- c - a cell of strings.
Description
createGUID creates a Globally Unique IDentifier (GUID), , a unique 128-bit integer used for CLSIDs and interface identifiers.
Example
createGUID()
createGUID(10)
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
getenv
Get the value of an environment variable.
Syntax
- s = getenv(env_name)
Input argument
- env_name - string scalar, character vector, string array, cell array of character vectors: environment variable name.
Output argument
- s - string scalar, character vector, string array, cell array of character vectors: the environment variable value.
Description
getenv returns the value of an environment variable if it exists.
If the environment variable does not exist, it will return ''.
If env_name is a nonscalar cell array of character vectors or string array, then val has the same dimensions and type as env_name.
If env_name is a string scalar, then s is a character vector.
Example
getenv('OS')
getenv('myenvvar')
getenv(["PATH"; "OS"])
getenv({'PATH'; 'OS'})
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
1.4.0 | Retrieve the values of several environment variables. |
Author
Allan CORNET
hostname
get host name of this computer.
Syntax
- s = hostname()
Output argument
- s - a char array: host name.
Description
hostname get host name of this computer.
Example
hostname()
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
ismac
Checks if version is for MacOS platform.
Syntax
- s = ismac()
Output argument
- s - a logical: true if it is a MacOS platform.
Description
ismac checks if it is a MacOs platform.
Example
if ismac
disp('Your platform is MacOs')
else
disp('Your platform is not MacOs')
end
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
ispc
Checks if version is for Windows platform.
Syntax
- s = ispc()
Output argument
- s - a logical: true if it is a Windows platform.
Description
ispc checks if it is a Windows platform.
Example
if ispc
disp('Your platform is Windows')
else
disp('Your platform is not Windows')
end
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
isunix
Checks if version is for GNU Linux or Unix platform.
Syntax
- s = isunix()
Output argument
- s - a logical: true if it is a GNU Linux or Unix platform.
Description
isunix checks if it is a GNU Linux or Unix platform.
MacOs platform is also detected as a GNU Linux or Unix platform.
Example
if isunix
disp('Your platform is Unix or Linux')
else
disp('Your platform is Unix or Linux')
end
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
searchenv
Searches for a file using environment paths.
Syntax
- c = searchenv(filename, env_name)
Input argument
- env_name - a string: environment variable name.
- filename - a string: filename searched in environment variable.
Output argument
- c - a cell of strings: full paths found in environment variable.
Description
searchenv Searches for a file using environment paths.
Example
[modules, paths] = getmodules();
env_value = '';
for p = paths
env_value = [env_value, pathsep, p];
end
setenv('MY_PATH_ENV', env_value);
c = searchenv('loader.m', 'MY_PATH_ENV')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
setenv
Set the value of an environment variable.
Syntax
- getenv(env_name, env_value)
Input argument
- env_name - a string: environment variable name.
- env_value - a string: environment variable value.
Description
setenv set the value of an environment variable.
Example
setenv('MY_ENV_VAR', 'funvalue')
getenv('MY_ENV_VAR')
setenv('MY_ENV_VAR', '')
getenv('MY_ENV_VAR')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
system
dos
unix
Shell command execution.
Syntax
- status = system(command)
- status = system(command, timeout)
- status = dos(command)
- status = unix(command)
- status = unix(commands)
- [status, output, duration] = system(command)
- [status, output, duration] = dos(command)
- [status, output, duration] = unix(command)
- [status, output, duration] = system(command, '-echo')
- [status, output, duration] = dos(command, '-echo')
- [status, output, duration] = unix(command, '-echo')
- [s, outputs, duration] = unix(commands)
- [s, outputs, duration] = unix(commands, timeouts)
Input argument
- command - a string: command to execute in command shell.
- commands - a cell of string or a string array: commands to execute in command shell in parallel.
- timeout - an integer value (scalar): kill process using timeout in seconds.
- timeouts - an integer value (scalar: applied to all commands or vector: one by command): kill process using timeout in seconds.
Output argument
- status - an integer value: exit code value of the command.
- output - a string: output of the command.
- duration - integer value: duration (milliseconds).
- s - an matrix of integer value: exit code value of the commands (same dimensions than commands).
- output - a string array: output of the commands.
- duration - an matrix of integer value: duration of each execution (milliseconds).
Description
system sends a string to the operating system for execution. Standard output and standard errors of the shell command are written in the calling shell.
[status, output] = system(command, '-echo') forces the output to the Command Window, even though it is also being assigned into a variable.
Callback functions cannot be called until system command is not finished.
Nelson will convert characters to the encoding that your operating system shell accepts (ANSI on Windows by default, UTF-8 on others systems).
command can be interrupted with CTRL-C key, in this case status code returned will be 258 (WAIT_TIMEOUT) on Windows and 134 on others platforms (128 + SIGABRT)output contains 'ABORTED'.
if timeout value is 0. timeout disabled.
Examples
[s,w] = system('dir');
[s,w] = system('dir','-echo');
[s,w] = system(["echo hello", "dir", "echo world"])
tic();[s, w, d] = system(["PING -n 5 127.0.0.1>nul", "PING -n 7 127.0.0.1>nul", "PING -n 10 127.0.0.1>nul"]), toc()
tic();[s, w, d] = system(["PING -n 5 127.0.0.1>nul", "PING -n 7 127.0.0.1>nul", "PING -n 10 127.0.0.1>nul"], [1, 5, 3]), toc()
To detach an system command, include the trailing character, &, in the command argument.
[s,w] = system('notepad &');
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
username
get user name currently used.
Syntax
- s = username()
Output argument
- s - a char array: user name.
Description
username get user name currently used.
Example
username()
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
winopen
Open file in appropriate application (Windows only).
Syntax
- winopen(filename)
Input argument
- command - a string: command to execute in command shell.
Description
winopen(filename) opens filename in the appropriate Microsoft Windows application.
The winopen function uses the appropriate Windows shell command, and performs the same action as if you double-click the file in the Windows Explorer.
If filename is not in the current directory, specify the absolute path for filename.
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
winqueryreg
Read the Windows registry (Windows only).
Syntax
- c = winqueryreg ('name', rootkey, subkey)
- v = winqueryreg (rootkey, subkey, value_name)
- v = winqueryreg (rootkey, subkey)
Input argument
- rootkey - a string: root key.
- subkey - a string: subkey path.
- value_name - a string: name of value.
Output argument
- c - a cell of strings.
- v - a string or int32.
Description
c = winqueryreg ('name', rootkey, subkey) returns a cell of strings with key names in rootkey\subkey.
v = winqueryreg (rootkey, subkey, value_name) returns the value associated to value_name in rootkey\subkey.
If the value is a 32-bit integer, winqueryreg returns the value as int32. If this value is a string, it is a string.
v = winqueryreg (rootkey, subkey) returns value in rootkey\subkey that has no value name property.
Supported root keys:
'HKEY_CLASSES_ROOT', 'HKCR',
'HKEY_CURRENT_USER', 'HKCU',
'HKEY_LOCAL_MACHINE', 'HKLM',
'HKEY_USERS', 'HKU',
'HKEY_CURRENT_CONFIG', 'HKCC'
Example
winqueryreg('name', 'HKEY_LOCAL_MACHINE', 'HARDWARE\DESCRIPTION\System')
winqueryreg('HKLM', 'HARDWARE\DESCRIPTION\System\CentralProcessor\1\', 'ProcessorNameString')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
Files and folders functions
Files and folders functions
Description
files, folders and paths functions
- cd - Changes Nelson current directory.
- copyfile - Copy files or folder.
- diff_file - diff two files or strings.
- dir - Returns file list.
- fileparts - Returns the path, filename and extension of a file path.
- filesep - Return the file separator character for the current platform.
- fullfile - Build full file name from parts.
- fullpath - Returns canonical full path.
- isdir - Returns true is the input argument is an directory.
- isfile - Returns true is the input argument is a file.
- isfolder - Returns true is the input argument is an directory.
- ls - List folder contents.
- mkdir - Creates a new directory.
- pathsep - Return the search path separator character for the current platform.
- pwd - Returns current directory.
- relativepath - Returns the relative path from an actual path to the target path.
- rmdir - Removes a directory.
- rmfile - Removes a file.
- tempdir - Returns the temporary directory path.
- tempname - Returns an unique temporary filename.
- userdir - Returns the current user's path.
cd
Changes Nelson current directory.
Syntax
- cd(dirname)
- cd dirname
- previous_path = cd(dirname)
- cd ..
- cd
Input argument
- dirname - a string: directory name to move.
Output argument
- previous_path - a string: previous directory.
Description
Changes the current working directory to dirname.
a = cd() without input argument returns the current working directory.
cd() without input argument displays the current working directory.
Example
previous = cd(tempdir())
cd
cd ..
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
copyfile
Copy files or folder.
Syntax
- copyfile(source, destination)
- [status, msg] = copyfile(source, destination)
- [status, msg] = copyfile(source, destination, 'f')
Input argument
- source - a string: file or directory.
- destination - a string: file or directory.
- 'f' or 'F' - force copy even destination is not writable.
Output argument
- status - a logical true or false
- msg - a string: error message
Description
copyfile(source , destination) copies the file or directory , source (and subdirectories) to the file or directory, destination.
If source is a directory, destination can not be a file.
copyfile replaces existing files without warning.
Example
copyfile([nelsonroot(), '/etc/startup.m'], [tempdir(), 'startup.m'])
[status, msg] = copyfile([nelsonroot(), '/etc/startup.m'], [tempdir(), 'startup.m'])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
1.4.0 | input arguments support scalar string array type |
Author
Allan CORNET
diff_file
diff two files or strings.
Syntax
- res = diff(filename_1, filename_2, with_eol)
Input argument
- filename_1 - a string: a filename.
- filename_2 - a string: a filename.
- with_eol - a logical: with end of line considered or not (true by default).
Output argument
- res - a string: '' if no diff detected.
- msg - a string: error message
Description
diff_file compares two files and returns diff as unified format.
if compared files are equals, res is an empty string.
Example
res = diff_file([nelsonroot(), '/etc/startup.m'], [nelsonroot(), '/etc/startup.m'])
res = diff_file([nelsonroot(), '/etc/startup.m'], [nelsonroot(), '/etc/finish.m'])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
dir
Returns file list.
Syntax
- dir
- dir(dirname)
- dir(dirname, '-s')
- res =dir()
- res = dir(dirname)
- res = dir(dirname, '-s')
Input argument
- dirname - a string: file or directory name.
- '-s' - a string: scan also subdirectories.
Output argument
- res - a struct with fields: name, date, bytes, isdir, datenum.
Description
dir displays the list of files and folders in the current folder.
* (wildcard) is supported in filename and path name.
Example
res = dir(nelsonroot())
res = dir(nelsonroot(), '-s')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
fileparts
Returns the path, filename and extension of a file path.
Syntax
- [p, f, e] = fileparts(fullpath)
- p = fileparts(fullpath, 'path')
- f = fileparts(fullpath, 'filename')
- e = fileparts(fullpath, 'extension')
Input argument
- fullpath - a string: file or directory name.
Output argument
- p - a string: path of the directory fullpath.
- f - a string: file name without extension of fullpath.
- e - a string: extension name of fullpath.
Description
[p ,f, e] = fileparts(fullpath) splits in its three parts: path, filename, extension including the dot.
Example
[p, f, e] = fileparts([nelsonroot(), '/etc/finish.m'])
p = fileparts([nelsonroot(), '/etc/finish.m'], 'path')
f = fileparts([nelsonroot(), '/etc/finish.m'], 'filename')
e = fileparts([nelsonroot(), '/etc/finish.m'], 'extension')
See also
isdir, isfile, pathsep, filesep.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
filesep
Return the file separator character for the current platform.
Syntax
- res = filesep()
Output argument
- res - a string: '/' or ''
Description
pathsep returns '' on Windows and '/' on others platforms.
Example
A = filesep
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
fullfile
Build full file name from parts.
Syntax
- R = fullfile(part1, ... , partN)
Input argument
- part1, ... , partN - a string or cell of string: filename to concat.
Output argument
- R - a character array or string array or cell array of character vectors.
Description
R = fullfile(part1, ... , partN) build full file name from parts.
Example
fullfile([nelsonroot(), '/./toto'])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
fullpath
Returns canonical full path.
Syntax
- R = fullpath(path)
Input argument
- path - a string or cell of string: filename to normalize.
Output argument
- R - a string or cell of string: canonical paths.
Description
fullpath(path) returns full path from a relative path.
Example
fullpath([nelsonroot(), '/../toto'])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
isdir
Returns true is the input argument is an directory.
Syntax
- r = isdir(dirname)
Input argument
- dirname - a string: directory name to check.
Output argument
- r - a logical: true if it is an directory.
Description
isdir(dirname) returns true if dirname is a directory.
isdir and isfolder are same.
Example
isdir(nelsonroot())
isdir([nelsonroot(), '/not_exist_dir'])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
isfile
Returns true is the input argument is a file.
Syntax
- r = isfile(name)
Input argument
- name - a string: filename to check.
Output argument
- r - a logical: true if it is a file.
Description
isfile(name) returns true if name is a file.
Example
isfile(nelsonroot())
isfile([nelsonroot(), '/etc/finish.m'])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
1.4.0 | input arguments support scalar string array type |
Author
Allan CORNET
isfolder
Returns true is the input argument is an directory.
Syntax
- r = isfolder(dirname)
Input argument
- dirname - a string: directory name to check.
Output argument
- r - a logical: true if it is an directory.
Description
isfolder(dirname) returns true if dirname is a directory.
Example
isdir(nelsonroot())
isdir([nelsonroot(), '/not_exist_dir'])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
ls
List folder contents.
Syntax
- ls
- ls(name)
- res = ls()
- res = ls(options)
Input argument
- name - a string: file or directory name.
- options - vary from system to system.
Output argument
- res - On Windows, res is an m-by-n character array of names. m is the number of names and n is the number of characters in the longest name. On Unix plaftorms is a character vector of names separated by tab and space characters.
Description
ls is implemented by calling the native operating system's directory listing command—available options will vary from system to system.
Example
res = ls(nelsonroot())
if ~ispc()
res = ls(nelsonroot(), '-l')
end
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
mkdir
Creates a new directory.
Syntax
- mkdir(dirname)
- mkdir(parentdir, dirname)
- status = mkdir(dirname)
- status = mkdir(parentdir, dirname)
- [status, msg] = mkdir(dirname)
- [status, msg] = mkdir(parentdir, dirname)
Input argument
- dirname - a string: directory name to create
- parentdir - a string: a directory in which the dirname directory will be created
Output argument
- status - a logical true or false
- msg - a string: error message
Description
Creates a directory named dirname in the directory parent.
If no parent directory is specified the present working directory is used.
If directory is created or already existing, status is true, otherwise it will be false.
Example
mkdir(tempdir(), 'subdir_example')
if isdir([tempdir(), 'subdir_example'])
disp('OK')
else
disp('NOT OK')
end
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
1.4.0 | input arguments support scalar string array type |
Author
Allan CORNET
pathsep
Return the search path separator character for the current platform.
Syntax
- res = pathsep()
Output argument
- res - a string: ';' or ':'
Description
pathsep returns ';' on Windows and ':' on others platforms.
Example
A = pathsep
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
pwd
Returns current directory.
Syntax
- pwd()
- r = pwd()
Output argument
- r - a string: current directory.
Description
Returns the current working directory.
pwd() without input argument displays the current working directory.
Example
r = pwd()
pwd()
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
relativepath
Returns the relative path from an actual path to the target path.
Syntax
- r = relativepath(path_1, path_2)
Input argument
- path_1 - a string: file or directory.
- path_2 - a string: file or directory.
Output argument
- r - a string: relative path.
Description
Returns the relative path from an actual path to the target path.
Example
relativepath(nelsonroot(), [nelsonroot(), '/lgpl-3.0.md'])
relativepath(nelsonroot(), [nelsonroot(), '/etc/finish.m'])
relativepath([nelsonroot(),'/bin'], [nelsonroot(), '/lgpl-3.0.md'])
relativepath('.', '.')
relativepath('.', '..')
relativepath('..', '.')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
rmdir
Removes a directory.
Syntax
- rmdir(dirname)
- rmdir(dirname, 's')
- res = rmdir(dirname)
- res = rmdir(dirname, 's')
- [res, msg] = rmdir(dirname)
- [res, msg] = rmdir(dirname, 's')
Input argument
- dirname - a string: file or directory name.
- 's' - a string: removes also subdirectories.
Output argument
- res - a logical: true or false.
- msg - a string: error message or ''.
Description
res = rmdir(dirname) removes the directory dirname.
If the directory is not empty, you must use the s argument.
Example
mkdir([tempdir(), 'test'])
rmdir([tempdir(), 'test'])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
rmfile
Removes a file.
Syntax
- rmfile(filename)
- res = rmfile(filename)
- [res, msg] = rmfile(filename)
- [res, msg] = rmfile(filename)
Input argument
- filename - a string: file name.
Output argument
- res - a logical: true or false.
- msg - a string: error message or ''.
Description
res = rmfile(filename) removes the file filename.
Example
fd = fopen([tempdir(), 'test_rmfile.txt'], 'wt')
fclose(fd)
isfile([tempdir(), 'test_rmfile.txt'])
rmfile([tempdir(), 'test_rmfile.txt'])
isfile([tempdir(), 'test_rmfile.txt'])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
tempdir
Returns the temporary directory path.
Syntax
- tempdir()
- p = tempdir()
Output argument
- p - a string: current temporary directory.
Description
Returns the name of the host system’s directory for temporary files.
Example
r = tempdir()
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
tempname
Returns an unique temporary filename.
Syntax
- f = tempname()
- f = tempname(path)
Input argument
- path - a string: an existing directory used instead of tempdir().
Output argument
- f - a string: an unique temporary filename.
Description
Returns the name of an unique temporary filename.
Example
r = tempname()
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
userdir
Returns the current user's path.
Syntax
- userdir()
- p = userdir()
Output argument
- p - a string: current user directory.
Description
Returns the name of the user's directory.
Example
r = userdir()
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
Memory manager functions
Memory manager functions
Description
memory manager functions
- acquirevar - Acquires variable value from a specified variables scope.
- assignin - Assignin value to a variable in a specified variables scope.
- clear - Remove variable from workspace.
- global - Defines a global variable.
- isglobal - Checks if a variable is global.
- isvar - Check for the existence of an variable.
- memory - Get memory information.
- persistent - Persistent variable.
- varislock - Checks if a variable is locked.
- varlock - Locks a variable.
- varunlock - Unlocks a variable.
- who - List variables in memory or in .nh5 or in .mat file.
- whos - List variables in memory or in .nh5 or in .mat file with sizes and types.
acquirevar
Acquires variable value from a specified variables scope.
Syntax
- value = acquirevar(scope, variable_name)
Input argument
- scope - a string: 'global', 'base', 'caller', 'local'.
- variable_name - a string: the name of symbol to search.
Output argument
- value - value of the variable searched.
Description
acquirevar search a symbol in a specific scope and copy the value in current scope.
Example
Y = 'variable in base scope';
function myfun()
disp(acquirevar('base', 'Y')
end
myfun()
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
assignin
Assignin value to a variable in a specified variables scope.
Syntax
- assignin(scope, variable_name, variable_value)
Input argument
- scope - a string: 'global', 'base', 'caller', 'local'.
- variable_name - a string: the name of variable destination.
- variable_value - a variable to assign.
Description
assignin assign value to a variable in a specified variables scope.
Example
assignin('base', 'X', 33);
Y = acquirevar('base', 'X');
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
clear
Remove variable from workspace.
Syntax
- clear
- clear variable_name
- clear global
- clear all
- clear mex
- clear variables
- clear functions
- clear function_name
- clear mexfunction_name
- clear variable_name_1 ... variable_name_N
- clear global variable_name
Input argument
- variable_name - a string: variable name.
- global - clears all global variables.
- all - clears all variables in all scopes
- mex - clears all mex functions in all scopes
- variables - clears all variables in current scope.
- functions - clears cache of macros functions and associated persistent variables.
- function_name - clears persistent variables of a function.
- mexfunction_name - clears mex function (see mexAtExit).
Description
clear is used to remove variable given by its name.
clear can also delete handle object if a function handle_TYPE_clear is defined.
Example
A = 3;
who
clear A
who
A
See also
who.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
global
Defines a global variable.
Syntax
- global variable_name
- global(variable_name)
- global variable_name1 ... variable_nameN
Input argument
- variable_name - a string: valid variable name.
Description
global make variable in global assign value to a variable in a specified variables scope.
Example
function myfun()
global y;
y = 1;
end
myfun()
who
global y
who
disp(y)
who
clear global y
disp(y)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
isglobal
Checks if a variable is global.
Syntax
- state = isglobal(variable_name)
Input argument
- variable_name - a string: variable name.
Output argument
- state - a logical: true if variable is global.
Description
isglobal returns true if variable_name has been declared as global variable and false otherwise.
Example
y = 3;
isglobal y
global b
b = 3
isglobal b
clear global b
isglobal b
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
isvar
Check for the existence of an variable.
Syntax
- tf = isvar(varname)
- tf = isvar(scope, varname)
Input argument
- scope - a string: 'global', 'base', 'caller', 'local'.
- varname - a string: variable name.
Output argument
- tf - a logical: true if varname exists.
Description
isvar checks for the existence of an variable.
Example
isvar('A')
A = 3
isvar('A')
isvar('global','B')
global B
isvar('global','B')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
memory
Get memory information.
Syntax
- memory
- usermem = memory()
- [usermem, systemmem] = memory()
Output argument
- usermem - Get information about user memory (an struct).
- systemmem - Get information about system memory (an struct)
Description
memory get memory information.
User Memory: returns Maximum Possible Array (MaxPossibleArrayBytes), Memory Available for All Arrays (MemAvailableAllArrays), Memory Used By Nelson (MemUsedNelson).
System Memory:
VirtualAddressSpace.Available: available swap file space
VirtualAddressSpace.Total: total swap file space
SystemMemory.Available: available system memory
PhysicalMemory.Available: available physical memory
PhysicalMemory.Total: total physical memory
Examples
memory()
A = ones(1000);
memory()
clear('A');
[u1, s1] = memory();
A = ones(1000);
[u2, s2] = memory();
disp(u2.MemUsedNelson - u1.MemUsedNelson);
clear('A');
[u3, s3] = memory();
disp(u3.MemUsedNelson - u2.MemUsedNelson);
[u1, s1] = memory()
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
persistent
Persistent variable.
Syntax
- persistent variable_name
- persistent('variable_name')
- persistent variable_name1, ..., variable_nameN
Input argument
- variable_name - a string: variable name.
Description
persistent defines a variable defined by his name variable_name as persistent in a function.
Before to use a persistent variable, it is necessary to initializ value.
Examples
function to define:
function r = test_persistent_function()
persistent calls;
if isempty(calls)
calls = 0;
end
disp(['nb calls to test_persistent_function: ', int2str(calls)]);
r= calls;
calls = calls + 1;
end
calls test_persistent_function
for i = 1:30
r = test_persistent_function();
end
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
varislock
Checks if a variable is locked.
Syntax
- state = varislock(scope, variable_name)
Input argument
- scope - a string: 'global', 'base', 'caller', 'local'.
- variable_name - a string: variable name.
Description
varislock returns true if variable_name has been declared as locked variable and false otherwise.
Example
y = 3;
varislock('local', 'y')
varlock('local', 'y')
varislock('local', 'y')
y = 4
varunlock('local', 'y')
varislock('local', 'y')
y = 4
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
varlock
Locks a variable.
Syntax
- varlock(scope, variable_name)
Input argument
- scope - a string: 'global', 'base', 'caller', 'local'.
- variable_name - a string: variable name.
Description
varlock locks a variable.
Locked variables cannot be killed.
ans variable cannot be locked.
Example
y = 3;
varislock('local', 'y')
varlock('local', 'y')
varislock('local', 'y')
y = 4
varunlock('local', 'y')
varislock('local', 'y')
y = 4
varlock('local', 'ans')
varislock('local', 'ans')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
varunlock
Unlocks a variable.
Syntax
- varunlock(scope, variable_name)
Input argument
- scope - a string: 'global', 'base', 'caller', 'local'.
- variable_name - a string: variable name.
Description
varunlock unlocks a variable.
Example
y = 3;
varislock('local', 'y')
varlock('local', 'y')
varislock('local', 'y')
y = 4
varunlock('local', 'y')
varislock('local', 'y')
y = 4
varlock('local', 'ans')
varislock('local', 'ans')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
who
List variables in memory or in .nh5 or in .mat file.
Syntax
- who
- s = who()
- who(scope)
- s = who(scope)
- who('-file', filename)
- s = who('-file', filename)
- who(... , var1, ..., varN)
- s = who(... , var1, ..., varN)
Input argument
- scope - a string: 'global', 'base', 'caller', 'local' or '-file'.
- filename - string: an existing filename .nh5 or .mat file.
- var1, ..., varN - string: variable name.
Output argument
- s - a cell of strings: list of variable's name.
Description
who displays current variable names.
Example
clear
who
A = 3
b= 3
who
s = who()
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
whos
List variables in memory or in .nh5 or in .mat file with sizes and types.
Syntax
- whos
- s = whos()
- whos(scope)
- s = whos(scope)
- whos('-file', filename)
- s = whos('-file', filename)
- whos(... , var1, ..., varN)
- s = whos(... , var1, ..., varN)
Input argument
- scope - a string: 'global', 'base', 'caller', 'local'.
- var1, ..., varN - a string: variable name.
- filename - string: an existing filename .nh5 or .mat file.
Output argument
- st - stores information about the variables in the structure array s.
Description
whos displays current variable names in memory or in .nh5 or .mat file.
Example
clear
whos
A = 3
b= 3
whos
s = whos()
save([tempdir(), 'example_who.nh5'], 'A', 'b')
whos([tempdir(), 'example_who.nh5'])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
Time functions
Time functions
Description
time functions
- addtodate - Modify date number by field.
- calendar - Calendar.
- clock - Return the current local date and time as a date vector.
- cputime - Return the CPU time used by your Nelon session.
- date - Return the Current date as character vector.
- datenum - Return the date/time input as a serial day number.
- datestr - Convert date and time to string format.
- datevec - Convert a serial date number into a date vector.
- eomday - Returns last day of month.
- etime - Time elapsed between date vectors.
- hour - Hours part of the input date and time.
- leapyear - Determine leap year.
- minute - Minutes part of the input date and time.
- now - Returns current date under the form of a Unix hour.
- second - Seconds part of the input date and time.
- sleep - Suspend code execution.
- tic - Starts a stopwatch timer.
- time - Return the current time as the number of seconds or nanoseconds since the epoch.
- timeit - Measure time required to run function.
- toc - Read the stopwatch timer.
- weekday - Return the day of week.
addtodate
Modify date number by field.
Syntax
- r = addtodate(d, q, f)
Input argument
- d - serial datenum.
- q - quantile to add to date
- f - 'year', 'month', 'day', 'hour', 'minute', 'second', or 'millisecond.
Output argument
- r - date number.
Description
r = addtodate(d, q, f) adds quantity q to the indicated date field f of a scalar serial date number d, returning the updated date number r.
Example
t = datenum('07-Apr-2008 23:00:00');datevec(t)
t2 = addtodate(t, -2, 'hour');datevec(t2)
t3 = addtodate(t, 4, 'hour');datevec(t3)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
calendar
Calendar.
Syntax
- calendar()
- c = calendar()
- c = calendar(d)
- c = calendar(y, m)
Input argument
- d - an integer value: a serial date number.
- y - an integer value: 'year' desired [1400, 9999].
- m - an integer value: 'month' desired [1, 12].
Output argument
- c - a 6x7 matrix.
Description
calendar() returns the currently monthly calendar.
If no output arguments are specified,the calendar is displayed on the screen instead of returning a matrix 6x7.
Example
calendar()
c = calendar(1973, 8)
c = calendar(datenum(1973, 8, 4))
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
clock
Return the current local date and time as a date vector.
Syntax
- v = clock()
Output argument
- v - a vector: [year, month, day, hours, minutes, seconds].
Description
calendar() returns the currently monthly calendar.
The date vector contains the following fields:
year
months [1, 12]
days [1, 31]
hours [0, 23]
minutes [0, 59]
seconds [0, 61]
seconds: field has a fractional part after the decimal point for extended accuracy.
To time the duration of an event, use tic and toc functions instead of clock.
The clock function is based on the system time and thus might not be reliable for time comparison operations.
Example
c = clock()
fix(c)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
cputime
Return the CPU time used by your Nelon session.
Syntax
- t = cputime()
Output argument
- t - a double: time in seconds.
Description
cputime() returns the CPU time used by Nelson session.
To measure performance, it is better to use tic and toc functions.
Example
t1 = cputime;
sleep(10);
t2 = cputime;
t2 - t1
% versus tic toc
tic()
sleep(10);
toc()
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
date
Return the Current date as character vector.
Syntax
- d = date()
Output argument
- d - a string: date string using format dd-MMM-yyy. MMM: English abbreviation for the month name.
Description
d = date() returns the current date as a character vector in the format dd-MMM-yyyy.
Example
d = date()
fix(c)
See also
now.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
datenum
Return the date/time input as a serial day number.
Syntax
- d = datetnum(datevec)
- d = datenum(datestr)
- d = datenum(datestr, format)
- d = datenum(datestr, pivotYear)
- d = datenum(DateString,format,pivotYear)
- d = datetnum(Y, M, D)
- d = datetnum(Y, M, D, H, MN, S)
Input argument
- datevec - a vector: [Y, M, D, H, MN, S] or matrix N x 6.
- format - a string specifying the date format, or leave it empty ('') for automatic format detection.
- datestr - a string, cell of string or string array: text representing a date.
- Y, M, D, H, MN, S - double: Year, Month, Day, Hours, Minutes, Secondes (scalar or vector).
- pivotYear: Start year of 100-year date range - integer value or present minus 50 years (default).
Output argument
- d - a double: serial date number (serial day 1 corresponds to 1-Jan-0000).
Description
d = datenum() returns the serial date number corresponding to current date.
d = datenum(datevec) converts date vector to serial date number.
d = datenum(datestr) and d = datenum(datestr, format) converts string to serial date number.
Supported format conversion:
dd-mmm-yyyy HH:MM:SS 10-Mar-2010 16:48:17
dd-mmm-yyyy 10-Mar-2010
mm/dd/yyyy 03/10/2010
mm/dd/yy 03/10/00
mm/dd 03/10
mmm.dd,yyyy HH:MM:SS Mar.10,2010 16:48:17
mmm.dd,yyyy Mar.10,2010
yyyy-mm-dd HH:MM:SS 2010-03-10 16:48:17
yyyy-mm-dd 2010-03-10
yyyy/mm/dd 2000/03/10
HH:MM:SS 16:48:17
HH:MM:SS PM 3:48:17 PM
HH:MM 16:48
HH:MM PM 3:35 PM
If format is not specified, the default format is dd-mmm-yyyy.
If format is specified and not using predefined format, the format must be specified as a character vector or string scalar composed of symbolic identifiers.
The format of the input text for representing dates and times, expressed as a character vector or string scalar composed of symbolic identifiers.
Symbolic Identifier | Description | Example |
---|---|---|
yyyy | Year in full | 1995, 2012 |
yy | Year in two digits | 89, 01 |
Quarter year using letter Q and one digit | Q1 | |
mmmm | Month using full name | March, December |
mmm | Month using first three letters | Mar, Dec |
mm | Month in two digits | 04, 12 |
m | Month using capitalized first letter | M, D |
dddd | Day using full name | Monday, Tuesday |
ddd | Day using first three letters | Mon, Tue |
dd | Day in two digits | 06, 21 |
d | Day using capitalized first letter | M, T |
HH | Hour in two digits (no leading zeros when symbolic identifier AM or PM is used) | 06, 6 AM |
MM | Minute in two digits | 11, 01 |
SS | Second in two digits | 06, 59 |
FFF | Millisecond in three digits | 056 |
AM or PM | AM or PM inserted in text representing time | 5:46:02 PM |
Example
d = datenum([1973,8,4,12,1,18])
datevec(d)
d = datenum('04–Aug-1973 12:01:18')
d = datenum(["04–Aug-1973 12:01:18"; "04–Aug-1974 11:01:18"])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
1.8.0 | date string parsing extended. |
1.10.0 | added: format '' means try to detect. |
Author
Allan CORNET
datestr
Convert date and time to string format.
Syntax
- dateAsString = datestr(dateVector)
- dateAsString = datestr(dateNumber)
- dateAsString = datestr(..., formatOut)
- dateAsString = datestr(dateAsStringIn)
- dateAsString = datestr(dateAsStringIn, formatOut, pivotYear)
- dateAsString = datestr(..., 'local')
Input argument
- dateVector - Date vectors or matrix.
- dateNumber - Serial date numbers: An array of positive double-precision floating-point numbers.
- formatOut - character vector, string scalar or integer value (-1 default): Output format for representing dates and times
- dateAsStringIn - character vector, cell array or string array: text denoting dates and times to convert.
- pivotYear - integer value: present minus 50 years (default).
- 'local' - returns the date in the language of the current locale.
Output argument
- dateAsString - character vector or two-dimensional character array: text denoting dates and time.
Description
dateAsString = datestr(dateVector) converts date vectors into text that represents the corresponding dates and times. It returns a character array with m rows, where m is the number of date vectors in dateVector.
dateAsString = datestr(dateNumber) converts serial date numbers into text representing dates and times. The output is a character array with m rows, where m is the number of date numbers in dateNumber.
dateAsString = datestr(..., formatOut) allows you to specify the format of the output text using formatOut. You can apply this option with any of the previous input types.
dateAsString = datestr(dateAsStringIn) converts the input string dateAsStringIn into a text format of day-month-year hour:minute:second. All dates in dateAsStringIn must follow the same format.
dateAsString = datestr(dateAsStringIn, formatOut, pivotYear) converts dateAsStringIn into the format specified by formatOut, while using an optional pivotYear to interpret two-digit years.
dateAsString = datestr(..., 'local') returns the date in the language of the system's current locale. If 'local' is omitted, the default language is US English. The 'local' option can be used with any of the previous syntaxes, and must be the last argument in the sequence.
Supported format conversion:
dd-mmm-yyyy HH:MM:SS 10-Mar-2010 16:48:17
dd-mmm-yyyy 10-Mar-2010
mm/dd/yyyy 03/10/2010
mm/dd/yy 03/10/00
mm/dd 03/10
mmm.dd,yyyy HH:MM:SS Mar.10,2010 16:48:17
mmm.dd,yyyy Mar.10,2010
yyyy-mm-dd HH:MM:SS 2010-03-10 16:48:17
yyyy-mm-dd 2010-03-10
yyyy/mm/dd 2000/03/10
HH:MM:SS 16:48:17
HH:MM:SS PM 3:48:17 PM
HH:MM 16:48
HH:MM PM 3:35 PM
If format is not specified, the default format is dd-mmm-yyyy.
If format is specified and not using predefined format, the format must be specified as a character vector or string scalar composed of symbolic identifiers.
The format of the input text for representing dates and times, expressed as a character vector or string scalar composed of symbolic identifiers.
Symbolic Identifier | Description | Example |
---|---|---|
yyyy | Year in full | 1995, 2012 |
yy | Year in two digits | 89, 01 |
Quarter year using letter Q and one digit | Q1 | |
mmmm | Month using full name | March, December |
mmm | Month using first three letters | Mar, Dec |
mm | Month in two digits | 04, 12 |
m | Month using capitalized first letter | M, D |
dddd | Day using full name | Monday, Tuesday |
ddd | Day using first three letters | Mon, Tue |
dd | Day in two digits | 06, 21 |
d | Day using capitalized first letter | M, T |
HH | Hour in two digits (no leading zeros when symbolic identifier AM or PM is used) | 06, 6 AM |
MM | Minute in two digits | 11, 01 |
SS | Second in two digits | 06, 59 |
FFF | Millisecond in three digits | 056 |
AM or PM | AM or PM inserted in text representing time | 5:46:02 PM |
Examples
dateVector = [2019, 4, 2, 9, 7, 18];
datestr(dateVector)
dateVector = [2019, 4, 2, 9, 7, 18];
formatOut = 'mm/dd/yy';
datestr(dateVector, formatOut)
datestr(now, 'mmmm dd, yyyy HH:MM:SS.FFF AM')
datestr('06:33 PM','HH:MM')
datestr('06:33','HH:MM PM')
formatOut = 'dd mmm yyyy';
datestr(datenum('18-05-45','dd-mm-yy',1900),formatOut)
datestr(datenum({'09/17/2017';'06/14/1906';'10/29/2014'}, 'mm/dd/yyyy')))
dateStringIn = '5/17/56';
formatOut = 1;
pivotYear = 1900;
datestr(dateStringIn, formatOut, pivotYear)
pivotYear = 2000;
datestr(dateStringIn,formatOut, pivotYear)
See also
History
Version | Description |
---|---|
1.8.0 | initial version |
Author
Allan CORNET
datevec
Convert a serial date number into a date vector.
Syntax
- [Y, M, D, H, MN, S] = datevec(dv)
- V = datevec(dv)
Input argument
- dv - a scalar, vector, or multidimensional array e: a serial date number.
Output argument
- Y, M, D, H, MN, S - double: Year, Month, Day, Hour, Minutes, Seconds.
- V - a vector of double: [Year, Month, Day, Hour, Minutes, Seconds].
Description
datevec converts a serial date number into a date vector.
To measure performance, it is better to use tic and toc functions.
Example
datevec(now())
datevec(720840)
V = datevec([720840, now()])
[Y, M, D, H, MN, S] = datevec([720840, now()])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
eomday
Returns last day of month.
Syntax
- E = eomday(Y, M)
Input argument
- Y - year: real.
- M - month: real.
Output argument
- E - last day of month: real.
Description
E = eomday(Y, M) returns the last day of the month M for the year Y.
Example
eomday(1900, 1:12)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
etime
Time elapsed between date vectors.
Syntax
- e = etime(t2, t1)
Input argument
- t2 - Date vectors: 1-by-6 vector or m-by-6 matrix.
- t1 - Date vectors: 1-by-6 vector or m-by-6 matrix.
Output argument
- e - a scalar or a vector: time elapsed (seconds).
Description
e = etime(t2, t1) returns the number of seconds between two date vectors or matrices of date vectors, t1 and t2.
Example
t1 = clock()
sleep(6)
t2 = clock()
etime(t2, t1)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
hour
Hours part of the input date and time.
Syntax
- h = hour(t)
- h = hour(t, formatIn)
Input argument
- t - serial date number or text inputs
- formatIn - valid date format
Output argument
- h - a double: integer value.
Description
h = hour(t) extracts the hour component from each date and time specified in t.
The output h is a double array containing integer values ranging from 0 to 23.
Example
h = hour(738427.656845093)
h = hour("2021/09/28 15:45:51", 'YYYY/M/DD HH:MM:SS')
See also
History
Version | Description |
---|---|
1.10.0 | initial version |
Author
Allan CORNET
leapyear
Determine leap year.
Syntax
- tf = leapyear(year)
Input argument
- year - year: scalar or array numeric value.
Output argument
- tf - Leap year determination result: scalar or array logical value.
Description
leapyear determines leap years.
Leap years is done by Gregorian calendar rules.
Example
tf = leapyear([2020 2021 2022])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
minute
Minutes part of the input date and time.
Syntax
- m = minute(t)
- m = minute(t, formatIn)
Input argument
- t - serial date number or text inputs
- formatIn - valid date format
Output argument
- m - a double: integer value.
Description
m = minute(t) extracts the minute component from each date and time specified in t.
The output m is a double array containing integer values ranging from 0 to 59.
Example
m = minute(738427.656845093)
m = minute("2021/09/28 15:45:51", 'YYYY/M/DD HH:MM:SS')
See also
History
Version | Description |
---|---|
1.10.0 | initial version |
Author
Allan CORNET
now
Returns current date under the form of a Unix hour.
Syntax
- n = now()
Output argument
- n - a double.
Description
now() returns the current date and time as a serial date number.
Example
datevec(now())
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
second
Seconds part of the input date and time.
Syntax
- s = second(t)
- s = second(t, formatIn)
Input argument
- t - serial date number or text inputs
- formatIn - valid date format
Output argument
- s - a double: integer value.
Description
s = second(t) extracts the second component from each date and time specified in t.
The output s is a double array containing integer values ranging from 0 to 59.
Example
s = second(738427.656845093)
s = second("2021/09/28 15:45:51", 'YYYY/M/DD HH:MM:SS')
See also
History
Version | Description |
---|---|
1.10.0 | initial version |
Author
Allan CORNET
sleep
Suspend code execution.
Syntax
- sleep(sec)
Input argument
- n - a double: duration of the sleep in seconds (decimal number).
Description
sleep stops Nelson processing any instruction for a speficied number of seconds.
CTRL-C interruption stops sleep.
Example
tic();sleep(1);toc()
tic();sleep(0.1);toc()
tic();sleep(0.01);toc()
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
tic
Starts a stopwatch timer.
Syntax
- tic()
- t = tic()
Output argument
- t - a unsigned integer 64 bit: value of internal timer of the tic function.
Description
The sequence of commands tic(); commands ; t = toc() returns the number of seconds required for the commands.
Consecutive tic commands overwrite the tic timer.
Example
tic()
sleep(10)
toc()
tic()
sleep(10)
t = toc()
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
time
Return the current time as the number of seconds or nanoseconds since the epoch.
Syntax
- t_s = time()
- t_s = time('s')
- t_ns = time('ns')
Output argument
- t_s - a double: value of current time as the number of seconds since the epoch.
- t_ns - a unsigned integer 64 bit: value of current time as the number of nanoseconds since the epoch.
Description
time returns the current time as the number of seconds or nanoseconds since the epoch.
The epoch is referenced to 00:00:00 UTC (Coordinated Universal Time) 1 Jan 1970.
Example
t1=time()
sleep(10)
t2 = time()
t2 - t1
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
timeit
Measure time required to run function.
Syntax
- t = timeit(f)
- t = timeit(f, nLhs)
- t = timeit(f, nLhs, x1, ..., xm)
Input argument
- f - Function handle: Function to run.
- nLhs - integer value: number of output arguments. (1: default)
- x1, ..., xm - Input arguments, specified as a comma-separated list of variables or expressions.
Output argument
- t - time (in seconds).
Description
t = timeit(f) measures the time elapsed required to run the function specified by the function handle f.
To perform a robust measurement, timeit calls function multiple times and returns the median of the measurements.
If the function runs fast, timeit might call the function many times.
Examples
f = str2func('@()sleep(6)');
tic();t = timeit(f), toc()
X = rand(100);
f = str2func('@(X) svd(X);');
tic(), t1 = timeit(f, 1, X), toc()
tic(), t2 = timeit(f, 3, X), toc()
See also
tic.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
toc
Read the stopwatch timer.
Syntax
- toc()
- t = toc()
- toc(timer_value)
- t = toc(timer_value)
Input argument
- timer_value - a unsigned integer 64 bit: value of internal timer of the tic function.
Output argument
- t - a double: number of seconds since last call to tic function (Precision in order of millisecond).
Description
The sequence of commands tic(); commands ; t = toc() returns the number of seconds required for the commands.
Consecutive calls to the toc function with no input return the elapsed since the most recent tic.
Consecutive calls to the toc function with the same timerVal input return the elapsed time since the tic function call that corresponds to that input.
Example
tic()
sleep(10)
toc()
sleep(10)
toc()
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
weekday
Return the day of week.
Syntax
- number = weekday(D)
- [number, name] = weekday(D)
- [number, name] = weekday(D, form)
- [number, name] = weekday(D, language)
- [number, name] = weekday(D, form, language)
Input argument
- D - Serial date numbers or text representing dates and times (vector, matrix, character vector, cell array of character vectors, string array or character array).
- form - a string: 'short' (default) or 'long'.
- language - a string: 'en_US' (default) or 'local'.
Output argument
- number - array of integers values in the range [1, 7].
- name - character array. if 'local' output uses current local if day name is available as translation.
Description
dayweek returns the day of the week as a number in number and as a string in name.
Example
[DayNumber, DayName] = weekday(datenum('12-21-2012'), 'long', 'en_US')
[DayNumber, DayName] = weekday(datenum('12-21-2012'), 'long', 'local')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
Random
Random
Description
random functions
- rand - Random Number.
- randn - Normally distributed random number.
- randperm - Random permutation of integers values.
- rng - Random Number Generator.
rand
Random Number.
Syntax
- M = rand
- M = rand(n)
- M = rand(x1, x2, ... , xN)
- M = rand(sz)
- M = rand(x1, x2, ... , xN, classname)
- M = rand(x1, x2, ... , xN, 'like', var)
Input argument
- n - a variable: n-by-n matrix will be generated.
- x1, x2, ... , xN - x1-by-...-by-xN values
- classname - a string: 'single' or 'double'
- var - a variable: single or double
Output argument
- M - a matrix of random numbers.
Description
rand returns a matrix with random elements uniformly distributed on the interval [0, 1].
seed can be modified using rng.
The Mersenne Twister designers consider 5489 as default seed. Nelson uses it as default seed (0).
Bibliography
M. Matsumoto and T. Nishimura, Mersenne Twister: A 623-dimensionally equidistributed uniform pseudorandom number generator, ACM Trans. on Modeling and Computer Simulation Vol. 8, No. 1, pp. 3–30, January 1998
Examples
rng('default');
rand
rng('default');
rand
rng('default');
rand(6)
rng('default');
rand(3, 2, 3)
rng('default');
rand(3, 2, 'single')
rng('default');
v = single([3, 3]);
rand(3, 2, 'like', v)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
randn
Normally distributed random number.
Syntax
- M = randn
- M = randn(n)
- M = randn(x1, x2, ... , xN)
- M = randn(sz)
- M = randn(x1, x2, ... , xN, classname)
- M = randn(x1, x2, ... , xN, 'like', var)
Input argument
- n - a variable: n-by-n matrix will be generated.
- x1, x2, ... , xN - x1-by-...-by-xN values
- classname - a string: 'single' or 'double'
- var - a variable: single or double
Output argument
- M - a matrix of random numbers.
Description
randn returns a matrix with normally distributed random elements having zero mean and variance one.
By default, randn uses the ziggurat algorithm implemented by Boost.
seed can be modified using rng.
Examples
rng('default');
randn
rng('default');
randn
rng('default');
randn(6)
rng('default');
randn(3, 2, 3)
rng('default');
randn(3, 2, 'single')
rng('default');
v = single([3, 3]);
randn(3, 2, 'like', v)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
randperm
Random permutation of integers values.
Syntax
- p = randperm(n)
Input argument
- n - Number of integers in sample interval (positive integer).
Output argument
- p - a row vector.
Description
p = randperm(n) returns a row vector containing a random permutation of 1:n.
Example
randperm(7)
See also
rand.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
rng
Random Number Generator.
Syntax
- lst = rng('enginelist')
- rng('default')
- s = rng('default')
- rng('shuffle')
- s = rng('shuffle')
- rng(seed)
- s = rng(seed)
- rng(seed, generator)
- s = rng(seed, generator)
- rng('shuffle', generator)
- s = rng('shuffle', generator)
- s = rng
- rng(s)
Input argument
- seed - an integer value: new seed for random generator
- generator - a string: 'twister', 'twister64', 'laggedfibonacci607'
- s - a struct
Output argument
- lst - a cell of strings.
- s - a struct
Description
lst = rng('enginelist') returns the list of available random number generator.
rng('default') puts the settings of the random number generator to default values.
s = rng('default') puts the settings of the random number generator to default values.
rng('shuffle') puts the settings of the random number generator to default values and returns previous generator as an struct.
s = rng('shuffle') seeds the random number generator based on the current time.
rng(seed) seeds the random number generator using the nonnegative integer.
s = rng(seed) seeds the random number generator using the nonnegative integer and returns previous generator as an struct.
rng(seed, generator) seeds the random number generator using the nonnegative integer and specify also the type of generator used.
s = rng(seed, generator) seeds the random number generator using the nonnegative integer and specify also the type of generator used and returns previous generator as an struct.
rng('shuffle', generator) seeds the random number generator based on the current time and specify also the type of generator used.
s = rng('shuffle', generator) seeds the random number generator based on the current time,specify also the type of generator used and returns previous generator as an struct.
s = rng returns current generator as an struct.
rng(s) restores the settings of the random number generator using a previous struct returned by s = rng.
Example
rng('default');
r = rng()
lst = rng('enginelist')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
Message Passing Interface
Message Passing Interface
Description
In the world of parallel computing the Message Passing Interface (MPI) is the de facto standard for implementing programs on multiple processors.
- MPI_Allreduce - Combines values from all processes and distributes the result back to all processes.
- MPI_Barrier - Blocks until all processes in the communicator have reached this routine.
- MPI_Bcast - Broadcasts a message from the process with rank "root" to all other processes of the communicator
- MPI_Comm_delete - Removes MPI_Comm object.
- MPI_Comm_get_name - Return the print name from the communicator.
- MPI_Comm_object - Creates MPI_Comm object.
- MPI_Comm_rank - Determines the rank of the calling process in the communicator.
- MPI_Comm_size - Determines the size of the group associated with a communicator.
- MPI_Comm_split - Partitions the group that is associated with the specified communicator into a specified number of disjoint subgroups.
- MPI_Comm_used - Returns list of current used MPI_Comm handle.
- MPI_Finalize - Terminate the MPI execution environment.
- MPI_Get_library_version - Return the version number of MPI library.
- MPI_Get_processor_name - Gets the name of the processor.
- MPI_Get_version - Return the version number of MPI.
- MPI_Init - Initialize the MPI execution environment.
- MPI_Initialized - Indicates whether MPI_Init has been called.
- MPI_Iprobe - Nonblocking test for a message.
- MPI_Probe - Blocking test for a message.
- MPI_Recv - Blocking receive for a message.
- MPI_Reduce - Reduces values on all processes to a single value.
- MPI_Send - Performs a blocking send.
- MPI examples - Some Nelson MPI examples.
- MPI overview - Access to MPI features from Nelson.
- mpiexec - Run an MPI script.
MPI_Allreduce
Combines values from all processes and distributes the result back to all processes.
Syntax
- r = MPI_Allreduce(Value, Operation, Comm)
Input argument
- Value - value to send: numeric or logical array (sparse not supported).
- Operation - a string: MPI_SUM, MPI_MAX, MPI_MIN, MPI_SUM, MPI_PROD, MPI_LAND, MPI_LOR, MPI_BAND, MPI_BOR, MPI_LXOR or MPI_BXOR
- Comm - a MPI_Comm object.
Output argument
- r - received value
Description
Combines values from all processes and distributes the result back to all processes.
Nelson does not check to ensure that the reduction operation are all the same size across the various processes in the group.
Please be sure that each process passes the same sized array to the MPI_Allreduce operation.
See also
Example
mpiexec([modulepath('mpi'), '/examples/help_examples/MPI_Allreduce.m'], 4)
if ~MPI_Initialized()
MPI_Init();
end
comm = MPI_Comm_object();
my_rank = MPI_Comm_rank ();
num_ranks = MPI_Comm_size();
A = [1 + my_rank:3 + my_rank]
B = MPI_Allreduce(A, 'MPI_PROD', comm);
if (my_rank == 0)
disp('Result:')
disp(B);
end
if MPI_Initialized()
MPI_Finalize();
end
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
MPI_Barrier
Blocks until all processes in the communicator have reached this routine.
Syntax
- r = MPI_Barrier(Comm)
Input argument
- Comm - a MPI_Comm object.
Output argument
- r - integer value: MPI_SUCCESS (0) or MPI_ERR_COMM (5).
Description
This function is used as a synchronization point for all processes in a group. All processes are blocked until every process calls MPI_Barrier.
See also
Example
mpiexec([modulepath('mpi'), '/examples/help_examples/MPI_Barrier.m'], 4)
if ~MPI_Initialized()
MPI_Init();
end
my_rank = MPI_Comm_rank ();
num_ranks = MPI_Comm_size();
comm = MPI_Comm_object('MPI_COMM_WORLD');
sleep(my_rank);
MPI_Barrier(comm);
disp(['I am ', int2str(my_rank), ' of ', int2str(num_ranks)]);
if MPI_Initialized()
MPI_Finalize();
end
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
MPI_Bcast
Broadcasts a message from the process with rank "root" to all other processes of the communicator
Syntax
- A = MPI_Bcast(A, Root)
- A = MPI_Bcast(A, Root, Comm)
Input argument
- A - a nelson variable.
- Root - a integer value: rank of broadcast root.
- Comm - a MPI_Comm object.
Output argument
- A - broadcasted array.
Description
This function is used to broadcast an array to all group members.
See also
Example
mpiexec([modulepath('mpi'), '/examples/help_examples/MPI_Bcast.m'], 4)
if ~MPI_Initialized()
MPI_Init();
end
my_rank = MPI_Comm_rank();
num_ranks = MPI_Comm_size();
root = 0;
if (my_rank == 0)
buff = 777;
else
buff = 0;
end
disp(['rank: ', int2str(my_rank), ': before Bcast, buff is ', int2str(buff)])
buff = MPI_Bcast(buff, root);
disp(['rank: ', int2str(my_rank), ': after Bcast, buff is ', int2str(buff)])
if MPI_Initialized()
MPI_Finalize();
end
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
MPI_Comm_delete
Removes MPI_Comm object.
Syntax
- MPI_Comm_delete(h)
- delete(h)
Input argument
- h - a handle: a MPI_Comm object.
Description
delete(h) deletes MPI_Comm object itself.
Do not forget to clear variable afterward.
Example
CLI required
if ~MPI_Initialized()
MPI_Init();
end
comm = MPI_Comm_object();
MPI_Comm_used
delete(COM_used())
MPI_Comm_used
if MPI_Initialized()
MPI_Finalize();
end
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
MPI_Comm_get_name
Return the print name from the communicator.
Syntax
- MPI_Comm_get_name(comm)
Input argument
- comm - a handle: a MPI_Comm object.
Description
MPI_Comm_get_name(comm) returns the print name from the communicator.
Example
CLI required
if ~MPI_Initialized()
MPI_Init();
end
comm = MPI_Comm_object();
MPI_Comm_get_name(comm)
delete(comm)
if MPI_Initialized()
MPI_Finalize();
end
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
MPI_Comm_object
Creates MPI_Comm object.
Syntax
- comm = MPI_Comm_object()
- comm = MPI_Comm_object(str)
Input argument
- str - a string: MPI_COMM_SELF, or MPI_COMM_WORLD.
Description
MPI_Comm_object(h) creates an MPI_Comm object.
Example
CLI required
if ~MPI_Initialized()
MPI_Init();
end
comm = MPI_Comm_object();
MPI_Comm_used
delete(MPI_Comm_used())
MPI_Comm_used
if MPI_Initialized()
MPI_Finalize();
end
See also
MPI_Comm_used, MPI_Comm_delete.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
MPI_Comm_rank
Determines the rank of the calling process in the communicator.
Syntax
- r = MPI_Comm_rank(Comm)
Input argument
- Comm - a MPI_Comm object.
Output argument
- r - an integer value: rank of the calling process in the group of Comm.
Description
Return the rank of the calling process in the specified communicator.
See also
Example
mpiexec([modulepath('mpi'), '/examples/MPI_helloworld.m'], 4)
if ~MPI_Initialized()
MPI_Init();
end
comm = MPI_Comm_object()
my_rank = MPI_Comm_rank (comm)
num_ranks = MPI_Comm_size(comm)
TAG= 1;
if (my_rank != 0)
rankvect = 0;
MPI_Send(rand(3,3) + my_rank, rankvect, TAG, comm);
else
disp('MPI master receive:')
for source = 1:num_ranks - 1
disp(['From slave ', int2str(source)])
message = MPI_Recv (source, TAG, comm);
disp(message)
end
end
if MPI_Initialized()
MPI_Finalize();
end
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
MPI_Comm_size
Determines the size of the group associated with a communicator.
Syntax
- r = MPI_Comm_size(Comm)
Input argument
- Comm - a MPI_Comm object.
Output argument
- r - an integer value: number of processes in the group of Comm.
Description
Determines the size of the group associated with a communicator.
See also
Example
mpiexec([modulepath('mpi'), '/examples/MPI_helloworld.m'], 4)
if ~MPI_Initialized()
MPI_Init();
end
comm = MPI_Comm_object()
my_rank = MPI_Comm_rank (comm)
num_ranks = MPI_Comm_size(comm)
TAG= 1;
if (my_rank != 0)
rankvect = 0;
MPI_Send(rand(3,3) + my_rank, rankvect, TAG, comm);
else
disp('MPI master receive:')
for source = 1:num_ranks - 1
disp(['From slave ', int2str(source)])
message = MPI_Recv (source, TAG, comm);
disp(message)
end
end
if MPI_Initialized()
MPI_Finalize();
end
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
MPI_Comm_split
Partitions the group that is associated with the specified communicator into a specified number of disjoint subgroups.
Syntax
- newcomm = MPI_Comm_split(comm, color, key)
Input argument
- comm - a MPI_Comm object.
- color - an integer value: The new communicator that the calling process is to be assigned to. The value of color must be non-negative.
- key - an integer value: The relative rank of the calling process in the group of the new communicator.
Output argument
- newcomm - MPI_Comm object: handle to a new communicator.
Description
Partitions the group that is associated with the specified communicator into a specified number of disjoint subgroups.
See also
Example
mpiexec([modulepath('mpi'), '/examples/help_examples/MPI_Comm_split.m'], 10)
if ~MPI_Initialized()
MPI_Init();
end
comm = MPI_Comm_object();
world_rank = MPI_Comm_rank();
world_size = MPI_Comm_size();
color = world_rank * inv(4);
% Split the communicator based on the color and use the
% original rank for ordering
row_comm = MPI_Comm_split(comm, color, world_rank);
row_rank = MPI_Comm_rank();
row_size = MPI_Comm_size();
disp(['WORLD RANK/SIZE: ',int2str(world_rank), '/', int2str(world_size), ' ROW RANK/SIZE: ', int2str(row_rank), '/', int2str(row_size)]);
if MPI_Initialized()
MPI_Finalize();
end
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
MPI_Comm_used
Returns list of current used MPI_Comm handle.
Syntax
- r = MPI_Comm_used()
Output argument
- h - a vector of MPI_Comm handle.
Description
Returns list of current used MPI_Comm handle.
See also
Example
CLI required
if ~MPI_Initialized()
MPI_Init();
end
comm = MPI_Comm_object();
MPI_Comm_used
delete(comm)
MPI_Comm_used
if MPI_Initialized()
MPI_Finalize();
end
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
MPI_Finalize
Terminate the MPI execution environment.
Syntax
- MPI_Finalize()
- r = MPI_Finalize()
Output argument
- r - a logical.
Description
Terminate the MPI execution environment.
MPI process are launched in CLI mode (no gui, no plot).
See also
Example
if ~MPI_Initialized()
MPI_Init();
end
if MPI_Initialized()
MPI_Finalize();
end
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
MPI_Get_library_version
Return the version number of MPI library.
Syntax
- name = MPI_Get_library_version()
Output argument
- name - a string: Version of MPI.
Description
This function returns the version number of MPI library.
See also
Example
if ~MPI_Initialized()
MPI_Init();
end
name = MPI_Get_library_version()
if MPI_Initialized()
MPI_Finalize();
end
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
MPI_Get_processor_name
Gets the name of the processor.
Syntax
- [name, namelen, info] = MPI_Get_processor_name()
Output argument
- name - a string: name of the processor that is using MPI.
- namelen - an integer value: Length (in characters) of the name.
- info - an integer value: 0 MPI_SUCCESS, 16 MPI_ERR_OTHER.
Description
This function get the name of the processor that is using MPI.
See also
Example
if ~MPI_Initialized()
MPI_Init();
end
[name, len, info] = MPI_Get_processor_name()
if MPI_Initialized()
MPI_Finalize();
end
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
MPI_Get_version
Return the version number of MPI.
Syntax
- [major, minor] = MPI_Get_version()
Output argument
- major - an integer value.
- minor - an integer value.
Description
Return the version number of MPI.
See also
Example
if ~MPI_Initialized()
MPI_Init();
end
[major, minor] = MPI_Get_version()
if MPI_Initialized()
MPI_Finalize();
end
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
MPI_Init
Initialize the MPI execution environment.
Syntax
- MPI_Init()
- r = MPI_Init()
Output argument
- r - a logical.
Description
Initialize the MPI execution environment.
MPI process are launched in CLI mode (no gui, no plot).
See also
MPI_Initialized, MPI_Finalize.
Example
if ~MPI_Initialized()
MPI_Init();
end
if MPI_Initialized()
MPI_Finalize();
end
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
MPI_Initialized
Indicates whether MPI_Init has been called.
Syntax
- r = MPI_Initialized()
Output argument
- r - a logical.
Description
Indicates whether MPI_Init has been called.
See also
Example
if ~MPI_Initialized()
MPI_Init();
end
if MPI_Initialized()
MPI_Finalize();
end
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
MPI_Iprobe
Nonblocking test for a message.
Syntax
- [flag, stat, info] = MPI_Iprobe(rank, tag)
- [flag, stat, info] = MPI_Iprobe(rank, tag, comm)
Input argument
- rank - an integer value: source rank.
- tag - an integer value: message tag.
- comm - a MPI_Comm object.
Output argument
- flag - an integer value: 1 if the message is ready to be received, 0 if it is not.
- stat - a struct: source rank, message tag, error, count, cancelled for the accepted message.
- info - an integer value: 0 (MPI_SUCCESS) other value is an error.
Description
Nonblocking test for a message.
See also
Example
mpiexec([modulepath('mpi'), '/examples/help_examples/MPI_Iprobe.m'], 4)
if ~MPI_Initialized()
MPI_Init();
end
comm = MPI_Comm_object();
world_rank = MPI_Comm_rank();
world_size = MPI_Comm_size();
[FLAG, STAT, INFO] = MPI_Iprobe(world_rank,1, comm)
if MPI_Initialized()
MPI_Finalize();
end
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
MPI_Probe
Blocking test for a message.
Syntax
- [flag, stat, info] = MPI_Probe(rank, tag)
- [flag, stat, info] = MPI_Probe(rank, tag, comm)
Input argument
- rank - an integer value: source rank.
- tag - an integer value: message tag.
- comm - a MPI_Comm object.
Output argument
- flag - an integer value: 1 if the message is ready to be received, 0 if it is not.
- stat - a struct: source rank, message tag, error, count, cancelled for the accepted message.
- info - an integer value: 0 (MPI_SUCCESS) other value is an error.
Description
Blocking test for a message.
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
MPI_Recv
Blocking receive for a message.
Syntax
- r = MPI_Recv(Source, Tag)
- [r, mpi_source, mpi_tag] = MPI_Reduce(Source, Tag, Comm)
Input argument
- Source - an integer value: rank of source.
- Tag - an integer value: message tag.
- Comm - a MPI_Comm object.
Output argument
- r - received value
Description
This function receives an array from a source node on a given communicator with the specified tag.
Throws an exception if there is an error.
Receive arrays of arbitrary complexity, including cell arrays, structures, strings, sparse, etc ...
See also
Example
mpiexec([modulepath('mpi'), '/examples/MPI_helloworld.m'], 4)
if ~MPI_Initialized()
MPI_Init();
end
comm = MPI_Comm_object()
my_rank = MPI_Comm_rank (comm)
num_ranks = MPI_Comm_size(comm)
TAG= 1;
if (my_rank != 0)
rankvect = 0;
MPI_Send(rand(3,3) + my_rank, rankvect, TAG, comm);
else
disp('MPI master receive:')
for source = 1:num_ranks - 1
disp(['From slave ', int2str(source)])
message = MPI_Recv (source, TAG, comm);
disp(message)
end
end
if MPI_Initialized()
MPI_Finalize();
end
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
MPI_Reduce
Reduces values on all processes to a single value.
Syntax
- r = MPI_Reduce(Value, Operation, Root)
- r = MPI_Reduce(Value, Operation, Root, Comm)
Input argument
- Value - value to send: numeric or logical array (sparse not supported).
- Operation - a string: MPI_SUM, MPI_MAX, MPI_MIN, MPI_SUM, MPI_PROD, MPI_LAND, MPI_LOR, MPI_BAND, MPI_BOR, MPI_LXOR or MPI_BXOR
- Root - a integer value: rank of root process.
- Comm - a MPI_Comm object.
Output argument
- r - received value
Description
Reduces values on all processes to a single value.
Nelson does not check to ensure that the reduction operation are all the same size across the various processes in the group.
Please be sure that each process passes the same sized array to the MPI_Allreduce operation.
See also
Example
mpiexec([modulepath('mpi'), '/examples/help_examples/MPI_Reduce.m'], 4)
if ~MPI_Initialized()
MPI_Init();
end
my_rank = MPI_Comm_rank ();
num_ranks = MPI_Comm_size();
A = [1 + my_rank:3 + my_rank]
B = MPI_Reduce(A, 'MPI_SUM', 0);
if (my_rank == 0)
disp('Result:')
B
end
if MPI_Initialized()
MPI_Finalize();
end
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
MPI_Send
Performs a blocking send.
Syntax
- MPI_Send(A, destination, tag)
- MPI_Send(A, destination, tag, comm)
Input argument
- A - an nelson array to send.
- destination - an integer value: rank of source.
- tag - an integer value: message tag.
- comm - a MPI_Comm object.
Description
This function sends an array to a destination node on a given communicator with a specific message tag.
Note that there has to be a matching receive issued by the destination node.
Throws an exception if there is an error.
See also
Example
mpiexec([modulepath('mpi'), '/examples/MPI_helloworld.m'], 4)
if ~MPI_Initialized()
MPI_Init();
end
comm = MPI_Comm_object()
my_rank = MPI_Comm_rank (comm)
num_ranks = MPI_Comm_size(comm)
TAG= 1;
if (my_rank != 0)
rankvect = 0;
MPI_Send(rand(3,3) + my_rank, rankvect, TAG, comm);
else
disp('MPI master receive:')
for source = 1:num_ranks - 1
disp(['From slave ', int2str(source)])
message = MPI_Recv (source, TAG, comm);
disp(message)
end
end
if MPI_Initialized()
MPI_Finalize();
end
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
MPI examples
MPI examples
Some Nelson MPI examples.
Description
See below for some basic examples about MPI interfaces available in Nelson.
Examples
mpiexec([modulepath('mpi'), '/examples/MPI_helloworld.m'], 4)
edit([modulepath('mpi'), '/examples/MPI_helloworld.m'])
mpiexec([modulepath('mpi'), '/examples/MPI_simpledemo.m'], 4)
edit([modulepath('mpi'), '/examples/MPI_simpledemo.m'])
mpiexec([modulepath('mpi'), '/examples/MPI_parallel_sum.m'], 40)
edit([modulepath('mpi'), '/examples/MPI_parallel_sum.m'])
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
MPI overview
MPI overview
Access to MPI features from Nelson.
Description
As many others MPI applications, MPI/Nelson as be started through the mpiexec/mpirun command.
MPI features are only avaible in CLI mode. But you can call in others mode with mpiexec builtin.
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
mpiexec
Run an MPI script.
Syntax
- mpiexec(script)
- mpiexec(script, nb_process)
- r = mpiexec(script, nb_process)
- [r, msg] = mpiexec(script, nb_process)
Input argument
- script - an filename with .m extension.
- nb_process - an integer value: number of process.
Output argument
- r - an integer value: maximum of the exit status values of all of the processes created by mpiexec.
Description
Run an MPI script in nelson.
MPI process are launched in CLI mode (no gui, no plot).
See also
Example
mpiexec([modulepath('mpi'), '/examples/help_examples/MPI_Allreduce.m'], 4)
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
Parallel
Parallel
Description
parallel functions
- afterAll - Run function after all functions finish running in the background.
- afterEach - Run function after each function finish running in the background.
- backgroundPool - Environment for running nelson's code in the background.
- cancel - Stop function running in the background.
- cancelAll - Stop all functions running in the background.
- fetchNext - Retrieve next unread outputs from FevalFuture array.
- fetchOutputs - Retrieve results from function running in the background pool.
- parfeval - Run function in background.
- wait - Wait for futures to be completed.
afterAll
Run function after all functions finish running in the background.
Syntax
- B = afterAll(F, fcn, n)
Input argument
- F - Input Future object (scalar or array).
- fcn - Function handle: Function to run after all input futures.
- n - Number of output arguments.
Output argument
- B - AfterAllFuture object.
Description
B = afterAll(F, fcn, n) returns a AfterAllFuture object B.
Function fcn is automatically runned after all elements in the Future array F were finished.
If any of the elements in F encounters an error, the Error property of B contains an error.
Example
pool = backgroundPool()
fptrRand = str2func('rand')
fptrMax = str2func('@(r) max(r)')
fptrMin = str2func('@(r) min(r)')
for idx= 1:10
f(idx) = parfeval(pool, fptrRand, 1, 1000, 1);
end
maxFuture = afterEach(f, fptrMax, 1);
minFuture = afterAll(maxFuture, fptrMin, 1);
fetchOutputs(minFuture)
fetchOutputs(maxFuture)
See also
backgroundPool, fetchOutputs, afterEach.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
afterEach
Run function after each function finish running in the background.
Syntax
- B = afterEach(F, fcn, n)
Input argument
- F - Input Future object (scalar or array).
- fcn - Function handle: Function to run after all input futures.
- n - Number of output arguments.
Output argument
- B - AfterEachFuture object.
Description
B = afterEach(F, fcn, n) returns a AfterEachFuture object B.
Function fcn is automatically runned after each element in the Future array F was finished.
If any of the elements in F encounters an error, the Error property of B contains an error.
Example
pool = backgroundPool()
fptrRand = str2func('rand')
fptrMax = str2func('@(r) max(r)')
fptrMin = str2func('@(r) min(r)')
for idx= 1:10
f(idx) = parfeval(pool, fptrRand, 1, 1000, 1);
end
maxFuture = afterEach(f, fptrMax, 1);
minFuture = afterAll(maxFuture, fptrMin, 1);
fetchOutputs(minFuture)
fetchOutputs(maxFuture)
See also
backgroundPool, fetchOutputs, afterAll.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
backgroundPool
Environment for running nelson's code in the background.
Syntax
- pool = backgroundPool()
Output argument
- pool - backgroundPool object.
Description
pool = backgroundPool() returns the background pool.
This allows to run other code in your Nelson's session at the same time.
Properties of backgroundPool object:
'FevalQueue': Queue of FevalFuture objects to run on the background pool (read only).
'NumWorkers': Number of workers (read only).
'Busy': Flag that indicates whether the background pool is busy, logical (read only).
Example
b = backgroundPool()
fptr = str2func('magic');
f = parfeval(b, fptr, 1, 9);
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
cancel
Stop function running in the background.
Syntax
- cancel(f)
Input argument
- f - FevalFuture object: scalar or array.
Description
cancel(f) will stop each running or queued element of the Future array f.
Future cancelled Findicates an error as property.
Some functions cannot be interrupted by pressing Ctrl+C or cancel, such as save function.
Example
fptr = str2func('pause');
for i = 1:100
f(i) = parfeval(backgroundPool, fptr, 0, 5);
end
f(70)
cancel(f(70))
f(70)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
cancelAll
Stop all functions running in the background.
Syntax
- cancel(fevalQueue)
Input argument
- fevalQueue - FevalQueue object: scalar.
Description
cancelAll(fevalQueue) stops all running or queued elements of the background pool.
Example
fptr = str2func('pause');
pool = backgroundPool;
pool.FevalQueue
f = parfeval(pool, fptr, 0, Inf);
f
pool.FevalQueue
cancelAll(pool.FevalQueue)
pool.FevalQueue
f
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
fetchNext
Retrieve next unread outputs from FevalFuture array.
Syntax
- [idx, y1, ... , ym] = fetchNext(f)
- [idx, y1, ... , ym] = fetchNext(f, timeout)
Input argument
- f - FevalFuture object
- timeout - timeout seconds: waits for a maximum of timeout seconds for a result in f to become available.
Output argument
- idx - Index of the FevalFuture array, returned as an integer scalar.
- y1, ... , ym - outputs
Description
[idx, y1, ... , ym] = fetchNext(f) retrieves index idx of the new readable FevalFuture object in the array f that is finished, and m results from that FevalFuture as Y1, ... , Ym.
Example
tic()
N = 100;
for idx = N:-1:1
F(idx) = parfeval(backgroundPool,str2func('rank'),1,magic(idx));
end
results = zeros(1,N);
for idx = 1:N
[finishedIdx, result] = fetchNext(F);
results(finishedIdx) = result;
disp(sprintf('Result: %d', result));
end
toc()
See also
parfeval, fetchOutputs, backgroundPool.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
fetchOutputs
Retrieve results from function running in the background pool.
Syntax
- [y1, ... , ym] = fetchOutputs(f)
Input argument
- f - FevalFuture object
Output argument
- y1, ... , ym - outputs
Description
[y1, ... , ym] = fetchOutputs(f) retrieves m results from a Future array f.
fetchOutputs waits for the function associated to f to finish before retrieving results.
If fetchOutputs is called, Read property of each element in f is set to true.
Examples
Sequential version
tic()
R1 = magic(5000);
R2 = magic(5000);
toc()
size(R1)
Parallel version
b = backgroundPool()
tic()
fptr = str2func('magic');
f1 = parfeval(b, fptr, 1, 5000);
f2 = parfeval(b, fptr, 1, 5000);
b
r1 = fetchOutputs(f1);
r2 = fetchOutputs(f2);
toc()
size(r1)
f1
f2
See also
parfeval, backgroundPool, fetchNext.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
parfeval
Run function in background.
Syntax
- f = parfeval(bPool, fptr, n, x1, ..., xm)
Input argument
- bPool - backgroundPool object returned by backgroundPool().
- fptr - Function handle: Function to run.
- n - Number of output arguments.
- x1, ..., xm - Input arguments, specified as a comma-separated list of variables or expressions.
Output argument
- f - FevalFuture object.
Description
f = parfeval(bPool, fptr, n, x1, ..., xm) starts the function fptr to run in the background.
backgroundPool has NumWorkers available. If there are more functions scheduled, functions wait than one entry is available in pool.
parfeval runs the function fptr on a background worker.
Example
b = backgroundPool()
fptr = str2func('cos');
f = parfeval(b, fptr, 1, 5);
r = fetchOutputs(f)
See also
backgroundPool, fetchOutputs, feval.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
wait
Wait for futures to be completed.
Syntax
- wait(f)
- wait(f, state)
- TF = wait(f, state, timeout)
Input argument
- f - FevalFuture object: scalar or array.
- state - state to wait: 'finished' (default) or 'running'
- timeout - seconds to wait: real numeric scalar.
Output argument
- TF - logical: If each element of the Future array f finishes before timeout seconds elapse, TF is true. Otherwise, TF is false.
Description
wait(f) pauses execution until each element of the Future array f is finished.
wait(f, state) pauses execution until each element of the Future array f has its 'State' property set to state.
tf = wait(f, state, timeout) pauses execution for a maximum of timeout seconds.
Example
fptr = str2func('pause');
for i = 1:15
f(i) = parfeval(backgroundPool, fptr, 0, 5);
end
tic()
R = wait(f, 'finished');
toc()
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
Help tools functions
Help tools functions
Description
help tools
- buildhelp - Build help of Nelson's modules.
- buildhelpmd - Build help of Nelson's modules for GitBook.
- buildhelpweb - Build help of Nelson's modules for website.
- docroot - Retrieve or update the root directory for Nelson Help system.
- headcomments - Display Nelson function header comments.
- htmltopdf - Convers html page to pdf.
- markdown - Converts markdown to html.
- xmldocbuild - Internal function to convert xml document files to html.
- xmldocchecker - Checks a xml documentation file.
- xmldoctohelp - Converts xml Nelson help files to Nelson format.
- xmldoctohtml - Converts xml Nelson help files to html.
- xmldoctomd - Converts xml Nelson help files to markdown format.
buildhelp
Build help of Nelson's modules.
Syntax
- buildhelp()
- buildhelp(module_name)
Input argument
- module_name - a string: module name (module must be loaded).
Description
buildhelp generates help files.
Example
buildhelp();
buildhelp('core');
See also
doc.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
buildhelpmd
Build help of Nelson's modules for GitBook.
Syntax
- buildhelpmd(dirdest)
- buildhelpmd(dirdest, module_name)
Input argument
- dirdest - a string: a path destination.
- module_name - a string: module name (module must be loaded).
Description
buildhelpmd generates help files for GitBook (markdown).
Example
buildhelpmd(tempdir());
buildhelpmd(tempdir(), 'core');
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
buildhelpweb
Build help of Nelson's modules for website.
Syntax
- buildhelpweb(destination_dir)
- buildhelpweb(destination_dir, language)
Input argument
- destination_dir - a string: destination directory.
- language - a string: language. if it is missing, current default language used.
Description
buildhelpweb generates help files for website.
internal function
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
docroot
Retrieve or update the root directory for Nelson Help system.
Syntax
- r = docroot()
- r = docroot(new_docroot)
Input argument
- new_docroot - a string: '', '.', or a URL.
Description
docroot retrieves or updates the root directory for Nelson Help.
When called without an argument, docroot returns the current root directory for Nelson Help. By default, it returns the URL of the help website used by Nelson.
When called with an argument, docroot updates the root directory for Nelson Help.
docroot('') resets the root directory for Nelson Help to the default value.
docroot('.') uses local help files and the local browser (restores behavior before v1.11.0).
Example
docroot()
doc rand
docroot('.')
doc rand
docroot('')
See also
doc.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
headcomments
Display Nelson function header comments.
Syntax
- headcomments(function_name)
- ce = headcomments(function_name)
Input argument
- function_name - a string: function name or a .m filename.
Output argument
- ce - a cell of strings
Description
head_comments displays the function header comments.
Comments are read from the associated .m file.
Nelson predefined functions have no header comments.
Example
comments = headcomments('cellstr'); md = markdown(comments);inserthtml(md)
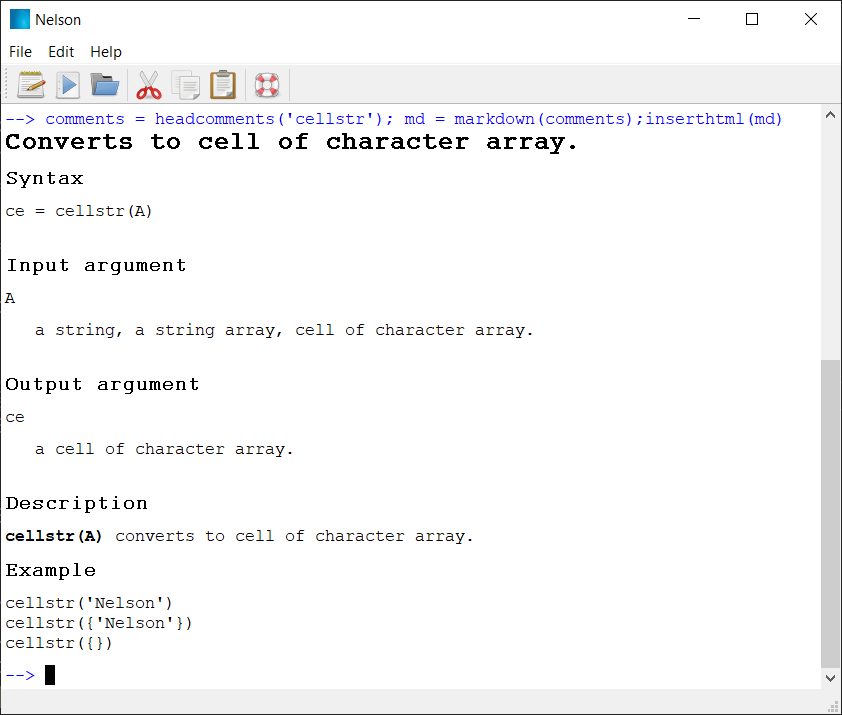
See also
doc, markdown, inserthtml, which.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
htmltopdf
Convers html page to pdf.
Syntax
- htmltopdf(html_filename, pdf_filename)
Input argument
- html_filename - a string: html filename.
- pdf_filename - a string: pdf filename (destination).
Description
htmltopdf converts html page to pdf.
Example
txt = {'## Example of Markdown text';
'>Nelson html to pdf conversion example'};
html = markdown(txt);
f = fopen([tempdir(), 'htmltopdf_example.html'], 'wt');
fwrite(f, html);
fclose(f);
htmltopdf([tempdir(), 'htmltopdf_example.html'], [tempdir(), 'htmltopdf_example.pdf'])
if ispc()
winopen([tempdir(), 'htmltopdf_example.pdf']);
end
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
markdown
Converts markdown to html.
Syntax
- html_txt = markdown(md_txt)
- status = markdown(md_filename, html_filename)
Input argument
- md_txt - a string: markdown text to convert.
- md_filename - a string: markdown filename to convert (source).
- html_filename - a string: html filename (destination).
Output argument
- status - a logical: html file generated or not.
Description
markdown converts Markdown text-to-HTML.
Example
txt = {'## Example of Markdown text';
'>Nelson supports markdown ...'};
html = markdown(txt);
filewrite([tempdir(), 'markdown_example.html'], html)
if ispc()
winopen([tempdir(), 'markdown_example.html']);
end
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
xmldocbuild
Internal function to convert xml document files to html.
Syntax
- status = xmldocbuild(source_dirs, destination_dir, main_title, export_format, overwrite)
Input argument
- source_dirs - a cell of string: list of xml filenames.
- destination_dir - a string: directory destination.
- main_title - a string: title of main index.
- export_format - a string: 'qt' or 'html'.
- overwrite - a logical: force overwrite if file destination already exists
Output argument
- status - a logical: files generated or not.
Description
xmldocbuild convert xml document files to html.
internal function
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
xmldocchecker
Checks a xml documentation file.
Syntax
- xmldocchecker(xmldocfilename)
- [errors_detected, warnings_detected] = xmldocchecker(xmldocfilename)
Input argument
- xmldocfilename - a string: xml document.
Output argument
- errors_detected - a cell of strings: errors detected.
- warnings_detected - a cell of strings: warnings detected.
Description
xmldocchecker is a tool to check that a xml document is valid.
Example
xmldocchecker([nelsonroot(),'/module_skeleton/help/en_US/xml/nelson_sum.xml'])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
xmldoctohelp
Converts xml Nelson help files to Nelson format.
Syntax
- status = xmldoctohelp(source_dirs, destination_dir, main_title, overwrite)
Input argument
- source_dirs - a cell of string: list of xml filenames.
- destination_dir - a string: directory destination.
- main_title - a string: title of main index.
- overwrite - a logical: force overwrite if file destination already exists
Output argument
- status - a logical: files generated or not.
Description
xmldoctohelp converts xml Nelson help files to Nelson format.
See also
xmldocbuild, buildhelp, buildhelpweb.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
xmldoctohtml
Converts xml Nelson help files to html.
Syntax
- status = xmldoctohtml(source_dirs, destination_dir, main_title, overwrite)
Input argument
- source_dirs - a cell of string: list of xml filenames.
- destination_dir - a string: directory destination.
- main_title - a string: title of main index.
- overwrite - a logical: force overwrite if file destination already exists
Output argument
- status - a logical: files generated or not.
Description
xmldoctohelp converts xml Nelson help files to html.
See also
xmldocbuild, buildhelp, buildhelpweb.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
xmldoctomd
Converts xml Nelson help files to markdown format.
Syntax
- status = xmldoctomd(source_dirs, destination_dir, main_title, overwrite)
Input argument
- source_dirs - a cell of string: list of xml filenames.
- destination_dir - a string: directory destination.
- main_title - a string: title of main index.
- overwrite - a logical: force overwrite if file destination already exists
Output argument
- status - a logical: files generated or not.
Description
xmldoctomd converts xml Nelson help files to markdown format.
See also
xmldocbuild, buildhelpmd, buildhelpweb.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
History manager
History manager
Description
history functions
- history - history manager.
history
history manager.
Syntax
- history()
- c = history()
- s = history('size')
- f = history('filename')
- l = history('enable_save')
- c = history('get')
- history('display')
- history('save')
- history('load')
- history('clear')
- history('duplicated')
- history('saveafter')
- history('removeexit')
- history('size', new_size)
- history('enable_save', true_false)
- history('delete', lines)
- history('append', str)
- history('filename', name)
- history('load', filename_history)
- history('save', filename_history)
- history('duplicated', true_false)
- history('removeexit', true_false)
- history('get', lines)
- history('saveafter', nb_commands)
Input argument
- new_size - a integer value: new size max of history.
- true_false - a logical.
- lines - a integer value or a vector of size 1x2.
- str - a string.
- name - a string: new default history filename
- filename_history - a string: filename
- nb_commands - a integer value: number of commands.
Output argument
- c - a cell of strings.
- l - a logical.
- s - a integer value.
- f - a string.
Description
history() displays the current Nelson history.
c = history() returns the current Nelson history in a cell of strings.
s = history('size') returns history size max.
f = history('filename') returns the history filename.
l = history('enable_save') returns the history manager state.
c = history('get') returns the current Nelson history in a cell of strings.
history('display') displays the current Nelson history.
history('save') saves current history file.
history('load') load current history file.
history('clear') clears history.
history('duplicated') get state about save of consecutive duplicated commands.
history('saveafter') get state about save the history after nth commands.
history('removeexit') get state about do not save exit in history file.
history('size', new_size) set history size max with new_size.
history('enable_save', true_false) set the history manager state: false for 'off', true for 'on'.
history('delete', lines) deletes lines by index: a scalar value or a vector 1x2.
history('append', str) append command to history.
history('filename', name) set the history filename.
history('load', filename_history) load history file.
history('save', filename_history) save history file
history('duplicated', true_false) set state about consecutive duplicated commands. true remove duplicated.
history('removeexit', true_false) set state about do not save exit in history file.
history('get', lines)returns the current Nelson history in a cell of strings by index: a scalar value or a vector 1x2.
history('saveafter', nb_commands) saves the history file after nb_commands statements are added to the file.
Tips: You can easily share your history file in the cloud by adding few lines code in your user startup file.
If nelson launched with '--nouserstartup' option, history file will be not loaded at startup and not saved at exit.
Examples
Example to share your history file in OneDrive cloud
OneDrivePath = getenv('OneDrive');
if (strcmp(OneDrivePath, '') == false)
NelsonOneDrivePath = [OneDrivePath, '/Nelson'];
mkdir(NelsonOneDrivePath);
NelsonOneDrivePathFilename = [NelsonOneDrivePath, '/', 'Nelson.history'];
history('filename', NelsonOneDrivePathFilename);
history('load', NelsonOneDrivePathFilename);
end
history()
c = history()
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
Console
Console
Description
Console
- clc - Clear Command Window.
- input - Display prompt and wait for user input.
- terminal_size - Query the size of the terminal window.
clc
Clear Command Window.
Syntax
- clc()
Description
clc() clears the console and move the cursor to the upper left corner.
Example
disp('Hello');
clc()
See also
disp.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
input
Display prompt and wait for user input.
Syntax
- r = input(prompt_str)
- r = input(prompt_str, 's')
Input argument
- prompt_str - a string: temp. prompt displayed
Output argument
- r - a string
Description
Display prompt and wait for user input. input returns a string which is the expression entered at keyboard.
Example
res = input('Please input a value ', 's');
r = execstr(['A = ', res, ';'], 'errcatch');
if (r)
disp('It was a value.');
disp(A)
else
disp('It was NOT a value.');
end
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
terminal_size
Query the size of the terminal window.
Syntax
- [r, c] = terminal_size()
Output argument
- [r, c] - a vector: rows and columns
Description
terminal_size() returns a vector with size of the terminal window in characters (rows and columns).
Example
terminal_size()
See also
disp.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
Gui module
Gui module
Description
functions about gui
- commandhistory - Command History
- errordlg - Creates a error dialog box.
- filebrowser - Current Folder Browser
- helpdlg - Creates a help dialog box.
- inserthtml - Insert html in GUI console.
- lookandfeel - default current application look and feel.
- msgbox - Creates a message dialog box.
- qt_verbose - show/hide Qt debug message.
- questdlg - Creates a question dialog box.
- uigetdir - Opens dialog box to select a directory.
- helpdlg - Creates a warning dialog box.
- workspace - Workspace Browser
commandhistory
Command History
Syntax
- commandhistory
Description
The Command History window presents a record of statements executed in both the current and past Nelson sessions.
Each session is timestamped with the short date format of your operating system, followed by the corresponding statements.
Entries within the Command History window can be selected for various actions and operations.
See also
History
Version | Description |
---|---|
1.1.0 | initial version |
Author
Allan CORNET
errordlg
Creates a error dialog box.
Syntax
- h = errordlg()
- h = errordlg(text_error)
- h = errordlg(text_error, title)
- h = errordlg(text_error, title, mode)
Input argument
- text_error - a string or a cell of string: the error message.
- title - a string: the title of the dialog box.
- mode - a string: 'mode', 'non-modal', 'replace'.
Output argument
- h - a QObject handle.
Description
errordlg creates an error dialog box.
h = errordlg(text_error, title, 'replace') specifies whether to replace an existing dialog box having the same title.
Examples
h = errordlg()
h = errordlg('error string')
h = errordlg('error string', 'dialog title')
h = errordlg('error string', 'dialog title')
h = errordlg('error string', 'dialog title', 'on')
See also
warndlg, questdlg, helpdlg, msgbox.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
filebrowser
Current Folder Browser
Syntax
- filebrowser
Description
The Current Folder browser in Nelson facilitates interactive file and folder management. Utilize it to navigate, create, access, relocate, and rename files and folders within the current directory.
See also
History
Version | Description |
---|---|
1.1.0 | initial version |
Author
Allan CORNET
helpdlg
Creates a help dialog box.
Syntax
- h = helpdlg()
- h = helpdlg(text_help)
- h = helpdlg(text_help, title)
- h = helpdlg(text_help, title, 'on')
Input argument
- text_help - a string or a cell of string: the help message.
- title - a string: the title of the dialog box.
Output argument
- h - a QObject handle.
Description
errordlg creates an help dialog box.
h = helpdlg(text_help, title, 'on') specifies whether to replace an existing dialog box having the same name.
Examples
h = helpdlg()
h = helpdlg('help string')
h = helpdlg('help string', 'dialog title')
h = helpdlg('help string', 'dialog title')
h = helpdlg('help string', 'dialog title', 'on')
See also
warndlg, errordlg, questdlg, msgbox.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
inserthtml
Insert html in GUI console.
Syntax
- inserthtml(html_txt)
Input argument
- html_txt - a string: html text
Description
inserthtml inserts html code in GUI console.
Example
inserthtml(markdown(fileread([nelsonroot(),'/CHANGELOG.md'])))
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
lookandfeel
default current application look and feel.
Syntax
- r = lookandfeel()
- ce = lookandfeel('available')
- p = lookandfeel(lf)
- ss = lookandfeel('stylesheet')
- pp = lookandfeel('stylesheet', ss)
Input argument
- lf - a string: look and feel to apply.
- ss - a string: style sheet to apply.
Output argument
- r - a string: current look and feel.
- ce - a cell of strings: list of look and feel available.
- ss - a string: current style sheet applied.
- p - a string: previous look and feel applied.
- pp - a string: previous style sheet applied.
Description
lookandfeel manages look and feel Nelson application.
Examples
currentlf = lookandfeel();
lfs = lookandfeel('available')
for lf = lfs'
lookandfeel(lf{1})
sleep(10);
end
lookandfeel(currentlf)
currentstylesheet = lookandfeel('stylesheet')
stylefilename = [modulepath('gui'), '/resources/darkstyle.qss'];
edit(stylefilename)
newstyle = fileread(stylefilename);
previousstylesheet = lookandfeel('stylesheet', newstyle)
sleep(10);
lookandfeel('stylesheet', previousstylesheet)
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
msgbox
Creates a message dialog box.
Syntax
- h = msgbox(message)
- h = msgbox(message, mode)
- h = msgbox(message, title)
- h = msgbox(message, title, mode)
- h = msgbox(message, title, icon)
- h = msgbox(message, title, icon, mode)
Input argument
- message - a string or a cell of string: the message to display.
- title - a string: the title of the dialog box.
- icon - a string: 'none', 'error', 'help', 'warn' or 'question'.
- mode - a string: 'modal', 'on' or 'nonmodal'.
Output argument
- h - a QObject handle.
Description
msgbox creates an message dialog box.
h = msgbox(message, title, 'on') specifies whether to replace an existing dialog box having the same name.
Examples
h = msgbox('help string')
h = msgbox('help string', 'dialog title')
h = msgbox('help string', 'dialog title')
h = msgbox('help string', 'dialog title', 'on')
See also
helpdlg, errordlg, questdlg, warndlg.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
qt_verbose
show/hide Qt debug message.
Syntax
- r = qt_verbose()
- p = qt_verbose(logical)
Input argument
- logical - a logical: true to show messages, false to hide.
Output argument
- r - logical: current value
- p - logical: previous value
Description
qt_verbose how/hide Qt debug message.
This function is usefull to debug Qt and Qml.
Example
h = qt_verbose()
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
questdlg
Creates a question dialog box.
Syntax
- buttonname = questdlg(question)
- buttonname = questdlg(question, title)
- buttonname = questdlg(question, title, default)
- buttonname = questdlg(question, title, text1, default)
- buttonname = questdlg(question, title, text1, text2, default)
- buttonname = questdlg(question, title, text1, text2, text3, default)
Input argument
- question - a string or a cell of string: the question.
- title - a string: the title of the dialog box.
- text1 - a string: text of button 1.
- text2 - a string: text of button 2.
- text3 - a string: text of button 3.
- default - a string: text of selected button by default.
Output argument
- buttonname - a string: text of the clicked button or ''.
Description
questdlg displays a string using a question dialog box and return the caption of the activated button.
The dialog has three default buttons: 'Yes', 'No', 'Cancel' with 'Yes' as default.
Examples
res = questdlg('What is the answer to the ultimate question of life, the universe and everything ?', 'A question for geeks', '41', '42', '43', '42')
res = questdlg ('Easy ?', 'Jeff', 'No', 'Okay', 'Okay')
res = questdlg('How are you ?', 'Health', 'Fine', 'Good', 'sick', 'Fine')
res = questdlg({'Is', 'this', 'a', 'multi line', 'test ?'}, 'Test :)')
See also
warndlg, errordlg, helpdlg, msgbox.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
uigetdir
Opens dialog box to select a directory.
Syntax
- dir_ans = uigetdir()
- dir_ans = uigetdir(path)
- dir_ans = uigetdir(path, title)
Input argument
- path - a string: initial path
- title - a string: title of the dialog box
Output argument
- dir_ans - a string (returned path) or 0 if dialogbox is canceled
Description
uigetdir opens a dialog box for selecting a directory.
If path is wrong or not given, the current working directory will be used.
Example
A = uigetdir();
See also
pwd.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
helpdlg
Creates a warning dialog box.
Syntax
- h = warndlg()
- h = warndlg(text_warning)
- h = warndlg(text_warning, title)
- h = warndlg(text_warning, title, 'on')
Input argument
- text_warning - a string or a cell of string: the warning message.
- title - a string: the title of the dialog box.
Output argument
- h - a QObject handle.
Description
errordlg creates an warning dialog box.
h = warndlg(text_warning, title, 'on') specifies whether to replace an existing dialog box having the same name.
Examples
h = warndlg()
h = warndlg('help string')
h = warndlg('help string', 'dialog title')
h = warndlg('help string', 'dialog title')
h = warndlg('help string', 'dialog title', 'on')
See also
helpdlg, errordlg, questdlg, msgbox.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
workspace
Workspace Browser
Syntax
- workspace
Description
The Workspace browser allows you to observe and actively oversee the contents of the workspace within Nelson, providing access and control over each variable or object present.
See also
History
Version | Description |
---|---|
1.1.0 | initial version |
Author
Allan CORNET
QML engine
QML engine
Description
The QML engine enables nelson programs to display and manipulate graphical content using Qt's QML framework.
- QObject_classname - Returns class name of an QObject handle.
- QObject_findchildren - Returns all children of this object with the given name.
- QObject_get - Retrieve a property value from an QObject handle.
- QObject_iswidgettype - Returns true if the QObject is a widget.
- QObject_iswindowtype - Returns true if the QObject is a window.
- QObject_methodsignature - Returns the signature of a method of a QObject handle.
- QObject_root - QObject root object.
- QObject_set - Set a property value of an QObject handle (set).
- QObject_undefine - Undefine a dynamic property of a QObject handle.
- QObject_used - Returns list of current used QObject handle.
- nelsonObject - nelson object callable from QML.
- qml_addimportpath - Adds path as directory where the qml engine searches for installed modules.
- qml_addpluginpath - Adds path as directory where the qml engine searches for native plugins.
- qml_clearcomponentcache - Clears the engine's internal component cache..
- qml_collectgarbage - Runs the Qml garbage collector.
- qml_createqquickview - Load a QML file and creates a window.
- qml_demos - QML demos.
- qml_evaluatefile - Evaluates a js file.
- qml_evaluatestring - Evaluates a js string.
- qml_importpathlist - Returns the list of directories where the engine searches for installed modules in a URL-based directory structure.
- qml_loadfile - Load a QML file.
- qml_loadstring - Load a QML string.
- qml_offlinestoragepath - Get the Property contains the directory to store offline user data.
- qml_pluginpathlist - Returns the list of directories where the engine searches for native plugins for imported modules.
- qml_setofflinestoragepath - Set the Property contains the directory to store offline user data.
- qt_constant - Returns Qt constant value.
- qt_version - Returns Qt version used.
QObject_classname
Returns class name of an QObject handle.
Syntax
- s = QObject_classname(h)
Input argument
- h - an QObject handle.
Output argument
- s - a string: class name.
Description
Returns class name of an QObject handle.
See also
QObject_set (set), QObject_get (get).
Example
h1 = QObject_root()
h1.className
QObject_classname(h1)
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
QObject_findchildren
Returns all children of this object with the given name.
Syntax
- hr = QObject_findchildren(h, objectName, recursive)
Input argument
- h - an QObject handle.
- objectName - a string.
- recursive - a logical: true (The search is performed recursively).
Output argument
- hr - a vector of QObject handle.
Description
Returns all children of this object with the given name.
See also
QObject_set (set), QObject_get (get).
Example
h1 = errordlg()
h2 = errordlg()
hr = QObject_findchildren(QObject_root(), 'errordlg', true)
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
QObject_get
Retrieve a property value from an QObject handle.
Syntax
- R = get(h, property_name)
Input argument
- h - an QObject handle.
- property_name - a string: property name.
Output argument
- R - The data type of the return value depends on the invoked method.
Description
R = get(h, property_name) returns the value of property asked.
See also
Example
h = errordlg();
h.visible % or get(h, 'visible')
h.windowTitle % or get(h, 'windowTitle')
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
QObject_iswidgettype
Returns true if the QObject is a widget.
Syntax
- R = QObject_iswidgettype(h)
Input argument
- h - an QObject handle.
Output argument
- R - a logical.
Description
Returns true if the QObject is a widget; otherwise returns false.
See also
Example
h = errordlg()
r = QObject_iswidgettype(h)
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
QObject_iswindowtype
Returns true if the QObject is a window.
Syntax
- R = QObject_iswindowtype(h)
Input argument
- h - an QObject handle.
Output argument
- R - a logical.
Description
Returns true if the QObject is a window; otherwise returns false.
See also
Example
h = errordlg()
r = QObject_iswindowtype(h)
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
QObject_methodsignature
Returns the signature of a method of a QObject handle.
Syntax
- res = QObject_methodsignature(h, method_name)
Input argument
- h - an QObject handle.
- method_name - a string : method name.
Output argument
- R - a string: method signature.
Description
Returns the signature of a method of a QObject handle.
See also
QObject_invoke (invoke), QObject_methods (methods).
Example
h = errordlg()
QObject_methodsignature(h, 'setVisible')
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
QObject_root
QObject root object.
Syntax
- r = QObject_root()
Output argument
- h - QObject handle of Nelson gui.
Description
Returns QObject handle of Nelson gui.
See also
QObject_set (set), QObject_get (get).
Example
h1 = QObject_root()
h1.windowTitle
h1.windowTitle = 'Your title'
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
QObject_set
Set a property value of an QObject handle (set).
Syntax
- R = set(h, property_name, value)
Input argument
- h - an QObject handle.
- property_name - a string: property name.
- value - a variable.
Output argument
- R - user-settable properties and possible values for the object identified by h.
Description
This routine can be used to modify the value of a specified property from an QObject object.
Example
h = errordlg()
h.visible = false; % or set(h, 'visible', false)
h.windowTitle = 'new title' % or set(h, 'windowTitle', 'new title')
h.visible = true;
See also
set.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
QObject_undefine
Undefine a dynamic property of a QObject handle.
Syntax
- QObject_undefine(h, property_name)
Input argument
- h - an QObject handle.
- property_name - a string : dynamic property name.
Output argument
- R - a string: method signature.
Description
Undefine a dynamic property of a QObject handle.
See also
QObject_set (set), QObject_get (get).
Example
h = errordlg()
set(h, 'myProp', 33)
h
get(h, 'myProp')
QObject_undefine(h, 'myProp')
get(h, 'myProp')
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
QObject_used
Returns list of current used QObject handle.
Syntax
- r = QObject_used()
Output argument
- h - a vector of QObject handle.
Description
Returns list of current used QObject handle.
See also
QObject_set (set), QObject_get (get).
Example
h1 = errordlg()
h2 = errordlg()
h3 = errordlg()
used = QObject_used()delete(used)
used = QObject_used()
delete(used)
used = QObject_used()
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
nelsonObject
nelson object callable from QML.
Syntax
- nelson.disp(msg)
- nelson.evaluate(cmd)
- nelson.processevent()
- nelson.call(function_name)
- nelson.call(function_name, arg1, ..., arg5)
Input argument
- msg - a string.
- cmd - a string.
- function_name - a string: nelson function name to call
- arg1, ..., arg5 - javascript variables
Description
nelson object contains some methods used as callback to call nelson from QML
See also
qml_pluginpathlist, qml_addimportpath.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
qml_addimportpath
Adds path as directory where the qml engine searches for installed modules.
Syntax
- qml_addimportpath(path)
Input argument
- path - a string : valid path.
Description
qml_addimportpath adds path as a directory where the engine searches for installed modules in a URL-based directory structure.
The newly added path will be first in qml_importpathlist.
Example
qml_importpathlist()
qml_addimportpath(tempdir)
qml_importpathlist()
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
qml_addpluginpath
Adds path as directory where the qml engine searches for native plugins.
Syntax
- qml_addpluginpath(path)
Input argument
- path - a string : valid path.
Description
qml_addpluginpath adds path as a directory where the engine searches for native plugins.
By default, the list contains only .. The newly added path will be first in the qml_pluginpathlist.
Example
qml_pluginpathlist()
qml_addpluginpath(tempdir)
qml_pluginpathlist()
See also
qml_pluginpathlist, qml_addimportpath.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
qml_clearcomponentcache
Clears the engine's internal component cache..
Syntax
- qml_clearcomponentcache
Description
This function causes the property metadata of all components previously loaded by the engine to be destroyed.
All previously loaded components and the property bindings for all extant objects created from those components will cease to function.
Example
qml_clearcomponentcache()
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
qml_collectgarbage
Runs the Qml garbage collector.
Syntax
- qml_collectgarbage
Description
The garbage collector will attempt to reclaim memory by locating and disposing of objects that are no longer reachable in the script environment.
Example
qml_collectgarbage()
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
qml_createqquickview
Load a QML file and creates a window.
Syntax
- h = qml_createqquickview(filename)
Input argument
- filename - a string: a QML filename.
Output argument
- h - a QObject handle.
Description
Load a QML file
It creates a QML component, a window, and load .qml file.
See also
Example
% see examples in [nelsonroot(), '/modules/qml_engine/examples']
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
qml_demos
QML demos.
Syntax
- qml_demos()
Description
qml_demos shows QML -- Nelson demos.
Example
qml_demos()
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
qml_evaluatefile
Evaluates a js file.
Syntax
- r = qml_evaluatefile(filename)
Input argument
- filename - a string: a js filename.
Output argument
- r - a double, logical, int or string.
Description
Evaluates a js file.
If returned value cannot be converted to a basic type, it will converted to string.
See also
Example
test_file = [tempdir() , '/example_qml_evaluatefile.js'];
f = fopen(test_file, 'wt');
fwrite(f, 'a = 2 + 4');
fclose(f);
qml_evaluatefile(test_file)
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
qml_evaluatestring
Evaluates a js string.
Syntax
- r = qml_evaluatestring(string_to_eval)
Input argument
- string_to_eval - a string: a js code.
Output argument
- r - a double, logical, int or string.
Description
Evaluates a js string.
If returned value cannot be converted to a basic type, it will converted to string.
See also
Example
qml_evaluatestring('a = 2 + 4')
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
qml_importpathlist
Returns the list of directories where the engine searches for installed modules in a URL-based directory structure.
Syntax
- p = qml_importpathlist()
Output argument
- p - a cell of strings: paths.
Description
Returns the list of directories where the engine searches for installed modules in a URL-based directory structure.
See also
Example
qml_importpathlist()
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
qml_loadfile
Load a QML file.
Syntax
- h = qml_loadfile(filename)
Input argument
- filename - a string: a QML filename.
Output argument
- h - a QObject handle.
Description
Load a QML file
It creates a QML component and load .qml file.
See also
Example
% see examples in [nelsonroot(), '/modules/qml_engine/examples']
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
qml_loadstring
Load a QML string.
Syntax
- h = qml_loadstring(str_to_eval)
Input argument
- str_to_eval - a string.
Output argument
- h - a QObject handle.
Description
Load a QML string
It creates a QML component and load .qml file.
See also
Example
% see examples in [nelsonroot(), '/modules/qml_engine/examples']
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
qml_offlinestoragepath
Get the Property contains the directory to store offline user data.
Syntax
- p = qml_offlinestoragepath()
Input argument
- path_data - a string
Output argument
- p - a string: path.
Description
Get the Property contains the directory to store offline user data.
See also
Example
qml_offlinestoragepath()
qml_setofflinestoragepath(tmpdir())
qml_offlinestoragepath()
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
qml_pluginpathlist
Returns the list of directories where the engine searches for native plugins for imported modules.
Syntax
- p = qml_pluginpathlist()
Output argument
- p - a string: path.
Description
Returns the list of directories where the engine searches for native plugins for imported modules.
See also
Example
qml_pluginpathlist()
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
qml_setofflinestoragepath
Set the Property contains the directory to store offline user data.
Syntax
- qml_setofflinestoragepath(path_data)
Input argument
- path_data - a string
Description
Set the Property contains the directory to store offline user data.
See also
Example
qml_setofflinestoragepath(tmpdir())
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
qt_constant
Returns Qt constant value.
Syntax
- v = qt_constant(constant_name)
- ce = qt_constant()
Input argument
- constant_name - a string: desired Qt constant.
Output argument
- v - a scalar integer value (Qt constant value).
- ce - a cell with all constant name available.
Description
v = qt_version(constant_name) returns Qt constant value.
Qt 5 family allows to get constant easily with qml_evaluatestring(constant_name), but it is no more available with Qt 6
Example
qt_constant('Qt.WindowModal')
c = qt_constant()
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
qt_version
Returns Qt version used.
Syntax
- v = qt_version()
Output argument
- v - a string : valid path.
Description
v = qt_version() returns the version number of Qt at run-time as a string (for example, "5.15.2").
Example
semver(qt_version(), '>5.15')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
Graphics functions
Graphics functions
Description
graphics functions
- abyss - Abyss colormap array.
- ancestor - Ancestor of graphics object.
- autumn - Autumn colormap array.
- axes - Create cartesian axes.
- axis - Set axis limits and aspect ratios.
- bar - Bar graph.
- bone - Bone colormap array.
- cla - Clear axes.
- clf - Clear figure.
- clim - Set colormap limits.
- close - Close one or more figures
- colorbar - Colorbar showing color scale.
- colormap - View and set current colormap.
- colstyle - Parse color and style from string.
- contour - Contour plot of matrix
- contour3 - Contour 3D plot of matrix
- cool - Cool colormap array.
- copper - Copper colormap array.
- copygraphics - Copy plot to clipboard.
- cylinder - Create cylinder.
- drawnow - Update figures and process callbacks
- figure - Creates an figure window.
- fill - Create filled 2-D patches.
- gca - get current axes graphics object.
- gcf - get current figure graphics object.
- Managing Callback Interruptions in Nelson
- gray - Gray colormap array.
- grid - Display or hide axes grid lines.
- groot - graphic root object.
- hggroup - Create group object.
- hist - Histogram plot.
- hold - Retain current plot when adding new plots.
- hot - Hot colormap array.
- im2double - Convert image to double precision.
- image - Display image from array.
- imagesc - Display image from array with scaled colors.
- imread - Read image from graphics file.
- imshow - Display image.
- imwrite - Write image to graphics file.
- is2D - Checks if ax is a 2-D Polar or Cartesian axes.
- isValidGraphicsProperty - Check property name is valid.
- isgraphics - Check for graphics object.
- ishold - Get current hold state.
- jet - Jet colormap array.
- legend - Add legend to axes.
- line - Create primitive line.
- loglog - Log-log scale plot.
- mesh - Mesh surface plot.
- meshz - Mesh surface plot with curtain.
- newplot - Prepare to produce a new plot.
- pan - Enable pan mode.
- parula - Parula colormap array.
- patch - Create patches of colored polygons
- pcolor - Pseudocolor plot.
- pie - Legacy pie chart.
- pink - Pink colormap array.
- plot - Linear 2-D plot.
- plot3 - 3-D line plot.
- quiver - Vector plot.
- refresh - Redraw current figure.
- rgbplot - Plot colormap.
- ribbon - Ribbon plot.
- rotate3d - Enable rotate mode.
- saveas - Save figure to specific file format.
- scatter - Scatter plot.
- semilogx - Semilog plot (x-axis has log scale).
- semilogy - Semilog plot (y-axis has log scale).
- sky - Sky colormap array.
- sphere - Create sphere.
- spring - Spring colormap array.
- spy - Visualize sparsity pattern of matrix.
- stairs - Stairstep graph.
- stem - Plot discrete sequence data.
- subplot - Create axes in tiled positions.
- summer - Summer colormap array.
- surf - surface plot.
- surface - Primitive surface plot.
- text - creates text descriptions to data points.
- title - Add title.
- turbo - Turbo colormap array.
- uicontrol - Create user interface component.
- validatecolor - Validate color values.
- view - Camera line of sigh.
- viridis - Viridis colormap array.
- waitfor - Wait for condition.
- waitforbuttonpress - Wait for click or key press.
- waterfall - waterfall plot.
- white - white colormap array.
- winter - Winter colormap array.
- xlabel - Label x-axis.
- xlim - set or get x-axis limits.
- ylabel - Label y-axis.
- ylim - set or get y-axis limits.
- zlabel - Label z-axis.
- zlim - set or get z-axis limits.
- zoom - Enable zoom mode.
abyss
Abyss colormap array.
Syntax
- c = abyss
- c = abyss(m)
Input argument
- m - a scalar integer value: Number of colors (256 as default value).
Output argument
- c - Abyss colormap array.
Description
abyss returns the colormap with abyss colors.
Example
f = figure();
surf(peaks);
colormap('abyss');
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
ancestor
Ancestor of graphics object.
Syntax
- p = ancestor(h, type)
- p = ancestor(h, type, 'toplevel')
Input argument
- h - graphics object
- type - a row vector character or cell of strings:
- 'toplevel' - a row vector character: return the highest parent in the object hierarchy that matches the condition.
Output argument
- p - a graphics object or []
Description
ancestor returns the handle of the specified object's ancestor of a given type.
Example
f = figure();
ax = gca();
s = surf(peaks);
AX = ancestor(s, 'axes')
F = ancestor(s, 'figure')
R = ancestor(s, 'root')
See also
gcf.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
autumn
Autumn colormap array.
Syntax
- c = autumn
- c = autumn(m)
Input argument
- m - a scalar integer value: Number of colors (256 as default value).
Output argument
- c - Autumn colormap array.
Description
autumn returns the colormap with autumn colors.
Example
f = figure();
surf(peaks);
colormap('autumn');
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
axes
Create cartesian axes.
Syntax
- ax = axes()
- ax = axes(parent)
- ax = axes(propertyName, propertyValue)
- ax = axes(parent, propertyName, propertyValue)
- axes(cax)
Input argument
- parent - a scalar graphics object value: parent container, specified as a figure.
- cax - axes to make current.
- propertyName - a scalar string or row vector character.
- propertyValue - a value.
Output argument
- ax - a graphics object: axes type.
Description
axes creates axes in the current figure and set it as the current axes.
axes(cax) set current axes.
Clicking on an axis automatically sets it as the current axes object.
Properties:
ALim Alpha limits: two-element vector of the form [amin, amax].
ALimMode Selection mode for ALim: 'manual' or 'auto' (default).
AmbientLightColor Background light color: RGB triplet or string color.
Box Box: 'on' or 'off'.
CLim Color limits: two-element vector of the form [cmin, cmax] or [0 1] (default).
CLimMode Selection mode for CLim: 'manual' or 'auto' (default).
CameraPosition Camera location: vector [x, y, z].
CameraPositionMode Selection mode for CameraPosition: 'manual' or 'auto' (default).
CameraTarget Camera target point: vector [x, y, z].
CameraTargetMode Selection mode for CameraTarget: 'manual' or 'auto' (default).
CameraUpVector Vector defining upwards direction: vector [x, y, z].
CameraUpVectorMode Selection mode for CameraUpVector: 'manual' or 'auto' (default).
CameraViewAngle Field of view: 0 (default) | scalar angle in range [0,180]
CameraViewAngleMode Selection mode for CameraViewAngle: 'manual' or 'auto' (default).
Children Array of graphics objects: A vector containing graphics objects to children of the current axis.
Clipping Clipping of objects to axes limits: 'on' (default) or 'off'.
Color Background color for the axes: RGB triplet, string color or hexadecimal color code.
ColorOrder Color order: three-column matrix of RGB triplets.
ColorOrderIndexColor order index: positive integer value, specifies the next color used.
DataAspectRatio Relative length of data units: vector [x, y, z].
DataAspectRatioMode Data aspect ratio mode: 'manual' or 'auto' (default).
FontAngle Character slant: 'italic' or 'normal' (default).
FontName Font name
FontSize Font size: scalar numeric value
FontUnits Font size units: 'inches', 'centimeters', 'normalized', 'pixels' or 'points' (default).
FontWeight Character thickness: 'bold' or 'normal' (default).
GridAlpha Grid-line transparency (0.15 (default) or value in the range [0, 1]).
GridColor Color of grid lines ([0.15, 0.15, 0.15] (default) or RGB triplet).
GridLineStyle Line style for grid lines: '--' , ':', '-.', 'none' or '-' (default).
HandleVisibility Visibility of object handle: 'on' (default) or 'off'.
HitTest Response to captured mouse clicks: 'on' (default) or 'off'.
BeingDeleted Flag indicating that the object is being deleted.
Interruptible Callback interruption:
Layer Placement of grid lines and tick marks: 'top' or 'bottom' (default).
LineStyleOrder Line style order: character vector, cell array of character vectors, string array or '-' solid line (default).
LineStyleOrderIndexColor order index: positive integer value, property specifies the next line style used.
LineWidth Line width: positive numeric value.
MinorGridLineStyle Line style for minor grid lines: '-', '--', '-.', 'none' or ':' (default).
NextPlot Properties to reset: 'add', 'replacechildren', 'replaceall' or 'replace' (default).
OuterPosition Size and location, including labels and margin: four-element vector.
Parent Parent container: Figure graphics object.
PlotBoxAspectRatio Relative length of each axis: vector [x, y, z].
PlotBoxAspectRatioMode Selection mode for PlotBoxAspectRatio: 'manual' or 'auto' (default).
Position Size and location, excluding margin for labels: four-element vector
PositionMode: 'manual' or 'auto' (default).
Projection Type of projection onto 2-D screen: 'perspective' or 'orthographic' (default).
Selected Selection state: 'on' or 'off' (default).
SelectionHighlight Display of selection graphics objects: 'on' (default) or 'off'.
Tag Object identifier: character vector, string scalar or '' (default).
TickDir Tick mark direction: 'out', 'both', 'none' or 'in' (default).
TickDirMode Selection mode for TickDir: 'manual' or 'auto' (default).
TickLength Tick mark length: two-element vector.
TightInset Margins for text labels: four-element vector [left bottom right top].
Title Text object for title: text object
Type Type of graphics object: 'axes'.
Units Position units: 'inches', 'centimeters', 'points', 'pixels', 'characters' or 'normalized' (default).
UserData User data: array or []
View Azimuth and elevation of view (default: [0 90])
Visible State of visibility: 'on' (default) or 'off'.
XAxisLocation x-axis location: 'top', 'origin' or 'bottom' (default).
XColorColor of axis line, tick values, and labels: RGB triplet.
XDir x-axis direction: 'reverse' or 'normal' (default).
XGrid Grid lines: 'on' or 'off' (default).
XLabel Text object for axis label: text object
XLim Minimum and maximum axis limits: two element vector [min max].
XLimMode Selection mode for axis limits: 'manual' or 'auto' (default).
XMinorGrid Minor grid lines: 'on' or 'off' (default).
XScale Scale of values along axis: 'log' or 'linear' (default).
XTick Tick values: vector of increasing values or [] (default).
XTickLabel Tick labels: cell array of character vectors or '' (default).
XTickLabelMode Selection mode for tick labels: 'manual' or 'auto' (default).
XTickMode Selection mode for tick values: 'manual' or 'auto' (default).
YAxisLocation y-axis location: 'top', 'origin' or 'bottom' (default).
YColorColor of axis line, tick values, and labels: RGB triplet.
YDir y-axis direction: 'reverse' or 'normal' (default).
YGrid Grid lines: 'on' or 'off' (default).
YLabel Text object for axis label : text object
YLim Minimum and maximum axis limits: two element vector [min max].
YLimMode Selection mode for axis limits: 'manual' or 'auto' (default).
YMinorGrid Minor grid lines: 'on' or 'off' (default).
YScale Scale of values along axis: 'log' or 'linear' (default).
YTick Tick values: vector of increasing values or [] (default).
YTickLabel Tick labels: cell array of character vectors or '' (default).
YTickLabelMode Selection mode for tick labels: 'manual' or 'auto' (default).
YTickModeSelection mode for tick values: 'manual' or 'auto' (default).
ZColorColor of axis line, tick values, and labels: RGB triplet.
ZDir z-axis direction: 'reverse' or 'normal' (default).
ZGrid Grid lines: 'on' or 'off' (default).
ZLabel Text object for axis label : text object
ZLim Minimum and maximum axis limits: two element vector [min max].
ZLimMode Selection mode for axis limits: 'manual' or 'auto' (default).
ZMinorGrid Minor grid lines: 'on' or 'off' (default).
ZScale Scale of values along axis: 'log' or 'linear' (default).
ZTick Tick values: vector of increasing values or [] (default).
ZTickLabel Tick labels: cell array of character vectors or '' (default).
ZTickLabelMode Selection mode for tick labels: 'manual' or 'auto' (default).
ZTickModeSelection mode for tick values: 'manual' or 'auto' (default).
CreateFcnCallback (function handle, string or cell) called when object is created. Set this property on an existing component has no effect.
DeleteFcnCallback (function handle, string or cell) called when object is deleted.
Some properties are available only for compatibility and have currently no effect on the axes.
Example
f = figure();
ax1 = axes('Position', [0.1 0.1 0.7 0.7]);
ax2 = axes('Position', [0.65 0.65 0.28 0.28]);
x = linspace(0,10);
y1 = sin(x);
y2 = cos(x);
plot(ax1, x, y1);
plot(ax2, x, y2);
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
1.2.0 | Clicking on an axis automatically sets it as the current axes object. |
-- | GridAlpha, GridColor propertiew for Axes. |
1.7.0 | CreateFcn, DeleteFcn callback added. |
-- | BeingDeleted property added. |
Author
Allan CORNET
axis
Set axis limits and aspect ratios.
Syntax
- axis([xmin, xmax, ymin, ymax, zmin, zmax, cmin, cmax])
- axis([xmin, xmax, ymin, ymax, zmin, zmax])
- axis([xmin, xmax, ymin, ymax])
- axis(style)
- axis(mode)
- axis(visibility)
- lim = axis()
- axis(ax, ...)
Input argument
- [xmin, xmax, ymin, ymax, zmin, zmax, cmin, cmax] - sets the limits in the X, Y, Z and color axes.
- [xmin, xmax, ymin, ymax, zmin, zmax] - sets only the limits in the X, Y, Z.
- [xmin, xmax, ymin, ymax] - sets only the limits in the X, Y.
- style - 'tight', 'equal', 'image', 'square', 'fill', 'vis3d' or 'normal' (default).
- cax - axes.
- visibility - 'off' or 'on' (default).
- mode - 'manual' (turns off automatic scaling of the axis based on the children of the current axis object) or 'auto' (choose automatically all axis limits) .
Output argument
- lim - For 2D: [xmin, xmax, ymin, ymax] or for 3D: [xmin, xmax, ymin, ymax, zmin, zmax]
Description
axes set axis limits and appearance.
Example
f = figure();
t = 0:0.01:2*pi;
x = cos(t);
subplot(2, 2, 1);
plot(t, x);
title ('normal plot');
subplot(2, 2, 2);
plot (t, x);
title('axis square');
axis('square');
subplot(2, 2, 3);
plot (t, x);
title('axis equal');
axis('equal');
subplot(2, 2, 4);
plot (t, x);
title('normal plot again');
axis('normal');
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
bar
Bar graph.
Syntax
- bar(Y)
- bar(X, Y)
- bar(..., width)
- bar(..., color)
- bar(..., propertyName, propertyValue)
- bar(ax, ...)
- b = bar(...)
Input argument
- X - x-coordinates: scalar, vector or string array.
- Y - y-coordinates: vector.
- width - scalar, 0.8 (default).
- color - a scalar string or row vector character: short name color.
- propertyName - a scalar string or row vector character.
- propertyValue - a value.
- ax - Axes object.
Output argument
- b - patch graphics object.
Description
bar(X, Y) creates a bar graph using two sets of X-Y data vectors.
When only one argument is provided (Y), it is interpreted as a vector containing Y values, and the X coordinates are generated as a sequence from 1 to the number of elements in the Y vector.
You can optionally specify the width of the bars.
A value of 1.0 will make each bar exactly touch its neighboring bars, while the default width is set to 0.8.
Examples
f = figure();
y = [ 91 75 123.5 105 150 131 203 179 249 226 281.5];
bar(y);
f = figure();
y = [ 91 75 123.5 105 150 131 203 179 249 226 281.5];
bar(y, 0.5);
f = figure();
x = 1900:10:2000;
y = [75 91 105 123.5 131 150 179 203 226 249 281.5];
bar(x, y, 'r');
f = figure();
x = [ "Summer", "Spring", "Winter", "Autumn"];
y = [ 2 1 4 3];
bar(x, y);
f = figure();
y = [91 75 123.5 105 150 131 203 179 249 226 281.5];
bar(y, 'FaceColor', [0 .5 .5], 'EdgeColor', [0 .9 .9], 'LineWidth', 1.5)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
bone
Bone colormap array.
Syntax
- c = bone
- c = bone(m)
Input argument
- m - a scalar integer value: Number of colors (256 as default value).
Output argument
- c - Bone colormap array.
Description
bone returns the colormap with bone colors.
Example
f = figure();
surf(peaks);
colormap('bone');
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
cla
Clear axes.
Syntax
- cla
- cla(ax)
- ca = cla(...)
Input argument
- ax - a scalar graphics object on an existing axes.
Output argument
- ca - a graphics object: used axes graphics object.
Description
cla clears the current axes.
Example
f = figure();
x = linspace(0, 2*pi);
y = sin(3 * x);
plot(x, y)
sleep(5)
cla
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
clf
Clear figure.
Syntax
- clf
- clf(f)
- F = clf(...)
Input argument
- f - a scalar graphics object on an existing figure.
Output argument
- F - a graphics object: used figure graphics object.
Description
clf clears the current figure.
Example
f = figure();
x = linspace(0, 2*pi);
y = sin(3 * x);
plot(x, y)
sleep(5)
clf
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
clim
Set colormap limits.
Syntax
- clim(limits)
- clim('auto')
- clim('manual')
- clim(ax, ...)
- lims = clim()
Input argument
- limits - New limits: [cmin cmax].
- 'auto' - enables automatic limit updates when values in the colormap indexing array change.
- 'manual' - disables automatic limit update.
- ax - Target object: axes graphics object.
Output argument
- lims - [cmin cmax]
Description
clim set or get colormap limits.
Examples
f = figure();
[X,Y] = meshgrid(-5:.5:5);
Z = X .^ 2 + Y .^ 2;
surf(Z);
limits = clim()
f = figure();
[X,Y] = meshgrid(-5:.5:5);
Z = X.^2 + Y.^2;
surf(Z);
clim([25 75])
limits = clim()
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
close
Close one or more figures
Syntax
- close()
- close('all')
- close(name)
- close(ID)
- close(GO)
- tf = close(...)
Input argument
- ID - a scalar integer value: figure ID.
- GO - a scalar graphics object on an existing figure.
- GO - a scalar graphics object on an existing figure.
Output argument
- tf - a scalar logical: true if figure was closed.
Description
close closes the current figure.
close(ID) closes the figure specified by figure ID.
close(GO) closes the figure specified by figure graphics object.
close('all') closes all figures.
Example
f = figure(1)
close();
h = figure(3)
close(h)
f1 = figure()
f2 = figure()
close('all')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
colorbar
Colorbar showing color scale.
Syntax
- colorbar()
- colorbar('off')
- colorbar(..., propertyName, propertyValue)
- colorbar(target, ...)
- colorbar(target, 'off')
- c = colorbar(...)
Input argument
- propertyName - a scalar string or row vector character.
- propertyValue - a value.
- target - Target: axes.
- 'off' - deletes colorbar associated with the current axes.
Output argument
- c - graphics object: axes on color bar.
Description
colorbaradds a color bar into a plot.
Examples
f = figure();
surf(peaks);
colormap('summer');
colorbar()
f = figure();
surf(peaks);
colormap('gray');
cb = colorbar(gca);
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
colormap
View and set current colormap.
Syntax
- colormap(map)
- colormap(target ,map)
- cmap = colormap()
- cmap = colormap(target)
Input argument
- map - colormap name, 'default' or RGB triplets (matrix).
- target - Target: figure or axes.
Output argument
- cmap - Colormap values: RGB triplets (matrix).
Description
colormapallows to view and set the colormap used into a plot.
Examples
f = figure()
x = linspace(-1, 1, 1024)' * ones(1, 1024);
y = x';
Z = exp(-(x .^ 2 + y .^ 2) / 0.4);
imagesc(Z);
colormap('summer')
f = figure()
x = linspace(-1, 1, 1024)' * ones(1, 1024);
y = x';
Z = exp(-(x .^ 2 + y .^ 2) / 0.4);
imagesc(Z);
colormap('gray')
f = figure()
x = linspace(-1, 1, 1024)' * ones(1, 1024);
y = x';
Z = exp(-(x .^ 2 + y .^ 2) / 0.4);
imagesc(Z);
map = [0 0 0.3;
0 0 0.4;
0 0 0.5;
0 0 0.6;
0 0 0.8;
0 0 1.0];
colormap(map)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
colstyle
Parse color and style from string.
Syntax
- [linespec, colorspec, markerspec, msg] = colstyle (str)
- [linespec, colorspec, markerspec, msg] = colstyle (str, 'plot')
Input argument
- str - a row vector of character or scalar string: line specification.
- 'plot' - linespec returns 'none' and not '' with this option.
Output argument
- linespec - a string: line type.
- colorspec - a string: color part.
- markerspec - a string: marker part.
- msg - a string: contain the error message string.
Description
colstyle parses color and style from string.
Example
[l, c, m, msg] = colstyle('r:x')
[l, c, m, msg] = colstyle('*')
[l, c, m, msg] = colstyle('*', 'plot')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
contour
Contour plot of matrix
Syntax
- contour(Z)
- contour(X, Y, Z)
- contour(..., levels)
- contour(..., LineSpec)
- contour(ax, ...)
- M = contour(...)
- [M, h] = contour(...)
Input argument
- X - x-coordinates: vector or matrix.
- Y - y-coordinates: vector or matrix.
- Z - z-coordinates: vector or matrix.
- levels - Contour levels: scalar or vector.
- LineSpec - Line style and color
- ax - a scalar graphics object value: parent container, specified as a axes.
Output argument
- M - Contour matrix.
- h - a graphics object: contour type.
Description
contour(Z) generates a contour plot representing isolines of the matrix Z. Each isoline corresponds to a specific height value on the x-y plane.
Nelson automatically selects the contour lines to display based on the values in Z. The column and row indices of Z serve as the x and y coordinates in the plane, respectively.
contour(X, Y, Z) allows the user to specify the x and y coordinates corresponding to the values in matrix Z. This enables more precise control over the positioning of the contour plot on the x-y plane.
The matrices X and Y provide the coordinates, while Z contains the height values for generating the contour plot.
Property Name-Value Pairs:
LevelList: The contour levels, specified as a vector of z values, determine the height levels at which the contour lines are drawn. By default, when not explicitly provided, the contour function automatically selects these values to cover the range of values present in the ZData property, ensuring comprehensive coverage of the data range. Default: empty matrix.
LevelListMode: Selection mode for LevelList: 'manual' or 'auto' (default).
LevelStep: Spacing between contour lines: scalar numeric value or 0 (default).
LevelStepMode:Selection mode for LevelStep: 'manual' or 'auto' (default).
EdgeColor: Color of contour lines: rgb color or 'flat' (default).
EdgeAlpha: Contour line transparency: scalar in range [0, 1] or 1 (default).
LineStyle: Line style: '--', ':', '-.' or '-' (default).
LineWidth: Line Width: positive value or 0.5 (default).
ContourMatrix: contour matrix.
XData: x values: vector or matrix or [] (default).
YData: y values: vector or matrix or [] (default).
ZData: z values: vector or matrix or [] (default).
XDataMode: Selection mode for XData: 'manual' or 'auto' (default).
YDataMode: Selection mode for YData: 'manual' or 'auto' (default).
DisplayName: Legend label: character vector, string scalar or '' (default).
Visible: State of visibility: on/off logical value, 'on' (default).
Parent: Parent: Axes object or Group object.
Children: Children.
HandleVisibility: Visibility of handle 'on', 'off'.
Type: Type of graphics object 'contour'.
Tag: Object identifier: character vector, string scalar or '' (default).
UserData: User data: array or [] (default).
CreateFcnCallback (function handle, string or cell) called when object is created. Set this property on an existing component has no effect.
DeleteFcnCallback (function handle, string or cell) called when object is deleted.
BeingDeleted Flag indicating that the object is being deleted.
Examples
f = figure();
subplot(2, 3, 1)
x = linspace(-2 * pi, 2 * pi);
y = linspace(0, 4 * pi);
[X, Y] = meshgrid(x, y);
Z = sin(X) + cos(Y);
contour(X, Y, Z);
subplot(2, 3, 2)
[X, Y, Z] = peaks;
contour(X, Y, Z, 20)
subplot(2, 3, 3)
[X, Y, Z] = peaks;
v = [1,1];
contour(X, Y, Z, v)
subplot(2, 3, 4)
[X, Y, Z] = peaks;
contour(X, Y, Z, '-.')
subplot(2, 3, 5)
Z = peaks;
[M, c] = contour(Z);
c.LineWidth = 3;
subplot(2, 3, 6)
[theta, r] = meshgrid (linspace (0,2*pi,64), linspace (0,1,64));
[X, Y] = pol2cart (theta, r);
Z = sin (2*theta) .* (1-r);
contour (X, Y, abs (Z), 10);
rng('default');
f = figure();
N = 50;
contour(1:N, 1:N, rand(N), 5)
f = figure();
Z = peaks;
Z(:,26) = NaN;
contour(Z)
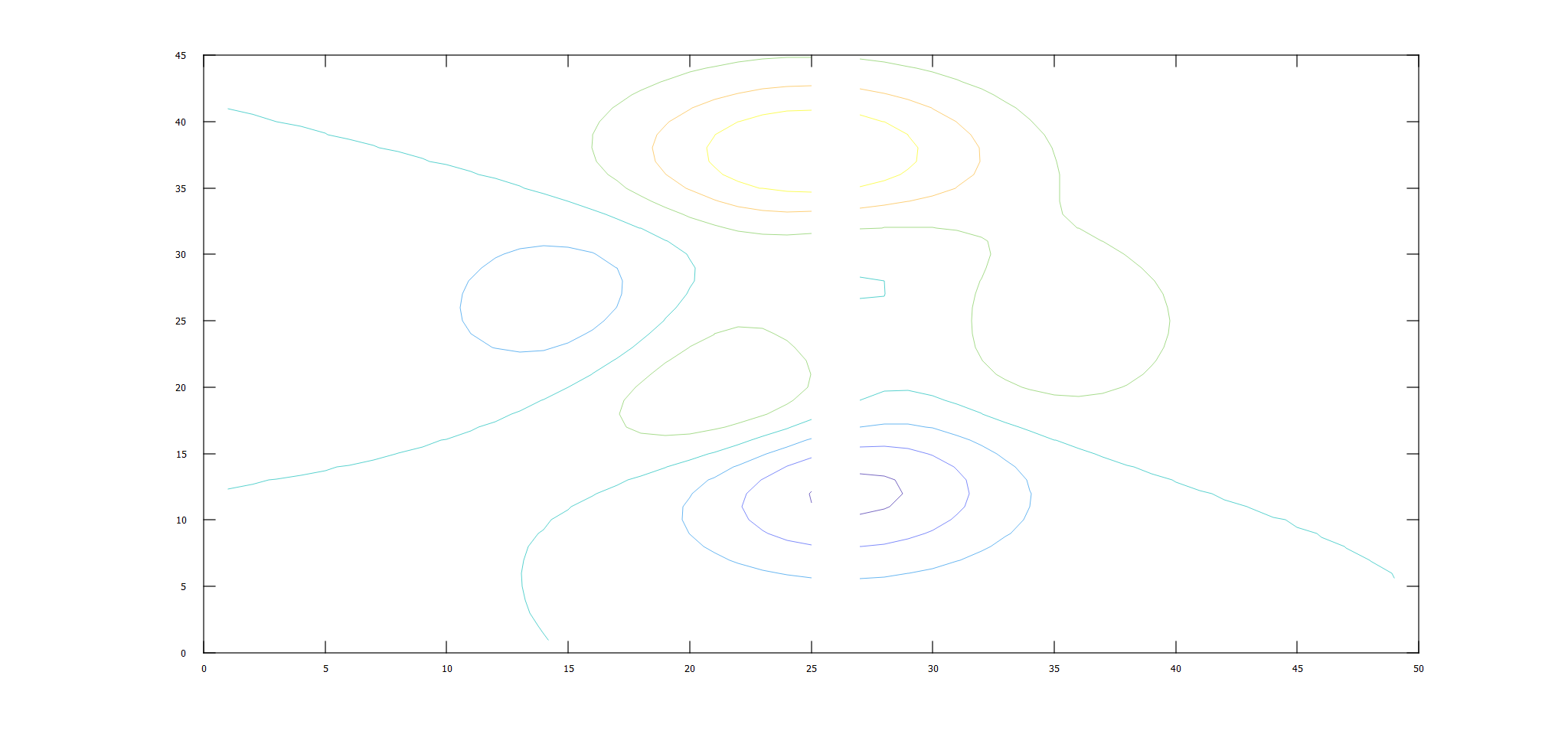
See also
History
Version | Description |
---|---|
1.3.0 | initial version |
1.7.0 | CreateFcn, DeleteFcn callback added. |
-- | BeingDeleted property added. |
Author
Allan CORNET
contour3
Contour 3D plot of matrix
Syntax
- contour3(Z)
- contour3(X, Y, Z)
- contour3(..., levels)
- contour3(..., LineSpec)
- contour3(ax, ...)
- M = contour3(...)
- [M, h] = contour3(...)
Input argument
- X - x-coordinates: vector or matrix.
- Y - y-coordinates: vector or matrix.
- Z - z-coordinates: vector or matrix.
- levels - Contour levels: scalar or vector.
- LineSpec - Line style and color
- ax - a scalar graphics object value: parent container, specified as a axes.
Output argument
- M - Contour matrix.
- h - a graphics object: contour type.
Description
contour3(Z) generates a 3-D contour plot illustrating the isolines of the matrix Z, where Z represents heights on the x-y plane.
The x and y coordinates in the plane correspond to the column and row indices of Z, respectively.
To specify the x and y coordinates for Z values, use contour3(X,Y,Z).
Example
f = figure();
[X,Y,Z] = sphere(50);
[M, C ]= contour3(X,Y,Z);
C.LineWidth = 3;
See also
History
Version | Description |
---|---|
1.3.0 | initial version |
Author
Allan CORNET
cool
Cool colormap array.
Syntax
- c = cool
- c = cool(m)
Input argument
- m - a scalar integer value: Number of colors (256 as default value).
Output argument
- c - Cool colormap array.
Description
cool returns the colormap with cool colors.
Example
f = figure();
surf(peaks);
colormap('cool');
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
copper
Copper colormap array.
Syntax
- c = copper
- c = copper(m)
Input argument
- m - a scalar integer value: Number of colors (256 as default value).
Output argument
- c - Copper colormap array.
Description
copper returns the colormap with copper colors.
Example
f = figure();
surf(peaks);
colormap('copper');
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
copygraphics
Copy plot to clipboard.
Syntax
- copygraphics(fig)
Input argument
- fig - figure object.
Description
copygraphics copy figure to clipboard.
Example
x = -2:0.25:2;
y = x;
[X,Y] = meshgrid(x);
F = X.*exp(-X.^2-Y.^2);
surf(X,Y,F);
copygraphics(gcf());
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
cylinder
Create cylinder.
Syntax
- [X, Y, Z] = cylinder()
- [X, Y, Z] = cylinder(r)
- [X, Y, Z] = cylinder(r, n)
- cylinder()
- cylinder(r)
- cylinder(r, n)
- cylinder(ax, ...)
Input argument
- r - Profile curve: vector.
- n - Number of points: positive whole number.
- ax - Target axes: 'axes' object.
Output argument
- X, Y, Z - x-, y-, and z- coordinates of a cylinder without drawing it.
Description
cylinder creates cylinder and plots it.
Examples
f1 = figure();
colormap(spring)
cylinder()
f2 = figure();
colormap(summer)
r = 4;
cylinder(r);
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
drawnow
Update figures and process callbacks
Syntax
- drawnow()
Description
drawnow flushes the event queue and updates the figure window.
Example
x = -pi:pi/20:pi;
plot(x, cos(x))
drawnow
title('Title Here ...')
grid on
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
figure
Creates an figure window.
Syntax
- f = figure()
- f = figure(ID)
- f = figure(H)
- f = figure(propertyName, propertyValue)
- f = figure(ID, propertyName, propertyValue)
- f = figure(H, propertyName, propertyValue)
Input argument
- ID - a scalar integer value: find or creates with ID.
- H - a scalar graphics object on an existing figure.
- propertyName - a scalar string or row vector character.
- propertyValue - a value.
Output argument
- f - a graphics object: figure handle.
Description
figure creates figure.
Clicking on an figure automatically sets it as the current figure object.
Properties:
AlphaMap: Transparency map for Axes content.
Children: Children of figure: empty array (default) or 1-D array of objects.
Color: Background color [R, G, B] or string (example: 'blue') or hexadecimal color code ('#FFAA00').
Colormap: Color map for axes content of figure: m-by-3 array of RGB triplets, parula (default).
CurrentAxes: Target axes in current figure: Axes object.
Name: Name (default '').
GraphicsSmoothing: GraphicsSmoothing (default 'on').
MenuBar: Figure menu bar display: 'none' or 'figure' (default).
NextPlot: Directive on how to add next plot: 'new', 'replace', 'replacechildren' or 'add' (default).
Number: Figure Number.
NumberTitle: Use number title: 'off' or 'on' (default).
Parent: Figure parent: root graphics object.
Position: Location and size of drawable area: [left, bottom, width, height]
'width' and 'height' define the size of the window. 'left' and 'bottom' define the position of the first addressable pixel in the lower left corner of the window
Resize: Resize figure: 'on' or 'off' (default).
Tag: Object identifier: string scalar, character vector, '' (default).
ToolBar: Figure toolbar display: 'none', 'auto' (default), 'figure'.
Type: Type 'figure'.
UserData: User data: array or [] (default).
Visible: State of visibility: 'off' or 'on' (default).
DrawLater: is used to delay a huge succession of graphics commands (implying several drawings or redrawings): 'on' or 'off' (default).
CloseRequestFcn: Close request callback: function handle, cell array, character vector with 'closereq' (default).
CreateFcn Callback (function handle, string or cell) called when object is created. Set this property on an existing component has no effect.
DeleteFcn Callback (function handle, string or cell) called when object is deleted.
KeyPressFcn Callback (function handle, string or cell) called when a key is pressed while the figure has the focus.
KeyReleaseFcn Callback (function handle, string or cell) called when a key is released while the figure has the focus.
ButtonDownFcn Callback (function handle, string or cell) called when a mouse button is pressed while the figure has the focus.
BeingDeleted Flag indicating that the object is being deleted.
Example
f = figure(1)
g = figure(2)
h = figure(3)
figure(g)
gcf()
figure('Name', 'Hello')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
1.2.0 | Clicking on an figure automatically sets it as the current figure object. |
1.7.0 | CreateFcn, DeleteFcn, CloseRequestFcn, KeyPressFcn, KeyReleaseFcn, ButtonDownFcn callback added. |
-- | BeingDeleted property added. |
1.8.0 | Resize property added. |
Author
Allan CORNET
fill
Create filled 2-D patches.
Syntax
- fill(X, Y, C)
- fill(..., propertyName, propertyValue)
- fill(ax, ...)
- go = fill(...)
Input argument
- X - x-coordinates: vector or matrix.
- Y - y-coordinates: vector or matrix.
- C - Color array: scalar, vector, m-by-n-by-3 array of RGB triplets.
- ax - a scalar graphics object value: parent container, specified as a axes.
- propertyName - a scalar string or row vector character.
- propertyValue - a value.
Output argument
- go - a graphics object: patch type.
Description
fill(X, Y, C) creates a 2D polygonal shape with vertices defined by X and Y coordinates, and fills the shape with color C.
fill(..., PropertyName, PropertyValue, ...) sets optional properties for the fill/patch object using name-value pairs.
go = fill(...) returns the handle go to the created patch object.
Property Name-Value Pairs:
'FaceColor': color of the filled shape. FaceColor can be a character vector or a 3-element RGB vector. Default: 'flat'.
'EdgeColor': color of the edges of the polygonal shape. EdgeColor can be a character vector or a 3-element RGB vector. Default: 'none'.
'LineWidth': width of the edges of the polygonal shape. Default: 0.5.
'LineStyle': style of the edges of the polygonal shape. LineStyle can be a character vector or a line style code. Default: '-'.
'FaceAlpha': transparency of the filled shape. FaceAlpha can be a scalar between 0 and 1. Default: 1.
'EdgeAlpha': transparency of the edges of the polygonal shape. EdgeAlpha can be a scalar between 0 and 1. Default: 1.
'Parent': handle of the parent object for the patch. Default: gca().
'Vertices': matrix of vertex coordinates. The matrix must have size N-by-2 or N-by-3, where N is the number of vertices. Default: the vertex coordinates are specified by the X, Y, and Z input arguments.
Examples
f = figure();
outerX = [0, 0.3, 1, 0.7, 1, 0.3, 0, -0.3, -1, -0.7, -1, -0.3, 0];
outerY = [1, 0.3, 0.3, 0, -0.3, -1, -0.3, -1, -0.3, 0, 0.3, 0.3, 1];
innerX = [0, 0.2, 0.5, 0.35, 0.5, 0.2, 0, -0.2, -0.5, -0.35, -0.5, -0.2, 0];
innerY = [0.6, 0.3, 0.3, 0, -0.3, -0.6, -0.3, -0.6, -0.3, 0, 0.3, 0.3, 0.6];
fill(outerX, outerY, 'y');
fill(innerX, innerY, 'r');
% Define the vertices of a colorful geometric pattern
x1 = [0, 1, 1, 0];
y1 = [0, 0, 1, 1];
x2 = [0.5, 1.5, 1.5, 0.5];
y2 = [0.5, 0.5, 1.5, 1.5];
x3 = [1, 2, 2, 1];
y3 = [1, 1, 2, 2];
% Define colors for the polygons
colors = ['r', 'g', 'b'];
% Create a figure with a white background
figure('Color', 'w');
% Fill the polygons with different colors
fill(x1, y1, colors(1));
hold on;
fill(x2, y2, colors(2));
fill(x3, y3, colors(3));
% Add labels to distinguish the regions
text(0.5, 0.5, 'Polygon 1', 'Color', 'w', 'HorizontalAlignment', 'center', 'FontWeight', 'bold');
text(1.25, 1.25, 'Polygon 2', 'Color', 'w', 'HorizontalAlignment', 'center', 'FontWeight', 'bold');
text(1.5, 0.5, 'Polygon 3', 'Color', 'w', 'HorizontalAlignment', 'center', 'FontWeight', 'bold');
axis equal;
title('Colorful Geometric Pattern');
Alpha channel
f = figure();
x = [10 30 40 30 10 0];
y = [0 0 20 40 40 20];
hold on
fill(x, y, 'cyan', 'FaceAlpha', 0.3);
fill(x + 2, y, 'magenta', 'FaceAlpha', 0.3);
fill(x + 1, y + 2, 'yellow', 'FaceAlpha', 0.3);
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
gca
get current axes graphics object.
Syntax
- ca = gca()
Output argument
- ca - a graphics object: axes graphics object.
Description
ca = gca() returns the current axes graphics object.
If there are no axes, gca() creates an axes and returns its graphics object.
Example
ca = gca()
isgraphics(ax, 'axes')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
gcf
get current figure graphics object.
Syntax
- cf = gcf()
Output argument
- cf - a graphics object: figure graphics object.
Description
cf = gcf() returns the current figure graphics object.
If a figure does not exist, gcf() creates a figure and returns its graphics object.
Example
cf = gcf();
root = groot();
isequal(root.CurrentFigure, cf)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
Managing Callback Interruptions in Nelson
Description
You can assign a callback function to a callback property using one of the following methods:
Function handle: Use this approach when your callback does not need extra input arguments.
Cell array: Ideal for situations where your callback requires additional input arguments. The cell array should include the function handle as the first element, followed by the input arguments.
Anonymous function: This method is suitable for simple callback code or when you want to reuse a function that isn't exclusively used as a callback.
Characters vector or scalar string containing commands.
Nelson provides control over whether a callback function can be interrupted during its execution. In some cases, allowing interruptions might be desirable, such as enabling users to stop an animation loop through an interrupting callback. However, in scenarios where the execution order of callbacks is crucial, it might be necessary to prevent interruptions to maintain the intended behavior, such as ensuring smooth responsiveness in applications that respond to pointer movements.
Callback Interruption Behavior:
Callbacks are executed in the order they are queued. When a callback is running and another user action triggers a second callback, this second callback attempts to interrupt the first one. The first callback is referred to as the "running callback," while the second is the "interrupting callback."
In some cases, specific commands within the running callback prompt Nelson to process any pending callbacks in the queue.
When Nelson encounters one of these commands such as drawnow, figure, waitfor, or pause it evaluates whether an interruption should occur.
No Interruption: If the running callback does not include any of these commands, Nelson will complete the running callback before executing the interrupting callback.
Interruption Conditions: If the running callback includes any of these commands, the behavior depends on the Interruptible property of the object that owns the running callback:
If Interruptible is set to 'on', Nelson allows the interruption. The running callback is paused, the interrupting callback is executed, and once it is finished, Nelson resumes the execution of the running callback.
If Interruptible is set to 'off', the interruption is blocked. The BusyAction property of the interrupting callback then dictates the next step:
If BusyAction is 'queue', the interrupting callback will be executed after the running callback completes.
If BusyAction is 'cancel', the interrupting callback is discarded and not executed.
By default, the Interruptible property is 'on', and BusyAction is 'queue'.
Notably, certain callbacks specifically DeleteFcn, CloseRequestFcn, and SizeChangedFcn will interrupt the running callback regardless of the Interruptible property's value.
Example
uicontrol demo Interruptible
addpath([modulepath('graphics','root'), '/examples/uicontrol'])
edit uicontrol_demo_interruptible
uicontrol_demo_interruptible
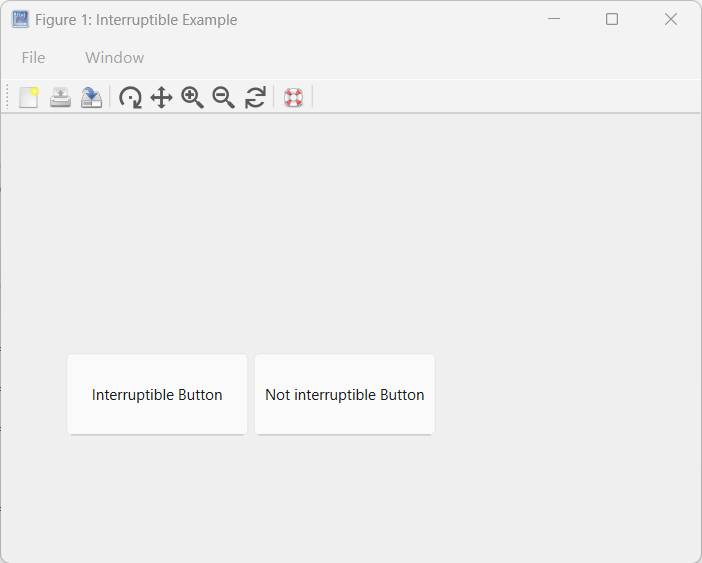
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
gray
Gray colormap array.
Syntax
- c = gray
- c = gray(m)
Input argument
- m - a scalar integer value: Number of colors (256 as default value).
Output argument
- c - Gray colormap array.
Description
gray returns the colormap with gray colors.
Example
f = figure();
surf(peaks);
colormap('gray');
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
grid
Display or hide axes grid lines.
Syntax
- grid
- grid('on')
- grid('off')
- grid('minor')
- grid(ax, ...)
Input argument
- 'on' - displays the major grid line.
- 'off' - removes all grid lines.
- 'minor' - toggles the visibility of the minor grid lines.
- ax - Target object: axes.
Description
grid() toggles the visibility of the major grid lines.
Example
f = figure();
x = linspace(0, 20);
y = cos(x);
plot(x, y)
grid on
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
groot
graphic root object.
Syntax
- g = groot()
Output argument
- g - a graphics object: root object.
Description
groot returns graphic root object.
Properties:
Children: Array of available figure objects.
CurrentFigure: Current figure graphics object.
Parent: empty array (No parent)
PointerLocation: Current location of pointer.
ScreenDepth: Number of bits that define each pixel color.
ScreenSize: Size of primary display (vector).
Tag: Object identifier: string scalar, character vector, '' (default).
Type: Type 'root'.
Units: 'pixels'.
UserData: User data: array or [] (default).
Example
g = groot()
g.ScreenDepth
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
hggroup
Create group object.
Syntax
- h = hggroup()
- h = hggroup(..., propertyName, propertyValue, ...)
- h = hggroup(ax, ...)
Input argument
- ax - graphics object: axes or hggroup.
- propertyName - a scalar string or row vector character.
- propertyValue - a value.
Output argument
- p - a graphics object of type: hggroup
Description
hggroup creates a hggroup object as a child of the current axes and returns its handle, h.
The hggroup object is used to group graphics objects, such as lines, patches, and text, so that they can be manipulated together.
Example
figure();
ax = gca();
g = hggroup();
h = text(0.1, 0.1, 'tttt', 'Parent', g);
h.Parent
h.Visible
h.Visible = 'off';
See also
gca.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
hist
Histogram plot.
Syntax
- hist(x)
- hist(x, nbins)
- hist(ax, ...)
- counts = hist(...)
- [counts, centers] = hist(...)
Input argument
- x - vector or matrix
- nbins - vector.
- ax - Axes object.
Output argument
- counts - Counts of the number of elements in each bin: row vector.
- centers - Bin centers: vector.
Description
A histogram is a graphical representation that illustrates the distribution of data values.
When you use the hist function, it organizes the elements in the vector Y into 10 equally spaced containers and provides the count of elements in each container as a row vector.
hist(Y, x) with a vector x, the function will return the distribution of values in Y among bins determined by the length of x, with centers specified by the values in x.
For instance, if x is a 5-element vector, hist will categorize the elements of Y into five bins, each centered on the x-axis at the values specified in x.
When you use hist(...) without specifying any output arguments, it generates a histogram plot. The bins are distributed along the x-axis between the minimum and maximum values found in the input vector Y.
Example
f = figure();
for i = 1:4
subplot(2, 2, i)
hist(randn(1000, 1), 50)
end
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
hold
Retain current plot when adding new plots.
Syntax
- hold('on')
- hold('off')
- hold('all')
- hold()
- hold(ax, ...)
Input argument
- 'on' - turn hold on.
- 'off' - turn hold off.
- 'all' - same as hold on.
- ax - Target axes: axes.
Output argument
- ax - a graphics object: axes type.
Description
hold allows to construct a plot sequence incrementally.
Example
f = figure();
x = linspace(-pi, pi);
y1 = cos(x);
plot(x, y1)
hold on
y2 = sin(x);
plot(x, y2)
hold off
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
hot
Hot colormap array.
Syntax
- c = hot
- c = hot(m)
Input argument
- m - a scalar integer value: Number of colors (256 as default value).
Output argument
- c - Hot colormap array.
Description
hot returns the colormap with hot colors.
Example
f = figure();
surf(peaks);
colormap('hot');
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
im2double
Convert image to double precision.
Syntax
- IM = im2double(I)
- IM = im2double(I,'indexed')
Input argument
- I - Input image: scalar, vector, matrix or multidimensional array with type single, double, int16, uint8, uint16 or logical.
Output argument
- IM - The converted image is returned as a numeric array with the same dimensions as the input image I with type double.
Description
IM = im2double(I) converts the input image I to double precision format. The input image IM can be a grayscale, truecolor, or binary image. When converting, im2double rescales the pixel values from their original integer format to a floating-point range of [0, 1].
For an indexed image, IM = im2double(I, 'indexed') converts the image I to double precision as well, but with an added offset of 1 to the pixel values during the conversion from integer types.
Example
I = reshape(uint8(linspace(1,255,25)),[5 5]);
IM1 = im2double(I)
IM2 = im2double(I, 'indexed')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
image
Display image from array.
Syntax
- image()
- image(C)
- image(X, Y, C)
- image('CData', C)
- image('XData', X, 'YData', Y,'CData', C)
- image(..., propertyName, propertyValue)
- image(parent, ...)
- go = image(...)
Input argument
- X - x-coordinates: vector or matrix.
- Y - y-coordinates: vector or matrix.
- C - Color array: m-by-n-by-3 array of RGB triplets.
- parent - a scalar graphics object value: parent container, specified as a axes.
- propertyName - a scalar string or row vector character.
- propertyValue - a value.
Output argument
- go - a graphics object: image type.
Description
image displays C data as an image.
Properties:
AlphaData Transparency data: scalar, array the same size as CData, or 1 (default).
AlphaDataMapping
CData Image color data: vector or matrix, 3-D array of RGB triplets.
CDataMapping Color data mapping method: 'scaled' or 'direct' (default).
Children [].
Parent Parent: axes object.
Tag Object identifier: string scalar, character vector, '' (default).
Type Type of graphics object: 'surface'.
UserData: User data: array or [] (default).
Visible State of visibility: 'off' or 'on' (default).
XData Placement along x-axis: two-element vector, scalar, [1 size(CData, 1)] (default).
YData Placement along y-axis: two-element vector, scalar, [1 size(CData, 2)] (default).
CreateFcnCallback (function handle, string or cell) called when object is created. Set this property on an existing component has no effect.
DeleteFcnCallback (function handle, string or cell) called when object is deleted.
BeingDeleted Flag indicating that the object is being deleted.
Example
f = figure();
L = linspace(0, 1);
R = L' * L;
G = L' * (L .^ 2);
B = L' * (0 *L + 1);
C(:, :, 1) = G;
C(:, :, 2) = G;
C(:, :, 3) = B;
im = image(C)
figure();
image();
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
1.7.0 | CreateFcn, DeleteFcn callback added. |
-- | BeingDeleted property added. |
Author
Allan CORNET
imagesc
Display image from array with scaled colors.
Syntax
- imagesc()
- imagesc(C)
- image(X, Y, C)
- imagesc('CData', C)
- imagesc('XData', X, 'YData', Y,'CData', C)
- imagesc(..., propertyName, propertyValue)
- imagesc(parent, ...)
- go = imagesc(...)
Input argument
- X - x-coordinates: vector or matrix.
- Y - y-coordinates: vector or matrix.
- C - Color array: m-by-n-by-3 array of RGB triplets.
- parent - a scalar graphics object value: parent container, specified as a axes.
- propertyName - a scalar string or row vector character.
- propertyValue - a value.
Output argument
- go - a graphics object: image type.
Description
imagessc displays C data as an image. This image is colormapped using the colormap for the current figure.
Properties:
AlphaData Transparency data: scalar, array the same size as CData, or 1 (default).
AlphaDataMapping
CData Image color data: vector or matrix, 3-D array of RGB triplets.
CDataMapping Color data mapping method: 'direct' or 'scaled' (default).
Children [].
Parent Parent: axes object.
Tag Object identifier: string scalar, character vector, '' (default).
Type Type of graphics object: 'surface'.
UserData: User data: array or [] (default).
Visible State of visibility: 'off' or 'on' (default).
XData Placement along x-axis: two-element vector, scalar, [1 size(CData, 1)] (default).
YData Placement along y-axis: two-element vector, scalar, [1 size(CData, 2)] (default).
CreateFcnCallback (function handle, string or cell) called when object is created. Set this property on an existing component has no effect.
DeleteFcnCallback (function handle, string or cell) called when object is deleted.
BeingDeleted Flag indicating that the object is being deleted.
Examples
f1 = figure();
C = [0 2 4 6; 8 10 12 14; 16 18 20 22];
imagesc(C)
f2 = figure();
C = [0 2 4 6; 8 10 12 14; 16 18 20 22];
imagesc(C)
colormap(gray)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
1.7.0 | CreateFcn, DeleteFcn callback added. |
-- | BeingDeleted property added. |
Author
Allan CORNET
imread
Read image from graphics file.
Syntax
- A = imread(filename)
- [A, map] = imread(filename)
- [A, map, transparency] = imread(filename)
Input argument
- filename - a row vector characters or scalar string: name of graphics file.
Output argument
- A - Image data: array.
- map - Colormap: m-by-3 matrix.
- transparency - Transparency information: matrix.
Description
imread reads the image data from the given file into a matrix.
Format | Description |
---|---|
BMP | Windows Bitmap |
GIF | Graphic Interchange Format (optional) |
JPG | Joint Photographic Experts Group |
JPEG | Joint Photographic Experts Group |
PNG | Portable Network Graphics |
PBM | Portable Bitmap |
PGM | Portable Graymap |
PPM | Portable Pixmap |
XBM | X11 Bitmap |
XPM | X11 Pixmap |
Example
f = figure();
filename = [tempdir, 'ngc6543a.gif'];
websave(filename, 'https://solarviews.com/raw/ds/ngc6543a.gif');
img = imread(filename);
imagesc(img);
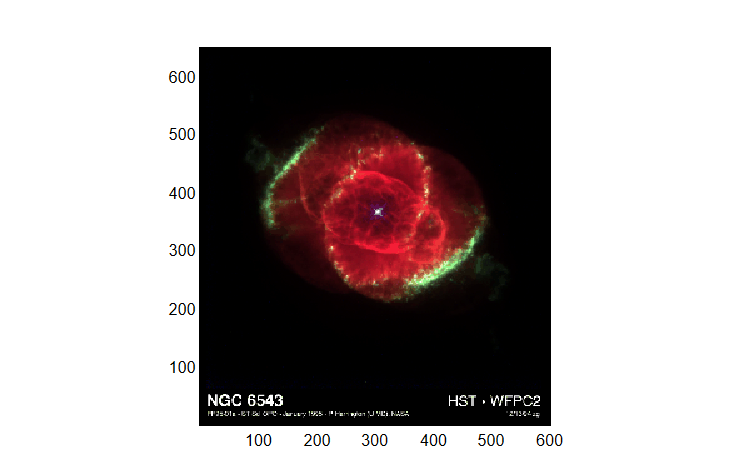
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
imshow
Display image.
Syntax
- imshow(filename)
- imshow(img)
- imshow(RGB)
- imshow(img, [low high])
- imshow(img, [])
- imshow(img, map)
- imshow(..., propertyName, propertyValue)
- go = imshow(...)
Input argument
- filename - row vector character: file name of the image to display.
- img - grayscale image: matrix.
- RGB - truecolor image: m-by-n-by-3 array.
- [low high] - grayscale image display range.
- map - colormap: c-by-3 matrix.
- propertyName - a scalar string or row vector character (for compatibility).
- propertyValue - a value (for compatibility).
Output argument
- go - a graphics object: image type.
Description
imshow(img) displays the image im.
Example
f = figure();
filename = [tempdir, 'apollo_8_earthrise_1968_as08-14-2383.jpg'];
websave(filename, 'https://www.nasa.gov/sites/default/files/thumbnails/image/apollo_8_earthrise_1968_as08-14-2383.jpg');
h = imshow(filename)
See also
imread, image, imagesc, colormap.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
imwrite
Write image to graphics file.
Syntax
- imwrite(A, filename)
- imwrite(A, map, filename)
- imwrite(..., fmt)
- imwrite(..., , propertyName, propertyValue)
Input argument
- A - matrix: 3D for color and 2D for gray or indexed image.
- map - Colormap of indexed image:m-by-3 array.
- fmt - Format of output file: 'bmp', 'png', 'jpg', ...
- filename - a row vector characters or scalar string: name of graphics file.
- propertyName - a scalar string or row vector character.
- propertyValue - a value.
Description
imwrite(A, filename) writes image data A to the file specified by filename
Property name:
Quality: quality of output file: scalar in the range [0, 100] (75 as default).
Alpha: matrix of values in the range [0, 1]: Transparency of each pixel.
Comment: character vector, string scalar, cell array of character vectors or string array: Comment added to image.
Author: character vector or string scalar: Author information.
Example
f = figure();
A = rand(69, 69);
A(:,:,2) = rand(69,69);
A(:,:,3) = rand(69,69);
imshow(A);
imwrite(A, [tempdir, '69x69-RGB.png']);
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
is2D
Checks if ax is a 2-D Polar or Cartesian axes.
Syntax
- tf = is2D(ax)
Input argument
- ax - a scalar graphic object: axe.
Output argument
- tf - a logical scalar.
Description
is2D returns Checks if ax is a 2-D Polar or Cartesian axes.
Example
f = figure();
ax = gca();
plot(ax, 1:10, sin(1:10));
assert_istrue(is2D(ax));
f = figure();
surf(peaks);
ax = gca();
assert_isfalse(is2D(ax));
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
isValidGraphicsProperty
Check property name is valid.
Syntax
- tf = isValidGraphicsProperty(typename, propertyname)
Input argument
- typename - a character vector or scalar string: 'axes', 'line', 'image', 'root', 'text', 'figure'.
- propertyname - a character vector or scalar string: property name to check.
Output argument
- tf - a scalar logical.
Description
isValidGraphicsProperty checks is property name is existing for graphical object class.
This function is an helper to check input parameters graphical functions.
Example
tf = isValidGraphicsProperty('figure', 'Type')
tf = isValidGraphicsProperty('figure', 'TypeType')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
isgraphics
Check for graphics object.
Syntax
- tf = isgraphics(GO)
- tf = isgraphics(GO, type)
Input argument
- GO - variable or graphics object.
- type - a character vector or scalar string: 'axes', 'line', 'image', 'root', 'text', 'figure'.
Output argument
- tf - a scalar logical.
Description
isgraphics checks is variable is an graphics object.
Example
f = figure()
tf = isgraphics(f)
tf = isgraphics(f, 'figure')
tf = isgraphics(f, 'text')
f = 3
tf = isgraphics(f)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
ishold
Get current hold state.
Syntax
- tf = ishold()
- tf = ishold(ax)
Input argument
- ax - scalar graphics object: axes.
Output argument
- tf - a scalar logical: true if it is hold on.
Description
tf = ishold(ax) returns the hold state of the specified axes object.
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
jet
Jet colormap array.
Syntax
- c = jet
- c = jet(m)
Input argument
- m - a scalar integer value: Number of colors (256 as default value).
Output argument
- c - Jet colormap array.
Description
jet returns the colormap with jet colors.
Example
f = figure();
surf(peaks);
colormap('jet');
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
legend
Add legend to axes.
Syntax
- legend()
- legend(label1, ..., labelN)
- legend(labels)
- legend('off')
- legend('hide')
- legend('show')
- legend('toggle')
- legend('boxon')
- legend('boxoff')
- legend(ax, ...)
- legend(..., 'Location', lcn)
- legend(..., propertyName, propertyValue)
- L = legend(...)
Input argument
- label1, ..., labelN - sets the legend labels: row vector characters.
- labels - cell array of character vectors or string array.
- 'off' - delete the legend.
- 'toggle' - toggle legend visibility.
- 'hide' - hide legend.
- 'show' - show legend.
- 'boxon' - display box around legend.
- 'boxoff' - hide box around legend.
- ax - axes to make current.
- lcn - Legend location: a string ('NE' default).
- propertyName - a scalar string or row vector character.
- propertyValue - a value.
Output argument
- L - a graphics object: axes type.
Description
legend creates a legend in the current figure.
Location for legend on the plot:
'northeast' or 'NE': Top right (default).
'north' or 'N': Top center.
'south' or 'S': Bottom center.
'east' or 'E': Middle right.
'west' or 'W': Middle left.
'northwest' or 'NW': Top left.
'southeast' or 'SE': Bottom right.
'southwest' or 'SW': Bottom left.
Example
f = figure();
x = linspace(0,10);
y1 = sin(x);
y2 = cos(x);
ax = gca();
plot(ax, x, y1);
plot(ax, x, y2);
legend('sin(x)', 'cos(x)', 'Location', 'N')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
line
Create primitive line.
Syntax
- go = line()
- po = line(x, y)
- go = line(x, y, z)
- go = line(ax, x, y, z)
- go = line(ax, x, y, z, propertyName, propertyValue)
Input argument
- x, y , z - a scalar graphics object value: parent container, specified as a figure.
- ax - Target axes: axes object.
- propertyName - a scalar string or row vector character.
- propertyValue - a value.
Output argument
- go - a graphics object: line type.
Description
line(x, y) creates a line in the current axes with vectors x and y.
line(x, y, z) creates a line in three-dimensional coordinates.
Properties:
Children [] (currently not used).
Color Line color: RGB triplet, [0, 0, 0] or hexadecimal color code.
DisplayName Legend label: character vector or string scalar, '' (default).
LineStyle Line style: '--', ':', '-.', 'none' or '-' (default).
LineWidth Line width: scalar positive value.
MarkerMarker symbol: 'o' (circle), '+' (Plus sign), '*' (asterik), '.' (point), 'x' (cross), '_' (horizontal line) , '|' (vertical line), 'square', 'diamond', '^' (Upward-pointing triangle), 'v' (Downward-pointing triangle), '>' (Right-pointing triangle), '<' (Left-pointing triangle), 'pentagram', 'hexagram', 'none'(default).
MarkerEdgeColor Marker outline color: RGB triplet.
MarkerFaceColor Marker fill color: RGB triplet.
MarkerSize Marker size: scalar positive value.
Parent Parent: axes graphics object.
Tag Object identifier: string scalar, character vector, '' (default).
Type Type of graphics object: 'line'
UserData User data: array, [] (default).
Visible State of visibility: 'off' or 'on' (default).
XData x values: vector, [0 1] (default).
YData y values: vector, [0 1] (default).
ZData z values: vector, [] (default).
CreateFcnCallback (function handle, string or cell) called when object is created. Set this property on an existing component has no effect.
DeleteFcnCallback (function handle, string or cell) called when object is deleted.
BeingDeleted Flag indicating that the object is being deleted.
Examples
f = figure();
x = linspace(0,10)';
y1 = sin(x);
y2 = cos(x);
line(x, y1, 'Color', [0 1 0])
line(x, y2, 'Color', [1 0 0])
f = figure();
x = [1 9];
y = [2 12];
line(x,y,'Color','red','LineStyle','--')
f = figure();
t = linspace(0,10*pi,400);
x = sin(t);
y = cos(t);
z = t;
line(x,y,z)
view(3)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
1.7.0 | CreateFcn, DeleteFcn callback added. |
-- | BeingDeleted property added. |
Author
Allan CORNET
loglog
Log-log scale plot.
Syntax
- loglog(X, Y)
- loglog(X, Y, LineSpec)
- loglog(Y)
- loglog(Y, LineSpec)
- loglog(ax, ...)
- loglog(..., propertyName, propertyValue)
- go = loglog(...)
Input argument
- X - Log scale coordinates: scalar, vector or matrix.
- Y - Log scale coordinates: scalar, vector or matrix.
- LineSpec - Line style, marker, and/or color: character vector or scalar string.
- ax - a scalar graphics object value: parent container, specified as a axes.
- propertyName - a scalar string or row vector character. see help of 'line' for property list.
- propertyValue - a value.
Output argument
- go - a graphics object: line type.
Description
loglog(X, Y) plots data using a base 10 logarithmic scale for the x-axis and the y-axis.
loglog has the exact same syntax as the plot command.
Examples
f = figure();
x = logspace(-1,2);
y = 2 .^ x;
loglog(x,y)
grid on
f = figure();
x = logspace(-1,2,20);
y = 10 .^ x;
loglog(x,y,'s','MarkerFaceColor',[0 0.447 0.741])
grid on
See also
semilogx, semilogy, line, plot, grid.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
mesh
Mesh surface plot.
Syntax
- mesh(X, Y, Z)
- mesh(Z)
- mesh(Z, C)
- mesh(X, Y, Z, C)
- mesh(parent, ...)
- mesh(..., propertyName, propertyValue)
- go = mesh(...)
Input argument
- X - x-coordinates: vector or matrix.
- Y - y-coordinates: vector or matrix.
- Z - z-coordinates: vector or matrix.
- C - Color array: m-by-n-by-3 array of RGB triplets.
- parent - a scalar graphics object value: parent container, specified as a axes.
- propertyName - a scalar string or row vector character.
- propertyValue - a value.
Output argument
- go - a graphics object: surface type.
Description
mesh creates a 3-D wireframe mesh.
You can customize the appearance of the plot using various options such as color, lighting, and shading.
Examples
f = figure();
[X, Y] = meshgrid(-8:.5:8);
R = sqrt(X.^2 + Y.^2) + eps;
Z = sin(R) ./ R;
mesh(X, Y, Z)
axis square
f = figure();
F = str2func('@(z) z .^ 3 - 1');
x = linspace(-2, 2, 100);
y = linspace(-2, 2, 100);
[X, Y] = meshgrid(x, y);
Z = X + 1i*Y;
W = F(Z);
mesh(real(W), imag(W), abs(W))
xlabel('Real')
ylabel('Imaginary')
zlabel('Magnitude')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
meshz
Mesh surface plot with curtain.
Syntax
- meshz(X, Y, Z)
- meshz(Z)
- meshz(Z, C)
- meshz(X, Y, Z, C)
- meshz(parent, ...)
- meshz(..., propertyName, propertyValue)
- go = meshz(...)
Input argument
- X - x-coordinates: vector or matrix.
- Y - y-coordinates: vector or matrix.
- Z - z-coordinates: vector or matrix.
- C - Color array: m-by-n-by-3 array of RGB triplets.
- parent - a scalar graphics object value: parent container, specified as a axes.
- propertyName - a scalar string or row vector character.
- propertyValue - a value.
Output argument
- go - a graphics object: surface type.
Description
meshz creates a 3-D surface plot with a wireframe plot on top.
The function takes the same input arguments as the mesh function.
Example
f = figure();
[X,Y] = meshgrid(-5:.5:5);
Z = Y.*sin(X) - X.*cos(Y);
s = meshz(X,Y,Z)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
newplot
Prepare to produce a new plot.
Syntax
- go = newplot()
- go = newplot(ax)
Input argument
- ax - specified figure or axes rather than the current figure and axes.
Output argument
- go - a graphics object: axes type.
Description
newplot prepares a figure and axes for graphics commands.
Example
h = newplot()
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
pan
Enable pan mode.
Syntax
- pan
- pan option
- pan(fig, ...)
- pan(ax, ...)
Input argument
- option - string: 'on', 'off', 'out', 'xon', 'yon' or 'toggle'.
- fig - Figure object: Target figure
- ax - a scalar graphics object value: parent container, specified as a axes.
Description
Utilize the pan mode to dynamically adjust axis limits for interactive data exploration.
Enable or disable the pan mode and configure additional basic settings using the pan function.
Pan mode is compatible with various charts like line, bar, histogram, and surface charts. These charts typically feature pan icon on the toolbar to facilitate pan functionality.
pan option configures the pan mode for all axes within the current figure.
Once pan mode is active, you can adjust the view of axes using the cursor, or keyboard:
Cursor: Click and drag the cursor in the axes.
Keyboard: To pan horizontally, press the left arrow (←) or the right arrow (→) key. To pan vertically, press the up arrow (↑) or the down arrow (↓) key.
The pan mode option can be specified using one of the following values:
'toggle': Toggles the pan mode. If pan mode is disabled, 'toggle' reverts to the most recently used pan option of 'on', 'xon', or 'yon'. This option behaves the same as calling pan without any arguments.
'xon': Enables pan mode for the x-dimension exclusively.
'yon': Activates pan mode for the y-dimension exclusively.
'on': Activates pan mode.
'off': Deactivates pan mode. Note that certain default interactions may persist regardless of the interaction mode.
Example
surf(peaks)
pan on
See also
History
Version | Description |
---|---|
1.2.0 | initial version |
Author
Allan CORNET
parula
Parula colormap array.
Syntax
- c = parula
- c = parula(m)
Input argument
- m - a scalar integer value: Number of colors (256 as default value).
Output argument
- c - Parula colormap array.
Description
parula returns the colormap with parula colors.
parula is the default colormap.
Example
f = figure();
surf(peaks);
colormap('parula');
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
patch
Create patches of colored polygons
Syntax
- patch(X, Y, C)
- patch(X, Y, Z, C)
- patch('XData', X, 'YData', Y)
- patch('XData', X, 'YData', Y, 'ZData', Z)
- patch('Faces', F, 'Vertices', V)
- patch(S)
- patch(..., propertyName, propertyValue)
- patch(ax, ...)
- go = patch(...)
Input argument
- X - x-coordinates: vector or matrix.
- Y - y-coordinates: vector or matrix.
- Z - z-coordinates: vector or matrix.
- C - Color array: scalar, vector, m-by-n-by-3 array of RGB triplets.
- ax - a scalar graphics object value: parent container, specified as a axes.
- propertyName - a scalar string or row vector character.
- propertyValue - a value.
- S - a structure with fields that correspond patch property names and field values.
Output argument
- go - a graphics object: patch type.
Description
patch(X, Y, C) creates a 2D polygonal shape with vertices defined by X and Y coordinates, and fills the shape with color C.
patch(X, Y, Z, C) creates a 3D polygonal shape with vertices defined by X, Y, and Z coordinates, and fills the shape with color C.
patch(..., PropertyName, PropertyValue, ...) sets optional properties for the patch object using name-value pairs.
patch('Faces', F, 'Vertices', V) creates one or more polygons .
go = patch(...) returns the handle go to the created patch object.
Property Name-Value Pairs:
'FaceColor': color of the filled shape. FaceColor can be a character vector or a 3-element RGB vector. Default: 'flat'.
'EdgeColor': color of the edges of the polygonal shape. EdgeColor can be a character vector or a 3-element RGB vector. Default: 'none'.
'LineWidth': width of the edges of the polygonal shape. Default: 0.5.
'LineStyle': style of the edges of the polygonal shape. LineStyle can be a character vector or a line style code. Default: '-'.
'FaceAlpha': transparency of the filled shape. FaceAlpha can be a scalar between 0 and 1. Default: 1.
'EdgeAlpha': transparency of the edges of the polygonal shape. EdgeAlpha can be a scalar between 0 and 1. Default: 1.
'Parent': handle of the parent object for the patch. Default: gca().
'Vertices': matrix of vertex coordinates. The matrix must have size N-by-2 or N-by-3, where N is the number of vertices. Default: the vertex coordinates are specified by the X, Y, and Z input arguments.
CreateFcnCallback (function handle, string or cell) called when object is created. Set this property on an existing component has no effect.
DeleteFcnCallback (function handle, string or cell) called when object is deleted.
BeingDeleted Flag indicating that the object is being deleted.
Examples
fig = figure('Color', 'k');
ax.Color = 'k';
f=0.1;
t=0:f^2:2*pi;
r=pi/4;
p=r*t+r;
patch([cos(p), 0], [sin(p), 0], 'y');
c = eye(3);
for a=2:2:6
patch([t/4+a, a+r*(1+cos(t/2)),a], [-f*cos(3*(a+t))-r,r*sin(t/2),-1], c(a/2,:));
patch(a +f*cos(t)'+r./[1,0.65], f*(2+sin(t)').*[1,1], 'k', 'EdgeColor', 'w', 'LineWidth', pi)
end
axis equal
axis off
f =figure('Color', 'w');
x = [-1 1 0 -1];
y = [-1/sqrt(3) -1/sqrt(3) 2*sqrt(3)/3 -1/sqrt(3)];
plot(x,y,'k','LineWidth',3);
t = 0:0.001:2*pi;
xc = cos(t)/3+x';
yc = sin(t)/3-y';
for i = 1:3
patch(xc(i,:),yc(i,:),'k');
end
patch(x,-y,'w','EdgeColor','w');
axis('equal')
axis('off')
Nerfertiti 3D mask
nefertiti_directory = [modulepath('graphics', 'root'), '/examples/nefertiti-mask/'];
load([nefertiti_directory, 'nefertiti-mask.nh5']);
figure('Color', [1, 1, 1]);
patch('Faces', Faces, 'Vertices', Vertices, 'FaceVertexCData', Colors, ...
'EdgeColor', 'none', ...
'FaceColor', 'interp', 'FaceAlpha', 1);
axis equal
axis off
view([0, 0, 1]);
Alpha channel
x = [1 3 4 3 1 0];
y = [0 0 2 4 4 2];
z = [0 0 0 0 0 0];
figure();
hold on
patch(x,y,z,'cyan','FaceAlpha',0.3)
patch(x+2,y,z,'magenta','FaceAlpha',0.3)
patch(x+1,y+2,z,'yellow','FaceAlpha',0.3)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
1.7.0 | CreateFcn, DeleteFcn callback added. |
-- | BeingDeleted property added. |
Author
Allan CORNET
pcolor
Pseudocolor plot.
Syntax
- pcolor(C)
- pcolor(X, Y, C)
- pcolor(parent, ...)
- go = pcolor(...)
Input argument
- X - x-coordinates: vector or matrix.
- Y - y-coordinates: vector or matrix.
- C - Color array: m-by-n-by-3 array of RGB triplets.
- parent - a scalar graphics object value: parent container, specified as a axes.
Output argument
- go - a graphics object: surface type.
Description
pcolor(C) creates a pseudocolor plot of the data in the matrix C, where each cell or 'face' in the plot is colored according to the corresponding value in the matrix.
The color of each face is determined by a colormap, which maps data values to colors.
Examples
X = linspace(0, 2*pi, 100);
Y = linspace(0, 2*pi, 100);
Z = sin(X' * Y);
f = figure()
pcolor(X, Y, Z)
f = figure();
rng('default');
ax1 = subplot(1, 2, 1);
C1 = rand(20, 10);
pcolor(ax1, C1)
ax2 = subplot(1, 2, 2);
C2 = rand(50, 10);
pcolor(ax2, C2)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
pie
Legacy pie chart.
Syntax
- pie(X)
- pie(X, explode)
- pie(X, labels)
- pie(X, explode, labels)
- pie(ax, ...)
- p = pie(...)
Input argument
- X - vector or matrix.
- explode - Offset slices: numeric vector or matrix, logical vector and matrix, string array or cell array of character vectors.
- labels - '%.0f%%' (default) or array of text labels
- ax - Axes object.
Output argument
- p - vector of patch and text objects.
Description
pie(X) generates a pie chart based on the data in the array variable X.
In cases where the sum of the elements in X is less than or equal to 1, the values in X directly represent the proportional areas of the pie slices.
If the sum of X is less than 1, the pie chart displays only a partial pie.
Alternatively, if the sum of X exceeds 1, the function normalizes the values by dividing each element by the sum of X.
This normalization ensures that the pie chart accurately reflects the relative proportions of the data.
In situations where X is a categorical variable, each slice of the pie corresponds to a category, and the area of each slice is determined by the ratio of the number of elements in the category to the total number of elements in X.
Examples
f = figure();
p = pie ([3, 2, 1], [0, 0, 1]);
f = figure();
p = pie([5 9 4 6 3],[0 1 0 1 0]);
f = figure();
p = pie([3 4 6 2],[0 1 0 0],["part1", "part2", "part3", "part4"]);
f = figure();
y2010 = [50 0 100 95];
y2011 = [65 22 97 120];
ax1 = subplot(1, 2, 1);
p1 = pie(ax1, y2010)
title('2010')
ax2 = subplot(1, 2, 2);
p2 = pie(ax2, y2011)
title('2011')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
pink
Pink colormap array.
Syntax
- c = pink
- c = pink(m)
Input argument
- m - a scalar integer value: Number of colors (256 as default value).
Output argument
- c - Pink colormap array.
Description
pink returns the colormap with pink colors.
Example
f = figure();
surf(peaks);
colormap('pink');
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
plot
Linear 2-D plot.
Syntax
- plot(Y)
- plot(X1, Y1, ...)
- plot(X1, Y1, LineSpec, ...)
- plot(..., propertyName, propertyValue, ...)
- plot(ax, ...)
- go = plot(...)
Input argument
- X1 - x-coordinates: vector or matrix.
- Y1 - y-coordinates: vector or matrix.
- LineSpec - Line style, marker, and/or color: character vector or scalar string.
- ax - a scalar graphics object value: parent container, specified as a axes.
- propertyName - a scalar string or row vector character. see help of 'line' for property list.
- propertyValue - a value.
Output argument
- go - a graphics object: line type.
Description
plot(Y) plots the columns of Y versus their index.
plot(X, Y) plots line defined by X versus Y pair.
go = plot(...) returns a column vector of line graphics objects.
LineSpec is a string used to change the characteristics of the line and is composed of three optional parts in any order:
The SymbolSpec specifies the symbol to be drawn at each data point:
'o': Circle symbol
'x': Times symbol
'+': Plus symbol
'*': Asterisk symbol
'.': Dot symbol
's': Square symbol
'd': Diamond symbol
'v': Downward-pointing triangle symbol
'^': Upward-pointing triangle symbol
'>': Left-pointing triangle symbol
'<': Right-pointing triangle symbol
The LineStyleSpec specifies the line style to use for each data series:
'-': Solid line style
'--': Dashed line style
'-.': Dot-Dash-Dot-Dash line style
':': Dotted line style
The ColorSpec specifies the line color to use for each data series:
'k': Color Black
'y': Color Yellow
'm': Color Magenta
'c': Color Cyan
'r': Color Red
'b': Color Blue
'g': Color Green
see line for more information about properties
Examples
Default abscissae using indices:
f = figure()
plot(sin(0:0.1:2*pi))
Using explicit abscissae:
f = figure()
x = [0:0.1:2*pi]';
plot(x, sin(x))
Multiple curves with shared abscissae:
f = figure()
x = [0:0.1:2*pi]';
plot(x, [cos(x), cos(2*x), cos(3*x)])
Color and Size of Markers:
f = figure();
x = -pi:pi/10:pi;
y = tan(sin(x)) - sin(tan(x));
plot(x ,y, '--rs','LineWidth', 2, 'MarkerEdgeColor','k', 'MarkerFaceColor','g', 'MarkerSize', 11)
Adding Title and Axis Labels:
f = figure();
x = linspace(0, 10, 150);
y = sin(5*x);
plot(x,y,'Color',[0,0.7,0.9])
title(_('2-D Line Plot'))
xlabel('x')
ylabel('sin(5x)')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
plot3
3-D line plot.
Syntax
- plot3(X1, Y1, Z1, ...)
- plot3(X1, Y1, Z1, LineSpec, ...)
- plot3(..., propertyName, propertyValue, ...)
- plot3(ax, ...)
- go = plot3(...)
Input argument
- X1 - x-coordinates: vector or matrix.
- Y1 - y-coordinates: vector or matrix.
- Z1 - z-coordinates: vector or matrix.
- LineSpec - Line style, marker, and/or color: character vector or scalar string.
- ax - a scalar graphics object value: parent container, specified as a axes.
- propertyName - a scalar string or row vector character. see help of 'line' for property list.
- propertyValue - a value.
Output argument
- go - a graphics object: line type.
Description
plot3(X1, Y1, Z1, ...)plots one or more lines in three-dimensional space.
go = plot3(...) returns a column vector of line graphics objects.
see line or plot for more information about properties
Examples
f = figure();
t = 0:pi/50:10*pi;
L = plot3(sin(t), cos(t), t);
axis square
f = figure();
t = 0:0.1:10*pi;
r = linspace (0, 1, length(t));
z = linspace (0, 1, length(t));
h = plot3 (r .* cos (t), r .* sin (t), z);
ylabel ('r .* sin (t)');
xlabel ('r .* cos (t)');
zlabel ('z');
title (_('plot3 display of 3-D helix'));
axis square
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
quiver
Vector plot.
Syntax
- quiver(X, Y, U, V)
- quiver(U, V)
- quiver(..., LineSpec)
- quiver(..., propertyName, propertyValue)
- quiver(parent, ...)
- gr = quiver(...)
Input argument
- X - x-coordinates of bases of arrows: scalar, vector or matrix.
- Y - y-coordinates of bases of arrows: scalar, vector or matrix.
- U - x-components: scalar, vector or matrix.
- V - y-components: scalar, vector or matrix.
- LineSpec - Line style, marker and/or color: character vector or scalar string.
- parent - a scalar graphics object value: parent container, specified as a axes.
- propertyName - a scalar string or row vector character.
- propertyValue - a value.
Output argument
- gr - group of graphics object: arrows.
Description
quiver(X, Y, U, V) plots velocity vectors as arrows with components (U,V) at the point (X, Y).
quiver try to scale the arrow lengths so that they do not overlap.
Current implementation is slow but it can be optimized using DrawLater property of figure. It could be optimized in an next version by an builtin.
Examples
f = figure();
f.DrawLater = 'on';
[X, Y] = meshgrid(0:pi/8:pi, -pi:pi/8:pi);
U1 = sin(X);
V1 = cos(Y);
quiver(U1,V1, 'r')
f.DrawLater = 'off';
f = figure();
f.DrawLater = 'on';
[X, Y] = meshgrid(0:pi/8:pi, -pi:pi/8:pi);
U1 = sin(X);
V1 = cos(Y);
U2 = sin(Y);
V2 = cos(X);
ax1 = subplot(1, 2, 1);
axis equal
title(ax1, 'Left Plot')
quiver(ax1, X, Y, U1, V1)
ax2 = subplot(1, 2, 2);
quiver(X,Y,U2,V2)
axis equal
title(ax2, 'Right Plot')
f.DrawLater = 'off';
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
refresh
Redraw current figure.
Syntax
- refresh()
- refresh(F)
Input argument
- F - figure graphics object.
Description
refresh erases and redraws the current figure.
refresh(F) redraws the figure identified by F.
See also
clf.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
rgbplot
Plot colormap.
Syntax
- rgbplot(cmap)
Input argument
- cmap - Colormap: three-column matrix of RGB triplets .
Description
rgbplot(cmap) plots the R (red), G (green), and B (blue) intensities of the specified cmap colormap.
Example
f = figure();
colormap = [0.2 0.1 0.5;
0.1 0.5 0.8;
0.2 0.7 0.6;
0.8 0.7 0.3;
0.9 1 0];
rgbplot(colormap);
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
ribbon
Ribbon plot.
Syntax
- ribbon(Z)
- ribbon(Y, Z)
- ribbon(Y, Z, width)
- ribbon(ax, ...)
- s = ribbon(...)
Input argument
- Z - z-coordinates: vector or matrix.
- Y - y-coordinates: vector or matrix.
- width - ribbon width.
- ax - a scalar graphics object value: parent container, specified as a axes.
Output argument
- s - a vector of surface objects.
Description
ribbon(Z) plots a 3D ribbon graph based on the matrix Z with the values of Y defining the y-axis of the graph.
ribbon(Y, Z) plots a 3D ribbon graph based on the matrix Y with the values of Z defining the z-axis of the graph.
s = ribbon(...) returns a vector of surface objects.
Note that Y and Z must have the same size.
Example
f = figure();
Y = peaks(25);
ribbon(Y)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
rotate3d
Enable rotate mode.
Syntax
- rotate3d
- rotate3d option
- rotate3d(fig, ...)
- rotate3d(ax, ...)
Input argument
- option - string: 'on', 'off' or 'toggle'.
- fig - Figure object: Target figure
- ax - a scalar graphics object value: parent container, specified as a axes.
Description
Utilize rotate mode to interactively rotate the 3-D view of the axes for data exploration. Enable or disable rotate mode and configure additional basic options using the rotate3d function.
rotate3d option establishes the rotate mode for all axes within the current figure. For instance, rotate3d on activates rotate mode, while rotate3d off deactivates it.
When rotate mode is enabled, you can adjust the view of axes using the cursor or the keyboard:
Cursor: Click and drag within the axes.
Keyboard: Use the right arrow (→) or left arrow (←) keys to adjust azimuth, and the up arrow (↑) or down arrow (↓) keys to modify elevation.
Example
surf(peaks)
rotate3d
See also
History
Version | Description |
---|---|
1.2.0 | initial version |
Author
Allan CORNET
saveas
Save figure to specific file format.
Syntax
- saveas(fig, filename)
- saveas(fig, filename, formattype)
Input argument
- fig - figure object.
- filename - character vector or scalar string: destination filename.
- formattype - character vector or scalar string: extension filename.
Description
saveas save figure to specific file format.
supported formats:
Option | Format | File extension |
---|---|---|
svg | SVG (scalable vector graphics) | .svg |
Full page Portable Document Format (PDF) color | ||
png | PNG 24-bit | .png |
jpg | JPEG 24-bit | .jpg |
Example
x = -2:0.25:2;
y = x;
[X,Y] = meshgrid(x);
F = X.*exp(-X.^2-Y.^2);
surf(X,Y,F);
saveas(gcf(), 'svg-file.svg');
See also
gcf.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
scatter
Scatter plot.
Syntax
- scatter(x, y)
- scatter(x, y, sz)
- scatter(x, y, sz, c)
- scatter(..., 'filled')
- scatter(..., marker)
- scatter(ax, ...)
- scatter(..., propertyName, propertyValue)
- s = scatter(...)
Input argument
- X - x-coordinates: vector or matrix.
- Y - y-coordinates: vector or matrix.
- sz - Marker size: numeric scalar, vector, [] (default: 36)
- c - Marker color: color name, RGB triplet or vector of colormap indices
- ax - a scalar graphics object value: parent container, specified as a axes.
- propertyName - a scalar string or row vector character. see help of 'line' for property list.
- propertyValue - a value.
Output argument
- s - a graphics object: line type or group of line.
Description
scatter(x, y) generates a scatter plot by placing circular markers at the coordinates defined by the vectors x and y.
If you intend to display a single dataset, ensure that both x and y are vectors of the same length.
To visualize multiple datasets on a shared set of axes, you can achieve this by using a matrix for either x or y, while keeping the other as a vector.
This allows you to overlay or compare multiple datasets within the same plot.
marker specifies the symbol to be drawn at each data point:
'o': Circle symbol
'x': Times symbol
'+': Plus symbol
'*': Asterisk symbol
'.': Dot symbol
's': Square symbol
'd': Diamond symbol
'v': Downward-pointing triangle symbol
'^': Upward-pointing triangle symbol
'>': Left-pointing triangle symbol
'<': Right-pointing triangle symbol
The ColorSpec specifies the marker color to use for each data series:
'k': Color Black
'y': Color Yellow
'm': Color Magenta
'c': Color Cyan
'r': Color Red
'b': Color Blue
'g': Color Green
see line for more information about properties
Examples
f = figure();
theta = linspace(0,1,600);
x = exp(theta).*sin(110*theta);
y = exp(theta).*cos(110*theta);
s = scatter(x,y ,'filled');
f = figure();
x = linspace(0,3*pi,255);
y = cos(x) + rand(1,255);
sz = 1:255;
c = 1:length(x);
scatter(x, y, sz, c, 'd', 'filled')
f = figure();
x = linspace(0, 3*pi, 255);
y = cos(x) + rand(1, 255);
c = linspace(1,10,length(x));
scatter(x, y, [], c, 'filled')
f = figure();
theta = linspace(0,2*pi,244);
x = sin(theta) + 0.75*rand(1,244);
y = cos(theta) + 0.75*rand(1,244);
sz = 45;
scatter(x,y,sz,'MarkerEdgeColor',[0 .6 .5], 'MarkerFaceColor',[0 .6 .7], 'LineWidth',3.5)
f = figure(),
x = linspace(0,3*pi,200);
y = cos(x) + rand(1,200);
% Top plot
ax1 = subplot(2,1, 1);
scatter(ax1,x,y)
% Bottom plot
ax2 = subplot(2,1, 2);
scatter(ax2,x,y,'filled','d')
f = figure();
x = rand(500,5);
y = randn(500,5) + (5:5:25);
s = scatter(x,y, 'filled');
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
semilogx
Semilog plot (x-axis has log scale).
Syntax
- semilogx(X, Y)
- semilogx(X, Y, LineSpec)
- semilogx(Y)
- semilogx(Y, LineSpec)
- semilogx(ax, ...)
- semilogx(..., propertyName, propertyValue)
- go = semilogx(...)
Input argument
- X - Log scale coordinates: scalar, vector or matrix.
- Y - Linear scale coordinates: scalar, vector or matrix.
- LineSpec - Line style, marker, and/or color: character vector or scalar string.
- ax - a scalar graphics object value: parent container, specified as a axes.
- propertyName - a scalar string or row vector character. see help of 'line' for property list.
- propertyValue - a value.
Output argument
- go - a graphics object: line type.
Description
semilogx(X, Y) plots data using a base 10 logarithmic scale for the x-axis and a normal (linear) scale for the y-axis.
semilogx has the exact same syntax as the plot command.
Examples
f = figure();
x = logspace(-1,2);
semilogx(x, x);
grid on
f = figure();
x = logspace(-1, 2, 15);
y = 13 + x;
semilogx(x, y, 'x', 'MarkerFaceColor', [0 0.447 0.741])
grid on
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
semilogy
Semilog plot (y-axis has log scale).
Syntax
- semilogy(X, Y)
- semilogy(X, Y, LineSpec)
- semilogy(Y)
- semilogy(Y, LineSpec)
- semilogy(ax, ...)
- semilogy(..., propertyName, propertyValue)
- go = semilogy(...)
Input argument
- X - Linear scale coordinates: scalar, vector or matrix.
- Y - Log scale coordinates: scalar, vector or matrix.
- LineSpec - Line style, marker, and/or color: character vector or scalar string.
- ax - a scalar graphics object value: parent container, specified as a axes.
- propertyName - a scalar string or row vector character. see help of 'line' for property list.
- propertyValue - a value.
Output argument
- go - a graphics object: line type.
Description
semilogy(X, Y) plots data using a base 10 logarithmic scale for the y-axis and a normal (linear) scale for the x-axis.
semilogy has the exact same syntax as the plot command.
Examples
f = figure();
x = 1:100;
y1 = x.^2;
y2 = x.^3;
semilogy(x,y1,'--',x,y2)
legend('x^2','x^3','Location','northwest')
f = figure();
y = [ 0.1 1 10
0.2 2 20
1.0 10 100
10 100 1000
1000 10000 100000];
semilogy(y)
grid on
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
sky
Sky colormap array.
Syntax
- c = sky
- c = sky(m)
Input argument
- m - a scalar integer value: Number of colors (256 as default value).
Output argument
- c - Sky colormap array.
Description
sky returns the colormap with sky colors.
Example
f = figure();
surf(peaks);
colormap('sky');
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
sphere
Create sphere.
Syntax
- [X, Y, Z] = sphere()
- [X, Y, Z] = sphere(n)
- sphere()
- sphere(n)
- sphere(ax, n)
Input argument
- n - Number of points: positive whole number.
- ax - Target axes: 'axes' object.
Output argument
- X, Y, Z - x-, y-, and z- coordinates of a sphere without drawing it.
Description
sphere creates sphere and plots it.
Example
f = figure();
colormap(gray);
subplot(1, 3, 1);
ax1 = gca();
sphere(ax1);
axis equal
title(_('20-by-20 faces (Default)'));
subplot(1, 3, 2);
ax2 = gca();
sphere(ax2, 50);
axis equal
title(_('50-by-50 faces'));
subplot(1, 3, 3);
ax3 = gca();
sphere(ax3,100);
axis equal
title(_('100-by-100 faces'));
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
spring
Spring colormap array.
Syntax
- c = spring
- c = spring(m)
Input argument
- m - a scalar integer value: Number of colors (256 as default value).
Output argument
- c - Spring colormap array.
Description
spring returns the colormap with spring colors.
Example
f = figure();
surf(peaks);
colormap('spring');
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
spy
Visualize sparsity pattern of matrix.
Syntax
- spy(S)
- spy(S, LineSpec)
- spy(S, LineSpec, MarkerSize)
Input argument
- S - matrix: sparse or dense.
- LineSpec - Line style, marker, and/or color: character vector or scalar string.
- MarkerSize - positive integer scalar value.
Description
spy(S) plots the sparsity pattern of the sparse matrix S.
Examples
f = figure();
rng('default');
S = sparse((rand(1, 10) + 1) * 100, (rand(1, 10) + 1) * 100 , (rand(1, 10) + 1) * 10);
spy(S);
f = figure();
rng('default');
S = sparse((rand(1, 10) + 1) * 100, (rand(1, 10) + 1) * 100 , (rand(1, 10) + 1) * 100);
spy(S, 45);
f = figure();
spy();
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
stairs
Stairstep graph.
Syntax
- stairs(Y)
- stairs(X, Y)
- stairs(..., LineSpec)
- stairs(..., Name, Value)
- stairs(ax, ...)
- h = stairs(...)
- [xb, yb] = stairs(...)
Input argument
- X - x values.
- Y - y values.
- LineSpec - Line style, marker and/or color: character vector or scalar string.
- propertyName - a scalar string or row vector character.
- propertyValue - a value.
- ax - Axes object.
Output argument
- h - line object.
- xb - x values for use with plot
- yb - y values for use with plot
Description
Stairstep graphs are a valuable tool for creating time-history plots of digitally sampled data.
stairs(Y) function is used to generate such graphs by plotting the elements of the vector Y.
If Y is a matrix, it draws one line for each column, with the color of the lines determined by the ColorOrder property of the axes.
In the case of a vector Y, the x-axis scale spans from 1 to the length of Y, while for a matrix Y, the x-axis scale ranges from 1 to the number of rows in Y.
stairs(X, Y) allows you to plot the elements in Y at specific locations defined by the vector X.
It's important to note that the elements in X must be in a monotonic order to create a valid stairstep graph.
Examples
f = figure();
f = figure();
x1 = linspace(0,2*pi)';
x2 = linspace(0,pi)';
X = [x1,x2];
Y = [sin(5*x1),exp(x2).*sin(5*x2)];
ax = gca();
stairs(ax, X,Y)
X = linspace(0,1,45)';
Y = [cos(3*X), exp(X).*sin(9*X)];
h = stairs(X,Y);
h(1).Marker = 'o';
h(1).MarkerSize = 5;
h(2).Marker = '+';
h(2).MarkerFaceColor = 'm';
See also
plot.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
stem
Plot discrete sequence data.
Syntax
- stem(Y)
- stem(X, Y)
- stem(..., 'filled')
- stem(..., LineSpec)
- stem(..., propertyName, propertyValue)
- stem(ax, ...)
- go = stem(...)
Input argument
- X - Locations to plot data values in Y.
- Y - Data sequence to display.
- LineSpec - Line style, marker and/or color: character vector or scalar string.
- propertyName - a scalar string or row vector character.
- propertyValue - a value.
- ax - Axes object.
Output argument
- gr - group of graphics object.
Description
A two-dimensional stem plot is a way to visualize data by representing it as lines extending from a horizontal baseline along the x-axis.
At the end of each line, there is a circle (which is the default marker), and the vertical position of this circle corresponds to the value of the data it represents.
stem(Y) creates a stem plot by taking the data sequence Y and drawing stems that extend from regularly spaced and automatically determined points along the x-axis.
If Y is a matrix, the stem function plots all elements in a row against the same x-value.
stem(X, Y) creates a stem plot that shows how X relates to the columns of Y.
Both X and Y can be vectors or matrices of the same size.
X can be either a row or a column vector, and Y should be a matrix with the same number of rows as the length of X.
If you want to specify whether to fill the circle at the end of each stem, you can use stem(...,'fill').
Moreover, by using stem(..., LineSpec), you can define the line style, marker symbol, and color for the stems and the top marker.
Refer to LineSpec for more details on how to customize the appearance of the stem plot.
Examples
f = figure();
x = 1:10;
y = 2*x;
h = stem (x, y, 'MarkerFaceColor', [1 0 1]);
title('stem plot modified with property/value pair');
f =figure();
% Defining base line - X input vector ranging from 0 to 2*pi
X = 0 : pi/100 : 2*pi;
% Defining the Y input vector as function of X
Y = exp(-3*X/4) .* cos(2*X);
% Third, we use the 'stem' function to plot discrete values
stem(X,Y)
See also
plot.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
subplot
Create axes in tiled positions.
Syntax
- subplot(m, n, p)
- subplot('mnp')
- subplot('Position', pos)
- ax = subplot(...)
Input argument
- m - Number of grid rows: scalar positive integer.
- n - Number of grid columns: scalar positive integer.
- p - Grid position for new axes: scalar or vector.
- pos - Custom position for new axes: [left bottom width height].
Output argument
- ax - a graphics object: axes type.
Description
subplot(n, m, p) divides the current figure into a 2-dimensional grid.
Each of which can contain a plot of some kind.
Examples
f = figure();
X = linspace(-pi, pi) * 2;
Y1 = cos(X) .* exp(-2 * X);
Y2 = cos(X * 2) .* exp(-2 * X);
Y3 = cos(X * 3) .* exp(-2 * X);
Y4 = cos(X * 4) .* exp(-2 * X);
subplot(4, 1, 1)
plot(X, Y1,'b');
subplot(4, 1, 2)
plot(X, Y2, 'r');
subplot(4, 1, 3);
plot(X, Y3, 'g');
subplot(4, 1, 4);
plot(X, Y4, 'k');
f = figure();
t = 0 : (2 * pi/100) : (2 * pi);
X = cos(t * 2) .* (2 + sin(t * 3) * 0.3);
Y = sin(t * 2) .* (2 + sin(t * 3) * 0.3);
Z = cos(t * 3) * 0.3;
subplot(2, 2, 1)
surf(peaks());
axis equal
view(3)
subplot(2, 2, 2);
plot(t, X);
subplot(2, 2, 3);
plot(t, Y);
subplot(2, 2, 4);
plot(t, Z);
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
summer
Summer colormap array.
Syntax
- c = summer
- c = autumn(m)
Input argument
- m - a scalar integer value: Number of colors (256 as default value).
Output argument
- c - Summer colormap array.
Description
summer returns the colormap with summer colors.
Example
f = figure();
surf(peaks);
colormap('summer');
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
surf
surface plot.
Syntax
- surf(X, Y, Z)
- surf(X, Y, Z, C)
- surf(Z)
- surf(Z, C)
- surf(parent, ...)
- surf(..., propertyName, propertyValue)
- go = surf(...)
Input argument
- X - x-coordinates: vector or matrix.
- Y - y-coordinates: vector or matrix.
- Z - z-coordinates: vector or matrix.
- C - Color array: m-by-n-by-3 array of RGB triplets.
- parent - a scalar graphics object value: parent container, specified as a axes.
- propertyName - a scalar string or row vector character.
- propertyValue - a value.
Output argument
- go - a graphics object: surface type.
Description
surf creates a 3D surface plot. It can be used to plot data in the form of a matrix or a function of two variables.
You can customize the appearance of the plot using various options such as color, lighting, and shading.
For example, you can use the colormap option to change the color of the surface, and the FaceLighting option to change the lighting of the surface.
Properties:
AlphaData Transparency data: array same size as ZData or 1 (default).
AlphaDataMapping Interpretation of AlphaData values: 'direct', 'none' or 'scaled' (default).
AmbientStrength Strength of ambient light: scalar in [0, 1].
BackFaceLighting Face lighting when normals point away from camera: 'unlit', 'lit' or 'reverselit' (default).
CData Vertex colors: 2-D or 3-D array.
CDataMapping Color mapping method: 'direct', 'scaled' (default).
CDataMode Selection mode for CData: 'manual', 'auto' (default).
Children currently not used: []
DiffuseStrength Strength of diffuse light: scalar in range [0, 1].
EdgeAlpha Edge transparency: scalar value in range[0, 1].
EdgeColor Edge line color: RGB triplets.
EdgeLighting Effect of light objects on edges: 'flat', 'gouraud' or 'none' (default).
FaceAlpha Face transparency: scalar in range [0, 1].
FaceColor Face color: RGB triplet.
FaceLighting Effect of light objects on faces: 'gouraud', 'none' or 'flat' (default).
LineStyle Line style: '--', ':', '-.', 'none' or '-' (default).
LineWidth Line width: positive value, 0.5 (default).
MarkerMarker symbol: 'o' (circle), '+' (Plus sign), '*' (asterik), '.' (point), 'x' (cross), '_' (horizontal line) , '|' (vertical line), 'square', 'diamond', '^' (Upward-pointing triangle), 'v' (Downward-pointing triangle), '>' (Right-pointing triangle), '<' (Left-pointing triangle), 'pentagram', 'hexagram', 'none'(default).
MarkerEdgeColor Marker outline color: RGB triplet.
MarkerFaceColor Marker fill color: RGB triplet.
MarkerSize Marker size: scalar positive value.
MeshStyle Edges to display: 'row', 'column' or 'both' (default).
Parent Parent: axes object.
SpecularColorReflectance Color of specular reflections: scalar in range [0, 1].
SpecularExponent Size of specular spot: scalar greater than or equal to 1.
SpecularStrength Strength of specular reflection: scalar in range [0, 1].
Tag Object identifier: character vector, string scalar or '' (default).
Type Type of graphics object: 'surface'.
UserData User data: array or [] (default).
VertexNormals Normal vectors for each surface vertex: m-by-n-by-3 array or [] (default).
Visible State of visibility: 'off' or 'on' (default).
XData x-coordinate data: vector or matrix.
XDataMode Selection mode for XData: 'manual' or 'auto'.
YData y-coordinate data: vector or matrix.
YDataMode Selection mode for YData: 'manual' or 'auto'.
ZData z-coordinate data: vector or matrix.
CreateFcnCallback (function handle, string or cell) called when object is created. Set this property on an existing component has no effect.
DeleteFcnCallback (function handle, string or cell) called when object is deleted.
BeingDeleted Flag indicating that the object is being deleted.
Some properties are available only for compatibility and have currently no effect on the surface.
Examples
f = figure();
[X, Y, Z] = peaks(35);
C(:, :, 1) = zeros(35);
C(:, :, 2) = ones(35) .* linspace(0.5, 0.6, 35);
C(:, :, 3) = ones(35) .* linspace(0, 1, 35);
S = surf(X, Y, Z, C)
f = figure();
[X,Y] = meshgrid(-8:.5:8);
R = sqrt(X.^2 + Y.^2) + eps;
Z = sin(R)./R;
h = surf(X, Y, Z);
axis square
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
1.7.0 | CreateFcn, DeleteFcn callback added. |
-- | BeingDeleted property added. |
Author
Allan CORNET
surface
Primitive surface plot.
Syntax
- surface(X, Y, Z)
- surface(X, Y, Z, C)
- surface(Z)
- surface(Z, C)
- surface(parent, ...)
- surface(..., propertyName, propertyValue)
- go = surface(...)
Input argument
- X - x-coordinates: vector or matrix.
- Y - y-coordinates: vector or matrix.
- Z - z-coordinates: vector or matrix.
- C - Color array: m-by-n-by-3 array of RGB triplets.
- parent - a scalar graphics object value: parent container, specified as a axes.
- propertyName - a scalar string or row vector character.
- propertyValue - a value.
Output argument
- go - a graphics object: surface type.
Description
surf and surface functions are both used to create 3D surface plots, but there are some slight differences between the two.
surf function is used to plot a surface defined by a function of two variables, or by a set of scattered data points.
It requires three input arguments: X, Y, and Z. X and Y define the coordinates of the data points, and Z defines the height of the surface at each point.
surf function also provides additional options for customizing the appearance of the plot, such as lighting and color.
surface function is used to plot a surface defined by a matrix of data. It requires three input arguments: X, Y, and Z. X and Y define the coordinates of the data points, and Z is a matrix that defines the height of the surface at each point.
The size of Z must match the size of X and Y. The surface function also provides additional options for customizing the appearance of the plot, such as lighting and color.
In summary, both surf and surface functions are used for 3D surface plots but surf is used for a surface defined by a function of two variables or by a set of scattered data points, while surface is used for a surface defined by a matrix of data, and the size of Z must match the size of X and Y.
Example
f = figure();
data = peaks(50);
ax1 = subplot(1, 2, 1);
s1 = surface(ax1, data);
ax2 = subplot(1, 2, 2);
s2 = surf(ax2, data);
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
text
creates text descriptions to data points.
Syntax
- text(x, y, txt)
- text(x, y, z, txt)
- text(... , propertyName, propertyValue)
- text(ax, ...)
- go = text(...)
Input argument
- x - x-coordinates: vector or matrix.
- y - y-coordinates: vector or matrix.
- z - z-coordinates: vector or matrix.
- parent - a scalar graphics object value: parent container, specified as a axes.
- text - Text to display: character vector, string scalar, string array or cell array.
- propertyName - a scalar string or row vector character.
- propertyValue - a value.
Output argument
- go - a graphics object: text type.
Description
figure creates figure.
Properties:
BackgroundColor: Color of text box background: RGB triplet.
Children: Children: [].
Color: Text color: RGB triplet, [0 0 0] (default) or hexadecimal color code.
EdgeColor: Color of box outline: RGB triplet.
Extent: Size and location of rectangle that encloses text: four-element vector.
FontAngle: Character slant: 'italic' or 'normal' (default).
FontName: Font name:
FontSize: Font size: scalar value greater than zero.
FontUnits: Font size units: 'inches', 'centimeters', 'normalized', 'pixels' or 'points' (default).
FontWeight: Character thickness: 'bold' or 'normal' (default).
HorizontalAlignment: Horizontal alignment of text with respect to position point: 'center', 'right', 'left' (default).
Interpreter: 'tex' (default) interpreter or 'none'.
LineStyle: Line style of box outline: 'none', '--', ':', '-.' or '-' (default).
LineWidth: Width of box outline: scalar numeric value.
Margin: Space around text within the text box: scalar numeric value.
Parent: Parent: axes object.
Position: Location of text: two-element vector of form [x y] or three-element vector of form [x y z].
Rotation: Text orientation: scalar value in degrees.
String: Text to display: character vector, cell array of character vectors, string array, numeric value or '' (default).
Tag: Object identifier: character vector, string scalar or '' (default).
Type: Type of graphics object: 'text'.
Units: Position and extent units: 'normalized', 'inches', 'centimeters', 'characters', 'points', 'pixels' or 'data' (default).
UserData: User data: array or [] (default).
VerticalAlignment: Vertical alignment of text with respect to position point.
Visible: State of visibility: 'off' or 'on' (default).
CreateFcnCallback (function handle, string or cell) called when object is created. Set this property on an existing component has no effect.
DeleteFcnCallback (function handle, string or cell) called when object is deleted.
BeingDeleted Flag indicating that the object is being deleted.
Some properties are available only for compatibility and have currently no effect on the text.
lists of the supported special characters for the 'tex' interpreter:
Superscript: ^{ } 'text^{superscript}'
Subscript: _{ } 'text_{subscript}'
Character Sequence | Symbol |
---|---|
\alpha | α |
\upsilon | υ |
\sim | ~ |
\angle | ∠ |
\phi | ϕ |
\leq | ≤ |
\ast | * |
\chi | χ |
\infty | ∞ |
\beta | β |
\psi | ψ |
\clubsuit | ♣ |
\gamma | γ |
\omega | ω |
\diamondsuit | ♦ |
\delta | δ |
\Gamma | Γ |
\heartsuit | ♥ |
\epsilon | ϵ |
\Delta | Δ |
\spadesuit | ♠ |
\zeta | ζ |
\Theta | Θ |
\leftrightarrow | ↔ |
\eta | η |
\Lambda | Λ |
\leftarrow | ← |
\theta | θ |
\Xi | Ξ |
\Leftarrow | ⇐ |
\vartheta | ϑ |
\Pi | Π |
\uparrow | ↑ |
\iota | ι |
\Sigma | Σ |
\rightarrow | → |
\kappa | κ |
\Upsilon | ϒ |
\Rightarrow | ⇒ |
\lambda | λ |
\Phi | Φ |
\downarrow | ↓ |
\mu | µ |
\Psi | Ψ |
\circ | º |
\nu | ν |
\Omega | Ω |
\pm | ± |
\xi | ξ |
\forall | ∀ |
\geq | ≥ |
\pi | π |
\exists | ∃ |
\propto | ∝ |
\rho | ρ |
\ni | ∍ |
\partial | ∂ |
\sigma | σ |
\cong | ≅ |
\bullet | • |
\varsigma | ς |
\approx | ≈ |
\div | ÷ |
\tau | τ |
\Re | ℜ |
\neq | ≠ |
\equiv | ≡ |
\oplus | ⊕ |
\aleph | ℵ |
\Im | ℑ |
\cup | ∪ |
\wp | ℘ |
\otimes | ⊗ |
\subseteq | ⊆ |
\oslash | ∅ |
\cap | ∩ |
\in | ∈ |
\supseteq | ⊇ |
\supset | ⊃ |
\lceil | ⌈ |
\subset | ⊂ |
\int | ∫ |
\cdot | · |
\o | ο |
\rfloor | ⌋ |
\neg | ¬ |
\nabla | ∇ |
\lfloor | ⌊ |
\times | x |
\ldots | ... |
\perp | ⊥ |
\surd | √ |
\prime | ´ |
\wedge | ∧ |
\varpi | ϖ |
\0 | ∅ |
\rceil | ⌉ |
\rangle | 〉 |
\mid | | |
\vee | ∨ |
\langle | 〈 |
\copyright | © |
Examples
f = figure(1)
t = text(0.5, 0.5, 'text here');
s = t.FontSize;
t.FontSize = 12;
t.Color = 'red';
figure();
ha = {'left', 'center', 'right'};
va = {'bottom', 'middle', 'top'};
color = {'red', 'green', 'blue'};
x = [0.25 0.5 0.75];
y = x;
for t = 0:45:359;
for nh = 1:numel (ha)
for nv = 1:numel (va)
text (x(nh), y(nv), 'Nelson', ...
'rotation', t, ...
'horizontalalignment', ha{nh}, ...
'verticalalignment', va{nv}, ...
'color', color{nv});
end
end
end
axis([0 1 0 1]);
title (_('Text alignment and rotation (0:45:360 degrees)'));
xlabel(_('Horizontal alignment'));
ylabel (_('Vertical alignment'));
figure();
h1 = text(0.5, 0.5, 'Nelson \copyright')
h1.String
% Nelson is full unicode, so
h2 = text(0.5, 0.3, 'OR Nelson ©')
h2.String
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
1.7.0 | CreateFcn, DeleteFcn callback added. |
-- | BeingDeleted property added. |
Author
Allan CORNET
title
Add title.
Syntax
- title(text)
- title(ax, text)
- title(..., propertyName, propertyValue)
- go = title(...)
Input argument
- text - Text to display: character vector, string scalar, string array or cell array.
- ax - a scalar graphics object value: parent container, specified as a axes.
- propertyName - a scalar string or row vector character.
- propertyValue - a value.
Output argument
- go - a graphics object: text type.
Description
title('text') adds the title to the current axes.
Visible property is inherited from the parent if not explicitly defined.
Example
f = figure();
x = linspace(-1, 1);
y = sin(2*pi*x);
plot(x, y);
title('Unicode ドラゴンボールZ(ゼット)', 14);
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
1.10.0 | Visible property is inherited from the parent if not explicitly defined. |
Author
Allan CORNET
turbo
Turbo colormap array.
Syntax
- c = turbo
- c = turbo(m)
Input argument
- m - a scalar integer value: Number of colors (256 as default value).
Output argument
- c - Turbo colormap array.
Description
turbo returns the colormap with turbo colors.
Example
f = figure();
surf(peaks);
colormap('turbo');
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
uicontrol
Create user interface component.
Syntax
- c = uicontrol()
- c = uicontrol(propertyName, propertyValue)
- c = uicontrol(parent)
- c = uicontrol(parent, propertyName, propertyValue, ...)
- uicontrol(c)
Input argument
- parent - figure graphics object.
- propertyName - property name: a scalar string or row vector character.
- propertyValue - property value: a value compatible with property name.
- c - an User Interface control object.
Output argument
- c - an User Interface control object.
Description
c = uicontrol creates a push button, which is the default user interface control, within the current figure and returns the associated uicontrol object. If no figure is currently open, Nelson generates one using the figure function.
c = uicontrol(propertyName, propertyValue) creates a user interface control with properties defined by one or more name-value pair arguments. For instance, specifying 'Style', 'button' will create a button.
c = uicontrol(parent) creates the default user interface control (push button) within the specified parent container, rather than defaulting to the current figure.
c = uicontrol(parent, propertyName, propertyValue) creates a user interface control within the specified parent container, allowing you to define its properties using one or more name-value pair arguments.
uicontrol(c) sets the focus to a previously defined user interface control, bringing it to the forefront for user interaction.
List of properties:
BackgroundColor: Background color, specified as an RGB triplet, a hexadecimal color code, or a valid color name.
BeingDeleted: Deletion status. on/off logical value.
BusyAction: Callback queuing specified as 'queue' (default) or 'cancel'. The property determines how Nelso handles the execution of interrupting callbacks.
ButtonDownFcn: Button-press callback function
CData:An optional icon can be specified as a 3-D array of truecolor RGB values. The array values can be either: Double-precision numbers ranging from 0.0 to 1.0, or uint8 numbers ranging from 0 to 255
Callback: Primary callback function: '' (default), function handle, cell array or character vector.
Children: UIControl children: empty array.
CreateFcn: Component creation function.
DeleteFcn: Component deletion function.
Enable: Operational state of user interface control.
FontAngle: Font angle: 'italic' or 'normal' (default).
FontName: Font name: system supported font name.
FontSize: Font size: positive number.
FontUnits: Font units: 'normalized', 'inches', 'centimeters', 'pixels' or 'points' (default).
FontWeight: Font weight: 'bold' or 'normal' (default).
ForeGround: Text color, specified as an RGB triplet, a hexadecimal color code, or valid color name.
HandleVisibility: Visibility of uiControl handle.
HorizontalAlignment: Alignment of uicontrol text 'left', 'right' or 'center' (default).
Interruptible: Callback interruption 'on' (default).
KeyPressFcn: Key press callback function.
KeyReleaseFcn: Key release callback function
ListboxTop: Index of top item in list box: integer value or 1 (default).
Max: Maximum value: number or 1 (default).
Min: Minimum value: number or 0 (default).
Parent: Parent object: Figure.
Position: Location and size: [left bottom width height].
SliderStep: Slider step size: [minorstep majorstep] or [0.01 0.10] (default).
String: Text to display: character vector, cell array of character vectors or string array.
Style: 'pushbutton' (default), 'togglebutton', 'checkbox', 'radiobutton', 'edit', 'text', 'slider'.
Tooltip: Tooltip: character vector or string scalar.
Type: 'uicontrol'
UserData: User data array or [] (default).
Value: Current value: number
Visible: State of visibility: 'on' (default).
Examples
Pushbutton
f = figure;
b = uicontrol(f,'Style','pushbutton', 'String', 'Click Me', 'Position', [100 100 60 30], 'Callback', 'disp(''Hello World!'')')
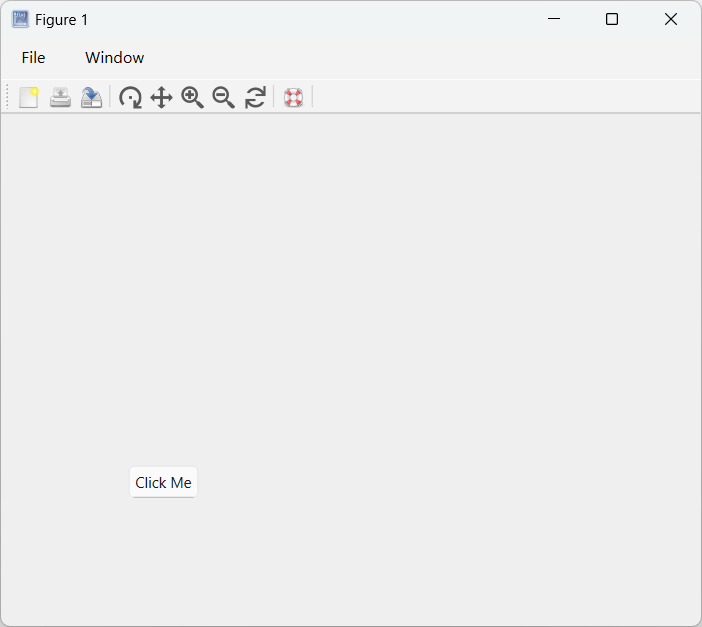
Checkbox
f = figure();
h = uicontrol('Style', 'checkbox', 'String', 'Click Me!', 'Position', [100, 100, 100, 50]);
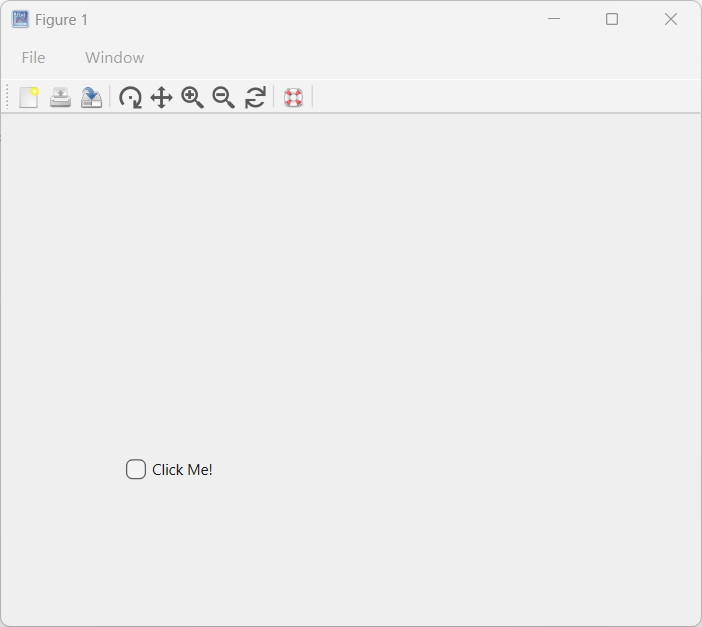
Edit
f = figure();
h = uicontrol('Style', 'edit', 'String', 'Click Me!', 'Position', [100, 100, 100, 50]);
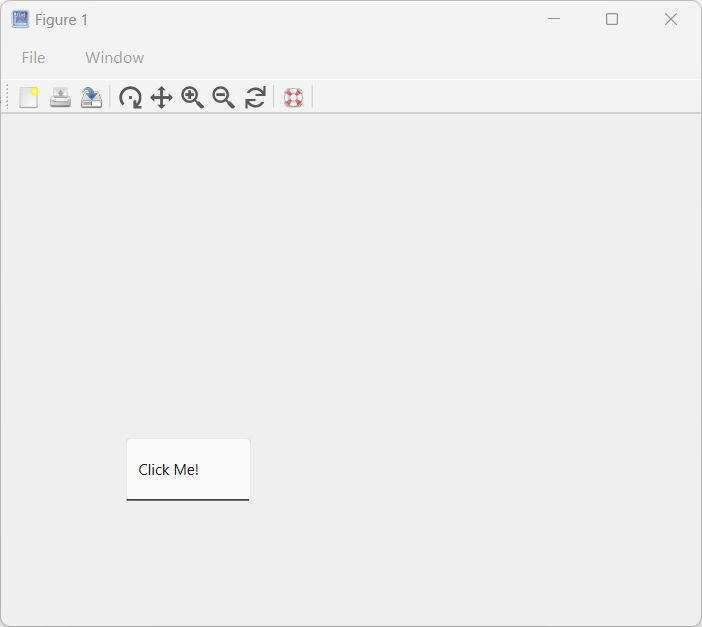
Image
hFig = figure('Position', [100, 100, 300, 300]);
imgSize = 50; % Size of the image
[X, Y] = meshgrid(1:imgSize, 1:imgSize);
CData = cat(3, X/imgSize, Y/imgSize, zeros(imgSize));
CData = im2double(CData); % Ensure the image is of type double
hButton = uicontrol('Style', 'pushbutton', 'Position', [100, 100, 100, 100], 'CData', CData, 'String', 'Click Me!');
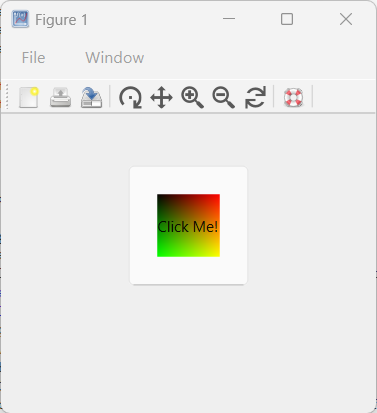
uicontrol demo
addpath([modulepath('graphics','root'), '/examples/uicontrol'])
edit uicontrol_demo
uicontrol_demo
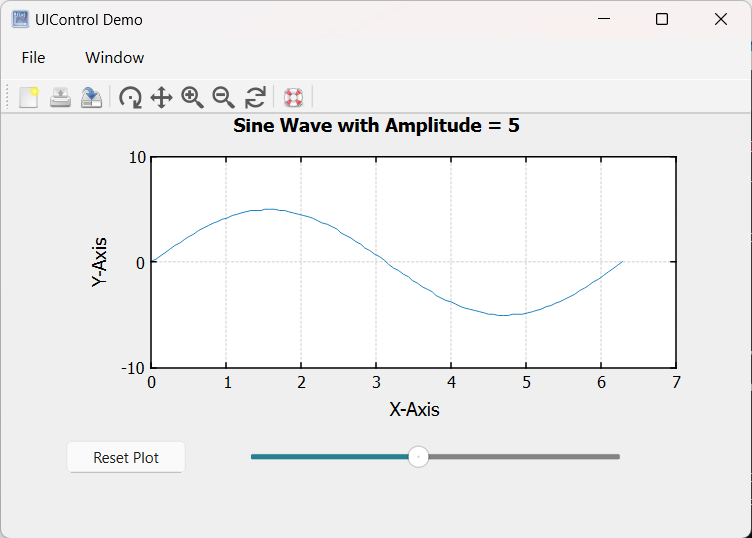
uicontrol demo Interruptible
addpath([modulepath('graphics','root'), '/examples/uicontrol'])
edit uicontrol_demo_interruptible
uicontrol_demo_interruptible
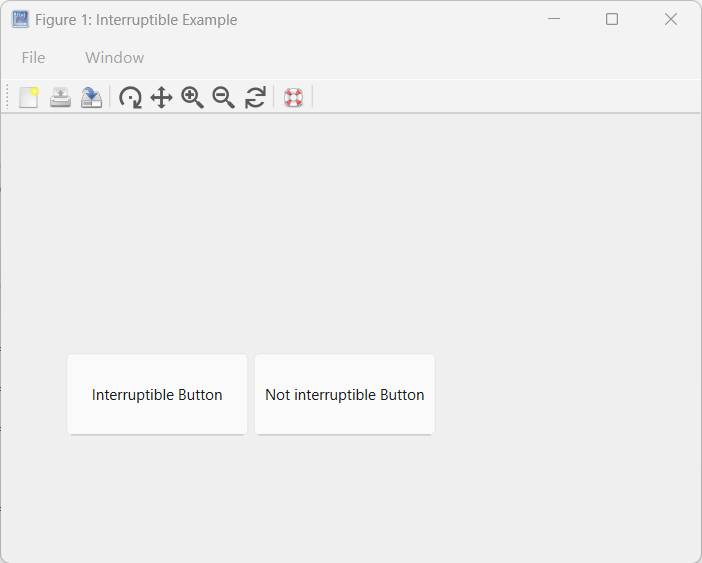
See also
figure, Managing Callback Interruptions in Nelson.
History
Version | Description |
---|---|
1.7.0 | initial version |
Author
Allan CORNET
validatecolor
Validate color values.
Syntax
- RGB = validatecolor(colors)
- RGB = validatecolor(colors, sz)
Input argument
- colors - 1-by-3 vector, m-by-3 matrix, character vector, cell array of character vectors or string array.
- sz - 'one' (default) or 'multiple'
Output argument
- RGB - RGB values: RGB triplet or matrix of RGB triplets.
Description
The validatecolor function is a color validation function that checks whether a given color is valid according to Nelson standards.
It takes a color argument as input and returns an error if the color is not valid.
Example
RGB = validatecolor('red')
RGB = validatecolor('purple')
RGB = validatecolor({'#8000FF','#00FF00','#FF9900'}, 'multiple')
RGB = validatecolor({'red','green','blue'},'multiple')
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
view
Camera line of sigh.
Syntax
- view(az, el)
- view([az, el])
- view([x, y, z])
- view(dim)
- view(ax, ...)
- [az, el] = view(...)
Input argument
- dim - Dimensions: 2 equivalent to view(0, 90) or 3 equivalent to view(-37.5, 30).
- az - Azimuth: scalar
- el - Elevation: scalar
- ax - a scalar graphics object value: parent container, specified as a axes.
Description
viewsets the view into a plot.
Examples
f = figure();
[X,Y] = meshgrid(-6:.5:6);
Z = Y .* sin(X) - X .* cos(Y);
surf(X, Y, Z)
f = figure();
[X,Y] = meshgrid(-6:.5:6);
Z = Y .* sin(X) - X .* cos(Y);
surf(X, Y, Z)
view(90, 0)
f = figure();
[X,Y] = meshgrid(-6:.5:6);
Z = Y .* sin(X) - X .* cos(Y);
surf(X, Y, Z)
view(2)
See also
axes.
History
Version | Description |
---|---|
1.0.0 | initial version |
1.2.0 | azimuth and elevation as output arguments. |
Author
Allan CORNET
viridis
Viridis colormap array.
Syntax
- c = viridis
- c = viridis(m)
Input argument
- m - a scalar integer value: Number of colors (256 as default value).
Output argument
- c - Viridis colormap array.
Description
viridis returns the colormap with viridis colors.
Bibliography
Color map created by Stéfan van der Walt and Nathaniel Smith
Example
f = figure();
surf(peaks);
view(2);
colormap('viridis');
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
waitfor
Wait for condition.
Syntax
- waitfor(obj)
- waitfor(obj, propertyName)
- waitfor(obj, propertyName, propertyValue)
Input argument
- obj - any scalar graphics object value.
- propertyName - property name: character vector or string scalar.
- propertyValue - property value: valid property value associated with propertyName.
Description
waitfor(obj) pauses the execution of statements until the specified object is closed (or deleted). Once the object is no longer present, waitfor returns, allowing the execution to continue. If the object does not exist at the time of the call, waitfor returns immediately.
waitfor(obj, propertyName) halts execution until the specified property of the object changes or the object is closed. For example, waitfor(hFig, 'UserData') pauses execution until the 'UserData' property of hFig changes. If the specified property name is invalid, an error stops execution.
waitfor(obj, propertyName, propertyValue) pauses execution until the specified property of the object changes to the given value. If the property is already equal to propvalue when waitfor is called, it returns immediately, allowing execution to resume.
Examples
h = figure()
waitfor(h);
% close figure to continue
hFig = figure('Position', [300, 300, 300, 150]);
hButton = uicontrol('Style', 'togglebutton', 'String', 'Toggle Me', 'Position', [100, 50, 100, 40], 'Value', 0);
hButton.Callback = @(src, event) set(src, 'Value', 1);
waitfor(hButton, 'Value');
% press toggle button
hFig = figure('Position', [300, 300, 300, 150]);
hButton = uicontrol('Style', 'togglebutton', 'String', 'Toggle Me', 'Position', [100, 50, 100, 40], 'Value', 0);
hButton.Callback = @(src, event) set(src, 'Value', 1);
waitfor(hButton, 'Value', 1);
% press toggle button
See also
figure, waitforbuttonpress, pause.
History
Version | Description |
---|---|
1.7.0 | initial version |
Author
Allan CORNET
waitforbuttonpress
Wait for click or key press.
Syntax
- w = waitforbuttonpress()
Output argument
- w - a scalar double value: 0 for mouse button pressed, 1 for key pressed.
Description
w = waitforbuttonpress() pauses the execution of code until the user interacts with the current figure by either clicking a mouse button or pressing a key.
Example
cf = gcf();
w = waitforbuttonpress;
axes;
See also
History
Version | Description |
---|---|
1.7.0 | initial version |
Author
Allan CORNET
waterfall
waterfall plot.
Syntax
- waterfall(X, Y, Z)
- waterfall(Z)
- waterfall(Z, C)
- waterfall(X, Y, Z, C)
- waterfall(parent, ...)
- waterfall(..., propertyName, propertyValue)
- go = waterfall(...)
Input argument
- X - x-coordinates: vector or matrix.
- Y - y-coordinates: vector or matrix.
- Z - z-coordinates: vector or matrix.
- C - Color array: m-by-n-by-3 array of RGB triplets.
- parent - a scalar graphics object value: parent container, specified as a axes.
- propertyName - a scalar string or row vector character.
- propertyValue - a value.
Output argument
- go - a graphics object: surface type.
Description
waterfall creates a waterfall plot, which is a mesh plot with a partial curtain along the y dimension.
This results in a 'waterfall' effect.
The function takes the same input arguments as the mesh function.
Examples
f = figure();
Z = peaks();
waterfall(Z);
title ("waterfall function");
f = figure();
[X,Y] = meshgrid(-5:.5:5);
Z = Y.*sin(X) - X.*cos(Y);
p = waterfall(X, Y, Z);
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
white
white colormap array.
Syntax
- c = white
- c = white(m)
Input argument
- m - a scalar integer value: Number of colors (256 as default value).
Output argument
- c - White colormap array.
Description
white returns the colormap with white colors.
Example
f = figure();
surf(peaks);
view(2);
colormap('white');
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
winter
Winter colormap array.
Syntax
- c = winter
- c = winter(m)
Input argument
- m - a scalar integer value: Number of colors (256 as default value).
Output argument
- c - Winter colormap array.
Description
winter returns the colormap with winter colors.
Example
f = figure();
surf(peaks);
colormap('winter');
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
xlabel
Label x-axis.
Syntax
- xlabel(text)
- xlabel(ax, text)
- xlabel(..., propertyName, propertyValue)
- go = xlabel(...)
Input argument
- text - Text to display: character vector, string scalar, string array or cell array.
- ax - a scalar graphics object value: parent container, specified as a axes.
- propertyName - a scalar string or row vector character.
- propertyValue - a value.
Output argument
- go - a graphics object: text type.
Description
xlabel('text') labels the x-axis of the current axes.
Example
f = figure();
x = linspace(-1, 1);
y = sin(2*pi*x);
plot(x, y);
xlabel('X axis Label - Unicode ドラゴンボールX(ゼット)')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
xlim
set or get x-axis limits.
Syntax
- lims = xlim()
- xlim([xmin, xmax])
- xlim('auto')
- xlim('manual')
- m = xlim('mode')
- xlim(ax, ...)
Input argument
- [xmin, xmax] - x-coordinates: vector or matrix.
- 'auto' - enable automatic limit selection.
- 'manual' - freeze the x-axis limits at their current value.
- 'mode' - returns the current x-axis limits mode.
- ax - a scalar graphics object value: parent container, specified as a axes.
Output argument
- lims - two-element vector: [xmin, xmax]
- m - 'auto' or 'manual'.
Description
xlim get or set the limits of the x-axis for the current plot.
Example
x = linspace(-1, 1);
y = sin(2*pi*x);
plot(x, y);
lim = xlim()
m = xlim('mode')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
ylabel
Label y-axis.
Syntax
- ylabel(text)
- ylabel(ax, text)
- ylabel(..., propertyName, propertyValue)
- go = ylabel(...)
Input argument
- text - Text to display: character vector, string scalar, string array or cell array.
- ax - a scalar graphics object value: parent container, specified as a axes.
- propertyName - a scalar string or row vector character.
- propertyValue - a value.
Output argument
- go - a graphics object: text type.
Description
ylabel('text') labels the y-axis of the current axes.
Example
f = figure();
x = linspace(-1, 1);
y = sin(2*pi*x);
plot(x, y);
ylabel('Y axis Label - Unicode ドラゴンボールY(ゼット)')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
ylim
set or get y-axis limits.
Syntax
- lims = ylim()
- ylim([ymin, ymax])
- ylim('auto')
- ylim('manual')
- m = ylim('mode')
- ylim(ax, ...)
Input argument
- [ymin, ymax] - y-coordinates: vector or matrix.
- 'auto' - enable automatic limit selection.
- 'manual' - freeze the y-axis limits at their current value.
- 'mode' - returns the current y-axis limits mode.
- ax - a scalar graphics object value: parent container, specified as a axes.
Output argument
- lims - two-element vector: [ymin, ymax]
- m - 'auto' or 'manual'.
Description
ylim get or set the limits of the y-axis for the current plot.
Example
x = linspace(-1, 1);
y = sin(2*pi*x);
plot(x, y);
lim = ylim()
m = ylim('mode')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
zlabel
Label z-axis.
Syntax
- zlabel(text)
- zlabel(ax, text)
- zlabel(..., propertyName, propertyValue)
- go = zlabel(...)
Input argument
- text - Text to display: character vector, string scalar, string array or cell array.
- ax - a scalar graphics object value: parent container, specified as a axes.
- propertyName - a scalar string or row vector character.
- propertyValue - a value.
Output argument
- go - a graphics object: text type.
Description
zlabel('text') labels the z-axis of the current axes.
Example
f = figure();
t = 0:pi/50:10*pi;
L = plot3(sin(t), cos(t), t);
axis square
zlabel('Z axis Label - Unicode ドラゴンボールZ(ゼット)')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
zlim
set or get z-axis limits.
Syntax
- lims = zlim()
- zlim([zmin, zmax])
- zlim('auto')
- zlim('manual')
- m = zlim('mode')
- zlim(ax, ...)
Input argument
- [zmin, zmax] - z-coordinates: vector or matrix.
- 'auto' - enable automatic limit selection.
- 'manual' - freeze the z-axis limits at their current value.
- 'mode' - returns the current z-axis limits mode.
- ax - a scalar graphics object value: parent container, specified as a axes.
Output argument
- lims - two-element vector: [zmin, zmax]
- m - 'auto' or 'manual'.
Description
zlim get or set the limits of the z-axis for the current plot.
Example
x = linspace(-1, 1);
y = sin(2*pi*x);
plot(x, y);
lim = zlim()
m = zlim('mode')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
zoom
Enable zoom mode.
Syntax
- zoom
- zoom option
- zoom(factor)
- zoom(fig, ...)
- zoom(ax, ...)
Input argument
- option - string: 'on', 'off', 'reset', 'out', 'xon', 'yon' or 'toggle'.
- factor - positive number: To zoom in, indicate a factor greater than 1. To zoom out, specify a factor between 0 and 1. When zooming out, the axes will zoom out by a factor of 1/factor.
- fig - Figure object: Target figure
- ax - a scalar graphics object value: parent container, specified as a axes.
Description
Utilize the zoom mode to dynamically adjust axis limits for interactive data exploration.
Enable or disable the zoom mode and configure additional basic settings using the zoom function.
Zoom mode is compatible with various charts like line, bar, histogram, and surface charts. These charts typically feature zoom in and zoom out icons on the toolbar to facilitate zooming functionality.
zoom option configures the zoom mode for all axes within the current figure.
For instance, zoom('on') activates zoom mode, zoom('xon') enables zoom mode exclusively for the x-dimension, while zoom('off') disables zoom mode altogether.
Once zoom mode is active, you can adjust the view of axes using the cursor, scroll wheel, or keyboard:
Cursor: Click to zoom in at the cursor position; Drag to zoom into a rectangular region.
Scroll wheel: Scroll up to zoom in, scroll down to zoom out.
Keyboard: Press the up arrow (↑) key to zoom in, and the down arrow (↓) key to zoom out.
The zoom mode option can be specified using one of the following values:
'toggle': Toggles the zoom mode. If zoom mode is disabled, 'toggle' reverts to the most recently used zoom option of 'on', 'xon', or 'yon'. This option behaves the same as calling zoom without any arguments.
'xon': Enables zoom mode for the x-dimension exclusively.
'yon': Activates zoom mode for the y-dimension exclusively.
'on': Activates zoom mode.
'off': Deactivates zoom mode. Note that certain default interactions may persist regardless of the interaction mode.
'reset': Establishes the current zoom level as the base zoom level. Once set, subsequent actions like zooming out, double-clicking within the axes, or clicking the Restore View icon on the axes toolbar will revert the axes to this baseline zoom level.
'out': Restores the current axes to its baseline zoom level.
Example
surf(peaks)
zoom on
zoom reset
zoom(1.5);
sleep(5);
zoom out
See also
History
Version | Description |
---|---|
1.2.0 | initial version |
Author
Allan CORNET
Help browser functions
Help browser functions
Description
help browser functions
- doc - Displays documentation.
- helpbrowser - internal function used by 'doc'.
doc
Displays documentation.
Syntax
- doc
- doc function_name
- doc('function_name')
Input argument
- function_name - a string: function name
Description
doclaunches help browser.
doc('function_name')displays the help about function designed by 'function_name'.
Examples
doc()
doc sin
doc is
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
helpbrowser
internal function used by 'doc'.
Description
helpbrowser function used by doc (not documented).
See also
doc.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
Text editor
Text editor
Description
Embedded Nelson text editor
- edit - function editor.
- editor - call the embedded text editor.
- smartindent - Smart indent a nelson file
edit
function editor.
Syntax
- edit()
- edit filename
- edit function_name
Input argument
- filename - a string: filename to open.
- function_name - a string: function name
Description
edit opens a new file called untitled.m in the nelson's editor.
If function_name is the name of a defined nelson function edit(function_name) try to open the associated file function_name.m .
edit(dirname) opens all .m available in dirname.
Example
edit('edit')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
1.5.0 | edit(dirname) added |
Author
Allan CORNET
editor
call the embedded text editor.
Syntax
- editor()
- editor(filename)
- editor('editor_command', cmd)
Input argument
- filename - a string: filename to open.
- cmd - a string representing the command to launch your preferred code editor.
Description
editor opens an existing file in the nelson's editor.
editor must be considered as internal and edit must be preferred.
Set another text editor as default: (example with VS code)
editor('editor_command', 'code')
To restore the default editor, use:
editor('editor_command', '')
Change text editor is persistent and will be saved in a configuration file.
Example
edit('edit')
if ispc()
editor('editor_command ', 'notepad')
else
editor('editor_command ', 'vim')
end
edit('edit')
% restore default editor
editor('editor_command ', '')
See also
edit.
History
Version | Description |
---|---|
1.0.0 | initial version |
1.10.0 | Option to change default text editor |
Author
Allan CORNET
smartindent
Smart indent a nelson file
Syntax
- smartindent(filename)
- smartindent(filename, indentsize)
- smartindent(filename, indentsize, dobackup)
Input argument
- filename - a string: filename to indent.
- indentsize - an integer value > 0. default: 2
- dobackup - a logical: false by default
Description
smartindent indents code to make reading statements such as while loops easier.
See also
edit.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
Tests manager
Tests manager
Description
Tests framework for Nelson
- test_makeref - Creates a '.ref' file for a test
- test_run - Runs tests
- skip_testsuite - Skip test suite on condition
test_makeref
Creates a '.ref' file for a test
Syntax
- status = test_makeref(filename)
Input argument
- filename - a string: filename, a test file.
Output argument
- status - a logical: true if .ref was generated.
Description
test_makeref function creates a '.ref' file from a test file.
test file must have <--CHECK REF--> tag.
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
test_run
Runs tests
Syntax
- status = test_run()
- status = test_run([])
- status = test_run('minimal_tests')
- status = test_run('-stoponfail')
- status = test_run(modules)
- status = test_run(file_to_test)
- status = test_run(modules, '-stoponfail')
- status = test_run(file_to_test, '-stoponfail')
- status = test_run(modules, option)
- status = test_run(file_to_test, option)
- status = test_run('minimal_tests', '-stoponfail')
- status = test_run('minimal_tests', option)
- status = test_run([], '-stoponfail')
- status = test_run([], option)
- status = test_run(modules, file_output)
- status = test_run(file_to_test, file_output)
- status = test_run([], file_output)
- status = test_run(modules, option, xunitfile)
- status = test_run(modules, '-stoponfail', xunitfile)
- status = test_run(modules, option, xunitfile, '-stoponfail')
Input argument
- module_name - a string or a cell of string: module name or list of modules.
- file_to_test - a string or a cell of string: file to test or list of filenames.
- options - a string or a cell of string: supported options 'all', 'all_tests', 'unitary_tests', 'nonreg_tests' or 'benchs'.
- xunitfile - a string: filename to export results as a .xml or .json file compatible with Xunit format.
- '-stoponfail' - a string: stop tests execution at first 'fails' detected.
Output argument
- status - a logical: true if tests pass.
Description
test_run function searchs 'test_*.m', 'bug_*.m', and 'bench_*.m' files, executes them, and displays a report about success or failures.
Each test or bench is executed in a separated process using the 'unix' command.
That enables the current command to continue, even if the test as created an unstable environment.
It also enables the tests to be independent from one another.
Some special tags can be inserted in the .m files to help the processing of the corresponding test.
These tags are expected to be found in Nelson comments:
<--NOT FIXED--> This test is skipped because it is a reported bug, but it is not yet fixed.
<--INTERACTIVE TEST--> This test is skipped because it is interactive test.
<--CLI MODE--> This test will be executed by nelson-cli executable (default).
<--ADV-CLI MODE--> This test will be executed by nelson-adv-cli executable.
<--GUI MODE--> This test will be executed by nelson-gui executable.
<--CHECK REF--> This test will compare .ref available in same directory with output generated. see test_makeref to generate .ref file.
<--ENGLISH IMPOSED--> This test will be executed with the en_US language.
<--WINDOWS ONLY--> This test will be executed only on Windows.
<--MACOS ONLY--> This test will be executed only on Macos.
<--UNIX ONLY--> This test will be executed only on Unix.
<--WITH DISPLAY--> This test will be executed only if a display output is available.
<--RELEASE ONLY--> This test will be executed only if nelson is an release (not in debug mode).
<--EXCEL REQUIRED--> This test will be executed only if excel is detected (on Windows).
<--MPI MODE--> This test will be executed in MPI mode.
<--AUDIO INPUT REQUIRED--> This test will be executed if an audio input is available.
<--AUDIO OUTPUT REQUIRED--> This test will be executed if an audio output is available.
<--C/C++ COMPILER REQUIRED--> This test will be executed if an C/C++ compiler is available.
<--INDEX 64 BIT REQUIRED--> This test will be executed if 64 bit index is available.
<--NO USER MODULES--> This test will be executed without load user modules.
<--IPC REQUIRED--> This test will be executed if IPC is available.
<--SEQUENTIAL TEST REQUIRED--> This test will be executed sequentialy (1 worker).
<--NATIVE ARCHITECTURE TEST REQUIRED--> This test will be executed if application's build and architecture are same.
<--FILE WATCHER REQUIRED--> This test will be executed if file watcher is available.
<--PYTHON ENVIRONMENT REQUIRED--> This test will be executed if file watcher is available.
Test can also skipped dynamically using skip_testsuite function.
To avoid to block the application, tests have an execution timer of 2 minutes and the benchs have a timer of 6 minutes.
test_run uses n th workers to execute and speed up tests executions.
Tests with <--SEQUENTIAL TEST REQUIRED--> are evaluated last.
Benchs are evaluated sequentialy.
Examples
test_run('string');
test_run({'string', 'time'})
test_run({'string', 'time'}, 'all', [tempdir(), 'tests.xml'])
See also
assert, test_makeref, skip_testsuite.
History
Version | Description |
---|---|
1.0.0 | initial version |
1.3.0 | PYTHON ENVIRONMENT REQUIRED tag added |
1.4.0 | skip_testsuite function reference |
Author
Allan CORNET
skip_testsuite
Skip test suite on condition
Syntax
- skip_testsuite()
- skip_testsuite(reason)
- skip_testsuite(condition)
- skip_testsuite(condition, reason)
Input argument
- condition - logical: true (default) or false
- reason - a string: reason to skip test suite
Description
The skip_testsuite function allows you to skip a test suite based on a specified condition.
condition: A boolean expression that determines whether to skip the test suite. If condition evaluates to true, the test suite will be skipped.
reason: A string explaining the reason for skipping the test suite. This parameter is useful for providing context to other developers or yourself in case the test suite is skipped.
Example
skip_testsuite(true, 'Test skipped')
See also
History
Version | Description |
---|---|
1.4.0 | initial version |
Author
Allan CORNET
Validators
Validators
Description
validators functions
- mustBeA - Checks that input value comes from one of specified classes.
- mustBeColumn - Checks that value is a column vector or raise an error.
- mustBeFile - Checks that input path refers to file.
- mustBeFinite - Checks that value is finite or raise an error.
- mustBeFloat - Checks that value is floating-point or raise an error.
- mustBeFolder - Checks that input path refers to folder.
- mustBeGreaterThan - Checks that value is greater than another value or issue error.
- mustBeGreaterThanOrEqual - Checks that value is greater than or equal to another value or issue error.
- mustBeInRange - Checks that value is in the specified range.
- mustBeInteger - Checks that value is integer or raise an error.
- mustBeLessThan - Checks that value is less than another value or issue error.
- mustBeLessThanOrEqual - Checks that value is less than or equal to another value or issue error.
- mustBeLogical - Checks that value is logical or raise an error.
- mustBeLogicalScalar - Checks that value is logical scalar or raise an error.
- mustBeMatrix - Checks that value is a matrix or raise an error.
- mustBeMember - Checks that value is member of specified array or issue error.
- mustBeNegative - Checks that value is negative or raise an error.
- mustBeNonNan - Checks that value is not NaN.
- mustBeNonSparse - Checks that value is not sparse.
- mustBeNonZero - Checks that value is not zero.
- mustBeNonempty - Checks that value is nonempty or raise an error.
- mustBeNonmissing - Checks that value is not missing.
- mustBeNonnegative - Checks that value is nonnegative or raise an error.
- mustBeNonpositive - Checks that value is non positive or raise an error.
- mustBeNonzeroLengthText - Checks that value is text with nonzero length or raise an error.
- mustBeNumeric - Checks that value is numeric or raise an error.
- mustBeNumericOrLogical - Checks that input is numeric or logical.
- mustBePositive - Checks that value is positive or raise an error.
- mustBeReal - Checks that value is real.
- mustBeRow - Checks that value is a row vector or raise an error.
- mustBeScalarOrEmpty - Checks that value is scalar or empty or raise an error.
- mustBeSparse - Checks that value is a sparse matrix or raise an error.
- mustBeText - Checks that value is piece of text or raise an error.
- mustBeTextScalar - Checks that value is single piece of text or raise an error.
- mustBeValidVariableName - Checks that value is valid variable name or raise an error.
- mustBeVector - Checks that value is vector or raise an error.
mustBeA
Checks that input value comes from one of specified classes.
Syntax
- mustBeA(var, classNames)
- mustBeA(var, classNames, argPosition)
- C++: void mustBeA(const ArrayOfVector& args, const wstringVector &classNames, int argPosition)
Input argument
- var - a variable.
- classNames - a variable: name of data type or class.
- argPosition - a positive integer value: Position of input argument.
Description
mustBeA checks that input value comes from one of specified classes.
Example
mustBeA(1, 'double')
mustBeA([], ["double", "single"])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
mustBeColumn
Checks that value is a column vector or raise an error.
Syntax
- mustBeColumn(var)
- mustBeColumn(var, argPosition)
- C++: void mustBeColumn(const ArrayOfVector& args, int argPosition)
Input argument
- var - a variable: all supported types and classes that implement iscolumn method.
- argPosition - a positive integer value: Position of input argument.
Description
mustBeColumn checks that value is a column vector or raise an error.
Example
mustBeColumn(true)
mustBeColumn([])
mustBeColumn(ones(3, 2, 4))
See also
History
Version | Description |
---|---|
1.10.0 | initial version |
Author
Allan CORNET
mustBeFile
Checks that input path refers to file.
Syntax
- mustBeFile(var)
- mustBeFile(var, argPosition)
- C++: void mustBeFile(const ArrayOfVector& args, int argPosition)
Input argument
- var - a variable: a scalar string array or row vector characters array.
- argPosition - a positive integer value: Position of input argument.
Description
mustBeFile checks that input path refers to file or raise an error.
Example
mustBeFile(tempdir())
mustBeFile([nelsonroot(), '/etc/startup.m'])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
mustBeFinite
Checks that value is finite or raise an error.
Syntax
- mustBeFinite(var)
- mustBeFinite(var, argPosition)
- C++: void mustBeFinite(const ArrayOfVector& args, int argPosition)
Input argument
- var - a variable: all supported types and classes that implement isfinite methods.
- argPosition - a positive integer value: Position of input argument.
Description
mustBeFinite checks that value is finite or raise an error.
Empty values are ignored.
Example
mustBeFinite(1)
mustBeFinite(Inf)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
mustBeFloat
Checks that value is floating-point or raise an error.
Syntax
- mustBeFloat(var)
- mustBeFloat(var, argPosition)
- C++: void mustBeFloat(const ArrayOfVector& args, int argPosition)
Input argument
- var - a variable: all supported types and classes that implement isfloat method.
- argPosition - a positive integer value: Position of input argument.
Description
mustBeFloat checks that value is floating-point (single or double) or raise an error.
Example
mustBeFloat(true)
mustBeFloat([])
mustBeFloat(single([true false]))
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
mustBeFolder
Checks that input path refers to folder.
Syntax
- mustBeFolder(var)
- mustBeFolder(var, argPosition)
- C++: void mustBeFolder(const ArrayOfVector& args, int argPosition)
Input argument
- var - a variable: a scalar string array or row vector characters array.
- argPosition - a positive integer value: Position of input argument.
Description
mustBeFolder checks that input path refers to folder or raise an error.
Example
mustBeFolder(tempdir())
mustBeFolder('hello_nelson')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
mustBeGreaterThan
Checks that value is greater than another value or issue error.
Syntax
- mustBeGreaterThan(var, c)
- mustBeGreaterThan(var, c, argPosition)
- C++: void mustBeGreaterThan(const ArrayOfVector& args, const ArrayOf &c, int argPosition)
Input argument
- var - a variable: logical or numeric array.
- c - a variable: scalar numeric value.
- argPosition - a positive integer value: Position of input argument.
Description
mustBeGreaterThan checks that value is greater than another value or issue error.
Example
mustBeGreaterThan(1, 0)
mustBeGreaterThan([2 3 4],2)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
mustBeGreaterThanOrEqual
Checks that value is greater than or equal to another value or issue error.
Syntax
- mustBeGreaterThanOrEqual(var, c)
- mustBeGreaterThanOrEqual(var, c, argPosition)
- C++: void mustBeGreaterThanOrEqual(const ArrayOfVector& args, const ArrayOf &c, int argPosition)
Input argument
- var - a variable: logical or numeric array.
- c - a variable: scalar numeric value.
- argPosition - a positive integer value: Position of input argument.
Description
mustBeGreaterThanOrEqual checks that value is greater than or equal to another value or issue error.
Example
mustBeGreaterThanOrEqual(1, 0)
mustBeGreaterThanOrEqual([2 3 4],5)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
mustBeInRange
Checks that value is in the specified range.
Syntax
- mustBeInRange(value, lower, upper)
- mustBeInRange(value, lower, upper, argPosition)
- mustBeInRange(value, lower, upper, boundflag1)
- mustBeInRange(value, lower, upper, boundflag1, argPosition)
- mustBeInRange(value, lower, upper, boundflag1, boundflag2)
- mustBeInRange(value, lower, upper, boundflag1, boundflag2, argPosition)
- C++: void mustBeInRange(const ArrayOfVector& args, const ArrayOf& lower, const ArrayOf& upper, const std::wstring& boundflag1, const std::wstring& boundflag2, int argPosition)
Input argument
- value - a numeric value: scalar or matrix
- lower - a scalar numeric or logical value.
- upper - a scalar numeric or logical value.
- boundflag1 - 'inclusive', 'exclusice', 'exclude-lower' or 'exclude-upper'.
- boundflag2 - 'inclusive', 'exclusice', 'exclude-lower' or 'exclude-upper'.
- argPosition - a positive integer value: Position of input argument.
Description
mustBeInRange checks that value is in the specified range or raise an error.
The only valid combination of the flags is exclude-lower with exclude-upper.
Example
mustBeInRange(3, 2, 4)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
mustBeInteger
Checks that value is integer or raise an error.
Syntax
- mustBeInteger(var)
- mustBeInteger(var, argPosition)
- C++: void mustBeInteger(const ArrayOfVector& args, int argPosition)
Input argument
- var - a variable: all supported types and classes that implement isnumeric, islogical, all, isreal, eq and floor methods.
- argPosition - a positive integer value: Position of input argument.
Description
mustBeInteger checks that value is integer or raise an error.
Example
mustBeInteger(-1)
mustBeInteger(Inf)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
mustBeLessThan
Checks that value is less than another value or issue error.
Syntax
- mustBeLessThan(var, c)
- mustBeLessThan(var, c, argPosition)
- C++: void mustBeLessThan(const ArrayOfVector& args, const ArrayOf &c, int argPosition)
Input argument
- var - a variable: logical or numeric array.
- c - a variable: scalar numeric value.
- argPosition - a positive integer value: Position of input argument.
Description
mustBeLessThan checks that value is less than another value or issue error.
Example
mustBeLessThan(1, 0)
mustBeLessThan(1, 2)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
mustBeLessThanOrEqual
Checks that value is less than or equal to another value or issue error.
Syntax
- mustBeLessThanOrEqual(var, c)
- mustBeLessThanOrEqual(var, c, argPosition)
- C++: void mustBeLessThanOrEqual(const ArrayOfVector& args, const ArrayOf &c, int argPosition)
Input argument
- var - a variable: logical or numeric array.
- c - a variable: scalar numeric value.
- argPosition - a positive integer value: Position of input argument.
Description
mustBeLessThanOrEqual checks that value is less than or equal to another value or issue error.
Example
mustBeLessThanOrEqual(1, 0)
mustBeLessThanOrEqual([2 3 4],2)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
mustBeLogical
Checks that value is logical or raise an error.
Syntax
- mustBeLogical(var)
- mustBeLogical(var, argPosition)
- C++: void mustBeLogical(const ArrayOfVector& args, int argPosition)
Input argument
- var - a variable: all supported types and classes that implement islogical and isempty methods.
- argPosition - a positive integer value: Position of input argument.
Description
mustBeLogical checks that value is logical or raise an error.
Empty values are ignored.
Example
mustBeLogical(true)
mustBeLogical([])
mustBeLogical([true false])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
mustBeLogicalScalar
Checks that value is logical scalar or raise an error.
Syntax
- mustBeLogicalScalar(var)
- mustBeLogicalScalar(var, argPosition)
- C++: void mustBeLogicalScalar(const ArrayOfVector& args, int argPosition)
Input argument
- var - a variable: all supported types and classes that implement islogical, isscalar methods.
- argPosition - a positive integer value: Position of input argument.
Description
mustBeLogicalScalar checks that value is logical scalar or raise an error.
Example
mustBeLogicalScalar(true)
mustBeLogicalScalar([])
mustBeLogicalScalar([true false])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
mustBeMatrix
Checks that value is a matrix or raise an error.
Syntax
- mustBeMatrix(var)
- mustBeMatrix(var, argPosition)
- C++: void mustBeMatrix(const ArrayOfVector& args, int argPosition)
Input argument
- var - a variable: all supported types and classes that implement ismatrix method.
- argPosition - a positive integer value: Position of input argument.
Description
mustBeMatrix checks that value is a matrix or raise an error.
Example
mustBeMatrix(true)
mustBeMatrix([])
mustBeMatrix(ones(3, 2, 4))
See also
History
Version | Description |
---|---|
1.10.0 | initial version |
Author
Allan CORNET
mustBeMember
Checks that value is member of specified array or issue error.
Syntax
- mustBeMember(var, c)
- mustBeMember(var, c, argPosition)
- C++: void mustBeMember(const ArrayOfVector& args, const ArrayOf &c, int argPosition)
Input argument
- var - a variable.
- c - a variable.
- argPosition - a positive integer value: Position of input argument.
Description
mustBeMember checks that value is member of an array or issue error.
Example
A = "red";
B = ["yellow","red","blue"];
mustBeMember(A,B)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
mustBeNegative
Checks that value is negative or raise an error.
Syntax
- mustBeNegative(var)
- mustBeNegative(var, argPosition)
- C++: void mustBeNegative(const ArrayOfVector& args, int argPosition)
Input argument
- var - a variable: all supported types and classes that implement isnumeric, islogical, all, isreal, and lt methods.
- argPosition - a positive integer value: Position of input argument.
Description
mustBeNegative checks that value is negative or raise an error.
Example
mustBeNegative(-1)
mustBeNegative(1)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
mustBeNonNan
Checks that value is not NaN.
Syntax
- mustBeNonNan(var)
- mustBeNonNan(var, argPosition)
- C++: void mustBeNonNan(const ArrayOfVector& args, int argPosition)
Input argument
- var - a variable: all supported types and classes that implement isnan methods.
- argPosition - a positive integer value: Position of input argument.
Description
mustBeNonNan checks that value is not NaN or raise an error.
Example
mustBeNonNan(1)
mustBeNonNan([])
mustBeNonNan(NaN)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
mustBeNonSparse
Checks that value is not sparse.
Syntax
- mustBeNonSparse(var)
- mustBeNonSparse(var, argPosition)
- C++: void mustBeNonSparse(const ArrayOfVector& args, int argPosition)
Input argument
- var - a variable: all supported types and classes that implement issparse method.
- argPosition - a positive integer value: Position of input argument.
Description
mustBeNonSparse checks that value is not sparse or raise an error.
Example
mustBeNonSparse(1)
mustBeNonSparse([])
mustBeNonSparse(sparse(3))
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
mustBeNonZero
Checks that value is not zero.
Syntax
- mustBeNonZero(var)
- mustBeNonZero(var, argPosition)
- C++: void mustBeNonZero(const ArrayOfVector& args, int argPosition)
Input argument
- var - a variable: all supported types and classes that implement eq, isnumeric and islogical methods.
- argPosition - a positive integer value: Position of input argument.
Description
mustBeNonZero checks that value is not zero or raise an error.
Example
mustBeNonZero(1)
mustBeNonZero([])
mustBeNonZero(NaN)
mustBeNonZero(0)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
mustBeNonempty
Checks that value is nonempty or raise an error.
Syntax
- mustBeNonempty(var)
- mustBeNonempty(var, argPosition)
- C++: void mustBeNonempty(const ArrayOfVector& args, int argPosition)
Input argument
- var - a variable: all supported types and classes that implement isempty methods.
- argPosition - a positive integer value: Position of input argument.
Description
mustBeNonempty checks that value is not empty or raise an error.
Example
mustBeNonempty(1)
mustBeNonempty([])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
mustBeNonmissing
Checks that value is not missing.
Syntax
- mustBeNonmissing(var)
- mustBeNonmissing(var, argPosition)
- C++: void mustBeNonmissing(const ArrayOfVector& args, int argPosition)
Input argument
- var - a variable: all supported types and classes that implement ismissing method.
- argPosition - a positive integer value: Position of input argument.
Description
mustBeNonmissing checks that value is not missing or raise an error.
Example
mustBeNonmissing(1)
mustBeNonmissing([])
mustBeNonmissing(["hello" string(NaN)])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
mustBeNonnegative
Checks that value is nonnegative or raise an error.
Syntax
- mustBeNonnegative(var)
- mustBeNonnegative(var, argPosition)
- C++: void mustBeNonnegative(const ArrayOfVector& args, int argPosition)
Input argument
- var - a variable: all supported types and classes that implement isnumeric, islogical, all, isreal, and ge methods.
- argPosition - a positive integer value: Position of input argument.
Description
mustBeNonnegative checks that value is nonnegative or raise an error.
Example
mustBeNonnegative(1)
mustBeNonnegative(-1)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
mustBeNonpositive
Checks that value is non positive or raise an error.
Syntax
- mustBeNonpositive(var)
- mustBeNonpositive(var, argPosition)
- C++: void mustBeNonpositive(const ArrayOfVector& args, int argPosition)
Input argument
- var - a variable: all supported types and classes that implement isnumeric, islogical, all, isreal, and le methods.
- argPosition - a positive integer value: Position of input argument.
Description
mustBeNonpositive checks that value is non positive or raise an error.
Example
mustBeNonpositive(-1)
mustBeNonpositive(1)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
mustBeNonzeroLengthText
Checks that value is text with nonzero length or raise an error.
Syntax
- mustBeNonzeroLengthText(var)
- mustBeNonzeroLengthText(var, argPosition)
- C++: void mustBeNonzeroLengthText(const ArrayOfVector& args, int argPosition)
Input argument
- var - a variable: a string array, a cell of strings, or row vector characters array.
- argPosition - a positive integer value: Position of input argument.
Description
mustBeNonzeroLengthText checks that value is text with nonzero length or raise an error.
Example
mustBeNonzeroLengthText('true')
mustBeNonzeroLengthText("hello")
mustBeNonzeroLengthText('')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
mustBeNumeric
Checks that value is numeric or raise an error.
Syntax
- mustBeNumeric(var)
- mustBeNumeric(var, argPosition)
- C++: void mustBeNumeric(const ArrayOfVector& args, int argPosition)
Input argument
- var - a variable: all supported types and classes that implement isnumeric method.
- argPosition - a positive integer value: Position of input argument.
Description
mustBeNumeric checks that value is numeric or raise an error.
Empty values are ignored.
Example
mustBeNumeric(1)
mustBeNumeric([])
mustBeNumeric({1})
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
mustBeNumericOrLogical
Checks that input is numeric or logical.
Syntax
- mustBeNumericOrLogical(var)
- mustBeNumericOrLogical(var, argPosition)
- C++: void mustBeNumericOrLogical(const ArrayOfVector& args, int argPosition)
Input argument
- var - a variable.
- argPosition - a positive integer value: Position of input argument.
Description
mustBeNumericOrLogical checks that value is numeric or logical otherwise raise an error.
Example
mustBeNumericOrLogical(1)
mustBeNumericOrLogical([])
mustBeNumericOrLogical({1})
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
mustBePositive
Checks that value is positive or raise an error.
Syntax
- mustBePositive(var)
- mustBePositive(var, argPosition)
- C++: void mustBePositive(const ArrayOfVector& args, int argPosition)
Input argument
- var - a variable: all supported types and classes that implement isnumeric, islogical, all, isreal, and gt methods.
- argPosition - a positive integer value: Position of input argument.
Description
mustBePositive checks that value is positive or raise an error.
Example
mustBePositive(1)
mustBePositive(-1)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
mustBeReal
Checks that value is real.
Syntax
- mustBeReal(var)
- mustBeReal(var, argPosition)
- C++: void mustBeReal(const ArrayOfVector& args, int argPosition)
Input argument
- var - a variable: all supported types and classes that implement isreal method.
- argPosition - a positive integer value: Position of input argument.
Description
mustBeReal checks that value is real or raise an error.
Example
mustBeReal(1)
mustBeReal(i)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
mustBeRow
Checks that value is a row vector or raise an error.
Syntax
- mustBeRow(var)
- mustBeRow(var, argPosition)
- C++: void mustBeRow(const ArrayOfVector& args, int argPosition)
Input argument
- var - a variable: all supported types and classes that implement isrow method.
- argPosition - a positive integer value: Position of input argument.
Description
mustBeRow checks that value is a row vector or raise an error.
Example
mustBeRow([1, 1])
mustBeRow([])
mustBeRow([1; 1])
See also
History
Version | Description |
---|---|
1.10.0 | initial version |
Author
Allan CORNET
mustBeScalarOrEmpty
Checks that value is scalar or empty or raise an error.
Syntax
- mustBeScalarOrEmpty(var)
- mustBeScalarOrEmpty(var, argPosition)
- C++: void mustBeScalarOrEmpty(const ArrayOfVector& args, int argPosition)
Input argument
- var - a variable: all supported types and classes that implement isscalar and isempty methods.
- argPosition - a positive integer value: Position of input argument.
Description
mustBeScalarOrEmpty checks that value is scalar or empty or raise an error.
Example
mustBeScalarOrEmpty(true)
mustBeScalarOrEmpty([])
mustBeScalarOrEmpty([true false])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
mustBeSparse
Checks that value is a sparse matrix or raise an error.
Syntax
- mustBeSparse(var)
- mustBeSparse(var, argPosition)
- C++: void mustBeSparse(const ArrayOfVector& args, int argPosition)
Input argument
- var - a variable: all supported types and classes that implement issparse method.
- argPosition - a positive integer value: Position of input argument.
Description
mustBeSparse checks that value is a sparse matrix or raise an error.
Example
mustBeSparse(true)
mustBeSparse(eye(3, 4))
mustBeSparse(sparse(eye(3, 4)))
See also
History
Version | Description |
---|---|
1.11.0 | initial version |
Author
Allan CORNET
mustBeText
Checks that value is piece of text or raise an error.
Syntax
- mustBeText(var)
- mustBeText(var, argPosition)
- C++: void mustBeText(const ArrayOfVector& args, int argPosition)
Input argument
- var - a variable: a string array, a cell of strings, or row vector characters array.
- argPosition - a positive integer value: Position of input argument.
Description
mustBeText that value is piece of text or raise an error.
Example
mustBeText('true')
mustBeText(["f", "ff"])
mustBeText("hello")
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
mustBeTextScalar
Checks that value is single piece of text or raise an error.
Syntax
- mustBeTextScalar(var)
- mustBeTextScalar(var, argPosition)
- C++: void mustBeTextScalar(const ArrayOfVector& args, int argPosition)
Input argument
- var - a variable: a scalar string array or row vector characters array.
- argPosition - a positive integer value: Position of input argument.
Description
mustBeTextScalar that value is single piece of text or raise an error.
Example
mustBeTextScalar('true')
mustBeTextScalar(["f", "ff"])
mustBeTextScalar("hello")
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
mustBeValidVariableName
Checks that value is valid variable name or raise an error.
Syntax
- mustBeValidVariableName(var)
- mustBeValidVariableName(var, argPosition)
- C++: void mustBeValidVariableName(const ArrayOfVector& args, int argPosition)
Input argument
- var - a variable: string or characters array.
- argPosition - a positive integer value: Position of input argument.
Description
mustBeValidVariableName checks that value is valid variable name or raise an error.
Example
mustBeValidVariableName('8t')
mustBeValidVariableName('t8')
mustBeValidVariableName("t8")
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
mustBeVector
Checks that value is vector or raise an error.
Syntax
- mustBeVector(var)
- mustBeVector(var, 'allow-all-empties')
- mustBeVector(var, argPosition)
- mustBeVector(var, 'allow-all-empties', argPosition)
- C++: void mustBeVector(const ArrayOfVector& args, bool allowsAllEmpties, int argPosition)
Input argument
- var - a variable: all supported types and classes that implement isvector methods.
- argPosition - a positive integer value: Position of input argument.
Description
mustBeVector checks that value is vector or raise an error.
Example
mustBeVector(true)
mustBeVector([1 2])
mustBeVector([])
mustBeVector([], 'allows-all-empties')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
Assertion functions
Assertion functions
Description
The aim of these functions is to provide tools to test some other functions.
- assert - Check that condition is true.
- assert_checkerror - Check that an command raises the expected error.
- assert_isapprox - Check that computed and expected values are approximately equal.
- assert_isequal - Check that computed and expected values are equal.
- assert_isfalse - Check that condition is false.
- assert_istrue - Check that condition is true.
assert
Check that condition is true.
Syntax
- assert(x)
- r = assert(x)
- [r, msg] = assert(x)
- assert(x, err_msg)
- r = assert(x, err_msg)
- [r, msg] = assert(x, err_msg)
Input argument
- x - a logical value
- err_msg - a string, the error message to be printed in case of failure (optional).
Output argument
- res - a logical value
- msg - a string value, the error message. If x == true, then msg == ''. If x == false, then msg contains the error message.
Description
Raises an error if x is false. Raises an error if x is not a logical.
Example
assert(4 == 3, _('error for comparaison.'))
See also
assert_istrue, assert_isfalse.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
assert_checkerror
Check that an command raises the expected error.
Syntax
- assert_checkerror(command, expected_error_message)
- r = assert_checkerror(command, expected_error_message)
- [r, msg] = assert_checkerror(command, expected_error_message)
- assert_checkerror(command, expected_error_message, expected_error_identifier)
- r = assert_checkerror(command, expected_error_message, expected_error_identifier)
- [r, msg] = assert_checkerror(command, expected_error_message, expected_error_identifier)
Input argument
- command - a string value
- expected_error_message - a string, the expected error message.
- expected_error_identifier - a string, the expected error identifier.
Output argument
- res - a logical value
- msg - a string value, the error message. If res == true, then errormsg == ''. If res == false, then msg contains the error message.
Description
If the command does not raise the expected error message, then assert_checkerror raises an error.
Examples
assert_checkerror('cos', _('Wrong number of input arguments.'));
assert_checkerror('cos', _('Wrong error message.'));
assert_checkerror('mustBeFinite(NaN)', _('Value must be finite.'), 'Nelson:validators:mustBeFinite')
See also
assert_istrue, assert_isfalse.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
assert_isapprox
Check that computed and expected values are approximately equal.
Syntax
- assert_isapprox(computed, expected)
- assert_isapprox(computed, expected, precision)
- res = assert_isapprox(computed, expected, precision)
- [res, msg] = assert_isapprox(computed, expected, precision)
Input argument
- computed - a value: numeric matrix, sparse double, a multidimensional matrix
- expected - a value: numeric matrix, sparse double, a multidimensional matrix
- expected - a double value. default precision is 0.
Output argument
- res - a logical value
- msg - a string value, the error message. If res == true, then errormsg == ''. If res == false, then msg contains the error message.
Description
assert_isapprox raises an error if computed value is not approximately equal to expected value.
This function compares two floating point numbers, which allows to check that two numbers are "approximately" equal, i.e. that the relative error is small.
Used function(s)
isapprox
Examples
assert_isapprox(1.23456, 1.23457, 1e-5)
assert_isapprox(1.23456, 1.23457, 1e-6)
[r, msg] =assert_isapprox(1.23456, 1.23457, 1e-6)
assert_isfalse(r);
assert_isequal(msg, _('Assertion failed: expected and computed values are too different.'));
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
assert_isequal
Check that computed and expected values are equal.
Syntax
- assert_isequal(computed, expected)
- res = assert_isequal(computed, expected)
- [res, msg] = assert_isequal(computed, expected)
Input argument
- computed - a value
- expected - a value
Output argument
- res - a logical value
- msg - a string value, the error message. If res == true, then errormsg == ''. If res == false, then msg contains the error message.
Description
assert_isequal raises an error if computed value is not equal to expected value (same type, same dimensions, same values comparaisons).
Used function(s)
isequaln
Bibliography
"Automated Software Testing for Matlab", Steven Eddins, 2009
Examples
A = eye(3, 3);
assert_isequal(A, A)
A = eye(3, 3);
B = single(A);
assert_isequal(A, B)
A = NaN;
B = A;
assert_isequal(A, B)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
assert_isfalse
Check that condition is false.
Syntax
- assert_isfalse(x)
- r = assert_isfalse(x)
- [r, msg] = assert_isfalse(x)
- assert_isfalse(x, err_msg)
- r = assert_isfalse(x, err_msg)
- [r, msg] = assert_isfalse(x, err_msg)
Input argument
- x - a logical value
- err_msg - a string, the error message to be printed in case of failure (optional).
Output argument
- res - a logical value
- msg - a string value, the error message. If x == false, then msg == ''. If x == true, then msg contains the error message.
Description
Raises an error if x is true. Raises an error if x is not a logical.
Examples
assert_isfalse(3 ~= 4)
assert_isfalse(3 == 4)
r = assert_isfalse(false)
[r, msg] = assert_isfalse(false)
[r, msg] = assert_isfalse(3 == 3, 'your error message.')
See also
assert_istrue, assert_checkerror.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
assert_istrue
Check that condition is true.
Syntax
- assert_istrue(x)
- r = assert_istrue(x)
- [r, msg] = assert_istrue(x)
- assert_istrue(x, err_msg)
- r = assert_istrue(x, err_msg)
- [r, msg] = assert_istrue(x, err_msg)
Input argument
- x - a logical value
- err_msg - a string, the error message to be printed in case of failure (optional).
Output argument
- res - a logical value
- msg - a string value, the error message. If x == true, then msg == ''. If x == false, then msg contains the error message.
Description
Raises an error if x is false. Raises an error if x is not a logical.
Examples
assert_istrue(3 == 3)
assert_istrue(3 == 4)
r = assert_istrue(false)
[r, msg] = assert_istrue(false)
[r, msg] = assert_istrue(3 == 4, 'your error message.')
See also
assert_isfalse, assert_checkerror.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
Stream manager
Stream manager
Description
stream functions
- diary - Diary of a session.
- fclose - Close an opened file.
- feof - Checks end of file.
- ferror - Test for i/o read/write errors.
- fgetl - Read string from a file without newline.
- fgets - Read string from a file, stopping after a newline, or EOF, or n characters have been read.
- fileread - Read contents of file as text.
- filewrite - Write text to a file.
- fopen - Open a file in Nelson.
- fprintf - Writes data to a file.
- fread - Read data in binary form to the file specified by the file descriptor fid.
- frewind - Set position of stream to the beginning.
- fscanf - Reads data from a file.
- fseek - Set the file pointer to a location.
- fsize - Returns size of an opened file.
- ftell - Returns the offset of the current byte relative to the beginning of a file.
- fwrite - Write data in binary form to the file specified by the file descriptor fid.
- load - load data from .nh5 or .mat file into Nelson's workspace.
- save - save workspace variables to .nh5 or .mat file
- sscanf - Read formatted data from strings.
diary
Diary of a session.
Syntax
- diary()
- diary(filename)
- diary('off')
- diary('on')
- onoff = diary('get', 'Diary')
- filename = diary('get', 'DiaryFile')
- diary('set', 'DiaryFile', filename)
- diary('set', 'Diary', onoff)
Input argument
- onoff - a string: 'on' or 'off'.
- filename - a string: filename of the current diary.
Output argument
- onoff - a string: 'on' or 'off'.
- filename - a string: filename to use for the diary.
Description
diary creates a log of keyboard input and the resulting text output.
diary toggles diary mode on and off.
diary('off') stops recording the session in the diary file.
diary('on') starts recording a session in a file called 'diary' in the current working directory.
diary('set', 'Diary', onoff) allows to start or stop the diary.
onoff = diary('get', 'Diary') returns the state 'on' or 'off' of the diary.
diary(filename) records the session in the file named filename.
filename = diary('get', 'DiaryFile') returns filename used as diary.
diary('set', 'DiaryFile', filename)) set the filename for the diary.
Example
filename = diary('get', 'DiaryFile')
onoff = diary('get', 'Diary')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
fclose
Close an opened file.
Syntax
- fclose(fid)
- fclose('all')
- status = fclose(fid)
- status = fclose('all')
Input argument
- fid - a file descriptor
Output argument
- status - an integer value: 0 if file is closed or -1 if not.
Description
fclose must be used to close a file opened by fopen.
fclose('all') closes all opened file with fopen.
Example
fd = fopen([tempdir(), filesep(), 'fclose_tst'],'wt');
status = fclose(fd)
status = fclose(fd)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
feof
Checks end of file.
Syntax
- status = feof(fid)
Input argument
- fid - a file descriptor
Output argument
- status - an integer value: 1 if file is closed or 0 if not.
Description
feof checks if end of file has been reached.
Example
fid = fopen([nelsonroot(), '/etc/startup.m'], 'rt');
feof(fid)
while ~feof(fid)
tline = fgetl(fid);
disp(tline);
end
feof(fid)
fclose(fid);
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
ferror
Test for i/o read/write errors.
Syntax
- msg = ferror(fid)
- [msg, code] = ferror(fid)
- ferror(fid, 'clear')
Input argument
- fid - a file descriptor
Output argument
- code - an integer value: 0 if no error. negative value if an error is detected.
- msg - an character vector: error message equivalent to error code.
Description
ferror inquires about file error status.
ferror(fid, 'clear') clears the error indicator for the specified file.
For more help about returned message, consult C run-time library manual for further details.
Example
filename = [tempdir(), 'test_ferror.csv'];
fid = fopen(filename, 'w');
res = fgets(fid);
[msg, code] = ferror(fid)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
fgetl
Read string from a file without newline.
Syntax
- res = fgetl(f)
Input argument
- f - a file descriptor
Output argument
- res - a string or -1
Description
Read string from a file, stopping after a newline or EOF have been read.
If there is no more character to read, fgets will return -1.
newline character removed of the string returned
characters encoding uses fopen parameter.
Example
fid = fopen([nelsonroot(), '/etc/startup.m']);
tline = fgetl(fid);
while ischar(tline)
disp(tline)
tline = fgetl(fid);
end
fclose(fid);
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
fgets
Read string from a file, stopping after a newline, or EOF, or n characters have been read.
Syntax
- res = fgets(f)
- res = fgets(f, n)
Input argument
- f - a file descriptor
- n - a scalar: number of characters
Output argument
- res - a string or -1
Description
Read string from a file, stopping after a newline, or EOF, or n characters have been read.
If there is no more character to read, fgets will return -1.
If n is omitted, fgets reads until the next newline.
characters encoding uses fopen parameter.
Examples
fid = fopen([nelsonroot(), '/etc/startup.m']);
tline = fgets(fid);
while ischar(tline)
disp(tline)
tline = fgets(fid);
end
fclose(fid);
fid = fopen([nelsonroot(), '/etc/startup.m']);
tline = fgets(fid, 5);
while ischar(tline)
disp(tline)
tline = fgets(fid, 5);
end
fclose(fid);
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
fileread
Read contents of file as text.
Syntax
- str = fileread(filename)
- str = fileread(filename, type)
- str = fileread(filename, 'char', eol)
- str = fileread(filename, 'char', eol, encoding)
Input argument
- filename - a string: a file name
- type - a string: 'char', 'cell' or 'string'. 'cell' will converts text file to a cell of string. 'string' will converts text file to a string array. 'char' by default.
- eol - a string: 'native', 'pc' or 'unix'. Set end of line. 'unix' by default.
- encoding - a string: 'UTF-8' (default), 'auto', 'ISO-8859-1', 'windows-1251', 'windows-1252', ...
Output argument
- str - a string, cell of strings or string array.
Description
fileread read contents of file as text.
if encoding is 'auto', nelson will try to detect best encoding to read contents of file as text.
Examples
str = fileread([nelsonroot(),'/CHANGELOG.md'])
str = fileread([nelsonroot(),'/CHANGELOG.md'], 'char')
str = fileread([nelsonroot(),'/CHANGELOG.md'], 'cell')
str = fileread([nelsonroot(),'/CHANGELOG.md'], 'string')
str = 'живете зело, земля, и иже и како люди';
filewrite([tempdir(), 'example_fileread.txt'], str, 'native', 'windows-1251')
T1 = fileread([tempdir(), 'example_fileread.txt'], 'char', 'native', 'windows-1251')
T2 = fileread([tempdir(), 'example_fileread.txt'], 'string', 'native', 'auto')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
filewrite
Write text to a file.
Syntax
- filewrite(filename, txt)
- filewrite(filename, txt, eol)
- filewrite(filename, txt, eol, encoding)
Input argument
- filename - a string: a filename
- txt - a string, cell of string or string array: content to save in file
- eol - a string: 'native' (system default), 'pc' [(char(13), char(10)], 'unix' [char(10)]
- encoding - a string: 'UTF-8' (default), 'ISO-8859-1', 'windows-1251', 'windows-1252', ...
Description
filewrite saves a character array, cell of string or string array to a file.
file saved uses by default UTF-8 (NO-BOM) encoding.
Examples
str = fileread([nelsonroot(),'/CHANGELOG.md'], 'string')
filewrite([tempdir(), 'CHANGELOG.md'], str)
characters encoding
str = 'живете зело, земля, и иже и како люди';
filewrite([tempdir(), 'example_filewrite.txt'], str, 'native', 'windows-1251')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
fopen
Open a file in Nelson.
Syntax
- fid = fopen(filename)
- fid = fopen(filename, permission)
- [fid, msg] = fopen(filename)
- [fid, msg] = fopen(filename, permission)
- [fid, msg] = fopen(filename, permission, machinefmt, encoding)
- [filename, permission, machinefmt, encoding] = fopen(fid)
- fids = fopen('all')
Input argument
- filename - a string: filename to open
- permission - a string: permission applied on file: 'r', 'w', 'a', 'r+', 'a+'
- machinefmt - a string: machine format applied on file: 'n' or 'native', 'b' or 'ieee-be', 'l' or 'ieee-le', 's' or 'ieee-be.l64', 'a' or 'ieee-le.l64'
- encoding - a string: ccharacter encoding applied on file: 'UTF-8', 'ISO-8859-1', 'windows-1251', 'windows-1252', ...
Output argument
- fid - an integer value: a file descriptor or -1 if there is an error.
- msg - a string: error message returned by fopen or ''.
- fids - a vector of integer values: list of files descriptor opened in Nelson.
Description
fopen opens a file in Nelson.
functions fprintf, fgetl, fgets, fread, and fwrite use character encoding for subsequent read and write operations.
Examples
fid = fopen([tempdir(), filesep(), 'fopen_tst'], 'wt');
[filename, permission] = fopen(fid)
fids = fopen('all')
status = fclose(fd)
[filename, permission] = fopen(stdin)
[filename, permission] = fopen(stdout)
[filename, permission] = fopen(stderr)
characters encoding
TEXT_REF = 'Виртуальная';
filename = [tempdir(), 'fwrite_example_Windows-1251.txt'];
F = fopen(filename, 'wt', 'n', 'windows-1251');
W = fwrite(F, TEXT_REF, 'char')
fclose(F);
F = fopen(filename, 'rt', 'n', 'windows-1251');
TXT_READ = fread(F, '*char')
fclose(F);
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
fprintf
Writes data to a file.
Syntax
- fprintf(format, v1, ... , vn)
- fprintf(fid, format, v1, ... , vn)
- R = fprintf(fid, format, v1, ... , vn)
Input argument
- fid - a file descriptor
- format - a string describing the format to used_function.
- v1, ... , vn - data to convert and print according to the previous format parameter.
Output argument
- R - an integer value: number of bytes that fprintf write.
Description
Write data in text form to the file specified by the file descriptor fid.
characters encoding uses fopen parameter.
If fid equals 1 redirection in stdout.
If fid equals 2 redirection in stderr.
The format follows C fprintf syntax.
Value type | format | comment |
---|---|---|
Integer | %i | base 10 |
Integer signed | %d | base 10 |
Integer unsigned | %u | base 10 |
Integer | %o | Octal (base 8) |
Integer | %x | Hexadecimal (lowercase) |
Integer | %X | Hexadecimal (uppercase) |
Floating-point number | %f | Fixed-point notation |
Floating-point number | %e | Exponential notation (lowercase) |
Floating-point number | %E | Exponential notation (uppercase) |
Floating-point number | %g | Exponential notation (compact format, lowercase) |
Floating-point number | %G | Exponential notation (compact format, uppercase) |
Character | %c | Single character |
String | %s | Character vector. |
To display a percent sign, you need to use a double percent sign (%%) in the format string.
Examples
fileID = fopen([tempdir(), 'fprintf.txt'],'wt');
fprintf(fileID, 'an example of %s.', 'text');
fclose(fileID);
R = fileread([tempdir(), 'fprintf.txt'])
fprintf(1, 'an value %g.', pi);
fprintf(2, "an value %g.", pi);
How to use backspace
reverseStr = '';
for idx = 1 : 100
percentDone = idx;
msg = sprintf('Percent done: %3.1f', percentDone);
fprintf([reverseStr, msg]);
reverseStr = repmat(sprintf('\b'), 1, length(msg));
end
Display a percent sign
fprintf(1, '%d%%.', 95)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
fread
Read data in binary form to the file specified by the file descriptor fid.
Syntax
- res = fread(fid)
- res = fread(fid, sz, precision)
- res = fread(fid, sz, precision, skip)
- res = fread(fid, sz, precision, arch)
- res = fread(fid, sz, precision, skip, arch)
- [res, count] = fread(fid, sz, precision, skip, arch)
Input argument
- fid - a file descriptor
- sz - Dimensions of output array: scalar, [m,n] or [m, Inf]
- precision - class of values to read
- skip - number of bytes to skip
- arch - a string specifying the data format for the file.
Output argument
- res - a vector of floating point or integer type numbers
- count - number of characters reads into res
Description
Read data in binary form to the file specified by the file descriptor fid.
supported architecture:
native , n: format of the current machine.
ieee-be, b: IEEE big endian.
ieee-le, l: IEEE little endian.
characters encoding uses fopen parameter.
Examples
A = rand(3,1)
fileID = fopen([tempdir(), 'doubledata.bin'],'w');
fwrite(fileID, A,'double');
fclose(fileID);
fileID = fopen([tempdir(), 'doubledata.bin'],'r');
R = fread(fileID, 'double')
fclose(fileID);
fileID = fopen([tempdir(), 'uint16nine.bin'],'w');
fwrite(fileID,[1:9],'uint16');
fclose(fileID);
fileID = fopen([tempdir(), 'uint16nine.bin'],'r');
A = fread(fileID,[4,Inf],'uint16')
fclose(fileID);
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
frewind
Set position of stream to the beginning.
Syntax
- frewind(fid)
Input argument
- fid - an integer value: file descriptor
Description
frewind puts the pointer at the beginning of file
Example
fileID = fopen([tempdir(), 'frewind.txt'],'wt');
fprintf(fileID, 'son is beautiful.');
frewind(fileID);
fprintf(fileID, 'sun');
fclose(fileID);
R = fileread([tempdir(), 'frewind.txt'])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
fscanf
Reads data from a file.
Syntax
- R = fscanf(fid, format)
- [R, count] = fscanf(fid, format)
- [R, count] = fscanf(fid, format, sizeR)
Input argument
- fid - a file descriptor
- format - a string describing the format to used function.
- sizeR - desired dimensions of R.
Output argument
- R - matrix or character vector.
Description
Read data in text from the file specified by the file descriptor fid.
characters encoding uses fopen parameter.
Value type | format | comment |
---|---|---|
Integer | %i | base 10 |
Integer signed | %d | base 10 |
Integer unsigned | %u | base 10 |
Integer | %o | Octal (base 8) |
Integer | %x | Hexadecimal (lowercase) |
Integer | %X | Hexadecimal (uppercase) |
Floating-point number | %f | Fixed-point notation |
Floating-point number | %e | Exponential notation (lowercase) |
Floating-point number | %E | Exponential notation (uppercase) |
Floating-point number | %g | Exponential notation (compact format, lowercase) |
Floating-point number | %G | Exponential notation (compact format, uppercase) |
Character | %c | Single character |
String | %s | Character vector. |
Example
M = rand(3, 2);
fw = fopen([tempdir, 'example_fscanf.txt'], 'wt');
fprintf(fw, "%f %f %f", M);
fclose(fw);
fd = fopen([tempdir, 'example_fscanf.txt'], 'r');
R = fscanf(fd, "%g %g %g");
fclose(fd);
R
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
fseek
Set the file pointer to a location.
Syntax
- fseek(fid, offset, origin)
- status = fseek(fid, offset, origin)
Input argument
- fid - an integer value: file descriptor
- offset - an integer value: number of bytes to move from origin.
- origin - an integer value or a string: location in the file.
Output argument
- status - an integer value: 0 or -1 if there is an error.
Description
fseek moves the file pointer to the location offset within the file fid.
origin can take as value:
'bof' or -1 : beginning of file.
'cof' or 0 : current position in file.
'eof' or 1 : end of file.
offset may be one of the predefined variables SEEK_CUR (current position, or 0), SEEK_SET (beginning, or -1), or SEEK_END (end of file, or 1).
Example
fileID = fopen([tempdir(), 'fseek.txt'],'wt');
fprintf(fileID, 'son is beautiful.');
fseek(fileID, SEEK_CUR, 'bof');
fprintf(fileID, 'sun');
fclose(fileID);
R = fileread([tempdir(), 'fseek.txt'])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
fsize
Returns size of an opened file.
Syntax
- s = fsize(fid)
Input argument
- fid - a file descriptor
Output argument
- s - an integer value: size of a file.
Description
fsize returns th size of a file opened by fopen.
Example
TXT = 'example about fsize.';
fileID = fopen([tempdir(), 'fsize.txt'],'wt');
fprintf(fileID, TXT);
fsize(fileID)
length(TXT)
status = fclose(fileID);
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
ftell
Returns the offset of the current byte relative to the beginning of a file.
Syntax
- p = ftell(fid)
Input argument
- fid - a file descriptor
Output argument
- p - an integer value: position of the file pointer as the number of characters from the beginning of the file.
Description
ftell returns the offset of the current byte relative to the beginning of the file associated with the named stream fid.
Example
TXT = 'example about ftell.';
fileID = fopen([tempdir(), 'ftell.txt'],'wt');
fprintf(fileID, TXT);
p1 = ftell(fileID)
fseek(fileID, SEEK_CUR, 'bof');
p2 = ftell(fileID)
status = fclose(fileID);
See also
fopen, fprintf, fclose, fseek.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
fwrite
Write data in binary form to the file specified by the file descriptor fid.
Syntax
- count = fwrite(fid, data)
- count = fwrite(fid, data, precision)
- count = fwrite(fid, data, precision, skip)
- count = fwrite(fid, data, precision, skip, arch)
- count = fwrite(fid, data, precision, arch)
Input argument
- fid - a file descriptor
- data - data to write
- precision - class of values to read
- skip - number of bytes to skip
- arch - a string specifying the data format for the file.
Output argument
- count - -1 or number of elements written
Description
Write data in binary form to the file specified by the file descriptor fid.
characters encoding uses fopen parameter.
supported architecture:
native , n: format of the current machine.
ieee-be, b: IEEE big endian.
ieee-le, l: IEEE little endian.
Example
A = rand(3,1)
fileID = fopen([tempdir(), 'doubledata.bin'],'w');
fwrite(fileID, A,'double');
fclose(fileID);
fileID = fopen([tempdir(), 'doubledata.bin'],'r');
R = fread(fileID, 'double')
fclose(fileID);
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
load
load data from .nh5 or .mat file into Nelson's workspace.
Syntax
- load(filename)
- st = load(filename)
- load(filename, var1, ..., varN)
- st = load(filename, var1, ..., varN)
- load(filename, '-mat')
- load(filename, '-nh5')
Input argument
- filename - a string: .nh5 or .mat filename.
- '-mat' or '-nh5' - forces to read file as nh5 or mat.
- var1, ..., varN - string: Names of variables to load into Nelson's workspace.
Output argument
- st - a structure with variables name as fieldnames.
Description
load loads data from .nh5 or .mat file to Nelson's workspace.
Example
A = ones(3, 4);
B = 'hello for open mat users';
save([tempdir(), 'example_load.mat'], 'A', 'B')
clear;
st = load([tempdir(), 'example_load.mat']);
who
st.A
st.B
clear
who
load([tempdir(), 'example_load.mat']);
who
A
B
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
save
save workspace variables to .nh5 or .mat file
Syntax
- save(filename)
- save(filename, version, var1, ..., varN)
- save(filename, '-append', ...)
- save(filename, '-mat', ...)
- save(filename, '-nh5', ...)
- save(filename, '-nocompression', ...)
Input argument
- filename - a string: .nh5 or .mat filename. extension defines format used .mat or .nh5 (default)
- var1, ..., varN - string: Names of variables to save from Nelson's workspace.
- version: '-v7.3', '-v7', '-v6', '-v4' - mat file version used ('-v7.3'). This option will force '-mat'.
- '-mat' - forces to save as mat file (default '-nh5').
- '-nh5' - forces to save as nh5 file (default '-nh5').
- '-append' - append variables to an existing .nh5/.mat file (-v7.3 only).
- '-nocompression' - disable .nh5/.mat file compression.
Description
save save workspace variables to .nh5 or .mat file.
Examples
A = ones(3, 4);
B = 'hello for open mat users';
save([tempdir(), 'example_load.mat'], 'A', 'B')
clear;
st = load([tempdir(), 'example_load.mat']);
who
st.A
st.B
clear
who
load([tempdir(), 'example_load.mat']);
who
A
B
append variables
C = eye(3, 4);
save([tempdir(), 'example_load.mat'], 'C', '-append')
clear;
st = load([tempdir(), 'example_load.mat']);
who
st.A
st.B
st.C
clear
who
load([tempdir(), 'example_load.mat']);
who
A
B
C
compression
C = eye(1000, 1000);
save([tempdir(), 'example_save_with_compression.mat'], 'C')
save([tempdir(), 'example_save_no_compression.mat'], 'C', '-nocompression')
with_compression = dir([tempdir(), 'example_save_with_compression.mat'])
no_compression = dir([tempdir(), 'example_save_no_compression.mat'])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
sscanf
Read formatted data from strings.
Syntax
- R = sscanf(str, format)
- R = sscanf(str, format, sizeR)
- [R, count] = sscanf(...)
- [R, count, errmsg] = sscanf(...)
- [R, count, errmsg, nextindex] = sscanf(...)
Input argument
- str - character array or string scalar.
- format - a string describing the format to used function, see fscanf for supported format.
- sizeR - desired dimensions of R.
Output argument
- R - matrix or character vector.
- count - number of elements read into output array.
- errmsg - Error message.
- nextindex - Position after last character scanned.
Description
Read formatted data from strings.
Example
str = "2.7183 3.1416 0.0073";
R = sscanf(str,'%f',[2 2])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
HDF5
HDF5
Description
Hierarchical Data Format
- h5create - Creates a data set.
- h5dump - dump the content of hdf5 file as text.
- h5ls - List the content of an HDF5 file.
- h5read - Read HDF5 data set.
- h5readatt - Read HDF5 attribute.
- h5write - Writes HDF5 data set.
- h5writeatt - Writes HDF5 attribute.
- isnh5file - Checks if filename a valid .nh5 file
- loadnh5 - load data from .nh5 file into Nelson's workspace.
- savenh5 - save workspace variables to .nh5 file
- whonh5 - List variables in an valid .nh5 file.
- whosnh5 - List variables in an valid .nh5 file with sizes and types.
h5create
Creates a data set.
Syntax
- h5create(filename, datasetname, size, Name1, Value1, ..., NameN, ValueN)
Input argument
- filename - a string: hdf5 filename.
- datasetname - a string: name of the data set.
- size - a row vector specifying the extents of the data set.
- Name1, Value1, ..., NameN, ValueN - Name-Value Pair Arguments.
Description
h5create creates a data set and specify its extent dimensions, datatype and chunk size.
Name-Values pair supported:
Name: Datatype (Nelson® datatypes).
Value: 'double' (default), 'uint64', 'uint32', 'uint16', 'uint8', 'single', 'int64', 'int32', 'int16', or 'int8'.
Name: ChunkSize, chunking layout
Value: []
Name: Deflate, gzip compression level (0-9)
Value: 0 (default)
Name: FillValue, fill value for numeric data sets.
Value: 0 (default)
Name: Fletcher32, enable fletcher32 checksum filter.
Value: logical: false by default
Name: Shuffle, enable shuffle filter.
Value: logical: false by default
Name: TextEncoding, Character encoding.
Value: 'system' or 'UTF-8' (default).
Example
h5create([tempdir(), 'myfile.h5'],'/myDataset1',[10 20]);
h5dump([tempdir(), 'myfile.h5'])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
h5dump
dump the content of hdf5 file as text.
Syntax
- h5dump(filename)
- R = h5dump(filename)
- h5dump(filename, location)
- R = h5dump(filename, location)
Input argument
- filename - a string: hdf5 filename.
- location - a string: name of the path to dump.
Output argument
- R - a string: dump of hdf5 file as text.
Description
h5dump dump the content of hdf5 file as text.
Example
h5create([tempdir(), 'myfile.h5'],'/myDataset2',[10 20]);
h5dump([tempdir(), 'myfile.h5'])
R = h5dump([tempdir(), 'myfile.h5'])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
h5ls
List the content of an HDF5 file.
Syntax
- h5ls(filename)
- R = h5ls(filename)
- h5ls(filename, location)
- R = h5ls(filename, location)
Input argument
- filename - a string: hdf5 filename.
- location - a string: name of the path to list.
Output argument
- R - a cell of strings with two columns (first column gives the names and the second one the type of the listed element).
Description
h5dump list the content of hdf5 file.
Example
h5create([tempdir(), 'myfile.h5'],'/myDataset2',[10 20]);
h5ls([tempdir(), 'myfile.h5'])
R = h5ls([tempdir(), 'myfile.h5'])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
h5read
Read HDF5 data set.
Syntax
- val = h5read(filename, location)
Input argument
- filename - a string: hdf5 filename.
- location - a string: full path identifying a data set.
Output argument
- val - a nelson's variable.
Description
h5read reads data set in location from the HDF5 file.
Example
h5_directory = [modulepath('hdf5','tests'), '/h5'];
double_data = [h5_directory, '/h5ex_t_float.h5'];
R = h5read(double_data,'/DS1')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
h5readatt
Read HDF5 attribute.
Syntax
- attval = h5readatt(filename, location, attname)
Input argument
- filename - a string: hdf5 filename.
- location - a string: full path identifying a group or variable.
- attname - a string: name of an attribute.
Output argument
- attval - a nelson's variable.
Description
h5readatt reads attribute named attname from the HDF5 file.
Example
h5create([tempdir(), 'myfile.h5'],'/myDataset1',[10 20]);
h5writeatt([tempdir(), 'myfile.h5'],'/','creation_date', '26-Dec-2018 16:55:32')
h5readatt([tempdir(), 'myfile.h5'],'/','creation_date')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
h5write
Writes HDF5 data set.
Syntax
- h5write(filename, location, value)
Input argument
- filename - a string: hdf5 filename.
- location - a string: full path identifying a data set.
- value - a value: supported types: double, uint64, uint32, uint16, uint8 single, int64, int32, int16, int8 or character array.
Description
h5write writes data to an entire data set, location, in the HDF5 file.
Example
h5filename = [tempdir(), 'doc_h5write.h5'];
R = rand(3, 4)
h5write(h5filename,'/rand', R);
h5write(h5filename,'/str', 'Hello');
h5dump(h5filename)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
h5writeatt
Writes HDF5 attribute.
Syntax
- h5writeatt(filename, location, attname, attvalue)
- h5writeatt(filename, location, attname, attvalue, 'TextEncoding', encoding)
Input argument
- filename - a string: hdf5 filename.
- location - a string: full path identifying a group or variable.
- attname - a string: name of an attribute.
- attvalue - a value: supported types: double, uint64, uint32, uint16, uint8 single, int64, int32, int16, or int8.
- encoding - a string: 'system' or 'UTF-8' ('UTF-8' default).
Description
h5writeatt writes attribute named attname with the value attvalue to the HDF5 file.
Example
h5create([tempdir(), 'myfile.h5'],'/myDataset1',[10 20]);
h5writeatt([tempdir(), 'myfile.h5'],'/','creation_date', '26-Dec-2018 16:55:32')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
isnh5file
Checks if filename a valid .nh5 file
Syntax
- tf = isnh5file(filename)
- [tf, version, header] = isnh5file(filename)
Input argument
- filename - a string: .nh5 filename.
Output argument
- tf - a logical: true if it is a valid .nh5 file.
- version - a string array: "-v1" or "" if it is undefined.
- header - a string array: header of nh5 file (date created).
Description
isnh5file checks if filename a valid .nh5 file.
Example
A = ones(3, 4);
savemat([tempdir(), 'example_isnh5.mat'], 'A')
R = isnh5file([tempdir(), 'example_isnh5.mat'])
h5save([tempdir(), 'example_isnh5.nh5'], 'A')
[R, VER, HE] = isnh5file([tempdir(), 'example_isnh5.nh5'])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
loadnh5
load data from .nh5 file into Nelson's workspace.
Syntax
- loadnh5(filename)
- st = loadnh5(filename)
- loadnh5(filename, var1, ..., varN)
- st = loadnh5(filename, var1, ..., varN)
Input argument
- filename - a string: .nh5 filename.
- var1, ..., varN - string: Names of variables to load into Nelson's workspace.
Output argument
- st - a structure with variables name as fieldnames.
Description
loadnh5 loads data from .nh5 file to Nelson's workspace.
.nh5 file uses hdf5 file as container.
Example
A = ones(3, 4);
B = 'hello for open mat users';
savenh5([tempdir(), 'example_h5load.nh5'], 'A', 'B')
clear;
st = loadnh5([tempdir(), 'example_h5load.nh5']);
who
st.A
st.B
clear
who
loadnh5([tempdir(), 'example_h5load.nh5']);
who
A
B
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
savenh5
save workspace variables to .nh5 file
Syntax
- savenh5(filename)
- savenh5(filename, var1, ..., varN)
- savenh5(filename, '-append', ...)
- savenh5(filename, '-nocompression', ...)
Input argument
- filename - a string: .nh5 filename.
- var1, ..., varN - string: Names of variables to save from Nelson's workspace.
- '-append' - append variables to an existing .nh5 file.
- '-nocompression' - disable .nh5 file compression.
Description
savenh5 save workspace variables to .nh5 file.
.nh5 file uses hdf5 file as container.
Examples
A = ones(3, 4);
B = 'hello for open mat users';
savenh5([tempdir(), 'example_h5load.nh5'], 'A', 'B')
clear;
st = loadnh5([tempdir(), 'example_h5load.nh5']);
who
st.A
st.B
clear
who
loadnh5([tempdir(), 'example_h5load.nh5']);
who
A
B
append variables
C = eye(3, 4);
savenh5([tempdir(), 'example_h5load.nh5'], 'C', '-append')
clear;
st = loadnh5([tempdir(), 'example_h5load.nh5']);
who
st.A
st.B
st.C
clear
who
loadnh5([tempdir(), 'example_h5load.nh5']);
who
A
B
C
compression
C = eye(1000, 1000);
savenh5([tempdir(), 'example_h5save_with_compression.nh5'], 'C')
savenh5([tempdir(), 'example_h5save_no_compression.nh5'], 'C', '-nocompression')
with_compression = dir([tempdir(), 'example_h5save_with_compression.nh5'])
no_compression = dir([tempdir(), 'example_h5save_no_compression.nh5'])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
whonh5
List variables in an valid .nh5 file.
Syntax
- whonh5(filename)
- ce = whonh5(filename)
- whonh5(filename, var1, ..., varN)
- ce = whonh5(filename, var1, ..., varN)
Input argument
- filename - a string: .nh5 filename.
- var1, ..., varN - string: Names of variables to inspect.
Output argument
- ce - cell of strings with variables names.
Description
whonh5 lists variables in an valid .nh5 file.
Example
A = ones(3, 4);
B = 'Nelson';
C = sparse(true);
D = sparse(3i);
savenh5([tempdir(), 'example_whonh5.nh5'], 'A', 'B', 'C', 'D')
whonh5([tempdir(), 'example_whonh5.nh5'])
ce = whonh5([tempdir(), 'example_whonh5.nh5'])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
whosnh5
List variables in an valid .nh5 file with sizes and types.
Syntax
- whosnh5(filename)
- st = whosnh5(filename)
- whosnh5(filename, var1, ..., varN)
- st = whosnh5(filename, var1, ..., varN)
Input argument
- filename - a string: .nh5 filename.
- var1, ..., varN - string: Names of variables to inspect.
Output argument
- st - stores information about the variables in the structure array st.
Description
whosnh5 lists variables in an valid .nh5 file.
Example
A = ones(3, 4);
B = 'Nelson';
C = sparse(true);
D = sparse(3i);
savenh5([tempdir(), 'example_whosnh5.nh5'], 'A', 'B', 'C', 'D')
whosnh5([tempdir(), 'example_whosnh5.nh5'])
st = whosnh5([tempdir(), 'example_whosnh5.nh5'])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
MATIO
MATIO
Description
MAT-File Read/Write I/O Functions
- ismatfile - Checks if filename a valid .mat file
- loadmat - load data from .mat file into Nelson's workspace.
- savemat - save workspace variables to .mat file
- whomat - List variables in an valid .mat file.
- whosmat - List variables in an valid .mat file with sizes and types.
ismatfile
Checks if filename a valid .mat file
Syntax
- [tf, ver, header] = ismatfile(filename)
Input argument
- filename - a string: .mat filename.
Output argument
- tf - a logical: true if it is a valid .mat file.
- ver - a string array: version of .mat file ("-v7.3", "-v7" or "-v6").
- header - a string array: header of .mat file (date).
Description
ismatfile checks if filename a valid .mat file.
Bibliography
Thanks to MATIO library (http://sourceforge.net/projects/matio/).
Example
A = ones(3, 4);
savemat([tempdir(), 'example_loadmat-v7.3.mat'], 'A', '-v7.3')
savemat([tempdir(), 'example_loadmat-v7.mat'], 'A', '-v7')
savemat([tempdir(), 'example_loadmat-v6.mat'], 'A', '-v6')
[tf, ver] = ismatfile([tempdir(), 'example_loadmat-v7.3.mat'])
[tf, ver] = ismatfile([tempdir(), 'example_loadmat-v7.mat'])
[tf, ver] = ismatfile([tempdir(), 'example_loadmat-v6.mat'])
[tf, ver, header] = ismatfile([tempdir(), 'example_not_existing.mat'])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
loadmat
load data from .mat file into Nelson's workspace.
Syntax
- loadmat(filename)
- st = loadmat(filename)
- loadmat(filename, var1, ..., varN)
- st = loadmat(filename, var1, ..., varN)
Input argument
- filename - a string: .mat filename.
- var1, ..., varN - string: Names of variables to load into Nelson's workspace.
Output argument
- st - a structure with variables name as fieldnames.
Description
loadmat loads data from .mat file to Nelson's workspace.
Bibliography
Thanks to MATIO library (http://sourceforge.net/projects/matio/).
Example
A = ones(3, 4);
B = 'hello for open mat users';
savemat([tempdir(), 'example_loadmat.mat'], 'A', 'B')
clear;
st = loadmat([tempdir(), 'example_loadmat.mat']);
who
st.A
st.B
clear
who
loadmat([tempdir(), 'example_loadmat.mat']);
who
A
B
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
savemat
save workspace variables to .mat file
Syntax
- savemat(filename)
- savemat(filename, version, var1, ..., varN)
- savemat(filename, '-append', ...)
- savemat(filename, '-nocompression', ...)
Input argument
- filename - a string: .nh5 filename.
- var1, ..., varN - string: Names of variables to save from Nelson's workspace.
- '-v7.3' - default mat file used.
- '-v7' - mat file version 7 used as output format.
- '-v6', '-v4' - mat file version 6 or 4 used as output format.
- '-append' - append variables to an existing .mat file (-v7.3 only).
- '-nocompression' - disable .mat file compression.
Description
savemat save workspace variables to .mat file.
Nelson's data types are converted into the Mat file equivalents.
Bibliography
Thanks to MATIO library (http://sourceforge.net/projects/matio/).
Examples
A = ones(3, 4);
B = 'hello for open mat users';
savemat([tempdir(), 'example_loadmat.mat'], 'A', 'B')
clear;
st = loadmat([tempdir(), 'example_loadmat.mat']);
who
st.A
st.B
clear
who
loadmat([tempdir(), 'example_loadmat.mat']);
who
A
B
append variables
C = eye(3, 4);
savemat([tempdir(), 'example_loadmat.mat'], 'C', '-append')
clear;
st = loadmat([tempdir(), 'example_loadmat.mat']);
who
st.A
st.B
st.C
clear
who
loadmat([tempdir(), 'example_loadmat.mat']);
who
A
B
C
compression
C = eye(1000, 1000);
savemat([tempdir(), 'example_savemat_with_compression.mat'], 'C')
savemat([tempdir(), 'example_savemat_no_compression.mat'], 'C', '-nocompression')
with_compression = dir([tempdir(), 'example_savemat_with_compression.mat'])
no_compression = dir([tempdir(), 'example_savemat_no_compression.mat'])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
whomat
List variables in an valid .mat file.
Syntax
- whomat(filename)
- ce = whomat(filename)
- whomat(filename, var1, ..., varN)
- ce = whomat(filename, var1, ..., varN)
Input argument
- filename - a string: .mat filename.
- var1, ..., varN - string: Names of variables to inspect.
Output argument
- ce - cell of strings with variables names.
Description
whomat lists variables in an valid .mat file.
Bibliography
Thanks to MATIO library (http://sourceforge.net/projects/matio/).
Example
A = ones(3, 4);
B = 'Nelson';
C = sparse(true);
D = sparse(3i);
savemat([tempdir(), 'example_whomat-v7.3.mat'], 'A', 'B', 'C', 'D', '-v7.3')
whomat([tempdir(), 'example_whomat-v7.3.mat'])
ce = whomat([tempdir(), 'example_whomat-v7.3.mat'])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
whosmat
List variables in an valid .mat file with sizes and types.
Syntax
- whosmat(filename)
- st = whosmat(filename)
- whosmat(filename, var1, ..., varN)
- st = whosmat(filename, var1, ..., varN)
Input argument
- filename - a string: .mat filename.
- var1, ..., varN - string: Names of variables to inspect.
Output argument
- st - stores information about the variables in the structure array st.
Description
whosmat lists variables in an valid .mat file.
Bibliography
Thanks to MATIO library (http://sourceforge.net/projects/matio/).
Example
A = ones(3, 4);
B = 'Nelson';
C = sparse(true);
D = sparse(3i);
savemat([tempdir(), 'example_whosmat-v7.3.mat'], 'A', 'B', 'C', 'D', '-v7.3')
whosmat([tempdir(), 'example_whosmat-v7.3.mat'])
st = whosmat([tempdir(), 'example_whosmat-v7.3.mat'])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
JavaScript Object Notation
JavaScript Object Notation
Description
Functions to transmit data objects consisting of attribute–value pairs and array data types (or any other serializable value).
- jsondecode - decodes a JSON string to Nelson object.
- jsonencode - encodes a Nelson object into a JSON string.
- jsonprettyprint - format an JSON string.
jsondecode
decodes a JSON string to Nelson object.
Syntax
- res = jsondecode(json_str)
Input argument
- json_str - a json string.
Output argument
- res - a nelson variable converted from JSON.
Description
jsondecode converts JSON object field names to Nelson structure field names
Bibliography
http://www.rfc-editor.org/rfc/rfc7159.txt
Examples
field1 = 'f1'; value1 = zeros(1,10);
field2 = 'f2'; value2 = {'a', 'b'};
field3 = 'f3'; value3 = {pi, pi*pi};
field4 = 'f4'; value4 = {'fourth'};
s = struct(field1,value1,field2,value2,field3,value3,field4,value4)
r = jsonencode(s)
r2 = jsondecode(r)
jsondecode(fileread([modulepath('json'), '/examples/patient.json']))
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
jsonencode
encodes a Nelson object into a JSON string.
Syntax
- res = jsonencode(obj)
- res = jsonencode(obj, 'ConvertInfAndNaN', true_or_false)
Input argument
- obj - a Nelson object: struct, cell, matrix.
- true_or_false - a logical: if true, Inf, NaN are converted to 'Inf' or 'Nan'.
Output argument
- res - a string: JSON text.
Description
jsonencode converts a Nelson variable to JSON text.
jsonencode does not support complex numbers, sparse arrays, function handle, and others handle.
jsonencode can be overloaded to manage your own type.
By default jsonencode Inf values are converted to the string "Inf", NaN values are converted to 'null'.
Warning: The shape of a matrix and data type are not always preserved.
Bibliography
http://www.rfc-editor.org/rfc/rfc7159.txt
Example
field1 = 'f1'; value1 = zeros(1,10);
field2 = 'f2'; value2 = {'a', 'b'};
field3 = 'f3'; value3 = {pi, pi*pi};
field4 = 'f4'; value4 = {'fourth'};
s = struct(field1,value1,field2,value2,field3,value3,field4,value4);
r = jsonencode(s)
filewrite([tempdir(), 'example.json'], r);
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
jsonprettyprint
format an JSON string.
Syntax
- res = jsonprettyprint(txt)
Input argument
- txt - a valid JSON text.
Output argument
- res - a string: a formatted JSON text (human readable).
Description
jsonprettyprint formats a JSON text string to be human readable.
Example
field1 = 'f1'; value1 = zeros(1,10);
field2 = 'f2'; value2 = {'a', 'b'};
field3 = 'f3'; value3 = {pi, pi*pi};
field4 = 'f4'; value4 = {'fourth'};
s = struct(field1,value1,field2,value2,field3,value3,field4,value4);
r = jsonencode(s)
jsonprettyprint(r)
See also
jsondecode, jsonencode, filewrite.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
File archiver functions
File archiver functions
Description
file archiver functions
unzip
Decompress zip file.
Syntax
- res = unzip(zipname)
- res = unzip(zipname, rootdir)
Input argument
- zipname - a string: zip archive filename.
- rootdir - a character vector or string scalar: root path for the files to decompress.
Output argument
- res - a cell array of character vectors containing the names of the files decompressed.
Description
unzip extracts archived contents. Timestamps and attributes are preserved for each file.
Example
zip([tempdir(), 'test.zip'], [nelsonroot(), '/module_skeleton']);
r = unzip([tempdir(), 'test.zip'], [tempdir(), createGUID()])
See also
zip.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
zip
Compress files into zip file.
Syntax
- res = zip(zipname, files)
- res = zip(zipname, files, rootdir)
Input argument
- zipname - a string: zip archive destination file.
- files - a character vector, a cell array of character vectors, or a string array: Names of files or folders to compress.
- rootdir - a character vector or string scalar: root path for the files to compress.
Output argument
- res - a cell array of character vectors containing the names of the files included in zip archive.
Description
zip compress files and directories into zip archive.
Each individual file must be smaller than 4 GB.
Number of files specified must be less than 65535.
Example
zip([tempdir(), 'test.zip'], [nelsonroot(), '/module_skeleton'])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
Audio playback
Audio playback
Description
Audio playback functions.
- audiodevinfo - Get audio devices information.
- audioinfo - Get audio file information.
- audiometadata - Get/Set metadata of audio file .
- audioplayer - Audio player object.
- audioplayer_delete - Removes audioplayer object.
- audioplayer_fieldnames - Returns the properties name of an audioplayer object.
- audioplayer_get - Get property value from audioplayer interface.
- audioplayer_pause - Pause an audioplayer object.
- audioplayer_set - Set object or interface property to specified value.
- audioplayer_stop - Stops an audioplayer object.
- audioplayer_used - Returns list of current used audioplayer handle.
- audioread - Read an audio file.
- audiosupportedformats - Get audio file supported formats.
- audiowrite - Writes an audio file.
- beep - Produces a beep sound.
- isplaying - get info about audio playback is in progress.
- lin2mu - Convert audio data from linear singal to mu-law.
- mu2lin - Convert audio data from mu-law to linear signal.
- play - Plays an audioplayer object.
- playblocking - Plays an audioplayer object with blocking.
- resume - Resumes an audioplayer object.
- sound - Convert matrix of signal data to sound and play it.
- soundsc - Scale data and play as sound.
- stop - Stops an audioplayer object.
audiodevinfo
Get audio devices information.
Syntax
- devices = audiodevinfo()
- devices = audiodevinfo('default')
- devices = audiodevinfo(io)
- name = audiodevinfo(io, id)
- id = audiodevinfo(io, name)
- id = audiodevinfo(io, rate, bits, channels)
- support = audiodevinfo(io, id, rate, bits, channels)
Input argument
- io - input (1) or output (0) device
- id - an integer value.
- name - a string: name of the audio device to search.
- rate - a double scalar: sample rate.
- bits - an integer value: bits per sample.
- channels - an integer value: number of audio channel.
Output argument
- devices - struct array
- name - a string: name of the audio device specified by io and id.
- id - an integer value.
- support - a logical: true if values supported or false.
Description
audiodevinfo returns a structure with available audio input and output devices.
devices = audiodevinfo('default') returns a structure with default used audio input and output devices.
Example
info = audiodevinfo()
OUTPUT_DEVICE = 0;
INPUT_DEVICE = 1;
for k = [1:audiodevinfo(OUTPUT_DEVICE)]
info.output(k)
end
for k = [1:audiodevinfo(INPUT_DEVICE)]
info.output(k)
end
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
audioinfo
Get audio file information.
Syntax
- info = audioinfo(filename)
Input argument
- filename - a string: an valid audio filename.
Output argument
- info - a struct: information about audio file.
Description
audioinfo returns a structure with information about audio file.
Many audio formats are supported as OGG, FLAC, WAV, RAW.
Example
wav_file = [modulepath('audio'), '/examples/haha.wav'];
info = audioinfo(wav_file)
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
audiometadata
Get/Set metadata of audio file .
Syntax
- info = audiometadata(filename)
- info_previous = audiometadata(filename, info_new)
Input argument
- filename - a string: an valid audio filename.
- info_new - a struct: new information about audio file to set.
Output argument
- info - a struct: information about audio file.
- info_previous - a struct: previous information about audio file.
Description
audiometadata returns a structure with metadata of an audio file.
audiometadata manages all tags available in the audio file.
Many audio formats are supported as OGG, FLAC, WAV, RAW.
Examples
wav_file = [modulepath('audio'), '/examples/haha.wav'];
info = audiometadata(wav_file)
wav_file = [modulepath('audio'), '/examples/haha.wav'];
modified_wav_file = [tempdir(), 'haha_modified_tags.wav'];
if isfile(modified_wav_file)
rmfile(modified_wav_file);
end
copyfile(wav_file, modified_wav_file);
info = audiometadata(modified_wav_file)
info.artist = 'Nelson';
audiometadata(modified_wav_file, info);
info = audiometadata(modified_wav_file)
if isfile(modified_wav_file)
rmfile(modified_wav_file);
end
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
audioplayer
Audio player object.
Syntax
- playerObj = audioplayer(y, fs)
- playerObj = audioplayer(y, fs, nbits)
- playerObj = audioplayer(y, fs, nbits, id)
Input argument
- y - a vector or matrix array: int8,uint8, int16, single or double.
- fs - a double value: sampling rate in Hz.
- nbits - a double value: bits per sample (16 by default).
- id - a double value: device identifier (-1 by default).
Output argument
- playerObj - audioplayer object
Description
audioplayer returns an audioplayer object to play data on an output device.
audioplayer object uses global scope and need to be deleted by user.
audioplayer can play multichannels data if your sound card supports it.
Example
signal = rand(2, 44100) - 0.5;
playObj = audioplayer(signal, 44100, 16)
play(playObj)
sleep(2)
delete(playObj)
playObj
See also
delete, play, stop, resume, pause.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
audioplayer_delete
Removes audioplayer object.
Syntax
- audioplayer_delete(h)
- delete(h)
Input argument
- h - a handle: an audioplayer object.
Description
delete(h) releases audioplayer object.
Do not forget to clear h afterward.
Example
audioplayer_used(),delete(audioplayer_used())
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
audioplayer_fieldnames
Returns the properties name of an audioplayer object.
Syntax
- l = audioplayer_fieldnames(h)
- l = fieldnames(h)
Input argument
- h - a audioplayer object.
Output argument
- l - a cell of strings.
Description
Example
signal = rand(2, 44100) - 0.5;
playObj = audioplayer(signal, 44100, 16)
fieldnames(playObj)
delete(playObj)
clear playObj
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
audioplayer_get
Get property value from audioplayer interface.
Syntax
- v = get(h, propertyname)
- v = audioplayer_get(h, propertyname)
- v = h.propertyname
Input argument
- h - an audioplayer object.
- propertyname - a string: the property's name of audioplayer object.
Output argument
- v - a nelson variable.
Description
The function returns the value of the property specified in the string, propertyname.
Example
signal = rand(2, 44100) - 0.5;
playObj = audioplayer(signal, 44100, 16)
playObj.Running
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
audioplayer_pause
Pause an audioplayer object.
Syntax
- pause(playObj)
Input argument
- playObj - an audioplayer object.
Description
pause pauses an audioplayer object.
Example
signal = rand(2, 44100) - 0.5;
playObj = audioplayer(signal, 44100, 16)
play(playObj)
sleep(2)
pause(playObj)
delete(playObj)
playObj
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
audioplayer_set
Set object or interface property to specified value.
Syntax
- set(h, propertyname, value)
- audioplayer_set(h, propertyname, value)
- h.propertyname = value
Input argument
- h - a audioplayer object.
- propertyname - a string: the property's name of audioplayer object.
- value - a string, boolean, double ...
Description
The function sets the property specified in the string propertyname to the given value.
Example
signal = rand(2, 44100) - 0.5;
playObj = audioplayer(signal, 44100, 16)
playObj.Tag = 'my audio object'
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
audioplayer_stop
Stops an audioplayer object.
Syntax
- stop(playObj)
Input argument
- playObj - an audioplayer object.
Description
stop stops an audioplayer object.
Example
signal = rand(2, 44100) - 0.5;
playObj = audioplayer(signal, 44100, 16)
play(playObj)
sleep(2)
stop(playObj)
delete(playObj)
playObj
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
audioplayer_used
Returns list of current used audioplayer handle.
Syntax
- r = audioplayer_used()
Output argument
- h - a vector of audioplayer handle.
Description
Returns list of current used audioplayer handle.
See also
audioplayer_set (set), audioplayer_get (get).
Example
audioplayer_used(),delete(audioplayer_used())
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
audioread
Read an audio file.
Syntax
- y = audioread(filename)
- [y, fs] = audioread(filename)
- [y, fs] = audioread(filename, range)
- [y, fs] = audioread(filename, type)
- [y, fs] = audioread(filename, range, type)
Input argument
- filename - a string: an existing filename.
- range - a vector: [start end].
- type - a string: 'double' or 'native'.
Output argument
- y - a matrix: audio data.
- fs - an integer value: sampling rate.
Description
audioread reads an audio file.
Supported format: 'wav', 'ogg', 'flac', 'mp3', 'caf', 'au', 'aiff'. See audiosupportedformats function to have all supported formats.
If type is 'native' then audio data depends on the file format (single, double, integers).
Example
wav_audio = [modulepath('audio'), '/examples/haha.wav'];
[y, fs] = audioread(wav_audio);
playObj = audioplayer(y, fs);
playblocking(playObj)
delete(playObj)
clear playObj
See also
playblocking, audioplayer, audiosupportedformats, audiowrite.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
audiosupportedformats
Get audio file supported formats.
Syntax
- formats = audiosupportedformats()
Output argument
- formats - struct array with 'Name', 'Extension', 'Subformats' fieldnames.
Description
audiosupportedformats returns a structure with supported audio file formats.
Example
formats = audiosupportedformats();
for k = [1: length(formats)]
formats(k).Name
formats(k).Extension
formats(k).Subformats
end
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
audiowrite
Writes an audio file.
Syntax
- audiowrite(filename, y, fs)
- audiowrite(filename, y, fs, fieldname, fieldvalue, ...)
Input argument
- filename - a string: filename to create.
- y - a matrix: audio data.
- fs - an integer value: sampling rate.
- fieldname - a string: 'BitsPerSample', 'BitRate', 'Quality', 'Title', 'Artist' or 'Comment' .
- fieldvalue - value associated to the fieldname.
Description
audiorwrite writes an audio file.
More 26 files format supported. See audiosupportedformats function to have all supported formats.
Example
wav_audio = [modulepath('audio'), '/examples/haha.wav'];
[y, fs] = audioread(wav_audio);
dest_ogg = [tempdir(), 'haha.ogg'];
audiowrite(dest_ogg, y, fs);
dest_flac = [tempdir(), 'haha.flac'];
audiowrite(dest_flac, y, fs);
dest_mp3 = [tempdir(), 'haha.mp3'];
audiowrite(dest_mp3, y, fs);
dest_caf = [tempdir(), 'haha.caf'];
audiowrite(dest_caf, y, fs);
See also
audioplayer, audiosupportedformats.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
beep
Produces a beep sound.
Syntax
- beep
- beep(str)
- str = beep
Input argument
- str - a string: 'on' or 'off'.
Output argument
- str - a string: 'on' or 'off'.
Description
beep produces an beep system.
Example
beep('off')
beep
beep('on')
beep
s = beep
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
isplaying
get info about audio playback is in progress.
Syntax
- play(playObj)
Input argument
- play - an audioplayer object.
Output argument
- play - an logical.
Description
isplaying get information about audio playback is in progress.
Example
signal = rand(2, 44100) - 0.5;
playObj = audioplayer(signal, 44100, 16)
play(playObj)
isplaying(playObj)
pause(playObj)
isplaying(playObj)
delete(playObj)
playObj
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
lin2mu
Convert audio data from linear singal to mu-law.
Syntax
- mu = lin2mu(y)
Input argument
- y - linear signal with -1 ≤ y ≤ 1.
Output argument
- mu - mu-law encoded 8-bit audio signals, with 0 ≤ mu ≤ 255.
Description
mu = lin2mu(y) converts audio data from linear to mu-law.
Bibliography
https://en.wikipedia.org/wiki/%CE%9C-law_algorithm
Example
mu = lin2mu([-1:0.5:1])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
mu2lin
Convert audio data from mu-law to linear signal.
Syntax
- y = mu2lin(mu)
Input argument
- mu - mu-law encoded 8-bit audio signals, with 0 ≤ mu ≤ 255.
Output argument
- y - linear signal.
Description
y = mu2lin(mu) converts audio data from mu-law to linear.
Bibliography
"A New Digital Technique for Implementation of Any Continuous PCM Companding Law," Villeret, Michel, et al. 1973 IEEE Int. Conf. on Communications, Vol 1, 1973, pg. 11.12-11.17.
Example
l = mu2lin([0:20:255])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
play
Plays an audioplayer object.
Syntax
- play(playObj)
- play(playObj, start)
- play(playObj, [start end])
Input argument
- playObj - an audioplayer object.
- start - an integer value: first sample to play.
- end - an integer value: last sample to play.
Description
play plays an audioplayer object.
Example
signal = rand(2, 44100) - 0.5;
playObj = audioplayer(signal, 44100, 16)
play(playObj)
sleep(2)
delete(playObj)
playObj
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
playblocking
Plays an audioplayer object with blocking.
Syntax
- playblocking(playObj)
- playblocking(playObj, start)
- playblocking(playObj, [start end])
Input argument
- playObj - an audioplayer object.
- start - an integer value: first sample to play.
- end - an integer value: last sample to play.
Description
playblocking plays an audioplayer object until playback is finished.
Example
signal = rand(2, 44100) - 0.5;
playObj = audioplayer(signal, 44100, 16)
playblocking(playObj)
delete(playObj)
playObj
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
resume
Resumes an audioplayer object.
Syntax
- resume(playObj)
Input argument
- playObj - an audioplayer object.
Description
resume resumes an audioplayer object.
Example
signal = rand(2, 44100) - 0.5;
playObj = audioplayer(signal, 44100, 16)
play(playObj)
pause(playObj)
stop(playObj)
resume(playObj)
playObj
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
sound
Convert matrix of signal data to sound and play it.
Syntax
- sound(y)
- sound(y, Fs)
- sound(y, Fs, nBits)
- sound(y, Fs, nBits)
Input argument
- y - column vector or m-by-2 matrix.
- Fs - sample rate, a positive number, 8192 by default.
- nBits - bit depth of sample values: 8, 16 (default), 24.
Description
sound plays audio signal y to the speaker at sample rate of Fs hertz and uses nBits bits per sample.
Example
signal = rand(2, 44100) - 0.5;
sound(signal, 44110, 16)
See also
audioplayer, playblocking, soundsc.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
soundsc
Scale data and play as sound.
Syntax
- soundsc(y)
- soundsc(y, Fs)
- soundsc(y, Fs, nBits)
- soundsc(y, Fs, nBits, yRange)
Input argument
- y - column vector or m-by-2 matrix.
- Fs - sample rate, a positive number, 8192 by default.
- nBits - bit depth of sample values: 8, 16 (default), 24.
- yRange - range of audio data to scale: | two-element vector or [-max(abs(y)),max(abs(y))] default.
Description
soundsc scales the values of audio signal y to fit in the range from –1.0 to 1.0 and play as sound.
Example
signal = rand(2, 44100) - 0.5;
soundsc(signal, 44110, 16)
See also
audioplayer, playblocking, sound.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
stop
Stops an audioplayer object.
Syntax
- stop(playObj)
Input argument
- playObj - an audioplayer object.
Description
stop stops an audioplayer object.
Example
signal = rand(2, 44100) - 0.5;
playObj = audioplayer(signal, 44100, 16)
play(playObj)
sleep(2)
stop(playObj)
delete(playObj)
playObj
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
SIO client
SIO client
Description
Nelson in the cloud
- doc - Displays documentation.
- sioemit - Emit an event to web client.
- siogetvariable - Emit an value of variable to web browser.
doc
Displays documentation.
Syntax
- doc
- doc function_name
- doc('function_name')
Input argument
- function_name - a string: function name
Description
doclaunches web browser.
doc('function_name')displays the help about function designed by 'function_name'.
Examples
doc()
doc sin
doc is
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
sioemit
Emit an event to web client.
Syntax
- sioemit(name, message)
- sioemit(name)
Input argument
- name - a string: event name
- message - a string: message to emit
Description
sioemit emits an event to client.
Example
sioemit('event_demo', jsonencode(eye(3,3)))
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
siogetvariable
Emit an value of variable to web browser.
Syntax
- siogetvariable(variable_name)
Input argument
- variable_name - a string: name of variable
Description
siogetvariable send value of a variable to web browser.
Example
A = eye(3, 3); siogetvariable('A')
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
Inter Process Communication
Inter Process Communication
Description
Inter Process Communication functions
getpid
Get nelson(s) Process IDentificator.
Syntax
- p = getpid()
- v = getpid('available')
Input argument
- 'available' - a string.
Output argument
- p - a double: current Process Identificator.
- v - a vector of double: list of nelson Processes Identification (with same arch) currently running for current user.
Description
p = getpid() returns current nelson process identificator currently running on computer.
v = getpid('available') returns list of nelson processes identificators (with same arch) running for current user.
win64 and win32 are two differents architecture but they can run in same time.
Example
p = getpid()
getpid('available')
unix('nelson-gui &')
sleep(5) % detached process need to wait to see available
getpid('available')
unix('nelson-gui &')
sleep(5) % detached process need to wait to see available
getpid('available')
unix('nelson-gui &')
sleep(5) % detached process need to wait to see available
getpid('available')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
ipc
Inter process communicator.
Syntax
- O = ipc(pid, 'eval', cmd)
- B = ipc(pid, 'isvar', name, scope)
- V = ipc(pid, 'get', name)
- V = ipc(pid, 'get', name, scope)
- TF = ipc(pid, 'minimize')
- ipc(pid, 'post', cmd)
- ipc(pid, 'post', cmd, scope)
- ipc(pid, 'put', var, name)
- ipc(pid, 'put', var, name, scope)
- ipc(pid, 'minimize', tf)
Input argument
- 'post' - a string: post a command to evaluate to another nelson's process in base scope (not blocking).
- 'eval' - a string: post a command to evaluate to another nelson's process in base scope (blocking).
- 'isvar' - a string: check if a variable exists into another nelson's process.
- 'put' - a string: send a variable into another nelson's process.
- 'get' - a string: get a variable from another nelson's process.
- 'minimize' - a string: minimize main window from another nelson's process.
Output argument
- B - a logical: true if variable exists.
- V - a variable from another nelson.
- TF - a logical: true if destination process is minimized.
- O - a character array: output of evaluate string.
Description
ipc allows to execute, get, put variables between multiple nelson's process.
All serializable nelson's types are supported. Unsupported types will be replaced by an empty matrix and a warning.
LIMITATION:
The limit for the size of data transferred is 5000x5000 double. On 32 bits architecture, 1024x1024 double.
Current limitation to limit memory usage.
Examples
master_pid = getpid()
initial_pids = getpid('available')
% Creates 4 nelsons process
N = 4;
for i = 1:N
cmd = sprintf('nelson-gui -e MASTER_PID=%d &', i);
unix(cmd);
sleep(5)
end
current_pids = getpid('available')
% wait clients ready
for p = current_pids
if p ~= master_pid
while(~ipc(p, 'isvar', 'MASTER_PID')), sleep(1), end
end
end
% Creates random matrix in others Nelson
n = 0;
for p = current_pids
if p ~= master_pid
cmd = sprintf('rng(%d);M = rand(10, 10); disp(M)', n);
ipc(p, 'post', cmd);
n = n + 1;
end
end
% Creates a matrix with matrix from others Nelson
C = [];
for p = current_pids
if p ~= master_pid
R = ipc(p, 'get', 'M');
C = [C; R]
n = n + 1;
end
end
% close all clients
for p = current_pids
if p ~= master_pid
ipc(p, 'post', 'exit')
end
end
ipc(getpid(), 'eval', 'dir')
ipc(getpid(), 'minimize', true)
ipc(getpid(), 'minimize')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
Dynamic link
Dynamic link
Description
By default, Nelson does not try to detect a C/C++ compiler on Windows. Do not forget to run 'configuremsvc' or 'configuremingw' once.
- Build C/C++ code on the fly - Build C/C++ code on the fly
- Supported C/C++ compilers
- libpointer datatype - C/Nelson equivalent data types
- cmake - call CMake tool
- configuremingw - Configure Nelson to use MinGW as default C compiler
- configuremsvc - Configure Nelson to use visual studio as default compiler
- dlcall - C or Fortran Foreign function call.
- dlclose - Removes dllib object.
- dlgeneratecleaner - Generates cleaner.m file for C++ gateway.
- dlgenerategateway - Generates C++ gateway.
- dlgenerateloader - Generates loader.m file for C++ gateway.
- dlgeneratemake - Generates a makefile for building a dynamic library.
- dlgenerateunloader - Generates unloader.m file for C++ gateway.
- dlgetnelsonincludes - Returns paths of Nelson include directories.
- dlgetnelsonlibraries - Returns paths to Nelson library files.
- dllib_used - Returns list of current used dllib handle.
- dllibinfo - Returns list of available symbols in an shared library.
- dllibisloaded - Checks if shared library is loaded.
- dlmake - call make or nmake tool
- dlopen - Loads an dynamic library.
- dlsym - Loads a C/Fortran symbol for an dynamic library.
- dlsym_delete - Removes dlsym object.
- dlsym_used - Returns list of current used dlsym handle.
- findcmake - find CMake path.
- getdynlibext - Returns the extension of dynamic libraries.
- havecompiler - Detect if a C/C++ compiler is configured.
- libpointer - Creates an C pointer object usuable in Nelson.
- libpointer_delete - Removes libpointer object.
- libpointer_isNull - Checks if libpointer handle points on NULL pointer.
- libpointer_plus - plus operator on libpointer handle.
- libpointer_reshape - Reshapes libpointer dimensions.
- libpointer_setdatatype - Set type of an libpointer handle.
- libpointer_used - Returns list of current used libpointer handle.
- loadcompilerconf - load compiler configuration.
- removecompilerconf - Remove used compiler configuration (on Windows).
- vswhere - Locate Visual Studio 2017, 2019 and newer installations
Build C/C++ code on the fly
Build C/C++ code on the fly
Description
Nelson provides a cross-platform command-line tool written in Nelson for compiling native addon modules for Nelson.
It takes away the pain of dealing with the various differences in build platforms.
Example
if ispc() && ~havecompiler()
configuremsvc()
end
C_CONTENT = ["double";
"functionC(double x)";
"{";
" return x + 8;";
"}"];
DEST_DIR = [tempdir(), 'example_C'];
mkdir(DEST_DIR);
C_DEST_FILE = [tempdir(), 'example_C/demo.c'];
filewrite(C_DEST_FILE, C_CONTENT)
dlgeneratemake(DEST_DIR, 'C_DEMO', {C_DEST_FILE}, {DEST_DIR})
[res, message] = dlmake(DEST_DIR)
lib = dlopen([DEST_DIR, '/C_DEMO', getdynlibext()])
c = dllibinfo(lib)
f = dlsym(lib, 'functionC', 'double', {'double'});
R = dlcall(f, 3) % 8 + 3
dlclose(lib)
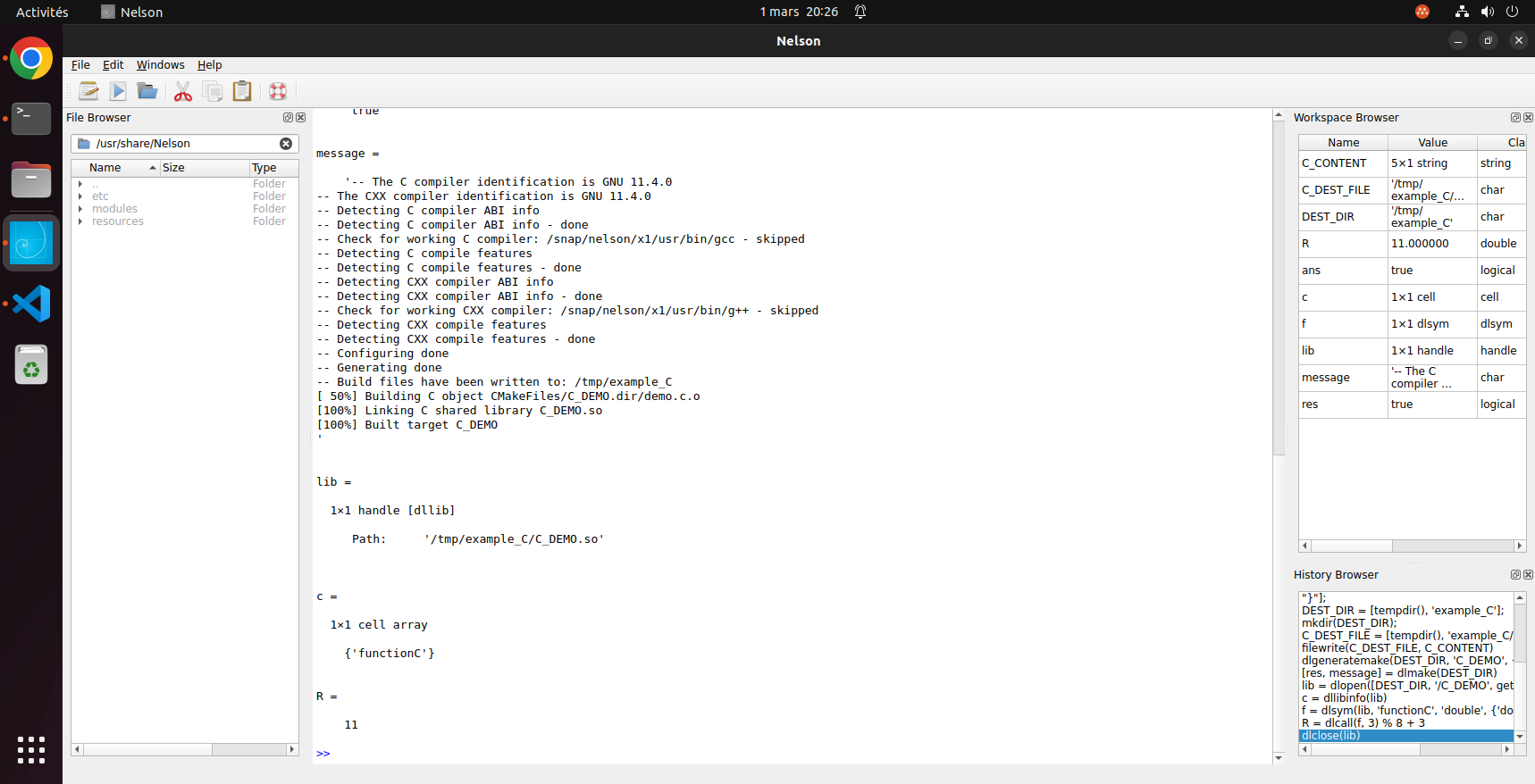
See also
configuremsvc, dlgeneratemake, dlmake, dlopen, dllibinfo, dlsym, dlcall.
History
Version | Description |
---|---|
1.2.0 | initial version |
Author
Allan CORNET
Supported C/C++ compilers
Description
Windows:
- Microsoft Visual Studio C/C++ 2022 all versions (Pro, Enterprise or Community)
- Microsoft Visual Studio C/C++ 2019 all versions (Pro, Enterprise or Community)
- Microsoft Visual Studio C/C++ 2017 all versions (Pro, Enterprise or Community)
- MinGW
GNU/Linux:
- GNU C/C++ compilers packaged with all distributions.
MacOS X:
- XCode C/C++ compilers.
See also
configuremsvc, vswhere, configuremingw.
Author
Allan CORNET
libpointer datatype
C/Nelson equivalent data types
Description
This table shows these Nelson types with their equivalent C types.
Nelson type | C type |
---|---|
logical (scalar) | uint8_t |
uint8 (scalar) | uint8_t |
int8 (scalar) | int8_t |
uint16 (scalar) | uint16_t |
int16 (scalar) | int16_t |
uint32 (scalar) | uint32_t |
int32 (scalar) | uint32_t |
uint64 (scalar) | uint64_t |
int64 (scalar) | int64_t |
float, single (scalar) | float |
double (scalar) | double |
cstring (string utf-8) | char * |
wstring (string unicode) | wchar_t * |
void | void |
logicalPtr (logical vector or matrix) | uint8_t * |
uint8Ptr (uint8 vector or matrix) | uint8_t * |
int8Ptr (int8 vector or matrix) | int8_t * |
uint16Ptr (uint16 vector or matrix) | uint16_t * |
int16Ptr (int16 vector or matrix) | int16_t * |
uint32Ptr (uint32 vector or matrix) | uint32_t * |
int32Ptr (int32 vector or matrix) | int32_t * |
int64Ptr (uint64 vector or matrix) | int64_t * |
uint64Ptr (uint64 vector or matrix) | uint64_t * |
floatPtr, singlePtr (single vector or matrix) | float * |
doublePtr (double vector or matrix) | double * |
voidPtr | void * |
libpointer | void *, uint8_t *, int8_t *, int16_t *, uint16_t *, ... |
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
cmake
call CMake tool
Syntax
- [status, message] = cmake(varargin)
Input argument
- varargin - command to send to CMake
Output argument
- res - a logical: true if cmake command is successfully
- message - a string: message generated by cmake command.
Description
cmake used internally to generate makefile used to build C/C++ code.
cmake used by dlgeneratemake.
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
configuremingw
Configure Nelson to use MinGW as default C compiler
Syntax
- [res, message] = configuremingw(mingw_path)
Input argument
- mingw_path - a string: mingw root path.
Output argument
- res - a logical: true if MinGW was found
- message - a string: empty if MinGW was found or an error message.
Description
By default, Nelson has no C/C++ compiler defined as default on Windows.
On others platforms, we will suppose that a C/C++ compiler is always available and it is not required to call this function.
On Windows, you need to call once configuremingw if you want to use MinGW as default C compiler.
Example
configuremingw('c:/mingw')
See also
Supported C/C++ compilers, havecompiler, configuremsvc.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
configuremsvc
Configure Nelson to use visual studio as default compiler
Syntax
- [res, message] = configuremsvc()
Output argument
- res - a logical: true if visual studio was found
- message - a string: empty if visual studio was found or an error message.
Description
By default, Nelson has no C/C++ compiler defined as default on Windows.
On others platforms, we will suppose that a C/C++ compiler is always available and it is not required to call this function.
On Windows, you need to call once configuremsvc if you want to use visual studio as default compiler.
After each update of Visual studio, it will be required to call again configuremsvc.
Example
configuremsvc()
See also
Supported C/C++ compilers, havecompiler.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
dlcall
C or Fortran Foreign function call.
Syntax
- [x1, ... , xN] = dlcall(dlsym_handle, arg1, ..., argN)
Input argument
- dlsym_handle - a dlsym handle.
- arg1, ..., argN - input arguments.
Output argument
- [x1, ... , xN] - output values.
Description
dlcall calls an external C or Fortran function loaded from an shared library.
dlcall validates input argument types before calling based on dlsym handle definition.
Examples
lib = dlopen([modulepath('nelson', 'builtin'), '/libnlsDynamic_link', getdynlibext()]);
V = double([1 2;3 4]);
% C prototype:
% int dynlibTestMultiplyDoubleArrayWithReturn(double *x, int size)
f = dlsym(lib, 'dynlibTestMultiplyDoubleArrayWithReturn', 'int32', {'doublePtr', 'int32'});
[r1, r2] = dlcall(f, V, int32(numel(V)))
delete(f);
delete(lib);
Call C getpid function
run([modulepath('dynamic_link'), '/examples/call_c.m']);
Call fortran DASUM (blas) function
run([modulepath('dynamic_link'), '/examples/call_fortran.m']);
See also
dlsym, C/Nelson equivalent data types.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
dlclose
Removes dllib object.
Syntax
- dllib_delete(h)
- delete(h)
- dlclose(h)
Input argument
- h - a handle: an dllib object.
Description
dlclose(h) or delete(h) releases dllib object.
Do not forget to clear h afterward.
Example
path_ref = modulepath('dynamic_link', 'builtin');
lib = dlopen(path_ref)
isvalid(lib)
dlclose(lib); // or delete(lib)
isvalid(lib)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
dlgeneratecleaner
Generates cleaner.m file for C++ gateway.
Syntax
- dlgeneratecleaner(destinationdir)
- dlgeneratecleaner(destinationdir, files)
Input argument
- destinationdir - a string: destination directory where is generated the cleaner.m file.
- files - a string or a cell of string: list of files to delete.
Description
dlgeneratecleaner generates a 'cleaner.m' to remove files.
Example
See module skeleton for example
dlgeneratecleaner(tempdir());
text = fileread([tempdir(), 'cleaner.m'])
See also
dlgenerateunloader, dlgenerategateway.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
dlgenerategateway
Generates C++ gateway.
Syntax
- dlgenerategateway(destinationdir, module_name, builtin_table)
Input argument
- destinationdir - a string: destination directory where is generated the gateway file.
- module_name - a string: module name exposed in Nelson.
- builtin_table - a cell composed of cell with {name exposed in Nelson, nb output arguments, nb input arguments}
Description
dlgenerategateway generates a C++ gateway used by addmodule.
Example
See module skeleton for example
dlgenerategateway(tempdir(), 'module_skeleton', {{'cpp_sum', 1, 2}; {'cpp_sub', 2, 3}});
text = fileread([tempdir(), 'Gateway.cpp'])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
dlgenerateloader
Generates loader.m file for C++ gateway.
Syntax
- dlgenerateloader(destinationdir, libraryname)
Input argument
- destinationdir - a string: destination directory where is generated the loader.m file.
- libraryname - a string or a cell of string: external dynamic library names.
Description
dlgenerateloader generates a 'loader.m' load external dynamic libraries.
Example
See module skeleton for example
dlgenerateloader(tempdir(), {'c_dynamic_library_1', 'c_dynamic_library_2'});
text = fileread([tempdir(), 'loader.m'])
See also
dlgenerateunloader, dlgenerategateway.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
dlgeneratemake
Generates a makefile for building a dynamic library.
Syntax
- [res, message] = dlgeneratemake(destinationdir, libname, c_cpp_files, include)
- [res, message] = dlgeneratemake(destinationdir, libname, c_cpp_files, includes, defines, external_libraries, build_configuration, c_flags, cxx_flags)
- [res, message] = dlgeneratemake(maketype, destinationdir, libname, c_cpp_files, include)
- [res, message] = dlgeneratemake(maketype, destinationdir, libname, c_cpp_files, includes, defines, external_libraries, build_configuration, c_flags, cxx_flags)
Input argument
- maketype - a string: 'executable' or 'dynamic_library'.
- destinationdir - a string: destination directory where is generated the makefile.
- libname - a string: destination dynamic library or executable name.
- c_cpp_files - a string or a cell of strings: .c or .cpp list files (full filename)
- include - a string or a cell of strings: directories where to find include files.
- defines - a string or a cell of strings: a list of defines
- external_libraries - a string or a cell of strings: a list of external libraries to link
- build_configuration - a string: 'Debug' or 'Release'
- c_flags - a string: C flags
- cxx_flags - a string: C flags
Output argument
- res - a logical: true if makefile was generated.
- message - a string: empty if makefile was generated or an error message.
Description
dlgeneratemake generates a makefile adapted to your system environment for building shared libraries.
Thanks to CMake to help Nelson in this task.
Example
See module skeleton for example
[status, message] = dlgeneratemake(currentpath, ...
'module_skeleton', ...
{[currentpath, '/cpp/cpp_sumBuiltin.cpp'], [currentpath, '/cpp/Gateway.cpp']}, ...
[{[currentpath, '/include']; [currentpath, '/../src/include']}; dlgetnelsonincludes()], ...
[], ...
[dlgetnelsonlibraries(); [currentpath, '/../src/business_code']]);
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
dlgenerateunloader
Generates unloader.m file for C++ gateway.
Syntax
- dlgenerateunloader(destinationdir, libraryname)
Input argument
- destinationdir - a string: destination directory where is generated the unloader.m file.
- libraryname - a string or a cell of string: external dynamic library names.
Description
dlgenerateunloader generates a 'unloader.m' unload external dynamic libraries.
Example
See module skeleton for example
dlgenerateunloader(tempdir(), {'c_dynamic_library_1', 'c_dynamic_library_2'});
text = fileread([tempdir(), 'unloader.m'])
See also
dlgenerateloader, dlgenerategateway.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
dlgetnelsonincludes
Returns paths of Nelson include directories.
Syntax
- C = dlgetnelsonincludes()
Output argument
- C - a cell array of paths to various include directories used by Nelson modules
Description
C = dlgetnelsonincludes() returns a cell array of paths to various include directories used by Nelson modules.
These paths are used internally for module development and building processes.
Example
See module skeleton for example
dlgetnelsonincludes()
See also
dlgetnelsonlibraries, dlgeneratemake.
History
Version | Description |
---|---|
1.10.0 | initial version |
Author
Allan CORNET
dlgetnelsonlibraries
Returns paths to Nelson library files.
Syntax
- C = dlgetnelsonlibraries()
Output argument
- C - a cell array of paths to various library directories used by Nelson modules
Description
C = dlgetnelsonlibraries() returns a cell array of paths to various library directories used by Nelson modules.
These paths are used internally for module development and building processes.
Example
See module skeleton for example
dlgetnelsonlibraries()
See also
dlgetnelsonincludes, dlgeneratemake.
History
Version | Description |
---|---|
1.10.0 | initial version |
Author
Allan CORNET
dllib_used
Returns list of current used dllib handle.
Syntax
- r = dllib_used()
Output argument
- h - a vector of dllib handle.
Description
Returns list of current used dllib handle.
See also
Example
dllib_used(),delete(dllib_used())
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
dllibinfo
Returns list of available symbols in an shared library.
Syntax
- c = dllibinfo(lib)
Input argument
- lib - a dllib handle: library already loaded.
Output argument
- c - a cell of strings.
Description
dllibinfo returns list of available symbols in an shared library.
Example
lib = dlopen(modulepath('dynamic_link', 'builtin'))
c = dllibinfo(lib)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
dllibisloaded
Checks if shared library is loaded.
Syntax
- tf = dllibisloaded(libraryname)
- [tf, lib] = dllibisloaded(libraryname)
Input argument
- libraryname - a string: dynamic library name.
Output argument
- tf - a logical: true if library is already loaded.
- lib - a dllib handle: library already loaded.
Description
dllibisloaded returns if share library is already loaded.
Example
path_1 = modulepath('dynamic_link', 'builtin');
r = dllibisloaded(path_1)
lib1 = dlopen(path_1);
[r, lib2] = dllibisloaded(path_1)
isequal(lib1, lib2)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
dlmake
call make or nmake tool
Syntax
- [res, message] = dlmake(destinationdir)
- [res, message] = dlgeneratemake(destinationdir, libname, c_cpp_files, includes, defines, external_libraries, build_configuration, c_flags, cxx_flags)
Input argument
- destinationdir - a string: destination directory where is the makefile to call.
Output argument
- res - a logical: true if makefile execution was successfully.
- message - a string: empty if makefile execution was successfully or an error message.
Description
dlmake used to provide an multiplatform way to build C/C++.
Example
basic example to call dlmake
dest = [tempdir(), 'dlmake_help'];
mkdir(dest);
txt = 'MESSAGE( STATUS "Hello world !")';
filewrite([dest, '/CMakeLists.txt'], txt);
[status, message] = dlmake(dest)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
dlopen
Loads an dynamic library.
Syntax
- lib = dlopen(libraryname)
Input argument
- libraryname - a string: dynamic library name.
Output argument
- lib - a dllib handle.
Description
dlopen loads an dynamic library.
dlopen returns a dllib handle with Path property.
get, ismethod, isprop, disp, delete, isvalid, used, eq, ne, isequal, horzcat, vertcat are overloaded for dllib type.
library is searched first in NELSON_LIBRARY_PATH and after in PATH on windows or LD_LIBRARY_PATH or DYLD_LIBRARY_PATH on linux or Macos.
NELSON_LIBRARY_PATH can modified with setenv.
Example
path_1 = modulepath('dynamic_link', 'builtin');
lib1 = dlopen(path_1)
isvalid(lib1)
dlclose(lib1)
isvalid(lib1)
clear lib1
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
dlsym
Loads a C/Fortran symbol for an dynamic library.
Syntax
- f = dlsym(lib, symbol_name, return_type, params_types)
Input argument
- lib - a dllib handle.
- symbolname - a string: symbol to load.
- return_type - a string: return type of the C/Fortran function.
- params_types - a cell of strings: arguments using a special syntax with differents data types.
Output argument
- f - a dlsym handle.
Description
dlsym retrieves the address of an exported function as an dlsym handle.
if symbolname not found, nelson try to find symbol equivalent based on these rules and in this order:
_symbolname
symbolname
symbolname_
_symbolname_
_SYMBOLNAME
SYMBOLNAME
SYMBOLNAME_
_SYMBOLNAME_
symbol name used is available in prototype field of the returned handle.
If multiple symbol names found, an error is raised with possible names.
Warning: Uses wrong datatype definitions a foreign function can terminate unexpectedly.
Examples
lib = dlopen(modulepath('dynamic_link', 'builtin'));
V = double([1 2;3 4]);
% C prototype:
% int dynlibTestMultiplyDoubleArrayWithReturn(double *x, int size)
f = dlsym(lib, 'dynlibTestMultiplyDoubleArrayWithReturn', 'int32', {'doublePtr', 'int32'});
[r1, r2] = dlcall(f, V, int32(numel(V)))
delete(f);
delete(lib);
Call C getpid function
run([modulepath('dynamic_link'), '/examples/call_c.m']);
Call fortran DASUM (blas) function
run([modulepath('dynamic_link'), '/examples/call_fortran.m']);
See also
dlcall, C/Nelson equivalent data types.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
dlsym_delete
Removes dlsym object.
Syntax
- dlsym_delete(h)
- delete(h)
Input argument
- h - a handle: an dlsym object.
Description
delete(h) releases dlsym object.
Do not forget to clear h afterward.
Example
dlsym_used(),delete(dlsym_used())
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
dlsym_used
Returns list of current used dlsym handle.
Syntax
- r = dlsym_used()
Output argument
- h - a vector of dlsym handle.
Description
Returns list of current used dlsym handle.
See also
Example
dlsym_used(),delete(dlsym_used())
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
findcmake
find CMake path.
Syntax
- [status, cmake_path] = findcmake()
Output argument
- status - a logical.
- cmake_path - a string: path of CMake or ''.
Description
find CMake path.
CMake is used internaly to generate makefiles used to build dynamic libraries on fly.
See also
Example
[status, cmake_path] = findcmake()
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
getdynlibext
Returns the extension of dynamic libraries.
Syntax
- ext = getdynlibext()
Output argument
- ext - a string: dynamic library extension
Description
getdynlibext() returns the extension of dynamic libraries.
Example
getdynlibext()
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
havecompiler
Detect if a C/C++ compiler is configured.
Syntax
- [status, compiler] = havecompiler()
Output argument
- status - a logical.
- compiler - a string: 'msvc', 'mingw', 'unix' or ''.
Description
havecompiler detects if C/C++ compiler is configured for Nelson.
On Unix platforms (linux, MacOs), havecompiler returns always true as status and unix as compiler.
Example
[status, message] = havecompiler()
See also
configuremsvc, configuremingw.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
libpointer
Creates an C pointer object usuable in Nelson.
Syntax
- ptr = libpointer()
- ptr = libpointer(datatype)
- ptr = libpointer(datatype, value)
Input argument
- datatype - a string: data type.
- value - a nelson variable compatible with datatype.
Output argument
- ptr - a libpointer handle.
Description
This is an advanced feature to manipulate C pointers.
ptr = libpointer() creates an NULL pointer.
Examples
p = libpointer('int8Ptr', int8([3 4]));
p.isNull()
p.DataType
p.Value
NLSDYNAMIC_LINK_IMPEXP double *multiplicationDoubleByReference(double *x)
{
*x *= 2;
return x;
}
x = 133.3;
xPtr = libpointer('doublePtr', x);
path_ref = modulepath('dynamic_link', 'builtin');
lib = dlopen(path_ref);
f = dlsym(lib, 'multiplicationDoubleByReference', 'libpointer', {'doublePtr'});
[r1, r2] = dlcall(f, xPtr);
r2
% r1 is an libpointer of type '' (voidPointer) and it need to be change type and size.
r1.setdatatype('doublePtr');
r1.reshape(1, 1);
get(r1)
See also
C/Nelson equivalent data types, isNull, libpointer.reshape, libpointer.setdatatype.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
libpointer_delete
Removes libpointer object.
Syntax
- libpointer_delete(h)
- delete(h)
Input argument
- h - a handle: an libpointer object.
Description
delete(h) releases libpointer object.
Do not forget to clear h afterward.
Example
libpointer_used(),delete(libpointer_used())
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
libpointer_isNull
Checks if libpointer handle points on NULL pointer.
Syntax
- tf = isNull(h)
- tf = h.isNull()
Input argument
- h - a libpointer handle.
Output argument
- tf - a logical.
Description
Checks if libpointer handle points on NULL pointer.
See also
Example
p = libpointer('int8Ptr', int8([3 4]));
p.isNull()
p2 = libpointer()
p2.isNull()
isNull(p2)
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
libpointer_plus
plus operator on libpointer handle.
Syntax
- h2 = h.plus(offset)
- h2 = h + offset
Input argument
- h - a libpointer handle.
- offset - a integer value: increment.
Description
plus operator on libpointer handle.
ouptut libpointer is valid only as long as the original input libpointer exists.
See also
Example
x = [1 2 3 4 5];
xPtr = libpointer('doublePtr', x);
y = xPtr + 2;
y.reshape(1, 3);
y.Value
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
libpointer_reshape
Reshapes libpointer dimensions.
Syntax
- tf = h.reshape(X, Y)
Input argument
- h - a libpointer handle.
- X - a scalar double: new X dimension.
- Y - a scalar double: new Y dimension.
Description
Set dimensions from libpointer object.
See also
Example
a = libpointer('doublePtr', eye(2, 2));
a.reshape(3, 3);
a.Value
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
libpointer_setdatatype
Set type of an libpointer handle.
Syntax
- h.setdatatype(datatype)
Input argument
- h - a libpointer handle.
- datatype - a string: new datatype.
Description
Set data type from libpointer object.
See also
libpointer, C/Nelson equivalent data types.
Example
a = libpointer();
a.isNull()
a.setdatatype('doublePtr');
a.reshape(1, 1)
a.Value
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
libpointer_used
Returns list of current used libpointer handle.
Syntax
- r = libpointer_used()
Output argument
- h - a vector of libpointer handle.
Description
Returns list of current used libpointer handle.
See also
Example
libpointer_used(),delete(libpointer_used())
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
loadcompilerconf
load compiler configuration.
Syntax
- res = loadcompilerconf()
- [res, compiler] = loadcompilerconf()
Output argument
- res - a logical
- compiler - a string: 'msvc', 'mingw', 'unix' or ''
Description
loadcompilerconf returns true if compiler was previously configured with configuremsvc or configuremingw.
loadcompilerconf returns always false on others platforms and 'unix' as compiler.
loadcompilerconf is called at Nelson's startup.
See also
removecompilerconf, configuremingw, configuremingw.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
removecompilerconf
Remove used compiler configuration (on Windows).
Syntax
- res = removecompilerconf()
Output argument
- res - a logical
Description
removecompilerconf returns true if compiler was previously configured with configuremsvc or configuremingw.
removecompilerconf returns always true on others platforms.
See also
configuremsvc, configuremingw.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
vswhere
Locate Visual Studio 2017, 2019 and newer installations
Syntax
- res = vswhere()
Output argument
- res - a struct with information about Visual studio
Description
vswhere allows to find easily Visual studio.
vswhere is currently only implemented on Windows platform.
Bibliography
https://github.com/Microsoft/vswhere
Example
vswhere()
See also
havecompiler, Supported C/C++ compilers.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
MEX functions
MEX functions
Description
MEX C API is allow to access Nelson, GNU Octave and commercial software functions and extend it's functionality.
- dlgeneratemexgateway - Generates C MEX gateway (internal function).
- engClose - Close Nelson engine session
- engEvalString - Evaluate expression in string in base scope
- engGetVariable - Copy variable from Nelson engine workspace
- engGetVisible - Determine visibility of Nelson engine session
- engOpen - Start Nelson process
- engOpenSingleUse - Start Nelson engine session for single and nonshared use.
- engOutputBuffer - Specify char buffer for Nelson output
- engPutVariable - Put variable into Nelson engine workspace
- engSetVisible - Show or hide Nelson engine session
- mex - Build MEX function
- mexAtExit - Register a function to be called when the MEX-file is cleared or when Nelson exits
- mexCallMATLAB - Call a NELSON function
- mexCallMATLABWithTrap - Call a NELSON function and capture error.
- mexext - Binary MEX file-name extension
dlgeneratemexgateway
Generates C MEX gateway (internal function).
Syntax
- dlgeneratemexgateway(destinationdir, function_name)
Input argument
- destinationdir - a string: destination directory where is generated the gateway file.
- function_name - a string: function name exposed in Nelson.
- interleavedcomplex - a logical: use interleaved complex representation.
Description
dlgeneratemexgateway generates a C MEX gateway used by mex (internal function).
See also
mex.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
engClose
Close Nelson engine session
Syntax
- #include "engine.h"
- int engClose(Engine *ep);
Input argument
- Engine *ep - handle to Nelson engine.
Output argument
- int - 0 on success and 1 on failure.
Description
engClose closes engine session and terminates the connection.
Example
edit([modulepath('mex', 'tests'), '/test_engine.c'])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
engEvalString
Evaluate expression in string in base scope
Syntax
- #include "engine.h"
- int engEvalString(Engine *ep, const char *string);
Input argument
- Engine *ep - handle to Nelson engine.
- const char *string - Expression to evaluate.
Output argument
- int - returns 1 if the engine session is closed or invalid. Otherwise, returns 0.
Description
Evaluate expression in string in base scope.
Example
edit([modulepath('mex'), '/examples/mex_engine_demo_2.c'])
See also
mex, engPutVariable, engGetVariable.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
engGetVariable
Copy variable from Nelson engine workspace
Syntax
- #include "engine.h"
- mxArray *engGetVariable(Engine *ep, const char *name);
Input argument
- Engine *ep - handle to Nelson engine.
- const char *name - name of mxArray in the Nelson workspace (base scope).
Output argument
- mxArray * - Pointer to an allocated mxArray structure. Do not forget to free.
Description
Copy variable from Nelson engine workspace.
The limit for the size of data transferred is 2048 MB.
Example
edit([modulepath('mex', 'tests'), '/test_engine.c'])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
engGetVisible
Determine visibility of Nelson engine session
Syntax
- #include "engine.h"
- int engGetVisible(Engine *ep, bool *value);
Input argument
- Engine *ep - handle to Nelson engine.
Output argument
- int - 0 if successful or 1 if an error occurs.
- bool * - true (visible) or false (minimize).
Description
Determine visibility of Nelson engine session
Example
edit([modulepath('mex', 'tests'), '/test_engine.c'])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
engOpen
Start Nelson process
Syntax
- #include "engine.h"
- Engine *engOpen(const char *startcmd);
Input argument
- startcmd - Nelson startup command (NULL).
Output argument
- Engine - handle to Nelson engine or NULL.
Description
engOpen starts a Nelson process for using Nelson as a computational engine.
Libraries path need to contain nelson path to find Nelson's libraries at runtime.
Set the value to the path returned by the following Nelson command:
res = modulepath('nelson', 'builtin')
on linux: export LD_LIBRARY_PATH=$LD_LIBRARY_PATH:res
export PATH=$PATH:res
on macos: export DYLIB_LIBRARY_PATH=$DYLIB_LIBRARY_PATH:res
export PATH=$PATH:res
on windows: set PATH=%PATH%;res
Example
edit([modulepath('mex', 'tests'), '/test_engine.c'])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
engOpenSingleUse
Start Nelson engine session for single and nonshared use.
Syntax
- #include "engine.h"
- Engine *engOpenSingleUse(const char *startcmd, void *dcom, int *retstatus);
Input argument
- startcmd - Nelson startup command (NULL).
- dcom - must be NULL.
Output argument
- Engine - handle to Nelson engine or NULL.
- retstatus - status; possible cause of failure.
Description
engOpenSingleUse start Nelson engine session for single and nonshared use.
Example
edit([modulepath('mex', 'tests'), '/test_engine.c'])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
engOutputBuffer
Specify char buffer for Nelson output
Syntax
- #include "engine.h"
- int engOutputBuffer(Engine *ep, char *p, int n);
Input argument
- Engine *ep - handle to Nelson engine.
- char *p - Pointer to character buffer.
- int n - Length of buffer.
Output argument
- int - returns 1 if the engine session is closed or invalid. Otherwise, returns 0.
Description
Specify char buffer for Nelson output.
To turn off output buffering in C, use: engOutputBuffer(ep, NULL, 0);
Example
edit([modulepath('mex'), '/examples/mex_engine_demo_2.c'])
See also
mex, engPutVariable, engGetVariable.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
engPutVariable
Put variable into Nelson engine workspace
Syntax
- #include "engine.h"
- int engPutVariable(Engine *ep, const char *name, const mxArray *pm);
Input argument
- Engine *ep - handle to Nelson engine.
- const char *name - name of mxArray in the Nelson workspace (base scope).
- const mxArray *pm - Pointer to mxArray.
Output argument
- int - 0 if successful or 1 if an error occurs.
Description
Put variable into Nelson engine workspace.
Example
edit([modulepath('mex', 'tests'), '/test_engine.c'])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
engSetVisible
Show or hide Nelson engine session
Syntax
- #include "engine.h"
- int engSetVisible(Engine *ep, bool value);
Input argument
- Engine *ep - handle to Nelson engine.
- bool value - set value to 1 to make the engine window visible, or to 0 to make it invisible.
Output argument
- int - 0 if successful or 1 if an error occurs.
Description
Show or hide Nelson engine session
Example
edit([modulepath('mex', 'tests'), '/test_engine.c'])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
mex
Build MEX function
Syntax
- mex(filenames)
- mex(filenames, option1, ..., optionN)
- mex(api, filenames)
- mex(api, filenames, option1, ..., optionN)
- mex('-output', mexName, filenames)
- mex(api, '-output', mexName, filenames)
- mex(api, '-output', mexName, filenames, option1, ..., optionN)
- mex('-client, 'engine', filenames)
- mex('-client', 'engine', 'filenames', api, option1, ..., optionN)
Input argument
- '-client', 'engine' - enable to build C/C++ source files into standalone engine application.
- api - a string: '-R2017b' (separated complex representation) or '-R2018a' (interleaved complex representation).
- filenames - a string or cell of characters: list of files to use. First filename used as mex name.
- mexName - a string: override naming convention.
- option1, ..., optionN - string: compilation or link option.
Description
To use mex, C/C++ compiler must be available and configured. See Supported C/C++ compilers section for more information.
Nelson includes an interface to allow legacy mex-files to be compiled and linked with Nelson.
A mex file is a type of computer file that provides an interface between Octave or the reference commercial software and functions written in C, C++.
Nelson have also his own C++ API to manage more easily internal nelson's objects.
PREDEFINED C MACRO:
MX_IS_NELSON macro is defined to easily detect if Nelson is used in C code.
MX_HAS_INTERLEAVED_COMPLEX macro is defined if C MEX API used is '-R2018a'.
Supported options: compilation or link.
CFLAGS=
-D The -D option defines C preprocessor macro.
-U The -U option undefines C preprocessor macro
-I Adds pathname to the list of folders to search for #include files.
-l Links with dynamic object library .lib, .so or .dylib.
-g Used for debugging (Debug configuration).
Example
edit([modulepath('mex', 'tests'), '/test_engine.m'])
See also
Supported C/C++ compilers, dlgenerategateway.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
mexAtExit
Register a function to be called when the MEX-file is cleared or when Nelson exits
Syntax
- #include "mex.h"
- int mexAtExit(void (*ExitFcn)(void));
Input argument
- ExitFcn - Pointer to function you wish to run on exit.
Output argument
- returned value - returns 0.
Description
Each MEX can register only one active exit subroutine at a time.
mexAtExit registers a subroutine to be called just when Nelson is finished or clear is called.
Example
edit([modulepath('mex', 'tests'), '/test_mexAtExit.m'])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
mexCallMATLAB
Call a NELSON function
Syntax
- #include "mex.h"
- int mexCallMATLAB(int nlhs, mxArray *plhs[], int nrhs, mxArray *prhs[], const char *command_name);
Input argument
- nlhs - number of desired output arguments.
- plhs - pointer to an array of mxArray (output).
- nrhs - number of desired input arguments.
- prhs - pointer to an array of mxArray (input).
- command_name - character string containing the name of the Nelson function called.
Output argument
- returned value - 0 if successful, and a nonzero value if unsuccessful.
Description
mexCallMATLAB calls an NELSON function.
If name detects an error, NELSON will terminate MEX and returns control to NELSON.
Example
edit([modulepath('mex', 'tests'), '/test_mexCallMATLAB.m'])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
mexCallMATLABWithTrap
Call a NELSON function and capture error.
Syntax
- #include "mex.h"
- mxArray *mexCallMATLABWithTrap(int nlhs, mxArray *plhs[], int nrhs, mxArray *prhs[], const char *functionName);
Input argument
- nlhs - number of desired output arguments.
- plhs - pointer to an array of mxArray (output).
- nrhs - number of desired input arguments.
- prhs - pointer to an array of mxArray (input).
- command_name - character string containing the name of the Nelson function called.
Output argument
- returned value - NULL if no error occurred; otherwise, a pointer to an mxArray (MException object).
Description
mexCallMATLABWithTrap calls an NELSON function and capture error.
If name detects an error, mexCallMATLABWithTrap returns an mxArray (MException object).
Example
edit([modulepath('mex', 'tests'), '/test_mexCallMATLABWithTrap.m'])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
mexext
Binary MEX file-name extension
Syntax
- ext = mexext()
- extlist = mexext('all')
Description
ext = mexext() returns the filename extension for the current platform.
extlist = mexext('all') returns the extensions for all platforms.
A mex file is a type of computer file that provides an interface between Octave or the reference commercial software and functions written in C, C++.
Nelson have also his own C++ API to manage more easily internal nelson's objects.
Nelson cannot load mex generated by others software, BUT you can easily rebuild it for each software target.
Mex generated by Nelson have a file extension beginning by .nex
Example
ext = mexext()
extlist = mexext('all')
See also
mex.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
Fortran to C
Fortran to C
Description
Converts Fortran 77 to C code
- f2c - Fortran to C converter.
f2c
Fortran to C converter.
Syntax
- f2c(src, dest)
- r = f2c(src, dest)
- [r, msg] = f2c(src, dest)
Input argument
- src - a string: fortran source file.
- dest - a string: destination directory.
Output argument
- r - a logical: true if success.
- msg - a string: error message or ''.
Description
f2c converts fortran 66, and fortran 77 files to C.
Example
f2c([modulepath(nelsonroot(),'f2c','root'), '/tests/dgemm.f'], tempdir());
fileread([tempdir(), 'dgemm.c'])
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
NIG
NIG
Description
Nelson Interface Generator
- nig - Nelson tool to interface C or Fortran functions with Nelson.
nig
Nelson tool to interface C or Fortran functions with Nelson.
Syntax
- nig(NIG_FUNCTIONS, DESTINATION_DIR)
Input argument
- NIG_FUNCTIONS - structure : functions definition
- DESTINATION_DIR - string: a valid path (destination)
Description
With nig, you can wrap your C/FORTRAN code into a same set, called an gateway, and use them in Nelson as Nelson builtin.
Examples
NIG_FUNCTION description
% Nelson Interface Generator (NIG) example
NIG_FUNCTION = struct();
NIG_FUNCTION.NELSON_NAME = 'example_nig_sum';
NIG_FUNCTION.NELSON_NAMESPACE = 'Example'; % optional
NIG_FUNCTION.MODULE_NAME = 'example';
NIG_FUNCTION.SYMBOL = 'sum';
NIG_FUNCTION.LANGUAGE = 'fortran';
NIG_FUNCTION.VARIABLES = struct([]);
IDX = length(NIG_FUNCTION.VARIABLES) + 1;
NIG_FUNCTION.VARIABLES(IDX).NAME = 'A';
NIG_FUNCTION.VARIABLES(IDX).TYPE = 'integer';
NIG_FUNCTION.VARIABLES(IDX).MODE = 'input';
IDX = length(NIG_FUNCTION.VARIABLES) + 1;
NIG_FUNCTION.VARIABLES(IDX).NAME = 'B';
NIG_FUNCTION.VARIABLES(IDX).TYPE = 'integer';
NIG_FUNCTION.VARIABLES(IDX).MODE = 'input';
IDX = length(NIG_FUNCTION.VARIABLES) + 1;
NIG_FUNCTION.VARIABLES(IDX).NAME = 'OUTPUT';
NIG_FUNCTION.VARIABLES(IDX).TYPE = 'integer';
NIG_FUNCTION.VARIABLES(IDX).MODE = 'output';
nig(NIG_FUNCTION, tempdir())
fileread([tempdir(),'/Gateway.cpp'])
fileread([tempdir(),'/example_nig_sumBuiltin.hpp'])
fileread([tempdir(),'/example_nig_sumBuiltin.cpp'])]
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
Web tools
Web tools
Description
Transferring data with URLs
- checkupdate - Check update for Nelson's application
- repo - Git repository tool for Nelson
- urlencode - Replace special characters in URLs with escape characters.
- weboptions - Specify parameters for RESTful web service
- webread - Read data from RESTful web service to Nelson's variable
- websave - Save data from RESTful web service to file
- webwrite - Write data to RESTful web service
checkupdate
Check update for Nelson's application
Syntax
- checkupdate()
- checkupdate('url', http_url_to_check)
- checkupdate('forcenogui', true_or_false)
- checkupdate('url', http_url_to_check, 'forcenogui', true_or_false)
- checkupdate('forcenogui', true_or_false)
- [res, msg, url_new_version] = checkupdate(...)
Input argument
- http_url_to_check - a string: URL to check the latest Nelson's application version.
- true_or_false - a logical: true (force CLI), false (detect default mode).
Output argument
- res - a logical: result of the update check.
- msg - a string: message providing information about the update check.
- url_new_version - a string: URL to download the new version if available.
Description
checkupdate checks if a new version of Nelson is available and opens a URL to download it.
This function is primarily used through the menu action available in the main window's help section.
Example
checkupdate
See also
History
Version | Description |
---|---|
1.2.0 | Initial version |
Author
Allan CORNET
repo
Git repository tool for Nelson
Syntax
- repo('clone', url, branch, destination)
- repo('clone', url, destination)
- repo('clone', url, branch, destination, username, password)
- repo('clone', url, destination, username, password)
- repo('export', url, branch_tag_sha1, destination)
- repo('export', url, destination)
- repo('export', url, branch_tag_sha1, destination, username, password)
- repo('export', url, destination, username, password)
- repo('checkout', destination, branch_tag_sha1)
- ce = repo('branch', destination)
- ce = repo('tag', destination)
- st = repo('log', destination)
- repo('fetch', destination)
- repo('fetch', destination, username, password)
- repo('remove_branch', destination, branch)
- current_branch = repo('current_branch', destination)
Input argument
- url - a string: URL to a git repository.
- branch - a string: branch name.
- destination - a string: local pathname.
- branch_tag_sha1 - a string: a branch name, tag or sha1.
- username - a string: username used if an authentification is required.
- password - a string: password used if an authentification is required.
Output argument
- ce - a cell: list of tags or branchs.
- st - a structure: contains log information.
- current_branch - a string: name of current branch.
Description
repo() allows to clone, checkout, fetch a git repository.
checkout command will be forced and remove untracked filed.
git https protocol works on all platforms. git ssh protocol works currently on macos and linux platforms.
report('export', ...) clone and remove .git directory.
Tips:
If you have this error: callback returned unsupported credentials type , checks your ~/.gitconfig file.
You don't have some ssh or https redirection.
Remove entries:
[url "git@github.com:"]
insteadOf = https://github.com/
Used function(s)
libgit2 (https://libgit2.org/)
Example
url = 'https://github.com/nelson-lang/module_skeleton.git';
destination = [tempdir(), 'demo_repo'];
if isdir(destination)
rmdir(destination, 's');
end
mkdir(destination);
repo('clone', url, destination)
repo('tag', destination)
repo('branch', destination)
repo('current_branch', destination)
repo('log', destination)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
urlencode
Replace special characters in URLs with escape characters.
Syntax
- new_url = webread(url)
Input argument
- url - a string: URL to a web service.
Output argument
- new_url - a string: encoded url.
Description
urlencode replaces special characters in URLs with escape characters.
Special characters in URLs need to be replaced with escape characters. For example, spaces should be replaced with '%20'.
Example
url = 'https://httpbin.org/get?query=hello world';
res = urlencode(url)
See also
History
Version | Description |
---|---|
1.11.0 | initial version |
Author
Allan CORNET
weboptions
Specify parameters for RESTful web service
Syntax
- options = weboptions()
- options = weboptions(name, value)
Input argument
- name - a string.
- value - a variable: value corresponding to name field.
Output argument
- options - a weboptions object.
Description
options = weboptions() returns default weboptions object.
weboptions object can be an optional input argument to the webread, websave, and webwrite builtin.
Name-Value Pair Arguments:
UserAgent User agent identification: a string or character vector.
Timeout Time out connection duration: positive numeric scalar or Inf value.
Username User identifier: a string or character vector.
Password User authentication password: a string or character vector.
KeyName Name of key: a string or character vector.
KeyValue Value of key: a string scalar, character vector, numeric or logical.
HeaderFields Names and values of header fields: m-by-2 array of strings or cell array of character vectors
ContentType Content type: a string scalar or character vector.
supported value: 'auto', 'text', 'audio', 'binary', 'json', 'raw'
ContentReader Content reader: an function handle.
MediaType Media type: a string or character vector.
supported value: 'auto', 'application/x-www-form-urlencoded'
RequestMethod HTTP request method: a string or character vector.
supported value: 'auto', 'get', 'post', 'put', 'delete', 'patch'
ArrayFormat: 'csv' (default), 'json', 'repeating' or 'php'
CertificateFilename Filename of root certificates: a string or character vector.
FollowLocation tells the library to follow any Location: header redirect that an HTTP server sends in a 30x response: a logical, false by default.
Example
weboptions()
options = weboptions('UserAgent', 'http://www.whoishostingthis.com/tools/user-agent/')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
1.6.0 | 'FollowLocation' option added |
Author
Allan CORNET
webread
Read data from RESTful web service to Nelson's variable
Syntax
- var = webread(url)
- var = webread(url, name1, value1, ... , nameN, valueN)
- var = webread(url, name1, value1, ... , nameN, valueN, options)
Input argument
- url - a string: URL to a web service.
- name1, value1, ... , nameN, valueN - Name-Value Pair Arguments.
- options - a weboptions object.
Output argument
- var - a variable: content from web.
Description
webread() reads content from the web to nelson's variable.
Examples
url = 'https://httpbin.org/get';
res = webread(url,weboptions('ContentType','json'));
More demos
edit([modulepath('webtools'),'/examples/webread_demo_1.m'])
Use function_handle with weboptions and webread
edit([modulepath('webtools'),'/examples/webread_demo_2.m'])
Read data from National Agricultural Statistics Service
edit([modulepath('webtools'),'/examples/webread_demo_3.m'])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
websave
Save data from RESTful web service to file
Syntax
- result_filename = websave(filename, url)
- result_filename = websave(filename, url, name1, value1, ... , nameN, valueN)
- result_filename = websave(filename, url, name1, value1, ... , nameN, valueN, options)
Input argument
- filename - a string: name of file to save content to.
- url - a string: URL to a web service.
- name1, value1, ... , nameN, valueN - Name-Value Pair Arguments.
- options - a weboptions object.
Output argument
- result_filename - a string: full filename path.
Description
websave() saves content from the web to filename.
websave function returns the full filename path as result_filename.
Example
url ='https://httpbin.org/get';
filename = [tempdir(), 'test.txt'];
destination_filename = websave(filename, url, weboptions('ContentType','json'));
txt = fileread(filename)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
webwrite
Write data to RESTful web service
Syntax
- response = webwrite(url, data)
- response = webwrite(url, data, options)
- response = webwrite(url, name1, value1, ... , nameN, valueN)
- response = webwrite(url, name1, value1, ... , nameN, valueN, options)
Input argument
- url - a string: URL to a web service.
- data - Data to post to a web service, an nelson's variable.
- name1, value1, ... , nameN, valueN - Name-Value Pair Arguments, Data to post to a web service.
- options - a weboptions object.
Output argument
- response - a variable: Response from a web service.
Description
webwrite write data to RESTful web service.
Examples
Send message to Slack
[Y, M, D, H, MN, S] = datevec(now);
datetime = sprintf('%d/%d/%d %d:%d:%d', Y, M, D, H, MN, S);
% hide url to slack
url = char([104 116 116 112 115 58 47 47 104 111 111 107 115 46 115 108 97 99 107 46 99 111 109 47 115 101 114 118 105 99 101 115 47 84 77 82 71 56 82 72 68 50 47 66 77 83 48 76 72 65 65 67 47 81 54 52 97 52 49 84 83 76 104 105 78 71 81 108 100 51 115 76 50 86 109 74 71]);
data = struct('text', ['hello from Nelson ', datetime], 'channel', '#test_webwrite');
R = webwrite(url, data);
Connect to your NetAtmo Weather station (oAuth2 connection)
USER_NAME = 'your username';
PASSWORD = 'your password';
CLIENT_ID = 'your client id';
CLIENT_SECRET = 'your client secret';
DEVICE_ID = 'your device id';
url = 'https://api.netatmo.com/oauth2/token';
args = {'grant_type', 'password', 'username', USER_NAME, 'password', PASSWORD, 'client_id', CLIENT_ID, 'client_secret', CLIENT_SECRET};
response = webwrite(url, args{:});
r = webread('https://api.netatmo.com/api/getstationsdata', 'access_token', response.access_token, 'device_id', DEVICE_ID);
disp('Devices')
disp(r.body.devices)
disp('Location Info')
disp(r.body.devices.place)
disp('Last values')
disp(r.body.devices.dashboard_data)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
Geometry
Geometry
Description
Functions about Geometry.
- rotx - 3x3 transformation matrix for rotations around x-axis
- roty - 3x3 transformation matrix for rotations around y-axis
- rotz - 3x3 transformation matrix for rotations around z-axis
rotx
3x3 transformation matrix for rotations around x-axis
Syntax
- rm = rotx(angle)
Input argument
- angle - angle in degree: scalar value.
Output argument
- rm - 3x3 transformation matrix: real-valued orthogonal matrix.
Description
rotx returns 3x3 transformation matrix for rotations around x-axis.
Bibliography
Goldstein, H., C. Poole and J. Safko, Classical Mechanics, 3rd Edition, San Francisco: Addison Wesley, 2002, pp. 142–144.
Example
r = rotx(90)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
roty
3x3 transformation matrix for rotations around y-axis
Syntax
- rm = roty(angle)
Input argument
- angle - angle in degree: scalar value.
Output argument
- rm - 3x3 transformation matrix: real-valued orthogonal matrix.
Description
roty returns 3x3 transformation matrix for rotations around y-axis.
Bibliography
Goldstein, H., C. Poole and J. Safko, Classical Mechanics, 3rd Edition, San Francisco: Addison Wesley, 2002, pp. 142–144.
Example
r = roty(90)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
rotz
3x3 transformation matrix for rotations around z-axis
Syntax
- rm = rotz(angle)
Input argument
- angle - angle in degree: scalar value.
Output argument
- rm - 3x3 transformation matrix: real-valued orthogonal matrix.
Description
rotz returns 3x3 transformation matrix for rotations around z-axis.
Bibliography
Goldstein, H., C. Poole and J. Safko, Classical Mechanics, 3rd Edition, San Francisco: Addison Wesley, 2002, pp. 142–144.
Example
r = rotz(90)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
COM engine
COM engine
Description
Component Object Model (COM) client interface: binary-interface standard for software components on Windows.
- COM examples - Some example to show how to use COM interface.
- COM_delete - Removes COM control or server.
- COM_fieldnames - Returns the properties name of an COM object.
- COM_get - Get property value from COM interface.
- COM_invoke - Invoke method on COM object or interface.
- COM_ismethod - Determines if input is an existing COM object method.
- COM_isprop - Determines if input is an existing COM object property.
- COM_methods - Returns the methods name of an COM object.
- COM_range - Private function: range helper.
- COM_set - Set object or interface property to specified value.
- COM_used - Returns list of current used COM handle.
- COM_xlsfinfo - Determines if file contains Microsoft Excel spreadsheet.
- COM_xlsread - Read Microsoft Excel spreadsheet file using COM.
- COM_xlswrite - Write Microsoft Excel spreadsheet file using COM.
- actxGetRunningServer - Handle to running instance of Automation server.
- actxcontrollist - Get available ActiveX controls.
- actxserver - Creates COM server.
- actxserverlist - Get available ActiveX servers.
- iscom - Determine whether input is COM or ActiveX object.
COM examples
Some example to show how to use COM interface.
Description
See [modulepath('com_engine'), '/examples'] directory.
Interacts with Internet explorer, Word, Excel, Speech voice, VB scripts ...
See also
actxserver, set, get, invoke.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
COM_delete
Removes COM control or server.
Syntax
- COM_delete(h)
- delete(h)
Input argument
- h - a handle: a COM object.
Description
delete(h) releases all the interfaces derived from the specified COM server or control, and then deletes the server or control itself.
This is different from releasing an interface, which releases and invalidates only the particular interface.
Do not forget to clear h afterward.
Example
pTextToSpeech = actxserver('Sapi.SpVoice')
delete(pTextToSpeech)
clear pTextToSpeech
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
COM_fieldnames
Returns the properties name of an COM object.
Syntax
- l = COM_fieldnames(h)
- l = fieldnames(h)
Input argument
- h - a COM object.
Output argument
- l - a cell of strings.
Description
Example
e = actxserver('Excel.Application');
fieldnames(e)
delete(e)
clear e
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
COM_get
Get property value from COM interface.
Syntax
- v = get(h, propertyname)
- v = COM_get(h, propertyname)
- v = h.propertyname
Input argument
- h - a COM object.
- propertyname - a string: the property's name of COM object.
Output argument
- v - a nelson variable.
Description
The function returns the value of the property specified in the string, propertyname.
Example
e = actxserver('Excel.Application');
get(e, 'Path')
e.Path
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
COM_invoke
Invoke method on COM object or interface.
Syntax
- S = invoke(h, methodname, arg1, arg2, ...)
- S = COM_invoke(h, methodname, arg1, arg2, ...)
Input argument
- h - a COM object.
- methodname - a string: the method name invoked on COM object.
- arg1, arg2, ... - a Nelson variable of type double, int, boolean, string, ... used as parameters of COM function invoked.
Output argument
- S - a COM object or data.
Description
If the method returns a COM interface, then ole_invoke returns a new COM object that represents the returned interface.
Example
pWord = actxserver('Word.Application')
pWord.Visible = true
invoke(pWord, 'Quit')
delete(pWord)
clear pWord
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
COM_ismethod
Determines if input is an existing COM object method.
Syntax
- r = COM_ismethod(h, methodname)
- r = ismethod(h, methodname)
Input argument
- h - a COM object.
- methodname - a string: method name tested as valid method for the COM object.
Output argument
- r - a logical.
Description
Example
e = actxserver('Excel.Application');
ismethod(e, 'Quit')
delete(e)
clear e
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
COM_isprop
Determines if input is an existing COM object property.
Syntax
- r = COM_isprop(h, propertyname)
- r = isprop(h, propertyname)
Input argument
- h - a COM object.
- propertyname - a string: property name tested as valid property for the COM object.
Output argument
- r - a logical.
Description
Example
e = actxserver('Excel.Application');
isprop(e, 'Window')
delete(e)
clear e
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
COM_methods
Returns the methods name of an COM object.
Syntax
- l = COM_methods(h)
- l = methods(h)
Input argument
- h - a COM object.
Output argument
- l - a cell of strings.
Description
Example
e = actxserver('Excel.Application');
methods(e)
delete(e)
clear e
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
COM_range
Private function: range helper.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
COM_set
Set object or interface property to specified value.
Syntax
- set(h, propertyname, value)
- COM_set(h, propertyname, value)
- h.propertyname = value
Input argument
- h - a COM object.
- propertyname - a string: the property's name of COM object.
- value - a string, boolean, double ...
Description
The function sets the property specified in the string propertyname to the given value.
Example
pWord = actxserver('Word.Application')
pWord.Visible = true
invoke(pWord, 'Quit')
delete(pWord)
clear pWord
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
COM_used
Returns list of current used COM handle.
Syntax
- r = COM_used()
Output argument
- h - a vector of COM handle.
Description
Returns list of current used COM handle.
See also
Example
delete(COM_used())
e = actxserver('Excel.Application');
used = COM_used()
delete(used)
used = COM_used()
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
COM_xlsfinfo
Determines if file contains Microsoft Excel spreadsheet.
Syntax
- status = xlsfinfo(filename)
- [status, sheets] = xlsfinfo(filename)
- [status, sheets, xlsformat] = xlsfinfo(filename)
Input argument
- filename - a string: a filename.
Output argument
- status - a string: file type
- sheets - a vector of strings: sheet names
- xlsformat - a string: excel file format
Description
Query Excel spreadsheet file filename for some information about its contents.
Example
[status, sheets, xlsformat] =COM_xlsfinfo([modulepath('com_engine'), '/examples/sample_xslx.xlsx'])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
COM_xlsread
Read Microsoft Excel spreadsheet file using COM.
Syntax
- numeric_data = COM_xlsread(filename)
- numeric_data = COM_xlsread(filename, sheet)
- numeric_data = COM_xlsread(filename, range)
- numeric_data = COM_xlsread(filename, sheet, range)
- numeric_data = COM_xlsread(filename, sheet, range)
- [numeric_data, txt_data, raw_data] = COM_xlsread(filename)
- [numeric_data, txt_data, raw_data] = COM_xlsread(filename, sheet)
- [numeric_data, txt_data, raw_data] = COM_xlsread(filename, range)
- [numeric_data, txt_data, raw_data] = COM_xlsread(filename, sheet, range)
- [numeric_data, txt_data, raw_data] = COM_xlsread(filename, sheet, range)
Input argument
- filename - a string: an existing filename.
- sheet - an integer or a string: sheet id or sheet name
- range - an string: an range xx:xx
Output argument
- numeric_data - a matrix or vector: string data converted to double.
- txt_data - a cell of strings with only strings.
- raw_data - a cell of strings: raw data without conversion.
Description
Example
data = {'Time', 'Temp'; 12 98; 13 99; Inf 97};
s = COM_xlswrite([tempdir(), 'example_xlswrite_2.xlsx'], data, 'Temperatures');
[numeric_data, txt_data, raw_data] = COM_xlsread([tempdir(), 'example_xlswrite_2.xlsx'])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
COM_xlswrite
Write Microsoft Excel spreadsheet file using COM.
Syntax
- COM_xlswrite(filename, v)
- COM_xlswrite(filename, v, sheet)
- COM_xlswrite(filename, v, range)
- COM_xlswrite(filename, v, sheet, range)
- status = COM_xlswrite(filename, v)
- status = COM_xlswrite(filename, v, sheet)
- status = COM_xlswrite(filename, v, range)
- status = COM_xlswrite(filename, v, sheet, range)
- [status, msg] = COM_xlswrite(filename, v)
- [status, msg] = COM_xlswrite(filename, v, sheet)
- [status, msg] = COM_xlswrite(filename, v, range)
- [status, msg] = COM_xlswrite(filename, v, sheet, range)
Input argument
- filename - a string: a full filename path.
- v - a string, cell, matrix: values to save.
- sheet - an integer or a string: sheet id or sheet name
- range - an string: an range xx:xx
Output argument
- status - a logical: true if save.
- msg - a string: '' if no error or an error message.
Description
COM_xlswrite Writes Microsoft Excel spreadsheet file using COM.
Inf is converted by Excel as 65535.
Examples
COM_xlswrite([tempdir(), 'example_xlswrite_1.xlsx'], rand(3, 3))
data = {'Time', 'Temp'; 12 98; 13 99; Inf 97};
s = COM_xlswrite([tempdir(), 'example_xlswrite_2.xlsx'], data, 'Temperatures');
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
actxGetRunningServer
Handle to running instance of Automation server.
Syntax
- h = actxGetRunningServer(progid)
Input argument
- progid - a string: the name of a COM server.
Output argument
- h - a COM object.
Description
h = actxGetRunningServer(progid) gets a reference to a running instance of the OLE/COM Automation server.
progid is the programmatic identifier of the Automation server object and h is the handle to the default interface of the server object.
The function returns an error if the server specified by progid is not currently running or if the server object is not registered.
When multiple instances of the Automation server are running, the operating system controls the behavior of this function.
Example
h = actxGetRunningServer('Excel.application')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
actxcontrollist
Get available ActiveX controls.
Syntax
- l = actxcontrollist()
Output argument
- l - a 1 by 3cell of strings.
Description
l = actxcontrollist() returns list of available controls
Example
l = actxcontrollist()
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
actxserver
Creates COM server.
Syntax
- h = actxserver(progid)
- h = actxserver(progid, 'machine', machineName)
Input argument
- progid - a string: the name of a COM server.
- machineName - a string: the name of the machine on which to start the server.
Output argument
- h - a COM object.
Description
h = actxserver(progid) creates a COM server using the progid identifier.
Examples
h = actxserver('Excel.application')
pTextToSpeech = actxserver('Sapi.SpVoice')
for i = 0:5
invoke(pTextToSpeech, 'Speak', int2str(5 - i));
end
invoke(pTextToSpeech, 'Speak', _('Welcome to COM Interface for Nelson !'));
delete(pTextToSpeech)
clear pTextToSpeech
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
actxserverlist
Get available ActiveX servers.
Syntax
- l = actxserverlist()
Output argument
- l - a 1 by 3cell of strings.
Description
l = actxserverlist() returns list of available servers.
Example
l = actxserverlist()
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
iscom
Determine whether input is COM or ActiveX object.
Syntax
- r = iscom(h)
Input argument
- h - a nelson variable.
Output argument
- r - a logical: true or false.
Description
r = iscom(h) returns logical true if handle h is a COM or a Microsoft® ActiveX® object. Otherwise, it returns false.
Example
pWord = actxserver('Word.Application')
iscom(pWord)
delete pWord
iscom(pWord)
clear pWord
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
Control System functions
Control System functions
Description
Algorithms for designing, analyzing and tuning linear control systems.
- abcdchk - Verifies the dimensional compatibility of matrices A, B, C, and D.
- acker - Pole placement gain selection using Ackermann's formula.
- append - Appends the inputs and outputs of the two models.
- augstate - Append state vector to output vector.
- balreal - Gramian-based balancing of state-space realizations.
- bdschur - Block-diagonal Schur factorization.
- bode - Bode plot of frequency response, magnitude and phase data.
- c2d - Convert model from continuous to discrete time.
- care - Continuous-time algebraic Riccati equation solution.
- cloop - Feedback connection of multiple models.
- compreal - Companion realization of transfer functions.
- ctrb - Controllability of state-space model.
- ctrbf - Compute controllability staircase form.
- d2c - Convert model from discrete to continuous time.
- damp - Natural frequency and damping ratio.
- dare - Discret-time algebraic Riccati equation solution.
- dcgain - Low-frequency (DC) gain of LTI system.
- dlqr - Linear-quadratic (LQ) state-feedback regulator for discrete-time state-space system.
- dlyap - Discrete-time Lyapunov equations.
- dsort - Sort discrete-time poles by magnitude.
- esort - Sort continuous-time poles by real part.
- evalfr - Evaluate frequency response at given frequency.
- feedback - Feedback connection of multiple models.
- freqresp - Evaluate system response over a grid of frequencies.
- gensig - Create periodic signals for simulating system response.
- gram - Controllability and observability Gramians.
- hsvd - Hankel singular values of dynamic system.
- impulse - Impulse response plot of dynamic system.
- initial - System response to initial states of state-space model.
- isct - Checks if dynamic system model is in continuous time.
- isdt - Checks if dynamic system model is in discret time.
- islti - Checks if variable is an linear model tf, ss or zpk.
- issiso - Checks if dynamic system model is single input and single output.
- isstatic - Checks if model is static or dynamic.
- kalman - Design Kalman filter for state estimation.
- lqe - Kalman estimator design for continuous-time systems.
- lqed - Calculates the discrete Kalman estimator configuration based on a continuous cost function.
- lqr - Linear-Quadratic Regulator (LQR) design.
- lqry - Form linear-quadratic (LQ) state-feedback regulator with output weighting.
- lsim - Plot simulated time response of dynamic system to arbitrary inputs.
- lyap - Continuous Lyapunov equation solution.
- minreal - Minimal realization or pole-zero cancellation.
- nyquist - Nyquist plot of frequency response.
- obsv - Observability of state-space model.
- obsvf - Compute observability staircase form.
- ord2 - Generate continuous second-order systems.
- padecoef - Computes the Pade approximation of time delays.
- parallel - Parallel connection of two models.
- pole - Poles of dynamic system.
- series - Series connection of two models.
- ss - State-space model.
- ss2tf - Convert state-space representation to transfer function.
- ssdata - Access state-space model data.
- ssdelete - Remove inputs, outputs and states from state-space system.
- ssselect - Extract subsystem from larger system.
- step - Step response plot of dynamic system.
- tf - Constructs a transfer function model.
- tf2ss - Convert transfer function filter parameters to state-space form.
- tfdata - Access transfer function model data.
- tzero - Invariant zeros of linear system.
- zero - Zeros and gain of SISO dynamic system.
abcdchk
Verifies the dimensional compatibility of matrices A, B, C, and D.
Syntax
- [msg, A, B, C, D] = abcdchk(a, b, c, d)
Input argument
- a (n x n) - Represents the system's state-transition matrix. It describes how the system's internal state evolves over time.
- b (n x m) - Describes the input-to-state mapping. It shows how control inputs affect the change in the system's state.
- c (p x n) - Represents the state-to-output mapping. It shows how the system's state variables are related to the system's outputs.
- d (p x m) - Describes the direct feedthrough from inputs to outputs. In many systems, this matrix is zero because there is no direct feedthrough.
Output argument
- msg - Returns an empty struct if matrix dimensions are consistent. Otherwise it returns the associated error message.
- a (n x n) - Represents the system's state-transition matrix. It describes how the system's internal state evolves over time.
- b (n x m) - Describes the input-to-state mapping. It shows how control inputs affect the change in the system's state.
- c (p x n) - Represents the state-to-output mapping. It shows how the system's state variables are related to the system's outputs.
- d (p x m) - Describes the direct feedthrough from inputs to outputs. In many systems, this matrix is zero because there is no direct feedthrough.
Description
abcdchk verify dimensional consistency of the matrices A, B, C, D, E.
It additionally adjusts the dimensions of any empty 0-by-0 matrices to ensure their alignment with the rest.
Example
A = [0 1; -2 -3];
B = [0; 1];
C = [1 0];
D = 0;
[msg, AA, BB, CC, DD] = abcdchk(A, B, C, D)
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
acker
Pole placement gain selection using Ackermann's formula.
Syntax
- K = acker(A, B, P)
Input argument
- A - State matrix: Nx-by-Nx matrix
- B - Input-to-state matrix: Nx-by-Nu matrix
- P - Desired closed-loop pole location vector.
Output argument
- K - feedback gain matrix.
Description
The function acker computes the feedback gain matrix K for a single-input system described by the state-space matrices A and B.
The closed-loop poles of the system under the feedback law u = -Kx are determined by the specified vector P, where P represents the desired pole locations.
The closed-loop poles are essentially the eigenvalues of the matrix A - B*K, calculated as P = eig(A - B*K).
It's important to note that this algorithm utilizes Ackermann's formula.
However, users should be aware that this method may not be numerically reliable, particularly for systems of order greater than 10 or for systems that are weakly controllable.
If the algorithm encounters numerical instability or if the closed-loop poles deviate significantly (more than 10%) from the desired locations specified in P, a warning message is issued to alert the user about potential issues.
Users are advised to exercise caution and consider alternative methods for higher-order or weakly controllable systems.
Example
A = [0 1 0; 0 0 1;-1 -5 -6];
B = [ 0; 0; 1];
P = [-10 -2-4i -2+4i];
K = acker(A, B, P)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
append
Appends the inputs and outputs of the two models.
Syntax
- sys = append(sys1, sys2, ..., sysN)
Input argument
- sys1, sys2, ..., sysN - LTI models.
Output argument
- sys - LTI model.
Description
sys = append(sys1, sys2, ..., sysN) combines the inputs and outputs of models sys1 through sysN, creating an augmented model represented by sys.
Example
sys1 = tf(1,[1 0]);
sys2 = tf([1 -1], [4 2]);
sys = append(sys1, 10, sys2)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
augstate
Append state vector to output vector.
Syntax
- sysa = augstate(sys)
- [Aa, Ba, Ca, Da] = augstate(A, B, C, D)
Input argument
- sys - LTI model.
Output argument
- sysa - State-space model with states appended to the outputs.
Description
The function sysa = augstate(sys) adds the state vector to the outputs of a state-space model.
Example
sys = ss(10, 10, 20, 0);
sysa = augstate(sys)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
balreal
Gramian-based balancing of state-space realizations.
Syntax
- [sysb, g] = balreal(sys)
- [sysb, g, T, Ti] = balreal(sys)
Input argument
- sys - LTI model.
Output argument
- sysb - LTI model.
- g - Diagonal vector of the balanced Gramian matrix.
- T - State similarity transform matrix.
- Ti - State similarity transform inverse matrix.
Description
balreal(sys) calculates a balanced realization, denoted as sysb, for the stable segment of the linear time-invariant (LTI) model sys.
This process is applicable to both continuous and discrete systems. If sys is not initially in state-space form, the function automatically converts it to state space using ss before proceeding with the balanced realization.
Example
sys = ss([-1, 0; 0.1, -3], [1, 0]', [0, 1], 0);
[sysb, g, T, Ti] = balreal(sys)
See also
gram.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
bdschur
Block-diagonal Schur factorization.
Syntax
- [T, B] = bdschur(A)
- [T, B] = bdschur(A, CONDMAX)
Input argument
- A - Square real matrix.
- CONDMAX - upper bound on the condition number of T. By default, CONDMAX = 1e4.
Output argument
- T - Transformation matrix.
- B - B = T \ A * T
Description
[T, B] = bdschur(A, CONDMAX) calculates a transformation matrix T, where B = T \ A * T results in a block diagonal matrix with each block being a quasi upper-triangular Schur matrix, ensuring the diagonalization of matrix A while preserving certain structural properties.
Used function(s)
MB03RD
Bibliography
http://slicot.org/objects/software/shared/doc/MB03RD.html
Example
A = [1. -1. 1. 2. 3. 1. 2. 3.;
1. 1. 3. 4. 2. 3. 4. 2.;
0. 0. 1. -1. 1. 5. 4. 1.;
0. 0. 0. 1. -1. 3. 1. 2.;
0. 0. 0. 1. 1. 2. 3. -1.;
0. 0. 0. 0. 0. 1. 5. 1.;
0. 0. 0. 0. 0. 0. 0.99999999 -0.99999999;
0. 0. 0. 0. 0. 0. 0.99999999 0.99999999];
[T, B] = bdschur(A)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
SLICOT Documentation
bode
Bode plot of frequency response, magnitude and phase data.
Syntax
- bode()
- bode(H)
- bode(H, wIn)
- bode(H, w, lineSpec)
- [magnitude, phase, w] = bode(H)
- [magnitude, phase, w] = bode(H, wIn)
Input argument
- H - a lti model.
- wIn - a cell {wmin, wmax} or a vector [wmin:wmax].
- lineSpec - Line style, marker, and color.
Output argument
- magnitude - Magnitude: size 1 x 1 x k (SISO).
- phase - Phase: size 1 x 1 x k (SISO).
- w - Frequencies: a vector: 1 x k.
Description
bode(sys) generates a Bode plot illustrating the frequency response of a dynamic system model, denoted as sys.
This plot visually represents the system's response in terms of both magnitude (measured in decibels, dB) and phase (measured in degrees) across varying frequencies.
The specific frequency points on the plot are automatically determined by bode based on the system's inherent dynamics.
Example
H = tf([1 0.1 7.5],[1 0.12 9 0 0]);
bode(H,{1 10}, '-.')
See also
plot.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
c2d
Convert model from continuous to discrete time.
Syntax
- [P, G] = c2d(A, B, Ts)
- sysd = c2d(sysc, Ts)
- sysd = c2d(sysc, Ts, method)
- sysd = c2d(sysc, Ts, 'prewarp', w0)
Input argument
- A - State matrix: Nx-by-Nx matrix.
- B - Input-to-state matrix: Nx-by-Nu matrix.
- Ts - Sample time: positive scalar.
- sysc - Continuous-time dynamic system: LTI model.
- method - Discretization method: 'zoh', 'tustin', 'prewarp'
- w0 - prewarp frequency.
Output argument
- P - phi
- G - gamma
- sysd - Discrete-time model
Description
The function sysd = c2d(sysc, Ts) discretizes the continuous-time dynamic system model sysc using a zero-order hold on the inputs with a sample time of Ts.
For instance, you can use sysd = c2d(sysc, Ts, method) to explicitly specify the discretization method.
Example
A = [1 0.5; 0.5 1 ];
B = [0 -1; 1 0 ];
C = [ -1 0; 0 1 ];
D = [ 1 0; 0 -1 ];
sys = ss(A, B, C, D);
Ts = 2;
sysd = c2d(sys, Ts, 'zoh')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
care
Continuous-time algebraic Riccati equation solution.
Syntax
- [X, L, G] = care(A, B, Q)
- [X, L, G] = care(A, B, Q, R, S, E)
Input argument
- A - Matrix representing the state with dimensions n x n, where n corresponds to the number of states.
- B - Matrix representing control with dimensions n x p, where p is the number of inputs.
- Q - Matrix describing the cost associated with the state, having dimensions n x n, where n is the number of states.
- R - Matrix representing the cost associated with control, with dimensions p x p, where p is the number of inputs.
- S - Matrix that is optionally real-valued with dimensions n x p.
- E - Matrix with dimensions n x n that serves as a descriptor matrix.
Output argument
- X - stabilized solution for the continuous-time Riccati equation of dimension n x n.
- L - Closed-loop pole vector.
- G - Gain matrix.
Description
The function care(A, B, Q) calculates the exclusive solution, denoted as X, for the continuous-time algebraic Riccati equation with matrices A, B, and Q, and also provides additional matrices L and G.
Example
a = [-3 2;1 1];
b = [0 ; 1];
c = [1 -1];
r = 3;
[x, l, g] = care(a, b, c'*c, r)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
SLICOT Documentation
cloop
Feedback connection of multiple models.
Syntax
- model = cloop(sys)
- model = cloop(sys, sign)
- model = cloop(sys, outputs, inputs)
Input argument
- sys - LTI model.
- sign - Type of feedback: -1 (default) or +1.
- outputs - vector indexes into the outputs.
- inputs - vector indexes into the inputs.
Output argument
- sys - Closed-loop system.
Description
cloop forms the closed-loop system when unity feedback is used.
This function is deprecated and has limitations, please see feedback. It is only applicable when the block in the feedback path is unity. Furthermore, its usage is restricted to system models expressed solely in transfer function form, and not in the more general "system".
Example
m = 1000;
b = 50;
u = 500;
A = [0 1; 0 -b/m];
B = [0; 1/m];
C = [0 1];
D = 0;
OUTPUTS = -1;
INPUTS = 1;
sys = ss(A, B, C, D);
R = cloop(sys, OUTPUTS, INPUTS)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
compreal
Companion realization of transfer functions.
Syntax
- [A, B, C, D, E] = compreal(numerator, denominator)
Input argument
- numerator - a vector or matrix
- denominator - a vector
Output argument
- A (n x n) - Represents the system's state-transition matrix. It describes how the system's internal state evolves over time.
- B (n x m) - Describes the input-to-state mapping. It shows how control inputs affect the change in the system's state.
- C (p x n) - Represents the state-to-output mapping. It shows how the system's state variables are related to the system's outputs.
- D (p x m) - Describes the direct feedthrough from inputs to outputs. In many systems, this matrix is zero because there is no direct feedthrough.
- E (n x n) - matrix.
Description
[A, B, C, D, E] = compreal(numerator, denominator) calculates a state-space realization represented by matrices A, B, C, D, and E.
The E matrix is an empty matrix (identity matrix) when there are at least as many poles as zeros.
However, if there are more zeros than poles, the E matrix becomes singular.
Example
numerator = [0 10 10];
denominator = [1 1 10];
[A, B, C, D, E] = compreal(numerator, denominator)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
ctrb
Controllability of state-space model.
Syntax
- Co = ctrb(A, B)
- Co = ctrb(sys)
Input argument
- sys - State-space model
- A - State matrix: Nx-by-Nx matrix
- B - Input-to-state matrix: Nx-by-Nu matrix
Output argument
- Co - Controllability matrix.
Description
Controllability in a dynamic system refers to the system's ability to be guided to any desired state within a finite timeframe through the application of suitable control signals.
This property is commonly known as reachability.
The function ctrb is employed to calculate a controllability matrix, either from state matrices or a state-space model.
The resulting matrix serves as a tool to assess and confirm the controllability of the system.
Example
A = [1 2; 0 3];
B = [1; 1];
C = eye(2);
D = zeros(2, 1);
sys = ss(A, B, C, D);
Co = ctrb(sys)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
ctrbf
Compute controllability staircase form.
Syntax
- [Abar, Bbar, Cbar, T, k] = ctrbf(A, B, C)
- [Abar, Bbar, Cbar, T, k] = ctrbf(A, B, C, tol)
Input argument
- A - State matrix: Nx-by-Nx matrix
- B - Input-to-state matrix: Nx-by-Nu matrix
- C - Output-to-state matrix: Ny-by-Nx matrix
- tol - scalar real (tolerance).
Output argument
- Abar - Observability staircase state matrix.
- Bbar - Observability staircase input matrix.
- Cbar - Observability staircase output matrix.
- T - Similarity transform matrix.
- k - Vector: number of observable states.
Description
ctrbf(A, B, C) decomposes the given state-space system, defined by matrices A, B, and C, into the controllability staircase form.
This results in transformed matrices Abar, Bbar, and Cbar, along with a similarity transformation matrix T and a vector k.
The length of vector k is equal to the order of the system represented by A, and each entry in k denotes the number of controllable states factored out at each step of the transformation matrix computation.
The non-zero elements in k indicate the number of iterations required for T calculation, and the sum of k corresponds to the number of states in Ac, the controllable portion of Abar.
Example
A = [-1.5 -0.5; 1 0];
B = [0.5; 0];
C = [0 1];
[Abar, Bbar, Cbar, T, k] = ctrbf(A, B, C)
See also
ctrb.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
d2c
Convert model from discrete to continuous time.
Syntax
- sysc = d2c(sysd)
- sysc = d2c(sysd, method)
- sysc = d2c(sysd, 'prewarp', w0)
Input argument
- sysd - Discret-time dynamic system: LTI model.
- method - Discretization method: 'zoh', 'tustin', 'prewarp'
- w0 - prewarp frequency.
Output argument
- sysc - continuous-time model
Description
The function sysc = d2c(sysd) transforms a discrete-time dynamic system model sysd into a continuous-time model, employing zero-order hold on the inputs.
For instance, you can use sysc = d2c(sysd, method) to explicitly define the conversion method.
Example
A = [0.25, 0.5; 0, 0.1];
B = [1; 0];
C = [-1, 0];
sys = ss(A, B, C, 0, 0.2);
sysc = d2c(sys, 'zoh')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
damp
Natural frequency and damping ratio.
Syntax
- [wn, zeta] = damp(sys)
- [wn, zeta, p, T] = damp(sys)
Input argument
- sys - LTI model.
Output argument
- wn - Natural frequency of each pole: vector.
- zeta - Damping ratio of each pole: vector.
- p - Poles of the dynamic system model: vector.
- T - Time Constant (seconds): vector.
Description
The function damp(sys) provides the natural frequencies (wn) and damping ratios (zeta) associated with the poles of the system represented by sys.
Example
sys = tf([2, 5, 1], [1, 0, 2, -6]);
[wn, zeta, p, T] = damp(sys)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
dare
Discret-time algebraic Riccati equation solution.
Syntax
- [X, L, G] = dare(A, B, Q)
- [X, L, G] = dare(A, B, Q, R, S, E)
Input argument
- A - Matrix representing the state with dimensions n x n, where n corresponds to the number of states.
- B - Matrix representing control with dimensions n x p, where p is the number of inputs.
- Q - Matrix describing the cost associated with the state, having dimensions n x n, where n is the number of states.
- R - Matrix representing the cost associated with control, with dimensions p x p, where p is the number of inputs.
- S - Matrix that is optionally real-valued with dimensions n x p.
- E - Matrix with dimensions n x n that serves as a descriptor matrix.
Output argument
- X - stabilized solution for the discret-time Riccati equation of dimension n x n.
- L - Closed-loop pole vector.
- G - Gain matrix.
Description
The function dare(A, B, Q) calculates the exclusive solution, denoted as X, for the discret-time algebraic Riccati equation with matrices A, B, and Q, and also provides additional matrices L and G.
Example
a = [-3 2;1 1];
b = [0 ; 1];
c = [1 -1];
r = 3;
[x, l, g] = dare(a, b, c'*c, r)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
SLICOT Documentation
dcgain
Low-frequency (DC) gain of LTI system.
Syntax
- k = dcgain(sys)
Input argument
- sys - a LTI model.
Output argument
- k - DC gain.
Description
k = dcgain(sys) computes the DC gain k of the LTI model sys.
Example
A = [1 2; 3 4];
B = [1 0; 0 1];
C = [1 1; 1 1];
D = [0 0; 0 0];
sys = ss(A, B, C, D);
K = dcgain(sys)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
dlqr
Linear-quadratic (LQ) state-feedback regulator for discrete-time state-space system.
Syntax
- [K, S, e] = dlqr(A, B, Q, R, N)
- [K, S, e] = dlqr(A, B, Q, R)
Input argument
- A - State matrix: n x n matrix.
- B - Input-to-state matrix: n x m matrix.
- Q - State-cost weighted matrix
- R - Input-cost weighted matrix
- N - Optional cross term matrix: 0 by default.
Output argument
- K - Optimal gain: row vector.
- S - Solution of the Algebraic Riccati Equation.
- e - Poles of the closed-loop system: column vector.
Description
The dlqr function is designed to minimize a quadratic cost function associated with a discrete linear time-invariant state-space system model.
Example
A = [0.9, 0.2; 0, 0.8];
B = [0; 2];
Q = [4, 0; 0, 4];
R = 3;
[K, S, e] = dlqr(A, B, Q, R)
See also
lqr.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
dlyap
Discrete-time Lyapunov equations.
Syntax
- X = dlyap(A, Q)
Input argument
- A - real matrix
- Q - real matrix
Output argument
- X - matrix: solution of the discrete-time Lyapunov equation.
Description
X = dlyap(A, Q) resolves the Discrete-time Lyapunov equation.
Example
A = [10, 20; -30, -40];
Q = [30, 10; 10, 10];
X = dlyap (A, Q)
See also
lyap.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
dsort
Sort discrete-time poles by magnitude.
Syntax
- s = dsort(p)
- [s, ndx] = dsort(p)
Input argument
- p - p: a vector
Output argument
- s - sorted vector by magnitude.
Description
dsort arranges the discrete-time poles within the vector p in a descending order based on their magnitude, with unstable poles taking precedence at the beginning of the sorted list.
Example
p = [-2.410 + 5.573i;
-2.410 - 5.573i;
1.503;
-0.972;
-2.590];
[s, ndx] = dsort(p)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
esort
Sort continuous-time poles by real part.
Syntax
- s = esort(p)
- [s, ndx] = esort(p)
Input argument
- p - p: a vector
Output argument
- s - sorted vector by real part.
Description
esort arranges the continuous-time poles within the vector p based on their real parts.
Unstable eigenvalues take precedence at the beginning of the sorted list, and the rest of the poles are organized in descending order according to their real parts.
Example
p = [-2.410 + 5.573i;
-2.410 - 5.573i;
1.503;
-0.972;
-2.590];
[s, ndx] = esort(p)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
evalfr
Evaluate frequency response at given frequency.
Syntax
- frsp = evalfr(sys, f)
Input argument
- sys - LTI model
- f - single frequency
Output argument
- frsp - frequency response
Description
The function evalfr(sys, f) computes the value of the transfer function for a given system model represented by sys at the complex number f.
Example
numerator = {[2, 0], [1, 3]};
denominator = {[4, 0, 3, -1], [1 , 3, 5]};
sys = tf(numerator, denominator);
z = 1 + j;
frsp = evalfr(sys, z)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
feedback
Feedback connection of multiple models.
Syntax
- sys = feedback(sys1, sys2)
- sys = feedback(sys1, sys2, sign)
Input argument
- sys1, sys2 - LTI models: Systems to connect in a feedback loop.
- sign - Type of feedback: -1 (default) or +1.
Output argument
- sys - Closed-loop system.
Description
sys = feedback(sys1, sys2) generates a model object, sys, representing the negative feedback interconnection of the model objects sys1 and sys2.
Example
G = tf([2 5 1], [1 2 3]);
C = tf([5, 10], [1, 10]);
sys = feedback(G, C, +1)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
freqresp
Evaluate system response over a grid of frequencies.
Syntax
- [H, wout] = freqresp(sys, w)
- H = freqresp(sys, w)
Input argument
- sys - LTI model
- w - Frequencies: vector
Output argument
- H - Frequency response values
- wout - Output frequencies corresponding to the frequency response: vector.
Description
freqresp computes the frequency response of a dynamic system sys at specified frequencies w.
To acquire magnitude and phase data, along with visual representations of the frequency response, utilize the bode function.
Examples
G = tf(1,[1 1]);
h1 = freqresp(G, 3)
num = [1 2];
den = [1 3 2];
sys = tf(num,den);
w = linspace(0, 100, 60);
[resp,freq] = freqresp(sys, w);
f = figure();
subplot(2, 1, 1);
plot(freq, 20 * log10(abs(squeeze(resp))));
ylabel(_('Amplitude (dB)'));
subplot(2, 1, 2);
plot(freq, angle(squeeze(resp)) * 180/pi);
ylabel(_('Phase (degrees)'));
xlabel(_('Frequency (Hz)'));
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
gensig
Create periodic signals for simulating system response.
Syntax
- [u, t] = gensig(type, tau)
- [u, t] = gensig(type, tau, Tf)
- [u, t] = gensig(type, tau, Tf, Ts)
Input argument
- type - Type of periodic signal: 'cos', 'tan', 'sin', 'pulse', 'square'
- tau - Period: positive scalar
- Tf - Duration: positive scalar or 5*tau (default)
- Ts - positive scalar or tau/64 (default)
Output argument
- u - Generated signal: column vector.
- t - Time vector: column vector.
Description
The function gensig(type, tau) creates a periodic signal with unit amplitude, characterized by the specified type and period.
The resulting signal, denoted as u, and its corresponding time vector, t, can be utilized for simulating the time response of a single-input dynamic system using lsim.
For multi-input systems, you can generate signals by making repeated calls to gensig and then assemble the resulting u vectors into a matrix. When simulating a dynamic system model with u and t, note that the software interprets the time vector t with units based on the TimeUnit property of the model.
To generate a signal with a specific duration Tf, use [u, t] = gensig(type, tau, Tf).
The time vector t spans from 0 to Tf in increments of tau/64.
For a signal with a defined sample time Ts, employ [u, t] = gensig(type, tau, Tf, Ts).
In this case, the time vector t ranges from 0 to Tf in increments of Ts.
This syntax is particularly useful for generating signals tailored for discrete-time model simulations, where Ts corresponds to the sample time of the model.
Example
f = figure();
tau = 3;
Tf = 6;
Ts = 0.1;
subplot(3, 2, 1)
[u,t] = gensig("sine",tau,Tf,Ts);
plot(t, u)
title('sine')
subplot(3, 2, 2)
[u,t] = gensig("square",tau,Tf,Ts);
plot(t, u)
title('square')
subplot(3, 2, 3)
[u,t] = gensig("cos",tau,Tf,Ts);
plot(t, u)
title('cosine')
subplot(3, 2, 4)
[u,t] = gensig("sin",tau,Tf,Ts);
plot(t, u)
title('sine')
subplot(3, 2, 5)
[u,t] = gensig("tan",tau,Tf,Ts);
plot(t, u)
title('tan')
See also
lsim.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
gram
Controllability and observability Gramians.
Syntax
- wc = gram(sys, 'o')
- wc = gram(sys, 'c')
Input argument
- sys - state-space model.
Output argument
- wc - observability or controllability Gramian.
Description
Example
sys = ss([-.1 -1;1 0], [1;0], [0 1], 0);
wc = gram(sys, 'c')
wc = gram(sys, 'o')
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
hsvd
Hankel singular values of dynamic system.
Syntax
- hsv = hsvd(sys)
Input argument
- sys - State-space model
Output argument
- hsv - Hankel singular values.
Description
hsv = hsvd(sys) calculates the Hankel singular values (hsv) for the dynamic system sys.
These singular values are computed in state coordinates that balance the energy transfers from input to state and from state to output.
The Hankel singular values serve as a measure of the impact of each state on the input/output characteristics of the system.
Analogous to how singular values relate to matrix rank, small Hankel singular values indicate states that may be omitted to streamline the model and simplify its representation.
Example
A = [ -0.04165 0.0000 4.9200 -4.9200 0.0000 0.0000 0.0000;
-5.2100 -12.500 0.0000 0.0000 0.0000 0.0000 0.0000;
0.0000 3.3300 -3.3300 0.0000 0.0000 0.0000 0.0000;
0.5450 0.0000 0.0000 0.0000 -0.5450 0.0000 0.0000;
0.0000 0.0000 0.0000 4.9200 -0.04165 0.0000 4.9200;
0.0000 0.0000 0.0000 0.0000 -5.2100 -12.500 0.0000;
0.0000 0.0000 0.0000 0.0000 0.0000 3.3300 -3.3300];
B = [ 0.0000 0.0000;
12.5000 0.0000;
0.0000 0.0000;
0.0000 0.0000;
0.0000 0.0000;
0.0000 12.500;
0.0000 0.0000];
C = [ 1.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000
0.0000 0.0000 0.0000 1.0000 0.0000 0.0000 0.0000
0.0000 0.0000 0.0000 0.0000 1.0000 0.0000 0.0000];
D = [];
sys = ss(A, B, C, D);
hsv = hsvd(sys)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
impulse
Impulse response plot of dynamic system.
Syntax
- [y, t, x] = impulse(sys)
- [y, t, x] = impulse(sys, tFinal)
- [y, t, x] = impulse(sys, [t0, tFinal])
- [y, t, x] = impulse(sys, t)
- impulse(...)
Input argument
- sys - a lti model.
- t - Time samples: vector.
- tFinal - End time for step response: scalar.
- [t0, tFinal] - Time range for step response: two-element vector.
Output argument
- y - Simulated response data: matrix or vector.
- tOut - Time vector: vector.
- x - State trajectories: matrix or vector.
Description
Example
sys = tf(4,[1 2 10]);
t = 0:0.05:5;
f = figure();
impulse(sys,t);
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
initial
System response to initial states of state-space model.
Syntax
- [y, t, x] = initial(sys, x0)
- [y, t, x] = initial(sys, x0, Tfinal)
- [y, t, x] = initial(sys, x0, t)
- [y, t, x] = initial(sys, x0, [t0, tFinal])
- initial(...)
Input argument
- sys - a lti model.
- x0 - Initial state values: vector.
- t - Time samples: vector.
- tFinal - End time for step response: scalar.
- [t0, tFinal] - Time range for step response: two-element vector.
Output argument
- y - Simulated response data: matrix or vector.
- tOut - Time vector: vector.
- x - State trajectories: matrix or vector.
Description
[y, tOut] = initial(sys, x0) calculates the unforced initial response (y) of the dynamic system sys from the specified initial state x0.
The time vector tOut is provided in the time units of sys, and the initial function automatically adapts time steps and simulation duration based on the system dynamics.
When you use [y, tOut] = initial(sys, x0, tFinal), the function simulates the response from t = 0 to the final time t = tFinal.
Similarly, [y, tOut] = initial(sys, x0, [t0, tFinal]) simulates the response from t0 to tFinal.
Additionally, [y, tOut] = initial(sys, x0, t) returns the initial response of sys at the specified times provided in the vector t.
Example
A = [-10 -20 -30;1 0 0; 0 1 0];
B = [1; 0; 0];
C = [0 0 1];
D = 0;
T = [0:0.1:1];
U = zeros(size(T, 1), size(T, 2));
X0 = [0.1 0.1 0.1];
sys = ss(A, B, C, D);
initial(sys, X0);
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
isct
Checks if dynamic system model is in continuous time.
Syntax
- res = isct(sys)
Input argument
- sys - a lti model.
Output argument
- res - a logical: true if dynamic system model is in continuous time.
Description
Checks if dynamic system model is in continuous time.
Example
A = [-15,-20; 10, 0];
B = [5; 0];
C = [0, 1];
D = 0;
sys1 = ss(A, B, C, D);
isct(sys1)
sys2 = ss(A, B, C, D, 0.2);
isct(sys2)
See also
isdt.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
isdt
Checks if dynamic system model is in discret time.
Syntax
- res = isdt(sys)
Input argument
- sys - a lti model.
Output argument
- res - a logical: true if dynamic system model is in discret time.
Description
Checks if dynamic system model is in discret time.
Example
A = [-15,-20; 10, 0];
B = [5; 0];
C = [0, 1];
D = 0;
sys1 = ss(A, B, C, D);
isdt(sys1)
sys2 = ss(A, B, C, D, 0.2);
isdt(sys2)
See also
isct.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
islti
Checks if variable is an linear model tf, ss or zpk.
Syntax
- res = islti(sys)
Input argument
- A - variable.
Output argument
- res - a logical: true if it is an linear model.
Description
Checks if variable is an linear model tf, ss or zpk.
Example
A = [-15,-20; 10, 0];
B = [5; 0];
C = [0, 1];
D = 0;
sys = ss(A, B, C, D);
islti(sys)
islti(A)
See also
isa.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
issiso
Checks if dynamic system model is single input and single output.
Syntax
- res = issiso(sys)
Input argument
- sys - a lti model.
Output argument
- res - a logical: true if dynamic system model is single input and single output.
Description
Checks if dynamic system model is single input and single output.
Example
A = [-15,-20; 10, 0];
B = [5; 0];
C = [0, 1];
D = 0;
sys = ss(A, B, C, D);
issiso(sys)
A = [1 2; 3 4];
B = [1 0; 0 1];
C = [1 1; 1 1];
D = [0 0; 0 0];
sys = ss(A, B, C, D);
issiso(sys)
See also
isdt.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
isstatic
Checks if model is static or dynamic.
Syntax
- res = isdt(sys)
Input argument
- sys - a lti model.
Output argument
- res - a logical: true if model is static.
Description
Checks if model is static.
Example
sys = tf(magic(3));
isstatic(sys)
See also
isct.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
kalman
Design Kalman filter for state estimation.
Syntax
- [kalmf, L, P, M, Z] = kalman(sys, Q, R, N)
- [kalmf, L, P, M, Z] = kalman(sys, Q, R, N, sensors, known)
Input argument
- sys - Plant model with process noise: state-space model.
- Q - Process noise covariance: scalar or matrix.
- R - Measurement noise covariance: scalar or matrix.
- N - Noise cross covariance: scalar or matrix.
- sensors - Measured outputs of sys: vector.
- known - Known inputs of sys: vector.
Output argument
- kalmf - Kalman estimator: state-space model
- L - Filter gains: matrix
- P - Steady-state error covariances: matrix
- M - Innovation gains of state estimators: matrix
- Z - Steady-state error covariances: matrix
Description
[kalmf, L, P] = kalman(sys, Q, R, N) generates a Kalman filter using the provided plant model sys and noise covariance matrices Q, R, and N.
The function calculates a Kalman filter suitable for application in a Kalman estimator, as depicted in the following diagram.
Example
A = [11.269 -0.4940 1.129; 1.0000 0 0;0 1.0000 0];
B = [-0.3832; 0.5919; 0.5191];
C = [1 0 0];
sys = ss(A,[B, B], C, 0);
Q = 1;
R = 1;
[kEst, l, p, m, z] = kalman(sys, Q, R, [])
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
lqe
Kalman estimator design for continuous-time systems.
Syntax
- [L, P, E] = lqe(A, G, C, Q, R, N)
- [L, P, E] = lqe(A, G, C, Q, R)
Input argument
- A - State matrix: n x n matrix.
- G - Defines a matrix linking the process noise to the states.
- C - The output matrix, with dimensions (q x n), where q is the number of outputs.
- Q - State-cost weighted matrix
- R - Input-cost weighted matrix
- N - Optional cross term matrix: 0 by default.
Output argument
- L - Kalman gain matrix.
- P - Solution of the Discrete Algebraic Riccati Equation.
- E - Closed-loop pole locations
Description
The function computes the optimal steady-state feedback gain matrix, denoted as L, minimizing a quadratic cost function for a linear discrete state-space system model.
Example
c = 1;
m = 1;
k = 1;
A = [0, 2; -k/m, -c/m];
B = [0; 2/m];
G = [2 0 ; 0 2];
C = [2 0];
Q = [0.02 0; 0 0.02];
R = 0.02;
[l, p, e] = lqe(A, G, C, Q, R)
See also
lqr.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
lqed
Calculates the discrete Kalman estimator configuration based on a continuous cost function.
Syntax
- [L, P, Z, E] = LQED(A, G, C, Q, R, Ts)
Input argument
- A - State matrix: n x n matrix.
- G - Defines a matrix linking the process noise to the states.
- C - The output matrix, with dimensions (q x n), where q is the number of outputs.
- Q - State-cost weighted matrix
- R - Input-cost weighted matrix
- N - Optional cross term matrix: 0 by default.
- Ts - sample time: scalare.
Output argument
- L - Kalman gain matrix.
- P - Solution of the Discrete Algebraic Riccati Equation.
- E - Closed-loop pole locations
- Z - Discrete estimator poles
Description
[L, P, Z, E] = LQED(A, G, C, Q, R, Ts) Calculates the discrete Kalman gain matrix L to minimize the discrete estimation error, equivalent to the estimation error in the continuous system.
Example
A = [10 1.2; 3.3 4];
B = [5 0; 0 6];
C = B;
D = [0,0;0,0];
R = [2,0;0,3];
Q = [5,0;0,4];
G = [6,0;0,7];
Ts = 0.004;
[L, P, Z, E] = lqed(A, G, C, Q, R, Ts)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
lqr
Linear-Quadratic Regulator (LQR) design.
Syntax
- [K, S, P] = lqr(sys, Q, R, N)
- [K, S, P] = lqr(A, B, Q, R, N)
Input argument
- sys - LTI model
- Q - State-cost weighted matrix
- R - Input-cost weighted matrix
- N - Optional cross term matrix: 0 by default.
- A - State matrix: n x n matrix.
- B - Input-to-state matrix: n x m matrix.
Output argument
- K - Optimal gain: row vector.
- S - Solution of the Algebraic Riccati Equation.
- p - Poles of the closed-loop system: column vector.
Description
In the context of continuous-time state-space matrices A and B, the command [K, S, P] = lqr(A, B, Q, R, N) computes the optimal gain matrix K, the solution S to the associated algebraic Riccati equation, and the closed-loop poles P.
This syntax is applicable exclusively to continuous-time models.
When applied to a continuous-time or discrete-time state-space model represented by sys, the command [K, S, P] = lqr(sys, Q, R, N) computes the optimal gain matrix K, the solution S to the associated algebraic Riccati equation, and the closed-loop poles P.
The weight matrices Q and R govern the importance of states and inputs, and the cross term matrix N is zero by default when not specified.
Example
A = [-0.313 56.7 0; -0.0139 -0.426 0; 0 56.7 0];
B = [0.232; 0.0203; 0];
C = [0 0 1];
D = 1;
Ts = 1.2;
sys1 = ss(A, B, C, D, Ts);
sys2 = ss(A, B, C, D);
P = 2;
Q = P * C' * C;
R = 2;
[K1, S1, e1] = lqr(sys1, Q, R)
[K2, S2, e2] = lqr(sys2, Q, R)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
lqry
Form linear-quadratic (LQ) state-feedback regulator with output weighting.
Syntax
- [K, S, e] = lqry(sys, Q, R, N)
Input argument
- sys - LTI model
- Q - State-cost weighted matrix
- R - Input-cost weighted matrix
- N - Optional cross term matrix: 0 by default.
Output argument
- K - Optimal gain: row vector.
- S - Solution of the Algebraic Riccati Equation.
- e - Poles of the closed-loop system: column vector.
Description
The function lqry computes and returns the optimal gain matrix (K), the Riccati solution (S), and the closed-loop eigenvalues (e) for a given state-space model (sys) with specified weights (Q, R, N).
The plant data is defined by the matrices A, B, C, and D, representing continuous- or discrete-time dynamics.
If the parameter N is not provided, it defaults to N=0.
The closed-loop eigenvalues are determined by the eigenvalues of the matrix A - B * K.
Example
A = [0.6, 0.25; 0, 0.9];
B = [0; 10];
C = [11, 0];
D = 0;
Q = 2;
R = 1;
[K, S, e] = lqry(A, B, C, D, Q, R)
See also
lqr.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
lsim
Plot simulated time response of dynamic system to arbitrary inputs.
Syntax
- lsim(sys, u, t)
- lsim(sys, u, t, x0)
- [y, tOut, x] = lsim(SYS, U, T, X0)
Input argument
- sys - a lti model.
- u - Input signal: matrix or vector.
- t - Time samples: vector.
- x0 - Initial state values: vector.
Output argument
- y - Simulated response data: matrix or vector.
- tOut - Time vector: vector.
- x - State trajectories: matrix or vector.
Description
The function lsim(sys, u, t) generates a plot illustrating the simulated time response of the dynamic system model sys to the input history (t, u).
The time samples for the simulation are specified by the vector t.
In the case of single-input systems, the input signal u is a vector with the same length as t.
For multi-input systems, u is an array with rows corresponding to time samples (length(t)) and columns corresponding to inputs to sys.
An additional usage of the function is demonstrated by the example lsim(sys, u, t, x0), where a vector x0 is provided to specify initial state values.
This is particularly relevant when sys is a state-space model.
Examples
A = [-10 -20 -30;1 0 0; 0 1 0];
B = [1; 0; 0];
C = [0 0 1];
D = 0;
T = [0:0.1:1];
U = zeros(size(T, 1), size(T, 2));
X0 = [0.1 0.1 0.1];
sys = ss(A, B, C, D);
lsim(sys, U, T, X0);
A = [-1.7 -0.3 1.1;
-0.2 -1.7 0.6;
1.0 0.6 -1.4];
B = [ 1.5 0.6;
-1.8 1.0;
0 0 ];
C = [ 0 -0.5 -0.1;
0.35 -0.1 -0.15
0.65 0 0.6];
D = [ 0.5 0;
0.05 0.75
0 0];
sys = ss(A,B,C,D);
Tf = 10;
Ts = 0.1;
[uSq,t] = gensig("square",4,Tf,Ts);
uP = gensig("pulse",3,Tf,Ts);
u = [uSq uP];
lsim(sys,u,t)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
lyap
Continuous Lyapunov equation solution.
Syntax
- X = lyap(A, Q)
Input argument
- A - real matrix
- Q - real matrix
Output argument
- X - matrix: solution of the Lyapunov equation.
Description
X = lyap(A, Q) resolves the Lyapunov equation.
Example
A = [10, 20; -30, -40];
Q = [30, 10; 10, 10];
X = lyap (A, Q)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
minreal
Minimal realization or pole-zero cancellation.
Syntax
- [Am, Bm, Cm, Dm] = minreal(A, B, C, D)
- [Am, Bm, Cm, Dm] = minreal(A, B, C, D, tol)
- sysOut = minreal(sysIn)
- sysOut = minreal(sysIn, tol)
Input argument
- A (n x n) - Represents the system's state-transition matrix. It describes how the system's internal state evolves over time.
- B (n x m) - Describes the input-to-state mapping. It shows how control inputs affect the change in the system's state.
- C (p x n) - Represents the state-to-output mapping. It shows how the system's state variables are related to the system's outputs.
- D (p x m) - Describes the direct feedthrough from inputs to outputs. In many systems, this matrix is zero because there is no direct feedthrough.
- tol - scalar real (tolerance).
- sysIn - LTI model.
Output argument
- Am, Bm, Cm, Dm - a minimal realization of the state-space system A, B, C, D.
- sysOut - a minimal realization of LTI input.
Description
minreal function reduces state-space models by eliminating uncontrollable or unobservable states.
In transfer functions or zero-pole-gain models, it cancels pole-zero pairs. The resulting model maintains the same response characteristics as the original model but with minimal order.
When using sysOut = minreal(sysIn, tol), you can customize the tolerance for state elimination or pole-zero cancellation.
The default tolerance is set to sqrt(eps), and increasing this value prompts more aggressive cancellations, potentially further simplifying the model.
Example
sysIn = ss([1 0;0 -2], [-1;0], [2 1], 0, 3.2);
sysOut = minreal(sysIn)
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
nyquist
Nyquist plot of frequency response.
Syntax
- nyquist(sys)
- nyquist(sys, w)
- [re, im, wout] = nyquist(sys)
- [re, im, wout] = nyquist(sys, w)
Input argument
- sys - Dynamic system
- w - Frequencies: vector or {wmin,wmax}
Output argument
- re - Real part of system response
- im - Imaginary part of system response
- wout - Frequencies
Description
The Nyquist function, nyquist(sys), generates a graphical representation known as a Nyquist plot, illustrating the frequency response of a dynamic system model represented by sys.
This plot visualizes both the real and imaginary components of the system's response across varying frequencies.
The contour depicted by nyquist encompasses both positive and negative frequencies.
Additionally, the plot incorporates arrows that signify the direction of increasing frequency for each branch.
Examples
f = figure();
sys = tf([1, 1, 3, 3], [1, -3, 3, -1])
nyquist(sys);
H = tf([2 5 1], [1 2 3]);
[re, im, wout] = nyquist(H);
f = figure();
H = tf([2 5 1], [1 2 3]);
nyquist(H);
See also
bode.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
obsv
Observability of state-space model.
Syntax
- Ob = obsv(A, C)
- Ob = obsv(sys)
Input argument
- sys - State-space model
- A - State matrix: Nx-by-Nx matrix
- C - State-to-output matrix: Ny-by-Nx matrix
Output argument
- Ob - Observability matrix.
Description
The obsv functionis designed to calculate the observability matrix for state-space systems.
Given an Nx-by-Nx matrix A representing the system dynamics and a Ny-by-Nx matrix C specifying the output, the function call obsv(A, C) generates the observability matrix.
It is advised against using the rank of the observability matrix for testing observability due to numerical instability.
The observability matrix Ob tends to be numerically singular for systems with more than a few states, making the rank-based approach unreliable for such cases.
Example
% Define the system matrices
A = [1 2; 3 4];
C = [7 8];
% Check observability using obsv function
O = obsv(A, C);
% Display the observability matrix
disp('Observability matrix:');
disp(O);
% Check if the system is observable
if rank(O) == size(A, 1)
disp('The system is observable.');
else
disp('The system is not observable.');
end
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
obsvf
Compute observability staircase form.
Syntax
- [Abar, Bbar, Cbar, T, k] = obsvf(A, B, C)
- [Abar, Bbar, Cbar, T, k] = obsvf(A, B, C, tol)
Input argument
- A - State matrix: Nx-by-Nx matrix
- B - Input-to-state matrix: Nx-by-Nu matrix
- C - Output-to-state matrix: Ny-by-Nx matrix
- tol - scalar real (tolerance).
Output argument
- Abar - Observability staircase state matrix.
- Bbar - Observability staircase input matrix.
- Cbar - Observability staircase output matrix.
- T - Similarity transform matrix.
- k - Vector: number of observable states.
Description
obsvf(A, B, C) decomposes the given state-space system, characterized by matrices A, B, and C, into the observability staircase form, resulting in transformed matrices Abar, Bbar, and Cbar.
It also provides a similarity transformation matrix T and a vector k.
The length of vector k corresponds to the number of states in A, and each entry in k signifies the number of observable states factored out at each step of the transformation matrix computation.
The non-zero elements in k indicate the number of iterations needed for T calculation, and the sum of k represents the number of states in Ao, the observable portion of Abar.
Example
A = [-1.5 -0.5; 1 0];
B = [0.5; 0];
C = [0 1];
[Abar, Bbar, Cbar, T, k] = obsvf(A, B, C)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
ord2
Generate continuous second-order systems.
Syntax
- [A, B, C, D] = ord2(wn, z)
- [num, den] = ord2(wn, z)
Input argument
- wn - natural frequency
- z - damping factor
Output argument
- A - State matrix: Nx-by-Nx matrix.
- B - Input-to-state matrix: Nx-by-Nu matrix.
- C - State-to-output matrix: Ny-by-Nx matrix.
- D - Feedthrough matrix: Ny-by-Nu matrix.
- num - polynomial coefficients: a row vector or as a cell array of row vectors.
- den - polynomial coefficients: a row vector or as a cell array of row vectors.
Description
ord2 offers a convenient way to obtain either the state-space representation or the transfer function of a second-order system based on its natural frequency and damping factor.
Example
wn = 5;
z = 0.7;
[A, B, C, D] = ord2(wn, z);
sys1 = ss(A, B, C, D)
[num, den] = ord2(wn, z);
sys2 = tf(num, den)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
padecoef
Computes the Pade approximation of time delays.
Syntax
- [numerator, denominator] = padecoef(T, N)
- [numerator, denominator] = padecoef(T)
Input argument
- T - Time delay: a scalar real positive.
- N - Order of approximation: a scalar real positive (default N = 1).
Output argument
- numerator - polynomials of order N: rows vector.
- denominator - polynomials of order N: rows vector.
Description
padecoef(T, N) computes the Nth-order Padé Approximation for the continuous-time delay system represented by the exponential term exp(-T*s) and returns it in the form of a transfer function.
Bibliography
http://en.wikipedia.org/wiki/Pad%C3%A9_approximant and Golub and Van Loan, Matrix Computations, Johns Hopkins University Press (Third edition, page 562).
Example
T = 2; N = 4;
[numerator, denominator] = padecoef(T, N)
See also
tf.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
parallel
Parallel connection of two models.
Syntax
- sys = parallel(sys1, sys2)
- sys = parallel(sys1, sys2)
Input argument
- sys1, sys2 - LTI models.
Output argument
- sys - LTI model.
Description
parallel function links two model objects in parallel.
It is versatile and can accept various types of models.
However, for successful connection, both systems must share the same nature, being either continuous or discrete, and must have identical sample times.
Static gains are treated as neutral and can be defined using regular matrices.
Example
sys1 = tf([1 4], [8 2 1]);
sys2 = tf(1, [8 2 1]);
sys = parallel(sys2, sys2)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
pole
Poles of dynamic system.
Syntax
- P = pole(sys)
Input argument
- sys - a LTI model.
Output argument
- P - Poles of dynamic system.
Description
P = pole(sys) returns the poles of sys.
Example
A = [-15, -20; 10, 0];
B = [5; 0];
C = [0, 10];
D = 0;
sys = ss(A, B, C, D);
P = pole(sys)
See also
zero.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
series
Series connection of two models.
Syntax
- sys = series(sys1, sys2)
- sys = series(sys1, sys2, outputs1, inputs2)
Input argument
- sys1, sys2 - LTI models.
- outputs1 - index vectors
- inputs2 - index vectors
Output argument
- sys - LTI model.
Description
series function links two model objects in a sequential manner.
It is versatile and can accept various types of models.
However, for successful connection, both systems must share the same nature, being either continuous or discrete, and must have identical sample times.
Static gains are treated as neutral and can be defined using regular matrices.
Example
[A, B, C, D] = ord2(1, 3);
sys1 = ss(A, B, C, D);
[A, B, C, D] = ord2(3, 6);
sys2 = ss(A, B, C, D)
outputs1 = 1;
inputs2 = 1;
sys = series(sys1, sys2, outputs1, inputs2)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
ss
State-space model.
Syntax
- sys = ss(A, B, C, D)
- sys = ss(A, B, C, D, TS)
- sys = ss(D)
- sys = ss(sysIn)
Input argument
- A - State matrix: Nx-by-Nx matrix.
- B - Input-to-state matrix: Nx-by-Nu matrix.
- C - State-to-output matrix: Ny-by-Nx matrix.
- D - Feedthrough matrix: Ny-by-Nu matrix.
- TS - Sample time: scalar.
- sysIn - SISO LTI model.
Output argument
- sys - Output state space system model.
Description
Creates a continuous-time state-space model using matrices A, B, C, and D, allowing for either real or complex-valued matrices.
This model is represented as sys = ss(A, B, C, D).
Examples
A = [-15,-20; 10, 0];
B = [5; 0];
C = [0, 1];
D = 0;
sys = ss(A, B, C, D)
num = [3 4];
den = [3 1 5];
Ts = 0.2;
sysIn = tf(num, den, Ts)
sys = ss(sysIn)
See also
tf.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
ss2tf
Convert state-space representation to transfer function.
Syntax
- [b, a] = ss2tf(A, B, C, D)
- [b, a] = ss2tf(A, B, C, D, ni)
Input argument
- A (n x n) - Represents the system's state-transition matrix. It describes how the system's internal state evolves over time.
- B (n x m) - Describes the input-to-state mapping. It shows how control inputs affect the change in the system's state.
- C (p x n) - Represents the state-to-output mapping. It shows how the system's state variables are related to the system's outputs.
- D (p x m) - Describes the direct feedthrough from inputs to outputs. In many systems, this matrix is zero because there is no direct feedthrough.
- ni - Input index:integer scalar or 1 (default).
Output argument
- b - Transfer function numerator coefficients: vector or matrix.
- a - Transfer function denominator coefficients: vector.
Description
[b, a] = ss2tf(A, B, C, D) transforms a state-space representation of a system into an equivalent transfer function.
The function ss2tf returns the Laplace-transform transfer function for continuous-time systems and the Z-transform transfer function for discrete-time systems.
[b, a] = ss2tf(A, B, C, D, ni) computes the transfer function resulting from exciting the nith input of a system with multiple inputs using a unit impulse.
Example
Fs = 16;
dt = 1/Fs;
Ac = [0 1 0 0; -2 0 1 0; 0 0 0 1; 1 0 -2 0];
A = expm(Ac*dt);
Bc = [0 0; 1 0; 0 0; 0 1];
B = Ac\(A-eye(4))*Bc;
C = [-2 0 1 0; 1 0 -2 0];
D = eye(2);
[b, a] = ss2tf(A, B, C, D, 2)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
ssdata
Access state-space model data.
Syntax
- [A, B, C, D] = ssdata(sys)
- [A, B, C, D, Ts] = ssdata(sys)
Input argument
- sys - LTI model.
Output argument
- A - State matrix: Nx-by-Nx matrix.
- B - Input-to-state matrix: Nx-by-Nu matrix.
- C - State-to-output matrix: Ny-by-Nx matrix.
- D - Feedthrough matrix: Ny-by-Nu matrix.
- TS - Sample time: scalar.
Description
The function ssdata(sys) retrieves the matrix data A, B, C, D from the state-space model (LTI array) represented by sys.
If sys is initially in the form of a transfer function or zero-pole-gain model (LTI array), it is automatically converted to the state-space representation before extracting the matrix data.
Example
sysIn = ss([1 0;0 -2], [-1;0], [2 1], 0, 3.2);
[a, b, c, d, Ts] = ssdata(sysIn)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
ssdelete
Remove inputs, outputs and states from state-space system.
Syntax
- sysOut = ssselect(sysIn, INPUTS, OUTPUTS)
- sysOut = ssselect(sysIn, INPUTS, OUTPUTS, STATES)
Input argument
- sysIn - state-space model
- INPUTS - indexes into the system inputs
- OUTPUTS - indexes into the system outputs
- STATES - states removed from the system.
Output argument
- sysOut - state-space model: subsystem with removed inputs, outputs and states.
Description
ssdelete removes inputs, outputs and states from state-space system.
Example
A = [33,2,5; 23,200,2; 9,2,45];
B = [4,5; 12,5; 82,1];
C = [34,56,2; 6,2,112];
D = [2,0; 0,19];
sys1 = ss(A, B, C, D);
inputs = 1;
outputs = 1;
R = ssdelete(sys1, inputs, outputs)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
ssselect
Extract subsystem from larger system.
Syntax
- sysOut = ssselect(sysIn, INPUTS, OUTPUTS)
- sysOut = ssselect(sysIn, INPUTS, OUTPUTS, STATES)
Input argument
- sysIn - state-space model
- INPUTS - indexes into the system inputs
- OUTPUTS - indexes into the system outputs
- STATES - specified states
Output argument
- sysOut - state-space model: subsystem from larger system.
Description
ssselect extracts subsystem from larger system.
Example
A = [33,2,5; 23,200,2; 9,2,45];
B = [4,5; 12,5; 82,1];
C = [34,56,2; 6,2,112];
D = [2,0; 0,19];
sys1 = ss(A, B, C, D)
inputs = 1;
outputs = 1;
R = ssselect(sys1, inputs, outputs)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
step
Step response plot of dynamic system.
Syntax
- [y, t, x] = step(sys)
- [y, t, x] = step(sys, t)
- [y, t, x] = step(sys, tFinal)
- [y, t, x] = step(sys, [t0, tFinal])
Input argument
- sys - a lti model.
- t - Time vector.
- tFinal - End time for step response: scalar.
- [t0, tFinal] - Time range for step response: two-element vector.
Output argument
- y - Simulated response data: matrix or vector.
- t - Time vector: vector.
- x - State trajectories: matrix or vector.
Description
The function defaults to applying a step at t0 = 0 with initial conditions U = 0, dU = 1, and td = 0.
The step function, used as [y, tOut] = step(sys), calculates the step response (y) of the dynamic system sys.
The time vector tOut is in the time units of sys, and the function automatically determines the time steps and simulation duration based on the system dynamics.
If you use [y, tOut] = step(sys, tFinal), the step response is computed from t = 0 to the specified end time t = tFinal.
Similarly, [y, tOut] = step(sys, [t0, tFinal]) computes the step response from t0 to tFinal.
Example
A = [-10 -20 -30;1 0 0; 0 1 0];
B = [1; 0; 0];
C = [0 0 1];
D = 0;
T = [0:0.1:1];
U = zeros(size(T, 1), size(T, 2));
X0 = [0.1 0.1 0.1];
sys = ss(A, B, C, D);
step(sys);
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
tf
Constructs a transfer function model.
Syntax
- sys = tf()
- sys = tf('s')
- sys = tf(numerator, denominator)
- sys = tf(numerator, denominator, Ts)
Input argument
- numerator - polynomial coefficients: a row vector or as a cell array of row vectors.
- denominator - polynomial coefficients: a row vector or as a cell array of row vectors.
- Ts - Sampling time Ts, default: in seconds
- sysIn - LTI model.
Output argument
- sys - Output tranfer function system model.
Description
sys = tf(numerator, denominator) is used to create a continuous-time transfer function model.
It is defined by specifying numerator and denominator of the transfer function.
When you include the Ts parameter, it allows you to create a discrete-time transfer function.
Setting Ts to -1 indicates an unspecified sampling time, and, in this scenario, the input arguments are treated as if they pertain to a continuous-time system.
Examples
numerator = 10;
denominator = [20, 33, 44];
sys = tf(numerator, denominator)
numerator = 10;
denominator = [20, 33, 44];
Ts = 1.5;
sys = tf(numerator, denominator, Ts)
See also
ss.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
tf2ss
Convert transfer function filter parameters to state-space form.
Syntax
- [A, B, C, D] = tf2ss(b, a)
Input argument
- b - Transfer function numerator coefficients: vector or matrix.
- a - Transfer function denominator coefficients: vector.
Output argument
- A (n x n) - Represents the system's state-transition matrix. It describes how the system's internal state evolves over time.
- B (n x m) - Describes the input-to-state mapping. It shows how control inputs affect the change in the system's state.
- C (p x n) - Represents the state-to-output mapping. It shows how the system's state variables are related to the system's outputs.
- D (p x m) - Describes the direct feedthrough from inputs to outputs. In many systems, this matrix is zero because there is no direct feedthrough.
Description
[A, B, C, D] = tf2ss(b, a) transforms a single-input transfer function, either continuous-time or discrete-time, into an equivalent state-space representation.
Example
Fs = 6;
dt = 1/Fs;
b = [1 -(1+cos(dt)) cos(dt)];
a = [1 -3*cos(dt) 1];
[A, B, C, D] = tf2ss(b, a)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
tfdata
Access transfer function model data.
Syntax
- [numerator, denominator] = tfdata(sys)
- [numerator, denominator, Ts] = tfdata(sys)
- sys = tf(numerator, denominator)
- sys = tf(numerator, denominator, Ts)
Input argument
- sys - a LTI model.
Output argument
- numerator - polynomial coefficients: a row vector or as a cell array of row vectors.
- denominator - polynomial coefficients: a row vector or as a cell array of row vectors.
- Ts - Sampling time Ts, default: in seconds
Description
The function tfdata(sys) retrieves the matrix data numerator, denominator from the transfer function model (LTI array) represented by sys.
If sys is initially in the form of a state-space model (LTI array), it is automatically converted to the transfer function representation before extracting the matrix data.
Example
numerator = 10;
denominator = [20, 33, 44];
sys = tf(numerator, denominator)
[num, den] = tfdata(sys)
See also
tf.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
tzero
Invariant zeros of linear system.
Syntax
- z = tzero(sys)
- z = tzero(A, B, C, D)
- z = tzero(A, B, C, D, E)
- [z, nrank] = tzero(sys)
- [z, nrank] = tzero(A, B, C, D)
- [z, nrank] = tzero(A, B, C, D, E)
Input argument
- sys - a LTI model.
- A - State matrix: Nx-by-Nx matrix.
- B - Input-to-state matrix: Nx-by-Nu matrix.
- C - State-to-output matrix: Ny-by-Nx matrix.
- D - Feedthrough matrix: Ny-by-Nu matrix.
- E - Nx-by-Nx matrix.
Output argument
- Z - Invariant zeros: column vector.
- nrank - Normal rank: positive integer.
Description
tzero function is employed to extract the invariant zeros of a Multiple Input, Multiple Output (MIMO) dynamic system described by the system model sys.
In cases where sys is a minimal realization, these invariant zeros coincide with the transmission zeros of the system.
Example
A = [1 2; 3 4];
B = [1; 0];
C = [1 0];
D = 0;
sys = ss(A, B, C, D);
z = tzero(sys)
[z, nrank] = tzero(sys)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
zero
Zeros and gain of SISO dynamic system.
Syntax
- Z = zero(sys)
- [Z, gain] = zero(sys)
Input argument
- sys - a LTI model.
Output argument
- Z - Zeros of the dynamic system.
- gain - Zero-pole-gain of the dynamic system.
Description
[Z, gain] = zero(sys) returns the zero-pole-gain of sys.
Example
sys = tf([4.2,0.25,-0.004],[1,9.6,17]);
[Z, gain] = zero(sys)
See also
pole.
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
Python engine
Python engine
Description
python_engine functions
- The power of calling Python from Nelson
- How to install python package
- Python Nelson types - Managing Data between Python and Nelson.
- Python operators - The representation of Python operators in Nelson.
- pyargs - Change default environment of Python interpreter.
- pyenv - Change default environment of Python interpreter.
- pyrun - Run Python statements from Nelson.
- pyrunfile - Run Python file from Nelson.
The power of calling Python from Nelson
Description
Python is a dominant force in various fields, including machine learning, data science, and general programming.
Its extensive libraries, such as NumPy, SciPy and Pandas, provide an arsenal of tools for scientific computing and data manipulation.
Combining the strengths of Nelson’s digital capabilities with Python’s vast ecosystem opens up a world of possibilities.
Why call Python from Nelson?
- Access to a rich ecosystem: Python has a vast collection of libraries for various tasks, from advanced statistical analysis to machine learning and beyond.
By calling Python from Nelson, users can access this rich ecosystem without sacrificing Nelson's core functionality.
- Seamless integration: Nelson's ability to call external functions allows for seamless integration with Python.
This means Nelson users can leverage Python capabilities within their familiar Nelson environment, improving productivity and workflow efficiency.
- Specialized Libraries: Although Nelson offers a wide range of built-in functions, there are some niche tasks that Python libraries excel at.
For example, deep learning tasks can be handled efficiently using Python libraries such as TensorFlow or PyTorch, seamlessly integrated into Nelson workflows.
- Rapid prototyping and development: Python is often preferred for rapid prototyping and development due to its concise syntax and extensive library support.
By leveraging Python from Nelson, users can leverage Python's rapid prototyping capabilities while maintaining Nelson's digital computing environment for production-level work.
- Community Collaboration: By integrating Python into Nelson workflows, users can leverage the collective wisdom of both communities, fostering collaboration and innovation.
In conclusion, calling Python from Nelson offers a powerful synergy that combines the strengths of both platforms.
Whether accessing specialized libraries, prototyping algorithms, or fostering collaboration, integrating Python into Nelson workflows can improve productivity and open up new possibilities for scientific computing and data analysis.
See also
How to install python package
Description
Nelson allows users to seamlessly integrate Python packages into their workflows.
Installing Python packages within Nelson expands its functionality and enables users to leverage a wide array of libraries for data analysis, machine learning, scientific computing, and more.
This help file provides a comprehensive guide on installing Python packages from within Nelson.
Tips and Considerations:
- Package Availability: Ensure that the Python package you intend to install is available on the Python Package Index (PyPI) or another compatible repository.
- Dependencies: Some Python packages may have dependencies on other packages. Make sure to install any required dependencies beforehand.
- Version Compatibility: Check the compatibility of the package with your current Python environment and Nelson version to avoid compatibility issues.
- Virtual Environments: Consider using virtual environments within Nelson to isolate package installations and manage dependencies for different projects.
- Permission Rights: When installing packages that require write access to the Python directory, ensure that you have the necessary permissions. On some systems, administrative privileges may be required to install packages globally. If you encounter permission errors, consider using a virtual environment or contacting your system administrator for assistance.
Examples
Get info from the pyenv environment:
pe = pyenv
Construct the command to install the package:
package_to_install = "scipy";
command_to_install = '"' + pe.Executable + '"' + " -m pip install " + package_to_install;
Construct the command to install the package:
[status, msg] = system(command_to_install);
See also
Python Nelson types
Managing Data between Python and Nelson.
Description
Managing data returned by Python functions:
Python return type, as shown in Python | Corresponding Nelson type (scalar) |
---|---|
bool | logical |
complex | double (complex) |
float | double |
Convert Python types to Nelson type explicitly:
Python return types or protocols shown in Nelson | Nelson conversion methods |
---|---|
py.str | char, string |
py.int | double, single, int8, uint8, int16, uint16, int32, uint32, int64, uint64 |
py.long | double, single, int8, uint8, int16, uint16, int32, uint32, int64, uint64 |
py.float | double, single, int8, uint8, int16, uint16, int32, uint32, int64, uint64 |
py.bool | double, single, int8, uint8, int16, uint16, int32, uint32, int64, uint64, logical |
py.bytes | double, single, int8, uint8, int16, uint16, int32, uint32, int64, uint64, logical |
py.bytearray | double, single, int8, uint8, int16, uint16, int32, uint32, int64, uint64, logical |
py.array.array | double, single, int8, uint8, int16, uint16, int32, uint32, int64, uint64 |
py.memoryview | double, single, int8, uint8, int16, uint16, int32, uint32, int64, uint64 |
py.numpy.ndarray | double, single, int8, uint8, int16, uint16, int32, uint32, int64, uint64 |
py.list | double, single, int8, uint8, int16, uint16, int32, uint32, int64, uint64, logical, string, cell |
py.tuple | double, single, int8, uint8, int16, uint16, int32, uint32, int64, uint64, logical, string, cell |
py.dict | struct |
Pass scalar Nelson type to Python:
Nelson scalar input argument type | Python type |
---|---|
NaN | float("nan") |
Inf | float("inf") |
double (real) | py.float |
single (real) | py.float |
double (complex) | py.complex |
single (complex) | py.complex |
int8 | py.int |
uint8 | py.int |
int16 | py.int |
uint16 | py.int |
int32 | py.int |
uint32 | py.int |
int64 | py.int |
uint64 | py.int |
string scalar | py.str |
char vector | py.str |
logical | py.bool |
struct | py.dict |
Pass 1-by-N Vector Nelson type to Python:
Nelson 1-by-N Vector input argument type | Python type |
---|---|
double (real) | array.array('d') |
single (real) | array.array('f') |
int8 | array.array('b') |
uint8 | array.array('B') |
int16 | array.array('h') |
uint16 | array.array('H') |
int32 | array.array('i') |
uint32 | array.array('I') |
int64 | array.array('q') |
uint64 | array.array('Q') |
double | memoryview |
single | memoryview |
logical | memoryview |
char vector | str |
string scalar | str |
cell vector | tuple |
Pass 2D Matrices and ND Arrays to Python:
The Python language offers a protocol for accessing memory buffers, akin to the data stored in Nelson arrays.
Nelson incorporates this Python buffer protocol for its arrays.
Examples
R = pyrun('', "A", 'A', magic(3))
R.double()
dictionary conversion nelson -- python
wheels = [1 2 3];
names = ["Monocycle" "Bicycle" "Tricycle"];
d = dictionary(wheels, names)
R = pyrun("A = d", "A", 'd', d)
dictionary(R)
See also
History
Version | Description |
---|---|
1.4.0 | initial version |
Author
Allan CORNET
Python operators
The representation of Python operators in Nelson.
Description
Nelson facilitates the utilization of the subsequent overloaded operators:
Python Operator Symbol | Python Methods | Nelson Methods |
---|---|---|
- (unary operator) | __neg__ | uminus, -a |
+ (unary operator) | __pos__ | uplus, +a |
+ (binary operator) | __add__, __radd__ | plus, + |
- (binary operator) | __sub__, __rsub__ | minus, - |
* (binary operator) | __mul__, __rmul__ | mtimes, * |
/ (binary operator) | __truediv__, __rtruediv__ | mrdivide, / |
== (binary operator) | __eq__ | eq, == |
> (binary operator) | __gt__ | gt, > |
< (binary operator) | __lt__ | lt, < |
!= (binary operator) | __ne__ | ne, ~= |
>= (binary operator) | __ge__ | ge, >= |
<= (binary operator) | __le__ | le, <= |
isequal builtin is also overloaded to manage python type.
For numpy types, isequal call numpy.array_equal from python.
Others python operators are currently not supported.
Example
pyrun('import numpy as np')
R = pyrun('R = np.asarray(A)', "R", 'A', magic(3))
R_A = R + R
R_B = R * 2
isequal(R_A, R_B)
See also
History
Version | Description |
---|---|
1.5.0 | initial version |
Author
Allan CORNET
pyargs
Change default environment of Python interpreter.
Syntax
- pyargs
- pa = pyargs(Name, Value)
Input argument
- Name - a string, or row characters array
- Value - variable value
Output argument
- pa - pyargs object.
Description
pyargs(Name, Value, ...) generates one or multiple keyword arguments for Python functions.
In Python, a keyword argument is a value associated with an identifier.
Ensure to position pyargs as the last input argument when calling a Python function.
Example
pa = pyargs('A', 1)
See also
History
Version | Description |
---|---|
1.3.0 | initial version |
Author
Allan CORNET
pyenv
Change default environment of Python interpreter.
Syntax
- pyenv
- pe = pyenv('Version', python_path)
- pe = pyenv(...)
Input argument
- python_path - a string, or row characters array: executable file name of Python or version (on Windows).
Output argument
- pe - PythonEnvironment object.
Description
Use pyenv to modify the default version or execution mode of the Python interpreter, ensuring these adjustments persist across various Nelson sessions.
The value set by pyenv is persistent across Nelson sessions.
Properties:
Version: string: Python version
Executable: string: Name of Python executable file
Library: string: Shared library file
Home: string: Home folder
Status: Process status: "NotLoaded" (default), "Loaded", "Terminated"
ExecutionMode: Execution mode: "InProcess" (default) or "OutOfProcess"
Use environment variables to force python environment at each startup (usefull for snapcraft or docker distribution):
__NELSON_PYTHON_VERSION__: example "3.10"
__NELSON_PYTHON_EXECUTABLE__: example "/usr/bin/python3"
__NELSON_PYTHON_LIBRARY__: example "libpython3.10.so.1.0"
__NELSON_PYTHON_HOME__: example "/usr"
All environment variables must exist and valid to be considered.
On Windows, the pyenv('Version', '3.11') function searches the Windows Registry for the Python version associated with the specified version. It first looks in the HKCU environment, and if not found, it searches in HKLM.
Examples
pe = pyenv
if ispc()
pe = pyenv('Version', '3.12')
See also
History
Version | Description |
---|---|
1.3.0 | initial version |
1.4.0 | environment variables to force python environment |
1.4.0 | On Windows find python by Windows registry. |
Author
Allan CORNET
pyrun
Run Python statements from Nelson.
Syntax
- pyrun(code)
- outvars = pyrun(code, outputs)
- outvars = pyrun(code, outputs, pyName, pyValue)
Input argument
- code - a string scalar, string array, character vector, character array or python code object.
- pyName, pyValue - Input arguments name and value
- outputs - string array: Python variable names.
Output argument
- outvars - One or more Nelson workspace variable names returned as valid Python types.
Description
pyrun(code) function executes Python statements present in the code string within the Python interpreter.
Variables generated by the pyrun function remain persistent, allowing their usage in subsequent pyrun calls.
outvars = pyrun(code, outputs) Python variables specified in outputs are returned to Nelson.
The values of these variables are captured in outvars.
outvars = pyrun(code, outputs, pyName, pyValue), the code is executed with assigned input and output variable names using Nelson data passed through one or more name-value arguments.
Examples
pyrun('a = b * c', 'b', 5, 'c', 10)
r = pyrun('d = a + c', 'd')
pyrun(["a = 3","print(a)"])
[R1, R2] = pyrun("a=b*c",["a","b"], 'b', 5, 'c', 10)
Python code object representing a script generated through the built-in compile function in Python
PYCODE = pyrun('X = compile(''Y = 3'', ''test'', ''exec'')', 'X')
y = pyrun(PYCODE, 'Y')
See also
pyrunfile, pyenv, Python types supported.
History
Version | Description |
---|---|
1.3.0 | initial version |
1.4.0 | Python code object allowed as first input argument |
Author
Allan CORNET
pyrunfile
Run Python file from Nelson.
Syntax
- pyrunfile(filename)
- pyrunfile(filename input)
- outvars = pyrunfile(filename, outputs)
- outvars = pyrunfile(filename, outputs, pyName, pyValue, ...)
Input argument
- filename - a string scalar, character vector: filename .py to run.
- "filename 'input'" - a string scalar, character vector: filename .py to run with input arguments.
- pyName, pyValue - Input arguments name and value
- outputs - string array: Python variable names.
Output argument
- outvars - One or more Nelson workspace variable names returned as valid Python types.
Description
pyrunfile(filenam) function executes Python file.
In contrast to the pyrun function, variables generated in the Python workspace through the pyrunfile function do not persist. This means that subsequent calls to pyrunfile won't be able to access these variables.
The code outvars = pyrunfile(file, outputs, pyName1, pyValue2, ..., pyNameN, pyValueN) executes the code with one or more name-value pair arguments.
Known limitation:
The pyrun and pyrunfile functions lack support for classes containing local variables initialized by other local variables via methods. In such cases, it's advisable to create a module and access it instead.
Examples
pyrunfile_example_1.py
content = "hello Nelson"
print(content)
pyrunfile from Nelson
pyrunfile('pyrunfile_example_1.py')
pyrunfile_example_2.py
import sys
print('greetings from:')
for arg in sys.argv[0:]:
print(arg)
pyrunfile from Nelson with arguments
pyrunfile('pyrunfile_example_2.py "Hello" "world"')
pyrunfile_example_3.py
def minus(a,c):
b = a-c
return b
z = minus(x, y)
pyrunfile from Nelson with values from Nelson
pyrunfile('pyrunfile_example_3.py', 'x', 5, 'y', 3)
See also
pyrun, pyenv, Python types supported.
History
Version | Description |
---|---|
1.4.0 | initial version |
Author
Allan CORNET
Spreadsheet
Spreadsheet
- csvread - Read comma-separated value (CSV) file.
- csvwrite - Write comma-separated value file.
- detectImportOptions - Create import options based on file content.
- dlmread - Read an numeric matrix from a text file file using a delimiter.
- dlmwrite - Write an numeric matrix to a text file file using a delimiter.
- readcell - Create cell array from file.
- readmatrix - Create matrix array from file.
- readtable - Create table from file.
- writecell - Write a cell to a file.
- writematrix - Write a matrix to a file.
- writetable - Write table to file.
csvread
Read comma-separated value (CSV) file.
Syntax
- M = csvread(filename)
- M = csvread(filename, R1, C1)
- M = csvread(filename, R1, C1, [R1 C1 R2 C2])
Input argument
- filename - a string: filename source.
- R1, C1 - nonnegative integer: offset. default : 0, 0
- [R1 C1 R2 C2] - nonnegative integer: Starting row offset, starting column offset, ending row offset and ending column offset.
Output argument
- M - a double matrix.
Description
M = csvread(filename, R1, C1, [R1 C1 R2 C2]) reads only the data within the range specified by row offsets R1 to R2 and column offsets C1 to C2.
M = csvread(filename, R1, C1) starts reading data at the row and column offsets specified by R1 and C1. For example, R1=0, C1=0 indicates the first value in the file.
To set row and column offsets without defining a delimiter, use an empty character as a placeholder, like M = csvread(filename, 3, 1).
M = csvread(filename) read a comma-separated value (CSV) formatted file into matrix M.
Complex Number Importing: csvread reads each complex number as a single unit, storing it in a complex numeric field.
Valid forms for complex numbers are:
Form: | Example: |
---|---|
±<real>±<imag>i|j | 3.1347-2.1i |
±<imag>i|j | -2.1j |
Note: Whitespace within a complex number is not allowed; csvread interprets any embedded spaces as field delimiters.
Example
A = [Inf, -Inf, NaN, 3];
filename = [tempdir(), 'csvread_example.csv'];
csvwrite(filename, A);
R = csvread(filename)
See also
History
Version | Description |
---|---|
1.10.0 | initial version |
Author
Allan CORNET
csvwrite
Write comma-separated value file.
Syntax
- csvwrite(filename, M)
- csvwrite(filename, M, r, c)
Input argument
- filename - a string: filename destination.
- M - an numeric or logical matrix.
- r, c - integer: offset. default : 0, 0
Description
csvwrite writes an numeric matrix to an CSV format file.
Example
A = [Inf, -Inf, NaN, 3];
filename = [tempdir(), 'dlmwrite_example.csv'];
csvwrite(filename, A);
R = csvread(filename)
A = eye(3, 2);
csvwrite(filename, A);
R = fileread(filename)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
detectImportOptions
Create import options based on file content.
Syntax
- options = detectImportOptions(filename)
Input argument
- filename - a string: filename source.
Output argument
- options - DelimitedTextImportOptions object.
Description
options = detectImportOptions(filename) identifies a table in a file and returns an import options object.
You can customize this object and use it with readtable, readcell or readmatrix to control how Nelson imports data as a table, cell array, or matrix.
The type of the returned options object depends on the file's extension.
Properties:
Delimiter: Field delimiter characters. example: {','}
LineEnding: End-of-line characters. example: {'\r\n'}
CommentStyle: Style of comments. example: {'#'}
EmptyLineRule: Procedure to handle empty lines. example: 'skip'
VariableNamesLine: Variable names location. example: 1
VariableNames: Variable names. example: {'Names' 'Age' 'Height' 'Weight'}
RowNamesColumn: Row names location. example: 0
DataLines: Data location, [l1 l2] Indicate the range of lines containing the data. l1 refers to the first line with data, while l2 refers to the last line. example: [2 Inf]
Example
Names = {'John'; 'Alice'; 'Bob'; 'Diana'};
Age = [28; 34; 22; 30];
Height = [175; 160; 180; 165];
Weight = [70; 55; 80; 60];
T = table(Names, Age, Height, Weight);
writetable(T, [tempdir,'readcell_1.csv'])
options = detectImportOptions([tempdir,'readcell_1.csv'])
C1 = readcell([tempdir,'readcell_1.csv'], options)
options.DataLines = [1 Inf]
C2 = readcell([tempdir,'readcell_1.csv'], options)
See also
readcell, readtable, readmatrix.
History
Version | Description |
---|---|
1.10.0 | initial version |
Author
Allan CORNET
dlmread
Read an numeric matrix from a text file file using a delimiter.
Syntax
- M = dlmread(filename)
- M = dlmread(filename, delimiter)
- M = dlmread(filename, delimiter, R1, C1)
- M = dlmread(filename, delimiter, [R1 C1 R2 C2])
Input argument
- filename - a string: filename source.
- delimiter - a string: ',' , '\t', ';' delimiter. default ','
- R1, C1 - nonnegative integer: offset. default : 0, 0
- [R1 C1 R2 C2] - nonnegative integer: Starting row offset, starting column offset, ending row offset and ending column offset.
Output argument
- M - a double matrix.
Description
M = dlmread(filename, delimiter, [R1 C1 R2 C2]) reads only the data within the range specified by row offsets R1 to R2 and column offsets C1 to C2. Alternatively, you can specify the range using spreadsheet notation, such as 'A1..B6' instead of [0 0 5 1].
M = dlmread(filename, delimiter, R1, C1) starts reading data at the row and column offsets specified by R1 and C1. For example, R1=0, C1=0 indicates the first value in the file.
To set row and column offsets without defining a delimiter, use an empty character as a placeholder, like M = dlmread(filename, '', 3, 1).
M = dlmread(filename, delimiter) reads data from the file using the specified delimiter and treats repeated delimiter characters as separate delimiters.
M = dlmread(filename) reads a numeric data file in ASCII-delimited format into matrix M. The dlmread function automatically detects the delimiter from the file and consolidates consecutive white spaces into a single delimiter.
Complex Number Importing: dlmread reads each complex number as a single unit, storing it in a complex numeric field.
Valid forms for complex numbers are:
Form: | Example: |
---|---|
±<real>±<imag>i|j | 3.1347-2.1i |
±<imag>i|j | -2.1j |
Note: Whitespace within a complex number is not allowed; dlmread interprets any embedded spaces as field delimiters.
Examples
A = [Inf, -Inf, NaN, 3];
filename = [tempdir(), 'dlmread_example.csv'];
dlmwrite(filename, A);
R = dlmread(filename)
Read a CSV file with a header
filename = [tempdir(), 'dlmread_example.csv'];
filewrite(filename, ['A,B,C,D,E,F',char(10)]);
A = magic(6);
dlmwrite(filename, A, '-append');
fileread(filename)
R = dlmread(filename, '', 1, 0)
See also
History
Version | Description |
---|---|
1.10.0 | initial version |
Author
Allan CORNET
dlmwrite
Write an numeric matrix to a text file file using a delimiter.
Syntax
- dlmwrite(filename, M)
- dlmwrite(filename, M, delimiter)
- dlmwrite(filename, M, '-append')
- dlmwrite(filename, M, '-append', delimiter)
- dlmwrite(filename, M, delimiter, r, c)
- dlmwrite(filename, M, '-append', delimiter, r, c)
- dlmwrite(filename, M, delimiter, r, c, eol)
- dlmwrite(filename, M, '-append', delimiter, r, c, eol)
- dlmwrite(filename, M, delimiter, r, c, eol, precision)
- dlmwrite(filename, M, '-append', delimiter, r, c, eol, precision)
Input argument
- filename - a string: filename destination.
- M - an numeric or logical matrix.
- delimiter - a string: ',' , '\t', ';' delimiter. default ','
- r, c - integer: offset. default : 0, 0
- eol - a string: 'pc' or 'unix'.
- precision - a integer or C format string. (default: 5)
Description
dlmwrite writes an numeric matrix to an ASCII format file.
Example
A = [Inf, -Inf, NaN, 3];
filename = [tempdir(), 'dlmwrite_example.csv'];
dlmwrite(filename, A);
R = dlmread(filename)
A = eye(3, 2);
dlmwrite(filename, A, ';', 4, 5);
R = fileread(filename)
See also
History
Version | Description |
---|---|
1.0.0 | initial version |
Author
Allan CORNET
readcell
Create cell array from file.
Syntax
- C = readcell(filename)
- C = readcell(filename, opts)
Input argument
- filename - a string: filename source.
- opts - DelimitedTextImportOptions object
Output argument
- C - a cell.
Description
C = readcell(filename) creates a cell array by importing column-oriented data from a text or spreadsheet file.
C = readcell(filename, opts) creates a cell array using the settings defined in the opts import options object. The import options object allows you to customize how readcell interprets the file, offering greater control, improved performance, and the ability to reuse the configuration compared to the default syntax.
Examples
Names = {'John'; 'Alice'; 'Bob'; 'Diana'};
Age = [28; 34; 22; 30];
Height = [175; 160; 180; 165];
Weight = [70; 55; 80; 60];
T = table(Names, Age, Height, Weight);
writetable(T, [tempdir,'readcell_1.csv'])
C = readcell([tempdir,'readcell_1.csv'])
Names = {'John'; 'Alice'; 'Bob'; 'Diana'};
Age = [28; 34; 22; 30];
Height = [175; 160; 180; 165];
Weight = [70; 55; 80; 60];
T = table(Names, Age, Height, Weight);
writetable(T, [tempdir,'readcell_1.csv'])
options = detectImportOptions([tempdir,'readcell_1.csv']);
C1 = readcell([tempdir,'readcell_1.csv'], options)
options.DataLines = [1 Inf]
C2 = readcell([tempdir,'readcell_1.csv'], options)
See also
writecell, detectImportOptions, writetable, readtable, fileread.
History
Version | Description |
---|---|
1.10.0 | initial version |
Author
Allan CORNET
readmatrix
Create matrix array from file.
Syntax
- M = readmatrix(filename)
- M = readmatrix(filename, opts)
- M = readmatrix(filename, opts, 'OutputType', type)
Input argument
- filename - a string: an existing filename source.
- opts - DelimitedTextImportOptions object
- type - a string: 'double', 'single', 'char', 'string', 'int8', 'int16', 'int32', 'int64', 'uint8', 'uint16', 'uint32', 'uint64'.
Output argument
- M - a matrix.
Description
M = readmatrix(filename) creates a matrix array by importing column-oriented data from a text or spreadsheet file.
M = readmatrix(filename, opts) creates a matrix array using the settings defined in the opts import options object. The import options object allows you to customize how readmatrix interprets the file, offering greater control, improved performance, and the ability to reuse the configuration compared to the default syntax.
Examples
filename = [tempdir,'readmatrix_1.csv'];
Names = {'John'; 'Alice'; 'Bob'; 'Diana'};
Age = [28; 34; 22; 30];
Height = [175; 160; 180; 165];
Weight = [70; 55; 80; 60];
T = table(Names, Age, Height, Weight);
writetable(T, filename)
M = readmatrix(filename)
filename = [tempdir,'readmatrix_2.csv'];
M = magic(6);
writematrix(M, filename)
options = detectImportOptions(filename)
options.DataLines = [2 4];
M2 = readmatrix(filename, options, 'OutputType', 'int64')
M3 = readmatrix(filename, options, 'OutputType', 'char')
See also
writematrix, detectImportOptions, writetable, readtable, fileread.
History
Version | Description |
---|---|
1.10.0 | initial version |
Author
Allan CORNET
readtable
Create table from file.
Syntax
- T = readtable(filename)
- T = readtable(filename, opts)
Input argument
- filename - a string: filename source.
- opts - DelimitedTextImportOptions object
Output argument
- T - a table.
Description
T = readtable(filename) creates a table by importing column-oriented data from a text or spreadsheet file.
T = readtable(filename, opts) creates a table using the settings defined in the opts import options object. The import options object allows you to customize how readtable interprets the file, offering greater control, improved performance, and the ability to reuse the configuration compared to the default syntax.
Examples
Names = {'John'; 'Alice'; 'Bob'; 'Diana'};
Age = [28; 34; 22; 30];
Height = [175; 160; 180; 165];
Weight = [70; 55; 80; 60];
T1 = table(Names, Age, Height, Weight);
writetable(T1, [tempdir,'readtable_1.csv'])
T2 = readtable([tempdir,'readtable_1.csv'])
Names = {'John'; 'Alice'; 'Bob'; 'Diana'};
Age = [28; 34; 22; 30];
Height = [175; 160; 180; 165];
Weight = [70; 55; 80; 60];
T = table(Names, Age, Height, Weight);
writetable(T, [tempdir,'readtable_1.csv'])
options = detectImportOptions([tempdir,'readtable_1.csv']);
T1 = readtable([tempdir,'readtable_1.csv'], options)
options.DataLines = [1 Inf]
T2 = readtable([tempdir,'readtable_1.csv'], options)
See also
writetable, detectImportOptions, readcell, fileread.
History
Version | Description |
---|---|
1.10.0 | initial version |
Author
Allan CORNET
writecell
Write a cell to a file.
Syntax
- writecell(C)
- writecell(C, filename)
- writecell(..., Name, Value)
Input argument
- C - an cell array.
- filename - a string: filename destination.
- Name, Value - Name-Value Arguments
Description
writecell writes an cell array to an CSV format file.
writecell does not support sparse matrices.
writecell outputs numeric data in the long G format.
Available Name-Value Arguments
Name-value pairs must follow all other arguments.
The order of name-value pairs doesn't matter
Delimiter and QuoteStrings options only apply to delimited text files.
FileType: Specifies the type of output file
Syntax: 'FileType','text'
Supports delimited text files (.txt, .dat, .csv)
WriteMode: Controls how data is written to the file
Syntax: 'WriteMode', mode
Options:
'overwrite' (default) - Creates new file or replaces existing content
'append' - Adds data to end of existing file
If the target file doesn't exist, a new file will be created regardless of mode.
Delimiter: Defines the character used to separate fields
Syntax: 'Delimiter', delimiter
Available Delimiters: Only applicable for delimited text files.
Specifier | Alternative | Description |
---|---|---|
','
|
'comma'
|
Comma (default) |
' '
|
'space'
|
Space character |
'\t'
|
'tab'
|
Tab character |
';'
|
'semi'
|
Semicolon |
'|'
|
'bar'
|
Vertical bar |
QuoteStrings: Controls text quoting behavior (Only applicable for delimited text files).
'QuoteStrings', option
with options
'minimal' (default) Quotes only text containing delimiters, line endings, or quotes.
'all' Quotes all text variables.
'none' Uses no quotes.
Example
C = {'ID', 'Product', 'Price'; 1, 'Laptop', 799.99; 2, 'Phone', 699.49; 3, 'Tablet', 499.00};
filename = [tempdir(), 'writecell_example.csv'];
writecell(C, filename);
R = fileread(filename)
See also
readcell, csvwrite, dlmread, fileread.
History
Version | Description |
---|---|
1.10.0 | initial version |
Author
Allan CORNET
writematrix
Write a matrix to a file.
Syntax
- writematrix(M)
- writematrix(M, filename)
- writematrix(..., Name, Value)
Input argument
- M - an numeric or logical matrix.
- filename - a string: filename destination.
- Name, Value - Name-Value Arguments
Description
writematrix writes an numeric matrix to an CSV format file.
writematrix does not support sparse matrices.
writematrix outputs numeric data in the long G format.
Available Name-Value Arguments
Name-value pairs must follow all other arguments.
The order of name-value pairs doesn't matter
Delimiter and QuoteStrings options only apply to delimited text files.
FileType: Specifies the type of output file
Syntax: 'FileType','text'
Supports delimited text files (.txt, .dat, .csv)
WriteMode: Controls how data is written to the file
Syntax: 'WriteMode', mode
Options:
'overwrite' (default) - Creates new file or replaces existing content
'append' - Adds data to end of existing file
If the target file doesn't exist, a new file will be created regardless of mode.
Delimiter: Defines the character used to separate fields
Syntax: 'Delimiter', delimiter
Available Delimiters: Only applicable for delimited text files.
Specifier | Alternative | Description |
---|---|---|
','
|
'comma'
|
Comma (default) |
' '
|
'space'
|
Space character |
'\t'
|
'tab'
|
Tab character |
';'
|
'semi'
|
Semicolon |
'|'
|
'bar'
|
Vertical bar |
QuoteStrings: Controls text quoting behavior (Only applicable for delimited text files).
'QuoteStrings', option
with options
'minimal' (default) Quotes only text containing delimiters, line endings, or quotes.
'all' Quotes all text variables.
'none' Uses no quotes.
Example
A = [Inf, -Inf, NaN, 3];
filename = [tempdir(), 'writematrix_example.csv'];
writematrix(A, filename);
R = fileread(filename)
See also
readcell, csvwrite, dlmread, fileread.
History
Version | Description |
---|---|
1.10.0 | initial version |
Author
Allan CORNET
writetable
Write table to file.
Syntax
- writetable(T)
- writetable(T, filename)
- writetable(..., Name, Value)
Input argument
- T - A table to be written to a file.
- filename - A string specifying the destination filename.
Description
writetable(T) writes the table T to a comma-delimited text file.
The file name is derived from the table's workspace variable name, with the .txt
extension appended.
If the file name cannot be derived from the table name, the default file name table.txt
is used.
Output formats supported:
- Text files: Each variable in T becomes a column, and variable names serve as column headers in the first line.
- XML files: Each variable in T becomes an XML node, with variable names as element node names.
To specify the file name explicitly, use writetable(T, filename). The file format is determined by the file extension:
- .txt, .dat, .csv: Delimited text files.
- .xml: XML files.
Additional options: Use writetable(..., Name, Value) for customization:
- WriteRowNames: Include row names in the output file (default:
false
). - FileType: Specify file format (
'text'
or'xml'
). - WriteVariableNames: Include variable names as column headings in text files (default:
true
). - WriteMode: Specify writing mode (
'overwrite'
or'append'
). - Delimiter: Define the field delimiter for text files (
','
,'\t'
, etc.). - QuoteStrings: Control how text is quoted in text files (
'minimal'
,'all'
, or'none'
). - AttributeSuffix: Specify attribute suffix for XML files (default:
'Attribute'
). - RowNodeName: Specify XML row node names (default:
'row'
). - TableNodeName: Specify XML root node name (default:
'table'
).
Example
Examples demonstrating various usages of
T = table([1; 2; 3], {'A'; 'B'; 'C'}, [10.5; 20.7; 30.2], 'VariableNames', {'ID', 'Name', 'Value'});
T.Value_Attribute = {'High'; 'Medium'; 'Low'};
% Basic usage - write to text file
writetable(T)
% Write to specific CSV file with custom delimiter
writetable(T, 'data.csv', 'Delimiter', ';')
% Write to XML with custom node names
writetable(T, 'data.xml', 'RowNodeName', 'record', 'TableNodeName', 'dataset')
% Append to existing file with row names
writetable(T, 'data.txt', 'WriteMode', 'append', 'WriteRowNames', true)
See also
History
Version | Description |
---|---|
1.10.0 | Initial version. |
Author
Allan CORNET
Changelog
All notable changes to this project will be documented in this file.
The format is based on Keep a Changelog, and this project adheres to Semantic Versioning.
1.11.0 (2025-01-11)
Added
- #1321
mustBeSparse
validator function. - #1322
cmdsep
: Command separator for current operating system. urlencode
: Replace special characters in URLs with escape characters.docroot
: Utility to retrieve or define the root directory of Nelson Help.ismodule
: second input argumentisprotected
added.editor('editor_command', cmd)
allows to change text editor in Nelson (for example: VS Code).NELSON_RUNTIME_PATH
environment variable added by installer on Windows.--vscode
command line argument added.- NixOS 24.11 packaging (see BUILDING_Linux.md).
Changed
- Help Center: Access documentation in your system's web browser. Previously, the documentation was opened in the embedded Help browser.
- CA certificate store update.
- fmt library dependency updated.
- BS::threadpool library dependency updated.
- Advanced terminal updated (common for all platforms without GUI, auto completion, search history).
- Python 3.13.1 supported.
Fixed
- #1324 Cell display could not be interrupted.
1.10.0 (2024-12-14)
Added
detectImportOptions
: Generate import options from the file's content.readtable
: Read table from file.writetable
: Write table to file.readcell
: Read cell array from file.writecell
: write cell array to file.readmatrix
: read matrix from file.writematrix
: write matrix to file.csvread
: Read comma-separated value (CSV) file.csvwrite
: Write comma-separated value (CSV) file.dlmread
: Read ASCII-delimited file of numeric data into matrix.realmin
: Smallest normalized floating-point number.- #1288
mustBeMatrix
,mustBeRow
,mustBeColumn
validator functions. join
: Combine strings.- #1292 Large Table Display.
- #1290
VariableTypes
property for table: Specify the data types of table in Nelson. hour
,minute
,second
component of input date and time.
Changed
narginchk
,nargoutchk
support for check only minimun argumentsnarginchk(3, Inf)
.- Fedora 41 CI
title
:Visible
property is inherited from the parent if not explicitly defined.- i18n: migration PO files to JSON.
dlmwrite
: rework the function to be more fast and robust.strjust
: rework the function to be more fast and robust.datenum
: support '' as format for compatibility.
Fixed
- #1303
datevec
result must be normalized. - #1297 some features have no help files.
- #1276 micromamba macos build.
1.9.0 (2024-10-26)
Added
-
Table direct computation:
- unary functions:
abs
,acos
,acosh
,acot
,acotd
,acoth
,acsc
,acscd
,acsch
,asec
,asecd
,asech
,asin
,asind
,asinh
,atan
,atand
,atanh
,ceil
,cosd
,cosh
,cospi
,cot
,cotd
,coth
,csc
,cscd
,csch
,exp
,fix
,floor
,log
,log10
,log1p
,log2
,nextpow2
,round
,sec
,secd
,sech
,sin
,sind
,sinh
,sinpi
,sqrt
,tan
,tand
,tanh
,var
,acosd
,not
. - binary functions:
plus
,minus
,times
,eq
,ge
,gt
,le
,ne
,lt
,rdivide
,rem
,power
,pow2
,or
,mod
,ldivide
.
- unary functions:
-
end
magic keyword can be overloaded for classes (applied totable
class). -
#1250
head
,tail
functions for table and array. -
#1248
removevars
,renamevars
functions for table.
Changed
- #1259 Add macOS Sequoia and remove macOS Monterey CI support.
- Qt 6.8 LTS support (used on Windows 64 bits binary).
- Python 3.13.0 on Windows.
- Boost 1.86 on Windows.
1.8.0 (2024-10-04)
Added
-
table
Data Type:-
Introduced the
table
data type, offering enhanced functionality for structured data manipulation. -
Overloaded methods specific to the
table
data type:disp
,display
for table display.horzcat
,vertcat
for horizontal and vertical concatenation.isempty
to check if the table is empty.isequal
,isequalto
for table comparison.properties
for accessing table metadata.subsasgn
for subscripted assignment.subsref
for subscripted referencing.
-
Conversion functions added:
array2table
: Convert an array to a table.cell2table
: Convert a cell array to a table.struct2table
: Convert a structure to a table.table2array
: Convert a table to an array.table2cell
: Convert a table to a cell array.table2struct
: Convert a table to a structure.
-
Utility functions introduced:
width
: Retrieve the number of columns in the tableheight
: Retrieve the number of rows in the tableistable
: Check if a variable is of thetable
data type
-
-
Resize
- Resize figure property. -
#36
datenum
format compatibility extended. -
#37
datestr
Convert date and time to string format.
Changed
- CodeQL Github action updated.
Fixed
- fix 'units' refresh for 'axes' object.
1.7.0 (2024-08-28)
Added
uicontrol
Create user interface control (button, slider, edit, list box, etc.).waitfor
Block execution and wait for condition.waitforbuttonpress
— Wait for click or key press.im2double
— Convert image to double.CloseRequestFcn
— Close request callback forfigure
.CreateFcn
— Create callback for all graphic objects.DeleteFcn
— Delete callback for all graphic objects.BusyAction
— Busy action for all graphic objects.Interruptible
— Interruptible property for all graphic objects.BeingDeleted
— Being deleted property for all graphic objects.KeyPressFcn
,KeyReleaseFcn
,ButtonDownFcn
properties forfigure
.
Changed
-
Refactor the internal implementation of the 'system' built-in function.
-
Python 3.12.5 on Windows.
1.6.0 (2024-06-29)
Added
unique
: Unique values.ndgrid
: Rectangular grid in N-D space.nthroot
: Real nth root of real numbers.allfinite
: Check if all array elements are finite.j
as imaginary unit number is also supported. example3+2j
equivalent to3+2i
.FollowLocation
option forweboptions
- oneAPI Threading Building Blocks optional dependency.
- Ubuntu 24.04 debian package.
- Ubuntu 24.04 CI
Changed
-
sort
: speed optimization. -
Windows dependencies updated and rebuild with minimal dependencies:
- Qt 6.7.1,
- Visual C++ 2022 Redistributable v14.40.33810.00,
- boost 1.85,
- Python 3.12.4,
- Intel Math Kernel Library 2024.1.1,
- Intel runtime,
- SLICOT,
- gettext 0.22.5,
- cmake 3.30.0 rc3,
- libsndfile 1.2.2,
- portaudio 19.7.5,
- taglib 2.0,
- libzip1 1.3.1,
- libcurl 8.8.0,
- icu4c 74.2,
- libffi 3.4.6,
- libxml2 2.11.7
-
Unicode® Standard, Version 15.1 support
-
simdutf 5.2.8
-
fast_float 6.1.1
-
dtl 1.2.0
Fixed
- #1210
bode
did not unwrap phase. - #1206
balance
yields wrong Transformation Matrix. - #1205
diag
may return wrong sub-diagonals. - #1202 buildhelpmd does not generate SUMMARY as expected.
- #1201 Matrix Exponential
expm
might give wrong results. - #1200 Matrix Parsing/Evaluation trouble.
1.5.0 (2024-05-31)
Added
-
dictionary
data type.dictionary
: Object that maps unique keys to values.configureDictionary
: Create dictionary with specified key and value types.insert
: Add entries to a dictionary.lookup
: Find value in dictionary by key.remove
: Remove dictionary entries.entries
: Key-value pairs of dictionary.keys
: Keys of dictionary.values
: Values of dictionary.types
: Types of dictionary keys and values.numEntries
: Number of key-value pairs in dictionary.isConfigured
: Determine if dictionary has types assigned to keys and values.isKey
: Determine if dictionary contains key.keyHash
: Generate hash code for dictionary key.keyMatch
: Determine if two dictionary keys are the same.
-
bernsteinMatrix
: Bernstein matrix. -
orderedfields
: Order fields of structure array. -
Python interface (part 3):
- #1160 Python operators in Nelson.
keyHash
,keyMatch
for python objects.isa
builtin support python types.- python dictionary to Nelson dictionary
dictionary(pyDict)
- conversion dictionary to python dictionary.
Changed
- help files generated sorted by name on all platforms.
- on windows, Qt libraries used are in debug mode.
Fixed
- #1195
strcmp({'a'},["a"])
did not return expected value.
1.4.0 (2024-04-27)
Added
-
Python interface (part 2):
- #1168 Run Python script file from Nelson.
- #1141 Help about Managing Data between Python and Nelson.
- #1149 python bytes, and bytearray types were not managed.
- #1163 pyenv searchs python by version on Windows.
- #1164 Embed python distribution on Windows.
- #1167 Help about how to install Python package from Nelson.
- numpy types support if numpy available.
pyenv
: can use environment variables to set values.
-
getenv
: Retrieve the values of several environment variables. -
pyrun
: Python code object allowed as first input argument. -
nelson --without_python
starts nelson without python engine. -
skip_testsuite
: allows to skip test suite dynamically on condition.
Changed
- Allow to call method of a variable of CLASS/HANDLE type like a function (currently, only plugged for python subtype).
- #1142 Github Actions updated.
- #1157 Qt 6.7 support (used on Windows 64 bits binary).
copyfile
,isfile
,isdir
,mkdir
allow string array type as input.- warning about 'Matrix is singular to working precision' for inv matrix.
- tests webtools skipped if connection fails or not available.
Fixed
- #1144 test_run markdown help file had a typo.
- #1143 Linux Snapcraft version did not allow to use python.
- #1148 pyrun('print(A)','A','A',string(NaN)) did not return expected value.
single(int64([1 2; 3 4]))
returned a wrong value.py.tuple
,py.list
compatibility increased.pyenv
did not manage python's path with space on Windows.- Matio 1.5.27 compatibility on ArchLinux.
- Ubuntu 24.04 LTS support.
- #1178 Fedora 40 support (CI).
- #1134 [CI] MacOS X Ventura restored.
1.3.0 (2024-03-30)
Added
-
Python interface (part 1):
- CMake: Optional Python3 detection.
pyenv
Change default environment of Python interpreter.pyrun
Run Python statements from Nelson.- Major types conversions are compatible (numpy in the next upcoming version).
-
ArchLinux packaging (https://aur.archlinux.org/packages/nelson-git).
-
contour
Contour plot of matrix. -
contour3
3-D contour plot. -
shiftdim
Shift array dimensions. -
xcorr2
2-D cross-correlation. -
deconv
Deconvolution and polynomial division. -
vecnorm
Vector-wise norm. -
normpdf
Normal probability density function. -
#310
gammaln
Logarithm of gamma function. -
#1112
gradient
Numerical gradient. -
#1126
isspace
Determine which characters are space characters.
Changed
- #1110 Eigen master branch (352ede96e4c331daae4e1be9a5f3f50fff951b8d) ready to use.
- #1134 [CI] MacOS X Ventura disabled (Install dependencies fails)
struct
supports scalar string array as field name.
Fixed
- #1110 add help about build and use C/C++ on fly.
- #1124 unexpected result from long statements on Multiple Lines.
- #1127 Nelson could crash if an mxn characters is displayed in the variable browser.
- #1125 Unsupported colon operator with char operands.
- Missing 'zoom in', 'zoom out' icons for help viewer in linux package.
gcd
without argument returned wrong error message.- #1133 [CI] [ARCH LINUX] Warning about MPI.
1.2.0 (2024-02-25)
Added
- Recursive completion on Graphic handle, struct, handle, class (properties, methods).
- Adding links between documents about mex and supported compilers.
- GitHub CI for macOS Sonoma (Apple Silicon) support.
Export to ...
context menu for console and text editor as pdf.CTRL + Mouse wheel
orCTRL + +/-
to zoom in/out on console, editor, help.- Toolbar for figure with print, zoom in, zoom out, rotation, pan, restore axes.
zoom
,pan
,rotate3d
functions.MenuBar
,ToolBar
figure properties.- Window menu on graphic window, list all others available windows.
feature
builtin (undocument features, debug, tests, ...) content can change with next releases.GridAlpha
,GridColor
,View
properties for Axes.- CTRL+C in help viewer, copy selected text.
checkupdate
function and check update menu.isScalarStringArray
iinternal API C++ method.
Changed
- Clicking on an axis automatically sets it as the current axes object.
- Clicking on an figure automatically sets it as the current figure object.
saveas
exports the figure as a PDF page with centered alignment.- Default color of grid for axes.
- Default figure size updated.
- Default
MarkerFaceColor
value for compatibility. - view function returns azimuth and elevation values.
- Camera view reworked.
- Minimal screen resolution supported 800x600.
Fixed
- Change directory with file browser line editor did not work as expected.
- Template to create a function with file browser was wrong.
- Do not allow to select multiple variable in workspace browser.
- File browser checks if files with the extension ".m" have a valid name before enable 'run' context menu.
- Paste in editor with multiple tab.
- Starting the Nelson desktop was taking longer than necessary.
1.1.0 (2024-01-29)
Added
- Nelson Desktop environment: file browser, command history, workspace browser, desktop layout.
- #1074 Roadmap v2.0.0
- #1044: LU matrix factorization.
- #1080
LineStyle
,LineWidth
properties were not implemented for surface objects. sky
,abyss
colormaps.
1.0.0 (2024-01-04)
Nelson 1.0.0 has been released.
Nelson is an interactive, fully functional environment for engineering and scientific applications. It implements a matrix-driven language (which is largely compatible with MATLAB and GNU Octave), with advanced features such as 2-D 3-D plotting, image manipulation and viewing, a codeless interface to external C/C++/FORTRAN libraries, native support for various C types, and a host of other features.
Features
-
Types managed by Nelson:
- double and double complex: scalar, vector, matrix 2D, N dimensions array, sparse matrix.
- single and single complex: scalar, vector, matrix 2D, N dimensions array, sparse matrix.
- logical: scalar, vector, matrix 2D, N dimensions array, sparse matrix.
- character array (UNICODE supported).
- string array (UNICODE supported).
- integers 8, 16, 32, 64 signed and unsigned: scalar, vector, matrix 2D, N dimensions array.
- handle objects.
- anonymous functions,
- all types can be overloaded.
-
OpenMP
andSIMD
extensions used. -
2D and 3D plotting with high-level plot commands.
-
Parallel Computing Module.
-
Fast Fourrier Transformation functions based on FFTW and MKL wrapper.
-
SLICOT (Subroutine Library in Systems and Control Theory) interfaces (optional).
-
Control System module.
-
Message Passing Interface (MPI): functions for parallel computing.
-
JSON decode/encode data support.
-
HDF5 high-level functions I/O,
-
HDF5 used as default data file format (.nh5) load/save workspace,
-
MAT-file compatible load/save workspace,
-
Foreign Function Interface C/Fortran.
-
Interfacing C/C++ or Fortran with Nelson (build and load external code on the fly).
-
MEX C API compatibility.
-
Nelson Engine API for C (compatible with MEX Engine). Call Nelson from your C code as engine.
-
RESTful API web service.
-
Inter-process communication between Nelson's process.
-
The QML engine enables nelson programs to display and manipulate graphical content using Qt's QML framework.
-
Component Object Model (COM) client interface: binary-interface standard for software components on Windows.
-
Write/Read xlsx files on Windows using COM.
-
Embedded Nelson code editor.
-
Help engine:
Generate help files using Nelson dedicated functions. View your generated help files as html, markdown, pdf, gitbook or directly in Nelson help viewer.
-
Tests engine:
Validate your algorithm using Nelson dedicated functions. Export the test results under the xUnit reports format.
-
Profiling and Code coverage tools for Nelson's language:
Nelson has a built-in profiler that is very useful to profile your code and find out what script or function is taking the most time.
-
Nelson cloud: Instant access to Nelson anywhere from an web browser.
-
Module skeleton to extend Nelson available here:
-
Nelson Modules Manager (nmm) : package manager for Nelson
Previous changelog
Changelog
All notable changes to this project will be documented in this file.
The format is based on Keep a Changelog, and this project adheres to Semantic Versioning.
1.11.0 (2025-01-11)
Added
- #1321
mustBeSparse
validator function. - #1322
cmdsep
: Command separator for current operating system. urlencode
: Replace special characters in URLs with escape characters.docroot
: Utility to retrieve or define the root directory of Nelson Help.ismodule
: second input argumentisprotected
added.editor('editor_command', cmd)
allows to change text editor in Nelson (for example: VS Code).NELSON_RUNTIME_PATH
environment variable added by installer on Windows.--vscode
command line argument added.- NixOS 24.11 packaging (see BUILDING_Linux.md).
Changed
- Help Center: Access documentation in your system's web browser. Previously, the documentation was opened in the embedded Help browser.
- CA certificate store update.
- fmt library dependency updated.
- BS::threadpool library dependency updated.
- Advanced terminal updated (common for all platforms without GUI, auto completion, search history).
- Python 3.13.1 supported.
Fixed
- #1324 Cell display could not be interrupted.
1.10.0 (2024-12-14)
Added
detectImportOptions
: Generate import options from the file's content.readtable
: Read table from file.writetable
: Write table to file.readcell
: Read cell array from file.writecell
: write cell array to file.readmatrix
: read matrix from file.writematrix
: write matrix to file.csvread
: Read comma-separated value (CSV) file.csvwrite
: Write comma-separated value (CSV) file.dlmread
: Read ASCII-delimited file of numeric data into matrix.realmin
: Smallest normalized floating-point number.- #1288
mustBeMatrix
,mustBeRow
,mustBeColumn
validator functions. join
: Combine strings.- #1292 Large Table Display.
- #1290
VariableTypes
property for table: Specify the data types of table in Nelson. hour
,minute
,second
component of input date and time.
Changed
narginchk
,nargoutchk
support for check only minimun argumentsnarginchk(3, Inf)
.- Fedora 41 CI
title
:Visible
property is inherited from the parent if not explicitly defined.- i18n: migration PO files to JSON.
dlmwrite
: rework the function to be more fast and robust.strjust
: rework the function to be more fast and robust.datenum
: support '' as format for compatibility.
Fixed
- #1303
datevec
result must be normalized. - #1297 some features have no help files.
- #1276 micromamba macos build.
1.9.0 (2024-10-26)
Added
-
Table direct computation:
- unary functions:
abs
,acos
,acosh
,acot
,acotd
,acoth
,acsc
,acscd
,acsch
,asec
,asecd
,asech
,asin
,asind
,asinh
,atan
,atand
,atanh
,ceil
,cosd
,cosh
,cospi
,cot
,cotd
,coth
,csc
,cscd
,csch
,exp
,fix
,floor
,log
,log10
,log1p
,log2
,nextpow2
,round
,sec
,secd
,sech
,sin
,sind
,sinh
,sinpi
,sqrt
,tan
,tand
,tanh
,var
,acosd
,not
. - binary functions:
plus
,minus
,times
,eq
,ge
,gt
,le
,ne
,lt
,rdivide
,rem
,power
,pow2
,or
,mod
,ldivide
.
- unary functions:
-
end
magic keyword can be overloaded for classes (applied totable
class). -
#1250
head
,tail
functions for table and array. -
#1248
removevars
,renamevars
functions for table.
Changed
- #1259 Add macOS Sequoia and remove macOS Monterey CI support.
- Qt 6.8 LTS support (used on Windows 64 bits binary).
- Python 3.13.0 on Windows.
- Boost 1.86 on Windows.
1.8.0 (2024-10-04)
Added
-
table
Data Type:-
Introduced the
table
data type, offering enhanced functionality for structured data manipulation. -
Overloaded methods specific to the
table
data type:disp
,display
for table display.horzcat
,vertcat
for horizontal and vertical concatenation.isempty
to check if the table is empty.isequal
,isequalto
for table comparison.properties
for accessing table metadata.subsasgn
for subscripted assignment.subsref
for subscripted referencing.
-
Conversion functions added:
array2table
: Convert an array to a table.cell2table
: Convert a cell array to a table.struct2table
: Convert a structure to a table.table2array
: Convert a table to an array.table2cell
: Convert a table to a cell array.table2struct
: Convert a table to a structure.
-
Utility functions introduced:
width
: Retrieve the number of columns in the tableheight
: Retrieve the number of rows in the tableistable
: Check if a variable is of thetable
data type
-
-
Resize
- Resize figure property. -
#36
datenum
format compatibility extended. -
#37
datestr
Convert date and time to string format.
Changed
- CodeQL Github action updated.
Fixed
- fix 'units' refresh for 'axes' object.
1.7.0 (2024-08-28)
Added
uicontrol
Create user interface control (button, slider, edit, list box, etc.).waitfor
Block execution and wait for condition.waitforbuttonpress
— Wait for click or key press.im2double
— Convert image to double.CloseRequestFcn
— Close request callback forfigure
.CreateFcn
— Create callback for all graphic objects.DeleteFcn
— Delete callback for all graphic objects.BusyAction
— Busy action for all graphic objects.Interruptible
— Interruptible property for all graphic objects.BeingDeleted
— Being deleted property for all graphic objects.KeyPressFcn
,KeyReleaseFcn
,ButtonDownFcn
properties forfigure
.
Changed
-
Refactor the internal implementation of the 'system' built-in function.
-
Python 3.12.5 on Windows.
1.6.0 (2024-06-29)
Added
unique
: Unique values.ndgrid
: Rectangular grid in N-D space.nthroot
: Real nth root of real numbers.allfinite
: Check if all array elements are finite.j
as imaginary unit number is also supported. example3+2j
equivalent to3+2i
.FollowLocation
option forweboptions
- oneAPI Threading Building Blocks optional dependency.
- Ubuntu 24.04 debian package.
- Ubuntu 24.04 CI
Changed
-
sort
: speed optimization. -
Windows dependencies updated and rebuild with minimal dependencies:
- Qt 6.7.1,
- Visual C++ 2022 Redistributable v14.40.33810.00,
- boost 1.85,
- Python 3.12.4,
- Intel Math Kernel Library 2024.1.1,
- Intel runtime,
- SLICOT,
- gettext 0.22.5,
- cmake 3.30.0 rc3,
- libsndfile 1.2.2,
- portaudio 19.7.5,
- taglib 2.0,
- libzip1 1.3.1,
- libcurl 8.8.0,
- icu4c 74.2,
- libffi 3.4.6,
- libxml2 2.11.7
-
Unicode® Standard, Version 15.1 support
-
simdutf 5.2.8
-
fast_float 6.1.1
-
dtl 1.2.0
Fixed
- #1210
bode
did not unwrap phase. - #1206
balance
yields wrong Transformation Matrix. - #1205
diag
may return wrong sub-diagonals. - #1202 buildhelpmd does not generate SUMMARY as expected.
- #1201 Matrix Exponential
expm
might give wrong results. - #1200 Matrix Parsing/Evaluation trouble.
1.5.0 (2024-05-31)
Added
-
dictionary
data type.dictionary
: Object that maps unique keys to values.configureDictionary
: Create dictionary with specified key and value types.insert
: Add entries to a dictionary.lookup
: Find value in dictionary by key.remove
: Remove dictionary entries.entries
: Key-value pairs of dictionary.keys
: Keys of dictionary.values
: Values of dictionary.types
: Types of dictionary keys and values.numEntries
: Number of key-value pairs in dictionary.isConfigured
: Determine if dictionary has types assigned to keys and values.isKey
: Determine if dictionary contains key.keyHash
: Generate hash code for dictionary key.keyMatch
: Determine if two dictionary keys are the same.
-
bernsteinMatrix
: Bernstein matrix. -
orderedfields
: Order fields of structure array. -
Python interface (part 3):
- #1160 Python operators in Nelson.
keyHash
,keyMatch
for python objects.isa
builtin support python types.- python dictionary to Nelson dictionary
dictionary(pyDict)
- conversion dictionary to python dictionary.
Changed
- help files generated sorted by name on all platforms.
- on windows, Qt libraries used are in debug mode.
Fixed
- #1195
strcmp({'a'},["a"])
did not return expected value.
1.4.0 (2024-04-27)
Added
-
Python interface (part 2):
- #1168 Run Python script file from Nelson.
- #1141 Help about Managing Data between Python and Nelson.
- #1149 python bytes, and bytearray types were not managed.
- #1163 pyenv searchs python by version on Windows.
- #1164 Embed python distribution on Windows.
- #1167 Help about how to install Python package from Nelson.
- numpy types support if numpy available.
pyenv
: can use environment variables to set values.
-
getenv
: Retrieve the values of several environment variables. -
pyrun
: Python code object allowed as first input argument. -
nelson --without_python
starts nelson without python engine. -
skip_testsuite
: allows to skip test suite dynamically on condition.
Changed
- Allow to call method of a variable of CLASS/HANDLE type like a function (currently, only plugged for python subtype).
- #1142 Github Actions updated.
- #1157 Qt 6.7 support (used on Windows 64 bits binary).
copyfile
,isfile
,isdir
,mkdir
allow string array type as input.- warning about 'Matrix is singular to working precision' for inv matrix.
- tests webtools skipped if connection fails or not available.
Fixed
- #1144 test_run markdown help file had a typo.
- #1143 Linux Snapcraft version did not allow to use python.
- #1148 pyrun('print(A)','A','A',string(NaN)) did not return expected value.
single(int64([1 2; 3 4]))
returned a wrong value.py.tuple
,py.list
compatibility increased.pyenv
did not manage python's path with space on Windows.- Matio 1.5.27 compatibility on ArchLinux.
- Ubuntu 24.04 LTS support.
- #1178 Fedora 40 support (CI).
- #1134 [CI] MacOS X Ventura restored.
1.3.0 (2024-03-30)
Added
-
Python interface (part 1):
- CMake: Optional Python3 detection.
pyenv
Change default environment of Python interpreter.pyrun
Run Python statements from Nelson.- Major types conversions are compatible (numpy in the next upcoming version).
-
ArchLinux packaging (https://aur.archlinux.org/packages/nelson-git).
-
contour
Contour plot of matrix. -
contour3
3-D contour plot. -
shiftdim
Shift array dimensions. -
xcorr2
2-D cross-correlation. -
deconv
Deconvolution and polynomial division. -
vecnorm
Vector-wise norm. -
normpdf
Normal probability density function. -
#310
gammaln
Logarithm of gamma function. -
#1112
gradient
Numerical gradient. -
#1126
isspace
Determine which characters are space characters.
Changed
- #1110 Eigen master branch (352ede96e4c331daae4e1be9a5f3f50fff951b8d) ready to use.
- #1134 [CI] MacOS X Ventura disabled (Install dependencies fails)
struct
supports scalar string array as field name.
Fixed
- #1110 add help about build and use C/C++ on fly.
- #1124 unexpected result from long statements on Multiple Lines.
- #1127 Nelson could crash if an mxn characters is displayed in the variable browser.
- #1125 Unsupported colon operator with char operands.
- Missing 'zoom in', 'zoom out' icons for help viewer in linux package.
gcd
without argument returned wrong error message.- #1133 [CI] [ARCH LINUX] Warning about MPI.
1.2.0 (2024-02-25)
Added
- Recursive completion on Graphic handle, struct, handle, class (properties, methods).
- Adding links between documents about mex and supported compilers.
- GitHub CI for macOS Sonoma (Apple Silicon) support.
Export to ...
context menu for console and text editor as pdf.CTRL + Mouse wheel
orCTRL + +/-
to zoom in/out on console, editor, help.- Toolbar for figure with print, zoom in, zoom out, rotation, pan, restore axes.
zoom
,pan
,rotate3d
functions.MenuBar
,ToolBar
figure properties.- Window menu on graphic window, list all others available windows.
feature
builtin (undocument features, debug, tests, ...) content can change with next releases.GridAlpha
,GridColor
,View
properties for Axes.- CTRL+C in help viewer, copy selected text.
checkupdate
function and check update menu.isScalarStringArray
iinternal API C++ method.
Changed
- Clicking on an axis automatically sets it as the current axes object.
- Clicking on an figure automatically sets it as the current figure object.
saveas
exports the figure as a PDF page with centered alignment.- Default color of grid for axes.
- Default figure size updated.
- Default
MarkerFaceColor
value for compatibility. - view function returns azimuth and elevation values.
- Camera view reworked.
- Minimal screen resolution supported 800x600.
Fixed
- Change directory with file browser line editor did not work as expected.
- Template to create a function with file browser was wrong.
- Do not allow to select multiple variable in workspace browser.
- File browser checks if files with the extension ".m" have a valid name before enable 'run' context menu.
- Paste in editor with multiple tab.
- Starting the Nelson desktop was taking longer than necessary.
1.1.0 (2024-01-29)
Added
- Nelson Desktop environment: file browser, command history, workspace browser, desktop layout.
- #1074 Roadmap v2.0.0
- #1044: LU matrix factorization.
- #1080
LineStyle
,LineWidth
properties were not implemented for surface objects. sky
,abyss
colormaps.
1.0.0 (2024-01-04)
Nelson 1.0.0 has been released.
Nelson is an interactive, fully functional environment for engineering and scientific applications. It implements a matrix-driven language (which is largely compatible with MATLAB and GNU Octave), with advanced features such as 2-D 3-D plotting, image manipulation and viewing, a codeless interface to external C/C++/FORTRAN libraries, native support for various C types, and a host of other features.
Features
-
Types managed by Nelson:
- double and double complex: scalar, vector, matrix 2D, N dimensions array, sparse matrix.
- single and single complex: scalar, vector, matrix 2D, N dimensions array, sparse matrix.
- logical: scalar, vector, matrix 2D, N dimensions array, sparse matrix.
- character array (UNICODE supported).
- string array (UNICODE supported).
- integers 8, 16, 32, 64 signed and unsigned: scalar, vector, matrix 2D, N dimensions array.
- handle objects.
- anonymous functions,
- all types can be overloaded.
-
OpenMP
andSIMD
extensions used. -
2D and 3D plotting with high-level plot commands.
-
Parallel Computing Module.
-
Fast Fourrier Transformation functions based on FFTW and MKL wrapper.
-
SLICOT (Subroutine Library in Systems and Control Theory) interfaces (optional).
-
Control System module.
-
Message Passing Interface (MPI): functions for parallel computing.
-
JSON decode/encode data support.
-
HDF5 high-level functions I/O,
-
HDF5 used as default data file format (.nh5) load/save workspace,
-
MAT-file compatible load/save workspace,
-
Foreign Function Interface C/Fortran.
-
Interfacing C/C++ or Fortran with Nelson (build and load external code on the fly).
-
MEX C API compatibility.
-
Nelson Engine API for C (compatible with MEX Engine). Call Nelson from your C code as engine.
-
RESTful API web service.
-
Inter-process communication between Nelson's process.
-
The QML engine enables nelson programs to display and manipulate graphical content using Qt's QML framework.
-
Component Object Model (COM) client interface: binary-interface standard for software components on Windows.
-
Write/Read xlsx files on Windows using COM.
-
Embedded Nelson code editor.
-
Help engine:
Generate help files using Nelson dedicated functions. View your generated help files as html, markdown, pdf, gitbook or directly in Nelson help viewer.
-
Tests engine:
Validate your algorithm using Nelson dedicated functions. Export the test results under the xUnit reports format.
-
Profiling and Code coverage tools for Nelson's language:
Nelson has a built-in profiler that is very useful to profile your code and find out what script or function is taking the most time.
-
Nelson cloud: Instant access to Nelson anywhere from an web browser.
-
Module skeleton to extend Nelson available here:
-
Nelson Modules Manager (nmm) : package manager for Nelson
Previous changelog
Changelog
All notable changes to this project will be documented in this file.
The format is based on Keep a Changelog.
0.7.12 (2023-12-23)
Changed
- #674 Migrate sources to nelson-lang github organization.
- #775
quit
,exit
,startup.m
,finish.m
behavior reworked for compatibility. - JSON for Modern C++ version 3.11.3 used on all platforms.
- {fmt} 10.1.1 (6f95000) used.
- Fedora 39 CI support.
Fixed
cellfun
did not check type of second input argument.
Added
-
filter
1-D digital filter. -
control system module (part 2):
freqresp
Evaluate system response over a grid of frequencies.step
Simulate continuous time model of a state space model or transfer function.lsim
Plot simulated time response of dynamic system to arbitrary inputs.dc2
Convert model from discrete to continuous time.c2d
Convert model from continuous to discrete time.augstate
Append state vector to output vector.kalman
Design Kalman filter for state estimation.evalfr
Evaluate system response at specific frequency.nyquist
Nyquist plot of frequency response.ord2
Generate continuous second-order systems.append
Group models by appending their inputs and outputs.feedback
Feedback connection of multiple models.parallel
Parallel connection of two models.series
Series connection of two models.ssdelete
Remove inputs, outputs and states from state-space system.ssselect
Extract subsystem from larger system.tzero
Invariant zeros of linear system.tf2ss
Convert transfer function filter parameters to state-space form.ss2tf
Convert state-space representation to transfer function.minreal
Minimal realization or pole-zero cancellation.ssdata
Access state-space model data.tfdata
Access transfer function data.gram
Controllability and observability Gramians.hsvd
Hankel singular values of a state-space or transfer function model.damp
Natural frequency and damping ratio.balreal
Gramian-based balancing of state-space realizations.lqry
Form linear-quadratic (LQ) state-feedback regulator with output weighting.- #957
dlqr
Linear-quadratic (LQ) state-feedback regulator for discrete-time state-space system. - #961
lqed
Discrete Kalman estimator design from continuous cost function. - #960
lqe
Kalman estimator design for continuous-time systems. - #955
lqr
Linear-Quadratic Regulator (LQR) design. - #943
dare
Solve discrete-time algebraic Riccati equations. - #951
care
Continuous-time algebraic Riccati equation solution. - #945
ctrbf
Compute controllability staircase form. - #946
ctrb
Controllability of state-space model. - #963
obsv
Observability matrix. - #964
obsvf
Compute observability staircase form. - #949
acker
Pole placement gain selection using Ackermann's formula. - #950
bdschur
Block-diagonal Schur factorization. - #952
cloop
Close unity feedback loops. - #953
compreal
Companion realization of transfer functions. - #959
gensig
Create periodic signals for simulating system response.
0.7.11 (2023-11-29)
Added
hist
Histogram plot.bar
Bar graph.scatter
Scatter plot.stem
Plot discrete sequence data.stairs
Stairstep graph.fill
2-D patch.pie
legacy pie chart.subsref
Subscripted reference.subsasgn
Redefine subscripted assignment.substruct
Create structure argument for subsasgn or subsref.deal
Distribute inputs to outputs.- Intel compiler support.
Changed
- axis limits recalculate with
hggroup
. axes
forces focus on current axe.- function_handle parenthese precedence.
patch
andfill
managesFaceAlpha
.- visibility title and labels.
- object constructor must be in '@' directory and no more in parent directory (compatibility).
subsref
,subsasgn
compatibility withsubstruct
.- To display a percent sign, you need to use a double percent sign (%%) in the format string (compatibility).
- French translation updated (100%, Thanks to weblate contributors)
- #997 Macos BigSur Github CI support removed.
- Qt 6.6.1 on win64 CI build.
Fixed
A = []; A(false) = zeros(3, 0)
did not return an empty matrix but an error.
0.7.10 (2023-10-27)
Added
-
private functions/folders support (to limit the scope of a function).
-
syntax extended to facilitate the creation of literal integers without loss of precision:
- example:
18446744073709551615u64
,18446744073709551615i64
(similar to rust syntax)
- example:
-
flintmax('like', p)
syntax added. -
int64
,uint64
warning about double-precision. -
#570 balance: Diagonal scaling to improve eigenvalue accuracy.
-
isobject
Check whether the input is an object. -
cell2mat
Convert cell array of matrices to single matrix. -
#948
blkdiag
Create a block diagonal matrix from 2D matrices of different sizes. -
kron
Kronecker tensor product. -
strjust
Justify strings. -
control system module (part 1):
- #967 control system module template.
- #944
mag2db
,db2mag
,pow2db
,db2pow
functions. - #968
zp2tf
: Zero-pole to transfer function conversion. - #954
dcgain
: Low-frequency (DC) gain of LTI system. - #965
padecoef
: Padé approximation of time delays. - #958
esort
: Sort continuous-time poles by real part. dsort
: Sort discrete-time poles by magnitude.- #962
lyap
: Continuous Lyapunov equation solution. dlyap
: Discret Lyapunov equation solution.abcdchk
Verifies the dimensional compatibility of matrices A, B, C, and D.ss
: State-space model.tf
: Transfer function model (display, horzcat, vertcat, size).isct
: checks if dynamic system model is in continuous time.isdt
: checks if dynamic system model is in discret time.isstatic
: checks if model is static or dynamic.islti
: checks if variable is an linear model tf, ss or zpk.issiso
: checks if dynamic system model is a single input and single output.zero
: Zeros and gain of SISO dynamic system.pole
: Poles of dynamic system.bode
: Bode plot of frequency response, magnitude and phase data.
Changed
- some modules (nig, modules_manager, help_browser) reworked to use private functions.
- Windows 64 bit CI and release use Qt 6.6.0
Fixed
- #940 title bar on dark theme on Windows.
- help viewer using dark theme.
- adjust position
xlabel
onfigure
. - #976 wrong output when reading a file with fscanf with size argument.
- #975 Legend color (and width) is not matching that of curve in figure.
- #988 anonymous function serialization '.^' and '^' are inversed.
0.7.9 (2023-09-18)
Changed
-
#488 overloading functions:
- all types including basic types can be overloaded.
- overload is now fully compatible using '@' syntax and precedence.
- all operators were reworked to support compatible overload.
-
.*
operator optimized. -
conv2
optimized. -
Boost 1.82 used on Windows.
-
Internals:
class
,function_handle
types reworked.- types order updated.
- rework validator module.
- functions finder reworked.
- file watcher reworked.
- operators reworked.
repmat
,ones
,NaN
,Inf
reworked.
-
function_handle
display is more compatible.
Added
- #491 Anonymous functions
--withoutfilewatcher
executable argument. disable file watcher for current session.<--FILE WATCHER REQUIRED -->
test_run option.- #853 MacOs 13 ventura CI
Fixed
- #916 openblas micromamba on macos required to link libgfortran
0.7.5 (2023-05-27)
Changed
BS::thread_pool
v3.5.0simdutf
to 3.2.9.{fmt}
to 10.0.0.fast_float
to 4.0.0.cast
reworked to be more compatible.colon
reworked to be more compatible (operator uses unary overload).- CMake 3.26.3 on Windows
0.7.4 (2023-04-27)
Added
- Qt 6.5 support.
- #802:
bitand
,bitor
,bitxor
functions. issorted
Determine if array is sorted.num2cell
Convert array to cell array with consistently sized cells.hggroup
Create group object.colorbar('off')
deletes colorbar associated with the current axes.waterfall
Waterfall plot.ribbon
ribbon plot.- figure property:
GraphicsSmoothing
Axes graphics smoothing. - text property:
FontSmoothing
. - surf property:
MeshStyle
. - chatGPT example.
- graphics examples about 3D polygons:
- utah teapot example.
- nefertiti mask example.
- stanford bunny example.
Changed
- Windows installer: allow to install for current user (no administrator rights required).
figure
without axes has a color.figure
can be created not visible.grid minor
toggles the visibility of the minor grid lines.mesh
reworked.- extraction on empty matrix for compatibility.
ones
,eye
,inf
,nan
allow negative index (replaced by 0) for compatibility.- Windows 64 bits version embeds Qt 6.5.
- allows
if
with empty statements
Fixed
weboptions
did not manage HeaderFields as expected.- update
cacert.pem
. - #895: Micromamba linux build fails after packages updates.
0.7.3 (2023-03-28)
Added
patch
Create patches of colored polygons.ancestor
Ancestor of graphics object.- hexadecimal color code managed example: '#DDFF00'.
validatecolor
Validate color values.- #851: Build with micromamba environment (linux and macOS)
Changed
- Figure property
Position
uses position based on bottom left position for compatibility. - internal: boost no more used to read/write json files.
- internal: taglib library is optional.
- version date updated with each build.
Fixed
- #866: Close menu on figure can crash on linux.
- graphic hierarchy was not fully destroyed after
close
ordelete
. - labels were not displayed correctly when the logarithmic scale was enabled.
- #869: missing help files in linux package.
0.7.2 (2023-02-27)
Changed
- cmake project reworked. It should be easier to package Nelson on linux platforms (Thanks to @JohanMabille)
- Debian package generated (beta - feedback welcome).
modulepath
reworked and extended.- C++ API:
IsCellOfStrings(ArrayOf)
replaced byArrayOf::isCellArrayOfCharacterVectors()
- C++ API: header
CheckHelpers.hpp
replaced byInputOutputArgumentsCheckers.hpp
- C++ API:
ToCellStringAsColumn
replaced byArrayOf::toCellArrayOfCharacterColumnVectors
api_nelson
methods moved to type modules- Remove internal circular dependency about error and warning.
- Exports minimum headers in package.
Fixed
disp
,display
did no more support overloading.image
did not save all values forXData
andYData
.- Github CI Monterey and Ubuntu 22.04 (dependencies install) fixed.
- some warnings.
0.7.1 (2023-01-29)
Added
drawnow
: Update figures and process callbacks.DrawLater
property added tofigure
graphics object.interp1
linear interpolation 1D.- #736:
bone
,cool
,copper
,hot
,jet
,pink
,turbo
,viridis
,white
colormaps. Visible
property tofigure
graphics object.- #809:
NumberTitle
property tofigure
graphics object. AlphaMap
andColormap
properties added toAxes
graphics object.LineStyleOrder
property of 'axes' used forplot
andplot3
.ColorOrderIndex
andLineStyleOrderIndex
properties added toaxes
graphics object.Interpreter
property added totext
graphics object.- tex special characters support for
text
andticks
graphics object. delete
for graphics objects.imread
Read image from graphics file.imwrite
Write image to graphics file.imshow
Display image.surface
Primitive surface plot.- #808:
pcolor
Pseudocolor plot. mesh
Mesh surface plot.meshz
Mesh surface plot with curtain.- #807:
loglog
Log-log scale plot. CHANGELOG
0.7.x family.
Changed
- Graphics objects property names check is strict (compatibility).
- Some speed optimization with graphics objects.
surf
reworked to usesurface
.
Fixed
- #823: default LineStyle for a line was wrong with marker.
CTRL+C
was not catched on advanced cli for linux and macos.- colors in
colorbar
were not in the good order. - warnings detected by CodeQL.
- #824: VariableCompleter was not filtered by prefix.
Previous changelog
Changelog
All notable changes to this project will be documented in this file.
The format is based on Keep a Changelog.
0.6.12 (2022-12-27)
Added
Graphics module:
First big step to do plot 2D and 3D with Nelson. Some features are missing and will come in next releases. Current version is usable and show plots possibilities of Nelson.
Do not hesitate to create features requests or bug reports. Contributions are welcome.
Implemented features:
figure
creates an figure window.cla
clears figure.axes
creates cartesian axes.axis
set axis limits and aspect ratios.cla
clears axes.zlim
set or get z-axis limits.ylim
set or get y-axis limits.xlim
set or get x-axis limits.clim
set colormap limits.line
creates primitive line.plot
2-D line plot.plot3
3-D line plot.rgbplot
Plot colormap.subplot
Create axes in tiled positions.newplot
Prepare to produce a new plot.semilogx
Semilog plot (x-axis has log scale).semilogy
Semilog plot (y-axis has log scale).squiver
Quiver or vector plot.surf
creates surface plot.text
creates text descriptions to data points.title
adds title.legend
adds legend to axes.xlabel
Label x-axis.ylabel
Label y-axis.zlabel
Label z-axis.grid
Display or hide axes grid lines.spy
Visualize sparsity pattern of matrix.image
displays image from array.imagesc
displays image from array with scaled colors.cylinder
creates cylinder.sphere
creates sphere.close
close one or more figures.gcf
get current figure graphics object.gca
get current axes graphics object.groot
return graphic root object.refresh
redraws current figure.ishold
get current hold state.hold
retain current plot when adding new plots.view
Camera line of sigh.saveas
Save figure to specific file format.copygraphics
Copy plot to clipboard.isValidGraphicsProperty
check property name is valid for a graphics object type name.isprop
,properties
,isequal
,eq
,display
,set
,get
for Graphics Object type.isgraphics
Check if it is an graphics object handles.colstyle
Parse color and style from string.is2D
Checks if ax is a 2-D Polar or Cartesian axes.colorbar
Colorbar showing color scale.colormap
View and set current colormap.- colormaps:
autumn
: Autumn colormap array.gray
: Gray colormap array.parula
: Parula colormap array.spring
: Spring colormap array.summer
: Summer colormap array.winter
: Winter colormap array.
░░ٌٌٌӇ░ٌٌٌƛ░ٌٌٌƤ░ٌٌٌƤ░ٌٌٌƳ ░ٌٌٌ░ٌٌٌƝ░ٌٌٌЄ░ٌٌٌƜ░ٌٌٌ░ٌٌٌ Ƴ░ٌٌٌЄ░ٌٌٌƛ░ٌٌٌƦ░ٌٌٌ!░
╚═════════ ೋღ❤ღೋ ═════════╝
0.6.11 (2022-11-25)
Added
-
normest
2-norm estimate. -
logical operators
&
,|
,&&
,||
for sparses matrix managed. -
doxygen documentation generated by Github CI for each release.
-
--noaudio
CLI argument disable audio (module) in Nelson. -
#783: Fedora 37 support.
-
an example to build mexFunction using Rust.
-
geometry module:
rotx
: 3x3 transformation matrix for rotations around x-axisroty
: 3x3 transformation matrix for rotations around y-axisrotz
: 3x3 transformation matrix for rotations around z-axis
-
spones
replaces non zero sparse matrix elements with ones. -
rot90
faster for 2D matrix.
Changed
-
timeout
--timeout
CLI argument andsystem
with timeout return error code258
on Windows and134
others platforms. -
test_run
catchs error code about test aborted (timeout). -
exit
does not allow value > 255 on Linux and MacOS. -
#776: internal dependencies between modules reworked.
-
Uses MKL or OpenBlas if detected on linux and MacOs (Nelson is faster on Linux and MacOs).
Fixed
-
basic terminal was closed immediately if stdin was null.
-
Github Actions CI warnings.
-
#778: ArchLinux Github CI was broken.
0.6.10 (2022-10-31)
Added
-
#750: Qt 6.4.0 support (win64 release embed Qt6.4.0).
-
#733: minimal build and optional installation on MacOs, Linux and Windows.
Fixed
-
#762: MacOs CI was broken (libomp no more detected).
-
#776: CodeQL Analyze was broken.
-
#739: special case with empty cell.
-
buildhelp did not return some errors.
Changed
-
#755: Boost 1.80 support (default on Windows)
-
#753: move from boost::filesystem to std::filesystem. (It should have no impact for users ...)
-
Path functions returns sensitive path on Windows.
cd ('c:/program files')
returns true pathC:/Program Files
. -
fileparts builtin updated to be more compatible.
-
normalizePath internal function reworked.
-
internal files functions reworked and factorized.
0.6.9 (2022-09-28)
Added
-
linear algebra module:
rref
: Gauss-Jordan elimination.orth
: Range space of a matrix.subspace
: Angle between two subspaces.planerot
: Givens plane rotation.condeig
: Condition number with respect to eigenvalues.bandwidth
: Lower and upper matrix bandwidth.isbanded
: Determine if matrix is within specific bandwidth.
-
audio module:
sound
: Convert matrix of signal data to sound and play it.soundsc
: Scale data and play as sound.mu2lin
: Convert audio data from mu-law to linear signal.lin2mu
: Convert audio data from linear singal to mu-law.
-
elementary functions module:
toeplitz
: Toeplitz matrix.hadamard
: Hadamard matrix.wilkinson
: Wilkinson's eigenvalue test matrix.vander
: Vandermonde matrix.rosser
: Classic symmetric eigenvalue test problem.nextpow2
: Next higher power of 2.permute
: Permute array dimensions.ipermute
: Inverse permute array dimensions.rot90
: Rotate array 90 degrees.
-
special functions module:
dot
: Dot product.cross
: Cross product.
-
string module:
isletter
: Determine which characters are letters.
-
data analysis module:
cumsum
: Cumulative sum.cumprod
: Cumulative product.
Changed
-
#715: update
{fmt}
to 9.1.0. -
Nelson uses
simdutf
library to convert characters encoding faster. -
shorcuts
&&
and||
reworked. -
assign behavior with empty matrix.
-
#702: Ubuntu 18.04 CI removed on Github.
-
Remove definitively Appveyor CI.
-
C++ API:
getContentAsDoubleScalar
,getContentAsSingleScalar
,getContentAsInteger8Scalar
, ... ,getContentAsInteger64Scalar
can check if it is an integer value. -
addgateway
,removegateway
,gatewayinfo
builtin moved inmodules_manager
module (reduces dependency)
Fixed
-
#722: MS MPI runtime v10.1.2.
-
#723: MacOS CI was broken.
-
#737: profiler failed with
sind
example. -
#739: special case with empty cell.
-
#741:
ones
andzeros
do not manager logical as typename.
0.6.8 (2022-08-27)
Added
-
#573:
diff
differences and discret derivatives. -
peaks
: peaks function. -
parallel module:
fetchNext
: Retrieve next unread outputs from FevalFuture array.afterAll
: Run function after all functions finish running in the background.afterEach
: Run function after each functions finish running in the background.
-
signal processing module:
hann
: Hann window.hamming
: Hamming window.bartlett
: Bartlett window.blackman
: Blackman window.sinc
: sinc function.filter2
: 2-D digital filter.fft2
: Two-dimensional discrete Fourier Transform.
-
time functions:
weekday
: Day of week.eomday
: Last day of month.etime
: Time elapsed between date vectors.date
: Current date as character vector.timeit
: Measure time required to run function.addtodate
: Modify date number by field.
-
trigonometric functions:
rad2deg
: Convert angle from radians to degrees.deg2rad
: Convert angle from degrees to radians.cart2pol
: Transforms Cartesian coordinates to polar or cylindrical.pol2cart
: Transforms polar or cylindrical coordinates to Cartesian.cart2sph
: Transforms Cartesian to spherical coordinates.sph2cart
: Transform spherical coordinates to Cartesian.sech
: Hyperbolic secant.secd
: Secant of argument in degrees.sec
: Secant of angle in radians.csch
: Hyperbolic cosecant.cscd
: Cosecant of argument in degrees.csc
: Cosecant of argument in radians.coth
: Hyperbolic cotangent.cot
: Cotangent of argument in radians.cotd
: Cotangent of argument in degrees.atand
: Inverse tangent in degrees.atan2d
: Four-quadrant inverse tangent in degrees.sinpi
: Computes sin(X * pi) accurately.cospi
: Computes cos(X * pi) accurately.asind
: Inverse sine in degrees.asinh
: Inverse hyperbolic sine.acosh
: Inverse hyperbolic cosine.acosd
: Inverse cosine in degrees.acoth
: Inverse hyperbolic cotangent.acsc
: Inverse cosecant in radians.acscd
: Inverse cosecant in degrees.acsch
: Inverse hyperbolic cosecant.asec
: Inverse secant in radians.asecd
: Inverse secant in degrees.asech
: Inverse hyperbolic secant.acot
: Inverse cotangent in radians.acotd
: Inverse cotangent in degrees.
-
prettier is used to format .xml, .md files.
-
Code format (.xml, .md, c++) checker added to Github CI.
Changed
-
system
builtin:- returns time execution
- timeout input argument can be set to kill process after n seconds.
-
#687: Uses BS thread pool 3.3.0.
-
Windows 32 bits threads support: due to threading limitations with MKL, openMP and others threads computation threads are limited to 4 threads on Windows 32 bits.
-
#683: MacOS Catalina removed on Github CI (no more officially supported).
-
Help chapter titles all start with a capital letter.
-
#771: Fedora 36 Github CI replaces Fedora 35 Github CI.
Fixed
- #689:
c = computer
returns an error. - #691: help generation crashs if copyright tag is empty.
- #35: datenum does not support vectorization.
or
,and
do not manage matrix operator scalar case.
0.6.7 (2022-07-27)
Added
-
str2func
manages anonymous function. -
Parallel functions (part 2):
cancelAll
: Stop all functions running in the background.cancel
: Stop function running in the background.wait
: Wait for futures to be completed.Diary
property forFevalFuture
object.
-
CITATION.cff with human- and machine-readable citation information for software.
Changed
- localization files
.pot
,.po
updated.
Fixed
- #679: uniformize path for includes and libraries.
0.6.6 (2022-06-27)
Added
-
Parallel functions (part 1):
parfeval
: Run function on parallel background thread pool worker.backgroundPool
: Environment for running code in the background.fetchOutputs
: Retrieve results from function running in the background.
-
#666:
ls
function: List folder contents. -
#655: help viewer embedded (available on all platforms).
Changed
-
LGTM replaced by CodeQL (Thanks to Github for their support)
-
#662: Snap uses Core22 and Qt6 (all dependencies are up-to-date).
-
#668:
maxNumCompThreads
detects numbers of physical cores on Windows. -
matrix of handles supported.
-
#676: use fmtlib 9.0
Fixed
0.6.5 (2022-05-26)
Added
-
#572:
pow2
: Base 2 exponentiation. -
audioread
,audiowrite
supports new file formats.mp3
,.flac
,.caf
(Thanks to libsndfile). -
MacOs 12 Monterey Github CI.
-
#634: Ubuntu 22.04 CI.
Changed
-
#631: Qt6 used as default distribution on some OS platforms.
For backport compatibility only Qt5.15.x and Qt6.3 (or more) are supported.
- Windows 64 bits: Qt6.3
- Windows 32 bits: Qt5.15.x
- Ubuntu 22.04: Qt6.3
- Ubuntu 20.04, 18.04: Qt5.15.x
- MacOs Monterey: Qt6.3
- MacOs BigSur, Catalina: Qt5.15.x
- Others OS platforms: Qt version based on distributed Qt version.
-
#636: docker image uses bullseye image.
-
system
builtin: multithread rework. -
#633: libsndfile 1.1.0 used.
-
Windows build uses
/permissive-
option. -
#646:
warndlg
,questdlg
,errordlg
,helpdlg
reworked to better Qt6 support.
Fixed
-
Dark theme detection with Gnome.
-
#642:
rand
was not thread safe. -
#648: Ubuntu 18.04 CI failed.
-
#651: MacOs monterey crashs at exit with mpi module.
0.6.4 (2022-04-24)
Changed
-
#590: Nelson's license moved to LGPL v3.0 and GPL v3.0 and uses SPDX format.
-
Nelson uses Hack font.
-
Linux 64 bits and 32 bits uses same main script.
-
#594:
.pot
used to generate en_US.
Added
-
'sscanf' reworked to manage unicode characters and speed optimization.
-
French translations imported from Weblate (Thanks to contributors)
-
Dark theme detected and applied on Linux, MacOS and Windows.
-
#559:
formattedDisplayText
function: Capture display output as string. -
Better detection Qt6 or Qt5 with CMake.
Fixed
-
Main Nelson's font was not applied on some OS platforms (ex: MacOS Catalina)
-
#622:
isreal(sqrt(i^4))
did not return true. -
Nelson's help indexing is faster (x100).
0.6.3 (2022-03-26)
Changed
- #596: Tests results display use emoji if terminal supports Unicode.
Added
-
Packaging:
-
add information for Software Center (Linux desktop, icons).
-
nelson
Main script to start Nelson (superceed others scripts). -
isunicodesupported
function: Detect whether the current terminal supports Unicode. -
dlsym
function: search nearest symbol name if value entry is not found. -
terminal_size
function:Query the size of the terminal window. -
#598:
sscanf
function read formatted data from strings.
Fixed
-
#599:
make install
step in CI for linux and MacOs. -
#601: embed all tests on linux and macos install.
0.6.2 (2022-02-26)
Changed
-
#576: C++17 Compiler required to build Nelson.
-
#581: Github CI platforms list extended (ArchLinux, Fedora, Ubuntu 18.04, MacOs BigSur).
-
#539: Visual studio 2022 build on Windows
- Visual studio 2022 solution upgraded,
- Github CI and Appveyor use VS 2022 image,
- boost 1.78 (VS 2022 x86, x64 build),
- Eigen 3.4 stable branch (Feb 06/22),
- MSVC 2022 support added to build C/C++ code easily "on fly" on Windows,
- slicot 5.0 (VS 2022 x86, x64 build),
- libffi (VS 2022 x86, x64 build),
- taglib 1.12 (VS 2022 x86, x64 build),
- hdf5 1.12.1 (VS 2022 x86, x64 build),
- matio 1.5.21 (VS 2022 x86, x64 build),
- all others windows dependencies rebuilt with VS 2022.
- #505: libCurl 7.81 on Windows.
- #524: oneApi 2022.1 on Windows.
Added
- Nelson uses
JuliaMono-Regular
font as default. - #567:
...
in cells if character vector is too long.
Fixed
- #587: implicit cast to string array for horzcat and vertcat operators.
- #562:
format long
complex do not display expected precision. - scale factor for integer values did not display as expected.
- #561:
0^0
did not return expected value. - #560: many warnings fixed (Thanks to new PVS-Studio and cppcheck).
- cmake
WITH_SLICOT
,WITH_FFTW
,ENABLE_CLANG_TIDY_FIX
were not documented. - #584: docker files updated to support C++17 and new libraries.
- #591: Innosetup display glitch with
Nelson's website
button.
0.6.1 (2022-01-31)
Changed
- display of all types reworked to be
pixel perfect
. (a small sentence but a big rework)
Added
format
extended to manage:compact
,loose
,longE
,longG
,hex
,bank
,rational
.- #507:
celldisp
: Display cell array contents. - #548:
hypot
builtin: Square root of sum of squares. - #555:
rsf2csf
function: Convert real Schur form to complex Schur form. CHANGELOG
0.6.x family.
Previous changelog
0.5.12 (2021-12-31)
Added
hankel
function: Hankel matrix.factor
function: Prime factors.primes
function: Prime numbers less than or equal to input value.isrow
function: Determine whether input is row vector.iscolumn
function: Determine whether input is column vector.
Fixed
-
#544: add
folder
fieldname todir
output. -
#541: common class between two elements for operators, horzcat and vertcat.
Compilation
-
Boost 1.78 support (default on Windows).
-
CMake 3.22.1 (on Windows).
0.5.11 (2021-11-26)
Added
hilb
function: Hilbert matrix.invhilb
function: Inverse of Hilbert matrix.cond
function: Condition number for inversion.rank
function: Rank of matrix.ismatrix
function: Determines whether input is matrix.squeeze
function: Removes dimensions of length 1.speye
function: Sparse identity matrix.randperm
function: Random permutation.cat
function: Concatenate arrays.SECURITY.md
file as recommended by Github.
Fixed
vercat
,horzcat
returns an empty array whose size is equal to the output size as when the inputs are nonempty.- #533:
find
with one lhs did not return expected result with complex. - #536:
test_websave_3
failed randomly due to distant server.
0.5.10 (2021-10-30)
-
Polynomial functions:
poly
: Polynomial with specified roots or characteristic polynomial.roots
: Polynomial roots.polyval
: Polynomial evaluation.polyvalm
: Matrix polynomial evaluation.polyint
: Polynomial integration.polyfit
: Polynomial curve fitting.polyder
: Polynomial differentiation.
-
pinv
: Moore-Penrose pseudoinverse. -
#520:
inputname
get variable name of function input. -
#525: use
fast_float
library to parse numbers . -
#528: Assignment in cell did not work in this case
[c{:}] = ind2sub (dv, i)
-
#534:
diag(ones(0, 1), -1)
did not return zero as result.
0.5.9 (2021-09-29)
-
leapyear
function: determine leap year. -
meshgrid
function: Cartesian rectangular grid in 2-D or 3-D. -
sub2ind
function: linear index to matrix subscript values. -
ind2sub
function: matrix subscript values to linear index. -
#518:
isStringScalar
checks if input is string array with one element. -
#516:
ind = 2; ind(false)
logical extraction on scalar should return empty matrix. -
#514:
C{3} = 4
should create a cell with good dimensions. -
#512: Assign must not change left assign type when it is possible.
-
#509: horzcat vertcat generic support for class object.
-
#508: Change default seed for 'rand' with Mersenne Twister algo.
-
#506: Modernize windows installer style.
Compilation:
0.5.8 (2021-08-25)
Features:
-
test_run
displays errors with file and line number. -
CTRL-C throws an error.
-
some warnings detected by LGTM or VS fixed.
-
allows .m file empty to be called.
-
#477: update files watcher algo.
-
#489: display builtin and associated overload.
-
#490: update default prompt.
Bug Fixes:
-
#480: publisher name updated for windows installer.
-
#483: extern modules no more build if boost not available.
-
#486:
inmem
help was missing. -
#464: simplify macos build (catalina & BigSur support only).
-
#499: rename
getContentAsUnsignedInt64Scalar
togetContentAsUnsignedInteger64Scalar
. -
#495: some mtimes call failed.
0.5.7 (2021-07-24)
Features:
-
macros in memory reworked to support also MEX.
-
C MEX compatibility, load and build fully compatible with other softwares.
-
inmem
builtin returns names of functions, MEX-files in memory. -
mexext
builtin returns binary MEX file-name extension. -
main function in .m no more require to be the first in file.
-
checks in the .m function that other local function names are not duplicated.
-
.m timestamp checked if
addpath(...,'-frozen')
is not enabled. -
function_handle reworked to have an compatible behavior.
-
struct
behavior withfunction_handle
. -
clear
reworked to support mex in memory. -
nargin
,nargout
behavior with mex updated. -
#474:
exist
: extended to manage mex function. -
#449:
conv2
: 2-D convolution andconv
: Convolution and polynomial multiplication.
Bug Fixes:
- #468: A(':') = [] was not managed.
0.5.6 (2021-06-27)
BREAKING CHANGE:
Features:
-
function ... endfunction
andfunction ... end
are equivalent (increase compatibility ;). -
file extension
.m
is managed by Nelson.-
About compatibility: scripts and functions developed with Nelson should work with other tools managing .m files. The reciprocal is not necessarily true.
-
.m
is default and alone file extension.
-
-
module skeleton updated to use to
.m
extension (Please update your code) -
run
builtin can also evaluate a macro function. -
macro functions also searched in current directory.
-
parser cleaned and generated with Bison 3.7.4
-
narginchk
builtin: checks number of input arguments. -
nargoutchk
builtin: checks number of outnput arguments. -
#448: data analysis module (Code refactoring).
Bug Fixes:
-
nmm('install', existing_module_directory)
did not work as expected. -
#451: var() returns an unexpected error.
Compilation:
-
#455: M1 macOS apple native support. It works but some gui features can crash due to young Qt support on M1.
-
Update fmt library to 8.0.
0.5.5 (2021-05-24)
Features:
-
Validators functions available from Nelson and C++ API (part 2):
mustBeFile
,mustBeNonempty
,mustBeNonNan
,mustBeNonZero
,mustBeNonSparse
,mustBeA
,mustBeReal
,mustBeInteger
,mustBeNonmissing
,mustBePositive
,mustBeNonpositive
,mustBeNonnegative
,mustBeNegative
,mustBeGreaterThan
,mustBeGreaterThanOrEqual
,mustBeLessThan
,mustBeNumericOrLogical
,mustBeText
,mustBeNonzeroLengthText
,mustBeMember
,mustBeInRange
.
-
test_run
managesSEQUENTIAL TEST REQUIRED
andNATIVE_ARCHITECTURE TEST REQUIRED
tags. -
benchs are executed sequentialy (better bench results).
-
all
,any
behavior with empty matrix updated. -
extends
all
to manage over all elements. -
ismember
builtin: Array elements that are members of another array. -
#439: split elementary_functions module and creates operators modules.
Changed:
- comment symbol is '%'. others previous supported comment symbol removed.
Bug Fixes:
- #435:
maxNumCompThreads
did not return number of threads but number of cores.
Compilation:
-
Move Windows build to GitHub CI. Appveyor is no more the principal build CI for Windows.
-
#441: Circle CI (ArchLinux build) fixed.
-
#357: Curl 7.76.1 on Windows.
0.5.4 (2021-04-24)
Features:
-
Validators functions available from Nelson and C++ API (part 1):
mustBeLogicalScalar
,mustBeLogical
,mustBeFloat
,mustBeFinite
,mustBeScalarOrEmpty
,mustBeVector
,mustBeValidVariableName
,mustBeTextScalar
,mustBeFolder
,mustBeNumeric
.
-
Functions using SIMD extensions:
ceil
,round
,fix
,floor
,abs
,conj
,exp
,sqrt
,log1p
,log10
,log
cos
,sin
,tan
atan2
,acos
,asin
- addition, substraction, multiplication, division vectors.
-
system
allows to run shell command execution in parallel. -
test_run
executes on parallel process. -
extends
assert_checkerror
to check also error identifier. -
isvector
,isscalar
support overload. -
isvarname
builtin: check if input is valid variable name. -
isdir
manages string array. -
time
returns current time as the number of seconds or nanoseconds since the epoch.
Bug Fixes:
0.5.3 (2021-03-24)
Features:
-
#373:
sign
builtin. -
#313:
atanh
builtin: inverse hyperbolic tangent. -
MException
comes default exception in Nelson. -
try, catch
extended to manageMException
. -
throw
,throwAsCaller
,rethrow
functions added. -
error
extended to manage identifier. -
callstack reworks, available with
MException
. -
for loop faster > x2.
-
assignment does not copy matrix.
-
reworks ArrayOfVector (internal).
-
C++ API nargincheck, nargoutcheck helpers added.
-
rename
mexception
toMException
Bug Fixes:
0.5.2 (2021-02-27)
Features:
-
triu
builtin: Upper triangular part of matrix. -
tril
builtin: Lower triangular part of matrix. -
istriu
checks if matrix is upper triangular part of matrix. -
istril
: checks if matrix is lower triangular part of matrix. -
isdiag
: checks if matrix is diagonal.
Compilation:
-
MacOS build uses openBLAS. lapacke included in openBLAS. No more thirdparty repository required for MacOS build.
-
rename ArrayOf::getLength to ArrayOf::getElementCount method.
-
rework simple assignement.
-
add benchs about loop to identify existing bottleneck for next iteration.
-
rework loop to prepare next iteration.
0.5.1 (2021-01-30)
Features:
-
qt_version
builtin: returns the version number of Qt at run-time. -
qt_constant
builtin: returns value of an Qt constant. -
#374:
num2str
builtin: converts numbers to character array.
Bug Fixes:
-
#388: Windows x64 build failed (elementary_functions module was too big).
-
#385:
corrcoef
,mean
,var
,cov
moved in statistics module.
Compilation:
-
0.5 family (CHANGELOG)
-
Eigen 3.3.9 used.
-
libsndfile 1.0.31 on Windows.
-
libboost 1.75 on Windows.
-
fix cirle CI build.
-
#394: Upgrade socket.IO dependency to v3.0.
-
#367: add fftw_init_threads and fftw_plan_with_nthreads to MKL wrapper for FFTW.
-
#356: MKL OneAPI v2021 support.
-
#355: Qt6 support.
-
#317: uses fmtlib.
Previous changelog:
0.4.12 (2020-12-30)
-
eig
builtin: Eigenvalues and eigenvectors. -
det
builtin: Matrix determinant. -
gcd
builtin: Greatest common divisor. -
find
builtin: Find indices and values of nonzero elements. -
ishermitian
builtin: Checks if an matrix is hermitian or skew-hermitian. -
strcat
builtin: concatenate strings horizontally. -
append
builtin: combine strings horizontally. -
corrcoef
function: correlation coefficients. -
cov
function: covariance. -
var
builtin: variance. -
magic
function: magic square. -
mpower
builtin: matrix support added. -
fft
is faster: plan was not correctly cached. -
|
,&
,./
and.^
operators are faster. -
inv
is faster. -
extends
fullfile
compatibility with string type. -
extends
assert_isequal
,isequal
to manage missing type. -
extends
issymmetric
to manage boolean type.
Bug Fixes:
-
#364:
isinf
,isnan
,conj
,double
,single
,real
,imag
are faster. -
#361:
abs
is faster. -
#360:
ctranpose
andtranspose
are faster. -
#353:
N = i; N(1)
returned wrong value. -
#351: binary operators and empty matrix (behavior described in book of Carl de Boor in An Empty Exercise)
Compilation:
- Qt 5.15.2 on Windows (AppVeyor CI).
0.4.11 (2020-11-24)
-
Nelson Engine API for C (compatible with MEX Engine 100%).
- engSetVisible,
- engGetVisible,
- engEvalString,
- engOutputBuffer.
-
sha256
builtin: get sha256 checksum of a file or a string. -
ipc
extended withminimize
argument. -
fullfile
builtin: build full file name from parts.
Bug Fixes:
-
#342: disable slicot on Macos CI.
-
#341: extend
ipc(pid, 'post', cmd, scope)
to manage scope destination. -
#314: Nelson crashs randomly at exit with Qt 5.15.0
Compilation:
- Qt 5.15.1 on Windows (AppVeyor CI).
0.4.10 (2020-10-29)
-
[IN PROGRESS] Nelson Engine API for C (compatible with MEX Engine).
- engOpen,
- engOpenSingleUse,
- engClose,
- engEvalString,
- engPutVariable,
- engGetVariable.
-
extends
mex
function to generate also executable. -
extends
dlgeneratemake
function to generate also executable. -
--minimize
command line argument added. minimize main GUI Window at startup.
Bug Fixes:
-
#340:
evalin
did not restore correctly variables after call. -
#339:
cd
,dir
,ls
had some compatibility troubles. -
#332: removes connect(2) call to /dev/shm/jack-0/default/jack_0 failed (err=No such file or directory).
-
#331: move ipc features to detected module
Compilation:
-
SEMAPHORE CI platform updated to Ubuntu 14.04 - GCC GNU 4.8.4 (supported until it is no more possible)
-
cmake binaries_directory supported (LGTM support).
-
fix some warnings detected with LGTM.
-
Eigen 3.3.8 stable on all platforms (mirror url also updated).
0.4.9 (2020-09-27)
-
ipc builtin: Inter-process communication between Nelson's process
-
getpid('running') renamed getpid('available').
-
--noipc command line argument added. disable IPC features.
-
dark theme detected and used on Macos X.
-
test_run reworked (faster to start).
-
test_run extended with '-stoponfail' option.
-
jsonencode faster for string encoding.
-
rework timeout thread.
Bug Fixes:
- #330: removes ALSA errors and warnings on linux.
Compilation:
-
#322: fix build with gcc 4.8 (ubuntu 14.04).
Nelson 0.4.9 will be last to support gcc 4.8
-
libsndfile 1.0.30 on Windows.
0.4.8 (2020-08-26)
-
multiplatforms files association based on Inter-process communication. open, load, execute files in latest created Nelson's process.
-
event loop and command queue updated.
-
fix play, playblocking, resume builtin.
-
getpid() returns current process identificator.
-
getpid('running') returns all nelson processes identificators currently running for current user.
-
hostname() returns current host name of your computer.
-
username() returns current user name used on your computer.
-
isvector checks if input is an vector.
Compilation:
-
libffi 3.3 was not detected on Macos X.
-
libicu4c 67.1 was not detected on Macos X.
-
Qt 5.15 official package was not detected on Macos X.
-
libsndfile 1.0.29 on Windows.
-
libcurl 7.72.0 on Windows.
-
libbost 1.74.0 on Windows.
-
CMake 3.18.1 used on Windows.
-
GitHub CI MacOS x Catalina.
0.4.7 (2020-07-31)
-
#311: betainc builtin: Incomplete beta function.
-
add icon to figure
-
some doxygen comments about mex functions.
-
#299: extends "complex" to manage sparse matrix.
Bug Fixes:
- #300: nmm returns wrong error.
Compilation:
- Qt 5.14.2 on Windows
0.4.6 (2020-06-27)
-
[IN PROGRESS] C MEX API:
-
extends mex function to manage interleaved complex option and c flags.
-
all C MEX API implemented, full API documentation and examples in progress.
-
mxMakeArrayReal, mxMakeArrayComplex functions.
-
mxGetImagData, mxSetImagData functions.
-
mxGetLogicals, mxIsLogicalScalar, mxIsLogicalScalarTrue functions.
-
mxGetInt8s, mxSetInt8s, mxGetComplexInt8s, mxSetComplexInt8s, mxGetUint8s, mxSetUint8s, mxGetComplexUint8s mxSetComplexUint8s, mxGetInt16s, mxSetInt16s, mxGetComplexInt16s, mxSetComplexInt16s, mxGetUint16s, mxGetComplexUint16s mxSetComplexUint16s, mxGetInt32s, mxSetInt32s, mxGetComplexInt32s, mxSetComplexInt32s, mxGetUint32s, mxSetUint32s mxGetComplexUint32s, mxSetComplexUint32s, mxSetUint16s, mxGetInt64s, mxSetInt64s, mxGetComplexInt64s, mxSetComplexInt64s mxGetUint64s, mxSetUint64s, mxGetComplexUint64s, mxSetComplexUint64s functions.
-
mxIsObject, mxIsFunctionHandle, mxIsOpaque functions.
-
mxIsInt8, mxIsInt16, mxIsInt32, mxIsInt64, mxIsUint8, mxIsUint16, mxIsUint32, mxIsUint64 functions.
-
mxCreateStringFromNChars, mxGetNChars
-
mxRemoveField, mxAddField, mxSetField, mxSetFieldByNumber, mxGetFieldNumber, mxGetFieldNameByNumber functions.
-
mexGetVariable, mexGetVariablePtr, mexPutVariable functions.
-
mexMakeArrayPersistent, mexMakeMemoryPersistent functions.
-
Compilation:
-
boost 1.73.0 on Windows.
-
ninja-build used with github actions CI.
0.4.5 (2020-05-23)
-
graphics object type added.
-
figure builtin: creates figure.
-
gcf builtin: get current figure.
-
groot builtin: returns graphic root object.
-
get, set, isvalid, class, fieldnames, delete, disp builtin overloaded to manage graphics objects.
-
test_run: tests are sorted on all platforms.
-
[IN PROGRESS] C MEX API:
-
C MEX supports build with MinGW compiler.
-
mxArray and ArrayOf conversion optimized.
-
C MEX interleaved complex support.
-
C MEX Sparse type fully supported.
-
mxGetClassName, mxSetClassName fully supported.
-
mxGetProperty, mxSetProperty fully supported (handle, graphics object, ...).
-
Bug Fixes:
- #295: sort did not return an wrong error message for struct.
Compilation:
-
libcurl 7.70.0 on Windows.
-
cmake 3.17.2 on Windows.
-
CA certificate (Wed Jan 1 04:12:10 2020 GMT)
0.4.4 (2020-04-29)
-
lookandfeel builtin: default current application look and feel.
-
clear builtin extended to clear mex functions.
-
mex function used to build MEX files.
-
[IN PROGRESS] MEX C API allows to access Nelson, GNU Octave and commercial software functions.
documentation and tests will be extended in next version.
Feedback and external tests are welcome.
Compilation:
-
MacOs X Catalina fully working.
-
Ubuntu 20.04 LTS supported.
0.4.3 (2020-03-30)
-
mean builtin: Mean elements of an array with nanflag and 'all' support.
-
sum and prod optimized.
-
save and load with .mat, .nh5 files support unicode filename on all platforms.
-
simplify builtin default prototype (breaking change). Evaluator is no more required for builtin.
-
NelsonPrint internal function added.
Bug Fixes:
-
#287: Parser error message are not localized.
-
#286: [end] = sin(1) did not return an syntax error.
-
#284: Nth dimensions assignation of an empty array with 2d matrix did not work.
Compilation:
-
MATIO 1.5.17 with unicode support
-
HDF5 1.12.0 support
-
BISON 3.5.0
0.4.2 (2020-02-25)
-
min, max builtin: Minimum/Maximum elements of an array with nanflag and 'all' support.
-
flipud: Flip array up to down.
-
fliplr: Flip array left to right.
-
flip: Flip order of elements.
-
flipdim: Flip array along specified dimension.
-
log2 builtin: Base 2 logarithm and floating-point number dissection.
-
colon operator optimized.
-
faster algorithm to convert variable to different data type.
-
replaces hashmap used for functions and variables.
-
some few speed optimization about evaluator.
0.4.1 (2020-01-27)
-
rework and speed optimization for times, divide, addition, subtraction operators.
-
sum builtin: sum of array elements.
-
linspace builtin: linearly spaced vector constructor.
-
logspace builtin: logarithmically spaced vectors constructor.
-
log10 builtin: Common logarithm (base 10).
-
log1p builtin: log(1+x) accurately for small values of x.
-
replaces dot animation by percent display about help indexing.
-
html style about table simplified.
Compilation:
-
uses ASIO C++ library in place of BOOST ASIO.
-
Add Qt 5.14.0 support.
-
OPEN MP support added.
Previous changelog:
0.3.12 (2019-12-27)
-
sort builtin: sort double, single, integers, cell and strings.
-
diag builtin: Get diagonal elements of matrix or create diagonal matrix.
-
Continous Integration tools for external modules (see module skeleton example).
-
modules installed with nmm are 'autoload' by default.
-
.nmz file extension used as module container.
-
nmm('package', module_name, destination_dir) package an external module.
-
nmm('install', module.nmz) installs a prebuilt external module.
-
'-frozen' option added to addpath builtin.
-
rmfield builtin: Remove fields from structure.
-
extends sprintf and fprintf to manage backspace characters.
-
fix display of vector with NaN or Inf as first element.
Bug Fixes:
- #260: disable files watch for internal modules.
0.3.11 (2019-11-26)
-
nmm: Nelson Modules Manager (package manager for Nelson)
- list : get list of installed modules,
- load : load an installed module for current session,
- autoload : load modules "marked" as autoload at startup,
- install : install a distant module,
- uninstall : uninstall an installed module.
-
Module skeleton moved to an dedicated git repository
- template with builtin and macros: https://github.com/nelson-lang/module_skeleton
- template with macros only: https://github.com/nelson-lang/module_skeleton_basic
-
usermodulesdir builtin: returns directory where user's modules are saved.
-
toolboxdir builtin: Root folder for specified toolbox.
-
nmm_build_help, nmm_build_loader: helper's functions to build module skeleton.
-
semver builtin: semantic versioner.
-
executable option added: '--nousermodules' disables load of user's modules.
-
add capability to load some user's modules: see nmm('autoload', ...) and nmm('load', ...)
-
add // <--NO USER MODULES--> tag for test_run (disable load of user modules for a test)
-
fullpath builtin: converts an relative path to full path name.
-
getLastReport builtin: returns last formatted error message.
-
extends repo to manage plain text authentification.
-
repo('export', ...) exports an git repository without .git directory.
-
getfield macro replaced by an builtin.
-
extends isequal, isequaln, isequalto for structure arrays.
Bug Fixes:
-
#261: add a detailed documentation about module.json used in external modules.
-
#259: extraction decomplexify values.
-
#257: dllibisloaded optimized.
-
#49: Some qml demos crashed on Windows 32 bits.
Compilation:
- Qt 5.13.2 on Windows.
0.3.10 (2019-10-29)
-
extends 'getmodules' to return module versions using new required 'module.json' (see module's template).
-
all core's modules are protected and cannot removed during an nelson's session.
-
increase max execution time for tests (2 minutes) and benchs (6 minutes).
-
split benchs and tests execution for CI.
-
repo builtin: clone, checkout branch or tag, ... from an GIT repository.
Compilation:
-
Visual studio 2019 Community and Pro upgrade (required) Dependencies updated:
- ICU 64.2 on Windows
- libffi updated VS 2019 build
- libxml 2.9.9 VS 2019 build
- libcurl 7.66.0_2
- CMake 5.15.3 update for Windows
- MKL 2019 update 5
- HDF5 1.10.5 VS 2019 build
- MATIO 1.5.17 VS 2019 build
-
appveyor script updated to build with VS 2019
-
Innosetup 6 support
Bug Fixes:
-
#254: fix Innosetup 6 warnings.
-
#252: help files of external modules were not loaded.
-
#245: Update MKL 2019 dependencies.
-
#202: Migrate to VS 2019.
0.3.9 (2019-09-25)
Features:
-
namedargs2cell builtin: Converts a struct containing name-value pairs to a cell.
-
extends 'size' builtin to find lengths of multiple array dimensions at a time.
-
matches builtin: Determine if pattern matches with strings.
-
webwrite function: Write data to RESTful web service.
-
improves error message raised by 'run' builtin.
-
extends size, length, ndims to manage function_handle type.
-
fscanf builtin: Read data from text file.
Bug Fixes:
-
#249: refactor code.
-
#236: mpiexec returned warning on docker as root user.
-
#235: add example about function_handle with webread.
-
#233: fix typo in native2unicode help file.
-
#227: Qt 5.13 support.
-
#220: setfield function added.
-
revert b22ae88a6536cd614f555af7fbd865cc607bea7f due to HDFFV-10579.
-
changes file or directory permission had a speed cost.
Compilation:
-
Qt 5.13.0 on Windows.
-
GitHub Actions CI (Ubuntu 18.04)
-
#244: Remove semaphore CI 2.0 build.
0.3.8 (2019-08-24)
Features:
-
RESTfull API webservice for Nelson:
- weboptions function: Set parameters for RESTful API web service.
- websave builtin: Save content from RESTful API web service to file.
- webread builtin: Read content from RESTful API web service to nelson's variable.
-
UNICODE support extended in Nelson:
- unicode2native builtin: Converts unicode characters representation to bytes representation.
- native2unicode builtin: Converts bytes representation representation to unicode string representation.
- nativecharset builtin: Find all charset matches that appear to be consistent with the input.
- text editor detects files charset and open files with it.
- fileread / filewrite builtin extended to use an characters encoding.
- fopen, fprintf, fgetl, fgets, fread, and fwrite builtin extended to manage characters encoding.
-
feof builtin: check for end of file.
-
ferror builtin: test for i/o read/write errors.
-
tempname function: Returns an unique temporary filename.
-
test_run uses nh5 files as result file (previously json)
Bug Fixes:
Compilation:
-
Visual studio 15.9.14.
-
Qt 5.12.4 on Windows.
-
CMake 3.9 required on linux and MacOS.
-
CircleCI moved to Arch Linux build.
0.3.7 (2019-07-23)
Features:
-
dec2base builtin: Convert decimal number to another base.
-
dec2bin builtin: Convert decimal number to base 2.
-
dec2hex builtin: Convert decimal number to base 16.
-
base2dec builtin: Convert number in a base to decimal.
-
bin2dec builtin: Convert number in base 2 to decimal.
-
hex2dec builtin: Convert number in base 16 to decimal.
-
flintmax builtin: Largest consecutive integer in floating-point format.
-
realmax builtin: Largest positive floating-point number.
-
struct builtin extended to convert object created by 'class' to structure.
Bug Fixes:
Compilation:
-
#212: MATIO 1.5.16 used all platforms.
-
#211: BOOST 1.70 on Windows platforms.
-
allocateArrayOf and new_with_exception no more set memory to zero by default. This speed up array constructors.
0.3.6 (2019-06-26)
Features:
-
num2bin builtin: Convert number to binary representation.
-
bin2num builtin: Convert two's complement binary string to number.
-
swapbytes builtin: endian converter.
-
zip/unzip builtin: Compress/Uncompress files natively into zip file (with Unicode support).
-
license function: Get license information for Nelson.
Compilation:
- update travis-CI script to support Ubuntu 16.04.
0.3.5 (2019-05-26)
Features:
-
Licensing change: Nelson is now released under the terms of the GNU Lesser General Public License (LGPL) v2.1. It is still also available under the terms of the GNU General Public License (GPL) v2.0.
You can build Nelson under LGPL v2.1 license on Unix/MacOS with
cd nelson cmake -DLGPL21_ONLY=ON -G "Unix Makefiles" .
On Windows, it is also easy, if you do not select SLICOT library during setup.
-
FFTW Wrapper allows to load dynamically FFTW library available on platform.
-
SLICOT Wrapper allows to load dynamically SLICOT library available on platform.
-
unix, dos, system builtin reworked (asynchronious, better pipes redirection, detached process). This function can be interrupted with CTRL-C key.
-
MSVC 2019 support added to build C/C++ code easily "on fly" on Windows.
Bug Fixes:
-
#198: history load and save will be disable if nelson is started with '--nouserstartup'.
-
#196: call cmake 3.11 from Nelson fails on linux.
Compilation:
-
remove hardcoded path between dynamic libraries on linux and macos. It will allow to package nelson easily.
-
BOOST 1.64 or more required
-
Build on MacOs X 10.13.6 and 10.14.5 (SD notary currently not supporterd.)
-
add Dockerfile for Arch, Debian, Fedora images used for CI.
-
SLICOT library removed from Nelson's source and moved here.
0.3.4 (2019-04-27)
Features:
-
Coverage and Profiling Tools for Nelson's language:
- profile function: Profile execution time for functions
- profsave function: Save profile report in HTML format
-
blanks builtin: creates an string of blank characters.
-
.nh5 files have an header to identify it easily.
-
isnh5file, ismatfile extended to return header string.
Bug Fixes:
- #193: func2str help was wrong.
Compilation:
-
MATIO 1.5.15
Thanks to MAT file I/O library (MATIO) to provide an easy support for MAT-file.
-
more 100 warnings fixed (Thanks to PVS-Studio analyzer and also Cppcheck).
-
.editorconfig file added.
-
Visual studio 15.9.11
-
Qt 5.12.2 on Windows
0.3.3 (2019-03-21)
Features:
-
load, save MAT-files:
- load overloads loadnh5, loadmat functions.
- save overloads savenh5, savemat functions.
- .mat file extension support added: data formatted (Nelson workspace).
- .mat file association on Windows. load MAT file as data formatted for Nelson.
- loadmat: load mat-file into Nelson's workspace
- savemat: save Nelson's workspace to .mat file.
- rename h5save to savenh5, h5load to loadnh5
-
whos: list variables with sizes and types.
- whosmat: list variables in an valid .mat file with sizes and types.
- whosnh5: list variables in an valid .nh5 file with sizes and types.
-
extends who to manage '-file' option.
- whomat: list variables in an valid .mat file.
- whonh5: list variables in an valid .nh5 file.
-
ismatfile: check if a file is a valid .mat file.
-
isnh5file: check if a file is a valid .nh5 file.
Compilation:
-
MATIO 1.5.13
Thanks to MAT file I/O library (MATIO) to provide an easy support for MAT-file.
-
BOOST 1.69 (Windows)
-
CMake 3.14 (Windows)
0.3.2 (2019-02-24)
Features:
-
h5save: save Nelson's workspace to .nh5 file.
-
h5load: load data form .nh5 file into Nelson's workspace.
-
save, load alias on h5save and h5load.
-
.nh5 file extension support added: data formatted (Nelson workspace).
-
.nh5 file association on Windows. load data formatted for Nelson.
Compilation:
-
Qt 5.12.1 on Windows
-
fix some 32 bit Warnings.
0.3.1 (2019-01-26)
Features:
- #173: convertStringsToChars and convertCharsToStrings builtin.
Bug Fixes:
-
#182: Nelson did not start without hdf5 dependency.
-
#179: isfolder alias on isdir.
-
#177: some tests failed when it executed from a binary version on windows.
-
#176: nfilename did not return canonical path name in some cases.
-
#9: tests were not embedded in linux & macos binaries (make package).
-
#4: nelson.pot generated from sources.
Compilation:
-
MKL 2019.1 updated for blas, lapack, lapacke, fftw wrappers on Windows.
-
Nelson deployed/installed on appveyor.
-
Nelson available as portable zip file for Windows.
-
Update Visual studio solution to SDK 10.17763
-
Update International Components for Unicode 63.1 (Windows and MacOs)
Previous changelog:
0.2.12 (2018-12-31)
Features:
HDF5 high-level functions:
- h5dump: Dump the content of hdf5 file as text.
- h5ls: List the content of an hdf5.
- h5writeatt: Writes HDF5 attribute.
- h5readatt: Read HDF5 attribute.
- h5write: Writes HDF5 data set.
- h5readatt: Read HDF5 data set.
- h5create: Creates a data set.
Bug Fixes:
Compilation:
- BOOST 1.68 on Windows
- Eigen 3.3.7
0.2.11 (2018-11-24)
Features:
-
Nelson in the cloud:
- easy way to deploy (npm package and docker)
- add 'sioemit' builtin. Send data from Nelson to client.
- add 'siogetvariable' function. Send variable value to cliend.
- add nelson_sio_client executable used by Nelson in the cloud framework.
-
addition, substration, mtimes, times, power with integers reworked.
-
integer cast optimized.
-
modernize/optimize C++ with LLVM tools.
0.2.10 (2018-10-20)
Features:
-
string array type added:
- strings, string builtin: string array constructor.
- isstring: Return true if variable var is a string array.
- parser updated to manage string array:
A = ["Nelson" "manages"; "string" "array"]
- isequal, isequaln, isequalto extended to manage string array.
- transpose, ctranspose extended to manage string array.
- horzcat, vertcat extended to manage string array.
- cell extended to manage string array.
- double extended to manage string array.
- tolower, toupper extended to manage string array.
- strtrim extended to manage string array.
- strlength extended to manage string array.
- count extended to manage string array.
- contains extended to manage string array.
- startsWith, endsWith extended to manage string array.
- replace, strrep extended to manage string array.
- str2double extended to manage string array.
- fprintf, sprintf extended to manage string array.
- strfind extended to manage string array.
- strcmp, strcmpi, strncmp, strncmpi extended to manage string array.
- fileread, filewrite extended to manage string array.
- operators ==, ~=, <, >, >=, <= extended to manage string array.
- operator plus extended to manage string array.
- loadbin, savebin extended to manage string array.
- MPI interface extended to manage string array.
-
deblank builtin: removes trailing whitespace from a cell of strings, a string array or a character vectors.
-
ismissing builtin: search missing values.
-
cellstr function: converts to cell array of character vectors.
-
operators ==, ~=, <, >, >=, <= reworked (Compatiblity Array Sizes increased).
-
==, ~=, isequal: speed optimization.
-
internal API C++ methods renamed:
- "stringConstructor" --> "characterArrayConstructor".
- "isString" --> "isCharacterArray"
- "isSingleString" --> "isRowVectorCharacterArray"
-
#164: operators ==, ~=, <, >, >=, <= code factorized.
-
#159: addpath must return an warning and not an error for an non existing path.
-
#157: fix warnings detected by LGTM.com.
-
#156: Moves dbstack in 'debugger' module.
0.2.9 (2018-09-23)
Features:
-
Overload and speed optimization:
- all operators use by default predefined functions. Nelson will search if operators are missing for a specific type.
- add overloadbasictypes function to change default behavior.
- gamma function speed x4 (overloading)
-
power element-wise operator reworked (overloading and mixed types).
-
colon operator reworked (overloading and mixed types).
-
ndarray subclass no more exists. class merged with basic type (speed optimization).
-
isequalto builtin added (returns true if all arguments x1, x2, ... , xn are equal i.e same type, same dimensions, same values or NaNs).
-
tanm, sinm builtin added.
-
strtrim builtin: removes leading and trailing whitespace from a cell of strings or a string.
-
cell & struct managed for complex transpose & transpose.
-
for, index column or matrix: behavior changed.
Warning: It is a breaking feature.
// Please replace: A = [1:4]'; for i = A, x = i + 1, end // by: A = [1:4]'; for i = A(:)', x = i + 1, end
Bug Fixes:
-
#133: Replaced uncommon term "trinary" by "ternary".
-
#119: Execution of simple expression "1+2+3" was rather slow.
-
#115:code about single & double operators was factorized
-
#114: Move gamma function in a dedicated module "special_functions".
Compilation:
- Enable LGTM.com analysis for C/C++ code.
0.2.8 (2018-08-26)
Features:
-
error manager reworked.
- internal C++ function did no more require reference to evaluator.
- error and warning internally managed as exceptions.
-
'dbstack' builtin get current instruction calling tree.
-
#16: lastwarn builtin (Last recorded warning message).
-
#15: warning builtin was extended (state and identifier added).
Bug Fixes:
-
#152: insertion did not return expected result for empty matrix.
-
#138: colon operator did not return expected for non scalar element.
Compilation:
- Qt 5.11.1 on Windows
0.2.7 (2018-07-29)
Features:
-
sqrt builtin (Square root)
-
log builtin (Natural logarithm)
-
angle function (Phase angle)
-
atan2 builtin (four-quadrant inverse tangent)
-
exp builtin (exponential)
-
#142: clear('functionName') clears all persistent variables of functionName function.
-
addition, substraction reworked (Compatiblity Array Sizes increased, code factorized).
-
&, |, &&, || operators reworked (Compatiblity Array Sizes increased).
-
havecompiler uses a persistent variable to speedup result.
Bug Fixes:
0.2.6 (2018-06-26)
Features:
-
Nelson provides a cross-platform command-line tool written in Nelson for compiling native addon modules for Nelson. It takes away the pain of dealing with the various differences in build platforms:
-
helper's functions to build C/C++ code easily on Windows, Linux, MacOS X:
- dlgeneratemake: generates a makefile for building a dynamic library.
- dlgeneratecleaner: generates cleaner.nls file for C++ gateway.
- dlgenerateloader: generates loader.m file for C++ gateway.
- dlgenerateunloader: generates unloader.m file for C++ gateway.
- dlgenerategateway: generates C++ gateway.
- findcmake: find CMake path.
- cmake function: call CMake tool.
- dlmake: call make or nmake tool.
-
detect and configure C/C++ compilers on Windows, Linux, MacOS:
-
On Windows:
- VS 2017 Professional, Entreprise, Community supported.
- MinGW-W64 for 32 and 64 bit supported.
- By default, Nelson does not try to detect a C/C++ compiler on Windows. Do not forget to run 'configuremsvc' or 'configuremingw' once.
-
On Linux:
- GNU C/C++ Compilers, Clang.
-
MacOS X:
- Xcode or GNU compiler available via Homebrew .
-
-
havecompiler function: returns if a compiler is configured.
-
configuremingw function: select and configure Mingw-w64 compilers.
-
configuremsvc function: select and configure Microsoft compilers.
-
loadcompilerconf function: load compiler configuration
-
removecompilerconf function: remove compiler configuration
-
vswhere function: detects easily modern Microsoft compilers.
-
-
module skeleton updated to build an example with C++ function (cpp_sum).
-
sprintf, fprintf functions: format data into a string or a file.
-
add "<--C/C++ COMPILER REQUIRED-->" tag managed by test engine.
-
add "<--INDEX 64 BIT REQUIRED-->" tag managed by test engine.
-
norm function: matrix and vectors norms.
-
#128: code indented with clang-format (webkit coding style)
Bug Fixes:
-
#139: fix(1e10) returned a wrong value.
-
#136: Corrected predecence of Colon ":" operator.
-
#134: Evaluation of Non-Scalar If-Condition Expression was not managed.
-
#116: fix display size of big sparse matrix.
Compilation:
- libffi 3.2.1 dll on Windows 32 & 64 bit
0.2.5 (2018-05-23)
Features:
- logm: matrix logarithm.
- sqrtm: square root of a square matrix.
- eval function: evaluates string for execution in Nelson.
- evalin function: evaluates string for execution in Nelson in a specified scope.
- evalc function: Evaluate Nelson code with console capture.
- winqueryreg function read the Windows registry (Windows only).
- factorial function.
- gamma function.
- plus, minus, le, ne, ge, gt, lt, eq, colon operators added for all integer types.
- le, ne, ge, gt, lt, eq for mixed single & double types.
- add generic overload mechanism for all integers: integer keyword.
- vertcat and horzcat: mixed concatenations logical with integers, single and double added.
- #110: add isproperty & ismethod to all handle types.
- add "isHandleProperty", "isHandleMethod", "getHandleCategory" C++ API Methods.
- #108: cast function: converts variable to a different data type.
- MPI Module loaded if MPI dependency is available.
Bug Fixes:
-
#125: cosm function was slow.
-
#123: addpath stopped to work after repeatedly call to the same path.
-
#121: home key did not work in GUI terminal on prompt.
-
#118: add information in DEVELOPMENT.md about how to buid Boost on old Ubuntu versions.
-
#109: add missing horzcat, vertcat for all handle types.
Compilation:
- BOOST 1.67 on Windows
- MSMPI 9.0.1 on Windows
- Update Visual Studio 15.7.1
0.2.4 (2018-04-30)
Features:
-
Foreign Function Interface: call C/Fortran functions that are compiled into shared libraries.
- dlopen: loads an dynamic library.
- dlclose: removes/unload dllib object.
- dlsym: loads a C/Fortran symbol for an dynamic library.
- dlcall: C or Fortran Foreign function call.
- dllibinfo: returns list of available symbols in an shared library.
- dllibisloaded: checks if shared library is loaded.
- libpointer: creates an C pointer object usuable in Nelson.
- getdynlibext : returns the extension of dynamic libraries.
-
#101: allows cell_vertcat_generic & cell_horzcat_generic.
Bug Fixes:
#106: update slicot url references.
#104: mpiexec did not work on some linux.
0.2.3 (2018-03-22)
Features:
-
'strlength': length of strings in an cell of strings.
-
'strrep' and 'replace' builtin : replace substring in a string.
-
export AUDIODEV=null disables audio module (used for Travis CI)
-
'xmldoctomd' : Converts xml Nelson help files to markdown format.
-
'buildhelpmd' : Build help of Nelson's modules for GitBook.
see Nelson's GitBook.
0.2.2 (2018-02-25)
Features:
- 'count' : computes the number of occurrences of an pattern.
- 'startsWith' : checks if string starts with pattern.
- 'endsWith' : checks if string ends with pattern.
- 'contains' : checks if string contains a pattern.
- audiometadata can modify metadata tags.
- Qt 5.10 used on Windows binaries
Bug Fixes:
#98: jsonencode was slow with a big file (> 60 Mo).
#97: fileread was slow with a big file (> 60 Mo).
#93: playblocking updated to manage range.
#92: play updated to manage range.
#90: factorize handle objects methods.
0.2.1 (2018-01-30)
Features:
-
pause: pause script execution.
-
keyboard: stop script execution and enter in debug mode.
-
Audio module:
- beep: do a beep sound.
- audiodevinfo: Information about audio device.
- audioplayer: creates an audio player object.
- play: plays audio object.
- playblocking: plays audio object and block execution until finish.
- pause: pauses audio object.
- stop: stops audio object.
- resume: resumes audio object.
- isplaying: returns if audio object is playing.
- audioread: read an audio file.
- audioread: write an audio file.
- audioinfo: get information about audio file.
- audiometadata: get metadata information about audio file.
-
JSON module:
- jsonencode: encodes a Nelson object into a JSON string.
- jsondecode: decodes a JSON string into a Nelson object.
- jsonprettyprint: JSON pretty printer.
-
#89: extends fileread behavior.
-
'filewrite' builtin allows to easily write to file a string array or a cell of strings.
-
'newline' function: returns newline character i.e char(10).
-
ndarraychar_disp was missing.
-
NIG slicot uses json files.
Bug Fixes:
Regenerates help files index if database is empty or wrong (>= Qt 5.9)
#87: struct did not support sparse matrix.
Compilation:
- Update VS 2017 solution to VS 2017 15.5.4
Previous changelog:
0.1.12 (2017-12-24)
Features:
- MPI interface for Nelson
- MPI_Initialized : Indicates whether MPI_Init has been called.
- MPI_Finalize : Terminates MPI execution environment.
- MPI_Init : Initialize the MPI execution environment.
- MPI_Get_processor_name : Gets the name of the processor.
- MPI_Get_version : Return the version number of MPI.
- MPI_Get_library_version : Return the version number of MPI library.
- MPI_Recv : Blocking receive for a message.
- MPI_Send : Performs a blocking send.
- MPI_Barrier : Blocks until all processes in the communicator have reached this routine.
- MPI_Bcast : Broadcasts a message from the process with rank "root" to all other processes of the communicator.
- MPI_Reduce : Reduces values on all processes to a single value.
- MPI_Allreduce : Combines values from all processes and distributes the result back to all processes.
- MPI_Probe : Blocking test for a message.
- MPI_Iprobe : Nonblocking test for a message.
- MPI_Comm_object : Creates MPI_Comm object.
- MPI_Comm_disp : displays MPI_Comm object.
- MPI_Comm_get_name : Return the print name from the communicator.
- MPI_Comm_delete : delete MPI_Comm object in Nelson environment.
- MPI_Comm_used : get used MPI_Comm objects in Nelson environment.
- MPI_Comm_isvalid : check MPI_Comm handle validity.
- MPI_Comm_split : Creates new communicators based on colors and keys.
- MPI_Comm_rank : Determines the rank of the calling process in the communicator.
- MPI_Comm_size : Determines the size of the group associated with a communicator.
- mpiexec : Run an MPI script.
Bug Fixes:
#85: paste in text editor should be limited to the text only.
#84: CTRL + A action in text editor.
#83: save action in text editor do a popup to reload file.
#82: home, end, page down, page up keys shorcut not implement in editor.
Compilation:
- Update VS 2017 solution to VS 2017 15.5.1
0.1.11 alpha (2017-11-25)
Features:
-
#75: Intel Math Kernel Library can be used to replace OpenBLAS and FFTW on Windows.
-
#72: Add a script to check missing help files.
-
add an embedded script editor for Nelson:
- 'edit' builtin added.
- syntax colorization.
- text completion.
- smart indentation.
- drag & drop files.
- files modification notified.
- files association: .nls .nlf
-
add 'smartindent' builtin to indent a .nls or .nlf files.
-
drag & drop .nls (run), .nlf (open with editor) in gui main window.
Bug Fixes:
#80: code editor: keys up, down, left, right behavior
#78: [p,f,e]=fileparts('c:/') did not return the good result
Compilation:
- BOOST 1.65.1 on Windows
0.1.10 alpha (2017-10-29)
Features:
-
SLICOT module:
-
SLICOT (Subroutine Library in Systems and Control Theory) builtin generated with NIG.
-
slicot_ab01od : Staircase form for multi-input systems using orthogonal state and input transformations.
-
slicot_ab04md : Discrete-time / continuous-time systems conversion by a bilinear transformation.
-
slicot_ab07nd : Inverse of a given linear system.
-
slicot_ab08nd : Construction of a regular pencil for a given system such that its generalized eigenvalues are invariant zeros of the system.
-
slicot_ag08bd : Zeros and Kronecker structure of a descriptor system pencil.
-
slicot_mb02md : Solution of Total Least-Squares problem using a SVD approach.
-
slicot_mb03od : Matrix rank determination by incremental condition estimation.
-
slicot_mb03pd : Matrix rank determination by incremental condition estimation (row pivoting).
-
slicot_mb03rd : Reduction of a real Schur form matrix to a block-diagonal form.
-
slicot_mb04gd : RQ factorization with row pivoting of a matrix.
-
slicot_mb04md : Balancing a general real matrix.
-
slicot_mb05od : Matrix exponential for a real matrix, with accuracy estimate.
-
slicot_mc01td : Checking stability of a given real polynomial.
-
slicot_sb01bd : Pole assignment for a given matrix pair (A,B).
-
slicot_sb02od : Solution of continuous- or discrete-time algebraic Riccati equations (generalized Schur vectors method).
-
slicot_sb03md : Solution of continuous- or discrete-time Lyapunov equations and separation estimation.
-
slicot_sb03od : Solution of stable continuous- or discrete-time Lyapunov equations (Cholesky factor).
-
slicot_sb04md : Solution of continuous-time Sylvester equations (Hessenberg-Schur method).
-
slicot_sb04qd : Solution of discrete-time Sylvester equations (Hessenberg-Schur method).
-
slicot_sb10jd : Converting a descriptor state-space system into regular state-space form.
-
slicot_sg02ad : Solution of continuous- or discrete-time algebraic Riccati equations for descriptor systems.
-
slicot_tb01id : Balancing a system matrix corresponding to a triplet (A, B, C).
-
slicot_tg01ad : Balancing the matrices of the system pencil corresponding to a descriptor triple (A-lambda E, B, C).
-
-
Nelson Interface Generator (NIG) allows to generate Nelson builtin from C/Fortran code.
-
add 'isfield' function to check existence of a fieldname in a struct.
-
add 'exist' function to check existence of variable, builtin, function, file or directory.
-
add 'isvar' function to check existence of variable.
-
add 'ismacro' function to check existence of macro.
-
add 'isbuiltin' function to check existence of builtin.
-
add 'f2c' function to convert fortran code to C from Nelson
-
optimize 'vertcat' and 'horzcat' builtin (remove duplicated code).
-
history file saved on your cloud drive if 'OneDrive' environment variable defined.
Bug Fixes:
#74: A=[]; A(end + 1) = 8 failed.
#71: unix & dos did not have help files.
#68: display of nd array of integer were not defined.
#14: error(error_struct) throws an error using the fields of error_struct.
Compilation:
-
SLICOT used for control functions.
-
add Fortran 2 C converter library (based on libf2c forked).
0.1.9 alpha (2017-09-02)
Features:
-
add 'fftshift' and 'ifftshift' functions to shift the zero-frequency component to the center of the spectrum.
-
add 'circshift' function.
-
colon as string managed for deletion, extraction and insertion.
-
add 'rem' builtin. Remainder after division.
-
add 'abs' builtin. Absolute value.
-
add 'repmat' builtin. Replicates and tiles an array.
-
add 'mod' builtin. Modulus after division.
-
add 'maxNumCompThreads' builtin. Set/Get maximum number of computional threads.
Compilation:
-
Nelson Visual Studio solution updated to use 2017 version.
-
On Windows, all dependencies updated to be compatible with VS 2017 runtime.
-
more 50 warnings fixed (Thanks to PVS-Studio analyzer and also Cppcheck).
Bug Fixes:
#60: Y = complex(rand(500), rand(500)) crashed Nelson.
#58: Manages ICU4C 59.1 on macos X.
0.1.8 alpha (2017-08-15)
Features:
-
FFTW module:
- fft, ifft, fftn, ifftn functions based on FFTW library.
- fftw function to manage FFTW wisdom data.
-
prod : product of array elements builtin added.
-
conj : complex conjugate builtin added.
-
transpose and complex conjugate transpose manage all types available in Nelson.
-
APPVEYOR updated to build windows 32 & 64 bits versions.
0.1.7 alpha (2017-07-16)
Features:
-
On Windows, Nelson can read/write all excel 97-2016 file formats (Excel required) based on COM:
- COM_xlsread : read a xls/xlsx file.
- COM_xlswrite : write a xls/xlsx file.
- COM_xlsfinfo : get information about xls/xlsx file.
-
Component Object Model (COM) client interface: binary-interface standard for software components on Windows.
- actxcontrollist : get list all available control services installed on current Windows.
- actxserverlist : get list all available active X services installed on current Windows.
- actxGetRunningServer : get COM handle of an existing COM server.
- actxserver : creates a COM server.
- COM_used : get list of COM handle currently used in current Nelson's session.
- iscom : determines if it is a COM handle.
- overload on : class, delete, disp, fieldnames, get, set, invoke, ismethod, isprop, isvalid, methods.
- add COM handle.
- see examples in [modulepath('com_engine'), '/examples/'] directory.
-
Better management of varargout without output.
-
Add "<--EXCEL REQUIRED-->" tag managed by test engine.
Bug Fixes:
#53: COM_xlsread did not support path with dot '.'
#50: qml demos did not start on adv-cli mode.
0.1.6 alpha (2017-06-19)
Features:
-
The QML engine enables nelson programs to display and manipulate graphical content using Qt's QML framework.
qml_demos // for demonstrations
-
Qt 5.9 used on Windows binaries
-
add CONTRIBUTING.md , CODE_OF_CONDUCT.md and ROADMAP.md files
-
add 'heldlg', 'msgbox', 'errordlg', 'warndlg' functions based on QML.
-
add 'questdlg' function (Creates a question dialog box) based on QML.
-
add '==', '~=', 'isequal', 'isequaln' overload for handle and QObject.
-
add ishandle, isprop, ismethod, method, properties builtin.
-
add set, get, invoke, isvalid builtin used by handle objects.
-
extends clear function to call delete if 'TYPEHANDLE_clear' function is defined.
-
add handle object type.
-
gui module also loaded in advanced cli mode.
-
add 'sind', 'cosd', 'tand' functions.
-
add more output information in the result file of test_run function.
Bug Fixes:
#47: add isfinite builtin.
#43: rename getContentsAsWideString to getContentAsWideString.
#27: >= operator was not implemented.
0.1.5 alpha (2017-04-17)
Features:
- Optimize constructors allocation for eye, ones, Inf, NaN, rand, randn functions.
- Add 'issymmetric' function.
- Add 'svd' function.
- Add 'rcond' function.
Bug Fixes:
#41: test_makeref starts a new clear session to create a ref file.
#39: inv([0 0;i() 3]) did not return [Inf, Inf; Inf, Inf] on ARM platform.
#25: ndims added.
#24: isnan & isinf added.
0.1.4 alpha (2017-03-19)
Compilation:
LAPACKE used for linear algebra functions. On Windows, OpenBLAS used. On others platforms reference library LAPACKE used.
Features:
- Add 'isnan' function.
- Rework double and single matrix 2D display.
- Add 'trace' function.
- Add 'schur' function.
- Add 'inv' function.
- Add 'expm' function (single, double, sparse double managed).
- Rename 'string' type to 'char' type, 'string' type will be added later.
- Add 'dlmwrite' function.
- Add 'mat2str' function (sparse managed).
- Add 'str2double' function.
- Add 'cosm' function.
- which(function_name, '-all') without output argument display paths.
Bug Fixes:
#34: Windows installer did not copy module_skeleton help files at the good place.
#31: doc function was too slow (indexing at each restart).
#30: Search path order for functions was wrong.
0.1.3 alpha (2017-02-11)
Features:
- datevec updated to manage vectors, matrix.
- Add 'nnz', 'nzmax', 'numel' functions.
- Rework 'searchenv' to return a cell of strings.
- Update persistent variables behavior (clear functions).
- Add 'ceil', 'floor', 'round', 'fix' functions.
- Add sparse support for acos, asin, atan, cos, cosh, sin, sinh, tan, tanh.
- Add website and bug reports link in help menu.
Bug Fixes:
#28: Each public builtin have a help file.
#26: 7.853981633974482790D-01 was not correctly parsed.
#22: last output argument of 'IJV' did not return nzmax.
#19: rand & randn did not use Column-major order.
#17: 'locales' directory renamed as 'locale' (more standard).
#13: mldivide and mrdivide builtin were missing.
0.1.2 alpha (2017-01-01)
Bug Fixes:
#11: colon constructor failed with some special values.
#10: module_skeleton did not build/load.
#8: test_nargin & test_nargout failed in Windows binary version.
0.1.1 alpha (2016-12-30)
Bug Fixes:
#5: help browser did not work on some Windows
0.1.0 alpha (2016-12-28)
- Initial import in github of Nelson
Nelson license:
Nelson is free software: you can redistribute it and/or modify it under the terms of the GNU Lesser General Public License, version 3.0
It is still also available under the terms of the GNU General Public License (GPL) v3.0.
Nelson is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License for more details.
You should have received a copy of the GNU Lesser General Public License along with Nelson. If not, see http://www.gnu.org/licenses/.
Nelson license:
Nelson is free software: you can redistribute it and/or modify it under the terms of the GNU Lesser General Public License, version 3.0
It is still also available under the terms of the GNU General Public License (GPL) v3.0.
Nelson is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License for more details.
You should have received a copy of the GNU Lesser General Public License along with Nelson. If not, see http://www.gnu.org/licenses/.
GNU LESSER GENERAL PUBLIC LICENSE
Version 3, 29 June 2007
Copyright (C) 2007 Free Software Foundation, Inc. https://fsf.org/
Everyone is permitted to copy and distribute verbatim copies of this license document, but changing it is not allowed.
This version of the GNU Lesser General Public License incorporates the terms and conditions of version 3 of the GNU General Public License, supplemented by the additional permissions listed below.
0. Additional Definitions.
As used herein, "this License" refers to version 3 of the GNU Lesser General Public License, and the "GNU GPL" refers to version 3 of the GNU General Public License.
"The Library" refers to a covered work governed by this License, other than an Application or a Combined Work as defined below.
An "Application" is any work that makes use of an interface provided by the Library, but which is not otherwise based on the Library. Defining a subclass of a class defined by the Library is deemed a mode of using an interface provided by the Library.
A "Combined Work" is a work produced by combining or linking an Application with the Library. The particular version of the Library with which the Combined Work was made is also called the "Linked Version".
The "Minimal Corresponding Source" for a Combined Work means the Corresponding Source for the Combined Work, excluding any source code for portions of the Combined Work that, considered in isolation, are based on the Application, and not on the Linked Version.
The "Corresponding Application Code" for a Combined Work means the object code and/or source code for the Application, including any data and utility programs needed for reproducing the Combined Work from the Application, but excluding the System Libraries of the Combined Work.
1. Exception to Section 3 of the GNU GPL.
You may convey a covered work under sections 3 and 4 of this License without being bound by section 3 of the GNU GPL.
2. Conveying Modified Versions.
If you modify a copy of the Library, and, in your modifications, a facility refers to a function or data to be supplied by an Application that uses the facility (other than as an argument passed when the facility is invoked), then you may convey a copy of the modified version:
- a) under this License, provided that you make a good faith effort to ensure that, in the event an Application does not supply the function or data, the facility still operates, and performs whatever part of its purpose remains meaningful, or
- b) under the GNU GPL, with none of the additional permissions of this License applicable to that copy.
3. Object Code Incorporating Material from Library Header Files.
The object code form of an Application may incorporate material from a header file that is part of the Library. You may convey such object code under terms of your choice, provided that, if the incorporated material is not limited to numerical parameters, data structure layouts and accessors, or small macros, inline functions and templates (ten or fewer lines in length), you do both of the following:
- a) Give prominent notice with each copy of the object code that the Library is used in it and that the Library and its use are covered by this License.
- b) Accompany the object code with a copy of the GNU GPL and this license document.
4. Combined Works.
You may convey a Combined Work under terms of your choice that, taken together, effectively do not restrict modification of the portions of the Library contained in the Combined Work and reverse engineering for debugging such modifications, if you also do each of the following:
- a) Give prominent notice with each copy of the Combined Work that the Library is used in it and that the Library and its use are covered by this License.
- b) Accompany the Combined Work with a copy of the GNU GPL and this license document.
- c) For a Combined Work that displays copyright notices during execution, include the copyright notice for the Library among these notices, as well as a reference directing the user to the copies of the GNU GPL and this license document.
- d) Do one of the following:
-
- Convey the Minimal Corresponding Source under the terms of this License, and the Corresponding Application Code in a form suitable for, and under terms that permit, the user to recombine or relink the Application with a modified version of the Linked Version to produce a modified Combined Work, in the manner specified by section 6 of the GNU GPL for conveying Corresponding Source.
-
- Use a suitable shared library mechanism for linking with the Library. A suitable mechanism is one that (a) uses at run time a copy of the Library already present on the user's computer system, and (b) will operate properly with a modified version of the Library that is interface-compatible with the Linked Version.
-
- e) Provide Installation Information, but only if you would otherwise be required to provide such information under section 6 of the GNU GPL, and only to the extent that such information is necessary to install and execute a modified version of the Combined Work produced by recombining or relinking the Application with a modified version of the Linked Version. (If you use option 4d0, the Installation Information must accompany the Minimal Corresponding Source and Corresponding Application Code. If you use option 4d1, you must provide the Installation Information in the manner specified by section 6 of the GNU GPL for conveying Corresponding Source.)
5. Combined Libraries.
You may place library facilities that are a work based on the Library side by side in a single library together with other library facilities that are not Applications and are not covered by this License, and convey such a combined library under terms of your choice, if you do both of the following:
- a) Accompany the combined library with a copy of the same work based on the Library, uncombined with any other library facilities, conveyed under the terms of this License.
- b) Give prominent notice with the combined library that part of it is a work based on the Library, and explaining where to find the accompanying uncombined form of the same work.
6. Revised Versions of the GNU Lesser General Public License.
The Free Software Foundation may publish revised and/or new versions of the GNU Lesser General Public License from time to time. Such new versions will be similar in spirit to the present version, but may differ in detail to address new problems or concerns.
Each version is given a distinguishing version number. If the Library as you received it specifies that a certain numbered version of the GNU Lesser General Public License "or any later version" applies to it, you have the option of following the terms and conditions either of that published version or of any later version published by the Free Software Foundation. If the Library as you received it does not specify a version number of the GNU Lesser General Public License, you may choose any version of the GNU Lesser General Public License ever published by the Free Software Foundation.
If the Library as you received it specifies that a proxy can decide whether future versions of the GNU Lesser General Public License shall apply, that proxy's public statement of acceptance of any version is permanent authorization for you to choose that version for the Library.
GNU GENERAL PUBLIC LICENSE
Version 3, 29 June 2007
Copyright (C) 2007 Free Software Foundation, Inc. https://fsf.org/
Everyone is permitted to copy and distribute verbatim copies of this license document, but changing it is not allowed.
Preamble
The GNU General Public License is a free, copyleft license for software and other kinds of works.
The licenses for most software and other practical works are designed to take away your freedom to share and change the works. By contrast, the GNU General Public License is intended to guarantee your freedom to share and change all versions of a program--to make sure it remains free software for all its users. We, the Free Software Foundation, use the GNU General Public License for most of our software; it applies also to any other work released this way by its authors. You can apply it to your programs, too.
When we speak of free software, we are referring to freedom, not price. Our General Public Licenses are designed to make sure that you have the freedom to distribute copies of free software (and charge for them if you wish), that you receive source code or can get it if you want it, that you can change the software or use pieces of it in new free programs, and that you know you can do these things.
To protect your rights, we need to prevent others from denying you these rights or asking you to surrender the rights. Therefore, you have certain responsibilities if you distribute copies of the software, or if you modify it: responsibilities to respect the freedom of others.
For example, if you distribute copies of such a program, whether gratis or for a fee, you must pass on to the recipients the same freedoms that you received. You must make sure that they, too, receive or can get the source code. And you must show them these terms so they know their rights.
Developers that use the GNU GPL protect your rights with two steps: (1) assert copyright on the software, and (2) offer you this License giving you legal permission to copy, distribute and/or modify it.
For the developers' and authors' protection, the GPL clearly explains that there is no warranty for this free software. For both users' and authors' sake, the GPL requires that modified versions be marked as changed, so that their problems will not be attributed erroneously to authors of previous versions.
Some devices are designed to deny users access to install or run modified versions of the software inside them, although the manufacturer can do so. This is fundamentally incompatible with the aim of protecting users' freedom to change the software. The systematic pattern of such abuse occurs in the area of products for individuals to use, which is precisely where it is most unacceptable. Therefore, we have designed this version of the GPL to prohibit the practice for those products. If such problems arise substantially in other domains, we stand ready to extend this provision to those domains in future versions of the GPL, as needed to protect the freedom of users.
Finally, every program is threatened constantly by software patents. States should not allow patents to restrict development and use of software on general-purpose computers, but in those that do, we wish to avoid the special danger that patents applied to a free program could make it effectively proprietary. To prevent this, the GPL assures that patents cannot be used to render the program non-free.
The precise terms and conditions for copying, distribution and modification follow.
TERMS AND CONDITIONS
0. Definitions.
"This License" refers to version 3 of the GNU General Public License.
"Copyright" also means copyright-like laws that apply to other kinds of works, such as semiconductor masks.
"The Program" refers to any copyrightable work licensed under this License. Each licensee is addressed as "you". "Licensees" and "recipients" may be individuals or organizations.
To "modify" a work means to copy from or adapt all or part of the work in a fashion requiring copyright permission, other than the making of an exact copy. The resulting work is called a "modified version" of the earlier work or a work "based on" the earlier work.
A "covered work" means either the unmodified Program or a work based on the Program.
To "propagate" a work means to do anything with it that, without permission, would make you directly or secondarily liable for infringement under applicable copyright law, except executing it on a computer or modifying a private copy. Propagation includes copying, distribution (with or without modification), making available to the public, and in some countries other activities as well.
To "convey" a work means any kind of propagation that enables other parties to make or receive copies. Mere interaction with a user through a computer network, with no transfer of a copy, is not conveying.
An interactive user interface displays "Appropriate Legal Notices" to the extent that it includes a convenient and prominently visible feature that (1) displays an appropriate copyright notice, and (2) tells the user that there is no warranty for the work (except to the extent that warranties are provided), that licensees may convey the work under this License, and how to view a copy of this License. If the interface presents a list of user commands or options, such as a menu, a prominent item in the list meets this criterion.
1. Source Code.
The "source code" for a work means the preferred form of the work for making modifications to it. "Object code" means any non-source form of a work.
A "Standard Interface" means an interface that either is an official standard defined by a recognized standards body, or, in the case of interfaces specified for a particular programming language, one that is widely used among developers working in that language.
The "System Libraries" of an executable work include anything, other than the work as a whole, that (a) is included in the normal form of packaging a Major Component, but which is not part of that Major Component, and (b) serves only to enable use of the work with that Major Component, or to implement a Standard Interface for which an implementation is available to the public in source code form. A "Major Component", in this context, means a major essential component (kernel, window system, and so on) of the specific operating system (if any) on which the executable work runs, or a compiler used to produce the work, or an object code interpreter used to run it.
The "Corresponding Source" for a work in object code form means all the source code needed to generate, install, and (for an executable work) run the object code and to modify the work, including scripts to control those activities. However, it does not include the work's System Libraries, or general-purpose tools or generally available free programs which are used unmodified in performing those activities but which are not part of the work. For example, Corresponding Source includes interface definition files associated with source files for the work, and the source code for shared libraries and dynamically linked subprograms that the work is specifically designed to require, such as by intimate data communication or control flow between those subprograms and other parts of the work.
The Corresponding Source need not include anything that users can regenerate automatically from other parts of the Corresponding Source.
The Corresponding Source for a work in source code form is that same work.
2. Basic Permissions.
All rights granted under this License are granted for the term of copyright on the Program, and are irrevocable provided the stated conditions are met. This License explicitly affirms your unlimited permission to run the unmodified Program. The output from running a covered work is covered by this License only if the output, given its content, constitutes a covered work. This License acknowledges your rights of fair use or other equivalent, as provided by copyright law.
You may make, run and propagate covered works that you do not convey, without conditions so long as your license otherwise remains in force. You may convey covered works to others for the sole purpose of having them make modifications exclusively for you, or provide you with facilities for running those works, provided that you comply with the terms of this License in conveying all material for which you do not control copyright. Those thus making or running the covered works for you must do so exclusively on your behalf, under your direction and control, on terms that prohibit them from making any copies of your copyrighted material outside their relationship with you.
Conveying under any other circumstances is permitted solely under the conditions stated below. Sublicensing is not allowed; section 10 makes it unnecessary.
3. Protecting Users' Legal Rights From Anti-Circumvention Law.
No covered work shall be deemed part of an effective technological measure under any applicable law fulfilling obligations under article 11 of the WIPO copyright treaty adopted on 20 December 1996, or similar laws prohibiting or restricting circumvention of such measures.
When you convey a covered work, you waive any legal power to forbid circumvention of technological measures to the extent such circumvention is effected by exercising rights under this License with respect to the covered work, and you disclaim any intention to limit operation or modification of the work as a means of enforcing, against the work's users, your or third parties' legal rights to forbid circumvention of technological measures.
4. Conveying Verbatim Copies.
You may convey verbatim copies of the Program's source code as you receive it, in any medium, provided that you conspicuously and appropriately publish on each copy an appropriate copyright notice; keep intact all notices stating that this License and any non-permissive terms added in accord with section 7 apply to the code; keep intact all notices of the absence of any warranty; and give all recipients a copy of this License along with the Program.
You may charge any price or no price for each copy that you convey, and you may offer support or warranty protection for a fee.
5. Conveying Modified Source Versions.
You may convey a work based on the Program, or the modifications to produce it from the Program, in the form of source code under the terms of section 4, provided that you also meet all of these conditions:
- a) The work must carry prominent notices stating that you modified it, and giving a relevant date.
- b) The work must carry prominent notices stating that it is released under this License and any conditions added under section 7. This requirement modifies the requirement in section 4 to "keep intact all notices".
- c) You must license the entire work, as a whole, under this License to anyone who comes into possession of a copy. This License will therefore apply, along with any applicable section 7 additional terms, to the whole of the work, and all its parts, regardless of how they are packaged. This License gives no permission to license the work in any other way, but it does not invalidate such permission if you have separately received it.
- d) If the work has interactive user interfaces, each must display Appropriate Legal Notices; however, if the Program has interactive interfaces that do not display Appropriate Legal Notices, your work need not make them do so.
A compilation of a covered work with other separate and independent works, which are not by their nature extensions of the covered work, and which are not combined with it such as to form a larger program, in or on a volume of a storage or distribution medium, is called an "aggregate" if the compilation and its resulting copyright are not used to limit the access or legal rights of the compilation's users beyond what the individual works permit. Inclusion of a covered work in an aggregate does not cause this License to apply to the other parts of the aggregate.
6. Conveying Non-Source Forms.
You may convey a covered work in object code form under the terms of sections 4 and 5, provided that you also convey the machine-readable Corresponding Source under the terms of this License, in one of these ways:
- a) Convey the object code in, or embodied in, a physical product (including a physical distribution medium), accompanied by the Corresponding Source fixed on a durable physical medium customarily used for software interchange.
- b) Convey the object code in, or embodied in, a physical product (including a physical distribution medium), accompanied by a written offer, valid for at least three years and valid for as long as you offer spare parts or customer support for that product model, to give anyone who possesses the object code either (1) a copy of the Corresponding Source for all the software in the product that is covered by this License, on a durable physical medium customarily used for software interchange, for a price no more than your reasonable cost of physically performing this conveying of source, or (2) access to copy the Corresponding Source from a network server at no charge.
- c) Convey individual copies of the object code with a copy of the written offer to provide the Corresponding Source. This alternative is allowed only occasionally and noncommercially, and only if you received the object code with such an offer, in accord with subsection 6b.
- d) Convey the object code by offering access from a designated place (gratis or for a charge), and offer equivalent access to the Corresponding Source in the same way through the same place at no further charge. You need not require recipients to copy the Corresponding Source along with the object code. If the place to copy the object code is a network server, the Corresponding Source may be on a different server (operated by you or a third party) that supports equivalent copying facilities, provided you maintain clear directions next to the object code saying where to find the Corresponding Source. Regardless of what server hosts the Corresponding Source, you remain obligated to ensure that it is available for as long as needed to satisfy these requirements.
- e) Convey the object code using peer-to-peer transmission, provided you inform other peers where the object code and Corresponding Source of the work are being offered to the general public at no charge under subsection 6d.
A separable portion of the object code, whose source code is excluded from the Corresponding Source as a System Library, need not be included in conveying the object code work.
A "User Product" is either (1) a "consumer product", which means any tangible personal property which is normally used for personal, family, or household purposes, or (2) anything designed or sold for incorporation into a dwelling. In determining whether a product is a consumer product, doubtful cases shall be resolved in favor of coverage. For a particular product received by a particular user, "normally used" refers to a typical or common use of that class of product, regardless of the status of the particular user or of the way in which the particular user actually uses, or expects or is expected to use, the product. A product is a consumer product regardless of whether the product has substantial commercial, industrial or non-consumer uses, unless such uses represent the only significant mode of use of the product.
"Installation Information" for a User Product means any methods, procedures, authorization keys, or other information required to install and execute modified versions of a covered work in that User Product from a modified version of its Corresponding Source. The information must suffice to ensure that the continued functioning of the modified object code is in no case prevented or interfered with solely because modification has been made.
If you convey an object code work under this section in, or with, or specifically for use in, a User Product, and the conveying occurs as part of a transaction in which the right of possession and use of the User Product is transferred to the recipient in perpetuity or for a fixed term (regardless of how the transaction is characterized), the Corresponding Source conveyed under this section must be accompanied by the Installation Information. But this requirement does not apply if neither you nor any third party retains the ability to install modified object code on the User Product (for example, the work has been installed in ROM).
The requirement to provide Installation Information does not include a requirement to continue to provide support service, warranty, or updates for a work that has been modified or installed by the recipient, or for the User Product in which it has been modified or installed. Access to a network may be denied when the modification itself materially and adversely affects the operation of the network or violates the rules and protocols for communication across the network.
Corresponding Source conveyed, and Installation Information provided, in accord with this section must be in a format that is publicly documented (and with an implementation available to the public in source code form), and must require no special password or key for unpacking, reading or copying.
7. Additional Terms.
"Additional permissions" are terms that supplement the terms of this License by making exceptions from one or more of its conditions. Additional permissions that are applicable to the entire Program shall be treated as though they were included in this License, to the extent that they are valid under applicable law. If additional permissions apply only to part of the Program, that part may be used separately under those permissions, but the entire Program remains governed by this License without regard to the additional permissions.
When you convey a copy of a covered work, you may at your option remove any additional permissions from that copy, or from any part of it. (Additional permissions may be written to require their own removal in certain cases when you modify the work.) You may place additional permissions on material, added by you to a covered work, for which you have or can give appropriate copyright permission.
Notwithstanding any other provision of this License, for material you add to a covered work, you may (if authorized by the copyright holders of that material) supplement the terms of this License with terms:
- a) Disclaiming warranty or limiting liability differently from the terms of sections 15 and 16 of this License; or
- b) Requiring preservation of specified reasonable legal notices or author attributions in that material or in the Appropriate Legal Notices displayed by works containing it; or
- c) Prohibiting misrepresentation of the origin of that material, or requiring that modified versions of such material be marked in reasonable ways as different from the original version; or
- d) Limiting the use for publicity purposes of names of licensors or authors of the material; or
- e) Declining to grant rights under trademark law for use of some trade names, trademarks, or service marks; or
- f) Requiring indemnification of licensors and authors of that material by anyone who conveys the material (or modified versions of it) with contractual assumptions of liability to the recipient, for any liability that these contractual assumptions directly impose on those licensors and authors.
All other non-permissive additional terms are considered "further restrictions" within the meaning of section 10. If the Program as you received it, or any part of it, contains a notice stating that it is governed by this License along with a term that is a further restriction, you may remove that term. If a license document contains a further restriction but permits relicensing or conveying under this License, you may add to a covered work material governed by the terms of that license document, provided that the further restriction does not survive such relicensing or conveying.
If you add terms to a covered work in accord with this section, you must place, in the relevant source files, a statement of the additional terms that apply to those files, or a notice indicating where to find the applicable terms.
Additional terms, permissive or non-permissive, may be stated in the form of a separately written license, or stated as exceptions; the above requirements apply either way.
8. Termination.
You may not propagate or modify a covered work except as expressly provided under this License. Any attempt otherwise to propagate or modify it is void, and will automatically terminate your rights under this License (including any patent licenses granted under the third paragraph of section 11).
However, if you cease all violation of this License, then your license from a particular copyright holder is reinstated (a) provisionally, unless and until the copyright holder explicitly and finally terminates your license, and (b) permanently, if the copyright holder fails to notify you of the violation by some reasonable means prior to 60 days after the cessation.
Moreover, your license from a particular copyright holder is reinstated permanently if the copyright holder notifies you of the violation by some reasonable means, this is the first time you have received notice of violation of this License (for any work) from that copyright holder, and you cure the violation prior to 30 days after your receipt of the notice.
Termination of your rights under this section does not terminate the licenses of parties who have received copies or rights from you under this License. If your rights have been terminated and not permanently reinstated, you do not qualify to receive new licenses for the same material under section 10.
9. Acceptance Not Required for Having Copies.
You are not required to accept this License in order to receive or run a copy of the Program. Ancillary propagation of a covered work occurring solely as a consequence of using peer-to-peer transmission to receive a copy likewise does not require acceptance. However, nothing other than this License grants you permission to propagate or modify any covered work. These actions infringe copyright if you do not accept this License. Therefore, by modifying or propagating a covered work, you indicate your acceptance of this License to do so.
10. Automatic Licensing of Downstream Recipients.
Each time you convey a covered work, the recipient automatically receives a license from the original licensors, to run, modify and propagate that work, subject to this License. You are not responsible for enforcing compliance by third parties with this License.
An "entity transaction" is a transaction transferring control of an organization, or substantially all assets of one, or subdividing an organization, or merging organizations. If propagation of a covered work results from an entity transaction, each party to that transaction who receives a copy of the work also receives whatever licenses to the work the party's predecessor in interest had or could give under the previous paragraph, plus a right to possession of the Corresponding Source of the work from the predecessor in interest, if the predecessor has it or can get it with reasonable efforts.
You may not impose any further restrictions on the exercise of the rights granted or affirmed under this License. For example, you may not impose a license fee, royalty, or other charge for exercise of rights granted under this License, and you may not initiate litigation (including a cross-claim or counterclaim in a lawsuit) alleging that any patent claim is infringed by making, using, selling, offering for sale, or importing the Program or any portion of it.
11. Patents.
A "contributor" is a copyright holder who authorizes use under this License of the Program or a work on which the Program is based. The work thus licensed is called the contributor's "contributor version".
A contributor's "essential patent claims" are all patent claims owned or controlled by the contributor, whether already acquired or hereafter acquired, that would be infringed by some manner, permitted by this License, of making, using, or selling its contributor version, but do not include claims that would be infringed only as a consequence of further modification of the contributor version. For purposes of this definition, "control" includes the right to grant patent sublicenses in a manner consistent with the requirements of this License.
Each contributor grants you a non-exclusive, worldwide, royalty-free patent license under the contributor's essential patent claims, to make, use, sell, offer for sale, import and otherwise run, modify and propagate the contents of its contributor version.
In the following three paragraphs, a "patent license" is any express agreement or commitment, however denominated, not to enforce a patent (such as an express permission to practice a patent or covenant not to sue for patent infringement). To "grant" such a patent license to a party means to make such an agreement or commitment not to enforce a patent against the party.
If you convey a covered work, knowingly relying on a patent license, and the Corresponding Source of the work is not available for anyone to copy, free of charge and under the terms of this License, through a publicly available network server or other readily accessible means, then you must either (1) cause the Corresponding Source to be so available, or (2) arrange to deprive yourself of the benefit of the patent license for this particular work, or (3) arrange, in a manner consistent with the requirements of this License, to extend the patent license to downstream recipients. "Knowingly relying" means you have actual knowledge that, but for the patent license, your conveying the covered work in a country, or your recipient's use of the covered work in a country, would infringe one or more identifiable patents in that country that you have reason to believe are valid.
If, pursuant to or in connection with a single transaction or arrangement, you convey, or propagate by procuring conveyance of, a covered work, and grant a patent license to some of the parties receiving the covered work authorizing them to use, propagate, modify or convey a specific copy of the covered work, then the patent license you grant is automatically extended to all recipients of the covered work and works based on it.
A patent license is "discriminatory" if it does not include within the scope of its coverage, prohibits the exercise of, or is conditioned on the non-exercise of one or more of the rights that are specifically granted under this License. You may not convey a covered work if you are a party to an arrangement with a third party that is in the business of distributing software, under which you make payment to the third party based on the extent of your activity of conveying the work, and under which the third party grants, to any of the parties who would receive the covered work from you, a discriminatory patent license (a) in connection with copies of the covered work conveyed by you (or copies made from those copies), or (b) primarily for and in connection with specific products or compilations that contain the covered work, unless you entered into that arrangement, or that patent license was granted, prior to 28 March 2007.
Nothing in this License shall be construed as excluding or limiting any implied license or other defenses to infringement that may otherwise be available to you under applicable patent law.
12. No Surrender of Others' Freedom.
If conditions are imposed on you (whether by court order, agreement or otherwise) that contradict the conditions of this License, they do not excuse you from the conditions of this License. If you cannot convey a covered work so as to satisfy simultaneously your obligations under this License and any other pertinent obligations, then as a consequence you may not convey it at all. For example, if you agree to terms that obligate you to collect a royalty for further conveying from those to whom you convey the Program, the only way you could satisfy both those terms and this License would be to refrain entirely from conveying the Program.
13. Use with the GNU Affero General Public License.
Notwithstanding any other provision of this License, you have permission to link or combine any covered work with a work licensed under version 3 of the GNU Affero General Public License into a single combined work, and to convey the resulting work. The terms of this License will continue to apply to the part which is the covered work, but the special requirements of the GNU Affero General Public License, section 13, concerning interaction through a network will apply to the combination as such.
14. Revised Versions of this License.
The Free Software Foundation may publish revised and/or new versions of the GNU General Public License from time to time. Such new versions will be similar in spirit to the present version, but may differ in detail to address new problems or concerns.
Each version is given a distinguishing version number. If the Program specifies that a certain numbered version of the GNU General Public License "or any later version" applies to it, you have the option of following the terms and conditions either of that numbered version or of any later version published by the Free Software Foundation. If the Program does not specify a version number of the GNU General Public License, you may choose any version ever published by the Free Software Foundation.
If the Program specifies that a proxy can decide which future versions of the GNU General Public License can be used, that proxy's public statement of acceptance of a version permanently authorizes you to choose that version for the Program.
Later license versions may give you additional or different permissions. However, no additional obligations are imposed on any author or copyright holder as a result of your choosing to follow a later version.
15. Disclaimer of Warranty.
THERE IS NO WARRANTY FOR THE PROGRAM, TO THE EXTENT PERMITTED BY APPLICABLE LAW. EXCEPT WHEN OTHERWISE STATED IN WRITING THE COPYRIGHT HOLDERS AND/OR OTHER PARTIES PROVIDE THE PROGRAM "AS IS" WITHOUT WARRANTY OF ANY KIND, EITHER EXPRESSED OR IMPLIED, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE. THE ENTIRE RISK AS TO THE QUALITY AND PERFORMANCE OF THE PROGRAM IS WITH YOU. SHOULD THE PROGRAM PROVE DEFECTIVE, YOU ASSUME THE COST OF ALL NECESSARY SERVICING, REPAIR OR CORRECTION.
16. Limitation of Liability.
IN NO EVENT UNLESS REQUIRED BY APPLICABLE LAW OR AGREED TO IN WRITING WILL ANY COPYRIGHT HOLDER, OR ANY OTHER PARTY WHO MODIFIES AND/OR CONVEYS THE PROGRAM AS PERMITTED ABOVE, BE LIABLE TO YOU FOR DAMAGES, INCLUDING ANY GENERAL, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES ARISING OUT OF THE USE OR INABILITY TO USE THE PROGRAM (INCLUDING BUT NOT LIMITED TO LOSS OF DATA OR DATA BEING RENDERED INACCURATE OR LOSSES SUSTAINED BY YOU OR THIRD PARTIES OR A FAILURE OF THE PROGRAM TO OPERATE WITH ANY OTHER PROGRAMS), EVEN IF SUCH HOLDER OR OTHER PARTY HAS BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES.
17. Interpretation of Sections 15 and 16.
If the disclaimer of warranty and limitation of liability provided above cannot be given local legal effect according to their terms, reviewing courts shall apply local law that most closely approximates an absolute waiver of all civil liability in connection with the Program, unless a warranty or assumption of liability accompanies a copy of the Program in return for a fee.
END OF TERMS AND CONDITIONS
How to Apply These Terms to Your New Programs
If you develop a new program, and you want it to be of the greatest possible use to the public, the best way to achieve this is to make it free software which everyone can redistribute and change under these terms.
To do so, attach the following notices to the program. It is safest to attach them to the start of each source file to most effectively state the exclusion of warranty; and each file should have at least the "copyright" line and a pointer to where the full notice is found.
<one line to give the program's name and a brief idea of what it does.>
Copyright (C) <year> <name of author>
This program is free software: you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation, either version 3 of the License, or
(at your option) any later version.
This program is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU General Public License for more details.
You should have received a copy of the GNU General Public License
along with this program. If not, see <https://www.gnu.org/licenses/>.
Also add information on how to contact you by electronic and paper mail.
If the program does terminal interaction, make it output a short notice like this when it starts in an interactive mode:
<program> Copyright (C) <year> <name of author>
This program comes with ABSOLUTELY NO WARRANTY; for details type `show w'.
This is free software, and you are welcome to redistribute it
under certain conditions; type `show c' for details.
The hypothetical commands `show w' and `show c' should show the appropriate parts of the General Public License. Of course, your program's commands might be different; for a GUI interface, you would use an "about box".
You should also get your employer (if you work as a programmer) or school, if any, to sign a "copyright disclaimer" for the program, if necessary. For more information on this, and how to apply and follow the GNU GPL, see https://www.gnu.org/licenses/.
The GNU General Public License does not permit incorporating your program into proprietary programs. If your program is a subroutine library, you may consider it more useful to permit linking proprietary applications with the library. If this is what you want to do, use the GNU Lesser General Public License instead of this License. But first, please read https://www.gnu.org/licenses/why-not-lgpl.html.